googleapis.drive
Modules
googleapis.drive
googleapis.drive.listenerModule googleapis.drive
Definitions
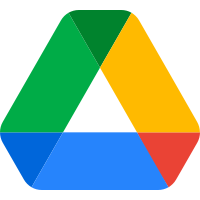
ballerinax/googleapis.drive Ballerina library
Overview
The connector provides the capability to programmatically manage files and folders in the Google Drive.
This module supports Google Drive API v3.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google Drive connector in your Ballerina application, update the .bal file as follows:
Step 1: Import the connector
Import the ballerinax/googleapis.drive module into the Ballerina projects shown below.
import ballerinax/googleapis.drive;
All the actions return a valid response or error. If the action is a success, then the requested resource is returned. If not, an error is returned.
Step 2: Create a new connector instance
Create a drive:ConnectionConfig with the OAuth2 tokens obtained, and initialize the connector with it.
configurable string refreshToken = ?; configurable string clientId = ?; configurable string clientSecret = ?; configurable string refreshUrl = drive:REFRESH_URL; drive:ConnectionConfig config = { auth: { clientId: clientId, clientSecret: clientSecret, refreshUrl: refreshUrl, refreshToken: refreshToken } }; drive:Client driveClient = new (config);
Step 3: Invoke the connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve a file using the connector.
Get file with given file ID
public function main() returns error? { drive:File response = check driveClient->getFile(fileId); log:printInfo("Successfully retreived the file."); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.drive: Client
Ballerina Google Drive connector provides capability to programmatically manage files and folders. Google Drive API provides operations related to files, channels and changes in Google Drive.
Constructor
Initialize the connector.
init (ConnectionConfig driveConfig)
- driveConfig ConnectionConfig - Configurations required to initialize the
Client
getFile
Retrieves file using the file ID.
Parameters
- fileId string - ID of the file to retrieve
- fields string? (default ()) - The paths of the fields you want included in the response
getFileContent
function getFileContent(string fileId) returns FileContent|error
Retrieves file content using the fileId.
Parameters
- fileId string - Id of the file to retrieve file content
Return Type
- FileContent|error - If successful,
FileContent
. Else anerror
downloadFile
Downloads file using the fileId.
Parameters
- fileId string - ID of the file to retrieve
getAllFiles
Retrieves all the files in the drive.
Parameters
- filterString string? (default ()) - Query used to find what you need. Read documentation for query string patterns. https://github.com/ballerina-platform/module-ballerinax-googleapis.drive/blob/main/drive/README.md#filter-files
- orderBy string? (default ()) - A comma-separated list of sort keys. Valid keys are 'createdTime', 'folder', 'modifiedByMeTime', 'modifiedTime', 'name', 'name_natural', 'quotaBytesUsed', 'recency', 'sharedWithMeTime', 'starred', and 'viewedByMeTime'
getFilesByName
Retrieves files by name.
Parameters
- fileName string - Name of the file to search (Partial search)
- orderBy string? (default ()) - A comma-separated list of sort keys. Valid keys are 'createdTime', 'folder', 'modifiedByMeTime', 'modifiedTime', 'name', 'name_natural', 'quotaBytesUsed', 'recency', 'sharedWithMeTime', 'starred', and 'viewedByMeTime'
getAllSpreadsheets
Retrieves all Google spreadsheets.
getSpreadsheetsByName
Retrieves Google spreadsheets by name
Parameters
- fileName string - Name of the file to search (Partial search)
- orderBy string? (default ()) - A comma-separated list of sort keys. Valid keys are 'createdTime', 'folder', 'modifiedByMeTime', 'modifiedTime', 'name', 'name_natural', 'quotaBytesUsed', 'recency', 'sharedWithMeTime', 'starred', and 'viewedByMeTime'
getDocumentsByName
Retrieves Google documents by name
Parameters
- fileName string - Name of the file to be searched (Partial search)
- orderBy string? (default ()) - A comma-separated list of sort keys. Valid keys are 'createdTime', 'folder', 'modifiedByMeTime', 'modifiedTime', 'name', 'name_natural', 'quotaBytesUsed', 'recency', 'sharedWithMeTime', 'starred', and 'viewedByMeTime'
getFormsByName
Retrieves Google forms by name.
Parameters
- fileName string - Name of the file to be searched (Partial search)
- orderBy string? (default ()) - A comma-separated list of sort keys. Valid keys are 'createdTime', 'folder', 'modifiedByMeTime', 'modifiedTime', 'name', 'name_natural', 'quotaBytesUsed', 'recency', 'sharedWithMeTime', 'starred', and 'viewedByMeTime'
getSlidesByName
Retrieves Google slides by name.
Parameters
- fileName string - Name of the file to be searched (Partial search)
- orderBy string? (default ()) - A comma-separated list of sort keys. Valid keys are 'createdTime', 'folder', 'modifiedByMeTime', 'modifiedTime', 'name', 'name_natural', 'quotaBytesUsed', 'recency', 'sharedWithMeTime', 'starred', and 'viewedByMeTime'
getFoldersByName
Retrieves folders by name.
Parameters
- folderName string - Name of the folder to be searched (Partial search)
- orderBy string? (default ()) - A comma-separated list of sort keys. Valid keys are 'createdTime', 'folder', 'modifiedByMeTime', 'modifiedTime', 'name', 'name_natural', 'quotaBytesUsed', 'recency', 'sharedWithMeTime', 'starred', and 'viewedByMeTime'.
deleteFile
Deletes file using the file ID.
Parameters
- fileId string - ID of the file to delete
copyFile
function copyFile(string fileId, string? destinationFolderId, string? newFileName) returns File|error
Copies file using the file ID.
Parameters
- fileId string - ID of the file to be copied
- destinationFolderId string? (default ()) - Folder ID of the destination
- newFileName string? (default ()) - Name of the New file
moveFile
Moves file using the file ID.
Parameters
- fileId string - ID of the file to be moved
- destinationFolderId string - Folder ID of the destination
renameFile
Renames a file.
Parameters
- fileId string - File ID that need to be renamed
- newFileName string - New file name that should be renamed to
updateFileMetadataById
function updateFileMetadataById(string fileId, FileMetadata? fileMetadata, record { addParents string, includePermissionsForView string, keepRevisionForever boolean, ocrLanguage string, removeParents string, supportsAllDrives boolean, useContentAsIndexableText boolean, anydata... }? optional) returns File|error
Updates file metadata using the file ID.
Parameters
- fileId string - ID of the file to be updated
- fileMetadata FileMetadata? (default ()) - 'FileMetadata' can added as a payload to change metadata
- optional record { addParents string, includePermissionsForView string, keepRevisionForever boolean, ocrLanguage string, removeParents string, supportsAllDrives boolean, useContentAsIndexableText boolean, anydata... }? (default ()) - 'UpdateFileMetadataOptional' used to add query parameters to the request
createFile
Creates new file.
Parameters
- fileName string - Name of the new file to be created
- mime MimeTypes? (default ()) - Type of file that is going to create. refer https://developers.google.com/drive/api/v3/mime-types You need to only specify the last word in the MIME type. For an example, If you want to create a Google document.. The value for this parameter should be "document" .. Google sheets -> "spreadsheet" etc
- folderId string? (default ()) - ID of the parent folder that the new file wants to get created
createFolder
Creates new folder.
Parameters
- folderName string - Name of the new folder to be created
- parentFolderId string? (default ()) - ID of the parent folder
uploadFile
Uploads new file.
Parameters
- localPath string - Path to the file object to be uploaded
- fileName string? (default ()) - File name for the uploading file (optional). It will take the base name, if not provided
- parentFolderId string? (default ()) - Parent folder ID (optional). It will be uploaded to the root, if not provided
uploadFileUsingByteArray
function uploadFileUsingByteArray(byte[] byteArray, string fileName, string? parentFolderId) returns File|error
Uploads new file using a Byte array.
Constants
googleapis.drive: REFRESH_URL
Enums
googleapis.drive: MimeTypes
Mime types for file operations.
Members
Records
googleapis.drive: About
Represents drive information.
Fields
- kind string? - Identifies what kind of resource this is. Value: the fixed string "drive#about"
- user User? - The authenticated user
- storageQuota StorageQuota? - The user's storage quota limits and usage. All fields are measured in bytes
- importFormats StringArrayValuePairs? - A map of source MIME type to possible targets for all supported imports
- exportFormats StringArrayValuePairs? - A map of source MIME type to possible targets for all supported exports
- maxImportSizes StringKeyValuePairs? - A map of maximum import sizes by MIME type, in bytes
- maxUploadSize float? - The maximum upload size in bytes
- appInstalled boolean? - Whether the user has installed the requesting app
- folderColorPalette string[]? - The currently supported folder colors as RGB hex strings
- driveThemes StringKeyValuePairs? - A list of themes that are supported for shared drives
- canCreateDrives boolean? - Indicates whether the user can create shared drives
googleapis.drive: Capabilities
Capabilities the current user has on this file. Each capability corresponds to a fine-grained action that a user may take.
Fields
- canAddChildren boolean - Whether the current user can add children to this folder. This is always false when the item is not a folder
- canAddFolderFromAnotherDrive boolean - Whether the current user can add a folder from another drive (different shared drive or My Drive) to this folder. This is false when the item is not a folder. Only populated for items in shared drives
- canAddMyDriveParent boolean - Whether the current user can add a parent for the item without removing an existing parent in the same request. Not populated for shared drive files
- canChangeCopyRequiresWriterPermission boolean - Whether the current user can change the copyRequiresWriterPermission restriction of this file
- canComment boolean - Whether the current user can comment on this file
- canCopy boolean - Whether the current user can copy this file. For an item in a shared drive, whether the current user can copy non-folder descendants of this item, or this item itself if it is not a folder
- canDelete boolean - Whether the current user can delete this file
- canDeleteChildren boolean - Whether the current user can delete children of this folder. This is false when the item is not a folder. Only populated for items in shared drives
- canDownload boolean - Whether the current user can download this file
- canEdit boolean - Whether the current user can edit this file. Other factors may limit the type of changes a user can make to a file. For example, see canChangeCopyRequiresWriterPermission or canModifyContent
- canListChildren boolean - Whether the current user can list the children of this folder. This is always false when the item is not a folder
- canModifyContent boolean - Whether the current user can modify the content of this file
- canModifyContentRestriction boolean - Whether the current user can modify restrictions on content of this file
- canMoveChildrenOutOfDrive boolean - Whether the current user can move children of this folder outside of the shared drive. This is false when the item is not a folder.Only populated for items in shared drives.
- canMoveChildrenWithinDrive boolean - Whether the current user can move children of this folder within this drive. This is false when the item is not a folder. Note that a request to move the child may still fail depending on the current user's access to the child and to the destination folder
- canMoveItemOutOfDrive boolean - Whether the current user can move this item outside of this drive by changing its parent. Note that a request to change the parent of the item may still fail depending on the new parent that is being added
- canMoveItemWithinDrive boolean - Whether the current user can move this item within this drive. Note that a request to change the parent of the item may still fail depending on the new parent that is being added and the parent that is being removed
- canReadRevisions boolean - Whether the current user can read the revisions resource of this file. For a shared drive item, whether revisions of non-folder descendants of this item, or this item itself if it is not a folder, can be read
- canReadDrive boolean - Whether the current user can read the shared drive to which this file belongs Only populated for items in shared drives
- canRemoveChildren boolean - Whether the current user can remove children from this folder. This is always false when the item is not a folder. For a folder in a shared drive, use canDeleteChildren or canTrashChildren instead
- canRemoveMyDriveParent boolean - Whether the current user can remove a parent from the item without adding another parent in the same request. Not populated for shared drive files
- canRename boolean - Whether the current user can rename this file
- canShare boolean - Whether the current user can modify the sharing settings for this file
- canTrash boolean - Whether the current user can move this file to trash
- canTrashChildren boolean - Whether the current user can trash children of this folder. This is false when the item is not a folder. Only populated for items in shared drives
- canUntrash boolean - Whether the current user can restore this file from trash
googleapis.drive: ConnectionConfig
Represents configuration parameters to create Google Drive client.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion string(default "1.1") - The HTTP version understood by the client
- http1Settings ClientHttp1Settings(default {}) - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings(default {}) - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- followRedirects FollowRedirects?(default ()) - Configurations associated with Redirection
- poolConfig PoolConfiguration?(default ()) - Configurations associated with request pooling
- cache CacheConfig(default {}) - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig?(default ()) - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig?(default ()) - Configurations associated with retrying
- cookieConfig CookieConfig?(default ()) - Configurations associated with cookies
- responseLimits ResponseLimitConfigs(default {}) - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket?(default ()) - SSL/TLS-related options
googleapis.drive: ContentHints
Additional information about the content of the file. These fields are never populated in responses.
Fields
- thumbnail Thumbnail - A thumbnail for the file. This will only be used if Google Drive cannot generate a standard thumbnail
- indexableText string - Text to be indexed for the file to improve fullText queries. This is limited to 128KB in length and may contain HTML elements
googleapis.drive: ContentRestrictions
Restrictions for accessing the content of the file. Only populated if such a restriction exists.
Fields
- readOnly boolean - Whether the content of the file is read-only. If a file is read-only, a new revision of the file may not be added,comments may not be added or modified, and the title of the file may not be modified
- reason string - Reason for why the content of the file is restricted. This is only mutable on requests that also set readOnly=true
- restrictingUser User - The user who set the content restriction. Only populated if readOnly is true
- restrictionTime string - The time at which the content restriction was set (formatted RFC 3339 timestamp) Only populated if readOnly is true
- 'type string -
googleapis.drive: CookieConfig
Client configuration for cookies.
Fields
- enabled boolean(default false) - User agents provide users with a mechanism for disabling or enabling cookies
- maxCookiesPerDomain int(default 50) - Maximum number of cookies per domain, which is 50
- maxTotalCookieCount int(default 3000) - Maximum number of total cookies allowed to be stored in cookie store, which is 3000
- blockThirdPartyCookies boolean(default true) - User can block cookies from third party responses and refuse to send cookies for third party requests, if needed
googleapis.drive: File
Represents File.
Fields
- Fields Included from *FileMetadata
- name string
- mimeType string
- description string
- starred boolean
- trashed boolean
- folderColorRgb string
- appProperties StringKeyValuePairs
- contentHints ContentHints
- copyRequiresWriterPermission boolean
- modifiedTime string
- originalFilename string
- properties StringKeyValuePairs
- viewedByMeTime string
- writersCanShare boolean
- anydata...
- Fields Included from *GeneratedFileMetadata
- id string
- kind string
- explicitlyTrashed boolean
- trashingUser User
- trashedTime string
- parents string[]
- spaces string[]
- version int
- webContentLink string
- webViewLink string
- iconLink string
- hasThumbnail boolean
- thumbnailLink string
- thumbnailVersion int
- viewedByMe boolean
- modifiedByMe boolean
- createdTime string
- modifiedByMeTime string
- sharedWithMeTime string
- sharingUser User
- owners User[]
- driveId string
- lastModifyingUser User
- shared boolean
- ownedByMe boolean
- capabilities Capabilities
- permissions Permissions[]
- permissionIds string[]
- hasAugmentedPermissions boolean
- fullFileExtension string
- fileExtension string
- md5Checksum string
- size int
- quotaBytesUsed int
- headRevisionId string
- imageMediaMetadata ImageMediaMetadata
- videoMediaMetadata VideoMediaMetadata
- isAppAuthorized boolean
- exportLinks StringKeyValuePairs
- shortcutDetails ShortcutDetails
- contentRestrictions ContentRestrictions
- anydata...
googleapis.drive: FileContent
Represents file content.
Fields
- content byte[] - A
byte[]
which represents the content of a file
- mimeType string - The MIME type for the file
googleapis.drive: FileMetadata
Represents file metadata.
Fields
- name string? - The name of the file. This is not necessarily unique within a folder.
Note that the name is constant for immutable items such as the top level folders of shared drives,
My Drive
root folder, and theApplication Data
folder
- mimeType string? - The MIME (Multipurpose Internet Mail Extensions) type of file. If no value is provided,
Google Drive attempts to automatically detect an appropriate value from uploaded content.
The value cannot be changed unless a new revision is uploaded.
If a file is created with a Google Doc MIME type, the uploaded content will be imported if possible.
The supported import formats are published in the
About
resource
- description string? - A short description of the file
- starred boolean? - Whether the user has starred the file
- trashed boolean? - Whether the file has been trashed, either explicitly or from a trashed parent folder. Only the owner may trash a file. The trashed item is excluded from all files. list responses returned for any user who does not own the file. However, all users with access to the file can see the trashed item metadata in an API response. All users with access can copy, download, export, and share the file
- folderColorRgb string? - The color for a folder as an RGB hex string
- appProperties StringKeyValuePairs? - A collection of arbitrary key-value pairs which are private to the requesting app
- contentHints ContentHints? - Additional information about the content of the file. These fields are never populated in responses.
- copyRequiresWriterPermission boolean? - Whether the options to copy, print, or download this file, should be disabled for readers and commenters
- modifiedTime string? - The last time the file was modified by anyone (RFC 3339 date-time)
- originalFilename string? - The original filename of the uploaded content if available, or else the original value of the name field. This is only available for files with binary content in Google Drive
- properties StringKeyValuePairs? - A collection of arbitrary key-value pairs which are visible to all apps
- viewedByMeTime string? - The last time the file was viewed by the user (RFC 3339 date-time)
- writersCanShare boolean? - Whether users with only writer permission can modify the file's permissions
googleapis.drive: FilesResponse
Response from file search
Fields
- kind string - Identifies what kind of resource this is. Value: the fixed string "drive#fileList"
- nextPageToken string? - The page token for the next page of files. This will be absent if the end of the files list has been reached. If the token is rejected for any reason, it should be discarded, and pagination should be restarted from the first page of results
- incompleteSearch boolean? - Whether the search process was incomplete. If true, then some search results may be missing, Since all documents were not searched. This may occur when searching multiple drives with the "allDrives" corpora, but all corpora could not be searched. When this happens, it is suggested that clients narrow their query by choosing a different corpus such as "user" or "drive".
- files File[] - The list of files. If nextPageToken is populated, then this list may be incomplete and an additional page of results should be fetched.
googleapis.drive: GeneratedFileMetadata
Represents generated file metadata
Fields
- id string? - The ID of the file/folder
- kind string? - Identifies what kind of resource this is. Value: the fixed string "drive#file"
- explicitlyTrashed boolean? - Whether the file has been explicitly trashed, as opposed to recursively trashed from a parent folder
- trashingUser User? - If the file has been explicitly trashed, the user who trashed it. Only populated for items in shared drives
- trashedTime string? - The time that the item was trashed (RFC 3339 date-time). Only populated for items in shared drives
- parents string[]? - The IDs of the parent folders which contain the file. If not specified as part of a create request, the file will be placed directly in the user's My Drive folder. If not specified as part of a copy request, the file will inherit any discoverable parents of the source file Update requests must use the addParents and removeParents parameters to modify the parents list.
- spaces string[]? - The list of spaces which contain the file. The currently supported values are 'drive', 'appDataFolder' and 'photos'
- 'version int? -
- webContentLink string? - A link for downloading the content of the file in a browser. This is only available for files with binary content in Google Drive
- webViewLink string? - A link for opening the file in a relevant Google editor or viewer in a browser
- iconLink string? - A static, unauthenticated link to the file's icon
- hasThumbnail boolean? - Whether this file has a thumbnail. This does not indicate whether the requesting app has access to the thumbnail.To check access,look for the presence of the thumbnailLink field
- thumbnailLink string? - A short-lived link to the file's thumbnail, if available. Typically lasts on the order of hours. Only populated when the requesting app can access the file's content. If the file isn't shared publicly, the URL returned in Files.thumbnailLink must be fetched using a credentialed request
- thumbnailVersion int? - The thumbnail version for use in thumbnail cache invalidation
- viewedByMe boolean? - Whether the file has been viewed by this user
- modifiedByMe boolean? - Whether the file has been modified by this user
- createdTime string? - The time at which the file was created (RFC 3339 date-time)
- modifiedByMeTime string? - The last time the file was modified by the user (RFC 3339 date-time)
- sharedWithMeTime string? - The time at which the file was shared with the user, if applicable (RFC 3339 date-time). Not populated for items in shared drives
- sharingUser User? - The user who shared the file with the requesting user, if applicable
- owners User[]? - The owners of the file. Currently, only certain legacy files may have more than one owner. Not populated for items in shared drives
- driveId string? - ID of the shared drive the file resides in. Only populated for items in shared drives
- lastModifyingUser User? - The last user to modify the file
- shared boolean? - Whether the file has been shared. Not populated for items in shared drives
- ownedByMe boolean? - Whether the user owns the file. Not populated for items in shared drives
- capabilities Capabilities? - Capabilities the current user has on this file. Each capability corresponds to a fine-grained action that a user may take
- permissions Permissions[]? - The full list of permissions for the file. This is only available if the requesting user can share the file. Not populated for items in shared drives
- permissionIds string[]? - List of permission IDs for users with access to this file
- hasAugmentedPermissions boolean? - Whether there are permissions directly on this file. This field is only populated for items in shared drives
- fullFileExtension string? - The full file extension extracted from the name field. May contain multiple concatenated extensions, such as "tar.gz".This is only available for files with binary content in Google Drive
- fileExtension string? - The final component of fullFileExtension. This is only available for files with binary content in Google Drive
- md5Checksum string? - The MD5 checksum for the content of the file. This is only applicable to files with binary content in Google Drive
- size int? - The size of the file's content in bytes. This is applicable to binary files in Google Drive and Google Docs files
- quotaBytesUsed int? - The number of storage quota bytes used by the file. This includes the head revision as well as previous revisions with keepForever enabled
- headRevisionId string? - The ID of the file's head revision. This is currently only available for files with binary content in Google Drive
- imageMediaMetadata ImageMediaMetadata? - Additional metadata about image media, if available
- videoMediaMetadata VideoMediaMetadata? - Additional metadata about video media. This may not be available immediately upon upload
- isAppAuthorized boolean? - Whether the file was created or opened by the requesting app
- exportLinks StringKeyValuePairs? - Links for exporting Docs Editors files to specific formats
- shortcutDetails ShortcutDetails? - Shortcut file details.Only populated for shortcut files, which have the mimeType field set to application/vnd.google-apps.shortcut
- contentRestrictions ContentRestrictions? - Restrictions for accessing the content of the file. Only populated if such a restriction exists
googleapis.drive: ImageMediaMetadata
Additional metadata about image media, if available
Fields
- width int - The width of the video in pixels
- height int - The height of the image in pixels
- rotation int - The number of clockwise 90 degree rotations applied from the image's original orientation
- location Location - Geographic location information stored in the image
- time string - The date and time the photo was taken (EXIF DateTime)
- cameraMake string - The make of the camera used to create the photo
- cameraModel string - The model of the camera used to create the photo
- exposureTime int - The length of the exposure in seconds
- aperture int - The aperture used to create the photo (f-number)
- flashUsed boolean - Whether a flash was used to create the photo
- focalLength int - The focal length used to create the photo, in millimeters
- isoSpeed int - The ISO speed used to create the photo
- meteringMode string - The metering mode used to create the photo
- sensor string - The type of sensor used to create the photo
- exposureMode string - The length of the exposure, in seconds
- colorSpace string - The color space of the photo
- whiteBalance string - The white balance mode used to create the photo
- exposureBias int - The exposure bias of the photo (APEX value)
- maxApertureValue int - The smallest f-number of the lens at the focal length used to create the photo (APEX value)
- subjectDistance int - The distance to the subject of the photo, in meters
- lens string - The lens used to create the photo
googleapis.drive: Location
Geographic location information stored in the image.
Fields
- latitude float - The latitude stored in the image
- longitude float - The longitude stored in the image
- altitude float - The altitude stored in the image
googleapis.drive: PermissionDetails
Details of whether the permissions on this shared drive item are inherited or directly on this item. This is an output-only field which is present only for shared drive items.
Fields
- permissionType string - The permission type for this user
- role string - The primary role for this user
- inheritedFrom string - The ID of the item from which this permission is inherited. This is an output-only field
- inherited boolean - Whether this permission is inherited. This field is always populated. This is an output-only field
googleapis.drive: Permissions
A permission for a file. A permission grants a user, group, domain or the world access to a file or a folder hierarchy.
Fields
- kind string - Identifies what kind of resource this is. Value: the fixed string "drive#permission"
- id string - The ID of this permission. This is a unique identifier for the grantee, and is published in User resources as permissionId. IDs should be treated as opaque values
- 'type string -
- emailAddress string - The email address of the user or group to which this permission refers
- domain string - The domain to which this permission refers
- role string - The role granted by this permission
- view string - Indicates the view for this permission. Only populated for permissions that belong to a view. published is the only supported value
- allowFileDiscovery boolean - Whether the permission allows the file to be discovered through search. This is only applicable for permissions of type domain or anyone
- displayName string - The "pretty" name of the value of the permission
- photoLink string - A link to the user's profile photo, if available
- expirationTime string - The time at which this permission will expire (RFC 3339 date-time)
- permissionDetails PermissionDetails[]? - Details of whether the permissions on this shared drive item are inherited or directly on this item This is an output-only field which is present only for shared drive items.
- deleted boolean - Whether the account associated with this permission has been deleted. This field only pertains to user and group permissions
googleapis.drive: ShortcutDetails
Shortcut file details. Only populated for shortcut files, which have the mimeType field set to application/vnd.google-apps.shortcut.
Fields
- targetId string - The ID of the file that this shortcut points to
- targetMimeType string - The MIME type of the file that this shortcut points to. The value of this field is a snapshot of the target's MIME type, captured when the shortcut is created
googleapis.drive: StorageQuota
Record Type to accept float values.
Fields
- float... - Rest field
googleapis.drive: StringArrayValuePairs
Record Type to accept string[] values.
Fields
- string[]... - Rest field
googleapis.drive: StringKeyValuePairs
Record Type to accpet string values.
Fields
- string... - Rest field
googleapis.drive: Thumbnail
A thumbnail for the file. This will only be used if Google Drive cannot generate a standard thumbnail.
Fields
- image byte - The thumbnail data encoded with URL-safe Base64 (RFC 4648 section 5)
- mimeType string - The MIME type of the thumbnail
googleapis.drive: User
Represents User.
Fields
- kind string? - Identifies what kind of resource this is. Value: the fixed string "drive#user".
- displayName string? - A plain text displayable name for this user
- photoLink string? - A link to the user's profile photo, if available
- me boolean? - Whether this user is the requesting user
- permissionId string? - The user's ID as visible in Permission resources
- emailAddress string? - The email address of the user. This may not be present in certain contexts if the user has not made. their email address visible to the requester
googleapis.drive: VideoMediaMetadata
Additional metadata about video media. This may not be available immediately upon upload.
Fields
- width int - The width of the video in pixelsn
- height int - The height of the video in pixels
- durationMillis float - The duration of the video in milliseconds
Import
import ballerinax/googleapis.drive;
Metadata
Released date: over 3 years ago
Version: 2.1.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: slbeta6
Pull count
Total: 7100
Current verison: 286
Weekly downloads
Keywords
Content & Files/File Management & Storage
Cost/Free
Vendor/Google
Contributors