googleapis.docs
Module googleapis.docs
Definitions
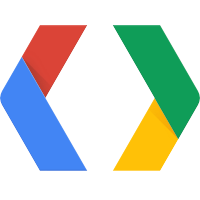
ballerinax/googleapis.docs Ballerina library
Overview
This is a generated connector for Google Docs API v1.0 OpenAPI specification. The Docs API provides access to read and write documents programmatically so that you can integrate data from various sources.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google Docs connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/googleapis.docs
module into the Ballerina project.
import ballerinax/googleapis.docs;
Step 2: Create a new connector instance
Create a docs:ClientConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
docs:ClientConfig clientConfig = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET>, refreshUrl: <REFRESH_URL>, refreshToken: <REFRESH_TOKEN> } }; docs:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve a Google Doc by document ID using the connector.
Retrieve a document by ID
public function main() { docs:Document|error response = baseClient->docsDocumentsGet("<DOCUMENT_ID>"); if (response is docs:Document) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.docs: Client
This is a generated connector for Google Docs API v1.0 OpenAPI specification. The Docs API provides access to reads and writes Google Docs documents.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Google account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://docs.googleapis.com/" - URL of the target service
createDocument
function createDocument(Document payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Document|error
Creates a blank document using the title given in the request. Other fields in the request, including any provided content, are ignored. Returns the created document.
Parameters
- payload Document -
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getDocument
function getDocument(string documentId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? suggestionsViewMode) returns Document|error
Gets the latest version of the specified document.
Parameters
- documentId string - The ID of the document to retrieve.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- suggestionsViewMode string? (default ()) - The suggestions view mode to apply to the document. This allows viewing the document with all suggestions inline, accepted or rejected. If one is not specified, DEFAULT_FOR_CURRENT_ACCESS is used.
batchUpdateDocuments
function batchUpdateDocuments(string documentId, BatchUpdateDocumentRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns BatchUpdateDocumentResponse|error
Applies one or more updates to the document. Each request is validated before being applied. If any request is not valid, then the entire request will fail and nothing will be applied. Some requests have replies to give you some information about how they are applied. Other requests do not need to return information; these each return an empty reply. The order of replies matches that of the requests. For example, suppose you call batchUpdate with four updates, and only the third one returns information. The response would have two empty replies, the reply to the third request, and another empty reply, in that order. Because other users may be editing the document, the document might not exactly reflect your changes: your changes may be altered with respect to collaborator changes. If there are no collaborators, the document should reflect your changes. In any case, the updates in your request are guaranteed to be applied together atomically.
Parameters
- documentId string - The ID of the document to update.
- payload BatchUpdateDocumentRequest -
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- BatchUpdateDocumentResponse|error - Successful response
Records
googleapis.docs: AutoText
A ParagraphElement representing a spot in the text that is dynamically replaced with content that can change over time, like a page number.
Fields
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. An AutoText may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this AutoText, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
- 'type string? - The type of this auto text.
googleapis.docs: Background
Represents the background of a document.
Fields
- color OptionalColor? - A color that can either be fully opaque or fully transparent.
googleapis.docs: BackgroundSuggestionState
A mask that indicates which of the fields on the base Background have been changed in this suggestion. For any field set to true, the Backgound has a new suggested value.
Fields
- backgroundColorSuggested boolean? - Indicates whether the current background color has been modified in this suggestion.
googleapis.docs: BatchUpdateDocumentRequest
Request message for BatchUpdateDocument.
Fields
- requests Request[]? - A list of updates to apply to the document.
- writeControl WriteControl? - Provides control over how write requests are executed.
googleapis.docs: BatchUpdateDocumentResponse
Response message from a BatchUpdateDocument request.
Fields
- documentId string? - The ID of the document to which the updates were applied to.
- replies Response[]? - The reply of the updates. This maps 1:1 with the updates, although replies to some requests may be empty.
- writeControl WriteControl? - Provides control over how write requests are executed.
googleapis.docs: Body
The document body. The body typically contains the full document contents except for headers, footers and footnotes.
Fields
- content StructuralElement[]? - The contents of the body. The indexes for the body's content begin at zero.
googleapis.docs: Bullet
Describes the bullet of a paragraph.
Fields
- listId string? - The ID of the list this paragraph belongs to.
- nestingLevel int? - The nesting level of this paragraph in the list.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: BulletSuggestionState
A mask that indicates which of the fields on the base Bullet have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- listIdSuggested boolean? - Indicates if there was a suggested change to the list_id.
- nestingLevelSuggested boolean? - Indicates if there was a suggested change to the nesting_level.
- textStyleSuggestionState TextStyleSuggestionState? - A mask that indicates which of the fields on the base TextStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.docs: Color
A solid color.
Fields
- rgbColor RgbColor? - An RGB color.
googleapis.docs: ColumnBreak
A ParagraphElement representing a column break. A column break makes the subsequent text start at the top of the next column.
Fields
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A ColumnBreak may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this ColumnBreak, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.docs: CreateFooterRequest
Creates a Footer. The new footer is applied to the SectionStyle at the location of the SectionBreak if specificed, otherwise it is applied to the DocumentStyle. If a footer of the specified type already exists, a 400 bad request error is returned.
Fields
- sectionBreakLocation Location? - A particular location in the document.
- 'type string? - The type of footer to create.
googleapis.docs: CreateFooterResponse
The result of creating a footer.
Fields
- footerId string? - The ID of the created footer.
googleapis.docs: CreateFootnoteRequest
Creates a Footnote segment and inserts a new FootnoteReference to it at the given location. The new Footnote segment will contain a space followed by a newline character.
Fields
- endOfSegmentLocation EndOfSegmentLocation? - Location at the end of a body, header, footer or footnote. The location is immediately before the last newline in the document segment.
- location Location? - A particular location in the document.
googleapis.docs: CreateFootnoteResponse
The result of creating a footnote.
Fields
- footnoteId string? - The ID of the created footnote.
googleapis.docs: CreateHeaderRequest
Creates a Header. The new header is applied to the SectionStyle at the location of the SectionBreak if specificed, otherwise it is applied to the DocumentStyle. If a header of the specified type already exists, a 400 bad request error is returned.
Fields
- sectionBreakLocation Location? - A particular location in the document.
- 'type string? - The type of header to create.
googleapis.docs: CreateHeaderResponse
The result of creating a header.
Fields
- headerId string? - The ID of the created header.
googleapis.docs: CreateNamedRangeRequest
Creates a NamedRange referencing the given range.
Fields
- name string? - The name of the NamedRange. Names do not need to be unique. Names must be at least 1 character and no more than 256 characters, measured in UTF-16 code units.
- range Range? - Specifies a contiguous range of text.
googleapis.docs: CreateNamedRangeResponse
The result of creating a named range.
Fields
- namedRangeId string? - The ID of the created named range.
googleapis.docs: CreateParagraphBulletsRequest
Creates bullets for all of the paragraphs that overlap with the given range. The nesting level of each paragraph will be determined by counting leading tabs in front of each paragraph. To avoid excess space between the bullet and the corresponding paragraph, these leading tabs are removed by this request. This may change the indices of parts of the text. If the paragraph immediately before paragraphs being updated is in a list with a matching preset, the paragraphs being updated are added to that preceding list.
Fields
- bulletPreset string? - The kinds of bullet glyphs to be used.
- range Range? - Specifies a contiguous range of text.
googleapis.docs: CropProperties
The crop properties of an image. The crop rectangle is represented using fractional offsets from the original content's four edges. - If the offset is in the interval (0, 1), the corresponding edge of crop rectangle is positioned inside of the image's original bounding rectangle. - If the offset is negative or greater than 1, the corresponding edge of crop rectangle is positioned outside of the image's original bounding rectangle. - If all offsets and rotation angle are 0, the image is not cropped.
Fields
- angle float? - The clockwise rotation angle of the crop rectangle around its center, in radians. Rotation is applied after the offsets.
- offsetBottom float? - The offset specifies how far inwards the bottom edge of the crop rectangle is from the bottom edge of the original content as a fraction of the original content's height.
- offsetLeft float? - The offset specifies how far inwards the left edge of the crop rectangle is from the left edge of the original content as a fraction of the original content's width.
- offsetRight float? - The offset specifies how far inwards the right edge of the crop rectangle is from the right edge of the original content as a fraction of the original content's width.
- offsetTop float? - The offset specifies how far inwards the top edge of the crop rectangle is from the top edge of the original content as a fraction of the original content's height.
googleapis.docs: CropPropertiesSuggestionState
A mask that indicates which of the fields on the base CropProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- angleSuggested boolean? - Indicates if there was a suggested change to angle.
- offsetBottomSuggested boolean? - Indicates if there was a suggested change to offset_bottom.
- offsetLeftSuggested boolean? - Indicates if there was a suggested change to offset_left.
- offsetRightSuggested boolean? - Indicates if there was a suggested change to offset_right.
- offsetTopSuggested boolean? - Indicates if there was a suggested change to offset_top.
googleapis.docs: DeleteContentRangeRequest
Deletes content from the document.
Fields
- range Range? - Specifies a contiguous range of text.
googleapis.docs: DeleteFooterRequest
Deletes a Footer from the document.
Fields
- footerId string? - The id of the footer to delete. If this footer is defined on DocumentStyle, the reference to this footer is removed, resulting in no footer of that type for the first section of the document. If this footer is defined on a SectionStyle, the reference to this footer is removed and the footer of that type is now continued from the previous section.
googleapis.docs: DeleteHeaderRequest
Deletes a Header from the document.
Fields
- headerId string? - The id of the header to delete. If this header is defined on DocumentStyle, the reference to this header is removed, resulting in no header of that type for the first section of the document. If this header is defined on a SectionStyle, the reference to this header is removed and the header of that type is now continued from the previous section.
googleapis.docs: DeleteNamedRangeRequest
Deletes a NamedRange.
Fields
- name string? - The name of the range(s) to delete. All named ranges with the given name will be deleted.
- namedRangeId string? - The ID of the named range to delete.
googleapis.docs: DeleteParagraphBulletsRequest
Deletes bullets from all of the paragraphs that overlap with the given range. The nesting level of each paragraph will be visually preserved by adding indent to the start of the corresponding paragraph.
Fields
- range Range? - Specifies a contiguous range of text.
googleapis.docs: DeletePositionedObjectRequest
Deletes a PositionedObject from the document.
Fields
- objectId string? - The ID of the positioned object to delete.
googleapis.docs: DeleteTableColumnRequest
Deletes a column from a table.
Fields
- tableCellLocation TableCellLocation? - Location of a single cell within a table.
googleapis.docs: DeleteTableRowRequest
Deletes a row from a table.
Fields
- tableCellLocation TableCellLocation? - Location of a single cell within a table.
googleapis.docs: Dimension
A magnitude in a single direction in the specified units.
Fields
- magnitude decimal? - The magnitude.
- unit string? - The units for magnitude.
googleapis.docs: Document
A Google Docs document.
Fields
- body Body? - The document body. The body typically contains the full document contents except for headers, footers and footnotes.
- documentId string? - Output only. The ID of the document.
- documentStyle DocumentStyle? - The style of the document.
- footers record {}? - Output only. The footers in the document, keyed by footer ID.
- footnotes record {}? - Output only. The footnotes in the document, keyed by footnote ID.
- headers record {}? - Output only. The headers in the document, keyed by header ID.
- inlineObjects record {}? - Output only. The inline objects in the document, keyed by object ID.
- lists record {}? - Output only. The lists in the document, keyed by list ID.
- namedRanges record {}? - Output only. The named ranges in the document, keyed by name.
- namedStyles NamedStyles? - The named styles. Paragraphs in the document can inherit their TextStyle and ParagraphStyle from these named styles.
- positionedObjects record {}? - Output only. The positioned objects in the document, keyed by object ID.
- revisionId string? - Output only. The revision ID of the document. Can be used in update requests to specify which revision of a document to apply updates to and how the request should behave if the document has been edited since that revision. Only populated if the user has edit access to the document. The format of the revision ID may change over time, so it should be treated opaquely. A returned revision ID is only guaranteed to be valid for 24 hours after it has been returned and cannot be shared across users. If the revision ID is unchanged between calls, then the document has not changed. Conversely, a changed ID (for the same document and user) usually means the document has been updated; however, a changed ID can also be due to internal factors such as ID format changes.
- suggestedDocumentStyleChanges record {}? - Output only. The suggested changes to the style of the document, keyed by suggestion ID.
- suggestedNamedStylesChanges record {}? - Output only. The suggested changes to the named styles of the document, keyed by suggestion ID.
- suggestionsViewMode string? - Output only. The suggestions view mode applied to the document. Note: When editing a document, changes must be based on a document with SUGGESTIONS_INLINE.
- title string? - The title of the document.
googleapis.docs: DocumentStyle
The style of the document.
Fields
- background Background? - Represents the background of a document.
- defaultFooterId string? - The ID of the default footer. If not set, there is no default footer. This property is read-only.
- defaultHeaderId string? - The ID of the default header. If not set, there is no default header. This property is read-only.
- evenPageFooterId string? - The ID of the footer used only for even pages. The value of use_even_page_header_footer determines whether to use the default_footer_id or this value for the footer on even pages. If not set, there is no even page footer. This property is read-only.
- evenPageHeaderId string? - The ID of the header used only for even pages. The value of use_even_page_header_footer determines whether to use the default_header_id or this value for the header on even pages. If not set, there is no even page header. This property is read-only.
- firstPageFooterId string? - The ID of the footer used only for the first page. If not set then a unique footer for the first page does not exist. The value of use_first_page_header_footer determines whether to use the default_footer_id or this value for the footer on the first page. If not set, there is no first page footer. This property is read-only.
- firstPageHeaderId string? - The ID of the header used only for the first page. If not set then a unique header for the first page does not exist. The value of use_first_page_header_footer determines whether to use the default_header_id or this value for the header on the first page. If not set, there is no first page header. This property is read-only.
- marginBottom Dimension? - A magnitude in a single direction in the specified units.
- marginFooter Dimension? - A magnitude in a single direction in the specified units.
- marginHeader Dimension? - A magnitude in a single direction in the specified units.
- marginLeft Dimension? - A magnitude in a single direction in the specified units.
- marginRight Dimension? - A magnitude in a single direction in the specified units.
- marginTop Dimension? - A magnitude in a single direction in the specified units.
- pageNumberStart int? - The page number from which to start counting the number of pages.
- pageSize Size? - A width and height.
- useCustomHeaderFooterMargins boolean? - Indicates whether DocumentStyle margin_header, SectionStyle margin_header and DocumentStyle margin_footer, SectionStyle margin_footer are respected. When false, the default values in the Docs editor for header and footer margin are used. This property is read-only.
- useEvenPageHeaderFooter boolean? - Indicates whether to use the even page header / footer IDs for the even pages.
- useFirstPageHeaderFooter boolean? - Indicates whether to use the first page header / footer IDs for the first page.
googleapis.docs: DocumentStyleSuggestionState
A mask that indicates which of the fields on the base DocumentStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- backgroundSuggestionState BackgroundSuggestionState? - A mask that indicates which of the fields on the base Background have been changed in this suggestion. For any field set to true, the Backgound has a new suggested value.
- defaultFooterIdSuggested boolean? - Indicates if there was a suggested change to default_footer_id.
- defaultHeaderIdSuggested boolean? - Indicates if there was a suggested change to default_header_id.
- evenPageFooterIdSuggested boolean? - Indicates if there was a suggested change to even_page_footer_id.
- evenPageHeaderIdSuggested boolean? - Indicates if there was a suggested change to even_page_header_id.
- firstPageFooterIdSuggested boolean? - Indicates if there was a suggested change to first_page_footer_id.
- firstPageHeaderIdSuggested boolean? - Indicates if there was a suggested change to first_page_header_id.
- marginBottomSuggested boolean? - Indicates if there was a suggested change to margin_bottom.
- marginFooterSuggested boolean? - Indicates if there was a suggested change to margin_footer.
- marginHeaderSuggested boolean? - Indicates if there was a suggested change to margin_header.
- marginLeftSuggested boolean? - Indicates if there was a suggested change to margin_left.
- marginRightSuggested boolean? - Indicates if there was a suggested change to margin_right.
- marginTopSuggested boolean? - Indicates if there was a suggested change to margin_top.
- pageNumberStartSuggested boolean? - Indicates if there was a suggested change to page_number_start.
- pageSizeSuggestionState SizeSuggestionState? - A mask that indicates which of the fields on the base Size have been changed in this suggestion. For any field set to true, the Size has a new suggested value.
- useCustomHeaderFooterMarginsSuggested boolean? - Indicates if there was a suggested change to use_custom_header_footer_margins.
- useEvenPageHeaderFooterSuggested boolean? - Indicates if there was a suggested change to use_even_page_header_footer.
- useFirstPageHeaderFooterSuggested boolean? - Indicates if there was a suggested change to use_first_page_header_footer.
googleapis.docs: EmbeddedDrawingProperties
The properties of an embedded drawing.
googleapis.docs: EmbeddedDrawingPropertiesSuggestionState
A mask that indicates which of the fields on the base EmbeddedDrawingProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: EmbeddedObject
An embedded object in the document.
Fields
- description string? - The description of the embedded object. The
title
anddescription
are both combined to display alt text.
- embeddedDrawingProperties EmbeddedDrawingProperties? - The properties of an embedded drawing.
- embeddedObjectBorder EmbeddedObjectBorder? - A border around an EmbeddedObject.
- imageProperties ImageProperties? - The properties of an image.
- linkedContentReference LinkedContentReference? - A reference to the external linked source content.
- marginBottom Dimension? - A magnitude in a single direction in the specified units.
- marginLeft Dimension? - A magnitude in a single direction in the specified units.
- marginRight Dimension? - A magnitude in a single direction in the specified units.
- marginTop Dimension? - A magnitude in a single direction in the specified units.
- size Size? - A width and height.
- title string? - The title of the embedded object. The
title
anddescription
are both combined to display alt text.
googleapis.docs: EmbeddedObjectBorder
A border around an EmbeddedObject.
Fields
- color OptionalColor? - A color that can either be fully opaque or fully transparent.
- dashStyle string? - The dash style of the border.
- propertyState string? - The property state of the border property.
- width Dimension? - A magnitude in a single direction in the specified units.
googleapis.docs: EmbeddedObjectBorderSuggestionState
A mask that indicates which of the fields on the base EmbeddedObjectBorder have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- colorSuggested boolean? - Indicates if there was a suggested change to color.
- dashStyleSuggested boolean? - Indicates if there was a suggested change to dash_style.
- propertyStateSuggested boolean? - Indicates if there was a suggested change to property_state.
- widthSuggested boolean? - Indicates if there was a suggested change to width.
googleapis.docs: EmbeddedObjectSuggestionState
A mask that indicates which of the fields on the base EmbeddedObject have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- descriptionSuggested boolean? - Indicates if there was a suggested change to description.
- embeddedDrawingPropertiesSuggestionState EmbeddedDrawingPropertiesSuggestionState? - A mask that indicates which of the fields on the base EmbeddedDrawingProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
- embeddedObjectBorderSuggestionState EmbeddedObjectBorderSuggestionState? - A mask that indicates which of the fields on the base EmbeddedObjectBorder have been changed in this suggestion. For any field set to true, there is a new suggested value.
- imagePropertiesSuggestionState ImagePropertiesSuggestionState? - A mask that indicates which of the fields on the base ImageProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
- linkedContentReferenceSuggestionState LinkedContentReferenceSuggestionState? - A mask that indicates which of the fields on the base LinkedContentReference have been changed in this suggestion. For any field set to true, there is a new suggested value.
- marginBottomSuggested boolean? - Indicates if there was a suggested change to margin_bottom.
- marginLeftSuggested boolean? - Indicates if there was a suggested change to margin_left.
- marginRightSuggested boolean? - Indicates if there was a suggested change to margin_right.
- marginTopSuggested boolean? - Indicates if there was a suggested change to margin_top.
- sizeSuggestionState SizeSuggestionState? - A mask that indicates which of the fields on the base Size have been changed in this suggestion. For any field set to true, the Size has a new suggested value.
- titleSuggested boolean? - Indicates if there was a suggested change to title.
googleapis.docs: EndOfSegmentLocation
Location at the end of a body, header, footer or footnote. The location is immediately before the last newline in the document segment.
Fields
- segmentId string? - The ID of the header, footer or footnote the location is in. An empty segment ID signifies the document's body.
googleapis.docs: Equation
A ParagraphElement representing an equation.
Fields
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A Equation may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
googleapis.docs: Footer
A document footer.
Fields
- content StructuralElement[]? - The contents of the footer. The indexes for a footer's content begin at zero.
- footerId string? - The ID of the footer.
googleapis.docs: Footnote
A document footnote.
Fields
- content StructuralElement[]? - The contents of the footnote. The indexes for a footnote's content begin at zero.
- footnoteId string? - The ID of the footnote.
googleapis.docs: FootnoteReference
A ParagraphElement representing a footnote reference. A footnote reference is the inline content rendered with a number and is used to identify the footnote.
Fields
- footnoteId string? - The ID of the footnote that contains the content of this footnote reference.
- footnoteNumber string? - The rendered number of this footnote.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A FootnoteReference may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this FootnoteReference, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: Header
A document header.
Fields
- content StructuralElement[]? - The contents of the header. The indexes for a header's content begin at zero.
- headerId string? - The ID of the header.
googleapis.docs: HorizontalRule
A ParagraphElement representing a horizontal line.
Fields
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A HorizontalRule may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this HorizontalRule, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: ImageProperties
The properties of an image.
Fields
- angle float? - The clockwise rotation angle of the image, in radians.
- brightness float? - The brightness effect of the image. The value should be in the interval [-1.0, 1.0], where 0 means no effect.
- contentUri string? - A URI to the image with a default lifetime of 30 minutes. This URI is tagged with the account of the requester. Anyone with the URI effectively accesses the image as the original requester. Access to the image may be lost if the document's sharing settings change.
- contrast float? - The contrast effect of the image. The value should be in the interval [-1.0, 1.0], where 0 means no effect.
- cropProperties CropProperties? - The crop properties of an image. The crop rectangle is represented using fractional offsets from the original content's four edges. - If the offset is in the interval (0, 1), the corresponding edge of crop rectangle is positioned inside of the image's original bounding rectangle. - If the offset is negative or greater than 1, the corresponding edge of crop rectangle is positioned outside of the image's original bounding rectangle. - If all offsets and rotation angle are 0, the image is not cropped.
- sourceUri string? - The source URI is the URI used to insert the image. The source URI can be empty.
- transparency float? - The transparency effect of the image. The value should be in the interval [0.0, 1.0], where 0 means no effect and 1 means completely transparent.
googleapis.docs: ImagePropertiesSuggestionState
A mask that indicates which of the fields on the base ImageProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- angleSuggested boolean? - Indicates if there was a suggested change to angle.
- brightnessSuggested boolean? - Indicates if there was a suggested change to brightness.
- contentUriSuggested boolean? - Indicates if there was a suggested change to content_uri.
- contrastSuggested boolean? - Indicates if there was a suggested change to contrast.
- cropPropertiesSuggestionState CropPropertiesSuggestionState? - A mask that indicates which of the fields on the base CropProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
- sourceUriSuggested boolean? - Indicates if there was a suggested change to source_uri.
- transparencySuggested boolean? - Indicates if there was a suggested change to transparency.
googleapis.docs: InlineObject
An object that appears inline with text. An InlineObject contains an EmbeddedObject such as an image.
Fields
- inlineObjectProperties InlineObjectProperties? - Properties of an InlineObject.
- objectId string? - The ID of this inline object.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInlineObjectPropertiesChanges record {}? - The suggested changes to the inline object properties, keyed by suggestion ID.
- suggestedInsertionId string? - The suggested insertion ID. If empty, then this is not a suggested insertion.
googleapis.docs: InlineObjectElement
A ParagraphElement that contains an InlineObject.
Fields
- inlineObjectId string? - The ID of the InlineObject this element contains.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. An InlineObjectElement may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this InlineObject, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: InlineObjectProperties
Properties of an InlineObject.
Fields
- embeddedObject EmbeddedObject? - An embedded object in the document.
googleapis.docs: InlineObjectPropertiesSuggestionState
A mask that indicates which of the fields on the base InlineObjectProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- embeddedObjectSuggestionState EmbeddedObjectSuggestionState? - A mask that indicates which of the fields on the base EmbeddedObject have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: InsertInlineImageRequest
Inserts an InlineObject containing an image at the given location.
Fields
- endOfSegmentLocation EndOfSegmentLocation? - Location at the end of a body, header, footer or footnote. The location is immediately before the last newline in the document segment.
- location Location? - A particular location in the document.
- objectSize Size? - A width and height.
- uri string? - The image URI. The image is fetched once at insertion time and a copy is stored for display inside the document. Images must be less than 50MB in size, cannot exceed 25 megapixels, and must be in one of PNG, JPEG, or GIF format. The provided URI can be at most 2 kB in length. The URI itself is saved with the image, and exposed via the ImageProperties.content_uri field.
googleapis.docs: InsertInlineImageResponse
The result of inserting an inline image.
Fields
- objectId string? - The ID of the created InlineObject.
googleapis.docs: InsertInlineSheetsChartResponse
The result of inserting an embedded Google Sheets chart.
Fields
- objectId string? - The object ID of the inserted chart.
googleapis.docs: InsertPageBreakRequest
Inserts a page break followed by a newline at the specified location.
Fields
- endOfSegmentLocation EndOfSegmentLocation? - Location at the end of a body, header, footer or footnote. The location is immediately before the last newline in the document segment.
- location Location? - A particular location in the document.
googleapis.docs: InsertSectionBreakRequest
Inserts a section break at the given location. A newline character will be inserted before the section break.
Fields
- endOfSegmentLocation EndOfSegmentLocation? - Location at the end of a body, header, footer or footnote. The location is immediately before the last newline in the document segment.
- location Location? - A particular location in the document.
- sectionType string? - The type of section to insert.
googleapis.docs: InsertTableColumnRequest
Inserts an empty column into a table.
Fields
- insertRight boolean? - Whether to insert new column to the right of the reference cell location. -
True
: insert to the right. -False
: insert to the left.
- tableCellLocation TableCellLocation? - Location of a single cell within a table.
googleapis.docs: InsertTableRequest
Inserts a table at the specified location. A newline character will be inserted before the inserted table.
Fields
- columns int? - The number of columns in the table.
- endOfSegmentLocation EndOfSegmentLocation? - Location at the end of a body, header, footer or footnote. The location is immediately before the last newline in the document segment.
- location Location? - A particular location in the document.
- rows int? - The number of rows in the table.
googleapis.docs: InsertTableRowRequest
Inserts an empty row into a table.
Fields
- insertBelow boolean? - Whether to insert new row below the reference cell location. -
True
: insert below the cell. -False
: insert above the cell.
- tableCellLocation TableCellLocation? - Location of a single cell within a table.
googleapis.docs: InsertTextRequest
Inserts text at the specified location.
Fields
- endOfSegmentLocation EndOfSegmentLocation? - Location at the end of a body, header, footer or footnote. The location is immediately before the last newline in the document segment.
- location Location? - A particular location in the document.
- text string? - The text to be inserted. Inserting a newline character will implicitly create a new Paragraph at that index. The paragraph style of the new paragraph will be copied from the paragraph at the current insertion index, including lists and bullets. Text styles for inserted text will be determined automatically, generally preserving the styling of neighboring text. In most cases, the text style for the inserted text will match the text immediately before the insertion index. Some control characters (U+0000-U+0008, U+000C-U+001F) and characters from the Unicode Basic Multilingual Plane Private Use Area (U+E000-U+F8FF) will be stripped out of the inserted text.
googleapis.docs: Link
A reference to another portion of a document or an external URL resource.
Fields
- bookmarkId string? - The ID of a bookmark in this document.
- headingId string? - The ID of a heading in this document.
- url string? - An external URL.
googleapis.docs: LinkedContentReference
A reference to the external linked source content.
Fields
- sheetsChartReference SheetsChartReference? - A reference to a linked chart embedded from Google Sheets.
googleapis.docs: LinkedContentReferenceSuggestionState
A mask that indicates which of the fields on the base LinkedContentReference have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- sheetsChartReferenceSuggestionState SheetsChartReferenceSuggestionState? - A mask that indicates which of the fields on the base SheetsChartReference have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: List
A List represents the list attributes for a group of paragraphs that all belong to the same list. A paragraph that is part of a list has a reference to the list's ID in its bullet.
Fields
- listProperties ListProperties? - The properties of a list which describe the look and feel of bullets belonging to paragraphs associated with a list.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this list.
- suggestedInsertionId string? - The suggested insertion ID. If empty, then this is not a suggested insertion.
- suggestedListPropertiesChanges record {}? - The suggested changes to the list properties, keyed by suggestion ID.
googleapis.docs: ListProperties
The properties of a list which describe the look and feel of bullets belonging to paragraphs associated with a list.
Fields
- nestingLevels NestingLevel[]? - Describes the properties of the bullets at the associated level. A list has at most nine levels of nesting with nesting level 0 corresponding to the top-most level and nesting level 8 corresponding to the most nested level. The nesting levels are returned in ascending order with the least nested returned first.
googleapis.docs: ListPropertiesSuggestionState
A mask that indicates which of the fields on the base ListProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- nestingLevelsSuggestionStates NestingLevelSuggestionState[]? - A mask that indicates which of the fields on the corresponding NestingLevel in nesting_levels have been changed in this suggestion. The nesting level suggestion states are returned in ascending order of the nesting level with the least nested returned first.
googleapis.docs: Location
A particular location in the document.
Fields
- index int? - The zero-based index, in UTF-16 code units. The index is relative to the beginning of the segment specified by segment_id.
- segmentId string? - The ID of the header, footer or footnote the location is in. An empty segment ID signifies the document's body.
googleapis.docs: MergeTableCellsRequest
Merges cells in a Table.
Fields
- tableRange TableRange? - A table range represents a reference to a subset of a table. It's important to note that the cells specified by a table range do not necessarily form a rectangle. For example, let's say we have a 3 x 3 table where all the cells of the last row are merged together. The table looks like this: [ ] A table range with table cell location = (table_start_location, row = 0, column = 0), row span = 3 and column span = 2 specifies the following cells: x x [ x x x ]
googleapis.docs: NamedRange
A collection of Ranges with the same named range ID. Named ranges allow developers to associate parts of a document with an arbitrary user-defined label so their contents can be programmatically read or edited at a later time. A document can contain multiple named ranges with the same name, but every named range has a unique ID. A named range is created with a single Range, and content inserted inside a named range generally expands that range. However, certain document changes can cause the range to be split into multiple ranges. Named ranges are not private. All applications and collaborators that have access to the document can see its named ranges.
Fields
- name string? - The name of the named range.
- namedRangeId string? - The ID of the named range.
- ranges Range[]? - The ranges that belong to this named range.
googleapis.docs: NamedRanges
A collection of all the NamedRanges in the document that share a given name.
Fields
- name string? - The name that all the named ranges share.
- namedRanges NamedRange[]? - The NamedRanges that share the same name.
googleapis.docs: NamedStyle
A named style. Paragraphs in the document can inherit their TextStyle and ParagraphStyle from this named style when they have the same named style type.
Fields
- namedStyleType string? - The type of this named style.
- paragraphStyle ParagraphStyle? - Styles that apply to a whole paragraph. Inherited paragraph styles are represented as unset fields in this message. A paragraph style's parent depends on where the paragraph style is defined: * The ParagraphStyle on a Paragraph inherits from the paragraph's corresponding named style type. * The ParagraphStyle on a named style inherits from the normal text named style. * The ParagraphStyle of the normal text named style inherits from the default paragraph style in the Docs editor. * The ParagraphStyle on a Paragraph element that is contained in a table may inherit its paragraph style from the table style. If the paragraph style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: NamedStyles
The named styles. Paragraphs in the document can inherit their TextStyle and ParagraphStyle from these named styles.
Fields
- styles NamedStyle[]? - The named styles. There is an entry for each of the possible named style types.
googleapis.docs: NamedStylesSuggestionState
The suggestion state of a NamedStyles message.
Fields
- stylesSuggestionStates NamedStyleSuggestionState[]? - A mask that indicates which of the fields on the corresponding NamedStyle in styles have been changed in this suggestion. The order of these named style suggestion states match the order of the corresponding named style within the named styles suggestion.
googleapis.docs: NamedStyleSuggestionState
A suggestion state of a NamedStyle message.
Fields
- namedStyleType string? - The named style type that this suggestion state corresponds to. This field is provided as a convenience for matching the NamedStyleSuggestionState with its corresponding NamedStyle.
- paragraphStyleSuggestionState ParagraphStyleSuggestionState? - A mask that indicates which of the fields on the base ParagraphStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
- textStyleSuggestionState TextStyleSuggestionState? - A mask that indicates which of the fields on the base TextStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: NestingLevel
Contains properties describing the look and feel of a list bullet at a given level of nesting.
Fields
- bulletAlignment string? - The alignment of the bullet within the space allotted for rendering the bullet.
- glyphFormat string? - The format string used by bullets at this level of nesting. The glyph format contains one or more placeholders, and these placeholder are replaced with the appropriate values depending on the glyph_type or glyph_symbol. The placeholders follow the pattern
%[nesting_level]
. Furthermore, placeholders can have prefixes and suffixes. Thus, the glyph format follows the pattern%[nesting_level]
. Note that the prefix and suffix are optional and can be arbitrary strings. For example, the glyph format%0.
indicates that the rendered glyph will replace the placeholder with the corresponding glyph for nesting level 0 followed by a period as the suffix. So a list with a glyph type of UPPER_ALPHA and glyph format%0.
at nesting level 0 will result in a list with rendered glyphsA.
B.
C.
The glyph format can contain placeholders for the current nesting level as well as placeholders for parent nesting levels. For example, a list can have a glyph format of%0.
at nesting level 0 and a glyph format of%0.%1.
at nesting level 1. Assuming both nesting levels have DECIMAL glyph types, this would result in a list with rendered glyphs1.
2.
2.1.
2.2.
3.
For nesting levels that are ordered, the string that replaces a placeholder in the glyph format for a particular paragraph depends on the paragraph's order within the list.
- glyphSymbol string? - A custom glyph symbol used by bullets when paragraphs at this level of nesting are unordered. The glyph symbol replaces placeholders within the glyph_format. For example, if the glyph_symbol is the solid circle corresponding to Unicode U+25cf code point and the glyph_format is
%0
, the rendered glyph would be the solid circle.
- glyphType string? - The type of glyph used by bullets when paragraphs at this level of nesting are ordered. The glyph type determines the type of glyph used to replace placeholders within the glyph_format when paragraphs at this level of nesting are ordered. For example, if the nesting level is 0, the glyph_format is
%0.
and the glyph type is DECIMAL, then the rendered glyph would replace the placeholder%0
in the glyph format with a number corresponding to list item's order within the list.
- indentFirstLine Dimension? - A magnitude in a single direction in the specified units.
- indentStart Dimension? - A magnitude in a single direction in the specified units.
- startNumber int? - The number of the first list item at this nesting level. A value of 0 is treated as a value of 1 for lettered lists and roman numeraled lists, i.e. for values of both 0 and 1, lettered and roman numeraled lists will begin at
a
andi
respectively. This value is ignored for nesting levels with unordered glyphs.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: NestingLevelSuggestionState
A mask that indicates which of the fields on the base NestingLevel have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- bulletAlignmentSuggested boolean? - Indicates if there was a suggested change to bullet_alignment.
- glyphFormatSuggested boolean? - Indicates if there was a suggested change to glyph_format.
- glyphSymbolSuggested boolean? - Indicates if there was a suggested change to glyph_symbol.
- glyphTypeSuggested boolean? - Indicates if there was a suggested change to glyph_type.
- indentFirstLineSuggested boolean? - Indicates if there was a suggested change to indent_first_line.
- indentStartSuggested boolean? - Indicates if there was a suggested change to indent_start.
- startNumberSuggested boolean? - Indicates if there was a suggested change to start_number.
- textStyleSuggestionState TextStyleSuggestionState? - A mask that indicates which of the fields on the base TextStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.docs: ObjectReferences
A collection of object IDs.
Fields
- objectIds string[]? - The object IDs.
googleapis.docs: OptionalColor
A color that can either be fully opaque or fully transparent.
Fields
- color Color? - A solid color.
googleapis.docs: PageBreak
A ParagraphElement representing a page break. A page break makes the subsequent text start at the top of the next page.
Fields
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A PageBreak may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this PageBreak, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: Paragraph
A StructuralElement representing a paragraph. A paragraph is a range of content that is terminated with a newline character.
Fields
- bullet Bullet? - Describes the bullet of a paragraph.
- elements ParagraphElement[]? - The content of the paragraph broken down into its component parts.
- paragraphStyle ParagraphStyle? - Styles that apply to a whole paragraph. Inherited paragraph styles are represented as unset fields in this message. A paragraph style's parent depends on where the paragraph style is defined: * The ParagraphStyle on a Paragraph inherits from the paragraph's corresponding named style type. * The ParagraphStyle on a named style inherits from the normal text named style. * The ParagraphStyle of the normal text named style inherits from the default paragraph style in the Docs editor. * The ParagraphStyle on a Paragraph element that is contained in a table may inherit its paragraph style from the table style. If the paragraph style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
- positionedObjectIds string[]? - The IDs of the positioned objects tethered to this paragraph.
- suggestedBulletChanges record {}? - The suggested changes to this paragraph's bullet.
- suggestedParagraphStyleChanges record {}? - The suggested paragraph style changes to this paragraph, keyed by suggestion ID.
- suggestedPositionedObjectIds record {}? - The IDs of the positioned objects that are suggested to be attached to this paragraph, keyed by suggestion ID.
googleapis.docs: ParagraphBorder
A border around a paragraph.
Fields
- color OptionalColor? - A color that can either be fully opaque or fully transparent.
- dashStyle string? - The dash style of the border.
- padding Dimension? - A magnitude in a single direction in the specified units.
- width Dimension? - A magnitude in a single direction in the specified units.
googleapis.docs: ParagraphElement
A ParagraphElement describes content within a Paragraph.
Fields
- autoText AutoText? - A ParagraphElement representing a spot in the text that is dynamically replaced with content that can change over time, like a page number.
- columnBreak ColumnBreak? - A ParagraphElement representing a column break. A column break makes the subsequent text start at the top of the next column.
- endIndex int? - The zero-base end index of this paragraph element, exclusive, in UTF-16 code units.
- equation Equation? - A ParagraphElement representing an equation.
- footnoteReference FootnoteReference? - A ParagraphElement representing a footnote reference. A footnote reference is the inline content rendered with a number and is used to identify the footnote.
- horizontalRule HorizontalRule? - A ParagraphElement representing a horizontal line.
- inlineObjectElement InlineObjectElement? - A ParagraphElement that contains an InlineObject.
- pageBreak PageBreak? - A ParagraphElement representing a page break. A page break makes the subsequent text start at the top of the next page.
- person Person? - A person or email address mentioned in a document. These mentions behave as a single, immutable element containing the person's name or email address.
- richLink RichLink? - A link to a Google resource (e.g., a file in Drive, a YouTube video, a Calendar event, etc.).
- startIndex int? - The zero-based start index of this paragraph element, in UTF-16 code units.
- textRun TextRun? - A ParagraphElement that represents a run of text that all has the same styling.
googleapis.docs: ParagraphStyle
Styles that apply to a whole paragraph. Inherited paragraph styles are represented as unset fields in this message. A paragraph style's parent depends on where the paragraph style is defined: * The ParagraphStyle on a Paragraph inherits from the paragraph's corresponding named style type. * The ParagraphStyle on a named style inherits from the normal text named style. * The ParagraphStyle of the normal text named style inherits from the default paragraph style in the Docs editor. * The ParagraphStyle on a Paragraph element that is contained in a table may inherit its paragraph style from the table style. If the paragraph style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
Fields
- alignment string? - The text alignment for this paragraph.
- avoidWidowAndOrphan boolean? - Whether to avoid widows and orphans for the paragraph. If unset, the value is inherited from the parent.
- borderBetween ParagraphBorder? - A border around a paragraph.
- borderBottom ParagraphBorder? - A border around a paragraph.
- borderLeft ParagraphBorder? - A border around a paragraph.
- borderRight ParagraphBorder? - A border around a paragraph.
- borderTop ParagraphBorder? - A border around a paragraph.
- direction string? - The text direction of this paragraph. If unset, the value defaults to LEFT_TO_RIGHT since paragraph direction is not inherited.
- headingId string? - The heading ID of the paragraph. If empty, then this paragraph is not a heading. This property is read-only.
- indentEnd Dimension? - A magnitude in a single direction in the specified units.
- indentFirstLine Dimension? - A magnitude in a single direction in the specified units.
- indentStart Dimension? - A magnitude in a single direction in the specified units.
- keepLinesTogether boolean? - Whether all lines of the paragraph should be laid out on the same page or column if possible. If unset, the value is inherited from the parent.
- keepWithNext boolean? - Whether at least a part of this paragraph should be laid out on the same page or column as the next paragraph if possible. If unset, the value is inherited from the parent.
- lineSpacing float? - The amount of space between lines, as a percentage of normal, where normal is represented as 100.0. If unset, the value is inherited from the parent.
- namedStyleType string? - The named style type of the paragraph. Since updating the named style type affects other properties within ParagraphStyle, the named style type is applied before the other properties are updated.
- shading Shading? - The shading of a paragraph.
- spaceAbove Dimension? - A magnitude in a single direction in the specified units.
- spaceBelow Dimension? - A magnitude in a single direction in the specified units.
- spacingMode string? - The spacing mode for the paragraph.
- tabStops TabStop[]? - A list of the tab stops for this paragraph. The list of tab stops is not inherited. This property is read-only.
googleapis.docs: ParagraphStyleSuggestionState
A mask that indicates which of the fields on the base ParagraphStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- alignmentSuggested boolean? - Indicates if there was a suggested change to alignment.
- avoidWidowAndOrphanSuggested boolean? - Indicates if there was a suggested change to avoid_widow_and_orphan.
- borderBetweenSuggested boolean? - Indicates if there was a suggested change to border_between.
- borderBottomSuggested boolean? - Indicates if there was a suggested change to border_bottom.
- borderLeftSuggested boolean? - Indicates if there was a suggested change to border_left.
- borderRightSuggested boolean? - Indicates if there was a suggested change to border_right.
- borderTopSuggested boolean? - Indicates if there was a suggested change to border_top.
- directionSuggested boolean? - Indicates if there was a suggested change to direction.
- headingIdSuggested boolean? - Indicates if there was a suggested change to heading_id.
- indentEndSuggested boolean? - Indicates if there was a suggested change to indent_end.
- indentFirstLineSuggested boolean? - Indicates if there was a suggested change to indent_first_line.
- indentStartSuggested boolean? - Indicates if there was a suggested change to indent_start.
- keepLinesTogetherSuggested boolean? - Indicates if there was a suggested change to keep_lines_together.
- keepWithNextSuggested boolean? - Indicates if there was a suggested change to keep_with_next.
- lineSpacingSuggested boolean? - Indicates if there was a suggested change to line_spacing.
- namedStyleTypeSuggested boolean? - Indicates if there was a suggested change to named_style_type.
- shadingSuggestionState ShadingSuggestionState? - A mask that indicates which of the fields on the base Shading have been changed in this suggested change. For any field set to true, there is a new suggested value.
- spaceAboveSuggested boolean? - Indicates if there was a suggested change to space_above.
- spaceBelowSuggested boolean? - Indicates if there was a suggested change to space_below.
- spacingModeSuggested boolean? - Indicates if there was a suggested change to spacing_mode.
googleapis.docs: Person
A person or email address mentioned in a document. These mentions behave as a single, immutable element containing the person's name or email address.
Fields
- personId string? - Output only. The unique ID of this link.
- personProperties PersonProperties? - Properties specific to a linked Person.
- suggestedDeletionIds string[]? - IDs for suggestions that remove this person link from the document. A Person might have multiple deletion IDs if, for example, multiple users suggest to delete it. If empty, then this person link isn't suggested for deletion.
- suggestedInsertionIds string[]? - IDs for suggestions that insert this person link into the document. A Person might have multiple insertion IDs if it is a nested suggested change (a suggestion within a suggestion made by a different user, for example). If empty, then this person link isn't a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this Person, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: PersonProperties
Properties specific to a linked Person.
Fields
- email string? - Output only. The email address linked to this Person. This field is always present.
- name string? - Output only. The name of the person if it is displayed in the link text instead of the person's email address.
googleapis.docs: PositionedObject
An object that is tethered to a Paragraph and positioned relative to the beginning of the paragraph. A PositionedObject contains an EmbeddedObject such as an image.
Fields
- objectId string? - The ID of this positioned object.
- positionedObjectProperties PositionedObjectProperties? - Properties of a PositionedObject.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionId string? - The suggested insertion ID. If empty, then this is not a suggested insertion.
- suggestedPositionedObjectPropertiesChanges record {}? - The suggested changes to the positioned object properties, keyed by suggestion ID.
googleapis.docs: PositionedObjectPositioning
The positioning of a PositionedObject. The positioned object is positioned relative to the beginning of the Paragraph it is tethered to.
Fields
- layout string? - The layout of this positioned object.
- leftOffset Dimension? - A magnitude in a single direction in the specified units.
- topOffset Dimension? - A magnitude in a single direction in the specified units.
googleapis.docs: PositionedObjectPositioningSuggestionState
A mask that indicates which of the fields on the base PositionedObjectPositioning have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- layoutSuggested boolean? - Indicates if there was a suggested change to layout.
- leftOffsetSuggested boolean? - Indicates if there was a suggested change to left_offset.
- topOffsetSuggested boolean? - Indicates if there was a suggested change to top_offset.
googleapis.docs: PositionedObjectProperties
Properties of a PositionedObject.
Fields
- embeddedObject EmbeddedObject? - An embedded object in the document.
- positioning PositionedObjectPositioning? - The positioning of a PositionedObject. The positioned object is positioned relative to the beginning of the Paragraph it is tethered to.
googleapis.docs: PositionedObjectPropertiesSuggestionState
A mask that indicates which of the fields on the base PositionedObjectProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- embeddedObjectSuggestionState EmbeddedObjectSuggestionState? - A mask that indicates which of the fields on the base EmbeddedObject have been changed in this suggestion. For any field set to true, there is a new suggested value.
- positioningSuggestionState PositionedObjectPositioningSuggestionState? - A mask that indicates which of the fields on the base PositionedObjectPositioning have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.docs: Range
Specifies a contiguous range of text.
Fields
- endIndex int? - The zero-based end index of this range, exclusive, in UTF-16 code units. In all current uses, an end index must be provided. This field is an Int32Value in order to accommodate future use cases with open-ended ranges.
- segmentId string? - The ID of the header, footer or footnote that this range is contained in. An empty segment ID signifies the document's body.
- startIndex int? - The zero-based start index of this range, in UTF-16 code units. In all current uses, a start index must be provided. This field is an Int32Value in order to accommodate future use cases with open-ended ranges.
googleapis.docs: ReplaceAllTextRequest
Replaces all instances of text matching a criteria with replace text.
Fields
- containsText SubstringMatchCriteria? - A criteria that matches a specific string of text in the document.
- replaceText string? - The text that will replace the matched text.
googleapis.docs: ReplaceAllTextResponse
The result of replacing text.
Fields
- occurrencesChanged int? - The number of occurrences changed by replacing all text.
googleapis.docs: ReplaceImageRequest
Replaces an existing image with a new image. Replacing an image removes some image effects from the existing image in order to mirror the behavior of the Docs editor.
Fields
- imageObjectId string? - The ID of the existing image that will be replaced.
- imageReplaceMethod string? - The replacement method.
- uri string? - The URI of the new image. The image is fetched once at insertion time and a copy is stored for display inside the document. Images must be less than 50MB in size, cannot exceed 25 megapixels, and must be in one of PNG, JPEG, or GIF format. The provided URI can be at most 2 kB in length. The URI itself is saved with the image, and exposed via the ImageProperties.source_uri field.
googleapis.docs: ReplaceNamedRangeContentRequest
Replaces the contents of the specified NamedRange or NamedRanges with the given replacement content. Note that an individual NamedRange may consist of multiple discontinuous ranges. In this case, only the content in the first range will be replaced. The other ranges and their content will be deleted. In cases where replacing or deleting any ranges would result in an invalid document structure, a 400 bad request error is returned.
Fields
- namedRangeId string? - The ID of the named range whose content will be replaced. If there is no named range with the given ID a 400 bad request error is returned.
- namedRangeName string? - The name of the NamedRanges whose content will be replaced. If there are multiple named ranges with the given name, then the content of each one will be replaced. If there are no named ranges with the given name, then the request will be a no-op.
- text string? - Replaces the content of the specified named range(s) with the given text.
googleapis.docs: Request
A single update to apply to a document.
Fields
- createFooter CreateFooterRequest? - Creates a Footer. The new footer is applied to the SectionStyle at the location of the SectionBreak if specificed, otherwise it is applied to the DocumentStyle. If a footer of the specified type already exists, a 400 bad request error is returned.
- createFootnote CreateFootnoteRequest? - Creates a Footnote segment and inserts a new FootnoteReference to it at the given location. The new Footnote segment will contain a space followed by a newline character.
- createHeader CreateHeaderRequest? - Creates a Header. The new header is applied to the SectionStyle at the location of the SectionBreak if specificed, otherwise it is applied to the DocumentStyle. If a header of the specified type already exists, a 400 bad request error is returned.
- createNamedRange CreateNamedRangeRequest? - Creates a NamedRange referencing the given range.
- createParagraphBullets CreateParagraphBulletsRequest? - Creates bullets for all of the paragraphs that overlap with the given range. The nesting level of each paragraph will be determined by counting leading tabs in front of each paragraph. To avoid excess space between the bullet and the corresponding paragraph, these leading tabs are removed by this request. This may change the indices of parts of the text. If the paragraph immediately before paragraphs being updated is in a list with a matching preset, the paragraphs being updated are added to that preceding list.
- deleteContentRange DeleteContentRangeRequest? - Deletes content from the document.
- deleteFooter DeleteFooterRequest? - Deletes a Footer from the document.
- deleteHeader DeleteHeaderRequest? - Deletes a Header from the document.
- deleteNamedRange DeleteNamedRangeRequest? - Deletes a NamedRange.
- deleteParagraphBullets DeleteParagraphBulletsRequest? - Deletes bullets from all of the paragraphs that overlap with the given range. The nesting level of each paragraph will be visually preserved by adding indent to the start of the corresponding paragraph.
- deletePositionedObject DeletePositionedObjectRequest? - Deletes a PositionedObject from the document.
- deleteTableColumn DeleteTableColumnRequest? - Deletes a column from a table.
- deleteTableRow DeleteTableRowRequest? - Deletes a row from a table.
- insertInlineImage InsertInlineImageRequest? - Inserts an InlineObject containing an image at the given location.
- insertPageBreak InsertPageBreakRequest? - Inserts a page break followed by a newline at the specified location.
- insertSectionBreak InsertSectionBreakRequest? - Inserts a section break at the given location. A newline character will be inserted before the section break.
- insertTable InsertTableRequest? - Inserts a table at the specified location. A newline character will be inserted before the inserted table.
- insertTableColumn InsertTableColumnRequest? - Inserts an empty column into a table.
- insertTableRow InsertTableRowRequest? - Inserts an empty row into a table.
- insertText InsertTextRequest? - Inserts text at the specified location.
- mergeTableCells MergeTableCellsRequest? - Merges cells in a Table.
- replaceAllText ReplaceAllTextRequest? - Replaces all instances of text matching a criteria with replace text.
- replaceImage ReplaceImageRequest? - Replaces an existing image with a new image. Replacing an image removes some image effects from the existing image in order to mirror the behavior of the Docs editor.
- replaceNamedRangeContent ReplaceNamedRangeContentRequest? - Replaces the contents of the specified NamedRange or NamedRanges with the given replacement content. Note that an individual NamedRange may consist of multiple discontinuous ranges. In this case, only the content in the first range will be replaced. The other ranges and their content will be deleted. In cases where replacing or deleting any ranges would result in an invalid document structure, a 400 bad request error is returned.
- unmergeTableCells UnmergeTableCellsRequest? - Unmerges cells in a Table.
- updateDocumentStyle UpdateDocumentStyleRequest? - Updates the DocumentStyle.
- updateParagraphStyle UpdateParagraphStyleRequest? - Update the styling of all paragraphs that overlap with the given range.
- updateSectionStyle UpdateSectionStyleRequest? - Updates the SectionStyle.
- updateTableCellStyle UpdateTableCellStyleRequest? - Updates the style of a range of table cells.
- updateTableColumnProperties UpdateTableColumnPropertiesRequest? - Updates the TableColumnProperties of columns in a table.
- updateTableRowStyle UpdateTableRowStyleRequest? - Updates the TableRowStyle of rows in a table.
- updateTextStyle UpdateTextStyleRequest? - Update the styling of text.
googleapis.docs: Response
A single response from an update.
Fields
- createFooter CreateFooterResponse? - The result of creating a footer.
- createFootnote CreateFootnoteResponse? - The result of creating a footnote.
- createHeader CreateHeaderResponse? - The result of creating a header.
- createNamedRange CreateNamedRangeResponse? - The result of creating a named range.
- insertInlineImage InsertInlineImageResponse? - The result of inserting an inline image.
- insertInlineSheetsChart InsertInlineSheetsChartResponse? - The result of inserting an embedded Google Sheets chart.
- replaceAllText ReplaceAllTextResponse? - The result of replacing text.
googleapis.docs: RgbColor
An RGB color.
Fields
- blue float? - The blue component of the color, from 0.0 to 1.0.
- green float? - The green component of the color, from 0.0 to 1.0.
- red float? - The red component of the color, from 0.0 to 1.0.
googleapis.docs: RichLink
A link to a Google resource (e.g., a file in Drive, a YouTube video, a Calendar event, etc.).
Fields
- richLinkId string? - Output only. The ID of this link.
- richLinkProperties RichLinkProperties? - Properties specific to a RichLink.
- suggestedDeletionIds string[]? - IDs for suggestions that remove this link from the document. A RichLink might have multiple deletion IDs if, for example, multiple users suggest to delete it. If empty, then this person link isn't suggested for deletion.
- suggestedInsertionIds string[]? - IDs for suggestions that insert this link into the document. A RichLink might have multiple insertion IDs if it is a nested suggested change (a suggestion within a suggestion made by a different user, for example). If empty, then this person link isn't a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this RichLink, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: RichLinkProperties
Properties specific to a RichLink.
Fields
- title string? - Output only. The title of the RichLink as displayed in the link. This title matches the title of the linked resource at the time of the insertion or last update of the link. This field is always present.
- uri string? - Output only. The URI to the RichLink. This is always present.
googleapis.docs: SectionBreak
A StructuralElement representing a section break. A section is a range of content which has the same SectionStyle. A section break represents the start of a new section, and the section style applies to the section after the section break. The document body always begins with a section break.
Fields
- sectionStyle SectionStyle? - The styling that applies to a section.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A SectionBreak may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
googleapis.docs: SectionColumnProperties
Properties that apply to a section's column.
Fields
- paddingEnd Dimension? - A magnitude in a single direction in the specified units.
- width Dimension? - A magnitude in a single direction in the specified units.
googleapis.docs: SectionStyle
The styling that applies to a section.
Fields
- columnProperties SectionColumnProperties[]? - The section's columns properties. If empty, the section contains one column with the default properties in the Docs editor. A section can be updated to have no more than three columns. When updating this property, setting a concrete value is required. Unsetting this property will result in a 400 bad request error.
- columnSeparatorStyle string? - The style of column separators. This style can be set even when there is one column in the section. When updating this property, setting a concrete value is required. Unsetting this property results in a 400 bad request error.
- contentDirection string? - The content direction of this section. If unset, the value defaults to LEFT_TO_RIGHT. When updating this property, setting a concrete value is required. Unsetting this property results in a 400 bad request error.
- defaultFooterId string? - The ID of the default footer. If unset, the value inherits from the previous SectionBreak's SectionStyle. If the value is unset in the first SectionBreak, it inherits from DocumentStyle's default_footer_id. This property is read-only.
- defaultHeaderId string? - The ID of the default header. If unset, the value inherits from the previous SectionBreak's SectionStyle. If the value is unset in the first SectionBreak, it inherits from DocumentStyle's default_header_id. This property is read-only.
- evenPageFooterId string? - The ID of the footer used only for even pages. If the value of DocumentStyle's use_even_page_header_footer is true, this value is used for the footers on even pages in the section. If it is false, the footers on even pages uses the default_footer_id. If unset, the value inherits from the previous SectionBreak's SectionStyle. If the value is unset in the first SectionBreak, it inherits from DocumentStyle's even_page_footer_id. This property is read-only.
- evenPageHeaderId string? - The ID of the header used only for even pages. If the value of DocumentStyle's use_even_page_header_footer is true, this value is used for the headers on even pages in the section. If it is false, the headers on even pages uses the default_header_id. If unset, the value inherits from the previous SectionBreak's SectionStyle. If the value is unset in the first SectionBreak, it inherits from DocumentStyle's even_page_header_id. This property is read-only.
- firstPageFooterId string? - The ID of the footer used only for the first page of the section. If use_first_page_header_footer is true, this value is used for the footer on the first page of the section. If it is false, the footer on the first page of the section uses the default_footer_id. If unset, the value inherits from the previous SectionBreak's SectionStyle. If the value is unset in the first SectionBreak, it inherits from DocumentStyle's first_page_footer_id. This property is read-only.
- firstPageHeaderId string? - The ID of the header used only for the first page of the section. If use_first_page_header_footer is true, this value is used for the header on the first page of the section. If it is false, the header on the first page of the section uses the default_header_id. If unset, the value inherits from the previous SectionBreak's SectionStyle. If the value is unset in the first SectionBreak, it inherits from DocumentStyle's first_page_header_id. This property is read-only.
- marginBottom Dimension? - A magnitude in a single direction in the specified units.
- marginFooter Dimension? - A magnitude in a single direction in the specified units.
- marginHeader Dimension? - A magnitude in a single direction in the specified units.
- marginLeft Dimension? - A magnitude in a single direction in the specified units.
- marginRight Dimension? - A magnitude in a single direction in the specified units.
- marginTop Dimension? - A magnitude in a single direction in the specified units.
- pageNumberStart int? - The page number from which to start counting the number of pages for this section. If unset, page numbering continues from the previous section. If the value is unset in the first SectionBreak, refer to DocumentStyle's page_number_start. When updating this property, setting a concrete value is required. Unsetting this property results in a 400 bad request error.
- sectionType string? - Output only. The type of section.
- useFirstPageHeaderFooter boolean? - Indicates whether to use the first page header / footer IDs for the first page of the section. If unset, it inherits from DocumentStyle's use_first_page_header_footer for the first section. If the value is unset for subsequent sectors, it should be interpreted as false. When updating this property, setting a concrete value is required. Unsetting this property results in a 400 bad request error.
googleapis.docs: Shading
The shading of a paragraph.
Fields
- backgroundColor OptionalColor? - A color that can either be fully opaque or fully transparent.
googleapis.docs: ShadingSuggestionState
A mask that indicates which of the fields on the base Shading have been changed in this suggested change. For any field set to true, there is a new suggested value.
Fields
- backgroundColorSuggested boolean? - Indicates if there was a suggested change to the Shading.
googleapis.docs: SheetsChartReference
A reference to a linked chart embedded from Google Sheets.
Fields
- chartId int? - The ID of the specific chart in the Google Sheets spreadsheet that is embedded.
- spreadsheetId string? - The ID of the Google Sheets spreadsheet that contains the source chart.
googleapis.docs: SheetsChartReferenceSuggestionState
A mask that indicates which of the fields on the base SheetsChartReference have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- chartIdSuggested boolean? - Indicates if there was a suggested change to chart_id.
- spreadsheetIdSuggested boolean? - Indicates if there was a suggested change to spreadsheet_id.
googleapis.docs: Size
A width and height.
Fields
- height Dimension? - A magnitude in a single direction in the specified units.
- width Dimension? - A magnitude in a single direction in the specified units.
googleapis.docs: SizeSuggestionState
A mask that indicates which of the fields on the base Size have been changed in this suggestion. For any field set to true, the Size has a new suggested value.
Fields
- heightSuggested boolean? - Indicates if there was a suggested change to height.
- widthSuggested boolean? - Indicates if there was a suggested change to width.
googleapis.docs: StructuralElement
A StructuralElement describes content that provides structure to the document.
Fields
- endIndex int? - The zero-based end index of this structural element, exclusive, in UTF-16 code units.
- paragraph Paragraph? - A StructuralElement representing a paragraph. A paragraph is a range of content that is terminated with a newline character.
- sectionBreak SectionBreak? - A StructuralElement representing a section break. A section is a range of content which has the same SectionStyle. A section break represents the start of a new section, and the section style applies to the section after the section break. The document body always begins with a section break.
- startIndex int? - The zero-based start index of this structural element, in UTF-16 code units.
- 'table Table? - A StructuralElement representing a table.
- tableOfContents TableOfContents? - A StructuralElement representing a table of contents.
googleapis.docs: SubstringMatchCriteria
A criteria that matches a specific string of text in the document.
Fields
- matchCase boolean? - Indicates whether the search should respect case: -
True
: the search is case sensitive. -False
: the search is case insensitive.
- text string? - The text to search for in the document.
googleapis.docs: SuggestedBullet
A suggested change to a Bullet.
Fields
- bullet Bullet? - Describes the bullet of a paragraph.
- bulletSuggestionState BulletSuggestionState? - A mask that indicates which of the fields on the base Bullet have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: SuggestedDocumentStyle
A suggested change to the DocumentStyle.
Fields
- documentStyle DocumentStyle? - The style of the document.
- documentStyleSuggestionState DocumentStyleSuggestionState? - A mask that indicates which of the fields on the base DocumentStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: SuggestedInlineObjectProperties
A suggested change to InlineObjectProperties.
Fields
- inlineObjectProperties InlineObjectProperties? - Properties of an InlineObject.
- inlineObjectPropertiesSuggestionState InlineObjectPropertiesSuggestionState? - A mask that indicates which of the fields on the base InlineObjectProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: SuggestedListProperties
A suggested change to ListProperties.
Fields
- listProperties ListProperties? - The properties of a list which describe the look and feel of bullets belonging to paragraphs associated with a list.
- listPropertiesSuggestionState ListPropertiesSuggestionState? - A mask that indicates which of the fields on the base ListProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: SuggestedNamedStyles
A suggested change to the NamedStyles.
Fields
- namedStyles NamedStyles? - The named styles. Paragraphs in the document can inherit their TextStyle and ParagraphStyle from these named styles.
- namedStylesSuggestionState NamedStylesSuggestionState? - The suggestion state of a NamedStyles message.
googleapis.docs: SuggestedParagraphStyle
A suggested change to a ParagraphStyle.
Fields
- paragraphStyle ParagraphStyle? - Styles that apply to a whole paragraph. Inherited paragraph styles are represented as unset fields in this message. A paragraph style's parent depends on where the paragraph style is defined: * The ParagraphStyle on a Paragraph inherits from the paragraph's corresponding named style type. * The ParagraphStyle on a named style inherits from the normal text named style. * The ParagraphStyle of the normal text named style inherits from the default paragraph style in the Docs editor. * The ParagraphStyle on a Paragraph element that is contained in a table may inherit its paragraph style from the table style. If the paragraph style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
- paragraphStyleSuggestionState ParagraphStyleSuggestionState? - A mask that indicates which of the fields on the base ParagraphStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: SuggestedPositionedObjectProperties
A suggested change to PositionedObjectProperties.
Fields
- positionedObjectProperties PositionedObjectProperties? - Properties of a PositionedObject.
- positionedObjectPropertiesSuggestionState PositionedObjectPropertiesSuggestionState? - A mask that indicates which of the fields on the base PositionedObjectProperties have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: SuggestedTableCellStyle
A suggested change to a TableCellStyle.
Fields
- tableCellStyle TableCellStyle? - The style of a TableCell. Inherited table cell styles are represented as unset fields in this message. A table cell style can inherit from the table's style.
- tableCellStyleSuggestionState TableCellStyleSuggestionState? - A mask that indicates which of the fields on the base TableCellStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: SuggestedTableRowStyle
A suggested change to a TableRowStyle.
Fields
- tableRowStyle TableRowStyle? - Styles that apply to a table row.
- tableRowStyleSuggestionState TableRowStyleSuggestionState? - A mask that indicates which of the fields on the base TableRowStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: SuggestedTextStyle
A suggested change to a TextStyle.
Fields
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
- textStyleSuggestionState TextStyleSuggestionState? - A mask that indicates which of the fields on the base TextStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
googleapis.docs: Table
A StructuralElement representing a table.
Fields
- columns int? - Number of columns in the table. It is possible for a table to be non-rectangular, so some rows may have a different number of cells.
- rows int? - Number of rows in the table.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A Table may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- tableRows TableRow[]? - The contents and style of each row.
- tableStyle TableStyle? - Styles that apply to a table.
googleapis.docs: TableCell
The contents and style of a cell in a Table.
Fields
- content StructuralElement[]? - The content of the cell.
- endIndex int? - The zero-based end index of this cell, exclusive, in UTF-16 code units.
- startIndex int? - The zero-based start index of this cell, in UTF-16 code units.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A TableCell may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTableCellStyleChanges record {}? - The suggested changes to the table cell style, keyed by suggestion ID.
- tableCellStyle TableCellStyle? - The style of a TableCell. Inherited table cell styles are represented as unset fields in this message. A table cell style can inherit from the table's style.
googleapis.docs: TableCellBorder
A border around a table cell. Table cell borders cannot be transparent. To hide a table cell border, make its width 0.
Fields
- color OptionalColor? - A color that can either be fully opaque or fully transparent.
- dashStyle string? - The dash style of the border.
- width Dimension? - A magnitude in a single direction in the specified units.
googleapis.docs: TableCellLocation
Location of a single cell within a table.
Fields
- columnIndex int? - The zero-based column index. For example, the second column in the table has a column index of 1.
- rowIndex int? - The zero-based row index. For example, the second row in the table has a row index of 1.
- tableStartLocation Location? - A particular location in the document.
googleapis.docs: TableCellStyle
The style of a TableCell. Inherited table cell styles are represented as unset fields in this message. A table cell style can inherit from the table's style.
Fields
- backgroundColor OptionalColor? - A color that can either be fully opaque or fully transparent.
- borderBottom TableCellBorder? - A border around a table cell. Table cell borders cannot be transparent. To hide a table cell border, make its width 0.
- borderLeft TableCellBorder? - A border around a table cell. Table cell borders cannot be transparent. To hide a table cell border, make its width 0.
- borderRight TableCellBorder? - A border around a table cell. Table cell borders cannot be transparent. To hide a table cell border, make its width 0.
- borderTop TableCellBorder? - A border around a table cell. Table cell borders cannot be transparent. To hide a table cell border, make its width 0.
- columnSpan int? - The column span of the cell. This property is read-only.
- contentAlignment string? - The alignment of the content in the table cell. The default alignment matches the alignment for newly created table cells in the Docs editor.
- paddingBottom Dimension? - A magnitude in a single direction in the specified units.
- paddingLeft Dimension? - A magnitude in a single direction in the specified units.
- paddingRight Dimension? - A magnitude in a single direction in the specified units.
- paddingTop Dimension? - A magnitude in a single direction in the specified units.
- rowSpan int? - The row span of the cell. This property is read-only.
googleapis.docs: TableCellStyleSuggestionState
A mask that indicates which of the fields on the base TableCellStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- backgroundColorSuggested boolean? - Indicates if there was a suggested change to background_color.
- borderBottomSuggested boolean? - Indicates if there was a suggested change to border_bottom.
- borderLeftSuggested boolean? - Indicates if there was a suggested change to border_left.
- borderRightSuggested boolean? - Indicates if there was a suggested change to border_right.
- borderTopSuggested boolean? - Indicates if there was a suggested change to border_top.
- columnSpanSuggested boolean? - Indicates if there was a suggested change to column_span.
- contentAlignmentSuggested boolean? - Indicates if there was a suggested change to content_alignment.
- paddingBottomSuggested boolean? - Indicates if there was a suggested change to padding_bottom.
- paddingLeftSuggested boolean? - Indicates if there was a suggested change to padding_left.
- paddingRightSuggested boolean? - Indicates if there was a suggested change to padding_right.
- paddingTopSuggested boolean? - Indicates if there was a suggested change to padding_top.
- rowSpanSuggested boolean? - Indicates if there was a suggested change to row_span.
googleapis.docs: TableColumnProperties
The properties of a column in a table.
Fields
- width Dimension? - A magnitude in a single direction in the specified units.
- widthType string? - The width type of the column.
googleapis.docs: TableOfContents
A StructuralElement representing a table of contents.
Fields
- content StructuralElement[]? - The content of the table of contents.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A TableOfContents may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
googleapis.docs: TableRange
A table range represents a reference to a subset of a table. It's important to note that the cells specified by a table range do not necessarily form a rectangle. For example, let's say we have a 3 x 3 table where all the cells of the last row are merged together. The table looks like this: [ ] A table range with table cell location = (table_start_location, row = 0, column = 0), row span = 3 and column span = 2 specifies the following cells: x x [ x x x ]
Fields
- columnSpan int? - The column span of the table range.
- rowSpan int? - The row span of the table range.
- tableCellLocation TableCellLocation? - Location of a single cell within a table.
googleapis.docs: TableRow
The contents and style of a row in a Table.
Fields
- endIndex int? - The zero-based end index of this row, exclusive, in UTF-16 code units.
- startIndex int? - The zero-based start index of this row, in UTF-16 code units.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A TableRow may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTableRowStyleChanges record {}? - The suggested style changes to this row, keyed by suggestion ID.
- tableCells TableCell[]? - The contents and style of each cell in this row. It is possible for a table to be non-rectangular, so some rows may have a different number of cells than other rows in the same table.
- tableRowStyle TableRowStyle? - Styles that apply to a table row.
googleapis.docs: TableRowStyle
Styles that apply to a table row.
Fields
- minRowHeight Dimension? - A magnitude in a single direction in the specified units.
googleapis.docs: TableRowStyleSuggestionState
A mask that indicates which of the fields on the base TableRowStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- minRowHeightSuggested boolean? - Indicates if there was a suggested change to min_row_height.
googleapis.docs: TableStyle
Styles that apply to a table.
Fields
- tableColumnProperties TableColumnProperties[]? - The properties of each column. Note that in Docs, tables contain rows and rows contain cells, similar to HTML. So the properties for a row can be found on the row's table_row_style.
googleapis.docs: TabStop
A tab stop within a paragraph.
Fields
- alignment string? - The alignment of this tab stop. If unset, the value defaults to START.
- offset Dimension? - A magnitude in a single direction in the specified units.
googleapis.docs: TextRun
A ParagraphElement that represents a run of text that all has the same styling.
Fields
- content string? - The text of this run. Any non-text elements in the run are replaced with the Unicode character U+E907.
- suggestedDeletionIds string[]? - The suggested deletion IDs. If empty, then there are no suggested deletions of this content.
- suggestedInsertionIds string[]? - The suggested insertion IDs. A TextRun may have multiple insertion IDs if it is a nested suggested change. If empty, then this is not a suggested insertion.
- suggestedTextStyleChanges record {}? - The suggested text style changes to this run, keyed by suggestion ID.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: TextStyle
Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
Fields
- backgroundColor OptionalColor? - A color that can either be fully opaque or fully transparent.
- baselineOffset string? - The text's vertical offset from its normal position. Text with
SUPERSCRIPT
orSUBSCRIPT
baseline offsets is automatically rendered in a smaller font size, computed based on thefont_size
field. Thefont_size
itself is not affected by changes in this field.
- bold boolean? - Whether or not the text is rendered as bold.
- fontSize Dimension? - A magnitude in a single direction in the specified units.
- foregroundColor OptionalColor? - A color that can either be fully opaque or fully transparent.
- italic boolean? - Whether or not the text is italicized.
- link Link? - A reference to another portion of a document or an external URL resource.
- smallCaps boolean? - Whether or not the text is in small capital letters.
- strikethrough boolean? - Whether or not the text is struck through.
- underline boolean? - Whether or not the text is underlined.
- weightedFontFamily WeightedFontFamily? - Represents a font family and weight of text.
googleapis.docs: TextStyleSuggestionState
A mask that indicates which of the fields on the base TextStyle have been changed in this suggestion. For any field set to true, there is a new suggested value.
Fields
- backgroundColorSuggested boolean? - Indicates if there was a suggested change to background_color.
- baselineOffsetSuggested boolean? - Indicates if there was a suggested change to baseline_offset.
- boldSuggested boolean? - Indicates if there was a suggested change to bold.
- fontSizeSuggested boolean? - Indicates if there was a suggested change to font_size.
- foregroundColorSuggested boolean? - Indicates if there was a suggested change to foreground_color.
- italicSuggested boolean? - Indicates if there was a suggested change to italic.
- linkSuggested boolean? - Indicates if there was a suggested change to link.
- smallCapsSuggested boolean? - Indicates if there was a suggested change to small_caps.
- strikethroughSuggested boolean? - Indicates if there was a suggested change to strikethrough.
- underlineSuggested boolean? - Indicates if there was a suggested change to underline.
- weightedFontFamilySuggested boolean? - Indicates if there was a suggested change to weighted_font_family.
googleapis.docs: UnmergeTableCellsRequest
Unmerges cells in a Table.
Fields
- tableRange TableRange? - A table range represents a reference to a subset of a table. It's important to note that the cells specified by a table range do not necessarily form a rectangle. For example, let's say we have a 3 x 3 table where all the cells of the last row are merged together. The table looks like this: [ ] A table range with table cell location = (table_start_location, row = 0, column = 0), row span = 3 and column span = 2 specifies the following cells: x x [ x x x ]
googleapis.docs: UpdateDocumentStyleRequest
Updates the DocumentStyle.
Fields
- documentStyle DocumentStyle? - The style of the document.
- fields string? - The fields that should be updated. At least one field must be specified. The root
document_style
is implied and should not be specified. A single"*"
can be used as short-hand for listing every field. For example to update the background, setfields
to"background"
.
googleapis.docs: UpdateParagraphStyleRequest
Update the styling of all paragraphs that overlap with the given range.
Fields
- fields string? - The fields that should be updated. At least one field must be specified. The root
paragraph_style
is implied and should not be specified. For example, to update the paragraph style's alignment property, setfields
to"alignment"
. To reset a property to its default value, include its field name in the field mask but leave the field itself unset.
- paragraphStyle ParagraphStyle? - Styles that apply to a whole paragraph. Inherited paragraph styles are represented as unset fields in this message. A paragraph style's parent depends on where the paragraph style is defined: * The ParagraphStyle on a Paragraph inherits from the paragraph's corresponding named style type. * The ParagraphStyle on a named style inherits from the normal text named style. * The ParagraphStyle of the normal text named style inherits from the default paragraph style in the Docs editor. * The ParagraphStyle on a Paragraph element that is contained in a table may inherit its paragraph style from the table style. If the paragraph style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
- range Range? - Specifies a contiguous range of text.
googleapis.docs: UpdateSectionStyleRequest
Updates the SectionStyle.
Fields
- fields string? - The fields that should be updated. At least one field must be specified. The root
section_style
is implied and must not be specified. A single"*"
can be used as short-hand for listing every field. For example to update the left margin, setfields
to"margin_left"
.
- range Range? - Specifies a contiguous range of text.
- sectionStyle SectionStyle? - The styling that applies to a section.
googleapis.docs: UpdateTableCellStyleRequest
Updates the style of a range of table cells.
Fields
- fields string? - The fields that should be updated. At least one field must be specified. The root
tableCellStyle
is implied and should not be specified. A single"*"
can be used as short-hand for listing every field. For example to update the table cell background color, setfields
to"backgroundColor"
. To reset a property to its default value, include its field name in the field mask but leave the field itself unset.
- tableCellStyle TableCellStyle? - The style of a TableCell. Inherited table cell styles are represented as unset fields in this message. A table cell style can inherit from the table's style.
- tableRange TableRange? - A table range represents a reference to a subset of a table. It's important to note that the cells specified by a table range do not necessarily form a rectangle. For example, let's say we have a 3 x 3 table where all the cells of the last row are merged together. The table looks like this: [ ] A table range with table cell location = (table_start_location, row = 0, column = 0), row span = 3 and column span = 2 specifies the following cells: x x [ x x x ]
- tableStartLocation Location? - A particular location in the document.
googleapis.docs: UpdateTableColumnPropertiesRequest
Updates the TableColumnProperties of columns in a table.
Fields
- columnIndices int[]? - The list of zero-based column indices whose property should be updated. If no indices are specified, all columns will be updated.
- fields string? - The fields that should be updated. At least one field must be specified. The root
tableColumnProperties
is implied and should not be specified. A single"*"
can be used as short-hand for listing every field. For example to update the column width, setfields
to"width"
.
- tableColumnProperties TableColumnProperties? - The properties of a column in a table.
- tableStartLocation Location? - A particular location in the document.
googleapis.docs: UpdateTableRowStyleRequest
Updates the TableRowStyle of rows in a table.
Fields
- fields string? - The fields that should be updated. At least one field must be specified. The root
tableRowStyle
is implied and should not be specified. A single"*"
can be used as short-hand for listing every field. For example to update the minimum row height, setfields
to"min_row_height"
.
- rowIndices int[]? - The list of zero-based row indices whose style should be updated. If no indices are specified, all rows will be updated.
- tableRowStyle TableRowStyle? - Styles that apply to a table row.
- tableStartLocation Location? - A particular location in the document.
googleapis.docs: UpdateTextStyleRequest
Update the styling of text.
Fields
- fields string? - The fields that should be updated. At least one field must be specified. The root
text_style
is implied and should not be specified. A single"*"
can be used as short-hand for listing every field. For example, to update the text style to bold, setfields
to"bold"
. To reset a property to its default value, include its field name in the field mask but leave the field itself unset.
- range Range? - Specifies a contiguous range of text.
- textStyle TextStyle? - Represents the styling that can be applied to text. Inherited text styles are represented as unset fields in this message. A text style's parent depends on where the text style is defined: * The TextStyle of text in a Paragraph inherits from the paragraph's corresponding named style type. * The TextStyle on a named style inherits from the normal text named style. * The TextStyle of the normal text named style inherits from the default text style in the Docs editor. * The TextStyle on a Paragraph element that is contained in a table may inherit its text style from the table style. If the text style does not inherit from a parent, unsetting fields will revert the style to a value matching the defaults in the Docs editor.
googleapis.docs: WeightedFontFamily
Represents a font family and weight of text.
Fields
- fontFamily string? - The font family of the text. The font family can be any font from the Font menu in Docs or from [Google Fonts] (https://fonts.google.com/). If the font name is unrecognized, the text is rendered in
Arial
.
- weight int? - The weight of the font. This field can have any value that is a multiple of
100
between100
and900
, inclusive. This range corresponds to the numerical values described in the CSS 2.1 Specification, section 15.6, with non-numerical values disallowed. The default value is400
("normal"). The font weight makes up just one component of the rendered font weight. The rendered weight is determined by a combination of theweight
and the text style's resolvedbold
value, after accounting for inheritance: * If the text is bold and the weight is less than400
, the rendered weight is 400. * If the text is bold and the weight is greater than or equal to400
but is less than700
, the rendered weight is700
. * If the weight is greater than or equal to700
, the rendered weight is equal to the weight. * If the text is not bold, the rendered weight is equal to the weight.
googleapis.docs: WriteControl
Provides control over how write requests are executed.
Fields
- requiredRevisionId string? - The revision ID of the document that the write request will be applied to. If this is not the latest revision of the document, the request will not be processed and will return a 400 bad request error. When a required revision ID is returned in a response, it indicates the revision ID of the document after the request was applied.
- targetRevisionId string? - The target revision ID of the document that the write request will be applied to. If collaborator changes have occurred after the document was read using the API, the changes produced by this write request will be transformed against the collaborator changes. This results in a new revision of the document which incorporates both the changes in the request and the collaborator changes, and the Docs server will resolve conflicting changes. When using
target_revision_id
, the API client can be thought of as another collaborator of the document. The target revision ID may only be used to write to recent versions of a document. If the target revision is too far behind the latest revision, the request will not be processed and will return a 400 bad request error and the request should be retried after reading the latest version of the document. In most cases arevision_id
will remain valid for use as a target revision for several minutes after it is read, but for frequently-edited documents this window may be shorter.
Import
import ballerinax/googleapis.docs;
Metadata
Released date: over 2 years ago
Version: 1.4.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.2.1
GraalVM compatible: Yes
Pull count
Total: 59
Current verison: 0
Weekly downloads
Keywords
Content & Files/Documents
Cost/Freemium
Vendor/Google
Contributors
Dependencies