googleapis.discovery
Module googleapis.discovery
Definitions
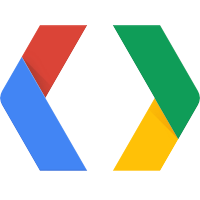
ballerinax/googleapis.discovery Ballerina library
Overview
This is a generated connector for Google Discovery REST API v1 OpenAPI specification. The Google Discovery API provides information about other Google APIs, such as what APIs are available, the resource, and method details for each API.
Quickstart
To use the Google Discovery connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/googleapis.discovery
module into the Ballerina project.
import ballerinax/googleapis.discovery;
Step 2: Create a new connector instance
Create a connector client instance
discovery:Client baseClient = check new;
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve list of available google apis
public function main() { discovery:DirectoryList|error response = baseClient->listAPIList(); if (response is discovery:DirectoryList) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.discovery: Client
This is a generated connector for Google Discovery REST API v1 OpenAPI specification. Provides information about other Google APIs, such as what APIs are available, the resource, and method details for each API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization doesn't require setting the API credentials.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://www.googleapis.com/discovery/v1" - URL of the target service
listAPIList
function listAPIList(string? alt, string? fields, string? quotaUser, string? userIp, string? name, boolean? preferred) returns DirectoryList|error
Retrieve the list of APIs supported at this endpoint.
Parameters
- alt string? (default ()) - Data format for the response.
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - An opaque string that represents a user for quota purposes. Must not exceed 40 characters.
- userIp string? (default ()) - Deprecated. Please use quotaUser instead.
- name string? (default ()) - Only include APIs with the given name.
- preferred boolean? (default ()) - Return only the preferred version of an API.
Return Type
- DirectoryList|error - Successful response
getAPIDescription
function getAPIDescription(string api, string 'version, string? alt, string? fields, string? quotaUser, string? userIp) returns RestDescription|error
Retrieve the description of a particular version of an api.
Parameters
- api string - The name of the API.
- 'version string - The version of the API.
- alt string? (default ()) - Data format for the response.
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - An opaque string that represents a user for quota purposes. Must not exceed 40 characters.
- userIp string? (default ()) - Deprecated. Please use quotaUser instead.
Return Type
- RestDescription|error - Successful response
Records
googleapis.discovery: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.discovery: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.discovery: DirectoryList
Fields
- discoveryVersion string? - Indicate the version of the Discovery API used to generate this doc.
- items DirectorylistItems[]? - The individual directory entries. One entry per api/version pair.
- kind string? - The kind for this response.
googleapis.discovery: DirectorylistIcons
Links to 16x16 and 32x32 icons representing the API.
Fields
- x16 string? - The URL of the 16x16 icon.
- x32 string? - The URL of the 32x32 icon.
googleapis.discovery: DirectorylistItems
Fields
- description string? - The description of this API.
- discoveryLink string? - A link to the discovery document.
- discoveryRestUrl string? - The URL for the discovery REST document.
- documentationLink string? - A link to human readable documentation for the API.
- icons DirectorylistIcons? - Links to 16x16 and 32x32 icons representing the API.
- id string? - The id of this API.
- kind string? - The kind for this response.
- labels string[]? - Labels for the status of this API, such as labs or deprecated.
- name string? - The name of the API.
- preferred boolean? - True if this version is the preferred version to use.
- title string? - The title of this API.
- 'version string? - The version of the API.
googleapis.discovery: JsonSchema
Fields
- '\$ref string? - A reference to another schema. The value of this property is the "id" of another schema.
- additionalProperties JsonSchema? -
- annotations JsonschemaAnnotations? - Additional information about this property.
- description string? - A description of this object.
- 'enum string[]? - Values this parameter may take (if it is an enum).
- enumDescriptions string[]? - The descriptions for the enums. Each position maps to the corresponding value in the "enum" array.
- format string? - An additional regular expression or key that helps constrain the value. For more details see: http://tools.ietf.org/html/draft-zyp-json-schema-03#section-5.23
- id string? - Unique identifier for this schema.
- items JsonSchema? -
- location string? - Whether this parameter goes in the query or the path for REST requests.
- maximum string? - The maximum value of this parameter.
- minimum string? - The minimum value of this parameter.
- pattern string? - The regular expression this parameter must conform to. Uses Java 6 regex format: http://docs.oracle.com/javase/6/docs/api/java/util/regex/Pattern.html
- properties record {}? - If this is a schema for an object, list the schema for each property of this object.
- readOnly boolean? - The value is read-only, generated by the service. The value cannot be modified by the client. If the value is included in a POST, PUT, or PATCH request, it is ignored by the service.
- repeated boolean? - Whether this parameter may appear multiple times.
- required boolean? - Whether the parameter is required.
- 'type string? - The value type for this schema. A list of values can be found here: http://tools.ietf.org/html/draft-zyp-json-schema-03#section-5.1
- variant JsonschemaVariant? - In a variant data type, the value of one property is used to determine how to interpret the entire entity. Its value must exist in a map of descriminant values to schema names.
googleapis.discovery: JsonschemaAnnotations
Additional information about this property.
Fields
- required string[]? - A list of methods for which this property is required on requests.
googleapis.discovery: JsonschemaVariant
In a variant data type, the value of one property is used to determine how to interpret the entire entity. Its value must exist in a map of descriminant values to schema names.
Fields
- discriminant string? - The name of the type discriminant property.
- 'map JsonschemaVariantMap[]? - The map of discriminant value to schema to use for parsing..
googleapis.discovery: JsonschemaVariantMap
Fields
- '\$ref string? -
- type_value string? -
googleapis.discovery: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.discovery: RestDescription
Fields
- auth RestdescriptionAuth? - Authentication information.
- basePath string? - [DEPRECATED] The base path for REST requests.
- baseUrl string? - [DEPRECATED] The base URL for REST requests.
- batchPath string? - The path for REST batch requests.
- canonicalName string? - Indicates how the API name should be capitalized and split into various parts. Useful for generating pretty class names.
- description string? - The description of this API.
- discoveryVersion string? - Indicate the version of the Discovery API used to generate this doc.
- documentationLink string? - A link to human readable documentation for the API.
- etag string? - The ETag for this response.
- exponentialBackoffDefault boolean? - Enable exponential backoff for suitable methods in the generated clients.
- features string[]? - A list of supported features for this API.
- icons DirectorylistIcons? - Links to 16x16 and 32x32 icons representing the API.
- id string? - The ID of this API.
- kind string? - The kind for this response.
- labels string[]? - Labels for the status of this API, such as labs or deprecated.
- methods record {}? - API-level methods for this API.
- name string? - The name of this API.
- ownerDomain string? - The domain of the owner of this API. Together with the ownerName and a packagePath values, this can be used to generate a library for this API which would have a unique fully qualified name.
- ownerName string? - The name of the owner of this API. See ownerDomain.
- packagePath string? - The package of the owner of this API. See ownerDomain.
- parameters record {}? - Common parameters that apply across all apis.
- protocol string? - The protocol described by this document.
- resources record {}? - The resources in this API.
- revision string? - The version of this API.
- rootUrl string? - The root URL under which all API services live.
- schemas record {}? - The schemas for this API.
- servicePath string? - The base path for all REST requests.
- title string? - The title of this API.
- 'version string? - The version of this API.
- version_module boolean? -
googleapis.discovery: RestdescriptionAuth
Authentication information.
Fields
- oauth2 RestdescriptionAuthOauth2? - OAuth 2.0 authentication information.
googleapis.discovery: RestdescriptionAuthOauth2
OAuth 2.0 authentication information.
Fields
- scopes record {}? - Available OAuth 2.0 scopes.
googleapis.discovery: RestdescriptionAuthOauth2Scopes
The scope value.
Fields
- description string? - Description of scope.
googleapis.discovery: RestMethod
Fields
- description string? - Description of this method.
- etagRequired boolean? - Whether this method requires an ETag to be specified. The ETag is sent as an HTTP If-Match or If-None-Match header.
- flatPath string? - The URI path of this REST method in (RFC 6570) format without level 2 features ({+var}). Supplementary to the path property.
- httpMethod string? - HTTP method used by this method.
- id string? - A unique ID for this method. This property can be used to match methods between different versions of Discovery.
- mediaUpload RestmethodMediaupload? - Media upload parameters.
- parameterOrder string[]? - Ordered list of required parameters, serves as a hint to clients on how to structure their method signatures. The array is ordered such that the "most-significant" parameter appears first.
- parameters record {}? - Details for all parameters in this method.
- path string? - The URI path of this REST method. Should be used in conjunction with the basePath property at the api-level.
- request RestmethodRequest? - The schema for the request.
- response RestmethodResponse? - The schema for the response.
- scopes string[]? - OAuth 2.0 scopes applicable to this method.
- supportsMediaDownload boolean? - Whether this method supports media downloads.
- supportsMediaUpload boolean? - Whether this method supports media uploads.
- supportsSubscription boolean? - Whether this method supports subscriptions.
- useMediaDownloadService boolean? - Indicates that downloads from this method should use the download service URL (i.e. "/download"). Only applies if the method supports media download.
googleapis.discovery: RestmethodMediaupload
Media upload parameters.
Fields
- accept string[]? - MIME Media Ranges for acceptable media uploads to this method.
- maxSize string? - Maximum size of a media upload, such as "1MB", "2GB" or "3TB".
- protocols RestmethodMediauploadProtocols? - Supported upload protocols.
googleapis.discovery: RestmethodMediauploadProtocols
Supported upload protocols.
Fields
- resumable RestmethodMediauploadProtocolsResumable? - Supports the Resumable Media Upload protocol.
- simple RestmethodMediauploadProtocolsSimple? - Supports uploading as a single HTTP request.
googleapis.discovery: RestmethodMediauploadProtocolsResumable
Supports the Resumable Media Upload protocol.
Fields
- multipart boolean? - True if this endpoint supports uploading multipart media.
- path string? - The URI path to be used for upload. Should be used in conjunction with the basePath property at the api-level.
googleapis.discovery: RestmethodMediauploadProtocolsSimple
Supports uploading as a single HTTP request.
Fields
- multipart boolean? - True if this endpoint supports upload multipart media.
- path string? - The URI path to be used for upload. Should be used in conjunction with the basePath property at the api-level.
googleapis.discovery: RestmethodRequest
The schema for the request.
Fields
- '\$ref string? - Schema ID for the request schema.
- parameterName string? - parameter name.
googleapis.discovery: RestmethodResponse
The schema for the response.
Fields
- '\$ref string? - Schema ID for the response schema.
googleapis.discovery: RestResource
Fields
- methods record {}? - Methods on this resource.
- resources record {}? - Sub-resources on this resource.
Import
import ballerinax/googleapis.discovery;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Marketing/Ads & Conversion
Cost/Free
Vendor/Google
Contributors
Dependencies