googleapis.cloudnaturallanguage
Module googleapis.cloudnaturallanguage
API
Definitions
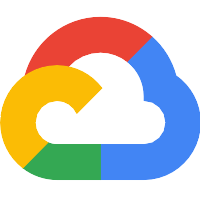
ballerinax/googleapis.cloudnaturallanguage Ballerina library
Overview
This is a generated connector for Google Cloud Natural Language API v1 OpenAPI specification. Provides natural language understanding technologies, such as sentiment analysis, entity recognition, entity sentiment analysis, and other text annotations, to developers.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google Cloud Natural Language connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/googleapis.cloudnaturallanguage
module into the Ballerina project.
import ballerinax/googleapis.cloudnaturallanguage;
Step 2: Create a new connector instance
Create a cloudnaturallanguage:ClientConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
cloudnaturallanguage:ClientConfig clientConfig = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET>, refreshUrl: <REFRESH_URL>, refreshToken: <REFRESH_TOKEN> } }; cloudnaturallanguage:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to classify a document into categories using the connector.
Classifies a document into categories
public function main() { cloudnaturallanguage:Document document = { content: "<content>" }; cloudnaturallanguage:ClassifyTextRequest classifyTextRequest = { document: document }; cloudnaturallanguage:ClassifyTextResponse|error response = baseClient->classifytextDocuments(classifyTextRequest); if (response is cloudnaturallanguage:ClassifyTextResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.cloudnaturallanguage: Client
This is a generated connector for Google Cloud Natural Language API v1 OpenAPI specification. Provides natural language understanding technologies, such as sentiment analysis, entity recognition, entity sentiment analysis, and other text annotations, to developers.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Google account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://language.googleapis.com/" - URL of the target service
analyzeentitiesDocuments
function analyzeentitiesDocuments(AnalyzeEntitiesRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns AnalyzeEntitiesResponse|error
Finds named entities (currently proper names and common nouns) in the text along with entity types, salience, mentions for each entity, and other properties.
Parameters
- payload AnalyzeEntitiesRequest - AnalyzeEntities request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- AnalyzeEntitiesResponse|error - Successful response
analyzeentitysentimentDocuments
function analyzeentitysentimentDocuments(AnalyzeEntitySentimentRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns AnalyzeEntitySentimentResponse|error
Finds entities, similar to AnalyzeEntities in the text and analyzes sentiment associated with each entity and its mentions.
Parameters
- payload AnalyzeEntitySentimentRequest - AnalyzeEntitySentiment request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- AnalyzeEntitySentimentResponse|error - Successful response
analyzesentimentDocuments
function analyzesentimentDocuments(AnalyzeSentimentRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns AnalyzeSentimentResponse|error
Analyzes the sentiment of the provided text.
Parameters
- payload AnalyzeSentimentRequest - AnalyzeSentiment request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- AnalyzeSentimentResponse|error - Successful response
analyzesyntaxDocuments
function analyzesyntaxDocuments(AnalyzeSyntaxRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns AnalyzeSyntaxResponse|error
Analyzes the syntax of the text and provides sentence boundaries and tokenization along with part of speech tags, dependency trees, and other properties.
Parameters
- payload AnalyzeSyntaxRequest - AnalyzeSyntax request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- AnalyzeSyntaxResponse|error - Successful response
annotatetextDocuments
function annotatetextDocuments(AnnotateTextRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns AnnotateTextResponse|error
A convenience method that provides all the features that analyzeSentiment, analyzeEntities, and analyzeSyntax provide in one call.
Parameters
- payload AnnotateTextRequest - AnnotateText request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- AnnotateTextResponse|error - Successful response
classifytextDocuments
function classifytextDocuments(ClassifyTextRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns ClassifyTextResponse|error
Classifies a document into categories.
Parameters
- payload ClassifyTextRequest - ClassifyText request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- ClassifyTextResponse|error - Successful response
Records
googleapis.cloudnaturallanguage: AnalyzeEntitiesRequest
The entity analysis request message.
Fields
- document Document? - ################################################################ # Represents the input to API methods.
- encodingType string? - The encoding type used by the API to calculate offsets.
googleapis.cloudnaturallanguage: AnalyzeEntitiesResponse
The entity analysis response message.
Fields
- entities Entity[]? - The recognized entities in the input document.
- language string? - The language of the text, which will be the same as the language specified in the request or, if not specified, the automatically-detected language. See Document.language field for more details.
googleapis.cloudnaturallanguage: AnalyzeEntitySentimentRequest
The entity-level sentiment analysis request message.
Fields
- document Document? - ################################################################ # Represents the input to API methods.
- encodingType string? - The encoding type used by the API to calculate offsets.
googleapis.cloudnaturallanguage: AnalyzeEntitySentimentResponse
The entity-level sentiment analysis response message.
Fields
- entities Entity[]? - The recognized entities in the input document with associated sentiments.
- language string? - The language of the text, which will be the same as the language specified in the request or, if not specified, the automatically-detected language. See Document.language field for more details.
googleapis.cloudnaturallanguage: AnalyzeSentimentRequest
The sentiment analysis request message.
Fields
- document Document? - ################################################################ # Represents the input to API methods.
- encodingType string? - The encoding type used by the API to calculate sentence offsets.
googleapis.cloudnaturallanguage: AnalyzeSentimentResponse
The sentiment analysis response message.
Fields
- documentSentiment Sentiment? - Represents the feeling associated with the entire text or entities in the text.
- language string? - The language of the text, which will be the same as the language specified in the request or, if not specified, the automatically-detected language. See Document.language field for more details.
- sentences Sentence[]? - The sentiment for all the sentences in the document.
googleapis.cloudnaturallanguage: AnalyzeSyntaxRequest
The syntax analysis request message.
Fields
- document Document? - ################################################################ # Represents the input to API methods.
- encodingType string? - The encoding type used by the API to calculate offsets.
googleapis.cloudnaturallanguage: AnalyzeSyntaxResponse
The syntax analysis response message.
Fields
- language string? - The language of the text, which will be the same as the language specified in the request or, if not specified, the automatically-detected language. See Document.language field for more details.
- sentences Sentence[]? - Sentences in the input document.
- tokens Token[]? - Tokens, along with their syntactic information, in the input document.
googleapis.cloudnaturallanguage: AnnotateTextRequest
The request message for the text annotation API, which can perform multiple analysis types (sentiment, entities, and syntax) in one call.
Fields
- document Document? - ################################################################ # Represents the input to API methods.
- encodingType string? - The encoding type used by the API to calculate offsets.
- features Features? - All available features for sentiment, syntax, and semantic analysis. Setting each one to true will enable that specific analysis for the input.
googleapis.cloudnaturallanguage: AnnotateTextResponse
The text annotations response message.
Fields
- categories ClassificationCategory[]? - Categories identified in the input document.
- documentSentiment Sentiment? - Represents the feeling associated with the entire text or entities in the text.
- entities Entity[]? - Entities, along with their semantic information, in the input document. Populated if the user enables AnnotateTextRequest.Features.extract_entities.
- language string? - The language of the text, which will be the same as the language specified in the request or, if not specified, the automatically-detected language. See Document.language field for more details.
- sentences Sentence[]? - Sentences in the input document. Populated if the user enables AnnotateTextRequest.Features.extract_syntax.
- tokens Token[]? - Tokens, along with their syntactic information, in the input document. Populated if the user enables AnnotateTextRequest.Features.extract_syntax.
googleapis.cloudnaturallanguage: ClassificationCategory
Represents a category returned from the text classifier.
Fields
- confidence float? - The classifier's confidence of the category. Number represents how certain the classifier is that this category represents the given text.
- name string? - The name of the category representing the document, from the predefined taxonomy.
googleapis.cloudnaturallanguage: ClassifyTextRequest
The document classification request message.
Fields
- document Document? - ################################################################ # Represents the input to API methods.
googleapis.cloudnaturallanguage: ClassifyTextResponse
The document classification response message.
Fields
- categories ClassificationCategory[]? - Categories representing the input document.
googleapis.cloudnaturallanguage: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.cloudnaturallanguage: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.cloudnaturallanguage: DependencyEdge
Represents dependency parse tree information for a token. (For more information on dependency labels, see http://www.aclweb.org/anthology/P13-2017
Fields
- headTokenIndex int? - Represents the head of this token in the dependency tree. This is the index of the token which has an arc going to this token. The index is the position of the token in the array of tokens returned by the API method. If this token is a root token, then the
head_token_index
is its own index.
- label string? - The parse label for the token.
googleapis.cloudnaturallanguage: Document
################################################################ # Represents the input to API methods.
Fields
- content string? - The content of the input in string format. Cloud audit logging exempt since it is based on user data.
- gcsContentUri string? - The Google Cloud Storage URI where the file content is located. This URI must be of the form: gs://bucket_name/object_name. For more details, see https://cloud.google.com/storage/docs/reference-uris. NOTE: Cloud Storage object versioning is not supported.
- language string? - The language of the document (if not specified, the language is automatically detected). Both ISO and BCP-47 language codes are accepted. Language Support lists currently supported languages for each API method. If the language (either specified by the caller or automatically detected) is not supported by the called API method, an
INVALID_ARGUMENT
error is returned.
- 'type string? - Required. If the type is not set or is
TYPE_UNSPECIFIED
, returns anINVALID_ARGUMENT
error.
googleapis.cloudnaturallanguage: Entity
Represents a phrase in the text that is a known entity, such as a person, an organization, or location. The API associates information, such as salience and mentions, with entities.
Fields
- mentions EntityMention[]? - The mentions of this entity in the input document. The API currently supports proper noun mentions.
- metadata record {}? - Metadata associated with the entity. For most entity types, the metadata is a Wikipedia URL (
wikipedia_url
) and Knowledge Graph MID (mid
), if they are available. For the metadata associated with other entity types, see the Type table below.
- name string? - The representative name for the entity.
- salience float? - The salience score associated with the entity in the [0, 1.0] range. The salience score for an entity provides information about the importance or centrality of that entity to the entire document text. Scores closer to 0 are less salient, while scores closer to 1.0 are highly salient.
- sentiment Sentiment? - Represents the feeling associated with the entire text or entities in the text.
- 'type string? - The entity type.
googleapis.cloudnaturallanguage: EntityMention
Represents a mention for an entity in the text. Currently, proper noun mentions are supported.
Fields
- sentiment Sentiment? - Represents the feeling associated with the entire text or entities in the text.
- text TextSpan? - Represents an output piece of text.
- 'type string? - The type of the entity mention.
googleapis.cloudnaturallanguage: Features
All available features for sentiment, syntax, and semantic analysis. Setting each one to true will enable that specific analysis for the input.
Fields
- classifyText boolean? - Classify the full document into categories.
- extractDocumentSentiment boolean? - Extract document-level sentiment.
- extractEntities boolean? - Extract entities.
- extractEntitySentiment boolean? - Extract entities and their associated sentiment.
- extractSyntax boolean? - Extract syntax information.
googleapis.cloudnaturallanguage: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.cloudnaturallanguage: PartOfSpeech
Represents part of speech information for a token. Parts of speech are as defined in http://www.lrec-conf.org/proceedings/lrec2012/pdf/274_Paper.pdf
Fields
- aspect string? - The grammatical aspect.
- case string? - The grammatical case.
- form string? - The grammatical form.
- gender string? - The grammatical gender.
- mood string? - The grammatical mood.
- number string? - The grammatical number.
- person string? - The grammatical person.
- proper string? - The grammatical properness.
- reciprocity string? - The grammatical reciprocity.
- tag string? - The part of speech tag.
- tense string? - The grammatical tense.
- voice string? - The grammatical voice.
googleapis.cloudnaturallanguage: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.cloudnaturallanguage: Sentence
Represents a sentence in the input document.
Fields
- sentiment Sentiment? - Represents the feeling associated with the entire text or entities in the text.
- text TextSpan? - Represents an output piece of text.
googleapis.cloudnaturallanguage: Sentiment
Represents the feeling associated with the entire text or entities in the text.
Fields
- magnitude float? - A non-negative number in the [0, +inf) range, which represents the absolute magnitude of sentiment regardless of score (positive or negative).
- score float? - Sentiment score between -1.0 (negative sentiment) and 1.0 (positive sentiment).
googleapis.cloudnaturallanguage: Status
The Status
type defines a logical error model that is suitable for different programming environments, including REST APIs and RPC APIs. It is used by gRPC. Each Status
message contains three pieces of data: error code, error message, and error details. You can find out more about this error model and how to work with it in the API Design Guide.
Fields
- code int? - The status code, which should be an enum value of google.rpc.Code.
- details record {}[]? - A list of messages that carry the error details. There is a common set of message types for APIs to use.
- message string? - A developer-facing error message, which should be in English. Any user-facing error message should be localized and sent in the google.rpc.Status.details field, or localized by the client.
googleapis.cloudnaturallanguage: TextSpan
Represents an output piece of text.
Fields
- beginOffset int? - The API calculates the beginning offset of the content in the original document according to the EncodingType specified in the API request.
- content string? - The content of the output text.
googleapis.cloudnaturallanguage: Token
Represents the smallest syntactic building block of the text.
Fields
- dependencyEdge DependencyEdge? - Represents dependency parse tree information for a token. (For more information on dependency labels, see http://www.aclweb.org/anthology/P13-2017
- partOfSpeech PartOfSpeech? - Represents part of speech information for a token. Parts of speech are as defined in http://www.lrec-conf.org/proceedings/lrec2012/pdf/274_Paper.pdf
- text TextSpan? - Represents an output piece of text.
Import
import ballerinax/googleapis.cloudnaturallanguage;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Business Intelligence/Language Analysis
Cost/Free
Vendor/Google
Contributors
Dependencies