googleapis.clouddatastore
Module googleapis.clouddatastore
API
Definitions
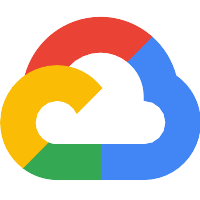
ballerinax/googleapis.clouddatastore Ballerina library
Overview
This is a generated connector for Google Cloud Datastore REST API v1 OpenAPI specification. The Google Cloud Datastore API provides the capability to access the schemaless NoSQL database to provide fully managed, robust, scalable storage for your application.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this guide
Clients
googleapis.clouddatastore: Client
This is a generated connector for Google Cloud Datastore REST API v1 OpenAPI specification. The Google Cloud Datastore API provides the capability to access the schemaless NoSQL database to provide fully managed, robust, scalable storage for your application.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Google account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://datastore.googleapis.com/" - URL of the target service
listIndexes
function listIndexes(string projectId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? filter, int? pageSize, string? pageToken) returns GoogleDatastoreAdminV1ListIndexesResponse|error
Lists the indexes that match the specified filters. Datastore uses an eventually consistent query to fetch the list of indexes and may occasionally return stale results.
Parameters
- projectId string - Project ID against which to make the request.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- filter string? (default ()) - Filter
- pageSize int? (default ()) - The maximum number of items to return. If zero, then all results will be returned.
- pageToken string? (default ()) - The next_page_token value returned from a previous List request, if any.
Return Type
- GoogleDatastoreAdminV1ListIndexesResponse|error - Successful response
createIndex
function createIndex(string projectId, GoogleDatastoreAdminV1Index payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns GoogleLongrunningOperation|error
Creates the specified index. A newly created index's initial state is CREATING
. On completion of the returned google.longrunning.Operation, the state will be READY
. If the index already exists, the call will return an ALREADY_EXISTS
status. During index creation, the process could result in an error, in which case the index will move to the ERROR
state. The process can be recovered by fixing the data that caused the error, removing the index with delete, then re-creating the index with create. Indexes with a single property cannot be created.
Parameters
- projectId string - Project ID against which to make the request.
- payload GoogleDatastoreAdminV1Index - Index to be created
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- GoogleLongrunningOperation|error - Successful response
getIndex
function getIndex(string projectId, string indexId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns GoogleDatastoreAdminV1Index|error
Gets an index.
Parameters
- projectId string - Project ID against which to make the request.
- indexId string - The resource ID of the index to get.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- GoogleDatastoreAdminV1Index|error - Successful response
deleteIndex
function deleteIndex(string projectId, string indexId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns GoogleLongrunningOperation|error
Deletes an existing index. An index can only be deleted if it is in a READY
or ERROR
state. On successful execution of the request, the index will be in a DELETING
state. And on completion of the returned google.longrunning.Operation, the index will be removed. During index deletion, the process could result in an error, in which case the index will move to the ERROR
state. The process can be recovered by fixing the data that caused the error, followed by calling delete again.
Parameters
- projectId string - Project ID against which to make the request.
- indexId string - The resource ID of the index to delete.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- GoogleLongrunningOperation|error - Successful response
allocateIdKeys
function allocateIdKeys(string projectId, AllocateIdsRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns AllocateIdsResponse|error
Allocates IDs for the given keys, which is useful for referencing an entity before it is inserted.
Parameters
- projectId string - Required. The ID of the project against which to make the request.
- payload AllocateIdsRequest - Keys to be allocated
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- AllocateIdsResponse|error - Successful response
beginTransaction
function beginTransaction(string projectId, BeginTransactionRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns BeginTransactionResponse|error
Begins a new transaction.
Parameters
- projectId string - Required. The ID of the project against which to make the request.
- payload BeginTransactionRequest - Transaction to be begun
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- BeginTransactionResponse|error - Successful response
commitTransaction
function commitTransaction(string projectId, CommitRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns CommitResponse|error
Commits a transaction, optionally creating, deleting or modifying some entities.
Parameters
- projectId string - Required. The ID of the project against which to make the request.
- payload CommitRequest - Transaction to be committed
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- CommitResponse|error - Successful response
exportEntities
function exportEntities(string projectId, GoogleDatastoreAdminV1ExportEntitiesRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns GoogleLongrunningOperation|error
Exports a copy of all or a subset of entities from Google Cloud Datastore to another storage system, such as Google Cloud Storage. Recent updates to entities may not be reflected in the export. The export occurs in the background and its progress can be monitored and managed via the Operation resource that is created. The output of an export may only be used once the associated operation is done. If an export operation is cancelled before completion it may leave partial data behind in Google Cloud Storage.
Parameters
- projectId string - Required. Project ID against which to make the request.
- payload GoogleDatastoreAdminV1ExportEntitiesRequest - Entities to be exported
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- GoogleLongrunningOperation|error - Successful response
importEntities
function importEntities(string projectId, GoogleDatastoreAdminV1ImportEntitiesRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns GoogleLongrunningOperation|error
Imports entities into Google Cloud Datastore. Existing entities with the same key are overwritten. The import occurs in the background and its progress can be monitored and managed via the Operation resource that is created. If an ImportEntities operation is cancelled, it is possible that a subset of the data has already been imported to Cloud Datastore.
Parameters
- projectId string - Required. Project ID against which to make the request.
- payload GoogleDatastoreAdminV1ImportEntitiesRequest - Entities to be imported
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- GoogleLongrunningOperation|error - Successful response
lookupEntity
function lookupEntity(string projectId, LookupRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns LookupResponse|error
Looks up entities by key.
Parameters
- projectId string - Required. The ID of the project against which to make the request.
- payload LookupRequest - Entities to be looked up
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- LookupResponse|error - Successful response
reserveIds
function reserveIds(string projectId, ReserveIdsRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Prevents the supplied keys' IDs from being auto-allocated by Cloud Datastore.
Parameters
- projectId string - Required. The ID of the project against which to make the request.
- payload ReserveIdsRequest - IDs to be reserved
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
rollbackTransaction
function rollbackTransaction(string projectId, RollbackRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Rolls back a transaction.
Parameters
- projectId string - Required. The ID of the project against which to make the request.
- payload RollbackRequest - Transaction to be rolled out
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
runQuery
function runQuery(string projectId, RunQueryRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns RunQueryResponse|error
Queries for entities.
Parameters
- projectId string - Required. The ID of the project against which to make the request.
- payload RunQueryRequest - Entities to be queried
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- RunQueryResponse|error - Successful response
getOperation
function getOperation(string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns GoogleLongrunningOperation|error
Gets the latest state of a long-running operation. Clients can use this method to poll the operation result at intervals as recommended by the API service.
Parameters
- name string - The name of the operation resource.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- GoogleLongrunningOperation|error - Successful response
deleteOperation
function deleteOperation(string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes a long-running operation. This method indicates that the client is no longer interested in the operation result. It does not cancel the operation. If the server doesn't support this method, it returns google.rpc.Code.UNIMPLEMENTED
.
Parameters
- name string - The name of the operation resource to be deleted.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listOperations
function listOperations(string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? filter, int? pageSize, string? pageToken) returns GoogleLongrunningListOperationsResponse|error
Lists operations that match the specified filter in the request. If the server doesn't support this method, it returns UNIMPLEMENTED
. NOTE: the name
binding allows API services to override the binding to use different resource name schemes, such as users/*/operations
. To override the binding, API services can add a binding such as "/v1/{name=users/*}/operations"
to their service configuration. For backwards compatibility, the default name includes the operations collection id, however overriding users must ensure the name binding is the parent resource, without the operations collection id.
Parameters
- name string - The name of the operation's parent resource.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- filter string? (default ()) - The standard list filter.
- pageSize int? (default ()) - The standard list page size.
- pageToken string? (default ()) - The standard list page token.
Return Type
- GoogleLongrunningListOperationsResponse|error - Successful response
cancelOperation
function cancelOperation(string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Starts asynchronous cancellation on a long-running operation. The server makes a best effort to cancel the operation, but success is not guaranteed. If the server doesn't support this method, it returns google.rpc.Code.UNIMPLEMENTED
. Clients can use Operations.GetOperation or other methods to check whether the cancellation succeeded or whether the operation completed despite cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to Code.CANCELLED
.
Parameters
- name string - The name of the operation resource to be cancelled.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Records
googleapis.clouddatastore: AllocateIdsRequest
The request for Datastore.AllocateIds.
Fields
- keys Key[]? - Required. A list of keys with incomplete key paths for which to allocate IDs. No key may be reserved/read-only.
googleapis.clouddatastore: AllocateIdsResponse
The response for Datastore.AllocateIds.
Fields
- keys Key[]? - The keys specified in the request (in the same order), each with its key path completed with a newly allocated ID.
googleapis.clouddatastore: ArrayValue
An array value.
Fields
- values Value[]? - Values in the array. The order of values in an array is preserved as long as all values have identical settings for 'exclude_from_indexes'.
googleapis.clouddatastore: BeginTransactionRequest
The request for Datastore.BeginTransaction.
Fields
- transactionOptions TransactionOptions? - Options for beginning a new transaction. Transactions can be created explicitly with calls to Datastore.BeginTransaction or implicitly by setting ReadOptions.new_transaction in read requests.
googleapis.clouddatastore: BeginTransactionResponse
The response for Datastore.BeginTransaction.
Fields
- 'transaction string? - The transaction identifier (always present).
googleapis.clouddatastore: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.clouddatastore: CommitRequest
The request for Datastore.Commit.
Fields
- mode string? - The type of commit to perform. Defaults to
TRANSACTIONAL
.
- mutations Mutation[]? - The mutations to perform. When mode is
TRANSACTIONAL
, mutations affecting a single entity are applied in order. The following sequences of mutations affecting a single entity are not permitted in a singleCommit
request: -insert
followed byinsert
-update
followed byinsert
-upsert
followed byinsert
-delete
followed byupdate
When mode isNON_TRANSACTIONAL
, no two mutations may affect a single entity.
- 'transaction string? - The identifier of the transaction associated with the commit. A transaction identifier is returned by a call to Datastore.BeginTransaction.
googleapis.clouddatastore: CommitResponse
The response for Datastore.Commit.
Fields
- indexUpdates int? - The number of index entries updated during the commit, or zero if none were updated.
- mutationResults MutationResult[]? - The result of performing the mutations. The i-th mutation result corresponds to the i-th mutation in the request.
googleapis.clouddatastore: CompositeFilter
A filter that merges multiple other filters using the given operator.
Fields
- filters Filter[]? - The list of filters to combine. Must contain at least one filter.
- op string? - The operator for combining multiple filters.
googleapis.clouddatastore: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.clouddatastore: Entity
A Datastore data object. An entity is limited to 1 megabyte when stored. That roughly corresponds to a limit of 1 megabyte for the serialized form of this message.
Fields
- 'key Key? - A unique identifier for an entity. If a key's partition ID or any of its path kinds or names are reserved/read-only, the key is reserved/read-only. A reserved/read-only key is forbidden in certain documented contexts.
- properties record {}? - The entity's properties. The map's keys are property names. A property name matching regex
__.*__
is reserved. A reserved property name is forbidden in certain documented contexts. The name must not contain more than 500 characters. The name cannot be""
.
googleapis.clouddatastore: EntityResult
The result of fetching an entity from Datastore.
Fields
- cursor string? - A cursor that points to the position after the result entity. Set only when the
EntityResult
is part of aQueryResultBatch
message.
- entity Entity? - A Datastore data object. An entity is limited to 1 megabyte when stored. That roughly corresponds to a limit of 1 megabyte for the serialized form of this message.
- 'version string? - The version of the entity, a strictly positive number that monotonically increases with changes to the entity. This field is set for
FULL
entity results. For missing entities inLookupResponse
, this is the version of the snapshot that was used to look up the entity, and it is always set except for eventually consistent reads.
googleapis.clouddatastore: Filter
A holder for any type of filter.
Fields
- compositeFilter CompositeFilter? - A filter that merges multiple other filters using the given operator.
- propertyFilter PropertyFilter? - A filter on a specific property.
googleapis.clouddatastore: GoogleDatastoreAdminV1beta1CommonMetadata
Metadata common to all Datastore Admin operations.
Fields
- endTime string? - The time the operation ended, either successfully or otherwise.
- labels record {}? - The client-assigned labels which were provided when the operation was created. May also include additional labels.
- operationType string? - The type of the operation. Can be used as a filter in ListOperationsRequest.
- startTime string? - The time that work began on the operation.
- state string? - The current state of the Operation.
googleapis.clouddatastore: GoogleDatastoreAdminV1beta1EntityFilter
Identifies a subset of entities in a project. This is specified as combinations of kinds and namespaces (either or both of which may be all, as described in the following examples). Example usage: Entire project: kinds=[], namespace_ids=[] Kinds Foo and Bar in all namespaces: kinds=['Foo', 'Bar'], namespace_ids=[] Kinds Foo and Bar only in the default namespace: kinds=['Foo', 'Bar'], namespace_ids=[''] Kinds Foo and Bar in both the default and Baz namespaces: kinds=['Foo', 'Bar'], namespace_ids=['', 'Baz'] The entire Baz namespace: kinds=[], namespace_ids=['Baz']
Fields
- kinds string[]? - If empty, then this represents all kinds.
- namespaceIds string[]? - An empty list represents all namespaces. This is the preferred usage for projects that don't use namespaces. An empty string element represents the default namespace. This should be used if the project has data in non-default namespaces, but doesn't want to include them. Each namespace in this list must be unique.
googleapis.clouddatastore: GoogleDatastoreAdminV1beta1ExportEntitiesMetadata
Metadata for ExportEntities operations.
Fields
- common GoogleDatastoreAdminV1beta1CommonMetadata? - Metadata common to all Datastore Admin operations.
- entityFilter GoogleDatastoreAdminV1beta1EntityFilter? - Identifies a subset of entities in a project. This is specified as combinations of kinds and namespaces (either or both of which may be all, as described in the following examples). Example usage: Entire project: kinds=[], namespace_ids=[] Kinds Foo and Bar in all namespaces: kinds=['Foo', 'Bar'], namespace_ids=[] Kinds Foo and Bar only in the default namespace: kinds=['Foo', 'Bar'], namespace_ids=[''] Kinds Foo and Bar in both the default and Baz namespaces: kinds=['Foo', 'Bar'], namespace_ids=['', 'Baz'] The entire Baz namespace: kinds=[], namespace_ids=['Baz']
- outputUrlPrefix string? - Location for the export metadata and data files. This will be the same value as the google.datastore.admin.v1beta1.ExportEntitiesRequest.output_url_prefix field. The final output location is provided in google.datastore.admin.v1beta1.ExportEntitiesResponse.output_url.
- progressBytes GoogleDatastoreAdminV1beta1Progress? - Measures the progress of a particular metric.
- progressEntities GoogleDatastoreAdminV1beta1Progress? - Measures the progress of a particular metric.
googleapis.clouddatastore: GoogleDatastoreAdminV1beta1ExportEntitiesResponse
The response for google.datastore.admin.v1beta1.DatastoreAdmin.ExportEntities.
Fields
- outputUrl string? - Location of the output metadata file. This can be used to begin an import into Cloud Datastore (this project or another project). See google.datastore.admin.v1beta1.ImportEntitiesRequest.input_url. Only present if the operation completed successfully.
googleapis.clouddatastore: GoogleDatastoreAdminV1beta1ImportEntitiesMetadata
Metadata for ImportEntities operations.
Fields
- common GoogleDatastoreAdminV1beta1CommonMetadata? - Metadata common to all Datastore Admin operations.
- entityFilter GoogleDatastoreAdminV1beta1EntityFilter? - Identifies a subset of entities in a project. This is specified as combinations of kinds and namespaces (either or both of which may be all, as described in the following examples). Example usage: Entire project: kinds=[], namespace_ids=[] Kinds Foo and Bar in all namespaces: kinds=['Foo', 'Bar'], namespace_ids=[] Kinds Foo and Bar only in the default namespace: kinds=['Foo', 'Bar'], namespace_ids=[''] Kinds Foo and Bar in both the default and Baz namespaces: kinds=['Foo', 'Bar'], namespace_ids=['', 'Baz'] The entire Baz namespace: kinds=[], namespace_ids=['Baz']
- inputUrl string? - The location of the import metadata file. This will be the same value as the google.datastore.admin.v1beta1.ExportEntitiesResponse.output_url field.
- progressBytes GoogleDatastoreAdminV1beta1Progress? - Measures the progress of a particular metric.
- progressEntities GoogleDatastoreAdminV1beta1Progress? - Measures the progress of a particular metric.
googleapis.clouddatastore: GoogleDatastoreAdminV1beta1Progress
Measures the progress of a particular metric.
Fields
- workCompleted string? - The amount of work that has been completed. Note that this may be greater than work_estimated.
- workEstimated string? - An estimate of how much work needs to be performed. May be zero if the work estimate is unavailable.
googleapis.clouddatastore: GoogleDatastoreAdminV1CommonMetadata
Metadata common to all Datastore Admin operations.
Fields
- endTime string? - The time the operation ended, either successfully or otherwise.
- labels record {}? - The client-assigned labels which were provided when the operation was created. May also include additional labels.
- operationType string? - The type of the operation. Can be used as a filter in ListOperationsRequest.
- startTime string? - The time that work began on the operation.
- state string? - The current state of the Operation.
googleapis.clouddatastore: GoogleDatastoreAdminV1EntityFilter
Identifies a subset of entities in a project. This is specified as combinations of kinds and namespaces (either or both of which may be all, as described in the following examples). Example usage: Entire project: kinds=[], namespace_ids=[] Kinds Foo and Bar in all namespaces: kinds=['Foo', 'Bar'], namespace_ids=[] Kinds Foo and Bar only in the default namespace: kinds=['Foo', 'Bar'], namespace_ids=[''] Kinds Foo and Bar in both the default and Baz namespaces: kinds=['Foo', 'Bar'], namespace_ids=['', 'Baz'] The entire Baz namespace: kinds=[], namespace_ids=['Baz']
Fields
- kinds string[]? - If empty, then this represents all kinds.
- namespaceIds string[]? - An empty list represents all namespaces. This is the preferred usage for projects that don't use namespaces. An empty string element represents the default namespace. This should be used if the project has data in non-default namespaces, but doesn't want to include them. Each namespace in this list must be unique.
googleapis.clouddatastore: GoogleDatastoreAdminV1ExportEntitiesMetadata
Metadata for ExportEntities operations.
Fields
- common GoogleDatastoreAdminV1CommonMetadata? - Metadata common to all Datastore Admin operations.
- entityFilter GoogleDatastoreAdminV1EntityFilter? - Identifies a subset of entities in a project. This is specified as combinations of kinds and namespaces (either or both of which may be all, as described in the following examples). Example usage: Entire project: kinds=[], namespace_ids=[] Kinds Foo and Bar in all namespaces: kinds=['Foo', 'Bar'], namespace_ids=[] Kinds Foo and Bar only in the default namespace: kinds=['Foo', 'Bar'], namespace_ids=[''] Kinds Foo and Bar in both the default and Baz namespaces: kinds=['Foo', 'Bar'], namespace_ids=['', 'Baz'] The entire Baz namespace: kinds=[], namespace_ids=['Baz']
- outputUrlPrefix string? - Location for the export metadata and data files. This will be the same value as the google.datastore.admin.v1.ExportEntitiesRequest.output_url_prefix field. The final output location is provided in google.datastore.admin.v1.ExportEntitiesResponse.output_url.
- progressBytes GoogleDatastoreAdminV1Progress? - Measures the progress of a particular metric.
- progressEntities GoogleDatastoreAdminV1Progress? - Measures the progress of a particular metric.
googleapis.clouddatastore: GoogleDatastoreAdminV1ExportEntitiesRequest
The request for google.datastore.admin.v1.DatastoreAdmin.ExportEntities.
Fields
- entityFilter GoogleDatastoreAdminV1EntityFilter? - Identifies a subset of entities in a project. This is specified as combinations of kinds and namespaces (either or both of which may be all, as described in the following examples). Example usage: Entire project: kinds=[], namespace_ids=[] Kinds Foo and Bar in all namespaces: kinds=['Foo', 'Bar'], namespace_ids=[] Kinds Foo and Bar only in the default namespace: kinds=['Foo', 'Bar'], namespace_ids=[''] Kinds Foo and Bar in both the default and Baz namespaces: kinds=['Foo', 'Bar'], namespace_ids=['', 'Baz'] The entire Baz namespace: kinds=[], namespace_ids=['Baz']
- labels record {}? - Client-assigned labels.
- outputUrlPrefix string? - Required. Location for the export metadata and data files. The full resource URL of the external storage location. Currently, only Google Cloud Storage is supported. So output_url_prefix should be of the form:
gs://BUCKET_NAME[/NAMESPACE_PATH]
, whereBUCKET_NAME
is the name of the Cloud Storage bucket andNAMESPACE_PATH
is an optional Cloud Storage namespace path (this is not a Cloud Datastore namespace). For more information about Cloud Storage namespace paths, see Object name considerations. The resulting files will be nested deeper than the specified URL prefix. The final output URL will be provided in the google.datastore.admin.v1.ExportEntitiesResponse.output_url field. That value should be used for subsequent ImportEntities operations. By nesting the data files deeper, the same Cloud Storage bucket can be used in multiple ExportEntities operations without conflict.
googleapis.clouddatastore: GoogleDatastoreAdminV1ExportEntitiesResponse
The response for google.datastore.admin.v1.DatastoreAdmin.ExportEntities.
Fields
- outputUrl string? - Location of the output metadata file. This can be used to begin an import into Cloud Datastore (this project or another project). See google.datastore.admin.v1.ImportEntitiesRequest.input_url. Only present if the operation completed successfully.
googleapis.clouddatastore: GoogleDatastoreAdminV1ImportEntitiesMetadata
Metadata for ImportEntities operations.
Fields
- common GoogleDatastoreAdminV1CommonMetadata? - Metadata common to all Datastore Admin operations.
- entityFilter GoogleDatastoreAdminV1EntityFilter? - Identifies a subset of entities in a project. This is specified as combinations of kinds and namespaces (either or both of which may be all, as described in the following examples). Example usage: Entire project: kinds=[], namespace_ids=[] Kinds Foo and Bar in all namespaces: kinds=['Foo', 'Bar'], namespace_ids=[] Kinds Foo and Bar only in the default namespace: kinds=['Foo', 'Bar'], namespace_ids=[''] Kinds Foo and Bar in both the default and Baz namespaces: kinds=['Foo', 'Bar'], namespace_ids=['', 'Baz'] The entire Baz namespace: kinds=[], namespace_ids=['Baz']
- inputUrl string? - The location of the import metadata file. This will be the same value as the google.datastore.admin.v1.ExportEntitiesResponse.output_url field.
- progressBytes GoogleDatastoreAdminV1Progress? - Measures the progress of a particular metric.
- progressEntities GoogleDatastoreAdminV1Progress? - Measures the progress of a particular metric.
googleapis.clouddatastore: GoogleDatastoreAdminV1ImportEntitiesRequest
The request for google.datastore.admin.v1.DatastoreAdmin.ImportEntities.
Fields
- entityFilter GoogleDatastoreAdminV1EntityFilter? - Identifies a subset of entities in a project. This is specified as combinations of kinds and namespaces (either or both of which may be all, as described in the following examples). Example usage: Entire project: kinds=[], namespace_ids=[] Kinds Foo and Bar in all namespaces: kinds=['Foo', 'Bar'], namespace_ids=[] Kinds Foo and Bar only in the default namespace: kinds=['Foo', 'Bar'], namespace_ids=[''] Kinds Foo and Bar in both the default and Baz namespaces: kinds=['Foo', 'Bar'], namespace_ids=['', 'Baz'] The entire Baz namespace: kinds=[], namespace_ids=['Baz']
- inputUrl string? - Required. The full resource URL of the external storage location. Currently, only Google Cloud Storage is supported. So input_url should be of the form:
gs://BUCKET_NAME[/NAMESPACE_PATH]/OVERALL_EXPORT_METADATA_FILE
, whereBUCKET_NAME
is the name of the Cloud Storage bucket,NAMESPACE_PATH
is an optional Cloud Storage namespace path (this is not a Cloud Datastore namespace), andOVERALL_EXPORT_METADATA_FILE
is the metadata file written by the ExportEntities operation. For more information about Cloud Storage namespace paths, see Object name considerations. For more information, see google.datastore.admin.v1.ExportEntitiesResponse.output_url.
- labels record {}? - Client-assigned labels.
googleapis.clouddatastore: GoogleDatastoreAdminV1Index
Datastore composite index definition.
Fields
- ancestor string? - Required. The index's ancestor mode. Must not be ANCESTOR_MODE_UNSPECIFIED.
- indexId string? - Output only. The resource ID of the index.
- kind string? - Required. The entity kind to which this index applies.
- projectId string? - Output only. Project ID.
- properties GoogleDatastoreAdminV1IndexedProperty[]? - Required. An ordered sequence of property names and their index attributes.
- state string? - Output only. The state of the index.
googleapis.clouddatastore: GoogleDatastoreAdminV1IndexedProperty
A property of an index.
Fields
- direction string? - Required. The indexed property's direction. Must not be DIRECTION_UNSPECIFIED.
- name string? - Required. The property name to index.
googleapis.clouddatastore: GoogleDatastoreAdminV1IndexOperationMetadata
Metadata for Index operations.
Fields
- common GoogleDatastoreAdminV1CommonMetadata? - Metadata common to all Datastore Admin operations.
- indexId string? - The index resource ID that this operation is acting on.
- progressEntities GoogleDatastoreAdminV1Progress? - Measures the progress of a particular metric.
googleapis.clouddatastore: GoogleDatastoreAdminV1ListIndexesResponse
The response for google.datastore.admin.v1.DatastoreAdmin.ListIndexes.
Fields
- indexes GoogleDatastoreAdminV1Index[]? - The indexes.
- nextPageToken string? - The standard List next-page token.
googleapis.clouddatastore: GoogleDatastoreAdminV1Progress
Measures the progress of a particular metric.
Fields
- workCompleted string? - The amount of work that has been completed. Note that this may be greater than work_estimated.
- workEstimated string? - An estimate of how much work needs to be performed. May be zero if the work estimate is unavailable.
googleapis.clouddatastore: GoogleLongrunningListOperationsResponse
The response message for Operations.ListOperations.
Fields
- nextPageToken string? - The standard List next-page token.
- operations GoogleLongrunningOperation[]? - A list of operations that matches the specified filter in the request.
googleapis.clouddatastore: GoogleLongrunningOperation
This resource represents a long-running operation that is the result of a network API call.
Fields
- done boolean? - If the value is
false
, it means the operation is still in progress. Iftrue
, the operation is completed, and eithererror
orresponse
is available.
- 'error Status? - The
Status
type defines a logical error model that is suitable for different programming environments, including REST APIs and RPC APIs. It is used by gRPC. EachStatus
message contains three pieces of data: error code, error message, and error details. You can find out more about this error model and how to work with it in the API Design Guide.
- metadata record {}? - Service-specific metadata associated with the operation. It typically contains progress information and common metadata such as create time. Some services might not provide such metadata. Any method that returns a long-running operation should document the metadata type, if any.
- name string? - The server-assigned name, which is only unique within the same service that originally returns it. If you use the default HTTP mapping, the
name
should be a resource name ending withoperations/{unique_id}
.
- response record {}? - The normal response of the operation in case of success. If the original method returns no data on success, such as
Delete
, the response isgoogle.protobuf.Empty
. If the original method is standardGet
/Create
/Update
, the response should be the resource. For other methods, the response should have the type XxxResponse, whereXxx
is the original method name. For example, if the original method name is TakeSnapshot(), the inferred response type isTakeSnapshotResponse
.
googleapis.clouddatastore: GqlQuery
A GQL query.
Fields
- allowLiterals boolean? - When false, the query string must not contain any literals and instead must bind all values. For example,
SELECT * FROM Kind WHERE a = 'string literal'
is not allowed, whileSELECT * FROM Kind WHERE a = @value
is.
- namedBindings record {}? - For each non-reserved named binding site in the query string, there must be a named parameter with that name, but not necessarily the inverse. Key must match regex
A-Za-z_$*
, must not match regex__.*__
, and must not be""
.
- positionalBindings GqlQueryParameter[]? - Numbered binding site @1 references the first numbered parameter, effectively using 1-based indexing, rather than the usual 0. For each binding site numbered i in
query_string
, there must be an i-th numbered parameter. The inverse must also be true.
googleapis.clouddatastore: GqlQueryParameter
A binding parameter for a GQL query.
Fields
- cursor string? - A query cursor. Query cursors are returned in query result batches.
- value Value? - A message that can hold any of the supported value types and associated metadata.
googleapis.clouddatastore: Key
A unique identifier for an entity. If a key's partition ID or any of its path kinds or names are reserved/read-only, the key is reserved/read-only. A reserved/read-only key is forbidden in certain documented contexts.
Fields
- partitionId PartitionId? - A partition ID identifies a grouping of entities. The grouping is always by project and namespace, however the namespace ID may be empty. A partition ID contains several dimensions: project ID and namespace ID. Partition dimensions: - May be
""
. - Must be valid UTF-8 bytes. - Must have values that match regex[A-Za-z\d\.\-_]{1,100}
If the value of any dimension matches regex__.*__
, the partition is reserved/read-only. A reserved/read-only partition ID is forbidden in certain documented contexts. Foreign partition IDs (in which the project ID does not match the context project ID ) are discouraged. Reads and writes of foreign partition IDs may fail if the project is not in an active state.
- path PathElement[]? - The entity path. An entity path consists of one or more elements composed of a kind and a string or numerical identifier, which identify entities. The first element identifies a root entity, the second element identifies a child of the root entity, the third element identifies a child of the second entity, and so forth. The entities identified by all prefixes of the path are called the element's ancestors. An entity path is always fully complete: all of the entity's ancestors are required to be in the path along with the entity identifier itself. The only exception is that in some documented cases, the identifier in the last path element (for the entity) itself may be omitted. For example, the last path element of the key of
Mutation.insert
may have no identifier. A path can never be empty, and a path can have at most 100 elements.
googleapis.clouddatastore: KindExpression
A representation of a kind.
Fields
- name string? - The name of the kind.
googleapis.clouddatastore: LatLng
An object that represents a latitude/longitude pair. This is expressed as a pair of doubles to represent degrees latitude and degrees longitude. Unless specified otherwise, this object must conform to the WGS84 standard. Values must be within normalized ranges.
Fields
- latitude decimal? - The latitude in degrees. It must be in the range [-90.0, +90.0].
- longitude decimal? - The longitude in degrees. It must be in the range [-180.0, +180.0].
googleapis.clouddatastore: LookupRequest
The request for Datastore.Lookup.
Fields
- keys Key[]? - Required. Keys of entities to look up.
- readOptions ReadOptions? - The options shared by read requests.
googleapis.clouddatastore: LookupResponse
The response for Datastore.Lookup.
Fields
- deferred Key[]? - A list of keys that were not looked up due to resource constraints. The order of results in this field is undefined and has no relation to the order of the keys in the input.
- found EntityResult[]? - Entities found as
ResultType.FULL
entities. The order of results in this field is undefined and has no relation to the order of the keys in the input.
- missing EntityResult[]? - Entities not found as
ResultType.KEY_ONLY
entities. The order of results in this field is undefined and has no relation to the order of the keys in the input.
googleapis.clouddatastore: Mutation
A mutation to apply to an entity.
Fields
- baseVersion string? - The version of the entity that this mutation is being applied to. If this does not match the current version on the server, the mutation conflicts.
- delete Key? - A unique identifier for an entity. If a key's partition ID or any of its path kinds or names are reserved/read-only, the key is reserved/read-only. A reserved/read-only key is forbidden in certain documented contexts.
- insert Entity? - A Datastore data object. An entity is limited to 1 megabyte when stored. That roughly corresponds to a limit of 1 megabyte for the serialized form of this message.
- update Entity? - A Datastore data object. An entity is limited to 1 megabyte when stored. That roughly corresponds to a limit of 1 megabyte for the serialized form of this message.
- upsert Entity? - A Datastore data object. An entity is limited to 1 megabyte when stored. That roughly corresponds to a limit of 1 megabyte for the serialized form of this message.
googleapis.clouddatastore: MutationResult
The result of applying a mutation.
Fields
- conflictDetected boolean? - Whether a conflict was detected for this mutation. Always false when a conflict detection strategy field is not set in the mutation.
- 'key Key? - A unique identifier for an entity. If a key's partition ID or any of its path kinds or names are reserved/read-only, the key is reserved/read-only. A reserved/read-only key is forbidden in certain documented contexts.
- 'version string? - The version of the entity on the server after processing the mutation. If the mutation doesn't change anything on the server, then the version will be the version of the current entity or, if no entity is present, a version that is strictly greater than the version of any previous entity and less than the version of any possible future entity.
googleapis.clouddatastore: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.clouddatastore: PartitionId
A partition ID identifies a grouping of entities. The grouping is always by project and namespace, however the namespace ID may be empty. A partition ID contains several dimensions: project ID and namespace ID. Partition dimensions: - May be ""
. - Must be valid UTF-8 bytes. - Must have values that match regex [A-Za-z\d\.\-_]{1,100}
If the value of any dimension matches regex __.*__
, the partition is reserved/read-only. A reserved/read-only partition ID is forbidden in certain documented contexts. Foreign partition IDs (in which the project ID does not match the context project ID ) are discouraged. Reads and writes of foreign partition IDs may fail if the project is not in an active state.
Fields
- namespaceId string? - If not empty, the ID of the namespace to which the entities belong.
- projectId string? - The ID of the project to which the entities belong.
googleapis.clouddatastore: PathElement
A (kind, ID/name) pair used to construct a key path. If either name or ID is set, the element is complete. If neither is set, the element is incomplete.
Fields
- id string? - The auto-allocated ID of the entity. Never equal to zero. Values less than zero are discouraged and may not be supported in the future.
- kind string? - The kind of the entity. A kind matching regex
__.*__
is reserved/read-only. A kind must not contain more than 1500 bytes when UTF-8 encoded. Cannot be""
.
- name string? - The name of the entity. A name matching regex
__.*__
is reserved/read-only. A name must not be more than 1500 bytes when UTF-8 encoded. Cannot be""
.
googleapis.clouddatastore: Projection
A representation of a property in a projection.
Fields
- property PropertyReference? - A reference to a property relative to the kind expressions.
googleapis.clouddatastore: PropertyFilter
A filter on a specific property.
Fields
- op string? - The operator to filter by.
- property PropertyReference? - A reference to a property relative to the kind expressions.
- value Value? - A message that can hold any of the supported value types and associated metadata.
googleapis.clouddatastore: PropertyOrder
The desired order for a specific property.
Fields
- direction string? - The direction to order by. Defaults to
ASCENDING
.
- property PropertyReference? - A reference to a property relative to the kind expressions.
googleapis.clouddatastore: PropertyReference
A reference to a property relative to the kind expressions.
Fields
- name string? - The name of the property. If name includes "."s, it may be interpreted as a property name path.
googleapis.clouddatastore: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.clouddatastore: Query
A query for entities.
Fields
- distinctOn PropertyReference[]? - The properties to make distinct. The query results will contain the first result for each distinct combination of values for the given properties (if empty, all results are returned).
- endCursor string? - An ending point for the query results. Query cursors are returned in query result batches and can only be used to limit the same query.
- filter Filter? - A holder for any type of filter.
- kind KindExpression[]? - The kinds to query (if empty, returns entities of all kinds). Currently at most 1 kind may be specified.
- 'limit int? - The maximum number of results to return. Applies after all other constraints. Optional. Unspecified is interpreted as no limit. Must be >= 0 if specified.
- offset int? - The number of results to skip. Applies before limit, but after all other constraints. Optional. Must be >= 0 if specified.
- 'order PropertyOrder[]? - The order to apply to the query results (if empty, order is unspecified).
- projection Projection[]? - The projection to return. Defaults to returning all properties.
- startCursor string? - A starting point for the query results. Query cursors are returned in query result batches and can only be used to continue the same query.
googleapis.clouddatastore: QueryResultBatch
A batch of results produced by a query.
Fields
- endCursor string? - A cursor that points to the position after the last result in the batch.
- entityResultType string? - The result type for every entity in
entity_results
.
- entityResults EntityResult[]? - The results for this batch.
- moreResults string? - The state of the query after the current batch.
- skippedCursor string? - A cursor that points to the position after the last skipped result. Will be set when
skipped_results
!= 0.
- skippedResults int? - The number of results skipped, typically because of an offset.
- snapshotVersion string? - The version number of the snapshot this batch was returned from. This applies to the range of results from the query's
start_cursor
(or the beginning of the query if no cursor was given) to this batch'send_cursor
(not the query'send_cursor
). In a single transaction, subsequent query result batches for the same query can have a greater snapshot version number. Each batch's snapshot version is valid for all preceding batches. The value will be zero for eventually consistent queries.
googleapis.clouddatastore: ReadOnly
Options specific to read-only transactions.
googleapis.clouddatastore: ReadOptions
The options shared by read requests.
Fields
- readConsistency string? - The non-transactional read consistency to use. Cannot be set to
STRONG
for global queries.
- 'transaction string? - The identifier of the transaction in which to read. A transaction identifier is returned by a call to Datastore.BeginTransaction.
googleapis.clouddatastore: ReadWrite
Options specific to read / write transactions.
Fields
- previousTransaction string? - The transaction identifier of the transaction being retried.
googleapis.clouddatastore: ReserveIdsRequest
The request for Datastore.ReserveIds.
Fields
- databaseId string? - If not empty, the ID of the database against which to make the request.
- keys Key[]? - Required. A list of keys with complete key paths whose numeric IDs should not be auto-allocated.
googleapis.clouddatastore: RollbackRequest
The request for Datastore.Rollback.
Fields
- 'transaction string? - Required. The transaction identifier, returned by a call to Datastore.BeginTransaction.
googleapis.clouddatastore: RunQueryRequest
The request for Datastore.RunQuery.
Fields
- partitionId PartitionId? - A partition ID identifies a grouping of entities. The grouping is always by project and namespace, however the namespace ID may be empty. A partition ID contains several dimensions: project ID and namespace ID. Partition dimensions: - May be
""
. - Must be valid UTF-8 bytes. - Must have values that match regex[A-Za-z\d\.\-_]{1,100}
If the value of any dimension matches regex__.*__
, the partition is reserved/read-only. A reserved/read-only partition ID is forbidden in certain documented contexts. Foreign partition IDs (in which the project ID does not match the context project ID ) are discouraged. Reads and writes of foreign partition IDs may fail if the project is not in an active state.
- query Query? - A query for entities.
- readOptions ReadOptions? - The options shared by read requests.
googleapis.clouddatastore: RunQueryResponse
The response for Datastore.RunQuery.
Fields
- batch QueryResultBatch? - A batch of results produced by a query.
- query Query? - A query for entities.
googleapis.clouddatastore: Status
The Status
type defines a logical error model that is suitable for different programming environments, including REST APIs and RPC APIs. It is used by gRPC. Each Status
message contains three pieces of data: error code, error message, and error details. You can find out more about this error model and how to work with it in the API Design Guide.
Fields
- code int? - The status code, which should be an enum value of google.rpc.Code.
- details record {}[]? - A list of messages that carry the error details. There is a common set of message types for APIs to use.
- message string? - A developer-facing error message, which should be in English. Any user-facing error message should be localized and sent in the google.rpc.Status.details field, or localized by the client.
googleapis.clouddatastore: TransactionOptions
Options for beginning a new transaction. Transactions can be created explicitly with calls to Datastore.BeginTransaction or implicitly by setting ReadOptions.new_transaction in read requests.
Fields
- readOnly ReadOnly? - Options specific to read-only transactions.
- readWrite ReadWrite? - Options specific to read / write transactions.
googleapis.clouddatastore: Value
A message that can hold any of the supported value types and associated metadata.
Fields
- arrayValue ArrayValue? - An array value.
- blobValue string? - A blob value. May have at most 1,000,000 bytes. When
exclude_from_indexes
is false, may have at most 1500 bytes. In JSON requests, must be base64-encoded.
- booleanValue boolean? - A boolean value.
- doubleValue decimal? - A double value.
- entityValue Entity? - A Datastore data object. An entity is limited to 1 megabyte when stored. That roughly corresponds to a limit of 1 megabyte for the serialized form of this message.
- excludeFromIndexes boolean? - If the value should be excluded from all indexes including those defined explicitly.
- geoPointValue LatLng? - An object that represents a latitude/longitude pair. This is expressed as a pair of doubles to represent degrees latitude and degrees longitude. Unless specified otherwise, this object must conform to the WGS84 standard. Values must be within normalized ranges.
- integerValue string? - An integer value.
- keyValue Key? - A unique identifier for an entity. If a key's partition ID or any of its path kinds or names are reserved/read-only, the key is reserved/read-only. A reserved/read-only key is forbidden in certain documented contexts.
- meaning int? - The
meaning
field should only be populated for backwards compatibility.
- nullValue string? - A null value.
- stringValue string? - A UTF-8 encoded string value. When
exclude_from_indexes
is false (it is indexed) , may have at most 1500 bytes. Otherwise, may be set to at most 1,000,000 bytes.
- timestampValue string? - A timestamp value. When stored in the Datastore, precise only to microseconds; any additional precision is rounded down.
Import
import ballerinax/googleapis.clouddatastore;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 0
Weekly downloads
Keywords
IT Operations/Databases
Cost/Freemium
Vendor/Google
Contributors
Dependencies