googleapis.cloudbuild
Module googleapis.cloudbuild
API
Definitions
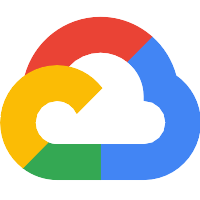
ballerinax/googleapis.cloudbuild Ballerina library
Overview
This is a generated connector for Google Cloud Build REST API v1 OpenAPI specification. The Google Cloud Build API provides the capability to manage builds on Google Cloud Platform.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this guide
Clients
googleapis.cloudbuild: Client
This is a generated connector for Google Cloud Build REST API v1 OpenAPI specification. The Google Cloud Build API provides the capability to manage builds on Google Cloud Platform.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Google account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://cloudbuild.googleapis.com/" - URL of the target service
listBuilds
function listBuilds(string projectId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? filter, int? pageSize, string? pageToken, string? parent) returns ListBuildsResponse|error
Lists previously requested builds. Previously requested builds may still be in-progress, or may have finished successfully or unsuccessfully.
Parameters
- projectId string - Required. ID of the project.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- filter string? (default ()) - The raw filter text to constrain the results.
- pageSize int? (default ()) - Number of results to return in the list.
- pageToken string? (default ()) - The page token for the next page of Builds. If unspecified, the first page of results is returned. If the token is rejected for any reason, INVALID_ARGUMENT will be thrown. In this case, the token should be discarded, and pagination should be restarted from the first page of results. See https://google.aip.dev/158 for more.
- parent string? (default ()) - The parent of the collection of
Builds
. Format:projects/{project}/locations/location
Return Type
- ListBuildsResponse|error - Successful response
createBuild
function createBuild(string projectId, Build payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? parent) returns Operation|error
Starts a build with the specified configuration. This method returns a long-running Operation
, which includes the build ID. Pass the build ID to GetBuild
to determine the build status (such as SUCCESS
or FAILURE
).
Parameters
- projectId string - Required. ID of the project.
- payload Build - Build to be created
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- parent string? (default ()) - The parent resource where this build will be created. Format:
projects/{project}/locations/{location}
getBuild
function getBuild(string projectId, string id, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? name) returns Build|error
Returns information about a previously requested build. The Build
that is returned includes its status (such as SUCCESS
, FAILURE
, or WORKING
), and timing information.
Parameters
- projectId string - Required. ID of the project.
- id string - Required. ID of the build.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- name string? (default ()) - The name of the
Build
to retrieve. Format:projects/{project}/locations/{location}/builds/{build}
cancelBuild
function cancelBuild(string projectId, string id, CancelBuildRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Build|error
Cancels a build in progress.
Parameters
- projectId string - Required. ID of the project.
- id string - Required. ID of the build.
- payload CancelBuildRequest - Build to be cancelled
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
retryBuild
function retryBuild(string projectId, string id, RetryBuildRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Operation|error
Creates a new build based on the specified build. This method creates a new build using the original build request, which may or may not result in an identical build. For triggered builds: * Triggered builds resolve to a precise revision; therefore a retry of a triggered build will result in a build that uses the same revision. For non-triggered builds that specify RepoSource
: * If the original build built from the tip of a branch, the retried build will build from the tip of that branch, which may not be the same revision as the original build. * If the original build specified a commit sha or revision ID, the retried build will use the identical source. For builds that specify StorageSource
: * If the original build pulled source from Google Cloud Storage without specifying the generation of the object, the new build will use the current object, which may be different from the original build source. * If the original build pulled source from Cloud Storage and specified the generation of the object, the new build will attempt to use the same object, which may or may not be available depending on the bucket's lifecycle management settings.
Parameters
- projectId string - Required. ID of the project.
- id string - Required. Build ID of the original build.
- payload RetryBuildRequest - Build to be retried
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listTriggers
function listTriggers(string projectId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken, string? parent) returns ListBuildTriggersResponse|error
Lists existing BuildTrigger
s. This API is experimental.
Parameters
- projectId string - Required. ID of the project for which to list BuildTriggers.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - Number of results to return in the list.
- pageToken string? (default ()) - Token to provide to skip to a particular spot in the list.
- parent string? (default ()) - The parent of the collection of
Triggers
. Format:projects/{project}/locations/{location}
Return Type
- ListBuildTriggersResponse|error - Successful response
createTrigger
function createTrigger(string projectId, BuildTrigger payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? parent) returns BuildTrigger|error
Creates a new BuildTrigger
. This API is experimental.
Parameters
- projectId string - Required. ID of the project for which to configure automatic builds.
- payload BuildTrigger - Trigger to be created
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- parent string? (default ()) - The parent resource where this trigger will be created. Format:
projects/{project}/locations/{location}
Return Type
- BuildTrigger|error - Successful response
getTrigger
function getTrigger(string projectId, string triggerId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? name) returns BuildTrigger|error
Returns information about a BuildTrigger
. This API is experimental.
Parameters
- projectId string - Required. ID of the project that owns the trigger.
- triggerId string - Required. Identifier (
id
orname
) of theBuildTrigger
to get.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- name string? (default ()) - The name of the
Trigger
to retrieve. Format:projects/{project}/locations/{location}/triggers/{trigger}
Return Type
- BuildTrigger|error - Successful response
deleteTrigger
function deleteTrigger(string projectId, string triggerId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? name) returns Response|error
Deletes a BuildTrigger
by its project ID and trigger ID. This API is experimental.
Parameters
- projectId string - Required. ID of the project that owns the trigger.
- triggerId string - Required. ID of the
BuildTrigger
to delete.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- name string? (default ()) - The name of the
Trigger
to delete. Format:projects/{project}/locations/{location}/triggers/{trigger}
updateTrigger
function updateTrigger(string projectId, string triggerId, BuildTrigger payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns BuildTrigger|error
Updates a BuildTrigger
by its project ID and trigger ID. This API is experimental.
Parameters
- projectId string - Required. ID of the project that owns the trigger.
- triggerId string - Required. ID of the
BuildTrigger
to update.
- payload BuildTrigger - Trigger to be updated
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- BuildTrigger|error - Successful response
runTrigger
function runTrigger(string projectId, string triggerId, RepoSource payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? name) returns Operation|error
Runs a BuildTrigger
at a particular source revision.
Parameters
- projectId string - Required. ID of the project.
- triggerId string - Required. ID of the trigger.
- payload RepoSource - Trigger to be ran
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- name string? (default ()) - The name of the
Trigger
to run. Format:projects/{project}/locations/{location}/triggers/{trigger}
receiveWebhookTrigger
function receiveWebhookTrigger(string projectId, string trigger, HttpBody payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? name, string? secret) returns Response|error
ReceiveTriggerWebhook [Experimental] is called when the API receives a webhook request targeted at a specific trigger.
Parameters
- projectId string - Project in which the specified trigger lives
- trigger string - Name of the trigger to run the payload against
- payload HttpBody - Webhook to be created
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- name string? (default ()) - The name of the
ReceiveTriggerWebhook
to retrieve. Format:projects/{project}/locations/{location}/triggers/{trigger}
- secret string? (default ()) - Secret token used for authorization if an OAuth token isn't provided.
receiveWebhook
function receiveWebhook(HttpBody payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? webhookKey) returns Response|error
ReceiveWebhook is called when the API receives a GitHub webhook.
Parameters
- payload HttpBody - Webhook to be received
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- webhookKey string? (default ()) - For GitHub Enterprise webhooks, this key is used to associate the webhook request with the GitHubEnterpriseConfig to use for validation.
getWorkerPool
function getWorkerPool(string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? projectId, string? triggerId) returns WorkerPool|error
Returns details of a WorkerPool
.
Parameters
- name string - Required. The name of the
WorkerPool
to retrieve. Format:projects/{project}/locations/{location}/workerPools/{workerPool}
.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- projectId string? (default ()) - Required. ID of the project that owns the trigger.
- triggerId string? (default ()) - Required. Identifier (
id
orname
) of theBuildTrigger
to get.
Return Type
- WorkerPool|error - Successful response
deleteWorkerPool
function deleteWorkerPool(string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? allowMissing, string? etag, boolean? validateOnly) returns Operation|error
Deletes a WorkerPool
.
Parameters
- name string - Required. The name of the
WorkerPool
to delete. Format:projects/{project}/locations/{workerPool}/workerPools/{workerPool}
.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- allowMissing boolean? (default ()) - If set to true, and the
WorkerPool
is not found, the request will succeed but no action will be taken on the server.
- etag string? (default ()) - Optional. If this is provided, it must match the server's etag on the workerpool for the request to be processed.
- validateOnly boolean? (default ()) - If set, validate the request and preview the response, but do not actually post it.
updateWorkerPool
function updateWorkerPool(string name, WorkerPool payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? updateMask, boolean? validateOnly) returns Operation|error
Updates a WorkerPool
.
Parameters
- name string - Output only. The resource name of the
WorkerPool
, with formatprojects/{project}/locations/{location}/workerPools/{worker_pool}
. The value of{worker_pool}
is provided byworker_pool_id
inCreateWorkerPool
request and the value of{location}
is determined by the endpoint accessed.
- payload WorkerPool - WorkerPool to be updated
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- updateMask string? (default ()) - A mask specifying which fields in
worker_pool
to update.
- validateOnly boolean? (default ()) - If set, validate the request and preview the response, but do not actually post it.
approveBuild
function approveBuild(string name, ApproveBuildRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Operation|error
Approves or rejects a pending build. If approved, the returned LRO will be analogous to the LRO returned from a CreateBuild call. If rejected, the returned LRO will be immediately done.
Parameters
- name string - Required. Name of the target build. For example: "projects/{$project_id}/builds/{$build_id}"
- payload ApproveBuildRequest - WorkerPool to be approved
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
cancelOperation
function cancelOperation(string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Starts asynchronous cancellation on a long-running operation. The server makes a best effort to cancel the operation, but success is not guaranteed. If the server doesn't support this method, it returns google.rpc.Code.UNIMPLEMENTED
. Clients can use Operations.GetOperation or other methods to check whether the cancellation succeeded or whether the operation completed despite cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to Code.CANCELLED
.
Parameters
- name string - The name of the operation resource to be cancelled.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
retryBuildByName
function retryBuildByName(string name, RetryBuildRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Operation|error
Creates a new build based on the specified build. This method creates a new build using the original build request, which may or may not result in an identical build. For triggered builds: * Triggered builds resolve to a precise revision; therefore a retry of a triggered build will result in a build that uses the same revision. For non-triggered builds that specify RepoSource
: * If the original build built from the tip of a branch, the retried build will build from the tip of that branch, which may not be the same revision as the original build. * If the original build specified a commit sha or revision ID, the retried build will use the identical source. For builds that specify StorageSource
: * If the original build pulled source from Google Cloud Storage without specifying the generation of the object, the new build will use the current object, which may be different from the original build source. * If the original build pulled source from Cloud Storage and specified the generation of the object, the new build will attempt to use the same object, which may or may not be available depending on the bucket's lifecycle management settings.
Parameters
- name string - The name of the
Build
to retry. Format:projects/{project}/locations/{location}/builds/{build}
- payload RetryBuildRequest - Build to be retried
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
runTriggerByName
function runTriggerByName(string name, RunBuildTriggerRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Operation|error
Runs a BuildTrigger
at a particular source revision.
Parameters
- name string - The name of the
Trigger
to run. Format:projects/{project}/locations/{location}/triggers/{trigger}
- payload RunBuildTriggerRequest - Trigger to be run
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
receiveWebhookTriggerByName
function receiveWebhookTriggerByName(string name, HttpBody payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? projectId, string? secret, string? trigger) returns Response|error
ReceiveTriggerWebhook [Experimental] is called when the API receives a webhook request targeted at a specific trigger.
Parameters
- name string - The name of the
ReceiveTriggerWebhook
to retrieve. Format:projects/{project}/locations/{location}/triggers/{trigger}
- payload HttpBody - Webhook trigger to be received
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- projectId string? (default ()) - Project in which the specified trigger lives
- secret string? (default ()) - Secret token used for authorization if an OAuth token isn't provided.
- trigger string? (default ()) - Name of the trigger to run the payload against
listBuildsByPath
function listBuildsByPath(string parent, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? filter, int? pageSize, string? pageToken, string? projectId) returns ListBuildsResponse|error
Lists previously requested builds. Previously requested builds may still be in-progress, or may have finished successfully or unsuccessfully.
Parameters
- parent string - The parent of the collection of
Builds
. Format:projects/{project}/locations/location
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- filter string? (default ()) - The raw filter text to constrain the results.
- pageSize int? (default ()) - Number of results to return in the list.
- pageToken string? (default ()) - The page token for the next page of Builds. If unspecified, the first page of results is returned. If the token is rejected for any reason, INVALID_ARGUMENT will be thrown. In this case, the token should be discarded, and pagination should be restarted from the first page of results. See https://google.aip.dev/158 for more.
- projectId string? (default ()) - Required. ID of the project.
Return Type
- ListBuildsResponse|error - Successful response
createBuildByPath
function createBuildByPath(string parent, Build payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? projectId) returns Operation|error
Starts a build with the specified configuration. This method returns a long-running Operation
, which includes the build ID. Pass the build ID to GetBuild
to determine the build status (such as SUCCESS
or FAILURE
).
Parameters
- parent string - The parent resource where this build will be created. Format:
projects/{project}/locations/{location}
- payload Build - Build to be created
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- projectId string? (default ()) - Required. ID of the project.
listGithubEnterpriseConfigs
function listGithubEnterpriseConfigs(string parent, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? projectId) returns ListGithubEnterpriseConfigsResponse|error
List all GitHubEnterpriseConfigs for a given project. This API is experimental.
Parameters
- parent string - Name of the parent project. For example: projects/{$project_number} or projects/{$project_id}
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- projectId string? (default ()) - ID of the project
Return Type
- ListGithubEnterpriseConfigsResponse|error - Successful response
createGithubEnterpriseConfig
function createGithubEnterpriseConfig(string parent, GitHubEnterpriseConfig payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? projectId) returns Operation|error
Create an association between a GCP project and a GitHub Enterprise server. This API is experimental.
Parameters
- parent string - Name of the parent project. For example: projects/{$project_number} or projects/{$project_id}
- payload GitHubEnterpriseConfig - Github Enterprise Config to be created
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- projectId string? (default ()) - ID of the project.
listTriggersByPath
function listTriggersByPath(string parent, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken, string? projectId) returns ListBuildTriggersResponse|error
Lists existing BuildTrigger
s. This API is experimental.
Parameters
- parent string - The parent of the collection of
Triggers
. Format:projects/{project}/locations/{location}
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - Number of results to return in the list.
- pageToken string? (default ()) - Token to provide to skip to a particular spot in the list.
- projectId string? (default ()) - Required. ID of the project for which to list BuildTriggers.
Return Type
- ListBuildTriggersResponse|error - Successful response
createTriggerByPath
function createTriggerByPath(string parent, BuildTrigger payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? projectId) returns BuildTrigger|error
Creates a new BuildTrigger
. This API is experimental.
Parameters
- parent string - The parent resource where this trigger will be created. Format:
projects/{project}/locations/{location}
- payload BuildTrigger - Trigger to be created
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- projectId string? (default ()) - Required. ID of the project for which to configure automatic builds.
Return Type
- BuildTrigger|error - Successful response
listWorkerPools
function listWorkerPools(string parent, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken) returns ListWorkerPoolsResponse|error
Lists WorkerPool
s.
Parameters
- parent string - Required. The parent of the collection of
WorkerPools
. Format:projects/{project}/locations/{location}
.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - The maximum number of
WorkerPool
s to return. The service may return fewer than this value. If omitted, the server will use a sensible default.
- pageToken string? (default ()) - A page token, received from a previous
ListWorkerPools
call. Provide this to retrieve the subsequent page.
Return Type
- ListWorkerPoolsResponse|error - Successful response
createWorkerPool
function createWorkerPool(string parent, WorkerPool payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? validateOnly, string? workerPoolId) returns Operation|error
Creates a WorkerPool
.
Parameters
- parent string - Required. The parent resource where this worker pool will be created. Format:
projects/{project}/locations/{location}
.
- payload WorkerPool - WorkerPool to be created
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- validateOnly boolean? (default ()) - If set, validate the request and preview the response, but do not actually post it.
- workerPoolId string? (default ()) - Required. Immutable. The ID to use for the
WorkerPool
, which will become the final component of the resource name. This value should be 1-63 characters, and valid characters are /a-z-/.
updateTriggerByName
function updateTriggerByName(string resourceName, BuildTrigger payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? projectId, string? triggerId) returns BuildTrigger|error
Updates a BuildTrigger
by its project ID and trigger ID. This API is experimental.
Parameters
- resourceName string - The
Trigger
name with format:projects/{project}/locations/{location}/triggers/{trigger}
, where {trigger} is a unique identifier generated by the service.
- payload BuildTrigger - Trigger to be updated
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- projectId string? (default ()) - Required. ID of the project that owns the trigger.
- triggerId string? (default ()) - Required. ID of the
BuildTrigger
to update.
Return Type
- BuildTrigger|error - Successful response
Records
googleapis.cloudbuild: ApprovalConfig
ApprovalConfig describes configuration for manual approval of a build.
Fields
- approvalRequired boolean? - Whether or not approval is needed. If this is set on a build, it will become pending when created, and will need to be explicitly approved to start.
googleapis.cloudbuild: ApprovalResult
ApprovalResult describes the decision and associated metadata of a manual approval of a build.
Fields
- approvalTime string? - Output only. The time when the approval decision was made.
- approverAccount string? - Output only. Email of the user that called the ApproveBuild API to approve or reject a build at the time that the API was called (the user's actual email that is tied to their GAIA ID may have changed). This field is not stored, rather, it is calculated on the fly using approver_id.
- comment string? - Optional. An optional comment for this manual approval result.
- decision string? - Required. The decision of this manual approval.
- url string? - Optional. An optional URL tied to this manual approval result. This field is essentially the same as comment, except that it will be rendered by the UI differently. An example use case is a link to an external job that approved this Build.
googleapis.cloudbuild: ApproveBuildRequest
Request to approve or reject a pending build.
Fields
- approvalResult ApprovalResult? - ApprovalResult describes the decision and associated metadata of a manual approval of a build.
googleapis.cloudbuild: ArtifactObjects
Files in the workspace to upload to Cloud Storage upon successful completion of all build steps.
Fields
- location string? - Cloud Storage bucket and optional object path, in the form "gs://bucket/path/to/somewhere/". (see Bucket Name Requirements). Files in the workspace matching any path pattern will be uploaded to Cloud Storage with this location as a prefix.
- paths string[]? - Path globs used to match files in the build's workspace.
- timing TimeSpan? - Start and end times for a build execution phase.
googleapis.cloudbuild: ArtifactResult
An artifact that was uploaded during a build. This is a single record in the artifact manifest JSON file.
Fields
- fileHash FileHashes[]? - The file hash of the artifact.
- location string? - The path of an artifact in a Google Cloud Storage bucket, with the generation number. For example,
gs://mybucket/path/to/output.jar#generation
.
googleapis.cloudbuild: Artifacts
Artifacts produced by a build that should be uploaded upon successful completion of all build steps.
Fields
- images string[]? - A list of images to be pushed upon the successful completion of all build steps. The images will be pushed using the builder service account's credentials. The digests of the pushed images will be stored in the Build resource's results field. If any of the images fail to be pushed, the build is marked FAILURE.
- objects ArtifactObjects? - Files in the workspace to upload to Cloud Storage upon successful completion of all build steps.
googleapis.cloudbuild: Build
A build resource in the Cloud Build API. At a high level, a Build
describes where to find source code, how to build it (for example, the builder image to run on the source), and where to store the built artifacts. Fields can include the following variables, which will be expanded when the build is created: - $PROJECT_ID: the project ID of the build. - $PROJECT_NUMBER: the project number of the build. - $BUILD_ID: the autogenerated ID of the build. - $REPO_NAME: the source repository name specified by RepoSource. - $BRANCH_NAME: the branch name specified by RepoSource. - $TAG_NAME: the tag name specified by RepoSource. - $REVISION_ID or $COMMIT_SHA: the commit SHA specified by RepoSource or resolved from the specified branch or tag. - $SHORT_SHA: first 7 characters of $REVISION_ID or $COMMIT_SHA.
Fields
- approval BuildApproval? - BuildApproval describes a build's approval configuration, state, and result.
- artifacts Artifacts? - Artifacts produced by a build that should be uploaded upon successful completion of all build steps.
- availableSecrets Secrets? - Secrets and secret environment variables.
- buildTriggerId string? - Output only. The ID of the
BuildTrigger
that triggered this build, if it was triggered automatically.
- createTime string? - Output only. Time at which the request to create the build was received.
- failureInfo FailureInfo? - A fatal problem encountered during the execution of the build.
- finishTime string? - Output only. Time at which execution of the build was finished. The difference between finish_time and start_time is the duration of the build's execution.
- id string? - Output only. Unique identifier of the build.
- images string[]? - A list of images to be pushed upon the successful completion of all build steps. The images are pushed using the builder service account's credentials. The digests of the pushed images will be stored in the
Build
resource's results field. If any of the images fail to be pushed, the build status is markedFAILURE
.
- logUrl string? - Output only. URL to logs for this build in Google Cloud Console.
- logsBucket string? - Google Cloud Storage bucket where logs should be written (see Bucket Name Requirements). Logs file names will be of the format
${logs_bucket}/log-${build_id}.txt
.
- name string? - Output only. The 'Build' name with format:
projects/{project}/locations/{location}/builds/{build}
, where {build} is a unique identifier generated by the service.
- options BuildOptions? - Optional arguments to enable specific features of builds.
- projectId string? - Output only. ID of the project.
- queueTtl string? - TTL in queue for this build. If provided and the build is enqueued longer than this value, the build will expire and the build status will be
EXPIRED
. The TTL starts ticking from create_time.
- results Results? - Artifacts created by the build pipeline.
- secrets Secret[]? - Secrets to decrypt using Cloud Key Management Service. Note: Secret Manager is the recommended technique for managing sensitive data with Cloud Build. Use
available_secrets
to configure builds to access secrets from Secret Manager. For instructions, see: https://cloud.google.com/cloud-build/docs/securing-builds/use-secrets
- serviceAccount string? - IAM service account whose credentials will be used at build runtime. Must be of the format
projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}
. ACCOUNT can be email address or uniqueId of the service account.
- 'source Source? - Location of the source in a supported storage service.
- sourceProvenance SourceProvenance? - Provenance of the source. Ways to find the original source, or verify that some source was used for this build.
- startTime string? - Output only. Time at which execution of the build was started.
- status string? - Output only. Status of the build.
- statusDetail string? - Output only. Customer-readable message about the current status.
- steps BuildStep[]? - Required. The operations to be performed on the workspace.
- substitutions record {}? - Substitutions data for
Build
resource.
- tags string[]? - Tags for annotation of a
Build
. These are not docker tags.
- timeout string? - Amount of time that this build should be allowed to run, to second granularity. If this amount of time elapses, work on the build will cease and the build status will be
TIMEOUT
.timeout
starts ticking fromstartTime
. Default time is ten minutes.
- timing record {}? - Output only. Stores timing information for phases of the build. Valid keys are: * BUILD: time to execute all build steps. * PUSH: time to push all specified images. * FETCHSOURCE: time to fetch source. * SETUPBUILD: time to set up build. If the build does not specify source or images, these keys will not be included.
- warnings Warning[]? - Output only. Non-fatal problems encountered during the execution of the build.
googleapis.cloudbuild: BuildApproval
BuildApproval describes a build's approval configuration, state, and result.
Fields
- config ApprovalConfig? - ApprovalConfig describes configuration for manual approval of a build.
- result ApprovalResult? - ApprovalResult describes the decision and associated metadata of a manual approval of a build.
- state string? - Output only. The state of this build's approval.
googleapis.cloudbuild: BuildOperationMetadata
Metadata for build operations.
Fields
- build Build? - A build resource in the Cloud Build API. At a high level, a
Build
describes where to find source code, how to build it (for example, the builder image to run on the source), and where to store the built artifacts. Fields can include the following variables, which will be expanded when the build is created: - $PROJECT_ID: the project ID of the build. - $PROJECT_NUMBER: the project number of the build. - $BUILD_ID: the autogenerated ID of the build. - $REPO_NAME: the source repository name specified by RepoSource. - $BRANCH_NAME: the branch name specified by RepoSource. - $TAG_NAME: the tag name specified by RepoSource. - $REVISION_ID or $COMMIT_SHA: the commit SHA specified by RepoSource or resolved from the specified branch or tag. - $SHORT_SHA: first 7 characters of $REVISION_ID or $COMMIT_SHA.
googleapis.cloudbuild: BuildOptions
Optional arguments to enable specific features of builds.
Fields
- diskSizeGb string? - Requested disk size for the VM that runs the build. Note that this is NOT "disk free"; some of the space will be used by the operating system and build utilities. Also note that this is the minimum disk size that will be allocated for the build -- the build may run with a larger disk than requested. At present, the maximum disk size is 1000GB; builds that request more than the maximum are rejected with an error.
- dynamicSubstitutions boolean? - Option to specify whether or not to apply bash style string operations to the substitutions. NOTE: this is always enabled for triggered builds and cannot be overridden in the build configuration file.
- env string[]? - A list of global environment variable definitions that will exist for all build steps in this build. If a variable is defined in both globally and in a build step, the variable will use the build step value. The elements are of the form "KEY=VALUE" for the environment variable "KEY" being given the value "VALUE".
- logStreamingOption string? - Option to define build log streaming behavior to Google Cloud Storage.
- logging string? - Option to specify the logging mode, which determines if and where build logs are stored.
- machineType string? - Compute Engine machine type on which to run the build.
- pool PoolOption? - Details about how a build should be executed on a
WorkerPool
. See running builds in a private pool for more information.
- requestedVerifyOption string? - Requested verifiability options.
- secretEnv string[]? - A list of global environment variables, which are encrypted using a Cloud Key Management Service crypto key. These values must be specified in the build's
Secret
. These variables will be available to all build steps in this build.
- sourceProvenanceHash string[]? - Requested hash for SourceProvenance.
- substitutionOption string? - Option to specify behavior when there is an error in the substitution checks. NOTE: this is always set to ALLOW_LOOSE for triggered builds and cannot be overridden in the build configuration file.
- volumes Volume[]? - Global list of volumes to mount for ALL build steps Each volume is created as an empty volume prior to starting the build process. Upon completion of the build, volumes and their contents are discarded. Global volume names and paths cannot conflict with the volumes defined a build step. Using a global volume in a build with only one step is not valid as it is indicative of a build request with an incorrect configuration.
- workerPool string? - This field deprecated; please use
pool.name
instead.
googleapis.cloudbuild: BuildStep
A step in the build pipeline.
Fields
- args string[]? - A list of arguments that will be presented to the step when it is started. If the image used to run the step's container has an entrypoint, the
args
are used as arguments to that entrypoint. If the image does not define an entrypoint, the first element in args is used as the entrypoint, and the remainder will be used as arguments.
- dir string? - Working directory to use when running this step's container. If this value is a relative path, it is relative to the build's working directory. If this value is absolute, it may be outside the build's working directory, in which case the contents of the path may not be persisted across build step executions, unless a
volume
for that path is specified. If the build specifies aRepoSource
withdir
and a step with adir
, which specifies an absolute path, theRepoSource
dir
is ignored for the step's execution.
- entrypoint string? - Entrypoint to be used instead of the build step image's default entrypoint. If unset, the image's default entrypoint is used.
- env string[]? - A list of environment variable definitions to be used when running a step. The elements are of the form "KEY=VALUE" for the environment variable "KEY" being given the value "VALUE".
- id string? - Unique identifier for this build step, used in
wait_for
to reference this build step as a dependency.
- name string? - Required. The name of the container image that will run this particular build step. If the image is available in the host's Docker daemon's cache, it will be run directly. If not, the host will attempt to pull the image first, using the builder service account's credentials if necessary. The Docker daemon's cache will already have the latest versions of all of the officially supported build steps (https://github.com/GoogleCloudPlatform/cloud-builders). The Docker daemon will also have cached many of the layers for some popular images, like "ubuntu", "debian", but they will be refreshed at the time you attempt to use them. If you built an image in a previous build step, it will be stored in the host's Docker daemon's cache and is available to use as the name for a later build step.
- pullTiming TimeSpan? - Start and end times for a build execution phase.
- script string? - A shell script to be executed in the step. When script is provided, the user cannot specify the entrypoint or args.
- secretEnv string[]? - A list of environment variables which are encrypted using a Cloud Key Management Service crypto key. These values must be specified in the build's
Secret
.
- status string? - Output only. Status of the build step. At this time, build step status is only updated on build completion; step status is not updated in real-time as the build progresses.
- timeout string? - Time limit for executing this build step. If not defined, the step has no time limit and will be allowed to continue to run until either it completes or the build itself times out.
- timing TimeSpan? - Start and end times for a build execution phase.
- volumes Volume[]? - List of volumes to mount into the build step. Each volume is created as an empty volume prior to execution of the build step. Upon completion of the build, volumes and their contents are discarded. Using a named volume in only one step is not valid as it is indicative of a build request with an incorrect configuration.
- waitFor string[]? - The ID(s) of the step(s) that this build step depends on. This build step will not start until all the build steps in
wait_for
have completed successfully. Ifwait_for
is empty, this build step will start when all previous build steps in theBuild.Steps
list have completed successfully.
googleapis.cloudbuild: BuildTrigger
Configuration for an automated build in response to source repository changes.
Fields
- approvalConfig ApprovalConfig? - ApprovalConfig describes configuration for manual approval of a build.
- autodetect boolean? - Autodetect build configuration. The following precedence is used (case insensitive): 1. cloudbuild.yaml 2. cloudbuild.yml 3. cloudbuild.json 4. Dockerfile Currently only available for GitHub App Triggers.
- build Build? - A build resource in the Cloud Build API. At a high level, a
Build
describes where to find source code, how to build it (for example, the builder image to run on the source), and where to store the built artifacts. Fields can include the following variables, which will be expanded when the build is created: - $PROJECT_ID: the project ID of the build. - $PROJECT_NUMBER: the project number of the build. - $BUILD_ID: the autogenerated ID of the build. - $REPO_NAME: the source repository name specified by RepoSource. - $BRANCH_NAME: the branch name specified by RepoSource. - $TAG_NAME: the tag name specified by RepoSource. - $REVISION_ID or $COMMIT_SHA: the commit SHA specified by RepoSource or resolved from the specified branch or tag. - $SHORT_SHA: first 7 characters of $REVISION_ID or $COMMIT_SHA.
- createTime string? - Output only. Time when the trigger was created.
- description string? - Human-readable description of this trigger.
- disabled boolean? - If true, the trigger will never automatically execute a build.
- filename string? - Path, from the source root, to the build configuration file (i.e. cloudbuild.yaml).
- filter string? - A Common Expression Language string.
- gitFileSource GitFileSource? - GitFileSource describes a file within a (possibly remote) code repository.
- github GitHubEventsConfig? - GitHubEventsConfig describes the configuration of a trigger that creates a build whenever a GitHub event is received.
- id string? - Output only. Unique identifier of the trigger.
- ignoredFiles string[]? - ignored_files and included_files are file glob matches using https://golang.org/pkg/path/filepath/#Match extended with support for "**". If ignored_files and changed files are both empty, then they are not used to determine whether or not to trigger a build. If ignored_files is not empty, then we ignore any files that match any of the ignored_file globs. If the change has no files that are outside of the ignored_files globs, then we do not trigger a build.
- includedFiles string[]? - If any of the files altered in the commit pass the ignored_files filter and included_files is empty, then as far as this filter is concerned, we should trigger the build. If any of the files altered in the commit pass the ignored_files filter and included_files is not empty, then we make sure that at least one of those files matches a included_files glob. If not, then we do not trigger a build.
- name string? - User-assigned name of the trigger. Must be unique within the project. Trigger names must meet the following requirements: + They must contain only alphanumeric characters and dashes. + They can be 1-64 characters long. + They must begin and end with an alphanumeric character.
- pubsubConfig PubsubConfig? - PubsubConfig describes the configuration of a trigger that creates a build whenever a Pub/Sub message is published.
- resourceName string? - The
Trigger
name with format:projects/{project}/locations/{location}/triggers/{trigger}
, where {trigger} is a unique identifier generated by the service.
- serviceAccount string? - The service account used for all user-controlled operations including UpdateBuildTrigger, RunBuildTrigger, CreateBuild, and CancelBuild. If no service account is set, then the standard Cloud Build service account ([PROJECT_NUM]@system.gserviceaccount.com) will be used instead. Format:
projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT_ID_OR_EMAIL}
- sourceToBuild GitRepoSource? - GitRepoSource describes a repo and ref of a code repository.
- substitutions record {}? - Substitutions for Build resource. The keys must match the following regular expression:
^_[A-Z0-9_]+$
.
- tags string[]? - Tags for annotation of a
BuildTrigger
- triggerTemplate RepoSource? - Location of the source in a Google Cloud Source Repository.
- webhookConfig WebhookConfig? - WebhookConfig describes the configuration of a trigger that creates a build whenever a webhook is sent to a trigger's webhook URL.
googleapis.cloudbuild: BuiltImage
An image built by the pipeline.
Fields
- digest string? - Docker Registry 2.0 digest.
- name string? - Name used to push the container image to Google Container Registry, as presented to
docker push
.
- pushTiming TimeSpan? - Start and end times for a build execution phase.
googleapis.cloudbuild: CancelBuildRequest
Request to cancel an ongoing build.
Fields
- id string? - Required. ID of the build.
- name string? - The name of the
Build
to cancel. Format:projects/{project}/locations/{location}/builds/{build}
- projectId string? - Required. ID of the project.
googleapis.cloudbuild: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.cloudbuild: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.cloudbuild: CreateGitHubEnterpriseConfigOperationMetadata
Metadata for CreateGithubEnterpriseConfig
operation.
Fields
- completeTime string? - Time the operation was completed.
- createTime string? - Time the operation was created.
- githubEnterpriseConfig string? - The resource name of the GitHubEnterprise to be created. Format:
projects/{project}/locations/{location}/githubEnterpriseConfigs/{id}
.
googleapis.cloudbuild: CreateWorkerPoolOperationMetadata
Metadata for the CreateWorkerPool
operation.
Fields
- completeTime string? - Time the operation was completed.
- createTime string? - Time the operation was created.
- workerPool string? - The resource name of the
WorkerPool
to create. Format:projects/{project}/locations/{location}/workerPools/{worker_pool}
.
googleapis.cloudbuild: DeleteGitHubEnterpriseConfigOperationMetadata
Metadata for DeleteGitHubEnterpriseConfig
operation.
Fields
- completeTime string? - Time the operation was completed.
- createTime string? - Time the operation was created.
- githubEnterpriseConfig string? - The resource name of the GitHubEnterprise to be deleted. Format:
projects/{project}/locations/{location}/githubEnterpriseConfigs/{id}
.
googleapis.cloudbuild: DeleteWorkerPoolOperationMetadata
Metadata for the DeleteWorkerPool
operation.
Fields
- completeTime string? - Time the operation was completed.
- createTime string? - Time the operation was created.
- workerPool string? - The resource name of the
WorkerPool
being deleted. Format:projects/{project}/locations/{location}/workerPools/{worker_pool}
.
googleapis.cloudbuild: FailureInfo
A fatal problem encountered during the execution of the build.
Fields
- detail string? - Explains the failure issue in more detail using hard-coded text.
- 'type string? - The name of the failure.
googleapis.cloudbuild: FileHashes
Container message for hashes of byte content of files, used in SourceProvenance messages to verify integrity of source input to the build.
Fields
- fileHash Hash[]? - Collection of file hashes.
googleapis.cloudbuild: GitFileSource
GitFileSource describes a file within a (possibly remote) code repository.
Fields
- path string? - The path of the file, with the repo root as the root of the path.
- repoType string? - See RepoType above.
- revision string? - The branch, tag, arbitrary ref, or SHA version of the repo to use when resolving the filename (optional). This field respects the same syntax/resolution as described here: https://git-scm.com/docs/gitrevisions If unspecified, the revision from which the trigger invocation originated is assumed to be the revision from which to read the specified path.
- uri string? - The URI of the repo (optional). If unspecified, the repo from which the trigger invocation originated is assumed to be the repo from which to read the specified path.
googleapis.cloudbuild: GitHubEnterpriseConfig
GitHubEnterpriseConfig represents a configuration for a GitHub Enterprise server.
Fields
- appId string? - Required. The GitHub app id of the Cloud Build app on the GitHub Enterprise server.
- createTime string? - Output only. Time when the installation was associated with the project.
- displayName string? - Name to display for this config.
- hostUrl string? - The URL of the github enterprise host the configuration is for.
- name string? - Optional. The full resource name for the GitHubEnterpriseConfig For example: "projects/{$project_id}/githubEnterpriseConfig/{$config_id}"
- peeredNetwork string? - Optional. The network to be used when reaching out to the GitHub Enterprise server. The VPC network must be enabled for private service connection. This should be set if the GitHub Enterprise server is hosted on-premises and not reachable by public internet. If this field is left empty, no network peering will occur and calls to the GitHub Enterprise server will be made over the public internet. Must be in the format
projects/{project}/global/networks/{network}
, where {project} is a project number or id and {network} is the name of a VPC network in the project.
- secrets GitHubEnterpriseSecrets? - GitHubEnterpriseSecrets represents the names of all necessary secrets in Secret Manager for a GitHub Enterprise server. Format is: projects//secrets/.
- sslCa string? - Optional. SSL certificate to use for requests to GitHub Enterprise.
- webhookKey string? - The key that should be attached to webhook calls to the ReceiveWebhook endpoint.
googleapis.cloudbuild: GitHubEnterpriseSecrets
GitHubEnterpriseSecrets represents the names of all necessary secrets in Secret Manager for a GitHub Enterprise server. Format is: projects//secrets/.
Fields
- oauthClientIdName string? - The resource name for the OAuth client ID secret in Secret Manager.
- oauthClientIdVersionName string? - The resource name for the OAuth client ID secret version in Secret Manager.
- oauthSecretName string? - The resource name for the OAuth secret in Secret Manager.
- oauthSecretVersionName string? - The resource name for the OAuth secret secret version in Secret Manager.
- privateKeyName string? - The resource name for the private key secret.
- privateKeyVersionName string? - The resource name for the private key secret version.
- webhookSecretName string? - The resource name for the webhook secret in Secret Manager.
- webhookSecretVersionName string? - The resource name for the webhook secret secret version in Secret Manager.
googleapis.cloudbuild: GitHubEventsConfig
GitHubEventsConfig describes the configuration of a trigger that creates a build whenever a GitHub event is received.
Fields
- enterpriseConfigResourceName string? - Optional. The resource name of the github enterprise config that should be applied to this installation. For example: "projects/{$project_id}/githubEnterpriseConfig/{$config_id}"
- installationId string? - The installationID that emits the GitHub event.
- name string? - Name of the repository. For example: The name for https://github.com/googlecloudplatform/cloud-builders is "cloud-builders".
- owner string? - Owner of the repository. For example: The owner for https://github.com/googlecloudplatform/cloud-builders is "googlecloudplatform".
- pullRequest PullRequestFilter? - PullRequestFilter contains filter properties for matching GitHub Pull Requests.
- push PushFilter? - Push contains filter properties for matching GitHub git pushes.
googleapis.cloudbuild: GitRepoSource
GitRepoSource describes a repo and ref of a code repository.
Fields
- ref string? - The branch or tag to use. Must start with "refs/" (required).
- repoType string? - See RepoType below.
- uri string? - The URI of the repo (required).
googleapis.cloudbuild: GoogleDevtoolsCloudbuildV2OperationMetadata
Represents the metadata of the long-running operation.
Fields
- apiVersion string? - Output only. API version used to start the operation.
- createTime string? - Output only. The time the operation was created.
- endTime string? - Output only. The time the operation finished running.
- requestedCancellation boolean? - Output only. Identifies whether the user has requested cancellation of the operation. Operations that have successfully been cancelled have Operation.error value with a google.rpc.Status.code of 1, corresponding to
Code.CANCELLED
.
- statusMessage string? - Output only. Human-readable status of the operation, if any.
- target string? - Output only. Server-defined resource path for the target of the operation.
- verb string? - Output only. Name of the verb executed by the operation.
googleapis.cloudbuild: Hash
Container message for hash values.
Fields
- 'type string? - The type of hash that was performed.
- value string? - The hash value.
googleapis.cloudbuild: HttpBody
Message that represents an arbitrary HTTP body. It should only be used for payload formats that can't be represented as JSON, such as raw binary or an HTML page. This message can be used both in streaming and non-streaming API methods in the request as well as the response. It can be used as a top-level request field, which is convenient if one wants to extract parameters from either the URL or HTTP template into the request fields and also want access to the raw HTTP body. Example: message GetResourceRequest { // A unique request id. string request_id = 1; // The raw HTTP body is bound to this field. google.api.HttpBody http_body = 2; } service ResourceService { rpc GetResource(GetResourceRequest) returns (google.api.HttpBody); rpc UpdateResource(google.api.HttpBody) returns (google.protobuf.Empty); } Example with streaming methods: service CaldavService { rpc GetCalendar(stream google.api.HttpBody) returns (stream google.api.HttpBody); rpc UpdateCalendar(stream google.api.HttpBody) returns (stream google.api.HttpBody); } Use of this type only changes how the request and response bodies are handled, all other features will continue to work unchanged.
Fields
- contentType string? - The HTTP Content-Type header value specifying the content type of the body.
- data string? - The HTTP request/response body as raw binary.
- extensions record {}[]? - Application specific response metadata. Must be set in the first response for streaming APIs.
googleapis.cloudbuild: HTTPDelivery
HTTPDelivery is the delivery configuration for an HTTP notification.
Fields
- uri string? - The URI to which JSON-containing HTTP POST requests should be sent.
googleapis.cloudbuild: InlineSecret
Pairs a set of secret environment variables mapped to encrypted values with the Cloud KMS key to use to decrypt the value.
Fields
- envMap record {}? - Map of environment variable name to its encrypted value. Secret environment variables must be unique across all of a build's secrets, and must be used by at least one build step. Values can be at most 64 KB in size. There can be at most 100 secret values across all of a build's secrets.
- kmsKeyName string? - Resource name of Cloud KMS crypto key to decrypt the encrypted value. In format: projects//locations//keyRings//cryptoKeys/
googleapis.cloudbuild: ListBuildsResponse
Response including listed builds.
Fields
- builds Build[]? - Builds will be sorted by
create_time
, descending.
- nextPageToken string? - Token to receive the next page of results. This will be absent if the end of the response list has been reached.
googleapis.cloudbuild: ListBuildTriggersResponse
Response containing existing BuildTriggers
.
Fields
- nextPageToken string? - Token to receive the next page of results.
- triggers BuildTrigger[]? -
BuildTriggers
for the project, sorted bycreate_time
descending.
googleapis.cloudbuild: ListGithubEnterpriseConfigsResponse
RPC response object returned by ListGithubEnterpriseConfigs RPC method.
Fields
- configs GitHubEnterpriseConfig[]? - A list of GitHubEnterpriseConfigs
googleapis.cloudbuild: ListWorkerPoolsResponse
Response containing existing WorkerPools
.
Fields
- nextPageToken string? - Continuation token used to page through large result sets. Provide this value in a subsequent ListWorkerPoolsRequest to return the next page of results.
- workerPools WorkerPool[]? -
WorkerPools
for the specified project.
googleapis.cloudbuild: NetworkConfig
Defines the network configuration for the pool.
Fields
- egressOption string? - Option to configure network egress for the workers.
- peeredNetwork string? - Required. Immutable. The network definition that the workers are peered to. If this section is left empty, the workers will be peered to
WorkerPool.project_id
on the service producer network. Must be in the formatprojects/{project}/global/networks/{network}
, where{project}
is a project number, such as12345
, and{network}
is the name of a VPC network in the project. See Understanding network configuration options
googleapis.cloudbuild: Notification
Notification is the container which holds the data that is relevant to this particular notification.
Fields
- filter string? - The filter string to use for notification filtering. Currently, this is assumed to be a CEL program. See https://opensource.google/projects/cel for more.
- httpDelivery HTTPDelivery? - HTTPDelivery is the delivery configuration for an HTTP notification.
- slackDelivery SlackDelivery? - SlackDelivery is the delivery configuration for delivering Slack messages via webhooks. See Slack webhook documentation at: https://api.slack.com/messaging/webhooks.
- smtpDelivery SMTPDelivery? - SMTPDelivery is the delivery configuration for an SMTP (email) notification.
- structDelivery record {}? - Escape hatch for users to supply custom delivery configs.
googleapis.cloudbuild: NotifierConfig
NotifierConfig is the top-level configuration message.
Fields
- apiVersion string? - The API version of this configuration format.
- kind string? - The type of notifier to use (e.g. SMTPNotifier).
- metadata NotifierMetadata? - NotifierMetadata contains the data which can be used to reference or describe this notifier.
- spec NotifierSpec? - NotifierSpec is the configuration container for notifications.
googleapis.cloudbuild: NotifierMetadata
NotifierMetadata contains the data which can be used to reference or describe this notifier.
Fields
- name string? - The human-readable and user-given name for the notifier. For example: "repo-merge-email-notifier".
- notifier string? - The string representing the name and version of notifier to deploy. Expected to be of the form of "/:". For example: "gcr.io/my-project/notifiers/smtp:1.2.34".
googleapis.cloudbuild: NotifierSecret
NotifierSecret is the container that maps a secret name (reference) to its Google Cloud Secret Manager resource path.
Fields
- name string? - Name is the local name of the secret, such as the verbatim string "my-smtp-password".
- value string? - Value is interpreted to be a resource path for fetching the actual (versioned) secret data for this secret. For example, this would be a Google Cloud Secret Manager secret version resource path like: "projects/my-project/secrets/my-secret/versions/latest".
googleapis.cloudbuild: NotifierSecretRef
NotifierSecretRef contains the reference to a secret stored in the corresponding NotifierSpec.
Fields
- secretRef string? - The value of
secret_ref
should be aname
that is registered in aSecret
in thesecrets
list of theSpec
.
googleapis.cloudbuild: NotifierSpec
NotifierSpec is the configuration container for notifications.
Fields
- notification Notification? - Notification is the container which holds the data that is relevant to this particular notification.
- secrets NotifierSecret[]? - Configurations for secret resources used by this particular notifier.
googleapis.cloudbuild: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.cloudbuild: Operation
This resource represents a long-running operation that is the result of a network API call.
Fields
- done boolean? - If the value is
false
, it means the operation is still in progress. Iftrue
, the operation is completed, and eithererror
orresponse
is available.
- 'error Status? - The
Status
type defines a logical error model that is suitable for different programming environments, including REST APIs and RPC APIs. It is used by gRPC. EachStatus
message contains three pieces of data: error code, error message, and error details. You can find out more about this error model and how to work with it in the API Design Guide.
- metadata record {}? - Service-specific metadata associated with the operation. It typically contains progress information and common metadata such as create time. Some services might not provide such metadata. Any method that returns a long-running operation should document the metadata type, if any.
- name string? - The server-assigned name, which is only unique within the same service that originally returns it. If you use the default HTTP mapping, the
name
should be a resource name ending withoperations/{unique_id}
.
- response record {}? - The normal response of the operation in case of success. If the original method returns no data on success, such as
Delete
, the response isgoogle.protobuf.Empty
. If the original method is standardGet
/Create
/Update
, the response should be the resource. For other methods, the response should have the type XxxResponse, whereXxx
is the original method name. For example, if the original method name is TakeSnapshot(), the inferred response type isTakeSnapshotResponse
.
googleapis.cloudbuild: OperationMetadata
Represents the metadata of the long-running operation.
Fields
- apiVersion string? - Output only. API version used to start the operation.
- cancelRequested boolean? - Output only. Identifies whether the user has requested cancellation of the operation. Operations that have been cancelled successfully have Operation.error value with a google.rpc.Status.code of 1, corresponding to
Code.CANCELLED
.
- createTime string? - Output only. The time the operation was created.
- endTime string? - Output only. The time the operation finished running.
- statusDetail string? - Output only. Human-readable status of the operation, if any.
- target string? - Output only. Server-defined resource path for the target of the operation.
- verb string? - Output only. Name of the verb executed by the operation.
googleapis.cloudbuild: PoolOption
Details about how a build should be executed on a WorkerPool
. See running builds in a private pool for more information.
Fields
- name string? - The
WorkerPool
resource to execute the build on. You must havecloudbuild.workerpools.use
on the project hosting the WorkerPool. Format projects/{project}/locations/{location}/workerPools/{workerPoolId}
googleapis.cloudbuild: PrivatePoolV1Config
Configuration for a V1 PrivatePool
.
Fields
- networkConfig NetworkConfig? - Defines the network configuration for the pool.
- workerConfig WorkerConfig? - Defines the configuration to be used for creating workers in the pool.
googleapis.cloudbuild: ProcessAppManifestCallbackOperationMetadata
Metadata for ProcessAppManifestCallback
operation.
Fields
- completeTime string? - Time the operation was completed.
- createTime string? - Time the operation was created.
- githubEnterpriseConfig string? - The resource name of the GitHubEnterprise to be created. Format:
projects/{project}/locations/{location}/githubEnterpriseConfigs/{id}
.
googleapis.cloudbuild: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.cloudbuild: PubsubConfig
PubsubConfig describes the configuration of a trigger that creates a build whenever a Pub/Sub message is published.
Fields
- serviceAccountEmail string? - Service account that will make the push request.
- state string? - Potential issues with the underlying Pub/Sub subscription configuration. Only populated on get requests.
- subscription string? - Output only. Name of the subscription. Format is
projects/{project}/subscriptions/{subscription}
.
- topic string? - The name of the topic from which this subscription is receiving messages. Format is
projects/{project}/topics/{topic}
.
googleapis.cloudbuild: PullRequestFilter
PullRequestFilter contains filter properties for matching GitHub Pull Requests.
Fields
- branch string? - Regex of branches to match. The syntax of the regular expressions accepted is the syntax accepted by RE2 and described at https://github.com/google/re2/wiki/Syntax
- commentControl string? - Configure builds to run whether a repository owner or collaborator need to comment
/gcbrun
.
- invertRegex boolean? - If true, branches that do NOT match the git_ref will trigger a build.
googleapis.cloudbuild: PushFilter
Push contains filter properties for matching GitHub git pushes.
Fields
- branch string? - Regexes matching branches to build. The syntax of the regular expressions accepted is the syntax accepted by RE2 and described at https://github.com/google/re2/wiki/Syntax
- invertRegex boolean? - When true, only trigger a build if the revision regex does NOT match the git_ref regex.
- tag string? - Regexes matching tags to build. The syntax of the regular expressions accepted is the syntax accepted by RE2 and described at https://github.com/google/re2/wiki/Syntax
googleapis.cloudbuild: RepoSource
Location of the source in a Google Cloud Source Repository.
Fields
- branchName string? - Regex matching branches to build. The syntax of the regular expressions accepted is the syntax accepted by RE2 and described at https://github.com/google/re2/wiki/Syntax
- commitSha string? - Explicit commit SHA to build.
- dir string? - Directory, relative to the source root, in which to run the build. This must be a relative path. If a step's
dir
is specified and is an absolute path, this value is ignored for that step's execution.
- invertRegex boolean? - Only trigger a build if the revision regex does NOT match the revision regex.
- projectId string? - ID of the project that owns the Cloud Source Repository. If omitted, the project ID requesting the build is assumed.
- repoName string? - Name of the Cloud Source Repository.
- substitutions record {}? - Substitutions to use in a triggered build. Should only be used with RunBuildTrigger
- tagName string? - Regex matching tags to build. The syntax of the regular expressions accepted is the syntax accepted by RE2 and described at https://github.com/google/re2/wiki/Syntax
googleapis.cloudbuild: Results
Artifacts created by the build pipeline.
Fields
- artifactManifest string? - Path to the artifact manifest. Only populated when artifacts are uploaded.
- artifactTiming TimeSpan? - Start and end times for a build execution phase.
- buildStepImages string[]? - List of build step digests, in the order corresponding to build step indices.
- buildStepOutputs string[]? - List of build step outputs, produced by builder images, in the order corresponding to build step indices. Cloud Builders can produce this output by writing to
$BUILDER_OUTPUT/output
. Only the first 4KB of data is stored.
- images BuiltImage[]? - Container images that were built as a part of the build.
- numArtifacts string? - Number of artifacts uploaded. Only populated when artifacts are uploaded.
googleapis.cloudbuild: RetryBuildRequest
Specifies a build to retry.
Fields
- id string? - Required. Build ID of the original build.
- name string? - The name of the
Build
to retry. Format:projects/{project}/locations/{location}/builds/{build}
- projectId string? - Required. ID of the project.
googleapis.cloudbuild: RunBuildTriggerRequest
Specifies a build trigger to run and the source to use.
Fields
- projectId string? - Required. ID of the project.
- 'source RepoSource? - Location of the source in a Google Cloud Source Repository.
- triggerId string? - Required. ID of the trigger.
googleapis.cloudbuild: Secret
Pairs a set of secret environment variables containing encrypted values with the Cloud KMS key to use to decrypt the value. Note: Use kmsKeyName
with available_secrets
instead of using kmsKeyName
with secret
. For instructions see: https://cloud.google.com/cloud-build/docs/securing-builds/use-encrypted-credentials.
Fields
- kmsKeyName string? - Cloud KMS key name to use to decrypt these envs.
- secretEnv record {}? - Map of environment variable name to its encrypted value. Secret environment variables must be unique across all of a build's secrets, and must be used by at least one build step. Values can be at most 64 KB in size. There can be at most 100 secret values across all of a build's secrets.
googleapis.cloudbuild: SecretManagerSecret
Pairs a secret environment variable with a SecretVersion in Secret Manager.
Fields
- env string? - Environment variable name to associate with the secret. Secret environment variables must be unique across all of a build's secrets, and must be used by at least one build step.
- versionName string? - Resource name of the SecretVersion. In format: projects//secrets//versions/*
googleapis.cloudbuild: Secrets
Secrets and secret environment variables.
Fields
- inline InlineSecret[]? - Secrets encrypted with KMS key and the associated secret environment variable.
- secretManager SecretManagerSecret[]? - Secrets in Secret Manager and associated secret environment variable.
googleapis.cloudbuild: SlackDelivery
SlackDelivery is the delivery configuration for delivering Slack messages via webhooks. See Slack webhook documentation at: https://api.slack.com/messaging/webhooks.
Fields
- webhookUri NotifierSecretRef? - NotifierSecretRef contains the reference to a secret stored in the corresponding NotifierSpec.
googleapis.cloudbuild: SMTPDelivery
SMTPDelivery is the delivery configuration for an SMTP (email) notification.
Fields
- fromAddress string? - This is the SMTP account/email that appears in the
From:
of the email. If empty, it is assumed to be sender.
- password NotifierSecretRef? - NotifierSecretRef contains the reference to a secret stored in the corresponding NotifierSpec.
- port string? - The SMTP port of the server.
- recipientAddresses string[]? - This is the list of addresses to which we send the email (i.e. in the
To:
of the email).
- senderAddress string? - This is the SMTP account/email that is used to send the message.
- server string? - The address of the SMTP server.
googleapis.cloudbuild: Source
Location of the source in a supported storage service.
Fields
- repoSource RepoSource? - Location of the source in a Google Cloud Source Repository.
- storageSource StorageSource? - Location of the source in an archive file in Google Cloud Storage.
- storageSourceManifest StorageSourceManifest? - Location of the source manifest in Google Cloud Storage. This feature is in Preview; see description here.
googleapis.cloudbuild: SourceProvenance
Provenance of the source. Ways to find the original source, or verify that some source was used for this build.
Fields
- fileHashes record {}? - Output only. Hash(es) of the build source, which can be used to verify that the original source integrity was maintained in the build. Note that
FileHashes
will only be populated ifBuildOptions
has requested aSourceProvenanceHash
. The keys to this map are file paths used as build source and the values contain the hash values for those files. If the build source came in a single package such as a gzipped tarfile (.tar.gz
), theFileHash
will be for the single path to that file.
- resolvedRepoSource RepoSource? - Location of the source in a Google Cloud Source Repository.
- resolvedStorageSource StorageSource? - Location of the source in an archive file in Google Cloud Storage.
- resolvedStorageSourceManifest StorageSourceManifest? - Location of the source manifest in Google Cloud Storage. This feature is in Preview; see description here.
googleapis.cloudbuild: Status
The Status
type defines a logical error model that is suitable for different programming environments, including REST APIs and RPC APIs. It is used by gRPC. Each Status
message contains three pieces of data: error code, error message, and error details. You can find out more about this error model and how to work with it in the API Design Guide.
Fields
- code int? - The status code, which should be an enum value of google.rpc.Code.
- details record {}[]? - A list of messages that carry the error details. There is a common set of message types for APIs to use.
- message string? - A developer-facing error message, which should be in English. Any user-facing error message should be localized and sent in the google.rpc.Status.details field, or localized by the client.
googleapis.cloudbuild: StorageSource
Location of the source in an archive file in Google Cloud Storage.
Fields
- bucket string? - Google Cloud Storage bucket containing the source (see Bucket Name Requirements).
- generation string? - Google Cloud Storage generation for the object. If the generation is omitted, the latest generation will be used.
- 'object string? - Google Cloud Storage object containing the source. This object must be a zipped (
.zip
) or gzipped archive file (.tar.gz
) containing source to build.
googleapis.cloudbuild: StorageSourceManifest
Location of the source manifest in Google Cloud Storage. This feature is in Preview; see description here.
Fields
- bucket string? - Google Cloud Storage bucket containing the source manifest (see Bucket Name Requirements).
- generation string? - Google Cloud Storage generation for the object. If the generation is omitted, the latest generation will be used.
- 'object string? - Google Cloud Storage object containing the source manifest. This object must be a JSON file.
googleapis.cloudbuild: TimeSpan
Start and end times for a build execution phase.
Fields
- endTime string? - End of time span.
- startTime string? - Start of time span.
googleapis.cloudbuild: UpdateGitHubEnterpriseConfigOperationMetadata
Metadata for UpdateGitHubEnterpriseConfig
operation.
Fields
- completeTime string? - Time the operation was completed.
- createTime string? - Time the operation was created.
- githubEnterpriseConfig string? - The resource name of the GitHubEnterprise to be updated. Format:
projects/{project}/locations/{location}/githubEnterpriseConfigs/{id}
.
googleapis.cloudbuild: UpdateWorkerPoolOperationMetadata
Metadata for the UpdateWorkerPool
operation.
Fields
- completeTime string? - Time the operation was completed.
- createTime string? - Time the operation was created.
- workerPool string? - The resource name of the
WorkerPool
being updated. Format:projects/{project}/locations/{location}/workerPools/{worker_pool}
.
googleapis.cloudbuild: Volume
Volume describes a Docker container volume which is mounted into build steps in order to persist files across build step execution.
Fields
- name string? - Name of the volume to mount. Volume names must be unique per build step and must be valid names for Docker volumes. Each named volume must be used by at least two build steps.
- path string? - Path at which to mount the volume. Paths must be absolute and cannot conflict with other volume paths on the same build step or with certain reserved volume paths.
googleapis.cloudbuild: Warning
A non-fatal problem encountered during the execution of the build.
Fields
- priority string? - The priority for this warning.
- text string? - Explanation of the warning generated.
googleapis.cloudbuild: WebhookConfig
WebhookConfig describes the configuration of a trigger that creates a build whenever a webhook is sent to a trigger's webhook URL.
Fields
- secret string? - Required. Resource name for the secret required as a URL parameter.
- state string? - Potential issues with the underlying Pub/Sub subscription configuration. Only populated on get requests.
googleapis.cloudbuild: WorkerConfig
Defines the configuration to be used for creating workers in the pool.
Fields
- diskSizeGb string? - Size of the disk attached to the worker, in GB. See Worker pool config file. Specify a value of up to 1000. If
0
is specified, Cloud Build will use a standard disk size.
- machineType string? - Machine type of a worker, such as
e2-medium
. See Worker pool config file. If left blank, Cloud Build will use a sensible default.
googleapis.cloudbuild: WorkerPool
Configuration for a WorkerPool
. Cloud Build owns and maintains a pool of workers for general use and have no access to a project's private network. By default, builds submitted to Cloud Build will use a worker from this pool. If your build needs access to resources on a private network, create and use a WorkerPool
to run your builds. Private WorkerPool
s give your builds access to any single VPC network that you administer, including any on-prem resources connected to that VPC network. For an overview of private pools, see Private pools overview.
Fields
- annotations record {}? - User specified annotations. See https://google.aip.dev/128#annotations for more details such as format and size limitations.
- createTime string? - Output only. Time at which the request to create the
WorkerPool
was received.
- deleteTime string? - Output only. Time at which the request to delete the
WorkerPool
was received.
- displayName string? - A user-specified, human-readable name for the
WorkerPool
. If provided, this value must be 1-63 characters.
- etag string? - Output only. Checksum computed by the server. May be sent on update and delete requests to ensure that the client has an up-to-date value before proceeding.
- name string? - Output only. The resource name of the
WorkerPool
, with formatprojects/{project}/locations/{location}/workerPools/{worker_pool}
. The value of{worker_pool}
is provided byworker_pool_id
inCreateWorkerPool
request and the value of{location}
is determined by the endpoint accessed.
- privatePoolV1Config PrivatePoolV1Config? - Configuration for a V1
PrivatePool
.
- state string? - Output only.
WorkerPool
state.
- uid string? - Output only. A unique identifier for the
WorkerPool
.
- updateTime string? - Output only. Time at which the request to update the
WorkerPool
was received.
Import
import ballerinax/googleapis.cloudbuild;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 2
Weekly downloads
Keywords
Finance/Billing
Cost/Paid
Vendor/Google
Contributors
Dependencies