googleapis.calendar
Module googleapis.calendar
API
Declarations
Definitions
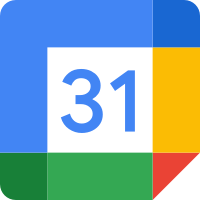
ballerinax/googleapis.calendar Ballerina library
Overview
The Google Calendar Connector provides the capability to manage events and calendar operations. It also provides the capability to support service account authorization that can provide delegated domain-wide access to the GSuite domain and support admins to do operations on behalf of the domain users.
This module supports Google Calendar API V3.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account.
- Obtain tokens - Follow the steps here to obtain tokens.
Quickstart
To use the Google Calendar connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/googleapis.calendar
module into the Ballerina project.
import ballerinax/googleapis.calendar;
Step 2: Create a new connector instance
Create a calendar:ConnectionConfig
with the OAuth2.0 tokens obtained and initialize the connector with it.
calendar:ConnectionConfig config = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET> refreshToken: <REFRESH_TOKEN>, refreshUrl: <REFRESH_URL>, } }; calendar:Client calendarClient = check new(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create quick event using the connector.string calendarId = "primary"; string title = "Sample Event"; public function main() returns error? { calendar:Event response = check calendarClient->quickAddEvent(calendarId, title); }
-
Use
bal run
command to compile and run the Ballerina program.
Functions
prepareUrlWithEventsOptionalParams
function prepareUrlWithEventsOptionalParams(string calendarId, int? count, string? pageToken, string? syncToken, EventFilterCriteria? optional) returns string
Prepare URL with optional parameters.
Parameters
- calendarId string - calendar Id
- count int? (default ()) - Number of events required in one page
- pageToken string? (default ()) - Token for retrieving next page
- syncToken string? (default ()) - Token for getting incremental sync
- optional EventFilterCriteria? (default ()) - Record that contains optional parameters
Return Type
- string - The prepared URL with encoded query
Clients
googleapis.calendar: Client
Ballerina Google Calendar connector provides the capability to access Google Calendar API. The connector let you perform calendar and event management operations.
Constructor
Initializes the connector. During initialization you can pass either BearerTokenConfig if you have a bearer token or OAuth2RefreshTokenGrantConfig if you have Oauth tokens. Create a Google account and obtain tokens following this guide.
init (ConnectionConfig config)
- config ConnectionConfig - Configurations required to initialize the client
getCalendars
function getCalendars(CalendarsToAccess? optional, string? userAccount) returns stream<Calendar, error?>|error
Gets calendars.
Parameters
- optional CalendarsToAccess? (default ()) - Record that contains optionals
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
createCalendar
function createCalendar(string title, string? userAccount) returns CalendarResource|error
Creates a calendar.
Parameters
- title string - Calendar name
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
Return Type
- CalendarResource|error - Created calendar on success or else an error
deleteCalendar
Deletes a calendar.
Parameters
- calendarId string - Calendar ID
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
Return Type
- error? -
()
or error on failure
createEvent
function createEvent(string calendarId, InputEvent event, EventsToAccess? optional, string? userAccount) returns Event|error
Creates an event.
Parameters
- calendarId string - Calendar ID
- event InputEvent - Record that contains event information
- optional EventsToAccess? (default ()) - Record that contains optional query parameters
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
quickAddEvent
function quickAddEvent(string calendarId, string text, string? sendUpdates, string? userAccount) returns Event|error
Creates an event at the moment with simple text.
Parameters
- calendarId string - Calendar ID
- text string - Event description
- sendUpdates string? (default ()) - Configuration for notifing the creation
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
updateEvent
function updateEvent(string calendarId, string eventId, InputEvent event, EventsToAccess? optional, string? userAccount) returns Event|error
Updates an existing event.
Parameters
- calendarId string - Calendar ID
- eventId string - Event ID
- event InputEvent - Record that contains updated information
- optional EventsToAccess? (default ()) - Record that contains optional query parameters
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
getEvents
function getEvents(string calendarId, EventFilterCriteria? filter, string? userAccount) returns stream<Event, error?>|error
Gets events.
Parameters
- calendarId string - Calendar ID
- filter EventFilterCriteria? (default ()) - Record that contains filtering criteria
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
getEvent
Gets an event.
Parameters
- calendarId string - Calendar ID
- eventId string - Event ID
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
deleteEvent
Deletes an event.
Parameters
- calendarId string - Calendar ID
- eventId string - Event ID
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
Return Type
- error? -
()
or else an error on failure
getEventsResponse
function getEventsResponse(string calendarId, int? count, string? pageToken, string? syncToken, EventFilterCriteria? filter, string? userAccount) returns EventResponse|error
Gets events response.
Parameters
- calendarId string - Calendar ID
- count int? (default ()) - Number of events required in one page
- pageToken string? (default ()) - Token for retrieving next page
- syncToken string? (default ()) - Token for getting incremental sync
- filter EventFilterCriteria? (default ()) - Record that contains filtering criteria
- userAccount string? (default ()) - The email address of the user for requesting delegated access in service account
Return Type
- EventResponse|error - EventResponse object on success or else an error
Enums
googleapis.calendar: OrderBy
Events order by.
Members
Records
googleapis.calendar: Attachment
Defines Attachment.
Fields
- fileUrl string - URL link to the attachment
- title string? - Attachment title
- mimeType string? - Internet media type (MIME type) of the attachment
- iconLink string? - URL link to the attachment's icon
- fileId string? - ID of the attached file
googleapis.calendar: Attendee
Defines attendee.
Fields
- id string? - The attendee's Profile ID
- email string - The attendee's email address
- displayName string? - The attendee's name
- organizer boolean? - Whether the attendee is the organizer of the event
- self boolean? - Whether this entry represents the calendar on which this copy of the event appears
- 'resource boolean? -
- optional boolean? - Whether this is an optional attendee
- responseStatus string? - The attendee's response status
- comment string? - The attendee's response comment
- additionalGuests int? - Number of additional guests
googleapis.calendar: Calendar
Represents Calendar.
Fields
- kind string - Type of the resource
- etag string - ETag of the resource
- id string - Identifier of the calendar
- summary string - Title of the calendar
- description string? - Description of the calendar
- location string? - Geographic location of the calendar
- timeZone string - The time zone of the calendar
- summaryOverride string? - The summary that the authenticated user has set for this calendar
- colorId string - The color id of the calendar.
- selected boolean? - Whether the calendar content shows up in the calendar UI
- backgroundColor string - The main color of the calendar in the hexadecimal format
- foregroundColor string - The foreground color of the calendar in the hexadecimal format
- hidden boolean? - Whether the calendar has been hidden from the list
- deleted boolean? - The effective access role that the authenticated user has on the calendar
- accessRole string - The effective access role that the authenticated user has on the calendar
- defaultReminders Reminder[] - The default reminders that the authenticated user has for this calendar
- conferenceProperties ConferenceProperties - Conferencing properties for this calendar
- primary boolean? - Whether the calendar is the primary calendar of the authenticated user
- notificationSettings NotificationSettings? - The notifications that the authenticated user is receiving for the calendar
googleapis.calendar: CalendarResource
Defines Calendar.
Fields
- kind string - Type of the resource
- etag string - ETag of the resource
- id string - Identifier of the calendar
- summary string - Title of the calendar
- description string? - Description of the calendar
- location string? - Geographic location of the calendar as free-form text
- timeZone string - The time zone of the calendar
- conferenceProperties ConferenceProperties - Conferencing properties for this calendar
googleapis.calendar: CalendarResponse
Defines calendar list response.
Fields
- kind string - Type of the collection
- etag string - ETag of the collection
- nextSyncToken string? - Token used to retrieve only the entries that have changed since this result was returned
- nextPageToken string? - Token used to access the next page of this result
- items Calendar[] - List of calendars
googleapis.calendar: CalendarsToAccess
Defines optionals for calendar list request.
Fields
- minAccessRole string? - The minimum access role for the user in the returned entries
- showDeleted boolean? - Whether to include deleted calendar list entries in the result
- showHidden boolean? - Whether to show hidden entries
googleapis.calendar: ConferenceData
Defines conference-related information.
Fields
- entryPoints EntryPoint[]? - Information about individual conference entry points
- conferenceSolution ConferenceSolution? - The conference solution
- conferenceId string? - The ID of the conference
- signature string - The signature of the conference data
- notes string? - Additional notes to display to the user
googleapis.calendar: ConferenceInputData
Defines conference-related information.
Fields
- createRequest CreateRequest? - A request to generate a new conference and attach it to the event
googleapis.calendar: ConferenceProperties
Defines conference properties.
Fields
- allowedConferenceSolutionTypes string[] - Types of conference solutions that are supported for this calendar
googleapis.calendar: ConferenceSolution
Defines conference solution.
Fields
- key Key - The key which can uniquely identify the conference solution for this event
- name string - The user-visible name of this solution
- iconUri string - The user-visible icon for this solution
googleapis.calendar: ConferenceSolutionKey
Defines conference solution key.
Fields
- 'type string -
googleapis.calendar: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig|JwtIssuerConfig - Configurations related to client authentication
googleapis.calendar: CreateRequest
Defines a request to generate a new conference and attach it to the event.
Fields
- requestId string - The client-generated unique ID for this request
- conferenceSolutionKey ConferenceSolutionKey - The conference solution
- status Status? - The status of the conference create request
googleapis.calendar: EntryPoint
Defines conference entry points.
Fields
- entryPointType string - The type of the conference entry point
- uri string - The URI of the entry point
- label string? - The label for the URI
- pin string? - The PIN to access the conference
- accessCode string? - The access code to access the conference
- meetingCode string? - The meeting code to access the conference
- passcode string? - The passcode to access the conference
- password string? - The password to access the conference
- regionCode string? - The region code of the conference
googleapis.calendar: Event
Defines Event.
Fields
- kind string - Type of the resource
- etag string - ETag of the resource
- id string - Opaque identifier of the event
- status string - Status of the event
- htmlLink string? - An absolute link to this event in the Google Calendar Web UI
- created string? - Creation time of the event
- updated string? - Last modification time of the event
- summary string? - Title of the event
- description string? - Description of the event
- location string? - Geographic location of the event as free-form text
- colorId string? - The color id of the event
- creator User? - The creator of the event
- organizer User? - The organizer of the event
- 'start Time? -
- end Time? - The end time of the event
- endTimeUnspecified boolean? - Whether the end time is actually unspecified
- recurrence string[]? - List of RRULE, EXRULE, RDATE and EXDATE lines for a recurring event, as specified in RFC5545
- recurringEventId string? - The id of the recurring event
- originalStartTime Time? - The start time of the event in recurring events
- transparency string? - Whether the event blocks time on the calendar
- visibility string? - Visibility of the event
- iCalUID string? - Event unique identifier as defined in RFC5545
- sequence int? - Sequence number as per iCalendar
- attendees Attendee[]? - The attendees of the event
- extendedProperties ExtendedProperties? - Extended properties of the event
- attendeesOmitted boolean? - Whether attendees may have been omitted from the event's representation
- hangoutLink string? - An absolute link to the Google+ hangout associated with this event
- conferenceData ConferenceData? - The conference-related information
- gadget Gadget? - A gadget that extends this event
- anyoneCanAddSelf boolean? - Whether anyone can invite themselves to the event
- guestsCanInviteOthers boolean? - Whether attendees other than the organizer can invite others to the event
- guestsCanModify boolean? - Whether attendees other than the organizer can modify the event
- guestsCanSeeOtherGuests boolean? - Whether attendees other than the organizer can see who the event's attendees are
- privateCopy boolean? - Whether enable event propagation
- locked boolean? - Whether this is a locked event copy
- reminders Reminders? - Information about the event's reminders
- 'source Source? -
- attachments Attachment[]? - File attachments for the event
- eventType string? - Specific type of the event [Default/ OutOfOffice]
googleapis.calendar: EventFilterCriteria
Defines get events optional parameters.
Fields
- iCalUID string? - Event unique identifier
- maxAttendees int? - The maximum number of attendees to include in the response
- orderBy OrderBy? - The order of the events returned in the result
- privateExtendedProperty string? - Extended private properties constraint specified as propertyName=value
- q string? - Free text search terms to find events that match these terms in any field, except for extended properties
- sharedExtendedProperty string? - Extended shared properties constraint specified as propertyName=value
- showDeleted boolean? - Whether to include deleted events (with status equals "cancelled") in the result
- showHiddenInvitations boolean? - Whether to include hidden invitations in the result
- singleEvents boolean? - Whether to expand recurring events into instances and only return single one-off events
- timeMax string? - Upper bound (exclusive) for an event's start time to filter by. Must be an RFC3339 timestamp
- timeMin string? - Lower bound (exclusive) for an event's end time to filter by. Must be an RFC3339 timestamp
- timeZone string? - Time zone used in the response
- updatedMin string? - Lower bound for an event's last modification time (as a RFC3339 timestamp) to filter by
googleapis.calendar: EventResponse
Defines event response.
Fields
- kind string - Type of the collection
- etag string - ETag of the collection
- summary string - Title of the calendar
- updated string - Last modification time of the calendar
- timeZone string - The time zone of the calendar
- accessRole string - The user's access role for this calendar
- defaultReminders Reminder[] - The default reminders on the calendar for the authenticated user
- nextSyncToken string? - Token used to retrieve only the entries that have changed since this result was returned
- nextPageToken string? - Token used to access the next page of this result
- items Event[] - List of events on the calendar
googleapis.calendar: EventsToAccess
Represents the optional query parameters of creating event.
Fields
- conferenceDataVersion int?(default ()) - Version number of conference data supported by the API client
- maxAttendees int?(default ()) - The maximum number of attendees to include in the response
- sendUpdates string?(default ()) - Whether to send notifications about the creation of the new event
- supportsAttachments boolean?(default ()) - Whether API client performing operation supports event attachment
googleapis.calendar: ExtendedProperties
Extended properties of the event.
Fields
- Fields Included from *Private
- string...
- Fields Included from *Shared
- string...
googleapis.calendar: Gadget
Defines gadget.
Fields
- preferences Preferences - Preferences
- 'type string? -
- title string? - Title of the gadget
- link string? - URL of the gadget
- iconLink string? - Icon URL of the gadget
- width string? - Width of the gadget in pixel
- height string? - Height of the gadget in pixel
- display string? - Display mode of the gadget
googleapis.calendar: InputEvent
Represents the elements representing event input.
Fields
- summary string? - Title of the event
- description string? - Description of the event
- location string? - Location of the event
- colorId string? - Color Id of the event
- id string? - Opaque identifier of the event
- 'start Time -
- end Time - End time of the event
- recurrence string[]? - List of RRULE, EXRULE, RDATE and EXDATE lines for a recurring event, as specified in RFC5545
- originalStartTime Time? - The start time of the event in recurring events
- transparency string? - Whether the event blocks time on the calendar
- visibility string? - Visibility of the event
- sequence int? - Sequence number as per iCalendar
- attendees Attendee[]? - The attendees of the event
- extendedProperties ExtendedProperties? - Properties of the event that appears on this calendar
- conferenceData ConferenceInputData? - The conference-related information
- anyoneCanAddSelf boolean? - Whether anyone can invite themselves to the event
- guestsCanInviteOthers boolean? - Whether attendees other than the organizer can invite others to the event
- guestsCanModify boolean? - Whether attendees other than the organizer can modify the event
- guestsCanSeeOtherGuests boolean? - Whether attendees other than the organizer can see who the event's attendees are
- reminders Reminders? - Information about the event's reminders
- 'source Source? -
- attachments Attachment[]? - Attachments of the events
googleapis.calendar: Key
Defines key.
Fields
- 'type string -
googleapis.calendar: Notification
Defines notification.
Fields
- 'type string -
- method string - The type of notification
googleapis.calendar: NotificationSettings
Defines notification settings.
Fields
- notifications Notification[] - The list of notifications set for this calendar
googleapis.calendar: Preferences
Defines preferences.
Fields
- string... - Rest field
googleapis.calendar: Private
Defines private event properties.
Fields
- string... - Rest field
googleapis.calendar: Reminder
Represents default reminders on the calendar for the authenticated user.
Fields
- method string - The method used by the reminder
- minutes int - Number of minutes before the start of the event when the reminder should trigger
googleapis.calendar: Reminders
Defines reminders.
Fields
- useDefault boolean - Whether the default reminders of the calendar apply to the event
- overrides Reminder[]? - List the reminders specific to the event
googleapis.calendar: Shared
Defines shared event properties.
Fields
- string... - Rest field
googleapis.calendar: Source
Defines source.
Fields
- url string - URL of the source pointing to a resource
- title string - Title of the source
googleapis.calendar: Status
Defines status.
Fields
- statusCode string - The current status of the conference create request
googleapis.calendar: Time
Defines time.
Fields
- date string? - The date, in the format "yyyy-mm-dd"
- dateTime string? - A combined date-time value formatted according to RFC3339
- timeZone string? - The time zone in which the time is specified
googleapis.calendar: User
Defines user.
Fields
- id string? - Id of the user
- email string - Email of the user
- displayName string? - Name of the user
- self boolean? - Whether the creator corresponds to the calendar on which this copy of the event appears
googleapis.calendar: WatchResponse
Defines watch response.
Fields
- kind string - Identifies this as a notification channel used to watch for changes to a resource
- id string - A UUID or similar unique string that identifies this channel
- resourceId string - An opaque ID that identifies the resource being watched on this channel
- resourceUri string - A version-specific identifier for the watched resource
- token string? - An arbitrary string delivered to the target address
- expiration string - Date and time of notification channel expiration
Import
import ballerinax/googleapis.calendar;
Metadata
Released date: about 1 year ago
Version: 3.2.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 10133
Current verison: 457
Weekly downloads
Keywords
Productivity/Calendars
Cost/Free
Vendor/Google
Contributors