googleapis.blogger
Module googleapis.blogger
API
Definitions
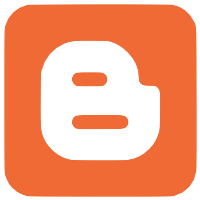
ballerinax/googleapis.blogger Ballerina library
Overview
This is a generated connector for Google Blogger API v3.0 OpenAPI specification. The Blogger API provides access to posts, comments and pages of a Blogger blog.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google Blogger connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/googleapis.blogger
module into the Ballerina project.
import ballerinax/googleapis.blogger;
Step 2: Create a new connector instance
Create a blogger:ClientConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
blogger:ClientConfig clientConfig = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET>, refreshUrl: <REFRESH_URL>, refreshToken: <REFRESH_TOKEN> } }; blogger:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve a blog by URL using the connector.
Retrieve a blog by URL
public function main() { blogger:Blog|error response = baseClient->getbyurlBloggerBlogs("<BLOG_URL>"); if (response is blogger:Blog) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.blogger: Client
This is a generated connector for Google Blogger API v3.0 OpenAPI specification. The Blogger API provides access to posts, comments and pages of a Blogger blog.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Google account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://blogger.googleapis.com" - URL of the target service
getbyurlBloggerBlogs
function getbyurlBloggerBlogs(string url, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? view) returns Blog|error
Gets a blog by url.
Parameters
- url string - Blog URL
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- view string? (default ()) - View type
getBloggerBlogs
function getBloggerBlogs(string blogId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? maxPosts, string? view) returns Blog|error
Gets a blog by id.
Parameters
- blogId string - Blog ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- maxPosts int? (default ()) - Maximum posts
- view string? (default ()) - View type
listbyblogBloggerComments
function listbyblogBloggerComments(string blogId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? endDate, boolean? fetchBodies, int? maxResults, string? pageToken, string? startDate, string[]? status) returns CommentList|error
Lists comments by blog.
Parameters
- blogId string - Blog ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- endDate string? (default ()) - End date
- fetchBodies boolean? (default ()) - Fetch bodies
- maxResults int? (default ()) - Maximum results
- pageToken string? (default ()) - Page token
- startDate string? (default ()) - Start date
- status string[]? (default ()) - Status
Return Type
- CommentList|error - Successful response
listBloggerPages
function listBloggerPages(string blogId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? fetchBodies, int? maxResults, string? pageToken, string[]? status, string? view) returns PageList|error
Lists pages.
Parameters
- blogId string - Blog ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- fetchBodies boolean? (default ()) - Fetch bodies
- maxResults int? (default ()) - Maximum results
- pageToken string? (default ()) - Page token
- status string[]? (default ()) - Status
- view string? (default ()) - View
insertBloggerPages
function insertBloggerPages(string blogId, Page payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? isDraft) returns Page|error
Inserts a page.
Parameters
- blogId string - Blog ID
- payload Page - Page request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- isDraft boolean? (default ()) - Is draft
getBloggerPages
function getBloggerPages(string blogId, string pageId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? view) returns Page|error
Gets a page by blog id and page id.
Parameters
- blogId string - Blog ID
- pageId string - Page ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- view string? (default ()) - View type
updateBloggerPages
function updateBloggerPages(string blogId, string pageId, Page payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? publish, boolean? revert) returns Page|error
Updates a page by blog id and page id.
Parameters
- blogId string - Blog ID
- pageId string - Page ID
- payload Page - Page request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- publish boolean? (default ()) - Publish
- revert boolean? (default ()) - Revert
deleteBloggerPages
function deleteBloggerPages(string blogId, string pageId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes a page by blog id and page id.
Parameters
- blogId string - Blog ID
- pageId string - Page ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
patchBloggerPages
function patchBloggerPages(string blogId, string pageId, Page payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? publish, boolean? revert) returns Page|error
Patches a page.
Parameters
- blogId string - Blog ID
- pageId string - Page ID
- payload Page - Page request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- publish boolean? (default ()) - Publish
- revert boolean? (default ()) - Revert
publishBloggerPages
function publishBloggerPages(string blogId, string pageId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Page|error
Publishes a page.
Parameters
- blogId string - Blog ID
- pageId string - Page ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
revertBloggerPages
function revertBloggerPages(string blogId, string pageId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Page|error
Reverts a published or scheduled page to draft state.
Parameters
- blogId string - Blog ID
- pageId string - Page ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getBloggerPageviews
function getBloggerPageviews(string blogId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string[]? range) returns Pageviews|error
Gets page views by blog id.
Parameters
- blogId string - Blog ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- range string[]? (default ()) - Range
listBloggerPosts
function listBloggerPosts(string blogId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? endDate, boolean? fetchBodies, boolean? fetchImages, string? labels, int? maxResults, string? orderBy, string? pageToken, string? startDate, string[]? status, string? view) returns PostList|error
Lists posts.
Parameters
- blogId string - Blog ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- endDate string? (default ()) - End date
- fetchBodies boolean? (default ()) - Fetch bodies
- fetchImages boolean? (default ()) - Fetch images
- labels string? (default ()) - Labels
- maxResults int? (default ()) - Maximum results
- orderBy string? (default ()) - Order by
- pageToken string? (default ()) - Page token
- startDate string? (default ()) - Start date
- status string[]? (default ()) - Status
- view string? (default ()) - View
insertBloggerPosts
function insertBloggerPosts(string blogId, Post payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? fetchBody, boolean? fetchImages, boolean? isDraft) returns Post|error
Inserts a post.
Parameters
- blogId string - Blog ID
- payload Post - Post request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- fetchBody boolean? (default ()) - Fetch body
- fetchImages boolean? (default ()) - Fetch images
- isDraft boolean? (default ()) - Is draft
getbypathBloggerPosts
function getbypathBloggerPosts(string blogId, string path, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? maxComments, string? view) returns Post|error
Gets a post by path.
Parameters
- blogId string - Blog ID
- path string - Path
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- maxComments int? (default ()) - Maximum comments
- view string? (default ()) - View
searchBloggerPosts
function searchBloggerPosts(string blogId, string q, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? fetchBodies, string? orderBy) returns PostList|error
Searches for posts matching given query terms in the specified blog.
Parameters
- blogId string - Blog ID
- q string - q
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- fetchBodies boolean? (default ()) - Fetch bodies
- orderBy string? (default ()) - Order by
getBloggerPosts
function getBloggerPosts(string blogId, string postId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? fetchBody, boolean? fetchImages, int? maxComments, string? view) returns Post|error
Gets a post by blog id and post id
Parameters
- blogId string - Blog ID
- postId string - Post ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- fetchBody boolean? (default ()) - Fetch body
- fetchImages boolean? (default ()) - Fetch images
- maxComments int? (default ()) - Maximum comments
- view string? (default ()) - View
updateBloggerPosts
function updateBloggerPosts(string blogId, string postId, Post payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? fetchBody, boolean? fetchImages, int? maxComments, boolean? publish, boolean? revert) returns Post|error
Updates a post by blog id and post id.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- payload Post - Post request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- fetchBody boolean? (default ()) - Fetch body
- fetchImages boolean? (default ()) - Fetch images
- maxComments int? (default ()) - Maximum comments
- publish boolean? (default ()) - Publish
- revert boolean? (default ()) - Revert
deleteBloggerPosts
function deleteBloggerPosts(string blogId, string postId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes a post by blog id and post id.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
patchBloggerPosts
function patchBloggerPosts(string blogId, string postId, Post payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? fetchBody, boolean? fetchImages, int? maxComments, boolean? publish, boolean? revert) returns Post|error
Patches a post.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- payload Post - Post request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- fetchBody boolean? (default ()) - Fetch body
- fetchImages boolean? (default ()) - Fetch images
- maxComments int? (default ()) - Maximum comments
- publish boolean? (default ()) - Publish
- revert boolean? (default ()) - Revert
listBloggerComments
function listBloggerComments(string blogId, string postId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? endDate, boolean? fetchBodies, int? maxResults, string? pageToken, string? startDate, string? status, string? view) returns CommentList|error
Lists comments.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- endDate string? (default ()) - End date
- fetchBodies boolean? (default ()) - Fetch bodies
- maxResults int? (default ()) - Maximum results
- pageToken string? (default ()) - Page token
- startDate string? (default ()) - Start date
- status string? (default ()) - Status
- view string? (default ()) - View type
Return Type
- CommentList|error - Successful response
getBloggerComments
function getBloggerComments(string blogId, string postId, string commentId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? view) returns Comment|error
Gets a comment by id.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- commentId string - Comment ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- view string? (default ()) - View
deleteBloggerComments
function deleteBloggerComments(string blogId, string postId, string commentId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes a comment by blog id, post id and comment id.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- commentId string - Comment ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
approveBloggerComments
function approveBloggerComments(string blogId, string postId, string commentId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Comment|error
Marks a comment as not spam by blog id, post id and comment id.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- commentId string - Comment ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
removecontentBloggerComments
function removecontentBloggerComments(string blogId, string postId, string commentId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Comment|error
Removes the content of a comment by blog id, post id and comment id.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- commentId string - Comment ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
markasspamBloggerComments
function markasspamBloggerComments(string blogId, string postId, string commentId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Comment|error
Marks a comment as spam by blog id, post id and comment id.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- commentId string - Comment ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
publishBloggerPosts
function publishBloggerPosts(string blogId, string postId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? publishDate) returns Post|error
Publishes a post.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- publishDate string? (default ()) - Publish date
revertBloggerPosts
function revertBloggerPosts(string blogId, string postId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Post|error
Reverts a published or scheduled post to draft state.
Parameters
- blogId string - Blog ID
- postId string - Post ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getBloggerUsers
function getBloggerUsers(string userId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns User|error
Gets one user by user_id.
Parameters
- userId string - User ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listbyuserBloggerBlogs
function listbyuserBloggerBlogs(string userId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, boolean? fetchUserInfo, string[]? role, string[]? status, string? view) returns BlogList|error
Lists blogs by user.
Parameters
- userId string - User ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- fetchUserInfo boolean? (default ()) - Fetch user information
- role string[]? (default ()) - Role
- status string[]? (default ()) - Default value of status is LIVE.
- view string? (default ()) - View type
getBloggerBloguserinfos
function getBloggerBloguserinfos(string userId, string blogId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? maxPosts) returns BlogUserInfo|error
Gets one blog and user info pair by blog id and user id.
Parameters
- userId string - User ID
- blogId string - Blog ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- maxPosts int? (default ()) - Maximum posts
Return Type
- BlogUserInfo|error - Successful response
listBloggerPostuserinfos
function listBloggerPostuserinfos(string userId, string blogId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? endDate, boolean? fetchBodies, string? labels, int? maxResults, string? orderBy, string? pageToken, string? startDate, string[]? status, string? view) returns PostUserInfosList|error
Lists post and user info pairs.
Parameters
- userId string - User ID
- blogId string - Blog ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- endDate string? (default ()) - End date
- fetchBodies boolean? (default ()) - Fetch bodies
- labels string? (default ()) - Labels
- maxResults int? (default ()) - Maximum results
- orderBy string? (default ()) - Order by
- pageToken string? (default ()) - Page token
- startDate string? (default ()) - Start date
- status string[]? (default ()) - Status
- view string? (default ()) - View type
Return Type
- PostUserInfosList|error - Successful response
getBloggerPostuserinfos
function getBloggerPostuserinfos(string userId, string blogId, string postId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? maxComments) returns PostUserInfo|error
Gets one post and user info pair, by post_id and user_id.
Parameters
- userId string - User ID
- blogId string - Blog ID
- postId string - Post ID
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- maxComments int? (default ()) - Maximum Comments
Return Type
- PostUserInfo|error - Successful response
Records
googleapis.blogger: Blog
Fields
- customMetaData string? - The JSON custom meta-data for the Blog.
- description string? - The description of this blog. This is displayed underneath the title.
- id string? - The identifier for this resource.
- kind string? - The kind of this entry. Always blogger#blog.
- locale BlogLocale? - The locale this Blog is set to.
- name string? - The name of this blog. This is displayed as the title.
- pages BlogPages? - The container of pages in this blog.
- posts BlogPosts? - The container of posts in this blog.
- published string? - RFC 3339 date-time when this blog was published.
- selfLink string? - The API REST URL to fetch this resource from.
- status string? - The status of the blog.
- updated string? - RFC 3339 date-time when this blog was last updated.
- url string? - The URL where this blog is published.
googleapis.blogger: BlogList
Fields
- blogUserInfos BlogUserInfo[]? - Admin level list of blog per-user information.
- items Blog[]? - The list of Blogs this user has Authorship or Admin rights over.
- kind string? - The kind of this entity. Always blogger#blogList.
googleapis.blogger: BlogLocale
The locale this Blog is set to.
Fields
- country string? - The country this blog's locale is set to.
- language string? - The language this blog is authored in.
- variant string? - The language variant this blog is authored in.
googleapis.blogger: BlogPages
The container of pages in this blog.
Fields
- selfLink string? - The URL of the container for pages in this blog.
- totalItems int? - The count of pages in this blog.
googleapis.blogger: BlogPerUserInfo
Fields
- blogId string? - ID of the Blog resource.
- hasAdminAccess boolean? - True if the user has Admin level access to the blog.
- kind string? - The kind of this entity. Always blogger#blogPerUserInfo.
- photosAlbumKey string? - The Photo Album Key for the user when adding photos to the blog.
- role string? - Access permissions that the user has for the blog (ADMIN, AUTHOR, or READER).
- userId string? - ID of the User.
googleapis.blogger: BlogPosts
The container of posts in this blog.
Fields
- items Post[]? - The List of Posts for this Blog.
- selfLink string? - The URL of the container for posts in this blog.
- totalItems int? - The count of posts in this blog.
googleapis.blogger: BlogUserInfo
Fields
- blog Blog? -
- blog_user_info BlogPerUserInfo? -
- kind string? - The kind of this entity. Always blogger#blogUserInfo.
googleapis.blogger: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.blogger: Comment
Fields
- author CommentAuthor? - The author of this Comment.
- blog CommentBlog? - Data about the blog containing this comment.
- content string? - The actual content of the comment. May include HTML markup.
- id string? - The identifier for this resource.
- inReplyTo CommentInreplyto? - Data about the comment this is in reply to.
- kind string? - The kind of this entry. Always blogger#comment.
- post CommentPost? - Data about the post containing this comment.
- published string? - RFC 3339 date-time when this comment was published.
- selfLink string? - The API REST URL to fetch this resource from.
- status string? - The status of the comment (only populated for admin users).
- updated string? - RFC 3339 date-time when this comment was last updated.
googleapis.blogger: CommentAuthor
The author of this Comment.
Fields
- displayName string? - The display name.
- id string? - The identifier of the creator.
- image CommentAuthorImage? - The creator's avatar.
- url string? - The URL of the creator's Profile page.
googleapis.blogger: CommentAuthorImage
The creator's avatar.
Fields
- url string? - The creator's avatar URL.
googleapis.blogger: CommentBlog
Data about the blog containing this comment.
Fields
- id string? - The identifier of the blog containing this comment.
googleapis.blogger: CommentInreplyto
Data about the comment this is in reply to.
Fields
- id string? - The identified of the parent of this comment.
googleapis.blogger: CommentList
Fields
- etag string? - Etag of the response.
- items Comment[]? - The List of Comments for a Post.
- kind string? - The kind of this entry. Always blogger#commentList.
- nextPageToken string? - Pagination token to fetch the next page, if one exists.
- prevPageToken string? - Pagination token to fetch the previous page, if one exists.
googleapis.blogger: CommentPost
Data about the post containing this comment.
Fields
- id string? - The identifier of the post containing this comment.
googleapis.blogger: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.blogger: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.blogger: Page
Fields
- author PageAuthor? - The author of this Page.
- blog PageBlog? - Data about the blog containing this Page.
- content string? - The body content of this Page, in HTML.
- etag string? - Etag of the resource.
- id string? - The identifier for this resource.
- kind string? - The kind of this entity. Always blogger#page.
- published string? - RFC 3339 date-time when this Page was published.
- selfLink string? - The API REST URL to fetch this resource from.
- status string? - The status of the page for admin resources (either LIVE or DRAFT).
- title string? - The title of this entity. This is the name displayed in the Admin user interface.
- updated string? - RFC 3339 date-time when this Page was last updated.
- url string? - The URL that this Page is displayed at.
googleapis.blogger: PageAuthor
The author of this Page.
Fields
- displayName string? - The display name.
- id string? - The identifier of the creator.
- image CommentAuthorImage? - The creator's avatar.
- url string? - The URL of the creator's Profile page.
googleapis.blogger: PageBlog
Data about the blog containing this Page.
Fields
- id string? - The identifier of the blog containing this page.
googleapis.blogger: PageList
Fields
- etag string? - Etag of the response.
- items Page[]? - The list of Pages for a Blog.
- kind string? - The kind of this entity. Always blogger#pageList.
- nextPageToken string? - Pagination token to fetch the next page, if one exists.
googleapis.blogger: Pageviews
Fields
- blogId string? - Blog Id.
- counts PageviewsCounts[]? - The container of posts in this blog.
- kind string? - The kind of this entry. Always blogger#page_views.
googleapis.blogger: PageviewsCounts
Fields
- count string? - Count of page views for the given time range.
- timeRange string? - Time range the given count applies to.
googleapis.blogger: Post
Fields
- author PostAuthor? - The author of this Post.
- blog PostBlog? - Data about the blog containing this Post.
- content string? - The content of the Post. May contain HTML markup.
- customMetaData string? - The JSON meta-data for the Post.
- etag string? - Etag of the resource.
- id string? - The identifier of this Post.
- images PostImages[]? - Display image for the Post.
- kind string? - The kind of this entity. Always blogger#post.
- labels string[]? - The list of labels this Post was tagged with.
- location PostLocation? - The location for geotagged posts.
- published string? - RFC 3339 date-time when this Post was published.
- readerComments string? - Comment control and display setting for readers of this post.
- replies PostReplies? - The container of comments on this Post.
- selfLink string? - The API REST URL to fetch this resource from.
- status string? - Status of the post. Only set for admin-level requests.
- title string? - The title of the Post.
- titleLink string? - The title link URL, similar to atom's related link.
- updated string? - RFC 3339 date-time when this Post was last updated.
- url string? - The URL where this Post is displayed.
googleapis.blogger: PostAuthor
The author of this Post.
Fields
- displayName string? - The display name.
- id string? - The identifier of the creator.
- image CommentAuthorImage? - The creator's avatar.
- url string? - The URL of the creator's Profile page.
googleapis.blogger: PostBlog
Data about the blog containing this Post.
Fields
- id string? - The identifier of the Blog that contains this Post.
googleapis.blogger: PostImages
Fields
- url string? -
googleapis.blogger: PostList
Fields
- etag string? - Etag of the response.
- items Post[]? - The list of Posts for this Blog.
- kind string? - The kind of this entity. Always blogger#postList.
- nextPageToken string? - Pagination token to fetch the next page, if one exists.
- prevPageToken string? - Pagination token to fetch the previous page, if one exists.
googleapis.blogger: PostLocation
The location for geotagged posts.
Fields
- lat decimal? - Location's latitude.
- lng decimal? - Location's longitude.
- name string? - Location name.
- span string? - Location's viewport span. Can be used when rendering a map preview.
googleapis.blogger: PostPerUserInfo
Fields
- blogId string? - ID of the Blog that the post resource belongs to.
- hasEditAccess boolean? - True if the user has Author level access to the post.
- kind string? - The kind of this entity. Always blogger#postPerUserInfo.
- postId string? - ID of the Post resource.
- userId string? - ID of the User.
googleapis.blogger: PostReplies
The container of comments on this Post.
Fields
- items Comment[]? - The List of Comments for this Post.
- selfLink string? - The URL of the comments on this post.
- totalItems string? - The count of comments on this post.
googleapis.blogger: PostUserInfo
Fields
- kind string? - The kind of this entity. Always blogger#postUserInfo.
- post Post? -
- post_user_info PostPerUserInfo? -
googleapis.blogger: PostUserInfosList
Fields
- items PostUserInfo[]? - The list of Posts with User information for the post, for this Blog.
- kind string? - The kind of this entity. Always blogger#postList.
- nextPageToken string? - Pagination token to fetch the next page, if one exists.
googleapis.blogger: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.blogger: User
Fields
- about string? - Profile summary information.
- blogs UserBlogs? - The container of blogs for this user.
- created string? - The timestamp of when this profile was created, in seconds since epoch.
- displayName string? - The display name.
- id string? - The identifier for this User.
- kind string? - The kind of this entity. Always blogger#user.
- locale UserLocale? - This user's locale
- selfLink string? - The API REST URL to fetch this resource from.
- url string? - The user's profile page.
googleapis.blogger: UserBlogs
The container of blogs for this user.
Fields
- selfLink string? - The URL of the Blogs for this user.
googleapis.blogger: UserLocale
This user's locale
Fields
- country string? - The country this blog's locale is set to.
- language string? - The language this blog is authored in.
- variant string? - The language variant this blog is authored in.
Import
import ballerinax/googleapis.blogger;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 2
Weekly downloads
Keywords
Content & Files/Blogs
Cost/Free
Vendor/Google
Contributors
Dependencies