googleapis.appsscript
Module googleapis.appsscript
API
Definitions
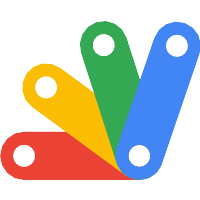
ballerinax/googleapis.appsscript Ballerina library
Overview
This is a generated connector for Google Apps Script API v1 OpenAPI specification. Manages and executes Google Apps Script projects.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google Apps Script connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/googleapis.appsscript
module into the Ballerina project.
import ballerinax/googleapis.appsscript;
Step 2: Create a new connector instance
Create a tasks:ClientConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
appsscript:ClientConfig clientConfig = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET>, refreshUrl: <REFRESH_URL>, refreshToken: <REFRESH_TOKEN> } }; appsscript:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list information about processes made by or on behalf of a user, such as process type and current status using the connector.
List information about processes made by or on behalf of a user.
public function main() { appsscript:ListUserProcessesResponse|error response = baseClient->listProcesses(); if (response is appsscript:ListUserProcessesResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.appsscript: Client
This is a generated connector for Google Apps Script API v1 OpenAPI specification. Manages and executes Google Apps Script projects.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Google account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://script.googleapis.com/" - URL of the target service
listProcesses
function listProcesses(string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken, string? userprocessfilterDeploymentid, string? userprocessfilterEndtime, string? userprocessfilterFunctionname, string? userprocessfilterProjectname, string? userprocessfilterScriptid, string? userprocessfilterStarttime, string[]? userprocessfilterStatuses, string[]? userprocessfilterTypes, string[]? userprocessfilterUseraccesslevels) returns ListUserProcessesResponse|error
List information about processes made by or on behalf of a user, such as process type and current status.
Parameters
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - The maximum number of returned processes per page of results. Defaults to 50.
- pageToken string? (default ()) - The token for continuing a previous list request on the next page. This should be set to the value of
nextPageToken
from a previous response.
- userprocessfilterDeploymentid string? (default ()) - Optional field used to limit returned processes to those originating from projects with a specific deployment ID.
- userprocessfilterEndtime string? (default ()) - Optional field used to limit returned processes to those that completed on or before the given timestamp.
- userprocessfilterFunctionname string? (default ()) - Optional field used to limit returned processes to those originating from a script function with the given function name.
- userprocessfilterProjectname string? (default ()) - Optional field used to limit returned processes to those originating from projects with project names containing a specific string.
- userprocessfilterScriptid string? (default ()) - Optional field used to limit returned processes to those originating from projects with a specific script ID.
- userprocessfilterStarttime string? (default ()) - Optional field used to limit returned processes to those that were started on or after the given timestamp.
- userprocessfilterStatuses string[]? (default ()) - Optional field used to limit returned processes to those having one of the specified process statuses.
- userprocessfilterTypes string[]? (default ()) - Optional field used to limit returned processes to those having one of the specified process types.
- userprocessfilterUseraccesslevels string[]? (default ()) - Optional field used to limit returned processes to those having one of the specified user access levels.
Return Type
- ListUserProcessesResponse|error - Successful response
listscriptprocessesProcesses
function listscriptprocessesProcesses(string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken, string? scriptId, string? scriptprocessfilterDeploymentid, string? scriptprocessfilterEndtime, string? scriptprocessfilterFunctionname, string? scriptprocessfilterStarttime, string[]? scriptprocessfilterStatuses, string[]? scriptprocessfilterTypes, string[]? scriptprocessfilterUseraccesslevels) returns ListScriptProcessesResponse|error
List information about a script's executed processes, such as process type and current status.
Parameters
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - The maximum number of returned processes per page of results. Defaults to 50.
- pageToken string? (default ()) - The token for continuing a previous list request on the next page. This should be set to the value of
nextPageToken
from a previous response.
- scriptId string? (default ()) - The script ID of the project whose processes are listed.
- scriptprocessfilterDeploymentid string? (default ()) - Optional field used to limit returned processes to those originating from projects with a specific deployment ID.
- scriptprocessfilterEndtime string? (default ()) - Optional field used to limit returned processes to those that completed on or before the given timestamp.
- scriptprocessfilterFunctionname string? (default ()) - Optional field used to limit returned processes to those originating from a script function with the given function name.
- scriptprocessfilterStarttime string? (default ()) - Optional field used to limit returned processes to those that were started on or after the given timestamp.
- scriptprocessfilterStatuses string[]? (default ()) - Optional field used to limit returned processes to those having one of the specified process statuses.
- scriptprocessfilterTypes string[]? (default ()) - Optional field used to limit returned processes to those having one of the specified process types.
- scriptprocessfilterUseraccesslevels string[]? (default ()) - Optional field used to limit returned processes to those having one of the specified user access levels.
Return Type
- ListScriptProcessesResponse|error - Successful response
createProjects
function createProjects(CreateProjectRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Project|error
Creates a new, empty script project with no script files and a base manifest file.
Parameters
- payload CreateProjectRequest - CreateProject request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getProjects
function getProjects(string scriptId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Project|error
Gets a script project's metadata.
Parameters
- scriptId string - The script project's Drive ID.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getcontentProjects
function getcontentProjects(string scriptId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? versionNumber) returns Content|error
Gets the content of the script project, including the code source and metadata for each script file.
Parameters
- scriptId string - The script project's Drive ID.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- versionNumber int? (default ()) - The version number of the project to retrieve. If not provided, the project's HEAD version is returned.
updatecontentProjects
function updatecontentProjects(string scriptId, Content payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Content|error
Updates the content of the specified script project. This content is stored as the HEAD version, and is used when the script is executed as a trigger, in the script editor, in add-on preview mode, or as a web app or Apps Script API in development mode. This clears all the existing files in the project.
Parameters
- scriptId string - The script project's Drive ID.
- payload Content - Content request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listProjectsDeployments
function listProjectsDeployments(string scriptId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken) returns ListDeploymentsResponse|error
Lists the deployments of an Apps Script project.
Parameters
- scriptId string - The script project's Drive ID.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - The maximum number of deployments on each returned page. Defaults to 50.
- pageToken string? (default ()) - The token for continuing a previous list request on the next page. This should be set to the value of
nextPageToken
from a previous response.
Return Type
- ListDeploymentsResponse|error - Successful response
createProjectsDeployments
function createProjectsDeployments(string scriptId, DeploymentConfig payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Deployment|error
Creates a deployment of an Apps Script project.
Parameters
- scriptId string - The script project's Drive ID.
- payload DeploymentConfig - DeploymentConfig request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- Deployment|error - Successful response
getProjectsDeployments
function getProjectsDeployments(string scriptId, string deploymentId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Deployment|error
Gets a deployment of an Apps Script project.
Parameters
- scriptId string - The script project's Drive ID.
- deploymentId string - The deployment ID.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- Deployment|error - Successful response
updateProjectsDeployments
function updateProjectsDeployments(string scriptId, string deploymentId, UpdateDeploymentRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Deployment|error
Updates a deployment of an Apps Script project.
Parameters
- scriptId string - The script project's Drive ID.
- deploymentId string - The deployment ID for this deployment.
- payload UpdateDeploymentRequest - UpdateDeployment request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- Deployment|error - Successful response
deleteProjectsDeployments
function deleteProjectsDeployments(string scriptId, string deploymentId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes a deployment of an Apps Script project.
Parameters
- scriptId string - The script project's Drive ID.
- deploymentId string - The deployment ID to be undeployed.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getmetricsProjects
function getmetricsProjects(string scriptId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? metricsfilterDeploymentid, string? metricsGranularity) returns Metrics|error
Get metrics data for scripts, such as number of executions and active users.
Parameters
- scriptId string - Required field indicating the script to get metrics for.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- metricsfilterDeploymentid string? (default ()) - Optional field indicating a specific deployment to retrieve metrics from.
- metricsGranularity string? (default ()) - Required field indicating what granularity of metrics are returned.
listProjectsVersions
function listProjectsVersions(string scriptId, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken) returns ListVersionsResponse|error
List the versions of a script project.
Parameters
- scriptId string - The script project's Drive ID.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - The maximum number of versions on each returned page. Defaults to 50.
- pageToken string? (default ()) - The token for continuing a previous list request on the next page. This should be set to the value of
nextPageToken
from a previous response.
Return Type
- ListVersionsResponse|error - Successful response
createProjectsVersions
function createProjectsVersions(string scriptId, Version payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Version|error
Creates a new immutable version using the current code, with a unique version number.
Parameters
- scriptId string - The script project's Drive ID.
- payload Version - Version description
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getProjectsVersions
function getProjectsVersions(string scriptId, int versionNumber, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Version|error
Gets a version of a script project.
Parameters
- scriptId string - The script project's Drive ID.
- versionNumber int - The version number.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
runScripts
function runScripts(string scriptId, ExecutionRequest payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Operation|error
Runs a function in an Apps Script project. The script project must be deployed for use with the Apps Script API and the calling application must share the same Cloud Platform project. This method requires authorization with an OAuth 2.0 token that includes at least one of the scopes listed in the Authorization section; script projects that do not require authorization cannot be executed through this API. To find the correct scopes to include in the authentication token, open the script project Overview page and scroll down to "Project OAuth Scopes." The error 403, PERMISSION_DENIED: The caller does not have permission
indicates that the Cloud Platform project used to authorize the request is not the same as the one used by the script.
Parameters
- scriptId string - The script ID of the script to be executed. Find the script ID on the Project settings page under "IDs."
- payload ExecutionRequest - Execution request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Records
googleapis.appsscript: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.appsscript: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.appsscript: Content
The Content resource.
Fields
- files File[]? - The list of script project files. One of the files is a script manifest; it must be named "appsscript", must have type of JSON, and include the manifest configurations for the project.
- scriptId string? - The script project's Drive ID.
googleapis.appsscript: CreateProjectRequest
Request to create a script project. Request to create a script project.
Fields
- parentId string? - The Drive ID of a parent file that the created script project is bound to. This is usually the ID of a Google Doc, Google Sheet, Google Form, or Google Slides file. If not set, a standalone script project is created.
- title string? - The title for the project.
googleapis.appsscript: Deployment
Representation of a single script deployment.
Fields
- deploymentConfig DeploymentConfig? - Metadata the defines how a deployment is configured.
- deploymentId string? - The deployment ID for this deployment.
- entryPoints EntryPoint[]? - The deployment's entry points.
- updateTime string? - Last modified date time stamp.
googleapis.appsscript: DeploymentConfig
Metadata the defines how a deployment is configured.
Fields
- description string? - The description for this deployment.
- manifestFileName string? - The manifest file name for this deployment.
- scriptId string? - The script project's Drive ID.
- versionNumber int? - The version number on which this deployment is based.
googleapis.appsscript: EntryPoint
A configuration that defines how a deployment is accessed externally.
Fields
- addOn GoogleAppsScriptTypeAddOnEntryPoint? - An add-on entry point.
- entryPointType string? - The type of the entry point.
- executionApi GoogleAppsScriptTypeExecutionApiEntryPoint? - An API executable entry point.
- webApp GoogleAppsScriptTypeWebAppEntryPoint? - A web application entry point.
googleapis.appsscript: ExecuteStreamResponse
The response for executing or debugging a function in an Apps Script project.
Fields
- result ScriptExecutionResult? - The result of an execution.
googleapis.appsscript: ExecutionError
An object that provides information about the nature of an error resulting from an attempted execution of a script function using the Apps Script API. If a run call succeeds but the script function (or Apps Script itself) throws an exception, the response body's error field contains a Status object. The Status
object's details
field contains an array with a single one of these ExecutionError
objects.
Fields
- errorMessage string? - The error message thrown by Apps Script, usually localized into the user's language.
- errorType string? - The error type, for example
TypeError
orReferenceError
. If the error type is unavailable, this field is not included.
- scriptStackTraceElements ScriptStackTraceElement[]? - An array of objects that provide a stack trace through the script to show where the execution failed, with the deepest call first.
googleapis.appsscript: ExecutionRequest
A request to run the function in a script. The script is identified by the specified script_id
. Executing a function on a script returns results based on the implementation of the script.
Fields
- devMode boolean? - If
true
and the user is an owner of the script, the script runs at the most recently saved version rather than the version deployed for use with the Apps Script API. Optional; default isfalse
.
- 'function string? - The name of the function to execute in the given script. The name does not include parentheses or parameters. It can reference a function in an included library such as
Library.libFunction1
.
- parameters anydata[]? - The parameters to be passed to the function being executed. The object type for each parameter should match the expected type in Apps Script. Parameters cannot be Apps Script-specific object types (such as a
Document
or aCalendar
); they can only be primitive types such asstring
,number
,array
,object
, orboolean
. Optional.
- sessionState string? - Deprecated. For use with Android add-ons only. An ID that represents the user's current session in the Android app for Google Docs or Sheets, included as extra data in the Intent that launches the add-on. When an Android add-on is run with a session state, it gains the privileges of a bound script—that is, it can access information like the user's current cursor position (in Docs) or selected cell (in Sheets). To retrieve the state, call
Intent.getStringExtra("com.google.android.apps.docs.addons.SessionState")
. Optional.
googleapis.appsscript: ExecutionResponse
An object that provides the return value of a function executed using the Apps Script API. If the script function returns successfully, the response body's response field contains this ExecutionResponse
object.
Fields
- result anydata? - The return value of the script function. The type matches the object type returned in Apps Script. Functions called using the Apps Script API cannot return Apps Script-specific objects (such as a
Document
or aCalendar
); they can only return primitive types such as astring
,number
,array
,object
, orboolean
.
googleapis.appsscript: File
An individual file within a script project. A file is a third-party source code created by one or more developers. It can be a server-side JS code, HTML, or a configuration file. Each script project can contain multiple files.
Fields
- createTime string? - Creation date timestamp. This read-only field is only visible to users who have WRITER permission for the script project.
- functionSet GoogleAppsScriptTypeFunctionSet? - A set of functions. No duplicates are permitted.
- lastModifyUser GoogleAppsScriptTypeUser? - A simple user profile resource.
- name string? - The name of the file. The file extension is not part of the file name, which can be identified from the type field.
- 'source string? - The file content.
- 'type string? - The type of the file.
- updateTime string? - Last modified date timestamp. This read-only field is only visible to users who have WRITER permission for the script project.
googleapis.appsscript: GoogleAppsScriptTypeAddOnEntryPoint
An add-on entry point.
Fields
- addOnType string? - The add-on's required list of supported container types.
- description string? - The add-on's optional description.
- helpUrl string? - The add-on's optional help URL.
- postInstallTipUrl string? - The add-on's required post install tip URL.
- reportIssueUrl string? - The add-on's optional report issue URL.
- title string? - The add-on's required title.
googleapis.appsscript: GoogleAppsScriptTypeExecutionApiConfig
API executable entry point configuration.
Fields
- access string? - Who has permission to run the API executable.
googleapis.appsscript: GoogleAppsScriptTypeExecutionApiEntryPoint
An API executable entry point.
Fields
- entryPointConfig GoogleAppsScriptTypeExecutionApiConfig? - API executable entry point configuration.
googleapis.appsscript: GoogleAppsScriptTypeFunction
Represents a function in a script project.
Fields
- name string? - The function name in the script project.
googleapis.appsscript: GoogleAppsScriptTypeFunctionSet
A set of functions. No duplicates are permitted.
Fields
- values GoogleAppsScriptTypeFunction[]? - A list of functions composing the set.
googleapis.appsscript: GoogleAppsScriptTypeProcess
Representation of a single script process execution that was started from the script editor, a trigger, an application, or using the Apps Script API. This is distinct from the Operation
resource, which only represents executions started via the Apps Script API.
Fields
- duration string? - Duration the execution spent executing.
- functionName string? - Name of the function the started the execution.
- processStatus string? - The executions status.
- processType string? - The executions type.
- projectName string? - Name of the script being executed.
- startTime string? - Time the execution started.
- userAccessLevel string? - The executing users access level to the script.
googleapis.appsscript: GoogleAppsScriptTypeUser
A simple user profile resource.
Fields
- domain string? - The user's domain.
- email string? - The user's identifying email address.
- name string? - The user's display name.
- photoUrl string? - The user's photo.
googleapis.appsscript: GoogleAppsScriptTypeWebAppConfig
Web app entry point configuration.
Fields
- access string? - Who has permission to run the web app.
- executeAs string? - Who to execute the web app as.
googleapis.appsscript: GoogleAppsScriptTypeWebAppEntryPoint
A web application entry point.
Fields
- entryPointConfig GoogleAppsScriptTypeWebAppConfig? - Web app entry point configuration.
- url string? - The URL for the web application.
googleapis.appsscript: ListDeploymentsResponse
Response with the list of deployments for the specified Apps Script project.
Fields
- deployments Deployment[]? - The list of deployments.
- nextPageToken string? - The token that can be used in the next call to get the next page of results.
googleapis.appsscript: ListScriptProcessesResponse
Response with the list of Process resources.
Fields
- nextPageToken string? - Token for the next page of results. If empty, there are no more pages remaining.
- processes GoogleAppsScriptTypeProcess[]? - List of processes matching request parameters.
googleapis.appsscript: ListUserProcessesResponse
Response with the list of Process resources.
Fields
- nextPageToken string? - Token for the next page of results. If empty, there are no more pages remaining.
- processes GoogleAppsScriptTypeProcess[]? - List of processes matching request parameters.
googleapis.appsscript: ListValue
ListValue
is a wrapper around a repeated field of values.
Fields
- values Value[]? - Repeated field of dynamically typed values.
googleapis.appsscript: ListVersionsResponse
Response with the list of the versions for the specified script project.
Fields
- nextPageToken string? - The token use to fetch the next page of records. if not exist in the response, that means no more versions to list.
- versions Version[]? - The list of versions.
googleapis.appsscript: Metrics
Resource containing usage stats for a given script, based on the supplied filter and mask present in the request.
Fields
- activeUsers MetricsValue[]? - Number of active users.
- failedExecutions MetricsValue[]? - Number of failed executions.
- totalExecutions MetricsValue[]? - Number of total executions.
googleapis.appsscript: MetricsValue
Metrics value that holds number of executions counted.
Fields
- endTime string? - Required field indicating the end time of the interval.
- startTime string? - Required field indicating the start time of the interval.
- value string? - Indicates the number of executions counted.
googleapis.appsscript: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.appsscript: Operation
A representation of an execution of an Apps Script function started with run. The execution response does not arrive until the function finishes executing. The maximum execution runtime is listed in the Apps Script quotas guide. After execution has started, it can have one of four outcomes: - If the script function returns successfully, the response field contains an ExecutionResponse object with the function's return value in the object's result
field. - If the script function (or Apps Script itself) throws an exception, the error field contains a Status object. The Status
object's details
field contains an array with a single ExecutionError object that provides information about the nature of the error. - If the execution has not yet completed, the done field is false
and the neither the response
nor error
fields are present. - If the run
call itself fails (for example, because of a malformed request or an authorization error), the method returns an HTTP response code in the 4XX range with a different format for the response body. Client libraries automatically convert a 4XX response into an exception class.
Fields
- done boolean? - This field indicates whether the script execution has completed. A completed execution has a populated
response
field containing the ExecutionResponse from function that was executed.
- 'error Status? - If a
run
call succeeds but the script function (or Apps Script itself) throws an exception, the response body's error field contains thisStatus
object.
- response record {}? - If the script function returns successfully, this field contains an ExecutionResponse object with the function's return value.
googleapis.appsscript: Project
The script project resource.
Fields
- createTime string? - When the script was created.
- creator GoogleAppsScriptTypeUser? - A simple user profile resource.
- lastModifyUser GoogleAppsScriptTypeUser? - A simple user profile resource.
- parentId string? - The parent's Drive ID that the script will be attached to. This is usually the ID of a Google Document or Google Sheet. This filed is optional, and if not set, a stand-alone script will be created.
- scriptId string? - The script project's Drive ID.
- title string? - The title for the project.
- updateTime string? - When the script was last updated.
googleapis.appsscript: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.appsscript: ScriptExecutionResult
The result of an execution.
Fields
- returnValue Value? -
Value
represents a dynamically typed value which is the outcome of an executed script.
googleapis.appsscript: ScriptStackTraceElement
A stack trace through the script that shows where the execution failed.
Fields
- 'function string? - The name of the function that failed.
- lineNumber int? - The line number where the script failed.
googleapis.appsscript: Status
If a run
call succeeds but the script function (or Apps Script itself) throws an exception, the response body's error field contains this Status
object.
Fields
- code int? - The status code. For this API, this value either: - 10, indicating a
SCRIPT_TIMEOUT
error, - 3, indicating anINVALID_ARGUMENT
error, or - 1, indicating aCANCELLED
execution.
- details record {}[]? - An array that contains a single ExecutionError object that provides information about the nature of the error.
- message string? - A developer-facing error message, which is in English. Any user-facing error message is localized and sent in the details field, or localized by the client.
googleapis.appsscript: Struct
Struct
represents a structured data value, consisting of fields which map to dynamically typed values.
Fields
- fields record {}? - Unordered map of dynamically typed values.
googleapis.appsscript: UpdateDeploymentRequest
Request with deployment information to update an existing deployment.
Fields
- deploymentConfig DeploymentConfig? - Metadata the defines how a deployment is configured.
googleapis.appsscript: Value
Value
represents a dynamically typed value which is the outcome of an executed script.
Fields
- boolValue boolean? - Represents a boolean value.
- bytesValue string? - Represents raw byte values.
- dateValue string? - Represents a date in ms since the epoch.
- listValue ListValue? -
ListValue
is a wrapper around a repeated field of values.
- nullValue string? - Represents a null value.
- numberValue decimal? - Represents a double value.
- protoValue record {}? - Represents a structured proto value.
- stringValue string? - Represents a string value.
- structValue Struct? -
Struct
represents a structured data value, consisting of fields which map to dynamically typed values.
googleapis.appsscript: Version
A resource representing a script project version. A version is a "snapshot" of a script project and is similar to a read-only branched release. When creating deployments, the version to use must be specified.
Fields
- createTime string? - When the version was created.
- description string? - The description for this version.
- scriptId string? - The script project's Drive ID.
- versionNumber int? - The incremental ID that is created by Apps Script when a version is created. This is system assigned number and is immutable once created.
Import
import ballerinax/googleapis.appsscript;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 2
Weekly downloads
Keywords
Content & Files/Documents
Cost/Free
Vendor/Google
Contributors
Dependencies