gitlab
Module gitlab
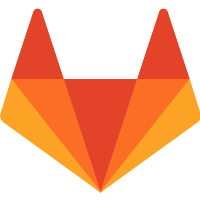
ballerinax/gitlab Ballerina library
Overview
This is a generated connector for GitLab GitLab REST API v4 OpenAPI specification.
Client endpoint for GitLab API currently supports operations related to access requests and access tokens in GitLab.
Prerequisites
- Create a GitLab account
- Obtain tokens
- Use this guide to obtain the PAT tokens related to your account
Quickstart
To use the GitLab connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/gitlab module into the Ballerina project.
import ballerinax/gitlab;
Step 2 - Create a new connector instance
You can now make the connection configuration using the PAT token
gitlab:ApiKeysConfig apiKeyConfig = { "privateToken": <GITLAB_PAT_TOKEN> }; gitlab:Client baseClient = check new Client(apiKeyConfig);
Step 3 - Invoke connector operation
- Get Version Information
public function main() { gitlab:VersionResponse|error gitVersion = baseClient->getVersion(); if (gitVersion is gitlab:VersionResponse) { log:printInfo("Git version " + gitVersion?.'version.toString()); } }
- Use
bal run
command to compile and run the Ballerina program
Clients
gitlab: Client
This is a generated connector for GitLab GitLab REST API v4 OpenAPI specification.
Client endpoint for GitLab API currently supports operations related to access requests and access tokens in GitLab.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a GitLab account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://gitlab.com/api/" - URL of the target service
getVersion
function getVersion() returns VersionResponse|error
Retrieve version information for this GitLab instance.
Return Type
- VersionResponse|error - Successful
accessrequestsprojectsGet
function accessrequestsprojectsGet(string id) returns ProjectAccessResponse|error
List access requests for a project
Parameters
- id string - The ID or URL-encoded path of the project owned by the authenticated userr.
Return Type
- ProjectAccessResponse|error - Successful
accessrequestsprojectsPost
function accessrequestsprojectsPost(string id) returns ProjectAccessRequest|error
Requests access for the authenticated user to a project
Parameters
- id string - The ID or URL-encoded path of the project owned by the authenticated user.
Return Type
- ProjectAccessRequest|error - Successful
accessrequestsprojectsapprovePut
function accessrequestsprojectsapprovePut(string id, int userId, int accessLevel) returns ProjectAccessApprove|error
Approves access for the authenticated user to a project
Parameters
- id string - The ID or URL-encoded path of the project owned by the authenticated user.
- userId int - The userID of the access requester
- accessLevel int (default 30) - A valid project access level. 0 = no access , 10 = guest, 20 = reporter, 30 = developer, 40 = Maintainer. Default is 30.'
Return Type
- ProjectAccessApprove|error - Successful
accessrequestprojectsdenyDelete
Denies a project access request for the given user
Parameters
- id string - The ID or URL-encoded path of the project owned by the authenticated user.
- userId int - The user ID of the access requester
accessrequestsgroupsGet
function accessrequestsgroupsGet(string id) returns GroupAccessResponse|error
List access requests for a group
Parameters
- id string - The ID or URL-encoded path of the group owned by the authenticated user.
Return Type
- GroupAccessResponse|error - Successful
accessrequestsgroupsPost
function accessrequestsgroupsPost(string id) returns GroupAccessRequest|error
Requests access for the authenticated user to a group
Parameters
- id string - The ID or URL-encoded path of the group owned by the authenticated user.
Return Type
- GroupAccessRequest|error - Successful
accessrequestsgroupsapprovePut
function accessrequestsgroupsapprovePut(string id, int userId, int accessLevel) returns GroupAccessApprove|error
Approves access for the authenticated user to a group
Parameters
- id string - The ID or URL-encoded path of the group owned by the authenticated user.
- userId int - The userID of the access requester
- accessLevel int (default 30) - A valid group access level. 0 = no access , 10 = Guest, 20 = Reporter, 30 = Developer, 40 = Maintainer, 50 = Owner. Default is 30.
Return Type
- GroupAccessApprove|error - Successful
accessrequestsgroupsdenyDelete
Denies a group access request for the given user
Parameters
- id string - The ID or URL-encoded path of the group owned by the authenticated user.
- userId int - The userID of the access requester
accesstokensGet
function accesstokensGet(string id) returns AccessToken|error
List access tokens for a project
Parameters
- id string - The ID or URL-encoded path of the project
Return Type
- AccessToken|error - Successful
accesstokensPost
function accesstokensPost(string id, string name, string[] scopes, string? expiresAt) returns AccessTokenList|error
Creates an access token for a project
Parameters
- id string - The ID or URL-encoded path of the project
- name string - The name of the project access token
- scopes string[] - Defines read and write permissions for the token
- expiresAt string? (default ()) - Date when the token expires. Time of day is Midnight UTC of that date.
Return Type
- AccessTokenList|error - Successful
accesstokensDelete
Revokes an access token
Records
gitlab: AccessToken
Access token info
Fields
- user_id int? - User ID
- name string? - The name of the project access token
- expires_at string? - The token expires at midnight UTC on that date
- id int? - The ID or URL-encoded path of the project
- active boolean? - If token is active or not
- created_at string? - The token creation date/time
- revoked boolean? - Is the token is revoked
gitlab: AccessTokenList
Access token info
Fields
- user_id int? - User ID
- name string? - The name of the project access token
- expires_at string? - The token expires at midnight UTC on that date
- id int? - The ID or URL-encoded path of the project
- active boolean? - If token is active or not
- created_at string? - The token creation date/time
- revoked boolean? - Is the token is revoked
gitlab: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- privateToken string - Represents API Key
Private-Token
gitlab: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
gitlab: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
gitlab: GroupAccessApprove
Access request info
Fields
- id int? - The ID or URL-encoded path of the project owned by the authenticated user
- username string? - Username
- name string? - Actual name
- state string? - If user is active or not
- created_at string? - Created date/time
- access_level int? - A valid access level (defaults: 30, the Developer role)
gitlab: GroupAccessRequest
Access request info
Fields
- id int? - The ID or URL-encoded path of the project owned by the authenticated user
- username string? - Username
- name string? - Actual name
- state string? - If user is active or not
- created_at string? - Created date/time
- requested_at string? - Requested date/time
gitlab: GroupAccessResponse
Access request info
Fields
- id int? - The ID or URL-encoded path of the project owned by the authenticated user
- username string? - Username
- name string? - Actual name
- state string? - If user is active or not
- created_at string? - Created date/time
- requested_at string? - Requested date/time
gitlab: ProjectAccessApprove
Access request info
Fields
- id int? - The ID or URL-encoded path of the project owned by the authenticated user
- username string? - Username
- name string? - Actual name
- state string? - If user is active or not
- created_at string? - Created date/time
- access_level int? - A valid access level (defaults: 30, the Developer role)
gitlab: ProjectAccessRequest
Access request info
Fields
- id int? - The ID or URL-encoded path of the project owned by the authenticated user
- username string? - Username
- name string? - Actual name
- state string? - If user is active or not
- created_at string? - Created date/time
- requested_at string? - Requested date/time
gitlab: ProjectAccessResponse
Access request info
Fields
- id int? - The ID or URL-encoded path of the project owned by the authenticated user
- username string? - Username
- name string? - Actual name
- state string? - If user is active or not
- created_at string? - Created date/time
- requested_at string? - Requested date/time
gitlab: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
gitlab: VersionResponse
GitLab version info
Fields
- 'version string? - GitLab version
- revision string? - Revision number
Import
import ballerinax/gitlab;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1
Current verison: 1
Weekly downloads
Keywords
IT Operations/Source Control
Cost/Freemium
Contributors
Dependencies