github.webhook
Modules
github.webhook
Module github.webhook
API
Declarations
Definitions
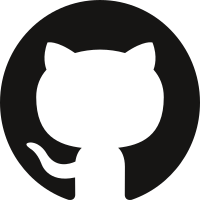
ballerinax/github.webhook Ballerina library
Overview
The GitHub connector Listener module allows you to listen to following events occur in GitHub.
Ping
,Fork
,Push
,Create
,Watch
Release
,Issue
,Label
,Milestone
Pull Request
,Pull Request Review
,Pull Request Review Comment
This module supports GitHub API v4 version and only allows to perform functions behalf of the currently logged in user.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Create account
-
Obtain token - To obtain a personal access token via which the connector can access the API, follow these steps
-
Configure the connector with obtained token
Quickstart
To use the GitHub connector in your Ballerina application, update the .bal file as follows:
Step 1: Import the GitHub Webhook Ballerina library
First, import the ballerinax/github.webhook and ballerina/websub modules into the Ballerina project as follows.
import ballerina/websub; import ballerinax/github.webhook as github;
Step 2: Initialize the GitHub Webhook Listener
Initialize the Webhook Listener by providing the port number.
listener github:Listener webhookListener = new (9090);
Step 3: Annotate the service with websub:SubscriberServiceConfig
Annotate the service with websub:SubscriberServiceConfig by providing necessary properties as follows.
@websub:SubscriberServiceConfig { target: [github:HUB, githubTopic], secret: githubSecret, callback: githubCallback, httpConfig: { auth: { token: accessToken } } } service /subscriber on webhookListener { }
Step 4: Provide remote functions corresponding to the events which you are interested on
The remote functions can be provided as follows.
remote function onPush(github:PushEvent event) returns github:Acknowledgement? { log:printInfo("Received push-event-message ", eventPayload = event); }
Step 5: Run the service
Use bal run
command to compile and run the Ballerina program.
Constants
Enums
github.webhook: IssueCommentActions
Represent issue comment event action types.
Members
github.webhook: IssuesActions
Represent issue event action types.
Members
github.webhook: LabelActions
Represent label event action types.
Members
github.webhook: MilestoneActions
Represent milestone event action types.
Members
github.webhook: PullRequestActions
Represent pull request event action types.
Members
github.webhook: PullRequestReviewActions
Represent pull request review event action types.
Members
github.webhook: PullRequestReviewCommentActions
Represent pull request review comment event action types.
Members
github.webhook: ReleaseActions
Represent release event action types.
Members
github.webhook: WatchActions
Represent watch event action types.
Members
Listeners
github.webhook: Listener
Listener for GitHub connector
Constructor
Initializes GitHub connector listener.
init (int|Listener listenTo, ListenerConfiguration? config)
- config ListenerConfiguration? () - Configurations for configure the underlying HTTP listener of the WebSub listener.
attach
function attach(SimpleWebhookService s, string[]|string? name) returns error?
detach
function detach(SimpleWebhookService s) returns error?
Parameters
'start
function 'start() returns error?
gracefulStop
function gracefulStop() returns error?
immediateStop
function immediateStop() returns error?
Records
github.webhook: Acknowledgement
Represent webhook acknowledgement
Fields
github.webhook: Asset
Represent GitHub Asset payload.
Fields
- url string - Asset URL
- browser_download_url string - Download URL for assets
- id int - Asset ID
- node_id string - Node ID
- name string - Name of the Asset
- label string - Label name
- state string - State of the assets
- content_type string - Content-Type of the Asset
- size int - Size of the asset
- download_count int - Downloaded count
- created_at string - Created date time
- updated_at string - Updated date time
- uploader User - Uploaded user
github.webhook: Body
Represents body of a Change occured
Fields
- 'from string -
github.webhook: Branch
Represent the payload of GitHub Branch
Fields
- label string - Label name
- ref string - Reference link
- sha string - Sha of the branch
- user User - Auther of the branch
- repo Repository - Repository name
github.webhook: Changes
Represents the Change payload.
Fields
- name Name? - Name of the Change
- title Title? - Title of the Change
- body Body? - Body of the Change
- color Color? - Color of the Change
- permission Permission? - Permission of the Change
- description Description? - Description of the Change
- due_on DueOn? - Due on date time
- note Note? - Notes of the Change
github.webhook: Color
Represents color of a Change occured
Fields
- 'from string -
github.webhook: CommentsLink
Represent comments link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: Commit
Represent GitHub commit payload.
Fields
- id string? - ID
- sha string? - Commit sha
- message string - Commit message
- author CommitAuthor - Author of the commit
- url string - Commit URL
- 'distinct boolean -
- committer CommitAuthor? - Commit author
- tree_id string? - Tree Id
- timestamp string? - Timestamp of the commit
- added string[]? - Added changes
- removed string[]? - Removed parts
- modified string[]? - Modified parts
github.webhook: CommitAuthor
Represent payload of GitHub commit author.
Fields
- name string - Name of the commit author
- email string - Email of the commit author
- username string? - GitHub username of the commit author
github.webhook: CommitsLink
Represent commits link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: CreateEvent
Represent GitHub create event.
Fields
- ref string - The git ref resource.
- ref_type string - The type of Git ref object created in the repository. Can be either branch or tag.
- master_branch string - The name of the repository's default branch (usually main).
- description string - The repository's current description.
- repository Repository - The repository where the event occurred.
- organization Organization? - Webhook payloads contain the organization object when the webhook is configured for an organization or the event occurs from activity in a repository owned by an organization.
- sender User - The user that triggered the event.
github.webhook: Description
Represents description of a Change occured
Fields
- 'from string -
github.webhook: DueOn
Represents due on of a Change occured
Fields
- 'from string -
github.webhook: EventNotification
Represent event notification payload.
Fields
- hubName string - Hub name
- eventId string - Event Id
- message string - Message
github.webhook: ForkEvent
Represent GitHub fork event.
Fields
- forkee Repository - Forkee repository
- repository Repository - Fork repository
- sender User - Fork send user
github.webhook: Hook
Represent GitHub Hook payload.
Fields
- 'type string -
- id int - ID
- name string - Name of the Hook
- active boolean - Whether the Hook is active
- events string[] - Events associated with the Hook
- config HookConfig - Hook configuration
- updated_at string - Updated date time
- created_at string - Created date time
- url string - Hook URL
- test_url string - test URL
- ping_url string - Ping URL
- last_response HookLastResponse - Last Hook response
github.webhook: HookConfig
Represent GitHub Hook config payload.
Fields
- content_type string - Content Type of the Hook request
- secret string? - Hook secret
- url string - Hook registration URL
- insecure_ssl string - Insecure SSL
github.webhook: HookLastResponse
Represent GitHub Hook last response.
Fields
- code string - Code associated with HookLastResponse
- status string - Hook status
- message string - Hook message
github.webhook: HtmlLink
Represent html link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: Invitation
Represent the payload of GitHub Invitation
Fields
- id int - ID
- login string - Login name for Invitation
- email string - Email of the Invitation
- role string - Role of the Invitation
- created_at string - Created date time
- inviter User - Inviter
- team_count int - Team count
- invitation_team_url string - Invitation team url
github.webhook: Issue
Represents the GitHub Issue payload.
Fields
- url string - Url of the payload
- repository_url string - Repository URl
- labels_url string - Labels URl
- comments_url string - Comments URl
- events_url string - Events URL
- html_url string - HTML URL
- id int - ID
- node_id string - Node ID
- number int - Issue number
- title string - Issue title
- user User - Author of the issue
- labels Label[] - Labels assigned to the issue
- state string - State of the issue.
- locked boolean - Whether the issue is locked
- assignee User - Assigne of the issue.
- assignees User[] - Assignes of the issue.
- milestone Milestone - Milestone of the issue
- comments int - Comments for the issue
- created_at string - Created at date time
- updated_at string - Updated date time
- closed_at string - Closed date time
- author_association string - Author associations
- active_lock_reason string - Active lock reasons
- body string - Description of the Issue
- performed_via_github_app string -
github.webhook: IssueComment
Represents the GitHub issue comment payload
Fields
- url string - Issue comment url
- html_url string - HTML url for the issue comment
- issue_url string - Issue URL
- id int - ID
- node_id string - Node ID
- user User - Author of the comment
- created_at string - Created date time
- updated_at string - Updated date time
- author_association string - Author association
- body string - Description of the issue comment
- performed_via_github_app string - Whether performed via GitHub Apps
github.webhook: IssueCommentEvent
Repesent GitHub issue comment event.
Fields
- action IssueCommentActions - Issue comment event action
- issue Issue - Associated issue.
- changes Changes? - Changes associated to the Issue comment
- comment IssueComment - Comment
- repository Repository - Issue comment associated repository
- sender User - Issue comment send user
github.webhook: IssueLink
Represent issue link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: IssuesEvent
Represent GitHub issue event.
Fields
- action IssuesActions - Issue event action
- issue Issue - Issue associated
- changes Changes? - Changes associated with the issue
- label Label? - Label of the issue event
- assignee User? - assignee of the issue event
- milestone Milestone? - Milestone associated with
- repository Repository - Repository of the issue events
- sender User - User associated with issue event
github.webhook: Label
Represents the GitHub Label payload.
Fields
- id int - Label ID
- node_id string - Node ID of the label
- url string - Label URL
- name string - Name of the label
- color string - Color of the label
- description string - Description of the label
- 'default boolean -
github.webhook: LabelEvent
Repesent GitHub label event.
Fields
- action LabelActions - Label event action
- label Label - Label payload
- issue Issue? - Issue associated with
- changes Changes? - Changes associated with
- repository Repository - Repository associated with
- sender User - Sender of the label event
github.webhook: License
Represent repository licence
Fields
- key string - License key
- name string - License name
- spdx_id string - Short identifier specified by https://spdx.org/licenses
- url string - URL to the license on https://choosealicense.com
- node_id string - GItHub node ID
github.webhook: Links
Represent the payload of Github Links
Fields
- 'self SelfLink? -
- html HtmlLink? - Html Link
- issue IssueLink? - Issue link
- comments CommentsLink? - Comments link
- review_comments ReviewCommentsLink? - Review comments link
- review_comment ReviewCommentLink? - Review comment
- commits CommitsLink? - Commits
- statuses StatusesLink? - Statuses
- pull_request PullRequestLink? - Pull request
github.webhook: Milestone
Represents the GitHub Milestone payload.
Fields
- url string - Milestone URL
- html_url string - HTML URL
- labels_url string - Lables URL
- id int - ID
- node_id string - Node ID
- number int - Milestone number
- title string - Title of the milestone
- description string - Description of the milestone
- creator User - Author of the milestone
- open_issues int - Number of open issues
- closed_issues int - Number of closed issues
- state string - State of the milestone
- created_at string - Created date time
- updated_at string - Updated date time
- due_on string - Due of date time
- closed_at string - Closed date time
github.webhook: MilestoneEvent
Represent GitHub milestone event.
Fields
- action MilestoneActions - Milestone event action
- milestone Milestone - Milestone payload
- changes Changes? - Changes associated with
- repository Repository - Repository associated with
- sender User - Sender of the label event
github.webhook: Name
Represents name of a Change occured
Fields
- 'from string -
github.webhook: Note
Represents note of a Change occured
Fields
- 'from string -
github.webhook: Organization
Represents the payload of an Organization
Fields
- login string - Login name of the Organization
- id int - ID of the organization
- node_id string - Node ID
- url string - URL of the organization
- name string - Name of the organization
- repos_url string - Reposiotries URL
- events_url string - Events URL
- hooks_url string - Hooks URL
- issues_url string - Issues URL
- members_url string - Members URl
- public_members_url string - Public members URl
- avatar_url string - Avatar URl
- description string - Description URL
github.webhook: Page
Represents the Page payload.
Fields
- page_name string - Name of the page
- title string - Title of the page
- summary string - Summery
- action string - Action
- sha string - Sha of the page
- html_url string - HTML URL of the page
github.webhook: Payload
Represent event Payload
Fields
- eventType string - Event type.
- eventData json - Event data.
github.webhook: Permission
Represents permission of a Change occured
Fields
- 'from string -
github.webhook: PingEvent
Represent GitHub ping event.
Fields
- zen string - Random string of GitHub zen.
- hook_id int - The ID of the webhook that triggered the ping.
- hook Hook - The webhook configuration.
- repository Repository - The repository where the event occurred.
- sender User - The user that triggered the event.
github.webhook: PullRequest
Represent payload of GitHub payload.
Fields
- url string - Pull request URL
- id int - ID
- node_id string - Node ID
- html_url string - Html Url
- diff_url string - Diff URL
- patch_url string - Patch URl
- issue_url string - Issues URL
- number int - Pull Request number
- state string - State of the PR
- locked boolean - Whether merge locked
- title string - Title of the Pull Request
- user User - Auther of the Pull Request
- body string - Body of the pull request
- created_at string - Created date time
- updated_at string - Updated date time
- closed_at string - Closed date time
- merged_at string - Merged date time
- merge_commit_sha string - Merge commit sha
- assignee User - Assignee of the PR
- assignees User[] - Assignees of the PR
- requested_reviewers User[] - Requested reviewers list
- requested_teams Team[] - Requested Items
- labels Label[] - Labels list
- milestone Milestone - Milestone of the PR
- draft boolean - Whether the PR is a draft
- commits_url string - Commits URL of the PR
- review_comments_url string - Revew comments URL of the PR
- review_comment_url string - Review comment URL
- comments_url string - Comments URL
- statuses_url string - Statuses URL
- head Branch - Head ref of the PR
- base Branch - base Ref of the PR
- _links Links - Links of the Pr
- author_association string - Author association
- merged boolean? - Whether the PR is merged
- mergeable boolean? - Whether the PR is mergeable
- rebaseable boolean? - Whether the PR is rebaseble
- mergeable_state string? - Mergeable state of the PR
- merged_by User? - Merged User
- comments int? - List of comments
- review_comments int? - Review comments
- maintainer_can_modify boolean? - Whether maintainer can modify the PR
- commits int? - Commits
- additions int? - Addition changes
- deletions int? - Deletion changes
- changed_files int? - Change files references
- active_lock_reason string - Active lock reason
github.webhook: PullRequestEvent
Represent GitHub Pull request event
Fields
- action PullRequestActions - Pull Request Event action
- number int - Pull request number
- changes Changes? - Changes associated with
- pull_request PullRequest - Pull Request payload
- assignee User? - Associated assignee to the Pull Request
- label Label? - Labels associated with
- requested_reviewer User? - Requested reviewer
- repository Repository - Repository associated with
- sender User - Pull request sender
github.webhook: PullRequestLink
Represent pull request link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: PullRequestReviewComment
Represent GitHub pull request review comment payload.
Fields
- url string - URL of the pull request review commment
- pull_request_review_id int - Pull Request review id
- id int - ID
- node_id string - Node ID
- diff_hunk string - Diff hunk of the PR review
- path string - Path for the Pull Request Review Comment
- position int - Position of the Review
- original_position int - Original posiiton of the Change
- commit_id string - Commit ID of the PR
- original_commit_id string - Original commit id
- user User - Author of the Pull Request Review
- body string - Body of the Pull Request Review
- created_at string - Created date time
- updated_at string - Updated date time
- html_url string - HTML url for the review
- pull_request_url string - URL for the Pull Request
- author_association string - Author association
- _links Links - Links associates
- start_line string - Start line number of the review
- original_start_line string - Original start line
- start_side string - Start side of the review
- line int - Line number
- original_line int - Original line
- side string - Side of the Pull Request Review
- in_reply_to_id int? - Reply to the PR ID
github.webhook: PullRequestReviewCommentEvent
Represent GitHub pull request review comment event.
Fields
- action string - Pull request review comment event action
- changes Changes? - Changes associated with
- pull_request PullRequest - Pull request associated with
- comment PullRequestReviewComment - Comments associated with
- repository Repository - Repository associated with
- sender User - Pull request review comment sender
github.webhook: PullRequestReviewEvent
Represent GitHub pull request review event.
Fields
- action PullRequestReviewActions - Pull Request Review Event action
- review Review - Pull Request Review payload.
- pull_request PullRequest - Pull Request associated with
- changes Changes? - Changes associated with
- repository Repository - Repository associated with
- sender User - Sender associated with
github.webhook: PushEvent
Represent GitHub push event
Fields
- ref string - The full git ref that was pushed. Example:
- before string - The SHA of the most recent commit on ref before the push.
- after string - The SHA of the most recent commit on ref after the push.
- created boolean - Created date time
- deleted boolean - Deleted date time
- forced boolean - Forced psuh
- base_ref string - Base git ref
- compare string - Comparison
- commits Commit[] - Array of commits
- head_commit Commit - Head ref commit
- repository Repository - The repository where the event occurred.
- pusher CommitAuthor - The user who pushed the commits.
- sender User - The user that triggered the event.
github.webhook: Release
Represent GitHub Release payload.
Fields
- url string - Release URL
- assets_url string - Assets URL
- upload_url string - Upload URL
- html_url string - HTML URL
- id int - ID
- node_id string - Node ID
- tag_name string - Tag name
- target_commitish string - Target commitsh
- name string - Name of the release
- draft boolean - Whether release is in draft state
- author User - Author of the release
- prerelease boolean - Whether release is a pre-release
- created_at string - Created date time
- published_at string - Published date time
- assets Asset[] - Release artifacts
- tarball_url string - Tarball URL
- zipball_url string - Zipball URL
- body string - Release body
github.webhook: ReleaseEvent
Represent GitHub release event.
Fields
- action ReleaseActions - The action that was performed.
- release Release - The release object.
- repository Repository - The repository where the event occurred.
- sender User - The user that triggered the event.
- changes Changes? - The previous version of the body if the action was edited.
github.webhook: Repository
Represent the GitHub Repository payload.
Fields
- id int - ID o fthe Repository
- node_id string - Node ID of the repository
- name string - Name of the Repository
- full_name string - Full name of the Repository
- owner User - Owner of the repository
- 'private boolean -
- html_url string - HTML URL of the repository.
- description string - Description of the repository.
- 'fork boolean -
- url string - URL of the repository
- forks_url string - Fork URL of the repository.
- keys_url string - Keys URl of the repository
- collaborators_url string - Collaborators URL of the repository
- teams_url string - Teams URL of the repository
- hooks_url string - Hooks URL
- issue_events_url string - Issue events URL
- events_url string - Events URl
- assignees_url string - Assignees URL
- branches_url string - Branches URL
- tags_url string - Tags URL
- blobs_url string - Blobs URL
- git_tags_url string - Git Tags URL
- git_refs_url string - Git Refs URL
- trees_url string - Trees URL
- statuses_url string - Statuses URL
- languages_url string - Languages URL.
- stargazers_url string - Stargazers URL
- contributors_url string - Contributors URL
- subscribers_url string - Subscribers URL
- subscription_url string - Subscription URL.
- commits_url string - Commits URL
- git_commits_url string - Git Commits URL
- comments_url string - Comments URL
- issue_comment_url string - Issue Comments URL
- contents_url string - Contents URL
- compare_url string - Compare URL
- merges_url string - Merges URL
- archive_url string - Archieve URL
- downloads_url string - Downloads URL
- issues_url string - Issues URL
- pulls_url string - Pulls URL
- milestones_url string - Milestone URLs
- notifications_url string - Notification URLs
- labels_url string - Labels URLs
- releases_url string - Releases URL
- updated_at string - Updated time
- git_url string - Git URL
- ssh_url string - SSH URL
- clone_url string - Clone URL
- svn_url string - SVN URL
- homepage string - Home page URL
- size int - Size of the repository
- stargazers_count int - Stargazers count
- watchers_count int - Watchers count
- language string - Language
- has_issues boolean - Whether issues are available
- has_downloads boolean - Whether downloads are available
- has_wiki boolean - Wther Wikis are available
- has_pages boolean - Whether GitHub pages available
- forks_count int - Number of forks
- mirror_url string - Mirror URl
- deployments_url string? - Deployment URL
- open_issues_count int - Open issues count
- license License - Licence URl
- forks int - Forks
- open_issues int - Open Issues
- watchers int - Watches
- default_branch string - Default branch
- archived boolean? - Whether archieved
- disabled boolean? - Whether disabled
- has_projects boolean? - Whether includes projects
- allow_squash_merge boolean? - Whether allow squash and merge
- allow_merge_commit boolean? - Whether allow merge commits
- allow_rebase_merge boolean? - Whether allow rebase merge
- delete_branch_on_merge boolean? - Whether delete branch on merge
- stargazers int? - Stargazers
- master_branch string? - Refrence to master branch
github.webhook: Review
Represents GitHub Review payload.
Fields
- id int - ID of the Review
- node_id string - Node ID
- user User - Auther of the review
- body string - Body of the review
- commit_id string - Commit
- submitted_at string - Submitted date time
- state string - State of the review
- html_url string - HTML of the Review
- pull_request_url string - Pull request URL
- author_association string - Author association
- _links Links - Links associations
github.webhook: ReviewCommentLink
Represent a review comment link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: ReviewCommentsLink
Represent review comments link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: SelfLink
Represent self link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: StartupMessage
Represent event startup message.
Fields
- hubName string - Hub name
- subscriberId string - Subscriber Id
github.webhook: StatusesLink
Represent statuses link of GitHub Link
Fields
- href string - Hyper-reference of the Link
github.webhook: Team
Represent Team payload.
Fields
- name string - Name of the Team
- id int - ID
- node_id string - Node ID
- slug string - Slug of the Team
- description string - Description of the Team
- privacy string - Privacy link of the Team
- url string - URL of the Team
- members_url string - Members URL of the team
- repositories_url string - Repositories URL of the Team
- permission string - Permissions of the Team
github.webhook: Title
Represents the title of a Change
Fields
- 'from string -
github.webhook: User
Represent the GitHub User payload.
Fields
- login string - The username used to login.
- id int - User ID
- node_id string? - Node ID
- avatar_url string - A URL pointing to the user's public avatar.
- gravatar_id string - Graveter ID
- url string - The HTTP URL for this user
- html_url string - HTML url
- followers_url string - Followers URL
- following_url string - Following URL
- gists_url string - Gists URL
- starred_url string - Starred URL
- subscriptions_url string - Subscription URL
- organizations_url string - Organization URL
- repos_url string - Repositories URL
- events_url string - Events URL
- received_events_url string - Received events URL.
- 'type string -
- site_admin boolean - is the user is Site Admin
- name string? - Name of the user
- email string? - Email of the user.
github.webhook: WatchEvent
Represent GitHub watch event.
Fields
- action WatchActions - The action that was performed. Currently, can only be started.
- repository Repository - The repository where the event occurred.
- sender User - The user that triggered the event.
Errors
Object types
github.webhook: SimpleWebhookService
Union types
github.webhook: GitHubEvent
GitHubEvent
Represent all events from GitHub.
Import
import ballerinax/github.webhook;
Metadata
Released date: almost 3 years ago
Version: 3.0.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: slbeta6
Pull count
Total: 17382
Current verison: 63
Weekly downloads
Keywords
IT Operations/Source Control
Cost/Freemium
Contributors