giphy
Module giphy
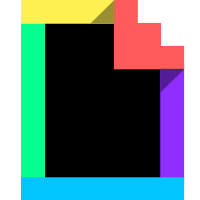
ballerinax/giphy Ballerina library
Overview
This is a generated connector for Giphy API v1 OpenAPI Specification.
Giphy API provides the capability to access GIFs and stickers.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Giphy account
- Obtain tokens - Follow this guide
Quickstart
To use the Giphy connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/giphy module into the Ballerina project.
import ballerinax/giphy;
Step 2: Create a new connector instance
Create a giphy:ApiKeysConfig
with the tokens obtained, and initialize the connector with it.
giphy:ApiKeysConfig configuration = <API_KEY_PAIR> giphy:Client giphyClient = check new(configuration);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get a random GIF using the connector.public function main() returns error? { giphy:Response entity = check giphyClient->randomGif(); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
giphy: Client
This is a generated connector for Giphy API v1 OpenAPI Specification. Giphy API provides functions to get GIFs and stickers.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Giphy account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.giphy.com/v1" - URL of the target service
getGifsById
function getGifsById(string? ids) returns PaginatedResponse|error
Get GIFs by ID
Parameters
- ids string? (default ()) - Filters results by specified GIF IDs, separated by commas.
Return Type
- PaginatedResponse|error - GIF or an error
randomGif
Random GIF
Parameters
- tag string? (default ()) - Filters results by specified tag.
- rating string? (default ()) - Filters results by specified rating.
searchGifs
function searchGifs(string q, int 'limit, int offset, string? rating, string? lang) returns PaginatedResponse|error
Search GIFs
Parameters
- q string - Search query term or prhase.
- 'limit int (default 25) - The maximum number of records to return.
- offset int (default 0) - An optional results offset.
- rating string? (default ()) - Filters results by specified rating.
- lang string? (default ()) - Specify default language for regional content; use a 2-letter ISO 639-1 language code.
Return Type
- PaginatedResponse|error - GIFs or an error
translateGif
Translate phrase to GIF
Parameters
- s string - Search term.
trendingGifs
function trendingGifs(int 'limit, int offset, string? rating) returns PaginatedResponse|error
Trending GIFs
Parameters
- 'limit int (default 25) - The maximum number of records to return.
- offset int (default 0) - An optional results offset.
- rating string? (default ()) - Filters results by specified rating.
Return Type
- PaginatedResponse|error - Treading GIFs or an error
getGifById
Get GIF by Id
Parameters
- gifId int - Filters results by specified GIF ID.
randomSticker
Random Sticker
Parameters
- tag string? (default ()) - Filters results by specified tag.
- rating string? (default ()) - Filters results by specified rating.
searchStickers
function searchStickers(string q, int 'limit, int offset, string? rating, string? lang) returns PaginatedResponse|error
Search Stickers
Parameters
- q string - Search query term or prhase.
- 'limit int (default 25) - The maximum number of records to return.
- offset int (default 0) - An optional results offset.
- rating string? (default ()) - Filters results by specified rating.
- lang string? (default ()) - Specify default language for regional content; use a 2-letter ISO 639-1 language code.
Return Type
- PaginatedResponse|error - Stickers or an error
translateSticker
Translate phrase to Sticker
Parameters
- s string - Search term.
trendingStickers
function trendingStickers(int 'limit, int offset, string? rating) returns PaginatedResponse|error
Trending Stickers
Parameters
- 'limit int (default 25) - The maximum number of records to return.
- offset int (default 0) - An optional results offset.
- rating string? (default ()) - Filters results by specified rating.
Return Type
- PaginatedResponse|error - Treading stickers or an error
Records
giphy: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apiKey string -
giphy: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
giphy: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
giphy: Gif
Fields
- bitly_url string? - The unique bit.ly URL for this GIF
- content_url string? - Currently unused
- create_datetime string? - The date this GIF was added to the GIPHY database.
- embded_url string? - A URL used for embedding this GIF
- featured_tags string[]? - An array of featured tags for this GIF (Note: Not available when using the Public Beta Key)
- id string? - This GIF's unique ID
- images GifImages? -
- import_datetime string? - The creation or upload date from this GIF's source.
- rating string? - The MPAA-style rating for this content. Examples include Y, G, PG, PG-13 and R
- slug string? - The unique slug used in this GIF's URL
- 'source string? - The page on which this GIF was found
- source_post_url string? - The URL of the webpage on which this GIF was found.
- source_tld string? - The top level domain of the source URL.
- tags string[]? - An array of tags for this GIF (Note: Not available when using the Public Beta Key)
- trending_datetime string? - The date on which this gif was marked trending, if applicable.
- 'type string? - Type of the gif. By default, this is almost always gif
- update_datetime string? - The date on which this GIF was last updated.
- url string? - The unique URL for this GIF
- user User? - The User Object contains information about the user associated with a GIF and URLs to assets such as that user's avatar image, profile, and more.
- username string? - The username this GIF is attached to, if applicable
giphy: GifImages
Fields
- downsized record {}? -
- downsized_large record {}? -
- downsized_medium record {}? -
- downsized_small record {}? -
- downsized_still record {}? -
- fixed_height record {}? -
- fixed_height_downsampled record {}? -
- fixed_height_small record {}? -
- fixed_height_small_still record {}? -
- fixed_height_still record {}? -
- fixed_width record {}? -
- fixed_width_downsampled record {}? -
- fixed_width_small record {}? -
- fixed_width_small_still record {}? -
- fixed_width_still record {}? -
- looping record {}? -
- original record {}? -
- original_still record {}? -
- preview record {}? -
- preview_gif record {}? -
giphy: Image
Fields
- frames string? - The number of frames in this GIF.
- height string? - The height of this GIF in pixels.
- mp4 string? - The URL for this GIF in .MP4 format.
- mp4_size string? - The size in bytes of the .MP4 file corresponding to this GIF.
- size string? - The size of this GIF in bytes.
- url string? - The publicly-accessible direct URL for this GIF.
- webp string? - The URL for this GIF in .webp format.
- webp_size string? - The size in bytes of the .webp file corresponding to this GIF.
- width string? - The width of this GIF in pixels.
giphy: Meta
The Meta Object contains basic information regarding the request, whether it was successful, and the response given by the API. Check responses
to see a description of types of response codes the API might give you under different cirumstances.
Fields
- msg string? - HTTP Response Message
- response_id string? - A unique ID paired with this response from the API.
- status int? - HTTP Response Code
giphy: PaginatedResponse
Fields
- data Gif[]? -
- meta Meta? - The Meta Object contains basic information regarding the request, whether it was successful, and the response given by the API. Check
responses
to see a description of types of response codes the API might give you under different cirumstances.
- pagination Pagination? - The Pagination Object contains information relating to the number of total results available as well as the number of results fetched and their relative positions.
giphy: Pagination
The Pagination Object contains information relating to the number of total results available as well as the number of results fetched and their relative positions.
Fields
- count int? - Total number of items returned.
- offset int? - Position in pagination.
- total_count int? - Total number of items available.
giphy: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
giphy: Response
Fields
- data Gif? -
- meta Meta? - The Meta Object contains basic information regarding the request, whether it was successful, and the response given by the API. Check
responses
to see a description of types of response codes the API might give you under different cirumstances.
giphy: User
The User Object contains information about the user associated with a GIF and URLs to assets such as that user's avatar image, profile, and more.
Fields
- avatar_url string? - The URL for this user's avatar image.
- banner_url string? - The URL for the banner image that appears atop this user's profile page.
- display_name string? - The display name associated with this user (contains formatting the base username might not).
- profile_url string? - The URL for this user's profile.
- twitter string? - The Twitter username associated with this user, if applicable.
- username string? - The username associated with this user.
Import
import ballerinax/giphy;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Content & Files/Images & Design
Cost/Free
Contributors
Dependencies