geonames
Module geonames
API
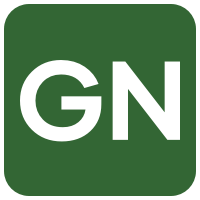
ballerinax/geonames Ballerina library
Overview
This is a generated connector for Geonames API OpenAPI specification. The Geonames API allows you to retrieve information about geo locations.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Geonames Account
- Obtaining tokens by following this link
Quickstart
To use the Geonames connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/geonames module into the Ballerina project.
import ballerinax/geonames;
Step 2: Create a new connector instance
You can use your organization name for authentication:
geonames:ApiKeysConfig apiKeyConfig = { username: <USERNAME> }; geonames:Client baseClient = check new Client(apiKeyConfig);
Step 3: Invoke connector operation
- You can search about a term.
geonames:SearchResponse score = check baseClient->search("sanfrancisco");
- Use
bal run
command to compile and run the Ballerina program.
Clients
geonames: Client
This is a generated connector for Geonames API OpenAPI specification. Geonames is a geographical database where users can retrieve geo location information.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Geonames account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "http://api.geonames.org/" - URL of the target service
search
function search(string? q, string? name, string? nameEquals, string? nameStartswith, int? maxRows, int? startRow, string? country, string? countryBias, string? continentCode, int? cities, string? operator, string? charset, string? searchlang, decimal? east, decimal? west, decimal? north, decimal? south) returns SearchResponse|error
Retrieves the names found for the search term as a XML or JSON document.
Parameters
- q string? (default ()) - The query to search over all attributes of a place.
- name string? (default ()) - The place name.
- nameEquals string? (default ()) - The exact place name.
- nameStartswith string? (default ()) - The place name starts with given characters.
- maxRows int? (default ()) - The maximal number of rows in the document returned by the service.
- startRow int? (default ()) - The starting row for the result.
- country string? (default ()) - The country codes to filter the results.
- countryBias string? (default ()) - The two letter country code to filter the results.
- continentCode string? (default ()) - The continent code to filter the results.
- cities int? (default ()) - The city population category.
- operator string? (default ()) - The the operator 'AND' searches for all terms in the placename parameter, the operator 'OR' searches for any term, default = AND.
- charset string? (default ()) - The encoding used for the document returned.
- searchlang string? (default ()) - The search will only consider names in the specified language
- east decimal? (default ()) - The bounding box, only features within the box are returned.
- west decimal? (default ()) - The bounding box, only features within the box are returned.
- north decimal? (default ()) - The bounding box, only features within the box are returned.
- south decimal? (default ()) - The bounding box, only features within the box are returned.
Return Type
- SearchResponse|error - The names found for the searchterm
searchPostalCode
function searchPostalCode(string? postalcode, string? placeName, string? postalcodeStartswith, string? placenameStartswith, string? country, string? countryBias, int? maxRows, string? style, string? operator, string? charset, string? isReduced, decimal? east, decimal? west, decimal? north, decimal? south) returns PostalCodeResponse|error
Retrieves a list of postal codes and places for the place name/postal code query.
Parameters
- postalcode string? (default ()) - The query to search over all attributes of a place. Either postal code or place name must be given.
- placeName string? (default ()) - Place name. Either postal code or place name must be given.
- postalcodeStartswith string? (default ()) - Postal code starts with
- placenameStartswith string? (default ()) - Place name starts with
- country string? (default ()) - Default is all countries. The country parameter may occur more than once,country=FR,country=GP.
- countryBias string? (default ()) - Records from the countryBias are listed first.
- maxRows int? (default ()) - The maximal number of rows in the document returned by the service.
- style string? (default ()) - Style
- operator string? (default ()) - Operator
- charset string? (default ()) - Charset
- isReduced string? (default ()) - Is reduced
- east decimal? (default ()) - The east value for bounding box.
- west decimal? (default ()) - The west value for bounding box.
- north decimal? (default ()) - The north value for bounding box.
- south decimal? (default ()) - The south value for bounding box.
Return Type
- PostalCodeResponse|error - List of postalcodes and places.
getTimezone
Timezone
Parameters
- lat decimal - The latitude to retrieve nearby postal codes.
- lng decimal - The longitude to retrieve nearby postal codes.
- lang string? (default ()) - The language which place name and country name will be returned.
postalCodeLookup
function postalCodeLookup(int? postalcode, string? country, int? maxRows) returns PostalCodes|error
Retrieves a list of places for the given postal code.
Parameters
- postalcode int? (default ()) - Postal code
- country string? (default ()) - Country
- maxRows int? (default ()) - The maximal number of rows in the document returned by the service.
Return Type
- PostalCodes|error - The timezone at the lat/lng
findNearbyPostalCodes
function findNearbyPostalCodes(int postalcode, string country, int? radius, int? maxRows) returns PostalCodes|error
Retrieving a list of postal codes and places for the latitude/longitude query.
Parameters
- postalcode int - Postal code
- country string - Country
- radius int? (default ()) - Radius
- maxRows int? (default ()) - The maximal number of rows in the document returned by the service.
Return Type
- PostalCodes|error - The timezone at the lat/lng
findNearByWeather
function findNearByWeather(decimal lat, decimal lng, int? radius) returns WeatherObservationResponse|error
Retrieves a weather station with the most recent weather observation.
Parameters
- lat decimal - The latitude to retrieve nearby postal codes.
- lng decimal - The longitude to retrieve nearby postal codes.
- radius int? (default ()) - Radius
Return Type
- WeatherObservationResponse|error - The timezone at the lat/lng
listWeatherStations
function listWeatherStations(decimal north, decimal south, decimal east, decimal west, int? maxRows) returns WeatherResponse|error
Retrieves a list of weather stations with the most recent weather observation.
Parameters
- north decimal - The north value for bounding box.
- south decimal - The south value for bounding box.
- east decimal - The east value for bounding box.
- west decimal - The west value for bounding box.
- maxRows int? (default ()) - The maximal number of rows in the document returned by the service.
Return Type
- WeatherResponse|error - Returns a list of weather stations with the most recent weather observation
listEarthquakes
function listEarthquakes(decimal north, decimal south, decimal east, decimal west, int? maxRows, decimal? minMagnitude, string? date) returns EarthquakeResponse|error
Retrieves a list of weather stations with the most recent weather observation.
Parameters
- north decimal - The north value for bounding box
- south decimal - The south value for bounding box
- east decimal - The east value for bounding box.
- west decimal - The west value for bounding box
- maxRows int? (default ()) - The maximal number of rows in the document returned by the service.
- minMagnitude decimal? (default ()) - Minimum magnitude
- date string? (default ()) - Date
Return Type
- EarthquakeResponse|error - Returns a list of earthquakes, ordered by magnitude
Records
geonames: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- username string - Represents API Key
username
geonames: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
geonames: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
geonames: Earthquake
Fields
- datetime string? -
- depth decimal? -
- lng decimal? -
- src string? -
- eqid string? -
- magnitude decimal? -
- lat decimal? -
geonames: EarthquakeResponse
Fields
- earthquakes Earthquake[]? -
geonames: GeonamesInner
Fields
- ing string? -
- geonameid int? -
- countrycode string? -
- name string? -
- fclName string? -
- toponymName string? -
- fcodeName string? -
- wikipedia string? -
- lat string? -
- fcl string? -
- population int? -
- fcode string? -
geonames: PostalCode
Fields
- adminCode1 string? -
- adminCode2 string? -
- adminCode3 string? -
- adminName1 string? -
- adminName2 string? -
- adminName3 string? -
- countryCode string? -
- distance string? -
- lat decimal? -
- lng decimal? -
- placeName string? -
- postalCode string? -
geonames: PostalCodeResponse
Fields
- geonames Geonames? -
geonames: PostalCodes
Fields
- postalcodes PostalCode[]? -
geonames: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
geonames: SearchResponse
Fields
- totalResultsCount int? -
- geonames Geonames? -
geonames: Timezone
Fields
- sunrise string? -
- lng decimal? -
- countryCode string? -
- gmtOffset int? -
- rawOffset int? -
- sunset string? -
- timezoneId string? -
- dstOffset int? -
- countryName string? -
- time string? -
- lat decimal? -
geonames: WeatherObservation
Fields
- clouds string? -
- cloudsCode string? -
- countrycode string? -
- datetime string? -
- dewPoint string? -
- elevation decimal? -
- hectoPascAltimeter decimal? -
- humidity decimal? -
- ICAO string? -
- lat decimal? -
- ing decimal? -
- observation string? -
- seaLevelPressure decimal? -
- stationName string? -
- temperature string? -
- weatherCondition string? -
- windSpeed string? -
- windDirection int? -
geonames: WeatherObservationResponse
Fields
- WeatherObservation WeatherObservation? -
Import
import ballerinax/geonames;
Metadata
Released date: about 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
IT Operations/Geographic Information Systems
Cost/Freemium
Contributors
Dependencies