freshbooks
Module freshbooks
API
Definitions
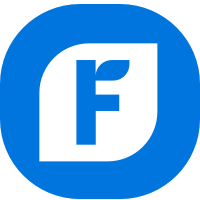
ballerinax/freshbooks Ballerina library
Overview
This is a generated connector for FreshBooks API v1 OpenAPI specification.
FreshBooks lets you create professional looking invoices in seconds, automatically record expenses with ease and track your time quickly and efficiently so you can focus on what matters most - serving the needs of your clients. The FreshBooks API is an interface for accessing your FreshBooks data using JSON.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a FreshBooks account.
- Obtain tokens by following this guide.
Quickstart
To use the FreshBooks connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/freshbooks
module into the Ballerina project.
import ballerinax/freshbooks;
Step 2: Create a new connector instance
Create a freshbooks:ClientConfig
with the <ACCESS_TOKEN>
obtained, and initialize the connector with it.
freshbooks:ClientConfig clientConfig = { auth : { token: `<ACCESS_TOKEN>` } }; freshbooks:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to list clients using the connector.
public function main() { string accountId = "<ACCOUNT_ID>"; freshbooks:ClientListResponse|error result = baseClient->listClients(accountId); if (result is freshbooks:ClientListResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
freshbooks: Client
This is a generated connector for FreshBooks API v1 OpenAPI specification. FreshBooks lets you create professional looking invoices in seconds, automatically record expenses with ease and track your time quickly and efficiently so you can focus on what matters most - serving the needs of your clients. The FreshBooks API is an interface for accessing your FreshBooks data using JSON.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a FreshBooks account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.freshbooks.com/" - URL of the target service
listClients
function listClients(string accountId) returns ClientListResponse|error
List all clients
Parameters
- accountId string - Account ID.
Return Type
- ClientListResponse|error - Success
createClient
function createClient(string accountId, ClientCreateObject payload) returns ClientObjectResponse|error
Create a new client
Return Type
- ClientObjectResponse|error - Success
getClient
function getClient(string accountId, string clientId) returns ClientObjectResponse|error
Get a single client with the parameter associated with clientId.
Return Type
- ClientObjectResponse|error - Success
updateClient
function updateClient(string accountId, string clientId, ClientCreateObject payload) returns ClientObjectResponse|error
Upadate a client
Parameters
- accountId string - Account ID.
- clientId string - Client ID.
- payload ClientCreateObject - Updated client data
Return Type
- ClientObjectResponse|error - Success
listEstimates
function listEstimates(string accountId) returns EstimateListResponse|error
Get an entire list of estimates that exist within your account.
Parameters
- accountId string - Account ID.
Return Type
- EstimateListResponse|error - Success
getEstimate
function getEstimate(string accountId, string estimateId) returns EstimateObjectResponse|error
Get a single estimate
Return Type
- EstimateObjectResponse|error - Success
updateEstimate
function updateEstimate(string accountId, string estimateId, EstimateUpdateObject payload) returns EstimateObjectResponse|error
Update an estimate
Parameters
- accountId string - Account ID.
- estimateId string - Estimate ID.
- payload EstimateUpdateObject - Updated estimate data
Return Type
- EstimateObjectResponse|error - Success
listExpenses
function listExpenses(string accountId) returns ExpenseListResponse|error
Get all expenses
Parameters
- accountId string - Account ID.
Return Type
- ExpenseListResponse|error - Success
createExpense
function createExpense(string accountId, ExpenseCreateObject payload) returns ExpenseObjectResponse|error
Create a new expense
Return Type
- ExpenseObjectResponse|error - Success
getExpense
function getExpense(string accountId, string expenseId) returns ExpenseObjectResponse|error
Get a single expense
Return Type
- ExpenseObjectResponse|error - Success
updateExpense
function updateExpense(string accountId, string expenseId, ExpenseUpdateObject payload) returns ExpenseObjectResponse|error
Update an expense
Parameters
- accountId string - Account ID.
- expenseId string - Expense ID.
- payload ExpenseUpdateObject - Updated expense data
Return Type
- ExpenseObjectResponse|error - Success
listInvoices
function listInvoices(string accountId) returns InvoiceListResponse|error
Get all invoices
Parameters
- accountId string - Account ID.
Return Type
- InvoiceListResponse|error - Success
createInvoice
function createInvoice(string accountId, InvoiceCreateObject payload) returns InvoiceObjectResponse|error
Create a new invoice
Return Type
- InvoiceObjectResponse|error - Success
getInvoice
function getInvoice(string accountId, string invoiceId) returns InvoiceObjectResponse|error
Get a single invoice
Return Type
- InvoiceObjectResponse|error - Success
updateInvoice
function updateInvoice(string accountId, string invoiceId, InvoiceUpdateObject payload) returns InvoiceObjectResponse|error
Update an invoice
Parameters
- accountId string - Account ID.
- invoiceId string - Invoice ID.
- payload InvoiceUpdateObject - Updated invoice data
Return Type
- InvoiceObjectResponse|error - Success
listTimeEntries
function listTimeEntries(string businessId, boolean? billable, boolean? billed, int? clientId, boolean? includeDeleted, boolean? team, boolean? includeUnlogged, boolean? startedFrom, boolean? startedTo, boolean? updatedSince, boolean? identityId) returns TimeEntryListResponse|error
Get time entries
Parameters
- businessId string - Bussiness ID.
- billable boolean? (default ()) - True returns entries that can be automatically added to an invoice
- billed boolean? (default ()) - True returns entries that have already been added to an invoice
- clientId int? (default ()) - Matches exact client ID
- includeDeleted boolean? (default ()) - True returns deleted time entries
- team boolean? (default ()) - True returns entries logged by all members of the business
- includeUnlogged boolean? (default ()) - True returns entries currently in progress for a running timer
- startedFrom boolean? (default ()) - matches entries that take place at or after the specified UTC date/time
- startedTo boolean? (default ()) - matches entries that take place before or at the specified UTC date/time
- updatedSince boolean? (default ()) - matches entries that have been updated at or after the specified UTC date/time
- identityId boolean? (default ()) - Matches entries logged against a specific teammate or user
Return Type
- TimeEntryListResponse|error - Success
createTimeEntry
function createTimeEntry(string businessId, TimeEntryCreateObject payload) returns TimeEntryObjectResponse|error
Create a new time entry
Return Type
- TimeEntryObjectResponse|error - Success
updateTimeEntry
function updateTimeEntry(string businessId, string timeEntryId, TimeEntryUpdateObject payload) returns TimeEntryObjectResponse|error
Update a time entry
Parameters
- businessId string - Bussiness ID.
- timeEntryId string - Time entry ID.
- payload TimeEntryUpdateObject - Updated time entry data
Return Type
- TimeEntryObjectResponse|error - Success
deleteTimeEntry
Delete a time entry
createProject
function createProject(string businessId, ProjectCreateObject payload) returns ProjectObjectResponse|error
Create a new project
Return Type
- ProjectObjectResponse|error - Success
listTaxes
function listTaxes(string accountId) returns TaxListResponse|error
Get details of all taxes
Parameters
- accountId string - Account ID.
Return Type
- TaxListResponse|error - Success
createTax
function createTax(string accountId, TaxCreateObject payload) returns TaxObjectResponse|error
Create a new tax
Return Type
- TaxObjectResponse|error - Success
getTax
function getTax(string accountId, string taxId) returns TaxObjectResponse|error
Get a single tax details
Return Type
- TaxObjectResponse|error - Success
updateTax
function updateTax(string accountId, string taxId, TaxUpdateObject payload) returns TaxObjectResponse|error
Update a tax
Parameters
- accountId string - Account ID.
- taxId string - Tax ID.
- payload TaxUpdateObject - Updated tax entry data
Return Type
- TaxObjectResponse|error - Success
deleteTax
Delete a tax
listExpenseCategories
function listExpenseCategories(string accountId) returns ExpenseCategoryListResponse|error
Get details of all expense categories
Parameters
- accountId string - Account ID.
Return Type
- ExpenseCategoryListResponse|error - Success
getExpenseCategory
function getExpenseCategory(string accountId, string categoryId) returns ExpenseCategoryObjectResponse|error
Get a single expense category details
Return Type
- ExpenseCategoryObjectResponse|error - Success
listPayments
function listPayments(string accountId) returns PaymemtListResponse|error
Get details of all payments
Parameters
- accountId string - Account ID.
Return Type
- PaymemtListResponse|error - Success
makePayment
function makePayment(string accountId, PaymemtCreateObject payload) returns PaymemtObjectResponse|error
Add a payment to a specific invoice
Return Type
- PaymemtObjectResponse|error - Success
getPayment
function getPayment(string accountId, string paymentId) returns PaymemtObjectResponse|error
Get a single payment details
Return Type
- PaymemtObjectResponse|error - Success
updatePayment
function updatePayment(string accountId, string paymentId, PaymemtCreateObject payload) returns PaymemtObjectResponse|error
Update a payment
Parameters
- accountId string - Account ID.
- paymentId string - Payment ID.
- payload PaymemtCreateObject - Updated tax entry data
Return Type
- PaymemtObjectResponse|error - Success
Records
freshbooks: ClientCreate
Fields
- fname string? - First name
- lname string? - Last name
- email string? - Email address
- organization string? - Name for client’s business
- vat_name string? - Value Added Tax name
- vat_number string? - Value Added Tax number
- status boolean? - Status
- note string? - Notes kept by admin about client
- home_phone string? - Home phone number
- userid int? - Duplicate of ID
- 'source string? - Source
- highlight_string string? - Highlight string
- p_city string? - Billing city
- p_code string? - Billing postal code
- p_country string? - Billing country
- p_province string? - Billing province
- p_street string? - Billing street address
- p_street2 string? - Billing street address second part
- currency_code string? - Three letter shortcode for preferred currency
- language string? - Shortcode indicating user language e.g. “en”
- last_activity string? - Last client activity action
- face string? - Face
- late_fee string? - Late fee
- late_reminders anydata[]? -
- contacts Contact[]? -
freshbooks: ClientCreateObject
Fields
- 'client ClientCreate? -
freshbooks: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
freshbooks: ClientListResponse
Fields
- response ClientlistresponseResponse? -
freshbooks: ClientlistresponseResponse
Fields
- result ClientlistresponseResponseResult? -
freshbooks: ClientlistresponseResponseResult
Fields
- clients ClientObject[]? -
- per_page int? - Record count per page
- total int? - Total records
- pages int? - Number of pages
- page int? - Page
freshbooks: ClientObject
Fields
- allow_late_notifications boolean? - Allow late notifications
- accounting_systemid string? - Unique identifier of business client exists on
- bus_phone string? - Business phone number
- company_industry string? - Description of industry client is in
- company_size string? - Size of client’s company
- currency_code string? - Three letter shortcode for preferred currency
- email string? - Email address
- fax string? - Fax number
- fname string? - First name
- home_phone string? - Home phone number
- ID int? - Unique to this business ID for client
- language string? - Shortcode indicating user language e.g. “en”
- last_activity string? - Last client activity action
- last_login string? - Last login time
- lname string? - Last name
- mob_phone string? - Mobile phone number
- note string? - Notes kept by admin about client
- num_logins int? - Number of logins
- organization string? - Name for client’s business
- p_city string? - Billing city
- p_code string? - Billing postal code
- p_country string? - Billing country
- p_province string? - Billing province
- p_street string? - Billing street address
- p_street2 string? - Billing street address second part
- pref_email boolean? - Prefers email over ground mail
- pref_gmail boolean? - Prefers ground mail over email
- s_city string? - Shipping address city
- s_code string? - Shipping postal code
- s_country string? - Shipping country
- s_province string? - Short form for province
- s_street string? - Shipping street address
- s_street2 string? - Shipping address second street info
- signup_date string? - Time of user signup
- subdomain string? - Unused in the new FreshBooks
- updated string? - Time of last modification to resource
- userid int? - Duplicate of ID
- vat_name string? - Value Added Tax name
- vat_number string? - Value Added Tax number
- vis_state int? - “visibility state”, active, deleted, or archived
freshbooks: ClientObjectResponse
Fields
- response ClientobjectresponseResponse? -
freshbooks: ClientobjectresponseResponse
Fields
- result ClientobjectresponseResponseResult? -
freshbooks: ClientobjectresponseResponseResult
Fields
- 'client ClientObject? -
freshbooks: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
freshbooks: Contact
Fields
- fname string? - First name
- lname string? - Last name
- email string? - Email address
- userid int? - Duplicate of ID
- face string? - Face
freshbooks: DeleteBody
Fields
- vis_state string? -
freshbooks: DeletionResponse
freshbooks: Estimate
Fields
- estimateid int? - Unique-to-this-system estimate ID
- ID int? - Unique-to-this-system estimate ID, duplicate of estimateid
- accounting_systemid string? - Unique ID for system
- ui_status string? - UI status fields give a descriptive name to states which can be used in filters, and apply to many invoices, estimates, and recurring profiles
- status int? - Estimate status
- amount EstimateAmount? - Total amount of estimate, to two decimal places
- code string? - Zip code for address on estimate
- discount_total EstimateupdateDiscountTotal? - Subfields - Amount and code
- description string? - Description of first line of estimate
- current_organization string? - Name of organization being estimated — denormalized from client
- invoiced boolean? - Indicator of whether this estimate has been converted to an invoice that was sent
- ownerid int? - ID of creator of estimate. 1 if business admin, other if created by e.g. a contractor
- sentid int? - Userid of user who sent the estimate, typically 1 for admin
- created_at string? - Time the estimate was created, YYYY-MM-DD HH:MM:SS format
- updated string? - Time estimate last updated at, YYYY-MM-DD HH:MM:SS format
- display_status string? - Description of status shown in FreshBooks UI, one of ‘draft’, ‘sent’, or ‘viewed’
- estimate_number string? - User-specified and visible estimate ID
- customerid int? - Unique-to-this-system client-ID
- create_date string? - Date estimate was created, YYYY-MM-DD format
- discount_value string? - Decimal-string amount
- po_number string? - Post Office box number for address on estimate
- currency_code string? - Three-letter currency code for estimate
- language string? - Two-letter language code, e.g. “en”
- terms string? - Terms listed on estimate
- notes string? - Notes listed on estimate
- address string? - First line of address on estimate
- vis_state int? - 0 for active, 1 for deleted
- street string? - Street for address on estimate
- street2 string? - Second line of street for address on estimate
- city string? - City for address on estimate
- province string? - Province for address on estimate
- country string? - Country for address on estimate
- organization string? - Name of organization being estimated
- fname string? - First name of Client on estimate
- lname string? - Last name of client being estimated
- vat_name string? - Value Added Tax name if provided
- vat_number string? - Value Added Tax number if provided
- lines EstimateLines[]? - Lines of the estimate
freshbooks: EstimateAmount
Total amount of estimate, to two decimal places
Fields
- amount string? - Estimate amount, to two decimal places
- code string? - Three-letter currency code
freshbooks: EstimateLines
Fields
- lineid int? - Unique-to-this-estimate line ID
- amount EstimateupdateAmount? - Amount total of an estimate line, calculated with unit cost, quantity and tax. subfields - amount and code
- updated string? - Time estimate line last updated at, YYYY-MM-DD HH:MM:SS format
- 'type int? - Estimate line type, 0 for normal estimate line
- qty int? - Quantity of the estimate line unit, multiplied against unit_cost
- unit_cost InvoicelinesUnitCost? - Unit cost of the line item. subfields - amount and code
- description string? - Description for the estimate line item
- name string? - Name for the estimate line item
- taxName1 string? - Name for the first tax on the estimate line
- taxAmount1 string? - First tax amount, in percentage, up to 3 decimal places
- taxName2 string? - Name for the second tax on the estimate line
- taxAmount2 string? - Second tax amount, in percentage, up to 3 decimal places
freshbooks: EstimateLinesWritable
Fields
- 'type int? - Estimate line type, 0 for normal estimate line
- qty int? - Quantity of the estimate line unit, multiplied against unit_cost
- unit_cost InvoicelinesUnitCost? - Unit cost of the line item. subfields - amount and code
- description string? - Description for the estimate line item
- name string? - Name for the estimate line item
- taxName1 string? - Name for the first tax on the estimate line
- taxAmount1 string? - First tax amount, in percentage, up to 3 decimal places
- taxName2 string? - Name for the second tax on the estimate line
- taxAmount2 string? - Second tax amount, in percentage, up to 3 decimal places
freshbooks: EstimateListResponse
Fields
- response EstimatelistresponseResponse? -
freshbooks: EstimatelistresponseResponse
Fields
- result EstimatelistresponseResponseResult? -
freshbooks: EstimatelistresponseResponseResult
Fields
- estimates Estimate[]? -
- per_page int? - Record count per page
- total int? - Total records
- pages int? - Number of pages
- page int? - Page
freshbooks: EstimateObjectResponse
Fields
- response EstimateobjectresponseResponse? -
freshbooks: EstimateobjectresponseResponse
Fields
- result EstimateobjectresponseResponseResult? -
freshbooks: EstimateobjectresponseResponseResult
Fields
- estimate Estimate? -
freshbooks: EstimateUpdate
Fields
- estimateid int? - Unique-to-this-system estimate ID
- ID int? - Unique-to-this-system estimate ID, duplicate of estimateid
- accounting_systemid string? - Unique ID for system
- ui_status string? - See Estimate UI Status table
- status string? - See Estimate Status table
- amount EstimateupdateAmount? - Amount total of an estimate line, calculated with unit cost, quantity and tax. subfields - amount and code
- discount_total EstimateupdateDiscountTotal? - Subfields - Amount and code
- description string? - Description of first line of estimate
- current_organization string? - Name of organization being estimated — denormalized from client
- invoiced boolean? - Indicator of whether this estimate has been converted to an invoice that was sent
- ownerid int? - ID of creator of estimate. 1 if business admin, other if created by e.g. a contractor
- sentid int? - Userid of user who sent the estimate, typically 1 for admin
- created_at string? - Time the estimate was created, YYYY-MM-DD HH:MM:SS format
- updated string? - Time estimate last updated at, YYYY-MM-DD HH:MM:SS format
- display_status string? - Description of status shown in FreshBooks UI, one of ‘draft’, ‘sent’, or ‘viewed’
- estimate_number string? - User-specified and visible estimate ID
- customerid int - Unique-to-this-system client-ID
- create_date string - Date estimate was created, YYYY-MM-DD format
- discount_value string? - Decimal-string amount
- po_number string? - Post Office box number for address on estimate
- currency_code string? - Three-letter currency code for estimate
- language string? - Two-letter language code, e.g. “en”
- terms string? - Terms listed on estimate
- notes string? - Notes listed on estimate
- address string? - First line of address on estimate
- vis_state int? - 0 for active, 1 for deleted
- street string? - Street for address on estimate
- street2 string? - Second line of street for address on estimate
- city string? - City for address on estimate
- province string? - Province for address on estimate
- country string? - Country for address on estimate
- organization string? - Name of organization being estimated
- fname string? - First name of Client on estimate
- lname string? - Last name of client being estimated
- vat_name string? - Value Added Tax name if provided
- vat_number string? - Value Added Tax number if provided
- lines EstimateLinesWritable[]? - Lines of the estimate
freshbooks: EstimateupdateAmount
Amount total of an estimate line, calculated with unit cost, quantity and tax. subfields - amount and code
Fields
- amount string? - Amount of estimate line item account, to two decimal places
- code string? - Three-letter currency code
freshbooks: EstimateupdateDiscountTotal
Subfields - Amount and code
Fields
- amount string? - Unit cost amount, to two decimal places
- code string? - Three-letter currency code
freshbooks: EstimateUpdateObject
Fields
- estimate EstimateUpdate? -
freshbooks: Expense
Fields
- categoryid int? - ID of related expense category
- markup_percent string? - String-decimal, note of percent to mark expense up
- projectid int? - ID of related project if applicable
- clientid int? - ID of related client if applicable
- taxName1 string? - Name of first tax
- taxPercent2 string? - string-decimal tax amount for second tax – indicates the maximum tax percentage for this expense, this does not add tax to the expense, instead use taxAmount2
- taxName2 string? - Name of second tax
- isduplicate boolean? - True/false is duplicated expense
- profileid int? - ID of related profile if applicable
- account_name string? - Name of related account if applicable
- transactionid int? - ID of related transaction if applicable
- invoiceid int? - ID of related invoice if applicable
- ID int? - Duplicate of expenseid
- taxAmount1 ExpensecreateTaxamount1? - The total for first tax applied to the subtotal amount of the expense
- taxAmount2 ExpensecreateTaxamount2? - The total for second tax applied to the subtotal amount of the expense
- vis_state int? - 0 for active, 1 for deleted
- status int? - Expense status
- bank_name string? - Name of bank expense was imported from, if applicable
- updated string? - Time invoice last updated at, YYYY-MM-DD HH:MM:SS format
- vendor string? - Name of vendor
- ext_systemid int? - ID of related contractor system if applicable
- staffid int? - ID of related staff member if applicable
- date string? - Date of expense, YYYY-MM-DD format
- has_receipt boolean? - True/false has receipt attached
- accounting_systemid string? - Unique ID for system
- background_jobid int? - (internal) ID for related background job if applicable
- notes string? - Notes about expense
- ext_invoiceid int? - ID of related contractor invoice if applicable
- amount ExpensecreateAmount? - Total amount of the expense
- expenseid int? - Unique to this business ID for expense
- accountid int? - ID of expense account if applicable
freshbooks: ExpenseCategory
Fields
- category string? - Name for this category, e.g. “Advertising”
- categoryid int? - Unique to this business ID for this category
- ID int? - Duplicate of categoryid
- is_editable boolean? - True/false can be edited
- is_cogs boolean? - True/false represents cost of goods sold
- parentid int? - Categorid of parent category
- vis_state int? - 0 for active, 1 for deleted
freshbooks: ExpenseCategoryListResponse
Fields
- response ExpensecategorylistresponseResponse? -
freshbooks: ExpensecategorylistresponseResponse
Fields
freshbooks: ExpensecategorylistresponseResponseResult
Fields
- categories ExpenseCategory[]? -
freshbooks: ExpenseCategoryObjectResponse
Fields
- response ExpensecategoryobjectresponseResponse? -
freshbooks: ExpensecategoryobjectresponseResponse
Fields
freshbooks: ExpensecategoryobjectresponseResponseResult
Fields
- category ExpenseCategory? -
freshbooks: ExpenseCreate
Fields
- categoryid int - ID of related expense category
- markup_percent string? - String-decimal, note of percent to mark expense up
- projectid int? - ID of related project if applicable
- clientid int? - ID of related client if applicable
- taxName1 string? - Name of first tax
- taxPercent2 string? - string-decimal tax amount for second tax – indicates the maximum tax percentage for this expense, this does not add tax to the expense, instead use taxAmount2
- taxName2 string? - Name of second tax
- isduplicate boolean? - True/false is duplicated expense
- profileid int? - ID of related profile if applicable
- account_name string? - Name of related account if applicable
- transactionid int? - ID of related transaction if applicable
- invoiceid int? - ID of related invoice if applicable
- ID int? - Duplicate of expenseid
- taxAmount1 ExpensecreateTaxamount1? - The total for first tax applied to the subtotal amount of the expense
- taxAmount2 ExpensecreateTaxamount2? - The total for second tax applied to the subtotal amount of the expense
- vis_state int? - 0 for active, 1 for deleted
- status int? - Expense statuses are not directly modifiable. Each is a description of the status of the expense
- bank_name string? - Name of bank expense was imported from, if applicable
- updated string? - Time invoice last updated at, YYYY-MM-DD HH:MM:SS format
- vendor string? - Name of vendor
- ext_systemid int? - ID of related contractor system if applicable
- staffid int - ID of related staff member if applicable
- date string - Date of expense, YYYY-MM-DD format
- has_receipt boolean? - True/false has receipt attached
- accounting_systemid string? - Unique ID for system
- background_jobid int? - (internal) ID for related background job if applicable
- notes string? - Notes about expense
- ext_invoiceid int? - ID of related contractor invoice if applicable
- amount ExpensecreateAmount - Total amount of the expense
- expenseid int? - Unique to this business ID for expense
- accountid int? - ID of expense account if applicable
freshbooks: ExpensecreateAmount
Total amount of the expense
Fields
- amount string? - String-decimal amount of the expense
- code string? - 3-letter currency code
freshbooks: ExpenseCreateObject
Fields
- expense ExpenseCreate? -
freshbooks: ExpensecreateTaxamount1
The total for first tax applied to the subtotal amount of the expense
Fields
- amount string? - String-decimal amount
- code string? - 3-letter currency code
freshbooks: ExpensecreateTaxamount2
The total for second tax applied to the subtotal amount of the expense
Fields
- amount string? - String-decimal amount
- code string? - 3-letter currency code
freshbooks: ExpenseListResponse
Fields
- response ExpenselistresponseResponse? -
freshbooks: ExpenselistresponseResponse
Fields
- result ExpenselistresponseResponseResult? -
freshbooks: ExpenselistresponseResponseResult
Fields
- expenses Expense[]? -
freshbooks: ExpenseObjectResponse
Fields
- response ExpenseobjectresponseResponse? -
freshbooks: ExpenseobjectresponseResponse
Fields
- result ExpenseobjectresponseResponseResult? -
freshbooks: ExpenseobjectresponseResponseResult
Fields
- expense Expense? -
freshbooks: ExpenseUpdate
Fields
- categoryid int? - ID of related expense category
- markup_percent string? - String-decimal, note of percent to mark expense up
- projectid int? - ID of related project if applicable
- clientid int? - ID of related client if applicable
- taxName1 string? - Name of first tax
- taxPercent2 string? - string-decimal tax amount for second tax – indicates the maximum tax percentage for this expense, this does not add tax to the expense, instead use taxAmount2
- taxName2 string? - Name of second tax
- isduplicate boolean? - True/false is duplicated expense
- profileid int? - ID of related profile if applicable
- account_name string? - Name of related account if applicable
- transactionid int? - ID of related transaction if applicable
- invoiceid int? - ID of related invoice if applicable
- ID int? - Duplicate of expenseid
- taxAmount1 ExpensecreateTaxamount1? - The total for first tax applied to the subtotal amount of the expense
- taxAmount2 ExpensecreateTaxamount2? - The total for second tax applied to the subtotal amount of the expense
- vis_state int? - 0 for active, 1 for deleted
- status int? - Expense statuses are not directly modifiable. Each is a description of the status of the expense
- bank_name string? - Name of bank expense was imported from, if applicable
- updated string? - Time invoice last updated at, YYYY-MM-DD HH:MM:SS format
- vendor string? - Name of vendor
- ext_systemid int? - ID of related contractor system if applicable
- staffid int? - ID of related staff member if applicable
- date string? - Date of expense, YYYY-MM-DD format
- has_receipt boolean? - True/false has receipt attached
- accounting_systemid string? - Unique ID for system
- background_jobid int? - (internal) ID for related background job if applicable
- notes string? - Notes about expense
- ext_invoiceid int? - ID of related contractor invoice if applicable
- amount ExpensecreateAmount? - Total amount of the expense
- expenseid int? - Unique to this business ID for expense
- accountid int? - ID of expense account if applicable
freshbooks: ExpenseUpdateObject
Fields
- expense ExpenseUpdate? -
freshbooks: Invoice
Fields
- ownerid int? - ID of creator of invoice. 1 if business admin, other if created by e.g. a contractor
- estimateid int? - ID of associated estimate, 0 if none
- sentid int? - Userid of user who sent the invoice, typically 1 for admin
- status int? - Invoice Status
- parent int? - ID of object this invoice was generated from, 0 if none
- display_status string? - Description of status shown in FreshBooks UI, one of ‘draft’, ‘created’, ‘sent’, ‘viewed’, or ‘outstanding’.
- autobill_status string? - One of retry, failed, or success
- payment_status string? - description of payment status. One of ‘unpaid’, ‘partial’, ‘paid’, and ‘auto-paid’. See the v3_status table on this page for descriptions of each.
- last_order_status string? - Describes status of last attempted payment
- dispute_status string? - Description of whether invoice has been disputed.
- deposit_status string? - description of deposits applied to invoice. One of ‘paid’, ‘unpaid’, ‘partial’, ‘none’, and ‘converted’.
- auto_bill boolean? - Whether this invoice has a credit card saved
- v3_status string? - Description of Invoice Status, see V3 Status Table.
- invoice_number string? - User-specified and visible invoice ID
- customerid int? - Unique-to-this-system client-ID
- create_date string? - Date invoice was created, YYYY-MM-DD format
- generation_date string? - date invoice generated from object, null if it wasn’t, YYYY-MM-DD if it was
- discount_value string? - percent amount being discounted from the subtotal, decimal-string amount ranging from 0 to 100
- discount_description string? - Public note about discount
- po_number string? - Reference number for address on invoice.
- template string? - (internal, deprecated) choice of rendering style
- currency_code string? - Three-letter currency code for invoice
- language string? - Two-letter language code, e.g. “en”
- terms string? - Terms listed on invoice
- notes string? - Notes listed on invoice
- address string? - First line of address on invoice
- deposit_amount InvoiceDepositAmount? - Amount required as deposit, null if none
- deposit_percentage string? - Percent of the invoice’s value required as a deposit
- gmail boolean? - Whether to send via ground mail
- show_attachments boolean? - Whether attachments on invoice are rendered
- vis_state int? - 0 for active, 1 for deleted
- street string? - Street for address on invoice
- street2 string? - Second line of street for address on invoice.
- city string? - City for address on invoice
- province string? - Province for address on invoice.
- code string? - Zip code for address on invoice
- country string? - Country for address on invoice
- organization string? - Name of organization being invoiced.
- fname string? - First name of Client on invoice
- lname string? - Last name of client being invoiced
- vat_name string? - Value Added Tax name if provided
- vat_number string? - Value Added Tax number if provided
- due_offset_days int? - Number of days from creation that invoice is due. If not set, the due date will default to the date of issue.
- lines InvoiceLines[]? - Lines of the invoice
- presentation record {}? - where invoice logo and styles are defined. See our postman collection for details.
- invoiceid int? - Unique-to-this-system invoice ID
- ID int? - Unique-to-this-system invoice ID, duplicate of invoiceid
- accounting_systemid string? - Unique ID for system
- accountid string? - Unique ID for system, repeat of accounting_systemid
- amount InvoiceAmount? - Total amount of invoice. subfields - amount, code
- paid InvoicePaid? - Subfields - amount and code
- outstanding InvoiceOutstanding? - Subfields - amount, code
- discount_total InvoiceDiscountTotal? - Subfields - amount and code
- created_at string? - Time the invoice was created, YYYY-MM-DD HH:MM:SS format
- current_organization string? - Name of organization being invoiced — denormalized from client
- date_paid string? - Date invoice was fully paid, YYYY-MM-DD format
- description string? - Description of first line of invoice
- due_date string? - date invoice is marked as due by, YYYY-MM-DD format, calculated from due_offset_days. If due_offset_days is not set, it will default to the date of issue.
- updated string? - Time invoice last updated at, YYYY-MM-DD HH:MM:SS format
freshbooks: InvoiceAmount
Total amount of invoice. subfields - amount, code
Fields
- amount string? - Total amount of invoice, to two decimal places
- code string? - Three-letter currency code
freshbooks: InvoiceCreate
Fields
- ownerid int? - ID of creator of invoice. 1 if business admin, other if created by e.g. a contractor
- estimateid int? - ID of associated estimate, 0 if none
- sentid int? - Userid of user who sent the invoice, typically 1 for admin
- status string? - See Invoice Status table.
- parent int? - ID of object this invoice was generated from, 0 if none
- display_status string? - Description of status shown in FreshBooks UI, one of ‘draft’, ‘created’, ‘sent’, ‘viewed’, or ‘outstanding’.
- autobill_status string? - One of retry, failed, or success
- payment_status string? - description of payment status. One of ‘unpaid’, ‘partial’, ‘paid’, and ‘auto-paid’. See the v3_status table on this page for descriptions of each.
- last_order_status string? - Describes status of last attempted payment
- dispute_status string? - Description of whether invoice has been disputed.
- deposit_status string? - description of deposits applied to invoice. One of ‘paid’, ‘unpaid’, ‘partial’, ‘none’, and ‘converted’.
- auto_bill boolean? - Whether this invoice has a credit card saved
- v3_status string? - Description of Invoice Status, see V3 Status Table.
- invoice_number string? - User-specified and visible invoice ID
- customerid int - Unique-to-this-system client-ID
- create_date string - Date invoice was created, YYYY-MM-DD format
- generation_date string? - date invoice generated from object, null if it wasn’t, YYYY-MM-DD if it was
- discount_value string? - percent amount being discounted from the subtotal, decimal-string amount ranging from 0 to 100
- discount_description string? - Public note about discount
- po_number string? - Reference number for address on invoice.
- template string? - (internal, deprecated) choice of rendering style
- currency_code string? - Three-letter currency code for invoice
- language string? - Two-letter language code, e.g. “en”
- terms string? - Terms listed on invoice
- notes string? - Notes listed on invoice
- address string? - First line of address on invoice
- deposit_amount InvoiceDepositAmount? - Amount required as deposit, null if none
- deposit_percentage string? - Percent of the invoice’s value required as a deposit
- gmail boolean? - Whether to send via ground mail
- show_attachments boolean? - Whether attachments on invoice are rendered
- vis_state int? - 0 for active, 1 for deleted
- street string? - Street for address on invoice
- street2 string? - Second line of street for address on invoice.
- city string? - City for address on invoice
- province string? - Province for address on invoice.
- code string? - Zip code for address on invoice
- country string? - Country for address on invoice
- organization string? - Name of organization being invoiced.
- fname string? - First name of Client on invoice
- lname string? - Last name of client being invoiced
- vat_name string? - Value Added Tax name if provided
- vat_number string? - Value Added Tax number if provided
- due_offset_days int? - Number of days from creation that invoice is due. If not set, the due date will default to the date of issue.
- lines InvoiceLinesWritable[]? - Lines of the invoice
- presentation record {}? - where invoice logo and styles are defined. See our postman collection for details.
freshbooks: InvoiceCreateObject
Fields
- invoice InvoiceCreate? -
freshbooks: InvoiceDepositAmount
Amount required as deposit, null if none
Fields
- amount string? - Total amount of invoice, to two decimal places
- code string? - Three-letter currency code
freshbooks: InvoiceDiscountTotal
Subfields - amount and code
Fields
- amount string? - Amount of discount, to two decimal places
- code string? - Three-letter currency code
freshbooks: InvoiceLines
Fields
- lineid int? - Unique-to-this-invoice line ID
- amount InvoicelinesAmount? - amount total of an invoice line, calculated with unit cost, quantity and tax. subfields - amount and code
- updated string? - Time invoice line last updated at, YYYY-MM-DD HH:MM:SS format
- 'type int? - invoice line type, 0 for normal invoice line, 1 for rebilling expense line
- expenseid int? - ID of unbilled expense, required when invoice line type is 1, otherwise should be excluded
- qty int? - Quantity of the invoice line unit, multiplied against unit_cost
- unit_cost InvoicelinesUnitCost? - Unit cost of the line item. subfields - amount and code
- description string? - Description for the invoice line item
- name string? - Name for the invoice line item
- taxName1 string? - Name for the first tax on the invoice line
- taxAmount1 string? - First tax amount, in percentage, up to 3 decimal places
- taxName2 string? - Name for the second tax on the invoice line
- taxAmount2 string? - Second tax amount, in percentage, up to 3 decimal places
freshbooks: InvoicelinesAmount
amount total of an invoice line, calculated with unit cost, quantity and tax. subfields - amount and code
Fields
- amount string? - Amount of invoice line item account, to two decimal places
- code string? - Three-letter currency code
freshbooks: InvoicelinesUnitCost
Unit cost of the line item. subfields - amount and code
Fields
- amount string? - Unit cost amount, to two decimal places
- code string? - Three-letter currency code
freshbooks: InvoiceLinesWritable
Fields
- 'type int? - invoice line type, 0 for normal invoice line, 1 for rebilling expense line
- expenseid int? - ID of unbilled expense, required when invoice line type is 1, otherwise should be excluded
- qty int? - Quantity of the invoice line unit, multiplied against unit_cost
- unit_cost InvoicelinesUnitCost? - Unit cost of the line item. subfields - amount and code
- description string? - Description for the invoice line item
- name string? - Name for the invoice line item
- taxName1 string? - Name for the first tax on the invoice line
- taxAmount1 string? - First tax amount, in percentage, up to 3 decimal places
- taxName2 string? - Name for the second tax on the invoice line
- taxAmount2 string? - Second tax amount, in percentage, up to 3 decimal places
freshbooks: InvoiceListResponse
Fields
- response InvoicelistresponseResponse? -
freshbooks: InvoicelistresponseResponse
Fields
- result InvoicelistresponseResponseResult? -
freshbooks: InvoicelistresponseResponseResult
Fields
- invoices Invoice[]? -
freshbooks: InvoiceObjectResponse
Fields
- response InvoiceobjectresponseResponse? -
freshbooks: InvoiceobjectresponseResponse
Fields
- result InvoiceobjectresponseResponseResult? -
freshbooks: InvoiceobjectresponseResponseResult
Fields
- invoice Invoice? -
freshbooks: InvoiceOutstanding
Subfields - amount, code
Fields
- amount string? - Amount outstanding on invoice, to two decimal places
- code string? - Three-letter currency code
freshbooks: InvoicePaid
Subfields - amount and code
Fields
- amount string? - Amount paid on invoice, to two decimal places
- code string? - Three-letter currency code
freshbooks: InvoiceUpdate
Fields
- ownerid int? - ID of creator of invoice. 1 if business admin, other if created by e.g. a contractor
- estimateid int? - ID of associated estimate, 0 if none
- sentid int? - Userid of user who sent the invoice, typically 1 for admin
- status string? - See Invoice Status table.
- parent int? - ID of object this invoice was generated from, 0 if none
- display_status string? - Description of status shown in FreshBooks UI, one of ‘draft’, ‘created’, ‘sent’, ‘viewed’, or ‘outstanding’.
- autobill_status string? - One of retry, failed, or success
- payment_status string? - description of payment status. One of ‘unpaid’, ‘partial’, ‘paid’, and ‘auto-paid’. See the v3_status table on this page for descriptions of each.
- last_order_status string? - Describes status of last attempted payment
- dispute_status string? - Description of whether invoice has been disputed.
- deposit_status string? - description of deposits applied to invoice. One of ‘paid’, ‘unpaid’, ‘partial’, ‘none’, and ‘converted’.
- auto_bill boolean? - Whether this invoice has a credit card saved
- v3_status string? - Description of Invoice Status, see V3 Status Table.
- invoice_number string? - User-specified and visible invoice ID
- customerid int? - Unique-to-this-system client-ID
- create_date string? - Date invoice was created, YYYY-MM-DD format
- generation_date string? - date invoice generated from object, null if it wasn’t, YYYY-MM-DD if it was
- discount_value string? - percent amount being discounted from the subtotal, decimal-string amount ranging from 0 to 100
- discount_description string? - Public note about discount
- po_number string? - Reference number for address on invoice.
- template string? - (internal, deprecated) choice of rendering style
- currency_code string? - Three-letter currency code for invoice
- language string? - Two-letter language code, e.g. “en”
- terms string? - Terms listed on invoice
- notes string? - Notes listed on invoice
- address string? - First line of address on invoice
- deposit_amount InvoiceDepositAmount? - Amount required as deposit, null if none
- deposit_percentage string? - Percent of the invoice’s value required as a deposit
- gmail boolean? - Whether to send via ground mail
- show_attachments boolean? - Whether attachments on invoice are rendered
- vis_state int? - 0 for active, 1 for deleted
- street string? - Street for address on invoice
- street2 string? - Second line of street for address on invoice.
- city string? - City for address on invoice
- province string? - Province for address on invoice.
- code string? - Zip code for address on invoice
- country string? - Country for address on invoice
- organization string? - Name of organization being invoiced.
- fname string? - First name of Client on invoice
- lname string? - Last name of client being invoiced
- vat_name string? - Value Added Tax name if provided
- vat_number string? - Value Added Tax number if provided
- due_offset_days int? - Number of days from creation that invoice is due. If not set, the due date will default to the date of issue.
- lines InvoiceLinesWritable[]? - Lines of the invoice
- presentation record {}? - where invoice logo and styles are defined. See our postman collection for details.
freshbooks: InvoiceUpdateObject
Fields
- invoice InvoiceUpdate? -
freshbooks: PaymemtCreateObject
Fields
- payment Payment? -
freshbooks: PaymemtListResponse
Fields
- response PaymemtlistresponseResponse? -
freshbooks: PaymemtlistresponseResponse
Fields
- result PaymemtlistresponseResponseResult? -
freshbooks: PaymemtlistresponseResponseResult
Fields
- payments Payment[]? -
freshbooks: PaymemtObjectResponse
Fields
- response PaymemtobjectresponseResponse? -
freshbooks: PaymemtobjectresponseResponse
Fields
- result PaymemtobjectresponseResponseResult? -
freshbooks: PaymemtobjectresponseResponseResult
Fields
- payment Payment? -
freshbooks: Payment
Fields
- 'accounting\ \_systemid string? - Unique identifier of business client exists on
- amount PaymentAmount? - Amount paid on invoice
- bulk_paymentid int? -
- clientid int? - ID of client who made the payment
- creditid int? - ID of related credit
- date string? - Date the payment was made, YYYY-MM-DD format
- from_credit boolean? - Whether or not the payment was converted from a Credit on a Client’s account
- gateway string? - The payment processor used, if any
- ID int? - Unique ID (across this business) for payment
- invoiceid int - ID of related invoice
- logid int? - Duplicate of ID
- note string? - Notes on payment, often used for credit card reference number
- orderid int? - ID of related orderid
- overpaymentid int? - ID of related overpayment if relevant
- send_client_notification boolean? - Whether to send the client a notification of this payment
- transactionid int? - Transaction ID
- 'type string? - “Check”, “Credit”, “Cash”, etc.
- updated string? - Date payment was last updated, YYYY-MM-DD
- vis_state int? - 0 for active, 1 for deleted
freshbooks: PaymentAmount
Amount paid on invoice
Fields
- amount string? - string-decimal amount
- code string? - three-letter currency code
freshbooks: Project
Fields
- due_date string? - Date of projected completion.
- fixed_price string? - used for flat-rate projects. Represents the amount being charged to the client for the project
- group ProjectGroup? - Subfields - pending_invitations, ID, members
- description string? - Description of project
- complete boolean? - Whether the project is completed or not
- title string? - The project’s title
- project_type string? - Type of project - fixed_price, hourly_rate
- budget int? - Budget for project
- updated_at string? - The time the project was last updated
- services ProjectServices? - Subfields - business_id, name, ID
- rate string? - The hourly rate of the project. Only applies to hourly_rate projects
- client_id int? - Unique ID of the client being billed for the project
- created_at string? - The date/time the project was created
- logged_duration int? - The time logged for the project in seconds
- ID int? - Unique ID for the project
- billing_method string? - The method of payment for the project
- internal boolean? - clarifies that the project is internally within the company (client is the company)
freshbooks: ProjectCreate
Fields
- due_date string? - Date of projected completion.
- fixed_price string? - used for flat-rate projects. Represents the amount being charged to the client for the project
- group ProjectGroup? - Subfields - pending_invitations, ID, members
- description string? - Description of project
- complete boolean? - Whether the project is completed or not
- title string - The project’s title
- project_type string? - Type of project - fixed_price, hourly_rate
- budget int? - Budget for project
- updated_at string? - The time the project was last updated
- services ProjectServices? - Subfields - business_id, name, ID
- rate string? - The hourly rate of the project. Only applies to hourly_rate projects
- client_id int? - Unique ID of the client being billed for the project
- created_at string? - The date/time the project was created
- logged_duration int? - The time logged for the project in seconds
- ID int? - Unique ID for the project
- billing_method string? - The method of payment for the project
- internal boolean? - clarifies that the project is internally within the company (client is the company)
freshbooks: ProjectCreateObject
Fields
- project ProjectCreate? -
freshbooks: ProjectGroup
Subfields - pending_invitations, ID, members
Fields
- pending_invitations string[]? - the pending invites to employees and contractors within the project
- ID int? - Unique ID of group membership
- members ProjectGroupMembers? - subfields - first_name, last_name, role, identity_id, active, company, ID, email
freshbooks: ProjectGroupMembers
subfields - first_name, last_name, role, identity_id, active, company, ID, email
Fields
- first_name string? - First name of the identity
- last_name string? - Last name of the identity
- role string? - Named role identity holds in group
- identity_id int? - The unique ID for the identity
- active boolean? - Whether the project is active or not
- company string? - The name of the business
- ID int? - Unique ID for the membership
- email string? - Email of identity
freshbooks: ProjectObjectResponse
Fields
- project Project? -
freshbooks: ProjectServices
Subfields - business_id, name, ID
Fields
- business_id int? - Unique ID for business
- name string? - The name of the service
- ID int? - Service ID
freshbooks: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
freshbooks: Tax
Fields
- 'accounting\ \_systemid string? - Unique identifier of business tax exists on
- updated string? - Date object was last updated, YYYY-MM-DD HH:MM:SS format
- name string? - Identifiable name for your tax, e.g. “GST”
- number string? - An external number that identifies your tax submission
- taxid int? - Unique identifier of this tax within this business
- amount decimal? - String-decimal representing percentage value of tax
- compound boolean? - Compound taxes are calculated on top of primary taxes
- ID int? - Unique ID to look this tax up later
freshbooks: TaxCreate
Fields
- 'accounting\ \_systemid string? - Unique identifier of business tax exists on
- updated string? - Date object was last updated, YYYY-MM-DD HH:MM:SS format
- name string - Identifiable name for your tax, e.g. “GST”
- number string? - An external number that identifies your tax submission
- taxid int? - Unique identifier of this tax within this business
- amount decimal? - String-decimal representing percentage value of tax
- compound boolean? - Compound taxes are calculated on top of primary taxes
- ID int? - Unique ID to look this tax up later
freshbooks: TaxCreateObject
Fields
- tax TaxCreate? -
freshbooks: TaxListResponse
Fields
- response TaxlistresponseResponse? -
freshbooks: TaxlistresponseResponse
Fields
- result TaxlistresponseResponseResult? -
freshbooks: TaxlistresponseResponseResult
Fields
- taxes Tax[]? -
freshbooks: TaxObjectResponse
Fields
- response TaxobjectresponseResponse? -
freshbooks: TaxobjectresponseResponse
Fields
- result TaxobjectresponseResponseResult? -
freshbooks: TaxobjectresponseResponseResult
Fields
- tax Project? -
freshbooks: TaxUpdate
Fields
- 'accounting\ \_systemid string? - Unique identifier of business tax exists on
- updated string? - Date object was last updated, YYYY-MM-DD HH:MM:SS format
- name string - Identifiable name for your tax, e.g. “GST”
- number string? - An external number that identifies your tax submission
- taxid int? - Unique identifier of this tax within this business
- amount decimal? - String-decimal representing percentage value of tax
- compound boolean? - Compound taxes are calculated on top of primary taxes
- ID int? - Unique ID to look this tax up later
freshbooks: TaxUpdateObject
Fields
- tax TaxUpdate? -
freshbooks: TimeEntry
Fields
- billable boolean? - True for entries that can be automatically added to an invoice
- billed boolean? - True for entries that have already been added to an invoice or manually marked as billed
- client_id int? - The unique identifier of the client to be billed for this entry
- duration int? - The length of time in seconds
- internal boolean? - True if the time entry is not assigned to a client
- is_logged boolean? - False if the time entry is being created from a running timer
- note string? - A short description of the work being done during the time
- project_id int? - The unique identifier of the project worked on during the time
- service_id int? - The unique identifier of the project service worked on during the time
- started_at string? - The date/time in UTC when the work started
- identity_id int? - The unique identifier of the teammate or user logging the time entry
freshbooks: TimeEntryCreate
Fields
- billable boolean? - True for entries that can be automatically added to an invoice
- billed boolean? - True for entries that have already been added to an invoice or manually marked as billed
- client_id int? - The unique identifier of the client to be billed for this entry
- duration int - The length of time in seconds
- internal boolean? - True if the time entry is not assigned to a client
- is_logged boolean - False if the time entry is being created from a running timer
- note string? - A short description of the work being done during the time
- project_id int? - The unique identifier of the project worked on during the time
- service_id int? - The unique identifier of the project service worked on during the time
- started_at string - The date/time in UTC when the work started
- identity_id int? - The unique identifier of the teammate or user logging the time entry
freshbooks: TimeEntryCreateObject
Fields
- time_entry TimeEntryCreate? -
freshbooks: TimeEntryListResponse
Fields
- response TimeentrylistresponseResponse? -
freshbooks: TimeentrylistresponseResponse
Fields
- result TimeentrylistresponseResponseResult? -
freshbooks: TimeentrylistresponseResponseResult
Fields
- time_entries TimeEntry[]? -
freshbooks: TimeEntryObjectResponse
Fields
- time_entry TimeEntry? -
freshbooks: TimeEntryUpdate
Fields
- billable boolean? - True for entries that can be automatically added to an invoice
- billed boolean? - True for entries that have already been added to an invoice or manually marked as billed
- client_id int? - The unique identifier of the client to be billed for this entry
- duration int? - The length of time in seconds
- internal boolean? - True if the time entry is not assigned to a client
- is_logged boolean? - False if the time entry is being created from a running timer
- note string? - A short description of the work being done during the time
- project_id int? - The unique identifier of the project worked on during the time
- service_id int? - The unique identifier of the project service worked on during the time
- started_at string? - The date/time in UTC when the work started
- identity_id int? - The unique identifier of the teammate or user logging the time entry
freshbooks: TimeEntryUpdateObject
Fields
- time_entry TimeEntryUpdate? -
Import
import ballerinax/freshbooks;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 4
Current verison: 3
Weekly downloads
Keywords
Finance/Accounting
Cost/Paid
Contributors
Dependencies