flatapi
Module flatapi
API
Definitions
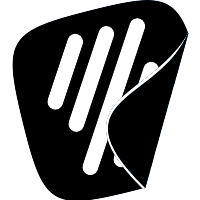
ballerinax/flatapi Ballerina library
Overview
This is a generated connector for Flat API v2.13.0 OpenAPI specification. The Flat API allows you to easily extend the abilities of the Flat Platform. Flat is a powerful cloud-based notation software that let you to create music scores and guitar tabs.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Flat Account
- Obtaining tokens
- Log into Flat Account
- Obtain tokens - Follow this link
Quickstart
To use the Flat connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/flatapi module into the Ballerina project.
import ballerinax/flatapi;
Step 2: Create a new connector instance
You can use Personal Access Token(PAT) for authentication:
flatapi:ClientConfig clientConfig = { auth: { token: <PAT_TOKEN> } }; flatapi:Client baseClient = check new Client(clientConfig, serviceUrl = "https://api.flat.io/v2");
Step 3: Invoke connector operation
- You can get detail about score created.
flatapi:ScoreDetails score = check baseClient->getScore(<SCORE_ID>);
- Use
bal run
command to compile and run the Ballerina program.
Clients
flatapi: Client
This is a generated connector for Flat API v2.13.0 OpenAPI specification. The Flat API allows you to easily extend the abilities of the Flat Platform, with a wide range of use cases including the following:
- Creating and importing new music scores using MusicXML, MIDI, Guitar Pro (GP3, GP4, GP5, GPX, GP), PowerTab, TuxGuitar and MuseScore files
- Browsing, updating, copying, exporting the user's scores (for example in MP3, WAV or MIDI)
- Managing educational resources with Flat for Education: creating & updating the organization accounts, the classes, rosters and assignments. The Flat API is built on HTTP. Our API is RESTful It has predictable resource URLs. It returns HTTP response codes to indicate errors. It also accepts and returns JSON in the HTTP body. The schema of this API follows the OpenAPI Initiative (OAI) specification, you can use and work with compatible Swagger tools. This API features Cross-Origin Resource Sharing (CORS) implemented in compliance with W3C spec. You can use your favorite HTTP/REST library for your programming language to use Flat's API. This specification and reference is available on Github. Getting Started and learn more:
- API Overview and introduction
- Authentication (Personal Access Tokens or OAuth2)
- SDKs
- Rate Limits
- Changelog
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Flat Platform Account and obtain tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.flat.io/v2" - URL of the target service
getAuthenticatedUser
function getAuthenticatedUser(boolean onlyId) returns UserDetails|error
Get current user profile
Parameters
- onlyId boolean (default false) - Only return the user id
Return Type
- UserDetails|error - Current user details
createScore
function createScore(ScoreCreation payload) returns ScoreDetails|error
Create a new score
Parameters
- payload ScoreCreation -
Return Type
- ScoreDetails|error - Score created
getScore
function getScore(string score, string? sharingKey) returns ScoreDetails|error
Get a score's metadata
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ScoreDetails|error - Score details
editScore
function editScore(string score, ScoreModification payload) returns ScoreDetails|error
Edit a score's metadata
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- payload ScoreModification -
Return Type
- ScoreDetails|error - Score details
deleteScore
Delete a score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- now boolean (default false) - If
true
, the score deletion will be scheduled to be done ASAP
untrashScore
Untrash a score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
getScoreSubmissions
function getScoreSubmissions(string score) returns AssignmentSubmission[]|error
List submissions related to the score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
Return Type
- AssignmentSubmission[]|error - List of submissions
forkScore
function forkScore(string score, ScoreFork payload, string? sharingKey) returns ScoreDetails|error
Fork a score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- payload ScoreFork -
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ScoreDetails|error - Score details
getScoreCollaborators
function getScoreCollaborators(string score, string? sharingKey) returns ResourceCollaborator[]|error
List the collaborators
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ResourceCollaborator[]|error - List of collaborators
addScoreCollaborator
function addScoreCollaborator(string score, ResourceCollaboratorCreation payload) returns ResourceCollaborator|error
Add a new collaborator
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- payload ResourceCollaboratorCreation -
Return Type
- ResourceCollaborator|error - The newly added collaborator metadata
getScoreCollaborator
function getScoreCollaborator(string score, string collaborator, string? sharingKey) returns ResourceCollaborator|error
Get a collaborator
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- collaborator string - Unique identifier of a collaborator permission, or unique identifier of a User, or unique identifier of a Group
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ResourceCollaborator|error - Collaborator information
removeScoreCollaborator
Delete a collaborator
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- collaborator string - Unique identifier of a collaborator permission, or unique identifier of a User, or unique identifier of a Group
listScoreTracks
function listScoreTracks(string score, string? sharingKey, string? assignment, boolean? listAutoTrack) returns ScoreTrack[]|error
List the audio or video tracks linked to a score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
- assignment string? (default ()) - An assignment id with which all the tracks returned will be related to
- listAutoTrack boolean? (default ()) - If true, and if available, return last automatically synchronized Flat's mp3 export as an additional track
Return Type
- ScoreTrack[]|error - List of tracks
addScoreTrack
function addScoreTrack(string score, ScoreTrackCreation payload) returns ScoreTrack|error
Add a new video or audio track to the score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- payload ScoreTrackCreation -
Return Type
- ScoreTrack|error - Created track
getScoreTrack
function getScoreTrack(string score, string track, string? sharingKey) returns ScoreTrack|error
Retrieve the details of an audio or video track linked to a score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- track string - Unique identifier of a score audio track
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ScoreTrack|error - Track details
updateScoreTrack
function updateScoreTrack(string score, string track, ScoreTrackUpdate payload) returns ScoreTrack|error
Update an audio or video track linked to a score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- track string - Unique identifier of a score audio track
- payload ScoreTrackUpdate -
Return Type
- ScoreTrack|error - Updated track
deleteScoreTrack
Remove an audio or video track linked to the score
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- track string - Unique identifier of a score audio track
getScoreComments
function getScoreComments(string score, string? sharingKey, string? 'type, string? sort, string? direction) returns ScoreComment[]|error
List comments
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
- 'type string? (default ()) - Filter the comments by type
- sort string? (default ()) - Sort
- direction string? (default ()) - Sort direction
Return Type
- ScoreComment[]|error - The comments of the score
postScoreComment
function postScoreComment(string score, ScoreCommentCreation payload, string? sharingKey) returns ScoreComment|error
Post a new comment
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- payload ScoreCommentCreation -
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ScoreComment|error - The new comment
updateScoreComment
function updateScoreComment(string score, string comment, ScoreCommentUpdate payload, string? sharingKey) returns ScoreComment|error
Update an existing comment
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- comment string - Unique identifier of a sheet music comment
- payload ScoreCommentUpdate -
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ScoreComment|error - The edited comment
deleteScoreComment
function deleteScoreComment(string score, string comment, string? sharingKey) returns Response|error
Delete a comment
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- comment string - Unique identifier of a sheet music comment
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
markScoreCommentResolved
function markScoreCommentResolved(string score, string comment, string? sharingKey) returns Response|error
Mark the comment as resolved
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- comment string - Unique identifier of a sheet music comment
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
markScoreCommentUnresolved
function markScoreCommentUnresolved(string score, string comment, string? sharingKey) returns Response|error
Mark the comment as unresolved
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- comment string - Unique identifier of a sheet music comment
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
getScoreRevisions
function getScoreRevisions(string score, string? sharingKey) returns ScoreRevision[]|error
List the revisions
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ScoreRevision[]|error - List of revisions
createScoreRevision
function createScoreRevision(string score, ScoreRevisionCreation payload) returns ScoreRevision|error
Create a new revision
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- payload ScoreRevisionCreation -
Return Type
- ScoreRevision|error - The new created revision metadata
getScoreRevision
function getScoreRevision(string score, string revision, string? sharingKey) returns ScoreRevision|error
Get a score revision
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- revision string - Unique identifier of a score revision. You can use
last
to fetch the information related to the last version created.
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ScoreRevision|error - Revision metadata
getScoreRevisionData
function getScoreRevisionData(string score, string revision, string format, string? sharingKey, string? parts, boolean? onlyCached, boolean? url) returns string|error
Get a score revision data
Parameters
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- revision string - Unique identifier of a score revision. You can use
last
to fetch the information related to the last version created.
- format string - The format of the file you will retrieve
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
- parts string? (default ()) - An optional a set of parts uuid to be exported. This parameter must be composed of parts uuids separated by commas. For example "59df645f-bb1c-f1b4-b573-d2afc4491f94,34ef645f-1aef-f3bc-1564-34cca4492b87".
- onlyCached boolean? (default ()) - Only return files already generated and cached in Flat's production cache. If the file is not availabe, a 404 will be returned
- url boolean? (default ()) - Returns a json with the
url
in it instead of redirecting
listCollections
function listCollections(string parent, string? sort, string? direction, int 'limit, string? next, string? previous) returns Collection[]|error
List the collections
Parameters
- parent string (default "root") - List the collection contained in this
parent
collection. This option doesn't provide a complete multi-level collection support. When sharing a collection with someone, this one will have asparent
sharedWithMe
.
- sort string? (default ()) - Sort
- direction string? (default ()) - Sort direction
- 'limit int (default 25) - This is the maximum number of objects that may be returned
- next string? (default ()) - An opaque string cursor to fetch the next page of data. The paginated API URLs are returned in the
Link
header when requesting the API. These URLs will contain anext
andprevious
cursor based on the available data.
- previous string? (default ()) - An opaque string cursor to fetch the previous page of data. The paginated API URLs are returned in the
Link
header when requesting the API. These URLs will contain anext
andprevious
cursor based on the available data.
Return Type
- Collection[]|error - List of collections
createCollection
function createCollection(CollectionCreation payload) returns Collection|error
Create a new collection
Parameters
- payload CollectionCreation -
Return Type
- Collection|error - Collection created
getCollection
function getCollection(string collection, string? sharingKey) returns Collection|error
Get collection details
Parameters
- collection string - Unique identifier of the collection. The following aliases are supported: -
root
: The root collection of the account -sharedWithMe
: Automatically contains new resources that have been shared individually -trash
: Automatically contains resources that have been deleted
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- Collection|error - Collection details
editCollection
function editCollection(string collection, CollectionModification payload) returns Collection|error
Update a collection's metadata
Parameters
- collection string - Unique identifier of the collection. The following aliases are supported: -
root
: The root collection of the account -sharedWithMe
: Automatically contains new resources that have been shared individually -trash
: Automatically contains resources that have been deleted
- payload CollectionModification -
Return Type
- Collection|error - Collection details
deleteCollection
Delete the collection
Parameters
- collection string - Unique identifier of the collection. The following aliases are supported: -
root
: The root collection of the account -sharedWithMe
: Automatically contains new resources that have been shared individually -trash
: Automatically contains resources that have been deleted
untrashCollection
Untrash a collection
Parameters
- collection string - Unique identifier of the collection. The following aliases are supported: -
root
: The root collection of the account -sharedWithMe
: Automatically contains new resources that have been shared individually -trash
: Automatically contains resources that have been deleted
listCollectionScores
function listCollectionScores(string collection, string? sharingKey, string? sort, string? direction, int 'limit, string? next, string? previous) returns ScoreDetails[]|error
List the scores contained in a collection
Parameters
- collection string - Unique identifier of the collection. The following aliases are supported: -
root
: The root collection of the account -sharedWithMe
: Automatically contains new resources that have been shared individually -trash
: Automatically contains resources that have been deleted
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
- sort string? (default ()) - Sort
- direction string? (default ()) - Sort direction
- 'limit int (default 25) - This is the maximum number of objects that may be returned
- next string? (default ()) - An opaque string cursor to fetch the next page of data. The paginated API URLs are returned in the
Link
header when requesting the API. These URLs will contain anext
andprevious
cursor based on the available data.
- previous string? (default ()) - An opaque string cursor to fetch the previous page of data. The paginated API URLs are returned in the
Link
header when requesting the API. These URLs will contain anext
andprevious
cursor based on the available data.
Return Type
- ScoreDetails[]|error - List of scores
addScoreToCollection
function addScoreToCollection(string collection, string score, string? sharingKey) returns ScoreDetails|error
Add a score to the collection
Parameters
- collection string - Unique identifier of the collection. The following aliases are supported: -
root
: The root collection of the account -sharedWithMe
: Automatically contains new resources that have been shared individually -trash
: Automatically contains resources that have been deleted
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
Return Type
- ScoreDetails|error - Score details
deleteScoreFromCollection
function deleteScoreFromCollection(string collection, string score, string? sharingKey) returns Response|error
Delete a score from the collection
Parameters
- collection string - Unique identifier of the collection. The following aliases are supported: -
root
: The root collection of the account -sharedWithMe
: Automatically contains new resources that have been shared individually -trash
: Automatically contains resources that have been deleted
- score string - Unique identifier of the score document. This can be a Flat Score unique identifier (i.e.
ScoreDetails.id
) or, if the score is also a Google Drive file, the Drive file unique identifier prefixed withdrive-
(e.g.drive-0B000000000
).
- sharingKey string? (default ()) - This sharing key must be specified to access to a score or collection with a
privacy
mode set toprivateLink
and the current user is not a collaborator of the document.
getUser
function getUser(string user) returns UserPublic|error
Get a public user profile
Parameters
- user string - This route parameter is the unique identifier of the user. You can specify an email instead of an unique identifier. If you are executing this request authenticated, you can use
me
as a value instead of the current User unique identifier to work on the current authenticated user.
Return Type
- UserPublic|error - The user public details
gerUserLikes
function gerUserLikes(string user, boolean? ids) returns ScoreDetails[]|error
List liked scores
Parameters
- user string - Unique identifier of a Flat user. If you authenticated, you can use
me
to refer to the current user.
- ids boolean? (default ()) - Return only the identifiers of the scores
Return Type
- ScoreDetails[]|error - List of liked scores
getUserScores
function getUserScores(string user, string? parent) returns ScoreDetails[]|error
List user's scores
Parameters
- user string - Unique identifier of a Flat user. If you authenticated, you can use
me
to refer to the current user.
- parent string? (default ()) - Filter the score forked from the score id
parent
Return Type
- ScoreDetails[]|error - The user scores
listOrganizationUsers
function listOrganizationUsers(string[]? sort, string? direction, string? next, string? previous, string[]? role, string? q, string[]? group, boolean? noActiveLicense, string[]? licenseExpirationDate, boolean? onlyIds, int 'limit) returns UserDetailsAdmin[]|error
List the organization users
Parameters
- sort string[]? (default ()) - The order to sort the user list
- direction string? (default ()) - Sort direction
- next string? (default ()) - An opaque string cursor to fetch the next page of data. The paginated API URLs are returned in the
Link
header when requesting the API. These URLs will contain anext
andprevious
cursor based on the available data.
- previous string? (default ()) - An opaque string cursor to fetch the previous page of data. The paginated API URLs are returned in the
Link
header when requesting the API. These URLs will contain anext
andprevious
cursor based on the available data.
- role string[]? (default ()) - Filter users by role
- q string? (default ()) - The query to search
- group string[]? (default ()) - Filter users by group
- noActiveLicense boolean? (default ()) - Filter users who don't have an active license
- licenseExpirationDate string[]? (default ()) - Filter users by license expiration date or
active
/notActive
- onlyIds boolean? (default ()) - Return only user ids
- 'limit int (default 25) - This is the maximum number of objects that may be returned
Return Type
- UserDetailsAdmin[]|error - List of users
createOrganizationUser
function createOrganizationUser(UserCreation payload) returns UserDetailsAdmin|error
Create a new user account
Parameters
- payload UserCreation -
Return Type
- UserDetailsAdmin|error - New user created
countOrgaUsers
function countOrgaUsers(string[]? role, string? q, string[]? group, boolean? noActiveLicense) returns UserDetailsAdmin[]|error
Count the organization users using the provided filters
Parameters
- role string[]? (default ()) - Filter users by role
- q string? (default ()) - The query to search
- group string[]? (default ()) - Filter users by group
- noActiveLicense boolean? (default ()) - Filter users who don't have an active license
Return Type
- UserDetailsAdmin[]|error - Number of users
updateOrganizationUser
function updateOrganizationUser(string user, UserAdminUpdate payload) returns UserDetailsAdmin|error
Update account information
Return Type
- UserDetailsAdmin|error - User updated
removeOrganizationUser
Remove an account from Flat
Parameters
- user string - Unique identifier of the Flat account
- convertToIndividual boolean? (default ()) - If
true
, the account will be only removed from the organization and converted into an individual account on our public website, https://flat.io. This operation will remove the education-related data from the account. Before realizing this operation, you need to be sure that the user is at least 13 years old and that this one has read and agreed to the Individual Terms of Services of Flat available on https://flat.io/legal.
listOrganizationInvitations
function listOrganizationInvitations(string? role, int 'limit, string? next, string? previous) returns OrganizationInvitation[]|error
List the organization invitations
Parameters
- role string? (default ()) - Filter users by role
- 'limit int (default 50) - This is the maximum number of objects that may be returned
- next string? (default ()) - An opaque string cursor to fetch the next page of data. The paginated API URLs are returned in the
Link
header when requesting the API. These URLs will contain anext
andprevious
cursor based on the available data.
- previous string? (default ()) - An opaque string cursor to fetch the previous page of data. The paginated API URLs are returned in the
Link
header when requesting the API. These URLs will contain anext
andprevious
cursor based on the available data.
Return Type
- OrganizationInvitation[]|error - List of invitations
createOrganizationInvitation
function createOrganizationInvitation(OrganizationInvitationCreation payload) returns OrganizationInvitation|error
Create a new invitation to join the organization
Parameters
- payload OrganizationInvitationCreation -
Return Type
- OrganizationInvitation|error - New invitation created
removeOrganizationInvitation
Remove an organization invitation
Parameters
- invitation string - Unique identifier of the invitation
listLtiCredentials
function listLtiCredentials() returns LtiCredentials[]|error
List LTI 1.x credentials
Return Type
- LtiCredentials[]|error - The list of LTI Credentials
createLtiCredentials
function createLtiCredentials(LtiCredentialsCreation payload) returns LtiCredentials|error
Create a new couple of LTI 1.x credentials
Parameters
- payload LtiCredentialsCreation -
Return Type
- LtiCredentials|error - The LTI Credentials
revokeLtiCredentials
Revoke LTI 1.x credentials
Parameters
- credentials string - Credentials unique identifier
listClasses
function listClasses(string state) returns ClassDetails[]|error
List the classes available for the current user
Parameters
- state string (default "active") - Filter the classes by state
Return Type
- ClassDetails[]|error - The list of classes
createClass
function createClass(ClassCreation payload) returns ClassDetails|error
Create a new class
Parameters
- payload ClassCreation -
Return Type
- ClassDetails|error - The new class details
getClass
function getClass(string 'class) returns ClassDetails|error
Get the details of a single class
Parameters
- 'class string - Unique identifier of the class
Return Type
- ClassDetails|error - The new class details
updateClass
function updateClass(string 'class, ClassUpdate payload) returns ClassDetails|error
Update the class
Return Type
- ClassDetails|error - The new class details
archiveClass
function archiveClass(string 'class) returns ClassDetails|error
Archive the class
Parameters
- 'class string - Unique identifier of the class
Return Type
- ClassDetails|error - The class details
unarchiveClass
function unarchiveClass(string 'class) returns ClassDetails|error
Unarchive the class
Parameters
- 'class string - Unique identifier of the class
Return Type
- ClassDetails|error - The class details
activateClass
function activateClass(string 'class) returns ClassDetails|error
Activate the class
Parameters
- 'class string - Unique identifier of the class
Return Type
- ClassDetails|error - The class details
addClassUser
Add a user to the class
deleteClassUser
Remove a user from the class
listClassStudentSubmissions
function listClassStudentSubmissions(string 'class, string user) returns AssignmentSubmission[]|error
List the submissions for a student
Return Type
- AssignmentSubmission[]|error - The list of submissions
listAssignments
function listAssignments(string 'class) returns Assignment[]|error
Assignments listing
Parameters
- 'class string - Unique identifier of the class
Return Type
- Assignment[]|error - List of assignments for the class
createAssignment
function createAssignment(string 'class, AssignmentCreation payload) returns Assignment|error
Assignment creation
Return Type
- Assignment|error - The assignment has been created
copyAssignment
function copyAssignment(string 'class, string assignment, AssignmentCopy payload) returns Assignment|error
Copy an assignment
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- payload AssignmentCopy -
Return Type
- Assignment|error - The new created assingment
archiveAssignment
function archiveAssignment(string 'class, string assignment) returns Assignment|error
Archive the assignment
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
Return Type
- Assignment|error - The assignment details
unarchiveAssignment
function unarchiveAssignment(string 'class, string assignment) returns Assignment|error
Unarchive the assignment.
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
Return Type
- Assignment|error - The assignment details
getSubmissions
function getSubmissions(string 'class, string assignment) returns AssignmentSubmission[]|error
List the students' submissions
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
Return Type
- AssignmentSubmission[]|error - The submissions
createSubmission
function createSubmission(string 'class, string assignment, AssignmentSubmissionUpdate payload) returns AssignmentSubmission|error
Create or edit a submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- payload AssignmentSubmissionUpdate -
Return Type
- AssignmentSubmission|error - The submission
exportSubmissionsReviewsAsCsv
CSV Grades exports
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
exportSubmissionsReviewsAsExcel
Excel Grades exports
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
getSubmission
function getSubmission(string 'class, string assignment, string submission) returns AssignmentSubmission|error
Get a student submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- submission string - Unique identifier of the submission
Return Type
- AssignmentSubmission|error - A submission
editSubmission
function editSubmission(string 'class, string assignment, string submission, AssignmentSubmissionUpdate payload) returns AssignmentSubmission|error
Edit a submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- submission string - Unique identifier of the submission
- payload AssignmentSubmissionUpdate -
Return Type
- AssignmentSubmission|error - The submission
deleteSubmission
function deleteSubmission(string 'class, string assignment, string submission) returns Response|error
Delete a submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- submission string - Unique identifier of the submission
getSubmissionHistory
function getSubmissionHistory(string 'class, string assignment, string submission) returns AssignmentSubmissionHistory[]|error
Get the history of the submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- submission string - Unique identifier of the submission
Return Type
- AssignmentSubmissionHistory[]|error - The history of the submission
getSubmissionComments
function getSubmissionComments(string 'class, string assignment, string submission) returns AssignmentSubmissionComment[]|error
List the feedback comments of a submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- submission string - Unique identifier of the submission
Return Type
- AssignmentSubmissionComment[]|error - The comments of the score
postSubmissionComment
function postSubmissionComment(string 'class, string assignment, string submission, AssignmentSubmissionCommentCreation payload) returns AssignmentSubmissionComment|error
Add a feedback comment to a submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- submission string - Unique identifier of the submission
- payload AssignmentSubmissionCommentCreation -
Return Type
- AssignmentSubmissionComment|error - The comment
updateSubmissionComment
function updateSubmissionComment(string 'class, string assignment, string submission, string comment, AssignmentSubmissionCommentCreation payload) returns AssignmentSubmissionComment|error
Update a feedback comment to a submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- submission string - Unique identifier of the submission
- comment string - Unique identifier of the comment
- payload AssignmentSubmissionCommentCreation -
Return Type
- AssignmentSubmissionComment|error - The comment
deleteSubmissionComment
function deleteSubmissionComment(string 'class, string assignment, string submission, string comment) returns Response|error
Delete a feedback comment to a submission
Parameters
- 'class string - Unique identifier of the class
- assignment string - Unique identifier of the assignment
- submission string - Unique identifier of the submission
- comment string - Unique identifier of the comment
enrollClass
function enrollClass(string enrollmentCode) returns ClassDetails|error
Join a class
Parameters
- enrollmentCode string - The enrollment code, available to the teacher in
ClassDetails
Return Type
- ClassDetails|error - The new class details
getGroupDetails
function getGroupDetails(string group) returns GroupDetails|error
Get group information
Parameters
- group string - Unique identifier of a Flat group
Return Type
- GroupDetails|error - The group details
listGroupUsers
function listGroupUsers(string group, string? 'source) returns UserPublic[]|error
List group's users
Parameters
- group string - Unique identifier of a Flat group
- 'source string? (default ()) - Filter the users by their source
Return Type
- UserPublic[]|error - The list of users member of the group
getGroupScores
function getGroupScores(string group, string? parent) returns ScoreDetails[]|error
List group's scores
Parameters
- group string - Unique identifier of a Flat group
- parent string? (default ()) - Filter the score forked from the score id
parent
Return Type
- ScoreDetails[]|error - The group's scores
Records
flatapi: Assignment
Assignment details
Fields
- state string? - State of the assignment
- 'type AssignmentType? - Type of the assignment
- title string? - Title of the assignment
- classroom string? - The unique identifier of the class where this assignment was posted
- description string? - Description and content of the assignment
- cover string? - The URL of the cover to display
- coverFile string? - The id of the cover to display
- attachments MediaAttachment[]? -
- submissions AssignmentSubmission[]? -
- creator string? - The User unique identifier of the creator of this assignment
- creationDate string? - The creation date of this assignment
- scheduledDate string? - The publication (scheduled) date of the assignment. If this one is specified, the assignment will only be listed to the teachers of the class.
- dueDate string? - The due date of this assignment, late submissions will be marked as paste due.
- maxPoints decimal? - If set, the grading will be enabled for the assignement
- googleClassroom GoogleClassroomCoursework? - A coursework on Google Classroom
- microsoftGraph MicrosoftGraphAssignment? - A Microsoft Teams asignment
- mfc AssignmentMfc? - A MusicFirst Classroom assignment
- canvas AssignmentCanvas? - A Canvas LMS assignment
- lti AssignmentLti? - An LTI assignment
flatapi: AssignmentCanvas
A Canvas LMS assignment
Fields
- id string? - Unique identifier of the course on Canvas assignment
- alternateLink string? - Link to Canvas assignment
flatapi: AssignmentCopy
Assignment copy operation
Fields
- classroom string? - The destination classroom where the assignment will be copied
- assignment string? - An optional destination assignment where the original assignement will be copied. Must be a draft.
- scheduledDate string? - The publication (scheduled) date of the assignment.
If this one is specified, the assignment will only be listed to the teachers of the class.
Alternatively the existing
scheduledDate
from the copied assignment will be used.
flatapi: AssignmentCreation
Assignment creation details
Fields
- 'type AssignmentType? - Type of the assignment
- state string? - State of the assignment
- title string? - Title of the assignment
- description string? - Description and content of the assignment
- attachments ClassAttachmentCreation[]? -
- dueDate string? - The due date of this assignment, late submissions will be marked as paste due. If not set, the assignment won't have a due date.
- scheduledDate string? - The publication (scheduled) date of the assignment. If this one is specified, the assignment will only be listed to the teachers of the class.
- nbPlaybackAuthorized decimal? - The number of playback authorized on the scores of the assignment.
- toolset string? - The id of the associated toolset
- coverFile string? - The id of the cover to display
- cover string? - The URL of the cover to display
- maxPoints decimal? - If set, the grading will be enabled for the assignement with this value as the maximum of points
- googleClassroom AssignmentcreationGoogleclassroom? - Google Classroom options for this assignment
- microsoftGraph AssignmentcreationMicrosoftgraph? - Microsoft Graph options for this assignment
- assigneeMode string? - Possible modes of assigning assignments
- assignedStudents string[]? - Identifiers for the students that have access to the assignment
flatapi: AssignmentcreationGoogleclassroom
Google Classroom options for this assignment
Fields
- topicId string? - Identifier of the topic where the assignment is created
flatapi: AssignmentcreationMicrosoftgraph
Microsoft Graph options for this assignment
Fields
- categories string[]? - List of categories this assignment belongs to
flatapi: AssignmentLti
An LTI assignment
Fields
- id string? - Resource ID in the LMS
flatapi: AssignmentMfc
A MusicFirst Classroom assignment
Fields
- id string? - Unique identifier of the course on MusicFirst Task
- alternateLink string? - Link to MusicFirst Classroom task
flatapi: AssignmentSubmission
Assignment Submission
Fields
- id string? - Unique identifier of the submission
- state AssignmentSubmissionState? - State of the submission
- classroom string? - Unique identifier of the classroom where the assignment was posted
- assignment string? - Unique identifier of the assignment
- creator string? - The User identifier of the student who created the submission
- creationDate string? - The date when the submission was created
- attachments MediaAttachment[]? -
- submissionDate string? - The date when the student submitted his work
- returnDate string? - The date when the teacher returned the work
- returnCreator string? - The User unique identifier of the teacher who returned the submission
- grade decimal? - Optional grade. If unset, no grade was set.
- draftGrade decimal? - Optional grade. If unset, no grade was set. This value is only visible by the teacher, and we will be set to
grade
once the teacher returns the submission
- maxPoints decimal? - Optional max points for the grade. If set, a corresponding
draftGrade
orgrade
will be set.
- googleClassroom GoogleClassroomSubmission? - A coursework submission on Google Classroom
- microsoftGraph MicrosoftGraphSubmission? - A Microsoft Teams submission
flatapi: AssignmentSubmissionComment
Feedback comment added to an assignment submission
Fields
- id string? - The comment unique identifier
- user string? - The author unique identifier
- submission string? - The submission unique identifier
- date string? - The date when the comment was posted
- modificationDate string? - The date of the last comment modification
- comment string? - The comment text
- unread boolean? - True if the comment is unread by the current user
flatapi: AssignmentSubmissionCommentCreation
Creation of a assignment submission comment
Fields
- comment string - The comment text
flatapi: AssignmentSubmissionHistory
History item of the submission
Fields
- date string? - The date when the submission was changed
- users string[]? - The user(s) unique identifier(s) who made the change
- state AssignmentSubmissionState? - State of the submission
- draftGrade decimal? - The numerator of the grade at this time in the submission grade history
- grade decimal? - The numerator of the grade at this time in the submission grade history
- maxPoints decimal? - The denominator of the grade at this time in the submission grade history
- attachment AssignmentsubmissionhistoryAttachment? -
flatapi: AssignmentsubmissionhistoryAttachment
Fields
- score string? - The score identifier that changed
- revision string? - The revision identifier that changed
flatapi: AssignmentSubmissionUpdate
Assignment Submission creation
Fields
- attachments ClassAttachmentCreation[]? -
- submit boolean? - If
true
, the submission will be marked as done
- draftGrade decimal? - Optional grade. If unset, no grade was set. This value is only visible by the teacher, and we will be set to
grade
once the teacher returns the submission
- grade decimal? - Optional grade. If unset, no grade was set.
- 'return boolean? - If
true
, the submission will be marked as done
- comments AssignmentsubmissionupdateComments? -
flatapi: AssignmentsubmissionupdateComments
Fields
- total decimal? - The total number of comments added to the submission
- unread decimal? - The number of unread comments for the current user
flatapi: ClassAttachmentCreation
Attachment creation for an assignment or stream post.
This attachment must contain a score
or an url
, all the details of this one will be resolved and returned as ClassAttachment
once the assignment or stream post is created.
Fields
- 'type string? - The type of the attachment posted
- score string? - A unique Flat score identifier. The user creating the assignment must at least have read access to the document. If the user has admin rights, new group permissions will be automatically added for the teachers and students of the class.
- worksheet string? - An unique worksheet identifier
- sharingMode MediaScoreSharingMode? - The sharing mode of the score for classes post and assignments
- lockScoreTemplate boolean? - To be used with a score attached in
sharingMode
copy
(score used as template). If true, students won't be able to change the original notes of the template.
- url string? - The URL of the attachment.
- googleDriveFileId string? - The ID of the Google Drive File
flatapi: ClassCreation
Creation of a classroom
Fields
- name string - The name of the new class
- section string? - The section of the new class
flatapi: ClassDetails
A classroom
Fields
- id string? - The unique identifier of the class
- state ClassState? - The state of a classroom
- name string? - The name of the class
- section string? - The section of the class
- description string? - An optionnal description for this class
- organization string? - The unique identifier of the Organization owning this class
- owner string? - The unique identifier of the User owning this class
- creationDate string? - The date when the class was create
- enrollmentCode string? - [Teachers only] The enrollment code that can be used by the students to join the class
- theme string? - The theme identifier using in Flat User Interface
- assignmentsCount decimal? - The number of assignments created in the class
- studentsGroup GroupDetails? - The details of a group
- teachersGroup GroupDetails? - The details of a group
- issues ClassdetailsIssues? - Detected issues for this class
- googleClassroom ClassdetailsGoogleclassroom? - Google Classroom course-related information
- googleDrive ClassdetailsGoogledrive? - Google Drive course-related information provided by Google Classroom
- microsoftGraph ClassdetailsMicrosoftgraph? -
- lti ClassdetailsLti? - Meta information provided by the LTI consumer
- canvas ClassdetailsCanvas? - Meta information provided by Canvs LMS
- mfc ClassdetailsMfc? - Meta information provided by Canvs LMS
- clever ClassdetailsClever? - Clever.com section-related information
flatapi: ClassdetailsCanvas
Meta information provided by Canvs LMS
Fields
- id string? - Unique identifier of the course on Canvas
- domain string? - Canvas instance domain (e.g. "canvas.instructure.com")
flatapi: ClassdetailsClever
Clever.com section-related information
Fields
- id string? - Clever section unique identifier
- creationDate string? - The creation date of the section on clever
- modificationDate string? - The last modification date of the section on clever
- subject string? - Normalized subject of the course
- termName string? - Name of the term when this course happens
- termStartDate string? - Beginning date of the term
- termEndDate string? - End date of the term
flatapi: ClassdetailsGoogleclassroom
Google Classroom course-related information
Fields
- id string? - The course identifier on Google Classroom
- alternateLink string? - Absolute link to this course in the Classroom web UI
flatapi: ClassdetailsGoogledrive
Google Drive course-related information provided by Google Classroom
Fields
- teacherFolderId string? - [Teachers only] The Drive directory identifier of the teachers' folder
- teacherFolderAlternateLink string? - [Teachers only] The Drive URL of the teachers' folder
flatapi: ClassdetailsIssues
Detected issues for this class
Fields
- sync ClassdetailsIssuesSync[]? - Synchronization issues for the class
flatapi: ClassdetailsIssuesSync
A sync issue
Fields
- id string? - The account user identifier
- email string? - The email address of the user concerned by this sync issue
- reason string? - The reason why the account cannot be synced
flatapi: ClassdetailsLti
Meta information provided by the LTI consumer
Fields
- contextId string? - Unique context identifier provided
- contextTitle string? - Context title
- contextLabel string? - Context label
flatapi: ClassdetailsMfc
Meta information provided by Canvs LMS
Fields
- id string? - Unique identifier of the course on MusicFirst Classroom
- alternateLink string? - Link to MusicFirst Classroom class
flatapi: ClassdetailsMicrosoftgraph
Fields
- id string? - The course identifier on Microsoft Graph
flatapi: ClassUpdate
Update of a classroom
Fields
- name string? - The name of the class
- section string? - The section of the class
flatapi: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
flatapi: Collection
Collection of scores
Fields
- id string? - Unique identifier of the collection
- title string? - The title of the collection
- htmlUrl string? - The url where the collection can be viewed in a web browser
- 'type CollectionType? - Type of the collection that influence the capabilitied available on the collections and how this collection is/can be populated.
- privacy CollectionPrivacy? - The collection main privacy mode(private).
- sharingKey string? - The private sharing key of the collection (available when the
privacy
mode is set toprivateLink
)
- app string? - If this directory is dedicated to an app, the unique idenfier of this app
- creationDate string? - The date when the collection was created
- user UserPublicSummary? - Public User details summary
- rights ResourceRights? - The rights of the current user on a score or collection
- collaborators ResourceCollaborator[]? - The list of the collaborators of the collection
- capabilities CollectionCapabilities? - Capabilities the current user has on this collection. Each capability corresponds to a fine-grained action that a user may take.
- collections string[]? - The List of parent collections, which includes all the collections this score is included. Please note that you might not have access to all of them.
flatapi: CollectionCapabilities
Capabilities the current user has on this collection. Each capability corresponds to a fine-grained action that a user may take.
Fields
- canEdit boolean? - Whether the current user can modify the metadata for the collection
- canShare boolean? - Whether the current user can modify the sharing settings for the collection
- canDelete boolean? - Whether the current user can delete the collection
- canAddScores boolean? - Whether the current user can add scores to the collection
If this collection has the
type
trash
, this property will be set tofalse
. UseDELETE /v2/scores/{score}
to trash a score.
- canDeleteScores boolean? - Whether the current user can delete scores from the collection
If this collection has the
type
trash
, this property will be set tofalse
. UsePOST /v2/scores/{score}/untrash
to restore a score.
flatapi: CollectionCreation
Fields
- title string - The title of the collection
- privacy CollectionPrivacy - The collection main privacy mode(private).
flatapi: CollectionModification
Edit the collection metadata
Fields
- title string? - The title of the collection
- privacy CollectionPrivacy? - The collection main privacy mode(private).
flatapi: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
flatapi: FlatErrorResponse
An API Error response
Fields
- code string? - A corresponding code for this error
- message string? - A printable message for this message
- id string? - An unique error identifier generated for the request
- param string? - The related parameter that caused the error
flatapi: GoogleClassroomCoursework
A coursework on Google Classroom
Fields
- id string? - Identifier of the coursework assigned by Classroom
- state string? - State of the coursework
- alternateLink string? - Absolute link to this coursework in the Classroom web UI
- topicId string? - Identifier of the topic where the assignment is created
flatapi: GoogleClassroomSubmission
A coursework submission on Google Classroom
Fields
- id string? - Identifier of the coursework submission assigned by Classroom
- state string? - State of the submission on Google Classroom
- alternateLink string? - Absolute link to this coursework in the Classroom web UI
flatapi: Group
A group of users
Fields
- id string? - The unique identifier of the group
- name string? - The display name of the group
- 'type string? - The type of the group:
generic
: A group created by a Flat userclassTeachers
: A group created automaticaly by Flat that contains the teachers of a classclassStudents
: A group created automaticaly by Flat that contains the studnets of a class
- usersCount decimal? - The number of users in this group
- readOnly boolean? -
True
if the group is set in read-only
- organization string? - If the group is related to an organization, this field will contain the unique identifier of the organization
- creationDate string? - The creation date of the group
flatapi: GroupDetails
The details of a group
Fields
- id string? - The unique identifier of the group
- name string? - The displayable name of the group
- 'type GroupType? - The type of the group
- organization string? - The unique identifier of the Organization owning the group
- creationDate string? - The date when the group was create
- usersCount decimal? - The number of students in this group
- readOnly boolean? -
true
if the properties and members of this group are in in read-only
flatapi: LtiCredentials
A couple of LTI 1.x OAuth credentials
Fields
- id string? - The unique identifier of this couple of credentials
- name string? - Name of the couple of credentials
- lms LmsName? - LMS name
- organization string? - The unique identifier of the Organization associated to these credentials
- creator string? - Unique identifier of the user who created these credentials
- creationDate string? - The creation date of thse credentials
- lastUsage string? - The last time these credentials were used
- consumerKey string? - OAuth 1 Consumer Key
- consumerSecret string? - OAuth 1 Consumer Secret
flatapi: LtiCredentialsCreation
Creation of a couple of LTI 1.x OAuth credentials
Fields
- name string - Name of the couple of credentials
- lms LmsName - LMS name
flatapi: MediaAttachment
Media attachment. The API will automatically resolve the details, oEmbed, and media available if possible and return them in this object
Fields
- 'type string? - The type of the assignment resolved:
rich
,photo
,video
are attachment types that are automatically resolved from alink
attachment.- A
flat
attachment is a score document where the unique identifier will be specified in thescore
property. Its sharing mode will be provided in thesharingMode
property.
- score string? - An unique Flat score identifier
- revision string? - An unique revision identifier of a score
- worksheet string? - An unique worksheet identifier
- track string? - A unique track identifier
- sharingMode MediaScoreSharingMode? - The sharing mode of the score for classes post and assignments
- lockScoreTemplate boolean? - To be used with a score attached in
sharingMode
copy
(score used as template). If true, students won't be able to change the original notes of the template.
- title string? - The resolved title of the attachment
- description string? - The resolved description of the attachment
- html string? - If the attachment type is
rich
orvideo
, the HTML code of the media to display
- htmlWidth string? - If the
html
is available, the width of the widget
- htmlHeight string? - If the
html
is available, the height of the widget
- url string? - The url of the attachment
- thumbnailUrl string? - If the attachment type is
rich
,video
,photo
orlink
, a displayable thumbnail for this attachment
- thumbnailWidth int? - If the
thumbnailUrl
is available, the width of the thumbnail
- thumbnailHeight int? - If the
thumbnailUrl
is available, the width of the thumbnail
- authorName string? - The resolved author name of the attachment
- authorUrl string? - The resolved author url of the attachment
- iconUrl string? - The URL of the icon
- mimeType string? - The mine type of the file
- googleDriveFileId string? - The ID of the Google Drive File
flatapi: MicrosoftGraphAssignment
A Microsoft Teams asignment
Fields
- id string? - Identifier of the assignement assigned by Microsoft Teams
- state string? - State of the assignment
- alternateLink string? - Absolute link to this assignement in the Microsoft Teams web UI
- categories string[]? - List of categories where this assignment is published under
flatapi: MicrosoftGraphSubmission
A Microsoft Teams submission
Fields
- id string? - Identifier of the submission assigned by Microsoft Teams
- state string? - State of the submission
- alternateLink string? - Absolute link to this submission in the Microsoft Teams web UI
flatapi: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://api.flat.io/oauth/access_token") - Refresh URL
flatapi: OrganizationInvitation
Details of an invitation to join an organization
Fields
- id string? - The invitation unique identifier
- organization string? - The unique identifier of the Organization owning this class
- organizationRole OrganizationRoles? - User's Organization Role (for Edu users only)
- customCode string? - Enrollment code to use when joining this organization
- email string? - The email address this invitation was sent to
- invitedBy string? - The unique identifier of the User who created this invitation
- usedBy string? - The unique identifier of the User who used this invitation
flatapi: OrganizationInvitationCreation
The parameters to create an organization invitation
Fields
- email string? - The email address you want to send the invitation to
- organizationRole OrganizationRoles? - User's Organization Role (for Edu users only)
flatapi: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
flatapi: ResourceCollaborator
A collaborator of a score. The userEmail
and group
are only available if the requesting user is a collaborator of the related score (in this case these permissions will eventualy not be listed and exposed publicly).
Fields
- Fields Included from *ResourceRights
- id string? - The unique identifier of the permission
- score string? - If this object is a permission of a score, this property will contain the unique identifier of the score
- collection string? - If this object is a permission of a collection, this property will contain the unique identifier of the collection
- user UserPublic? - Public User details
- group Group? - A group of users
- userEmail string? - If the collaborator is not a user of Flat yet, this field will contain his email.
- invited boolean? - If this property is
true
, this is still a pending invitation
flatapi: ResourceCollaboratorCreation
Add a collaborator to a resource.
Fields
- user string? - The unique identifier of a Flat user
- group string? - The unique identifier of a Flat group
- userEmail string? - Fill this field to invite an individual user by email.
- userToken string? - Token received in an invitation to join the score.
- aclRead boolean? -
True
if the related user can read the score. (probably true if the user has a permission on the document).
- aclWrite boolean? -
True
if the related user can modify the score.
- aclAdmin boolean? -
True
if the related user can can manage the current document, i.e. changing the document permissions and deleting the document
flatapi: ResourceRights
The rights of the current user on a score or collection
Fields
- aclRead boolean? -
True
if the current user can read the current document
- aclWrite boolean? -
True
if the current user can modify the current document. If this is a right of a Collection, the capabilities of the associated user can be lower than this permission, check out thecapabilities
property as the end-user to have the complete possibilities with the collection.
- aclAdmin boolean? -
True
if the current user can manage the current document (i.e. share, delete) If this is a right of a Collection, the capabilities of the associated user can be lower than this permission, check out thecapabilities
property as the end-user to have the complete possibilities with the collection.
- isCollaborator boolean? -
True
if the current user is a collaborator of the current document (direct or via group).
flatapi: ScoreComment
Comment added on a sheet music
Fields
- id string? - The comment unique identifier
- 'type string? - The type of the comment
- user string? - The author unique identifier
- score string? - The unique identifier of the score where the comment was posted
- revision string? - The unique identifier of revision the comment was posted
- replyTo string? - When the comment is a reply to another comment, the unique identifier of the parent comment
- date string? - The date when the comment was posted
- modificationDate string? - The date of the last comment modification
- comment string? - The comment text that can includes mentions using the following
format:
@[id:username]
.
- rawComment string? - A raw version of the comment, that can be displayed without parsing the mentions.
- context ScoreCommentContext? - The context of the comment (for inline/contextualized comments). A context will include all the information related to the location of the comment (i.e. score parts, range of measure, time position).
- mentions string[]? - The list of user identifier mentioned on the score
- resolved boolean? - For inline comments, the comment can be marked as resolved and will be hidden in the future responses
- resolvedBy string? - If the user is marked as resolved, this will contain the unique identifier of the User who marked this comment as resolved
- spam boolean? -
true if the message has been detected as spam and hidden from other users MISSING[
]
flatapi: ScoreCommentContext
The context of the comment (for inline/contextualized comments). A context will include all the information related to the location of the comment (i.e. score parts, range of measure, time position).
Fields
- partUuid string - The unique identifier (UUID) of the score part
- staffIdx decimal? - (Deprecated, use
staffUuid
) The identififer of the staff
- staffUuid string? - The unique identififer (UUID) of the staff
- measureUuids string[] - The list of measure UUIds
- startTimePos decimal -
- stopTimePos decimal -
- startDpq decimal -
- stopDpq decimal -
flatapi: ScoreCommentCreation
Creation of a comment
Fields
- revision string? - The unique indentifier of the revision of the score where the comment was added. If this property is unspecified or contains "last", the API will automatically take the last revision created.
- comment string - The comment text that can includes mentions using the following format:
@[id:username]
.
- rawComment string? - A raw version of the comment, that can be displayed without the mentions. If you use mentions, this property must be set.
- mentions string[]? - The list of user identifiers mentioned in this comment
- replyTo string? - When the comment is a reply to another comment, the unique identifier of the parent comment
- context ScoreCommentContext? - The context of the comment (for inline/contextualized comments). A context will include all the information related to the location of the comment (i.e. score parts, range of measure, time position).
flatapi: ScoreCommentsCounts
A computed version of the total, unique, weekly and monthly number of comments added on the documents (this doesn't include inline comments).
Fields
- total decimal? - The total number of comments added to the score
- unique decimal? - The unique (1/user) number of comments added to the score
- weekly decimal? - The weekly unique number of comments added to the score
- monthly decimal? - The monthly unique number of comments added to the score
flatapi: ScoreCommentUpdate
Update of a comment
Fields
- revision string? - The unique indentifier of the revision of the score where the comment was added. If this property is unspecified or contains "last", the API will automatically take the last revision created.
- comment string? - The comment text that can includes mentions using the following format:
@[id:username]
.
- rawComment string? - A raw version of the comment, that can be displayed without the mentions. If you use mentions, this property must be set.
- context ScoreCommentContext? - The context of the comment (for inline/contextualized comments). A context will include all the information related to the location of the comment (i.e. score parts, range of measure, time position).
flatapi: ScoreCreation
A new created score
Fields
- title string? - The title of the new score. If the title is too long, the API may trim this one.
If this title is not specified, the API will try to (in this order):
- Use the title contained in the file (e.g.
movement-title
orcredit-words
for MusicXML files). - Use the name of the file for files from a specified
source
(e.g. Google Drive) or the one in thefilename
property - Set a default title (e.g. "New Music Score")
- Use the title contained in the file (e.g.
- filename string? - If this is an imported file, its filename
- privacy ScorePrivacy - The score main privacy mode(public, private, privateLink and organizationPublic).
- data string? - The data of the score file. It must be a MusicXML 3 file (
vnd.recordare.musicxml
orvnd.recordare.musicxml+xml
), a MIDI file (audio/midi
) or a Flat.json (aka Adagio.json) file. Binary payloads (vnd.recordare.musicxml
andaudio/midi
) can be encoded in Base64, in this case thedataEncoding
property must match the encoding used for the API request.
- dataEncoding string? - The optional encoding of the score data. This property must match the encoding used for the
data
property.
- 'source ScoreSource? -
- collection string? - Unique identifier of a collection where the score will be created.
If no collection identifier is provided, the score will be stored in the
root
directory.
- googleDriveFolder string? - If the user uses Google Drive and this properties is specified, the file will be created in this directory. The currently user creating the file must be granted to write in this directory.
flatapi: ScoreDetails
The score and all its details
Fields
- Fields Included from *ScoreSummary
- id string
- sharingKey string
- title string
- privacy ScorePrivacy
- user UserPublicSummary
- htmlUrl string
- anydata...
- subtitle string? - Subtitle of the score
- lyricist string? - Lyricist of the score
- arranger string? - Arranger of the score
- composer string? - Composer of the score
- description string? - Description of the creation
- tags string[]? - Tags describing the score
- creationType ScoreCreationType? - The type of creation (an orginal, an arrangement)
- license ScoreLicense? - License of the creation. Read more about the Creative Commons licenses on https://creativecommons.org/licenses/
- licenseText string? - Additional license text written on the exported/printed score
- durationTime decimal? - In seconds, an approximative duration of the score
- numberMeasures int? - The number of measures in the score
- mainTempoQpm decimal? - The main tempo of the score (in QPM)
- rights ResourceRights? - The rights of the current user on a score or collection
- collaborators ResourceCollaborator[]? - The list of the collaborators of the score
- creationDate string? - The date when the score was created
- modificationDate string? - The date of the last revision of the score
- publicationDate string? - The date when the score was published on Flat
- organization string? - If the score has been created in an organization, the identifier of this organization. This property is especially used with the score privacy
organizationPublic
.
- parentScore string? - If the score has been forked, the unique identifier of the parent score.
- instruments string[]? - An array of the instrument identifiers used in the last version of the score. This is mainly used to display a list of the instruments in the Flat's UI or instruments icons. The format of the strings is
{instrument-group}.{instrument-id}
.
- samples string[]? - An array of the audio samples identifiers used the different score parts.
The format of the strings is
{instrument-group}.{sample-id}
.
- googleDriveFileId string? - If the user uses Google Drive and the score exists on Google Drive, this field will contain the unique identifier of the Flat score on Google Drive. You can access the document using the url:
https://drive.google.com/open?id={googleDriveFileId}
- likes ScoreLikesCounts? - A computed version of the weekly, monthly and total of number of likes for a score
- comments ScoreCommentsCounts? - A computed version of the total, unique, weekly and monthly number of comments added on the documents (this doesn't include inline comments).
- views ScoreViewsCounts? - A computed version of the total, weekly, and monthly number of views of the score
- plays ScorePlaysCounts? - A computed version of the total, weekly, and monthly number of plays of the score
- collections string[]? - The List of parent collections, which includes all the collections this score is included. Please note that you might not have access to all of them.
flatapi: ScoreFork
Options to fork the score
Fields
- collection string? - Unique identifier of a collection where the score will be copied.
If no collection identifier is provided, the score will be stored in the
root
directory.
flatapi: ScoreLikesCounts
A computed version of the weekly, monthly and total of number of likes for a score
Fields
- total decimal? - The total number of likes of the score
- weekly decimal? - The number of new likes during the last week
- monthly decimal? - The number of new likes during the last month
flatapi: ScoreModification
Edit the score metadata
Fields
- title string? - The title of the score
- subtitle string? - The subtitle of the score
- composer string? - The composer of the score
- lyricist string? - The lyricist of the score
- arranger string? - The arranger of the score
- privacy ScorePrivacy? - The score main privacy mode(public, private, privateLink and organizationPublic).
- sharingKey string? - When using the
privacy
modeprivateLink
, this property can be used to set a custom sharing key, otherwise a new key will be generated.
- description string? - Description of the creation
- tags string[]? - Tags describing the score
- creationType ScoreCreationType? - The type of creation (an orginal, an arrangement)
- license ScoreLicense? - License of the creation. Read more about the Creative Commons licenses on https://creativecommons.org/licenses/
- licenseText string? - The rights info written on the score
flatapi: ScorePlaysCounts
A computed version of the total, weekly, and monthly number of plays of the score
Fields
- total decimal? - The total number of plays of the score
- weekly decimal? - The weekly number of plays of the score
- monthly decimal? - The monthly number of plays of the score
flatapi: ScoreRevision
A score revision metadata
Fields
- id string? - The unique identifier of the revision.
- user string? - The user identifier who created the revision
- collaborators string[]? -
- creationDate string? - The date when this revision was created
- event string? - The last event (action id) of the revision
- description string? - A description associated to the revision
- autosave boolean? - True if this revision was automatically generated by Flat and not on purpose by the user.
- statistics ScoreRevisionStatistics? - The statistics related to the score revision (additions and deletions)
flatapi: ScoreRevisionCreation
A new created revision
Fields
- data string - The data of the score file. It must be a MusicXML 3 file (
vnd.recordare.musicxml
orvnd.recordare.musicxml+xml
), a MIDI file (audio/midi
) or a Flat.json (aka Adagio.json) file. Binary payloads (vnd.recordare.musicxml
andaudio/midi
) can be encoded in Base64, in this case thedataEncoding
property must match the encoding used for the API request.
- dataEncoding string? - The optional encoding of the score data. This property must match the encoding used for the
data
property.
- autosave boolean? - Must be set to
true
if the revision was created automatically.
- description string? - A description associated to the revision
flatapi: ScoreRevisionStatistics
The statistics related to the score revision (additions and deletions)
Fields
- additions decimal? - The number of additions operations in the last revision
- deletions decimal? - The number of deletions operations in the last revision
flatapi: ScoreSource
Fields
- googleDrive string? - If the score is a file on Google Drive, this field property must contain its identifier. To use this method, the Drive file must be public or the Flat Drive App must have access to the file.
flatapi: ScoreSummary
A summary of the score details
Fields
- id string? - The unique identifier of the score
- sharingKey string? - The private sharing key of the score (available when the
privacy
mode is set toprivateLink
)
- title string? - The title of the score
- privacy ScorePrivacy? - The score main privacy mode(public, private, privateLink and organizationPublic).
- user UserPublicSummary? - Public User details summary
- htmlUrl string? - The url where the score can be viewed in a web browser
flatapi: ScoreTrack
An audio track for a score
Fields
- id string? - The unique identifier of the score track
- title string? - Title of the track
- score string? - The unique identifier of the score
- creator string? - The unique identifier of the track creator
- creationDate string? - The creation date of the track
- modificationDate string? - The modification date of the track
- default boolean? - True if the track should be used as default audio source
- state ScoreTrackState? - State of the track
- 'type ScoreTrackType? - The type of an audio track
- url string? - The URL of the track
- mediaId string? - The unique identifier of the track when hosted on an external service.
For example, if the url is
https://www.youtube.com/watch?v=dQw4w9WgXcQ
,mediaId
will bedQw4w9WgXcQ
- synchronizationPoints ScoreTrackPoint[]? -
flatapi: ScoreTrackCreation
Creation of a new track. This one must contain the URL of the track or the corresponding file
Fields
- title string? - Title of the track
- default boolean? - True if the track should be used as default audio source
- state ScoreTrackState? - State of the track
- url string? - The URL of the track
- synchronizationPoints ScoreTrackPoint[]? -
flatapi: ScoreTrackPoint
A track synchronization point
Fields
- 'type string - The type of the synchronization point. If the type is
measure
, the measure uuid must be present inmeasureUuid
- measureUuid string? - The measure unique identifier
- time decimal - The corresponding time in seconds
flatapi: ScoreTrackUpdate
Update an existing track.
Fields
- title string? - Title of the track
- default boolean? - True if the track should be used as default audio source
- state ScoreTrackState? - State of the track
- synchronizationPoints ScoreTrackPoint[]? -
flatapi: ScoreViewsCounts
A computed version of the total, weekly, and monthly number of views of the score
Fields
- total decimal? - The total number of views of the score
- weekly decimal? - The weekly number of views of the score
- monthly decimal? - The monthly number of views of the score
flatapi: UserAdminUpdate
User update as an organization admin
Fields
- password string? - Password of the account
- organizationRole OrganizationRoles? - User's Organization Role (for Edu users only)
- username string? - Username of the account
- firstname string? - First name of the user
- lastname string? - Last name of the user
- email string? - Email of the account
flatapi: UserBasics
Fields
- id string? - The user unique identifier
- 'type string? - The type of user account
- username string? - The user name (unique for the organization)
- printableName string? - The name that can be directly printed (name, firstname & lastname, or username)
- firstname string? - Firstname of the user (for education users)
- lastname string? - Lastname of the user (for education users)
- name string? - A displayable name for the user (for consumer users)
- picture string? - The URL of the picture to display
- isPowerUser boolean? - User license status. 'true' if user is an individual Power user
- isFlatTeam boolean? - Will be 'true' if user is part of the Flat Team
flatapi: UserCreation
User creation
Fields
- username string - Username of the new account
- firstname string? - First name of the user
- lastname string? - Last name of the user
- email string? - Email of the new account
- password string - Password of the new account
- locale FlatLocales? - The user language
flatapi: UserDetails
User details
Fields
- Fields Included from *UserPublic
- bio string
- registrationDate string
- likedScoresCount int
- followersCount int
- followingCount int
- ownedPublicScoresCount int
- coverPicture string
- profileTheme string
- instruments string[]
- organization string
- organizationRole string|()
- classRole string|()
- htmlUrl string
- id string
- type string
- username string
- printableName string
- firstname string
- lastname string
- name string
- picture string|()
- isPowerUser boolean
- isFlatTeam boolean
- anydata...
- id string? - Identifier of the user
- 'type string? - The type of account
- privateProfile boolean? - Tell either this user profile is private or not (individual accounts only)
- locale FlatLocales? - The user language
- pictureFile string? - The ID of the user profile picture
- coverPictureFile string? - The ID of the user profile cover picture
flatapi: UserDetailsAdmin
User details (view for organization teacher / admin)
Fields
- Fields Included from *UserPublicSummary
- email string? - Email of the user
- lastActivityDate string? - Date of the last user activity
- license UserdetailsadminLicense? - Current active license of the user
flatapi: UserdetailsadminLicense
Current active license of the user
Fields
- id string? - ID of the current license
- expirationDate string? - Date when the license expires
- 'source LicenseSources? - Source of the license
- mode LicenseMode? - Mode of the license
- active boolean? - ID of the current license
flatapi: UserPublic
Public User details
Fields
- Fields Included from *UserPublicSummary
- bio string? - User's biography
- registrationDate string? - Date the user signed up
- likedScoresCount int? - Number of the scores liked by the user
- followersCount int? - Number of followers the user have
- followingCount int? - Number of people the user follow
- ownedPublicScoresCount int? - Number of public scores the user have
- coverPicture string? - Cover picture (backgroud) for the profile
- profileTheme string? - Theme (background) for the profile
- instruments string[]? - An array of the instrument identifiers.
The format of the strings is
{instrument-group}.{instrument-id}
.
flatapi: UserPublicSummary
Public User details summary
Fields
- Fields Included from *UserBasics
- organization string? - Organization ID (for Edu users only)
- organizationRole OrganizationRoles? - User's Organization Role (for Edu users only)
- classRole ClassRoles? - User's Class Role (for Edu users only)
- htmlUrl string? - Link to user profile (for Indiv. users only)
String types
flatapi: AssignmentSubmissionState
AssignmentSubmissionState
State of the submission
flatapi: AssignmentType
AssignmentType
Type of the assignment
flatapi: ClassState
ClassState
The state of a classroom
flatapi: CollectionPrivacy
CollectionPrivacy
The collection main privacy mode(private).
flatapi: CollectionType
CollectionType
Type of the collection that influence the capabilitied available on the collections and how this collection is/can be populated.
flatapi: FlatLocales
FlatLocales
The user language
flatapi: GroupType
GroupType
The type of the group
flatapi: LicenseMode
LicenseMode
Mode of the license
flatapi: LicenseSources
LicenseSources
Source of the license
flatapi: LmsName
LmsName
LMS name
flatapi: MediaScoreSharingMode
MediaScoreSharingMode
The sharing mode of the score for classes post and assignments
flatapi: ScoreCreationType
ScoreCreationType
The type of creation (an orginal, an arrangement)
flatapi: ScoreLicense
ScoreLicense
License of the creation. Read more about the Creative Commons licenses on https://creativecommons.org/licenses/
flatapi: ScorePrivacy
ScorePrivacy
The score main privacy mode(public, private, privateLink and organizationPublic).
flatapi: ScoreTrackState
ScoreTrackState
State of the track
flatapi: ScoreTrackType
ScoreTrackType
The type of an audio track
Import
import ballerinax/flatapi;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Lifestyle & Entertainment/News & Lifestyle
Cost/Freemium
Contributors