figshare
Module figshare
API
Definitions
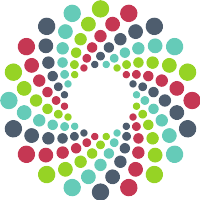
ballerinax/figshare Ballerina library
Overview
This is a generated connector for Figshare API v2.0.0 OpenAPI specification. Figshare is a repository where users can make all of their research outputs available in a citable, shareable and discoverable manner.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Figshare account
- Obtain tokens - Follow this link
Quickstart
To use the Figshare connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/figshare module into the Ballerina project.
import ballerinax/figshare;
Step 2: Create a new connector instance
You can now enter the credentials in the Figshare client configuration and create Figshare client by passing the configuration:
configurable http:BearerTokenConfig & readonly auth = ?; figshare:ClientConfig clientConfig = {auth : auth}; figshare:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
- You can get public articles as follows with
listPublicArticles
method.figshare:Article[] articles = check baseClient->listPublicArticles();
- Use
bal run
command to compile and run the Ballerina program.
Clients
figshare: Client
This is a generated connector for Figshare API v2.0.0 OpenAPI specification. Figshare is a repository where users can make all of their research outputs available in a citable, shareable and discoverable manner.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Figshare account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.figshare.com/v2" - URL of the target service
getPrivateAccount
Private Account information
listPrivateArticles
function listPrivateArticles(int? page, int pageSize, int? 'limit, int? offset) returns Article[]|error
Private Articles
Parameters
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
createPrivateArticle
function createPrivateArticle(ArticleCreate payload) returns Location|error
Create new Article
Parameters
- payload ArticleCreate - Article description
listAccountArticleReports
function listAccountArticleReports(int? groupId) returns AccountReport[]|error
Account Article Report
Parameters
- groupId int? (default ()) - A group ID to filter by
Return Type
- AccountReport[]|error - OK. An array of account report entries
generateAccountArticleReport
function generateAccountArticleReport() returns AccountReport|error
Initiate a new Report
Return Type
- AccountReport|error - OK. AccountReport created.
searchPrivateArticles
function searchPrivateArticles(PrivateArticleSearch payload) returns Article[]|error
Private Articles search
Parameters
- payload PrivateArticleSearch - Search Parameters
getPrivateArticleDetails
function getPrivateArticleDetails(int articleId) returns ArticleCompletePrivate|error
Article details
Parameters
- articleId int - Article unique identifier
Return Type
- ArticleCompletePrivate|error - OK. Article representation
updatePrivateArticle
function updatePrivateArticle(int articleId, ArticleUpdate payload) returns Response|error
Update article
deletePrivateArticle
Delete article
Parameters
- articleId int - Article unique identifier
listPrivateArticleAuthors
List article authors
Parameters
- articleId int - Article unique identifier
replacePrivateArticleAuthors
function replacePrivateArticleAuthors(int articleId, AuthorsCreator payload) returns Response|error
Replace article authors
addPrivateArticleAuthors
function addPrivateArticleAuthors(int articleId, AuthorsCreator payload) returns Response|error
Add article authors
deletePrivateArticleAuthor
Delete article author
listPrivateArticleCategories
List article categories
Parameters
- articleId int - Article unique identifier
replacePrivateArticleCategories
function replacePrivateArticleCategories(int articleId, CategoriesCreator payload) returns Response|error
Replace article categories
Parameters
- articleId int - Article unique identifier
- payload CategoriesCreator - A record of type
CategoriesCreator
which contains the necessary data to create or update article category
addPrivateArticleCategories
function addPrivateArticleCategories(int articleId, CategoriesCreator payload) returns Response|error
Add article categories
Parameters
- articleId int - Article unique identifier
- payload CategoriesCreator - A record of type
CategoriesCreator
which contains the necessary data to create or update article category
deletePrivateArticleCategory
Delete article category
getPrivateArticleConfidentialityDetails
function getPrivateArticleConfidentialityDetails(int articleId) returns ArticleConfidentiality|error
Article confidentiality details
Parameters
- articleId int - Article unique identifier
Return Type
- ArticleConfidentiality|error - OK. Article categories
updatePrivateArticleConfidentiality
function updatePrivateArticleConfidentiality(int articleId, ConfidentialityCreator payload) returns Response|error
Update article confidentiality
Parameters
- articleId int - Article unique identifier
- payload ConfidentialityCreator - A record of type
ConfidentialityCreator
which contains the necessary data to update confidentiality settings
deletePrivateArticleConfidentiality
Delete article confidentiality
Parameters
- articleId int - Article unique identifier
getPrivateArticleEmbargoDetails
function getPrivateArticleEmbargoDetails(int articleId) returns ArticleEmbargo|error
Article Embargo Details
Parameters
- articleId int - Article unique identifier
Return Type
- ArticleEmbargo|error - OK. Embargo for article
updatePrivateArticleEmbargo
function updatePrivateArticleEmbargo(int articleId, ArticleEmbargoUpdater payload) returns Response|error
Update Article Embargo
Parameters
- articleId int - Article unique identifier
- payload ArticleEmbargoUpdater - Embargo description
deletePrivateArticleEmbargo
Delete Article Embargo
Parameters
- articleId int - Article unique identifier
listPrivateArticleFiles
function listPrivateArticleFiles(int articleId) returns PrivateFile[]|error
List article files
Parameters
- articleId int - Article unique identifier
Return Type
- PrivateFile[]|error - OK. Article files list
initiatePrivateArticleUpload
function initiatePrivateArticleUpload(int articleId, FileCreator payload) returns Location|error
Initiate Upload
Parameters
- articleId int - Article unique identifier
- payload FileCreator - A record of type
FileCreator
which contains the necessary data to initiate a new file upload within the article
getPrivateArticleFile
function getPrivateArticleFile(int articleId, int fileId) returns PrivateFile|error
Single File
Return Type
- PrivateFile|error - OK. Article private file
completePrivateArticleUpload
Complete Upload
deletePrivateArticleFile
File Delete
getPrivateArticlePrivateLink
function getPrivateArticlePrivateLink(int articleId) returns PrivateLink[]|error
List private links
Parameters
- articleId int - Article unique identifier
Return Type
- PrivateLink[]|error - OK. Article private links
createPrivateArticlePrivateLink
function createPrivateArticlePrivateLink(int articleId, PrivateLinkCreator payload) returns Location|error
Create private link
Parameters
- articleId int - Article unique identifier
- payload PrivateLinkCreator - A record of type
FileCreator
which contains the necessary data to create or update private link
updatePrivateArticlePrivateLink
function updatePrivateArticlePrivateLink(int articleId, string linkId, PrivateLinkCreator payload) returns Response|error
Update private link
Parameters
- articleId int - Article unique identifier
- linkId string - Private link token
- payload PrivateLinkCreator - A record of type
FileCreator
which contains the necessary data to create or update private link
deletePrivateArticlePrivateLink
Disable private link
publishPrivateArticle
Private Article Publish
Parameters
- articleId int - Article unique identifier
reserveDoiPrivateArticle
function reserveDoiPrivateArticle(int articleId) returns ArticleDOI|error
Private Article Reserve DOI
Parameters
- articleId int - Article unique identifier
Return Type
- ArticleDOI|error - OK
reservePrivateArticleHandle
function reservePrivateArticleHandle(int articleId) returns ArticleHandle|error
Private Article Reserve Handle
Parameters
- articleId int - Article unique identifier
Return Type
- ArticleHandle|error - OK
updateArticleThumbVersion
function updateArticleThumbVersion(int articleId, int versionId, FileId payload) returns Response|error
Article Version Update Thumb
Parameters
- articleId int - Article unique identifier
- versionId int - Article version identifier
- payload FileId - File ID
searchPrivateAuthors
function searchPrivateAuthors(PrivateAuthorsSearch payload) returns Author[]|error
Search Authors
Parameters
- payload PrivateAuthorsSearch - Search Parameters
getPrivateAuthorDetails
function getPrivateAuthorDetails(int authorId) returns AuthorComplete|error
Author details
Parameters
- authorId int - Author unique identifier
Return Type
- AuthorComplete|error - OK. Article representation
listPrivateCategories
Private Account Categories
listPrivateCollections
function listPrivateCollections(int? page, int pageSize, int? 'limit, int? offset, string 'order, string orderDirection) returns Collection[]|error
Private Collections List
Parameters
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
- 'order string (default "published_date") - The field by which to order. Default varies by endpoint/resource.
- orderDirection string (default "desc") - Sorting order (desc or asc)
Return Type
- Collection[]|error - OK. An array of collections
createPrivateCollection
function createPrivateCollection(CollectionCreate payload) returns CollectionComplete|error
Create collection
Parameters
- payload CollectionCreate - Collection description
Return Type
- CollectionComplete|error - Created
searchPrivateCollections
function searchPrivateCollections(PrivateCollectionSearch payload) returns Collection[]|error
Private Collections Search
Parameters
- payload PrivateCollectionSearch - Search Parameters
Return Type
- Collection[]|error - OK. An array of collections
getPrivateCollectionDetails
function getPrivateCollectionDetails(int collectionId) returns CollectionComplete|error
Collection details
Parameters
- collectionId int - Collection Unique identifier
Return Type
- CollectionComplete|error - OK. Collection representation
updatePrivateCollection
function updatePrivateCollection(int collectionId, CollectionUpdate payload) returns Response|error
Update collection
Parameters
- collectionId int - Collection Unique identifier
- payload CollectionUpdate - Collection description
deletePrivateCollection
Delete collection
Parameters
- collectionId int - Collection Unique identifier
listPrivateCollectionArticles
List collection articles
Parameters
- collectionId int - Collection unique identifier
replacePrivateCollectionArticles
function replacePrivateCollectionArticles(int collectionId, ArticlesCreator payload) returns Response|error
Replace collection articles
addPrivateCollectionArticles
function addPrivateCollectionArticles(int collectionId, ArticlesCreator payload) returns Location|error
Add collection articles
deletePrivateCollectionArticle
Delete collection article
Parameters
- collectionId int - Collection unique identifier
- articleId int - Collection article unique identifier
listPrivateCollectionAuthors
List collection authors
Parameters
- collectionId int - Collection unique identifier
replacePrivateCollectionAuthors
function replacePrivateCollectionAuthors(int collectionId, AuthorsCreator payload) returns Response|error
Replace collection authors
addPrivateCollectionAuthors
function addPrivateCollectionAuthors(int collectionId, AuthorsCreator payload) returns Location|error
Add collection authors
deletePrivateCollectionAuthor
Delete collection author
Parameters
- collectionId int - Collection unique identifier
- authorId int - Collection Author unique identifier
listPrivateCollectionCategories
List collection categories
Parameters
- collectionId int - Collection unique identifier
replacePrivateCollectionCategories
function replacePrivateCollectionCategories(int collectionId, CategoriesCreator payload) returns Response|error
Replace collection categories
Parameters
- collectionId int - Collection unique identifier
- payload CategoriesCreator - Categories list
addPrivateCollectionCategories
function addPrivateCollectionCategories(int collectionId, CategoriesCreator payload) returns Location|error
Add collection categories
Parameters
- collectionId int - Collection unique identifier
- payload CategoriesCreator - Categories list
deletePrivateCollectionCategory
Delete collection category
Parameters
- collectionId int - Collection unique identifier
- categoryId int - Collection category unique identifier
listPrivateCollectionPrivateLinks
function listPrivateCollectionPrivateLinks(int collectionId) returns PrivateLink[]|error
List collection private links
Parameters
- collectionId int - Collection unique identifier
Return Type
- PrivateLink[]|error - OK. Collection private links
createPrivateCollectionPrivateLink
function createPrivateCollectionPrivateLink(int collectionId, CollectionPrivateLinkCreator payload) returns Location|error
Create collection private link
Parameters
- collectionId int - Collection unique identifier
- payload CollectionPrivateLinkCreator - A record of type
CollectionPrivateLinkCreator
which contains the necessary data to create or update collection private link
updatePrivateCollectionPrivateLink
function updatePrivateCollectionPrivateLink(int collectionId, string linkId, CollectionPrivateLinkCreator payload) returns Response|error
Update collection private link
Parameters
- collectionId int - Collection unique identifier
- linkId string - Private link token
- payload CollectionPrivateLinkCreator - A record of type
CollectionPrivateLinkCreator
which contains the necessary data to create or update collection private link
deletePrivateCollectionPrivateLink
Disable private link
publishPrivateCollection
Private Collection Publish
Parameters
- collectionId int - Collection Unique identifier
reserveDoiForPrivateCollection
function reserveDoiForPrivateCollection(int collectionId) returns CollectionDOI|error
Private Collection Reserve DOI
Parameters
- collectionId int - Collection Unique identifier
Return Type
- CollectionDOI|error - OK
getPrivateCollectionReserveHandle
function getPrivateCollectionReserveHandle(int collectionId) returns CollectionHandle|error
Private Collection Reserve Handle
Parameters
- collectionId int - Collection Unique identifier
Return Type
- CollectionHandle|error - OK
searchPrivateFunding
function searchPrivateFunding(FundingSearch payload) returns FundingInformation[]|error
Search Funding
Parameters
- payload FundingSearch - Search Parameters
Return Type
- FundingInformation[]|error - OK. An array of funding information
getPrivateInstitutionDetails
function getPrivateInstitutionDetails() returns Institution|error
Private Account Institutions
Return Type
- Institution|error - OK. An array of institutions
listPrivateInstitutionAccounts
function listPrivateInstitutionAccounts(int? page, int pageSize, int? 'limit, int? offset, int? isActive, string? institutionUserId, string? email) returns ShortAccount[]|error
Private Account Institution Accounts
Parameters
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
- isActive int? (default ()) - Filter by active status
- institutionUserId string? (default ()) - Filter by institution_user_id
- email string? (default ()) - Filter by email
Return Type
- ShortAccount[]|error - OK. An array of Accounts
createPrivateInstitutionAccounts
function createPrivateInstitutionAccounts(AccountCreate payload) returns Response|error
Create new Institution Account
Parameters
- payload AccountCreate - Account description
searchPrivateInstitutionAccounts
function searchPrivateInstitutionAccounts(InstitutionAccountsSearch payload) returns ShortAccount[]|error
Private Account Institution Accounts Search
Parameters
- payload InstitutionAccountsSearch - Search Parameters
Return Type
- ShortAccount[]|error - OK. An array of Accounts
updatePrivateInstitutionAccounts
function updatePrivateInstitutionAccounts(int accountId, AccountUpdate payload) returns Response|error
Update Institution Account
Parameters
- accountId int - Account identifier the user is associated to
- payload AccountUpdate - Account description
getPrivateInstitutionArticles
function getPrivateInstitutionArticles(int? page, int pageSize, int? 'limit, int? offset, string 'order, string orderDirection, string? publishedSince, string? modifiedSince, int? status, string? resourceDoi, int? itemType) returns Article[]|error
Private Institution Articles
Parameters
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
- 'order string (default "published_date") - The field by which to order. Default varies by endpoint/resource.
- orderDirection string (default "desc") - Sorting order (desc or asc)
- publishedSince string? (default ()) - Filter by article publishing date. Will only return articles published after the date. date(ISO 8601) YYYY-MM-DD
- modifiedSince string? (default ()) - Filter by article modified date. Will only return articles published after the date. date(ISO 8601) YYYY-MM-DD
- status int? (default ()) - only return collections with this status
- resourceDoi string? (default ()) - only return collections with this resource_doi
- itemType int? (default ()) - Only return articles with the respective type. Mapping for item_type is: 1 - Figure, 2 - Media, 3 - Dataset, 5 - Poster, 6 - Journal contribution, 7 - Presentation, 8 - Thesis, 9 - Software, 11 - Online resource, 12 - Preprint, 13 - Book, 14 - Conference contribution, 15 - Chapter, 16 - Peer review, 17 - Educational resource, 18 - Report, 19 - Standard, 20 - Composition, 21 - Funding, 22 - Physical object, 23 - Data management plan, 24 - Workflow, 25 - Monograph, 26 - Performance, 27 - Event, 28 - Service, 29 - Model
getPrivateInstitutionEmbargoOptionsDetails
function getPrivateInstitutionEmbargoOptionsDetails() returns GroupEmbargoOptions[]|error
Private Account Institution embargo options
Return Type
- GroupEmbargoOptions[]|error - OK. An array of embargo options
listPrivateInstitutionGroups
Private Account Institution Groups
getPrivateGroupEmbargoOptionsDetails
function getPrivateGroupEmbargoOptionsDetails(int groupId) returns GroupEmbargoOptions[]|error
Private Account Institution Group Embargo Options
Parameters
- groupId int - Group identifier
Return Type
- GroupEmbargoOptions[]|error - OK. An array of embargo options
getAccountInstitutionCuration
function getAccountInstitutionCuration(int curationId) returns CurationDetail|error
Institution Curation Review
Parameters
- curationId int - ID of the curation
Return Type
- CurationDetail|error - OK. A curation review.
getAccountInstitutionCurationComments
function getAccountInstitutionCurationComments(int curationId, int? 'limit, int? offset) returns CurationComment|error
Institution Curation Review Comments
Parameters
- curationId int - ID of the curation
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
Return Type
- CurationComment|error - OK. A curation review's comments.
addAccountInstitutionCurationComments
function addAccountInstitutionCurationComments(int curationId, CurationCommentCreate payload) returns Response|error
POST Institution Curation Review Comment
Parameters
- curationId int - ID of the curation
- payload CurationCommentCreate - The content/value of the comment.
listAccountInstitutionCurations
function listAccountInstitutionCurations(int? groupId, int? articleId, string? status, int? 'limit, int? offset) returns Curation|error
Institution Curation Reviews
Parameters
- groupId int? (default ()) - Filter by the group ID
- articleId int? (default ()) - Retrieve the reviews for this article
- status string? (default ()) - Filter by the status of the review
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
listPrivateInstitutionRoles
Private Account Institution Roles
listPrivateInstitutionAccountGroupRoles
function listPrivateInstitutionAccountGroupRoles(int accountId) returns AccountGroupRoles|error
List Institution Account Group Roles
Parameters
- accountId int - Account identifier the user is associated to
Return Type
- AccountGroupRoles|error - OK. Account Group Roles
createPrivateInstitutionAccountGroupRoles
function createPrivateInstitutionAccountGroupRoles(int accountId, AccountGroupRolesCreate payload) returns Response|error
Add Institution Account Group Roles
Parameters
- accountId int - Account identifier the user is associated to
- payload AccountGroupRolesCreate - Account description
deletePrivateInstitutionAccountGroupRole
function deletePrivateInstitutionAccountGroupRole(int accountId, int groupId, int roleId) returns Response|error
Delete Institution Account Group Role
Parameters
- accountId int - Account identifier for which to remove the role
- groupId int - Group identifier for which to remove the role
- roleId int - Role identifier
getPrivateInstitutionUserAccount
Private Account Institution User
Parameters
- accountId int - Account identifier the user is associated to
listPrivateLicenses
Private Account Licenses
listPrivateProjects
function listPrivateProjects(int? page, int pageSize, int? 'limit, int? offset, string 'order, string orderDirection, string? storage, string? roles) returns ProjectPrivate[]|error
Private Projects
Parameters
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
- 'order string (default "published_date") - The field by which to order.
- orderDirection string (default "desc") - Sorting order (desc or asc)
- storage string? (default ()) - only return collections from this institution
- roles string? (default ()) - Any combination of owner, collaborator, viewer separated by comma. Examples: "owner" or "owner,collaborator".
Return Type
- ProjectPrivate[]|error - OK. An array of projects
createPrivateProject
function createPrivateProject(ProjectCreate payload) returns Location|error
Create project
Parameters
- payload ProjectCreate - Project description
searchPrivateProjects
function searchPrivateProjects(ProjectsSearch payload) returns ProjectPrivate[]|error
Private Projects search
Parameters
- payload ProjectsSearch - Search Parameters
Return Type
- ProjectPrivate[]|error - OK. An array of projects
getPrivateProjectDetails
function getPrivateProjectDetails(int projectId) returns ProjectCompletePrivate|error
View project details
Parameters
- projectId int - Project unique identifier
Return Type
- ProjectCompletePrivate|error - OK. Project representation
updatePrivateProject
function updatePrivateProject(int projectId, ProjectUpdate payload) returns Response|error
Update project
deletePrivateProject
Delete project
Parameters
- projectId int - Project unique identifier
listPrivateProjectArticles
function listPrivateProjectArticles(int projectId, int? page, int pageSize, int? 'limit, int? offset) returns Article[]|error
List project articles
Parameters
- projectId int - Project unique identifier
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
createPrivateProjectArticles
function createPrivateProjectArticles(int projectId, ArticleProjectCreate payload, int? page, int pageSize, int? 'limit, int? offset) returns Location|error
Create project article
Parameters
- projectId int - Project unique identifier
- payload ArticleProjectCreate - Article description
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
getPrivateProjectArticleDetails
function getPrivateProjectArticleDetails(int projectId, int articleId) returns ProjectArticle|error
Project article details
Parameters
- projectId int - Project unique identifier
- articleId int - Project Article unique identifier
Return Type
- ProjectArticle|error - OK. Article representation
deletePrivateProjectArticle
Delete project article
Parameters
- projectId int - Project unique identifier
- articleId int - Project Article unique identifier
listPrivateProjectArticleFiles
function listPrivateProjectArticleFiles(int projectId, int articleId) returns PrivateFile[]|error
Project article list files
Parameters
- projectId int - Project unique identifier
- articleId int - Project Article unique identifier
Return Type
- PrivateFile[]|error - OK. List of files
getPrivateProjectArticleFile
function getPrivateProjectArticleFile(int projectId, int articleId, int fileId) returns PrivateFile|error
Project article file details
Parameters
- projectId int - Project unique identifier
- articleId int - Project Article unique identifier
- fileId int - File unique identifier
Return Type
- PrivateFile|error - OK. File representation
listPrivateProjectCollaborators
function listPrivateProjectCollaborators(int projectId) returns ProjectCollaborator[]|error
List project collaborators
Parameters
- projectId int - Project unique identifier
Return Type
- ProjectCollaborator[]|error - OK. List of Collaborators
invitePrivateProjectCollaborators
function invitePrivateProjectCollaborators(int projectId, ProjectCollaboratorInvite payload) returns ResponseMessage|error
Invite project collaborators
Parameters
- projectId int - Project unique identifier
- payload ProjectCollaboratorInvite - viewer or collaborator role. User user_id or email of user
Return Type
- ResponseMessage|error - Created
removePrivateProjectCollaborator
Remove project collaborator
leavePrivateProject
Private Project Leave
Parameters
- projectId int - Project unique identifier
listPrivateProjectNotes
function listPrivateProjectNotes(int projectId, int? page, int pageSize, int? 'limit, int? offset) returns ProjectNote[]|error
List project notes
Parameters
- projectId int - Project unique identifier
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
Return Type
- ProjectNote[]|error - OK. List of project notes
createProjectNote
function createProjectNote(int projectId, ProjectNoteCreate payload) returns Location|error
Create project note
getPrivateProjectNote
function getPrivateProjectNote(int projectId, int noteId) returns ProjectNotePrivate|error
Project note details
Return Type
- ProjectNotePrivate|error - OK. Note representation
updatePrivateProjectNote
function updatePrivateProjectNote(int projectId, int noteId, ProjectNoteCreate payload) returns Response|error
Update project note
Parameters
- projectId int - Project unique identifier
- noteId int - Note unique identifier
- payload ProjectNoteCreate - Note message
deletePrivateProjectNote
Delete project note
publishPrivateProject
function publishPrivateProject(int projectId) returns ResponseMessage|error
Private Project Publish
Parameters
- projectId int - Project unique identifier
Return Type
- ResponseMessage|error - OK
listPublicArticles
function listPublicArticles(int? page, int pageSize, int? 'limit, int? offset, string 'order, string orderDirection, int? institution, string? publishedSince, string? modifiedSince, int? group, string? resourceDoi, int? itemType, string? doi, string? 'handle) returns Article[]|error
Public Articles
Parameters
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
- 'order string (default "published_date") - The field by which to order. Default varies by endpoint/resource.
- orderDirection string (default "desc") - Sorting order (desc or asc)
- institution int? (default ()) - only return articles from this institution
- publishedSince string? (default ()) - Filter by article publishing date. Will only return articles published after the date. date(ISO 8601) YYYY-MM-DD
- modifiedSince string? (default ()) - Filter by article modified date. Will only return articles published after the date. date(ISO 8601) YYYY-MM-DD
- group int? (default ()) - only return articles from this group
- resourceDoi string? (default ()) - only return articles with this resource_doi
- itemType int? (default ()) - Only return articles with the respective type. Mapping for item_type is: 1 - Figure, 2 - Media, 3 - Dataset, 5 - Poster, 6 - Journal contribution, 7 - Presentation, 8 - Thesis, 9 - Software, 11 - Online resource, 12 - Preprint, 13 - Book, 14 - Conference contribution, 15 - Chapter, 16 - Peer review, 17 - Educational resource, 18 - Report, 19 - Standard, 20 - Composition, 21 - Funding, 22 - Physical object, 23 - Data management plan, 24 - Workflow, 25 - Monograph, 26 - Performance, 27 - Event, 28 - Service, 29 - Model
- doi string? (default ()) - only return articles with this doi
- 'handle string? (default ()) - only return articles with this handle
searchPublicArticles
function searchPublicArticles(ArticleSearch payload) returns Article[]|error
Public Articles Search
Parameters
- payload ArticleSearch - Search Parameters
getArticleById
function getArticleById(int articleId) returns ArticleComplete|error
View article details
Parameters
- articleId int - Article Unique identifier
Return Type
- ArticleComplete|error - OK. Article representation
listArticleFiles
function listArticleFiles(int articleId) returns PublicFile[]|error
List article files
Parameters
- articleId int - Article Unique identifier
Return Type
- PublicFile[]|error - OK. List of article files
getPublicArticleFileById
function getPublicArticleFileById(int articleId, int fileId) returns PublicFile|error
Article file details
Return Type
- PublicFile|error - OK. File representation
listPublicArticleVersions
function listPublicArticleVersions(int articleId) returns ArticleVersions[]|error
List article versions
Parameters
- articleId int - Article Unique identifier
Return Type
- ArticleVersions[]|error - OK. Article version representations
getArticleVersionDetails
function getArticleVersionDetails(int articleId, int vNumber) returns ArticleComplete|error
Article details for version
Return Type
- ArticleComplete|error - OK. Article representation
getArticleVersionConfidentiality
function getArticleVersionConfidentiality(int articleId, int vNumber) returns ArticleConfidentiality|error
Public Article Confidentiality for article version
Return Type
- ArticleConfidentiality|error - OK. Confidentiality representation
embargoArticleVersion
function embargoArticleVersion(int articleId, int vNumber) returns ArticleEmbargo|error
Public Article Embargo for article version
Return Type
- ArticleEmbargo|error - OK. Embargo representation
listPublicCategories
Public Categories
listPublicCollections
function listPublicCollections(int? page, int pageSize, int? 'limit, int? offset, string 'order, string orderDirection, int? institution, string? publishedSince, string? modifiedSince, int? group, string? resourceDoi, string? doi, string? 'handle) returns Collection[]|error
Public Collections
Parameters
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
- 'order string (default "published_date") - The field by which to order. Default varies by endpoint/resource.
- orderDirection string (default "desc") - Sorting order (desc or asc)
- institution int? (default ()) - only return collections from this institution
- publishedSince string? (default ()) - Filter by collection publishing date. Will only return collections published after the date. date(ISO 8601) YYYY-MM-DD
- modifiedSince string? (default ()) - Filter by collection modified date. Will only return collections published after the date. date(ISO 8601) YYYY-MM-DD
- group int? (default ()) - only return collections from this group
- resourceDoi string? (default ()) - only return collections with this resource_doi
- doi string? (default ()) - only return collections with this doi
- 'handle string? (default ()) - only return collections with this handle
Return Type
- Collection[]|error - OK. An array of collections
searchCollections
function searchCollections(CollectionSearch payload) returns Collection[]|error
Public Collections Search
Parameters
- payload CollectionSearch - Search Parameters
Return Type
- Collection[]|error - OK. An array of collections
getCollectionDetailsById
function getCollectionDetailsById(int collectionId) returns CollectionComplete|error
Collection details
Parameters
- collectionId int - Collection Unique identifier
Return Type
- CollectionComplete|error - OK. Collection representation
listCollectionArticles
function listCollectionArticles(int collectionId, int? page, int pageSize, int? 'limit, int? offset) returns Article[]|error
Public Collection Articles
Parameters
- collectionId int - Collection Unique identifier
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
listCollectionVersions
function listCollectionVersions(int collectionId) returns CollectionVersions[]|error
Collection Versions list
Parameters
- collectionId int - Collection Unique identifier
Return Type
- CollectionVersions[]|error - OK. An array of versions
getCollectionVersionDetails
function getCollectionVersionDetails(int collectionId, int versionId) returns CollectionComplete|error
Collection Version details
Return Type
- CollectionComplete|error - OK. Collection for that version
downloadFile
Public File Download
Parameters
- fileId int - File Id
uploadHRFeedFile
function uploadHRFeedFile(HrfeedUploadBody payload) returns ResponseMessage|error
Private Institution HRfeed Upload
Parameters
- payload HrfeedUploadBody - Request payload to upload HRFeed File
Return Type
- ResponseMessage|error - OK
listArticlesByInstitution
function listArticlesByInstitution(string institutionStringId, string resourceId, string filename) returns Article[]|error
Public Licenses
Parameters
- institutionStringId string - Institution Id
- resourceId string - Resource Id
- filename string - File name
listPublicLicenses
Public Licenses
listPublicProjects
function listPublicProjects(int? page, int pageSize, int? 'limit, int? offset, string 'order, string orderDirection, int? institution, string? publishedSince, int? group) returns Project[]|error
Public Projects
Parameters
- page int? (default ()) - Page number. Used for pagination with page_size
- pageSize int (default 10) - The number of results included on a page. Used for pagination with page
- 'limit int? (default ()) - Number of results included on a page. Used for pagination with query
- offset int? (default ()) - Where to start the listing(the offset of the first result). Used for pagination with limit
- 'order string (default "published_date") - The field by which to order. Default varies by endpoint/resource.
- orderDirection string (default "desc") - Sorting order (desc or asc)
- institution int? (default ()) - only return collections from this institution
- publishedSince string? (default ()) - Filter by article publishing date. Will only return articles published after the date. date(ISO 8601) YYYY-MM-DD
- group int? (default ()) - only return collections from this group
searchPublicProjects
function searchPublicProjects(ProjectsSearch payload) returns Project[]|error
Public Projects Search
Parameters
- payload ProjectsSearch - Search Parameters
getProjectById
function getProjectById(int projectId) returns ProjectComplete|error
Public Project
Parameters
- projectId int - Project Unique identifier
Return Type
- ProjectComplete|error - OK. Project representation
listArticlesByProject
Public Project Articles
Parameters
- projectId int - Project Unique identifier
Records
figshare: Account
Fields
- active int? - Account activity status
- created_date string? - Date when account was created
- email string? - User email
- first_name string? - First Name
- group_id int? - Account group id
- id int? - Account id
- institution_id int? - Account institution
- institution_user_id string? - Account institution user id
- last_name string? - Last Name
- maximum_file_size int? - Maximum upload size for account
- modified_date string? - Date of last account modification
- pending_quota_request boolean? - True if a quota request is pending
- quota int? - Account quota
- used_quota int? - Account total used quota
- used_quota_private int? - Account used private quota
- used_quota_public int? - Account public used quota
figshare: AccountCreate
Fields
- email string - Email of account
- first_name string(default "") - First Name
- group_id int? - Not applicable to regular users. This field is reserved to institutions/publishers with access to assign to specific groups
- institution_user_id string(default "") - Institution user id
- is_active boolean? - Is account active
- last_name string - Last Name
- quota int? - Account quota
- symplectic_user_id string(default "") - Symplectic user id
figshare: AccountGroupRoles
figshare: AccountGroupRolesCreate
figshare: AccountReport
Fields
- account_id int? - The ID of the account which generated this report.
- created_date string? - Date when the AccountReport was requested
- download_url string? - The download link for the generated XLSX
- group_id int? - The group ID that was used to filter the report, if any.
- id int? - A unique ID for the AccountRecord
- status string? - Status of the report
figshare: AccountUpdate
Fields
- group_id int? - Not applicable to regular users. This field is reserved to institutions/publishers with access to assign to specific groups
- is_active boolean? - Is account active
figshare: Article
Fields
- defined_type int? - Type of article identificator
- defined_type_name string? - Name of the article type identificator
- doi string? - DOI
- group_id decimal? - Group ID
- 'handle string? - Handle
- id int? - Unique identifier for article
- published_date string? - Posted date
- thumb string? - Thumbnail image
- timeline Timeline? -
- title string? - Title of article
- url string? - Api endpoint for article
- url_private_api string? - Private Api endpoint for article
- url_private_html string? - Private site endpoint for article
- url_public_api string? - Public Api endpoint for article
- url_public_html string? - Public site endpoint for article
figshare: ArticleConfidentiality
Fields
- is_confidential boolean? - True if article is confidential
- reason string? - Reason for confidentiality
figshare: ArticleCreate
Fields
- authors record {}[](default []) - List of authors to be associated with the article. The list can contain the following fields: id, name, first_name, last_name, email, orcid_id. If an id is supplied, it will take priority and everything else will be ignored. No more than 10 authors. For adding more authors use the specific authors endpoint.
- categories int[](default []) - List of category ids to be associated with the article(e.g [1, 23, 33, 66])
- custom_fields record {}? - List of key, values pairs to be associated with the article
- defined_type string? - <b>One of:</b> <code>figure</code> <code>online resource</code> <code>preprint</code> <code>book</code> <code>conference contribution</code> <code>media</code> <code>dataset</code> <code>poster</code> <code>journal contribution</code> <code>presentation</code> <code>thesis</code> <code>software</code>
- description string(default "") - The article description. In a publisher case, usually this is the remote article description
- doi string(default "") - Not applicable for regular users. In an institutional case, make sure your group supports setting DOIs. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- funding string(default "") - Grant number or funding authority
- funding_list FundingCreate[]? - Funding creation / update items
- group_id int? - Not applicable to regular users. This field is reserved to institutions/publishers with access to assign to specific groups
- 'handle string(default "") - Not applicable for regular users. In an institutional case, make sure your group supports setting Handles. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- keywords string[](default []) - List of tags to be associated with the article. Tags can be used instead
- license int(default 0) - License id for this article.
- references string[](default []) - List of links to be associated with the article (e.g ["http://link1", "http://link2", "http://link3"])
- resource_doi string(default "") - Not applicable to regular users. In a publisher case, this is the publisher article DOI.
- resource_title string(default "") - Not applicable to regular users. In a publisher case, this is the publisher article title.
- tags string[](default []) - List of tags to be associated with the article. Keywords can be used instead
- timeline TimelineUpdate? -
- title string - Title of article
figshare: ArticleDOI
Fields
- doi string? - Reserved DOI
figshare: ArticleEmbargo
Fields
- embargo_date string? - Date when embargo lifts
- embargo_options record {}[]? - List of embargo permissions that are associated with the article. If the type is logged_in and the group_ids list is empty, then the whole institution can see the article; if there are multiple group_ids, then only users that are under those groups can see the article.
- embargo_reason string? - Reason for embargo
- embargo_title string? - Title for embargo
- embargo_type string? - Embargo type
- is_embargoed boolean? - True if embargoed
figshare: ArticleEmbargoUpdater
Fields
- embargo_date string - Date when the embargo expires and the article gets published, '0' value will set up permanent embargo
- embargo_options record {}[]? - List of embargo permissions to be associated with the article. The list must contain
id
and can also containgroup_ids
(a field that only applies to 'logged_in' permissions). The new list replaces old options in the database, and an empty list removes all permissions for this article. Administration permission has to be set up alone but logged in and IP range permissions can be set up together.
- embargo_reason string? - Reason for setting embargo
- embargo_title string? - Title for embargo
- embargo_type string - Embargo can be enabled at the article or the file level. Possible values: article, file
- is_embargoed boolean - Embargo status
figshare: ArticleHandle
Fields
- 'handle string? - Reserved Handle
figshare: ArticleProjectCreate
Fields
- authors record {}[](default []) - List of authors to be associated with the article. The list can contain the following fields: id, name, first_name, last_name, email, orcid_id. If an id is supplied, it will take priority and everything else will be ignored. No more than 10 authors. For adding more authors use the specific authors endpoint.
- categories int[](default []) - List of category ids to be associated with the article(e.g [1, 23, 33, 66])
- custom_fields record {}? - List of key, values pairs to be associated with the article
- defined_type string? - <b>One of:</b> <code>figure</code> <code>online resource</code> <code>preprint</code> <code>book</code> <code>conference contribution</code> <code>media</code> <code>dataset</code> <code>poster</code> <code>journal contribution</code> <code>presentation</code> <code>thesis</code> <code>software</code>
- description string(default "") - The article description. In a publisher case, usually this is the remote article description
- doi string(default "") - Not applicable for regular users. In an institutional case, make sure your group supports setting DOIs. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- funding string(default "") - Grant number or funding authority
- funding_list FundingCreate[]? - Funding creation / update items
- 'handle string(default "") - Not applicable for regular users. In an institutional case, make sure your group supports setting Handles. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- keywords string[](default []) - List of tags to be associated with the article. Tags can be used instead
- license int(default 0) - License id for this article.
- references string[](default []) - List of links to be associated with the article (e.g ["http://link1", "http://link2", "http://link3"])
- resource_doi string(default "") - Not applicable to regular users. In a publisher case, this is the publisher article DOI.
- resource_title string(default "") - Not applicable to regular users. In a publisher case, this is the publisher article title.
- tags string[](default []) - List of tags to be associated with the article. Keywords can be used instead
- timeline TimelineUpdate? -
- title string - Title of article
figshare: ArticlesCreator
Fields
- articles int[] - List of article ids
figshare: ArticleUpdate
Fields
- authors record {}[]? - List of authors to be associated with the article. The list can contain the following fields: id, name, first_name, last_name, email, orcid_id. If an id is supplied, it will take priority and everything else will be ignored. No more than 10 authors. For adding more authors use the specific authors endpoint.
- categories int[]? - List of category ids to be associated with the article(e.g [1, 23, 33, 66])
- custom_fields record {}? - List of key, values pairs to be associated with the article
- defined_type string? - <b>One of:</b> <code>figure</code> <code>online resource</code> <code>preprint</code> <code>book</code> <code>conference contribution</code> <code>media</code> <code>dataset</code> <code>poster</code> <code>journal contribution</code> <code>presentation</code> <code>thesis</code> <code>software</code>
- description string? - The article description. In a publisher case, usually this is the remote article description
- doi string? - Not appliable for regular users. In an institutional case, make sure your group supports setting DOIs. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- funding string? - Grant number or funding authority
- funding_list FundingCreate[]? - Funding creation / update items
- group_id int? - Not applicable to regular users. This field is reserved to institutions/publishers with access to assign to specific groups
- 'handle string? - Not appliable for regular users. In an institutional case, make sure your group supports setting Handles. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- keywords string[]? - List of tags to be associated with the article. Tags can be used instead
- license int? - License id for this article.
- references string[]? - List of links to be associated with the article (e.g ["http://link1", "http://link2", "http://link3"])
- resource_doi string? - Not applicable to regular users. In a publisher case, this is the publisher article DOI.
- resource_title string? - Not applicable to regular users. In a publisher case, this is the publisher article title.
- tags string[]? - List of tags to be associated with the article. Keywords can be used instead
- timeline TimelineUpdate? -
- title string? - Title of article
figshare: ArticleVersions
Fields
- url string? - Api endpoint for the item version
- 'version int? - Version number
figshare: Author
Fields
- full_name string? - Author full name
- id int? - Author id
- is_active boolean? - True if author has published items
- orcid_id string? - Author Orcid
- url_name string? - Author url name
figshare: AuthorsCreator
Fields
- authors record {}[] - List of authors to be associated with the article. The list can contain the following fields: id, name, first_name, last_name, email, orcid_id. If an id is supplied, it will take priority and everything else will be ignored. No more than 10 authors. For adding more authors use the specific authors endpoint.
figshare: CategoriesCreator
Fields
- categories int[] - List of category ids
figshare: Category
Fields
- id int? - Category id
- parent_id int? - Parent category
- title string? - Category title
figshare: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
figshare: Collaborator
Fields
- name string? - Collaborator name
- role_name string? - Collaborator role
- user_id int? - Collaborator id
figshare: Collection
Fields
- doi string? - Collection DOI
- 'handle string? - Collection Handle
- id int? - Collection id
- published_date string? - Date when collection was published
- timeline Timeline? -
- title string? - Collection title
- url string? - Api endpoint
figshare: CollectionCreate
Fields
- articles int[]? - List of articles to be associated with the collection
- authors record {}[](default []) - List of authors to be associated with the article. The list can contain the following fields: id, name, first_name, last_name, email, orcid_id. If an id is supplied, it will take priority and everything else will be ignored. No more than 10 authors. For adding more authors use the specific authors endpoint.
- categories int[](default []) - List of category ids to be associated with the article(e.g [1, 23, 33, 66])
- custom_fields record {}? - List of key, values pairs to be associated with the article
- description string(default "") - The article description. In a publisher case, usually this is the remote article description
- doi string(default "") - Not applicable for regular users. In an institutional case, make sure your group supports setting DOIs. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- funding string(default "") - Grant number or funding authority
- funding_list FundingCreate[]? - Funding creation / update items
- group_id int? - Not applicable to regular users. This field is reserved to institutions/publishers with access to assign to specific groups
- 'handle string(default "") - Not applicable for regular users. In an institutional case, make sure your group supports setting Handles. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- keywords string[](default []) - List of tags to be associated with the article. Tags can be used instead
- references string[](default []) - List of links to be associated with the article (e.g ["http://link1", "http://link2", "http://link3"])
- resource_doi string(default "") - Not applicable to regular users. In a publisher case, this is the publisher article DOI.
- resource_id string? - Not applicable to regular users. In a publisher case, this is the publisher article id
- resource_link string? - Not applicable to regular users. In a publisher case, this is the publisher article link
- resource_title string(default "") - Not applicable to regular users. In a publisher case, this is the publisher article title.
- resource_version int? - Not applicable to regular users. In a publisher case, this is the publisher article version
- tags string[](default []) - List of tags to be associated with the article. Keywords can be used instead
- timeline TimelineUpdate? -
- title string - Title of article
figshare: CollectionDOI
Fields
- doi string? - Reserved DOI
figshare: CollectionHandle
Fields
- 'handle string? - Reserved Handle
figshare: CollectionPrivateLinkCreator
Fields
- expires_date string? - Date when this private link should expire - optional. By default private links expire in 365 days.
- read_only boolean? - Optional, default true. Set to false to give private link users editing rights for this collection.
figshare: CollectionUpdate
Fields
- articles int[]? - List of articles to be associated with the collection
- authors record {}[]? - List of authors to be associated with the article. The list can contain the following fields: id, name, first_name, last_name, email, orcid_id. If an id is supplied, it will take priority and everything else will be ignored. No more than 10 authors. For adding more authors use the specific authors endpoint.
- categories int[]? - List of category ids to be associated with the article(e.g [1, 23, 33, 66])
- custom_fields record {}? - List of key, values pairs to be associated with the article
- description string? - The article description. In a publisher case, usually this is the remote article description
- doi string? - Not applicable for regular users. In an institutional case, make sure your group supports setting DOIs. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- funding string? - Grant number or funding authority
- funding_list FundingCreate[]? - Funding creation / update items
- group_id int? - Not applicable to regular users. This field is reserved to institutions/publishers with access to assign to specific groups
- 'handle string? - Not applicable for regular users. In an institutional case, make sure your group supports setting Handles. This setting is applied by figshare via opening a ticket through our support/helpdesk system.
- keywords string[]? - List of tags to be associated with the article. Tags can be used instead
- references string[]? - List of links to be associated with the article (e.g ["http://link1", "http://link2", "http://link3"])
- resource_doi string? - Not applicable to regular users. In a publisher case, this is the publisher article DOI.
- resource_id string? - Not applicable to regular users. In a publisher case, this is the publisher article id
- resource_link string? - Not applicable to regular users. In a publisher case, this is the publisher article link
- resource_title string? - Not applicable to regular users. In a publisher case, this is the publisher article title.
- resource_version int? - Not applicable to regular users. In a publisher case, this is the publisher article version
- tags string[]? - List of tags to be associated with the article. Keywords can be used instead
- timeline TimelineUpdate? -
- title string? - Title of article
figshare: CollectionVersions
Fields
- id int? - Version number
- url string? - Api endpoint for the collection version
figshare: CommonSearch
Fields
- group int? - only return collections from this group
- institution int? - only return collections from this institution
- 'limit int? - Number of results included on a page. Used for pagination with query
- modified_since string? - Filter by article modified date. Will only return articles published after the date. date(ISO 8601) YYYY-MM-DD
- offset int? - Where to start the listing(the offset of the first result). Used for pagination with limit
- order_direction string? - Sorting order (desc or asc)
- page int? - Page number. Used for pagination with page_size
- page_size int? - The number of results included on a page. Used for pagination with page
- published_since string? - Filter by article publishing date. Will only return articles published after the date. date(ISO 8601) YYYY-MM-DD
- search_for string? - Search term
figshare: ConfidentialityCreator
Fields
- reason string? - Reason for confidentiality
figshare: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
figshare: Curation
Fields
- account_id int? - The ID of the account of the owner of the article of this review.
- article_id int? - The ID of the article of this review.
- assigned_to int? - The ID of the account to which this review is assigned.
- comments_count int? - The number of comments in the review.
- created_date string? - The creation date of the review.
- group_id int? - The group in which the article is present.
- id int? - The review id
- modified_date string? - The date the review has been modified.
- review_date string? - The last time a comment has been added to the review.
- status string? - The status of the review.
- 'version int? - The Version number of the article in review.
figshare: CurationComment
Fields
- account_id int? - The ID of the account which generated this comment.
- id int? - The ID of the comment.
- text string? - The value/content of the comment.
- 'type string? - The ID of the account which generated this comment.
figshare: CurationCommentCreate
Fields
- text string - The contents/value of the comment
figshare: CustomArticleField
Fields
- is_mandatory boolean? - True if field completion is mandatory
- name string? - Custom metadata name
- value string? - Custom metadata value
figshare: ErrorMessage
Fields
- code int? - A machine friendly error code, used by the dev team to identify the error.
- message string? - A human friendly message explaining the error.
figshare: FileCreator
Fields
- link string? - Url for an existing file that will not be uploaded to Figshare
- md5 string? - MD5 sum pre-computed on client side.
- name string? - File name including the extension; can be omitted only for linked files.
- size int? - File size in bytes; can be omitted only for linked files.
figshare: FileId
Fields
- file_id int? - File ID
figshare: FundingCreate
Fields
- id int? - A funding ID as returned by the Funding Search endpoint
- title string? - The title of the new user created funding
figshare: FundingInformation
Fields
- funder_name string? - Funder's name
- grant_code string? - The grant code
- id int? - Funding id
- is_user_defined boolean? - Return whether the grant has been introduced manually
- title string? - The funding name
- url string? - The grant url
figshare: FundingSearch
Fields
- search_for string? - Search term
figshare: Group
Fields
- association_criteria string? - HR code associated with group, if code exists
- id int? - Group id
- name string? - Group name
- parent_id int? - Parent group if any
- resource_id string? - Group resource id
figshare: GroupEmbargoOptions
Fields
- id int? - Embargo option id
- ip_name string? - IP range name; value appears if type is ip_range
- 'type string? - Embargo permission type
figshare: HrfeedUploadBody
Fields
- hrfeed string? - You can find an example in the Hr Feed section
figshare: Institution
Fields
- domain string? - Institution domain
- id int? - Institution id
- name string? - Institution name
figshare: InstitutionAccountsSearch
Fields
- email string? - filter by email
- institution_user_id string? - filter by institution_user_id
- is_active int? - Filter by active status
- 'limit int? - Number of results included on a page. Used for pagination with query
- offset int? - Where to start the listing(the offset of the first result). Used for pagination with limit
- page int? - Page number. Used for pagination with page_size
- page_size int? - The number of results included on a page. Used for pagination with page
- search_for string? - Search term
figshare: License
Fields
- name string? - License name
- url string? - License url
- value int? - License value
figshare: Location
Fields
- location string? - Url for item
figshare: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://api.figshare.com/v2/token") - Refresh URL
figshare: PrivateAuthorsSearch
Fields
- group_id int? - Return only authors in this group or subgroups of the group
- institution_id int? - Return only authors associated to this institution
- is_active boolean? - Return only active authors if True
- is_public boolean? - Return only authors that have published items if True
- 'limit int? - Number of results included on a page. Used for pagination with query
- offset int? - Where to start the listing(the offset of the first result). Used for pagination with limit
- orcid string? - Orcid of author
- 'order string? - The field by which to order. Default varies by endpoint/resource.
- order_direction string? - Sorting order (desc or asc)
- page int? - Page number. Used for pagination with page_size
- page_size int? - The number of results included on a page. Used for pagination with page
- search_for string? - Search term
figshare: PrivateLink
Fields
- expires_date string? - Date when link will expire
- id string? - Private link id
- is_active boolean? - True if private link is active
figshare: PrivateLinkCreator
Fields
- expires_date string? - Date when this private link should expire - optional. By default private links expire in 365 days.
figshare: Project
Fields
- id int? - Project id
- published_date string? - Date when project was published
- title string? - Project title
- url string? - Api endpoint
figshare: ProjectCollaborator
Fields
- name string? - Collaborator name
- role_name string? - Collaborator role
- status string? - Status of collaborator invitation
- user_id int? - Collaborator id
figshare: ProjectCollaboratorInvite
Fields
- comment string? - Text sent when inviting the user to the project
- email string? - Collaborator email
- role_name string - Role of the the collaborator inside the project
- user_id int? - User id of the collaborator
figshare: ProjectCreate
Fields
- description string? - Project description
- funding string? - Grant number or organization(s) that funded this project. Up to 2000 characters permitted.
- funding_list FundingCreate[]? - Funding creation / update items
- group_id int? - Only if project type is group.
- title string - The title for this project - mandatory. 3 - 1000 characters.
figshare: ProjectNote
Fields
- 'abstract string? - Note Abstract - short/truncated content
- created_date string? - Date when note was created
- id int? - Project note id
- modified_date string? - Date when note was last modified
- user_id int? - User who wrote the note
- user_name string? - Username of the one who wrote the note
figshare: ProjectNoteCreate
Fields
- text string - Text of the note
figshare: ProjectUpdate
Fields
- description string? - Project description
- funding string? - Grant number or organization(s) that funded this project. Up to 2000 characters permitted.
- funding_list FundingCreate[]? - Funding creation / update items
- title string? - The title for this project - mandatory. 3 - 1000 characters.
figshare: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
figshare: PublicFile
Fields
- computed_md5 string? - File computed md5
- download_url string? - Url for file download
- id int? - File id
- is_link_only boolean? - True if file is hosted somewhere else
- name string? - File name
- size int? - File size
- supplied_md5 string? - File supplied md5
figshare: ResponseMessage
Fields
- message string? - Response message text
figshare: Role
Fields
- category string? - Role category
- description string? - Role description
- id int? - Role id
- name string? - Role name
figshare: ShortAccount
Fields
- active int? - Account activity status
- email string? - User email
- first_name string? - First Name
- id int? - Account id
- institution_id int? - Account institution
- institution_user_id string? - Account institution user id
- last_name string? - Last Name
figshare: TimelineUpdate
Fields
- firstOnline string? - Online posted date
- publisherAcceptance string? - Date when the item was accepted for publication
- publisherPublication string? - Publish date
figshare: UploadFilePart
Fields
- endOffset int? - Indexes on byte range. zero-based and inclusive
- locked boolean? - When a part is being uploaded it is being locked, by setting the locked flag to true. No changes/uploads can happen on this part from other requests.
- partNo int? - File part id
- startOffset int? - Indexes on byte range. zero-based and inclusive
- status string? - part status
figshare: UploadInfo
Fields
- md5 string? - md5 provided on upload initialization
- name string? - name of file on upload server
- parts UploadFilePart[]? - Uploads parts
- size int? - size of file in bytes
- status string? - Upload status
- token string? - token received after initializing a file upload
figshare: User
Fields
- first_name string? - First Name
- id int? - User id
- is_active boolean? - Account activity status
- is_public boolean? - Account public status
- job_title string? - User Job title
- last_name string? - Last Name
- name string? - Full Name
- orcid_id string? - Orcid associated to this User
- url_name string? - Name that appears in website url
Import
import ballerinax/figshare;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Content & Files/Documents
Cost/Freemium
Contributors