facebook.ads
Module facebook.ads
API
Definitions
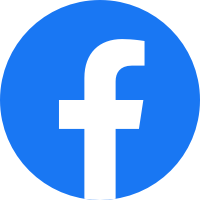
ballerinax/facebook.ads Ballerina library
Overview
This is a generated connector for Facebook Marketing API v12.0 OpenAPI specification. Facebook Marketing APIs are a collection of Graph API endpoints that can be used to help you advertise on Facebook.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Facebook account.
- Obtain tokens by following this guide.
Quickstart
To use the Facebook Ads connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/facebook.ads
module into the Ballerina project.
import ballerinax/facebook.ads;
Step 2 - Create a new connector instance
You can now make the connection configuration using the <API_KEY>.
ads:ApiKeysConfig clientConfig = { accessToken : "<API_KEY>" }; ads:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to ceate a campaign using the connector.
public function main() { string adAccountId = "403797561212027"; ads:Campaign properties = { name: "New Furniture Campaign", objective: "LINK_CLICKS", status: "PAUSED", special_ad_categories: "NONE" }; ads:CampaignResponse|error result = baseClient->createCampaign(adAccountId, properties); if (result is ads:CampaignResponse) { log:printInfo(result.toString()); } else { log:printInfo(result.toString()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
facebook.ads: Client
This is a generated connector for Facebook Marketing API v12.0 OpenAPI specification. Facebook is an American online social media and social networking service owned by Facebook, Inc.Facebook Marketing APIs are a collection of Graph API endpoints that can be used to help you advertise on Facebook.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Facebook account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://graph.facebook.com/v12.0" - URL of the target service
getCampaigns
function getCampaigns(string adAccountId, string? datePreset, string[]? effectiveStatus, boolean? isCompleted, TimeRange? timeRange, string[]? fields, string[]? summary) returns CampaignList|error
Returns campaigns under this ad account.
Parameters
- adAccountId string - ID of the ad account.
- datePreset string? (default ()) - Predefined date range used to aggregate insights metrics.
- effectiveStatus string[]? (default ()) - Effective status for the campaigns
- isCompleted boolean? (default ()) - If true, we return completed campaigns.
- timeRange TimeRange? (default ()) - Date range used to aggregate insights metrics
- fields string[]? (default ()) - Fields of the campaign
- summary string[]? (default ()) - Aggregated information about the edge, such as counts
Return Type
- CampaignList|error - Success
createCampaign
function createCampaign(string adAccountId, Campaign properties) returns CampaignResponse|error
Cerates a campaign.
Return Type
- CampaignResponse|error - Success
dissociateCampaign
function dissociateCampaign(string adAccountId, string deleteStrategy, string? beforeDate, int? objectCount) returns CampaignDissociateResponse|error
Dissociate a campaign from an AdAccount.
Parameters
- adAccountId string - ID of the ad account.
- deleteStrategy string - Delete strategy
- beforeDate string? (default ()) - Set a before date to delete campaigns before this date
- objectCount int? (default ()) - Object count
Return Type
- CampaignDissociateResponse|error - Success
updateCampaign
function updateCampaign(string campaignId, CampaignUpdate properties) returns CampaignResponse|error
Updates a campaign.
Parameters
- campaignId string - ID of the campaign.
- properties CampaignUpdate - Campaign update properties
Return Type
- CampaignResponse|error - Success
deleteCampaign
function deleteCampaign(string campaignId) returns CampaignResponse|error
Deletes a campaign.
Parameters
- campaignId string - ID of the campaign.
Return Type
- CampaignResponse|error - Success
getAdSets
function getAdSets(string adAccountId, string? datePreset, TimeRange? timeRange, string[]? fields) returns AdSetList|error
Returns all ad sets from one ad account
Parameters
- adAccountId string - ID of the ad account.
- datePreset string? (default ()) - Predefined date range used to aggregate insights metrics.
- timeRange TimeRange? (default ()) - Time Range. Note if time range is invalid, it will be ignored.
- fields string[]? (default ()) - Fields of the ad set
createAdSet
function createAdSet(string adAccountId, AdSet properties) returns AdSetResponse|error
Cerates an ad set.
Return Type
- AdSetResponse|error - Success
getAdSet
function getAdSet(string adSetId, string? datePreset, TimeRange? timeRange, string[]? fields) returns AdSet|error
Return date related to an ad set.
Parameters
- adSetId string - ID of the ad set.
- datePreset string? (default ()) - Predefined date range used to aggregate insights metrics.
- timeRange TimeRange? (default ()) - Time Range. Note if time range is invalid, it will be ignored.
- fields string[]? (default ()) - Fields of the ad set
updateAdSet
function updateAdSet(string adSetId, AdSetUpdate properties) returns AdSetResponse|error
Updates an ad set.
Return Type
- AdSetResponse|error - Success
deleteAdSet
function deleteAdSet(string adSetId) returns AdSetResponse|error
Deletes a ad set.
Parameters
- adSetId string - ID of the ad set.
Return Type
- AdSetResponse|error - Success
getAds
function getAds(string adAccountId, string? datePreset, string[]? effectiveStatus, TimeRange? timeRange, int? updatedSince, string[]? fields, string[]? summary) returns AdList|error
Returns ads under this ad account.
Parameters
- adAccountId string - ID of the ad account.
- datePreset string? (default ()) - Predefined date range used to aggregate insights metrics.
- effectiveStatus string[]? (default ()) - Effective status for the ads
- timeRange TimeRange? (default ()) - Date range used to aggregate insights metrics
- updatedSince int? (default ()) - Updated since.
- fields string[]? (default ()) - Fields of the campaign
- summary string[]? (default ()) - Aggregated information about the edge, such as counts
createAd
function createAd(string adAccountId, Ad properties) returns AdResponse|error
Cerates an ad.
Return Type
- AdResponse|error - Success
getAd
function getAd(string adId, string? datePreset, TimeRange? timeRange, int? updatedSince, string[]? fields) returns Ad|error
Returns data of an ad.
Parameters
- adId string - ID of the ad
- datePreset string? (default ()) - Predefined date range used to aggregate insights metrics.
- timeRange TimeRange? (default ()) - Date range used to aggregate insights metrics
- updatedSince int? (default ()) - Updated since.
- fields string[]? (default ()) - Fields of the campaign
updateAd
function updateAd(string adId, AdUpdate properties) returns AdResponse|error
Updates an ad.
Return Type
- AdResponse|error - Success
deleteAd
function deleteAd(string adId) returns AdResponse|error
Deletes an ad.
Parameters
- adId string - ID of the ad
Return Type
- AdResponse|error - Success
Records
facebook.ads: Ad
Ad properties
Fields
- adlabels record {}[]? - Ad labels associated with this ad
- adset_id int? - The ID of the ad set, required on creation
- adset_spec record {}? - The ad set spec for this ad. When the spec is provided, adset_id field is not required.
- audience_id string? - The ID of the ad set, required on creation
- bid_amount int? - Bid amount for this ad which will be used in auction instead of the ad set bid_amount, if specified.
- conversion_domain string? - The domain where conversions happen. Required to create or update an ad in a campaign that shares data with a pixel.
- creative record {} - This field is required for create. The ID or creative spec of the ad creative to be used by this ad.
- date_format string? - The format of the date.
- display_sequence int? - The sequence of the ad within the same campaign
- engagement_audience string? - Flag to create a new audience based on users who engage with this ad
- execution_options string[]? - An execution setting
- include_demolink_hashes boolean? - Include the demolink hashes
- name string - Name of the ad
- priority int? - Priority
- status string? - Only ACTIVE and PAUSED are valid during creation. Other statuses can be used for update
- tracking_specs record {}? - With Tracking Specs, you log actions taken by people on your ad.
facebook.ads: AdList
Ad list response
Fields
- data Ad[]? - An array of ads
- paging Paging? - A cursor-paginated edge.
- summary ListSummary? - List summary
facebook.ads: AdResponse
Adgroup operation response
Fields
- id string? - Ad ID
- success boolean? - Sucess status
facebook.ads: AdSet
Ad set properties
Fields
- adlabels record {}[]? - Specifies list of labels to be associated with this object. This field is optional
- adset_schedule AdSetSchedule[]? - Ad set schedule, representing a delivery schedule for a single day
- attribution_spec AttributionSpec[]? - Array of attribution specs
- bid_amount int? - Bid cap or target cost for this ad set. The bid cap used in a lowest cost bid strategy is defined as the maximum bid you want to pay for a result based on your optimization_goal.
- bid_strategy string? - Bid strategy for this campaign to suit your specific business goals
- billing_event string? - The billing event
- campaign_spec CampignSpec? - Campaign specifications
- contextual_bundling_spec ContextualBundlingSpec? - Settings of Contextual Bundle to support ads serving in Facebook contextual surfaces
- daily_budget int? - The daily budget defined in your account currency, allowed only for ad sets with a duration (difference between end_time and start_time) longer than 24 hours.
- daily_imps int? - Daily impressions. Available only for campaigns with buying_type=FIXED_CPM.
- daily_min_spend_target int? - Daily minimum spend target of the ad set defined in your account currency. To use this field, daily budget must be specified in the Campaign.
- daily_spend_cap int? - Daily spend cap of the ad set defined in your account currency. To use this field, daily budget must be specified in the Campaign.
- destination_type string? - The billing event
- end_time string? - End time, required when lifetime_budget is specified
- execution_options string[]? - An execution setting
- existing_customer_budget_percentage int? - Existing customer budget percentage
- is_dynamic_creative boolean?(default false) - Indicates the ad set must only be used for dynamic creatives. Dynamic creative ads can be created in this ad set. Defaults to false
- lifetime_budget int? - Lifetime budget of this campaign. All adsets under this campaign will share this budget. You can either set budget at the campaign level or at the adset level, not both.
- lifetime_imps int? - Lifetime impressions. Available only for campaigns with buying_type=FIXED_CPM
- lifetime_min_spend_target int? - Lifetime minimum spend target of the ad set defined in your account currency. To use this field, lifetime budget must be specified in the Campaign.
- lifetime_spend_cap int? - Lifetime spend cap of the ad set defined in your account currency. To use this field, lifetime budget must be specified in the Campaign.
- multi_optimization_goal_weight string? - Multi optimization goal weight
- name string - Ad set name, max length of 400 characters.
- optimization_goal string? - What the ad set is optimizing for.
- optimization_sub_event string? - What the ad set is optimizing for.
- pacing_type string[]? - Defines the pacing type, standard by default or using ad scheduling
- promoted_object PromotedObject? - The object this campaign is promoting across all its ads
- start_time string? - The start time of the set
- status string? - Only ACTIVE and PAUSED are valid during creation. Other statuses can be used for update
- targeting record {}? - An ad set's targeting structure. "countries" is required.
- time_based_ad_rotation_id_blocks int[]? - Specify ad creative that displays at custom date ranges in a campaign as an array. A list of Adgroup IDs
- time_based_ad_rotation_intervals int[]? - Date range when specific ad creative displays during a campaign. Provide date ranges in an array of UNIX timestamps where each timestamp represents the start time for each date range.
- time_start string? - Time start
- time_stop string? - Time stop
- tune_for_category string? - Tune for category
facebook.ads: AdsetBudgets
Adset Budgets
Fields
- adset_id string? - Adset ID
- daily_budget int? - Daily budget
- lifetime_budget int? - Lifetime budget
facebook.ads: AdSetList
Ad set list response
Fields
- data AdSet[]? - An array of ad sets
- paging Paging? - A cursor-paginated edge.
- summary ListSummary? - List summary
facebook.ads: AdSetResponse
Ad set operation response
Fields
- id string? - Ad set ID
- success boolean? - Sucess status
facebook.ads: AdSetSchedule
Ad set schedule, representing a delivery schedule for a single day
Fields
- start_minute int? - A 0 based minute of the day representing when the schedule starts
- end_minute int - A 0 based minute of the day representing when the schedule ends
- days int[] - Array of ints representing which days the schedule is active. Valid values are 0-6 with 0 representing Sunday, 1 representing Monday, ... and 6 representing Saturday.
- timezone_type string?(default "USER") - Timezone type
facebook.ads: AdSetUpdate
Ad set update properties
Fields
- account_id string? - Ad Account ID
- adlabels record {}[]? - Specifies list of labels to be associated with this object. This field is optional
- adset_schedule AdSetSchedule[]? - Ad set schedule, representing a delivery schedule for a single day
- attribution_spec AttributionSpec[]? - Array of attribution specs
- bid_amount int? - Bid cap or target cost for this ad set. The bid cap used in a lowest cost bid strategy is defined as the maximum bid you want to pay for a result based on your optimization_goal.
- bid_strategy string? - Bid strategy for this campaign to suit your specific business goals
- billing_event string? - The billing event
- contextual_bundling_spec ContextualBundlingSpec? - Settings of Contextual Bundle to support ads serving in Facebook contextual surfaces
- daily_budget int? - The daily budget defined in your account currency, allowed only for ad sets with a duration (difference between end_time and start_time) longer than 24 hours.
- daily_imps int? - Daily impressions. Available only for campaigns with buying_type=FIXED_CPM.
- daily_min_spend_target int? - Daily minimum spend target of the ad set defined in your account currency. To use this field, daily budget must be specified in the Campaign.
- daily_spend_cap int? - Daily spend cap of the ad set defined in your account currency. To use this field, daily budget must be specified in the Campaign.
- destination_type string? - The billing event
- end_time string? - End time, required when lifetime_budget is specified
- execution_options string[]? - An execution setting
- existing_customer_budget_percentage int? - Existing customer budget percentage
- is_dynamic_creative boolean? - Indicates the ad set must only be used for dynamic creatives. Dynamic creative ads can be created in this ad set. Defaults to false
- lifetime_budget int? - Lifetime budget of this campaign. All adsets under this campaign will share this budget. You can either set budget at the campaign level or at the adset level, not both.
- lifetime_imps int? - Lifetime impressions. Available only for campaigns with buying_type=FIXED_CPM
- lifetime_min_spend_target int? - Lifetime minimum spend target of the ad set defined in your account currency. To use this field, lifetime budget must be specified in the Campaign.
- lifetime_spend_cap int? - Lifetime spend cap of the ad set defined in your account currency. To use this field, lifetime budget must be specified in the Campaign.
- multi_optimization_goal_weight string? - Multi optimization goal weight
- name string? - Ad set name, max length of 400 characters.
- optimization_goal string? - What the ad set is optimizing for.
- optimization_sub_event string? - What the ad set is optimizing for.
- pacing_type string[]? - Defines the pacing type, standard by default or using ad scheduling
- promoted_object PromotedObject? - The object this campaign is promoting across all its ads
- start_time string? - The start time of the set
- status string? - Only ACTIVE and PAUSED are valid during creation. Other statuses can be used for update
- targeting record {}? - An ad set's targeting structure. "countries" is required.
- time_based_ad_rotation_id_blocks int[]? - Specify ad creative that displays at custom date ranges in a campaign as an array. A list of Adgroup IDs
- time_based_ad_rotation_intervals int[]? - Date range when specific ad creative displays during a campaign. Provide date ranges in an array of UNIX timestamps where each timestamp represents the start time for each date range.
- time_start string? - Time start
- time_stop string? - Time stop
- tune_for_category string? - Tune for category
facebook.ads: AdUpdate
Ad update properties
Fields
- adlabels record {}[]? - Ad labels associated with this ad
- audience_id string? - The ID of the audience
- bid_amount int? - Bid amount for this ad which will be used in auction instead of the ad set bid_amount, if specified. Any updates to the ad set bid_amount will overwrite this value with the new ad set value.
- conversion_domain string? - The domain where conversions happen. Required to create or update an ad in a campaign that shares data with a pixel.
- creative record {} - This field is required for create. The ID or creative spec of the ad creative to be used by this ad.
- display_sequence int? - The sequence of the ad within the same campaign
- engagement_audience string? - Flag to create a new audience based on users who engage with this ad
- execution_options string[]? - An execution setting
- include_demolink_hashes boolean? - Include the demolink hashes
- name string? - Name of the ad
- status string? - Only ACTIVE and PAUSED are valid during creation. Other statuses can be used for update
- tracking_specs record {}? - With Tracking Specs, you log actions taken by people on your ad.
facebook.ads: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- accessToken string - Represents Access Token
access_token
facebook.ads: AttributionSpec
Conversion attribution spec used for attributing conversions for optimization. Supported window lengths differ by optimization goal and campaign objective.
Fields
- event_type string? - Event type
- window_days int? - Window days
facebook.ads: Campaign
Campaign properties
Fields
- adlabels record {}[]? - Ad Labels associated with this campaign
- buying_type string? - This field will help Facebook make optimizations to delivery, pricing, and limits. All ad sets in this campaign must match the buying type.
Possible values are
- AUCTION (default)
- RESERVED (for reach and frequency ads)
- bid_strategy string? - Bid strategy for this campaign to suit your specific business goals
- campaign_optimization_type string? - Campaign optimization type
- daily_budget int? - Daily budget of this campaign. All adsets under this campaign will share this budget.
- execution_options string[]? - An execution setting
- is_skadnetwork_attribution boolean? - To create an iOS 14 campaign, enable SKAdNetwork attribution for this campaign
- is_using_l3_schedule boolean? - Is using l3 schedule
- iterative_split_test_configs string[]? - Array of Iterative Split Test Configs created under this campaign
- lifetime_budget int? - Lifetime budget of this campaign. All adsets under this campaign will share this budget. You can either set budget at the campaign level or at the adset level, not both.
- name string? - Name for this campaign
- objective string? - Campaign's objective. If it is specified the API will validate that any ads created under the campaign match that objective.
- promoted_object PromotedObject? - The object this campaign is promoting across all its ads
- special_ad_categories string[] - Special ad categories
- special_ad_category_country string? - Special ad category country
- spend_cap int? - A spend cap for the campaign, such that it will not spend more than this cap. Defined as integer value of subunit in your currency with a minimum value of $100 USD (or approximate local equivalent).
- start_time string? - Start time
- stop_time string? - Stop time
- status string? - Only ACTIVE and PAUSED are valid during creation. Other statuses can be used for update
- upstream_events record {}? - Upstream events
facebook.ads: CampaignDissociateResponse
Campaign dissociate operation response
Fields
- objects_left_to_delete_count int? - Count of objects left to delete
- deleted_object_ids string[]? - Deleted object IDs
facebook.ads: CampaignList
Campaign list response
Fields
- data Campaign[]? - An array of campaigns
- paging Paging? - A cursor-paginated edge.
- summary ListSummary? - List summary
facebook.ads: CampaignResponse
Campaign operation response
Fields
- id string? - Campaign ID
- success boolean? - Sucess status
facebook.ads: CampaignUpdate
Campaign operationupdate properties
Fields
- adlabels record {}[]? - Ad Labels associated with this campaign
- adset_bid_amounts record {}? - A map of child adset IDs to their respective bid amounts required in the process of toggling campaign from autobid to manual bid
- adset_budgets AdsetBudgets? - Adset Budgets
- bid_strategy string? - Bid strategy for this campaign to suit your specific business goals
- budget_rebalance_flag boolean? - Whether to automatically rebalance budgets daily for all the adsets under this campaign
- campaign_optimization_type string? - Campaign optimization type
- daily_budget int? - Daily budget of this campaign. All adsets under this campaign will share this budget.
- execution_options string[]? - An execution setting
- is_skadnetwork_attribution boolean? - To create an iOS 14 campaign, enable SKAdNetwork attribution for this campaign
- is_using_l3_schedule boolean? - Is using l3 schedule
- iterative_split_test_configs string[]? - An Array of Iterative Split Test Configs created under this campaign
- lifetime_budget int? - Lifetime budget of this campaign. All adsets under this campaign will share this budget. You can either set budget at the campaign level or at the adset level, not both.
- name string? - Name for this campaign
- objective string? - Campaign's objective. If it is specified the API will validate that any ads created under the campaign match that objective.
- promoted_object PromotedObject? - The object this campaign is promoting across all its ads
- smart_promotion_type string? - Samrt promotion type
- special_ad_categories string[] - Special ad categories
- special_ad_category_country string? - Special ad category country
- spend_cap int? - A spend cap for the campaign, such that it will not spend more than this cap. Defined as integer value of subunit in your currency with a minimum value of $100 USD (or approximate local equivalent).
- start_time string? - Start time
- stop_time string? - Stop time
- status string? - Only ACTIVE and PAUSED are valid during creation. Other statuses can be used for update
- upstream_events record {}? - Upstream events
facebook.ads: CampignSpec
Campaign specifications
Fields
- name string? - Name of the campaign
- objective string? - Campaign's objective. If it is specified the API will validate that any ads created under the campaign match that objective.
- buying_type string? - This field will help Facebook make optimizations to delivery, pricing, and limits. All ad sets in this campaign must match the buying type.
Possible values are
- AUCTION (default)
- RESERVED (for reach and frequency ads)
facebook.ads: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
facebook.ads: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
facebook.ads: ContextualBundlingSpec
Settings of Contextual Bundle to support ads serving in Facebook contextual surfaces
Fields
- status string? - Status
facebook.ads: ListSummary
List summary
Fields
- insights record {}? - Analytics summary for all objects
- total_count int? - Total number of objects
facebook.ads: OmnichannelObject
Omnichannel object
Fields
- app record {}[]? - App objects
- pixel record {}[]? - Pixel objects
- onsite record {}[]? - Onsite objects
facebook.ads: Paging
A cursor-paginated edge.
Fields
- cursors PagingCursors? - Pagination cursor.
- next anydata? - The Graph API endpoint that will return the next page of data. If not included, this is the last page of data. Due to how pagination works with visibility and privacy, it is possible that a page may be empty but contain a next paging link. Stop paging when the next link no longer appears.
- previous anydata? - The Graph API endpoint that will return the previous page of data. If not included, this is the first page of data.
facebook.ads: PagingCursors
Pagination cursor.
Fields
- before string? - This is the cursor that points to the start of the page of data that has been returned.
- after string? - This is the cursor that points to the end of the page of data that has been returned.
facebook.ads: PromotedObject
The object this campaign is promoting across all its ads
Fields
- application_id int? - The ID of a Facebook Application. Usually related to mobile or canvas games being promoted on Facebook for installs or engagement
- custom_event_type string? - The event from an App Event of a mobile app, not in the standard event list
- object_store_url string? - The uri of the mobile / digital store where an application can be bought / downloaded
- page_id string? - The ID of a Facebook Page
- custom_event_str string? - The event from an App Event of a mobile app, not in the standard event list.
- omnichannel_object OmnichannelObject? - Omnichannel object
- post_conversions record {}[]? - Post conversions
facebook.ads: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
facebook.ads: TimeRange
Date range used to aggregate insights metrics
Fields
- since string? - A date in the format of "YYYY-MM-DD", which means from the beginning midnight of that day
- until string? - A date in the format of "YYYY-MM-DD", which means to the beginning midnight of the following day
Import
import ballerinax/facebook.ads;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 3
Current verison: 0
Weekly downloads
Keywords
Marketing/Social Media Accounts
Cost/Free
Contributors
Dependencies