eventbrite
Module eventbrite
API
Definitions
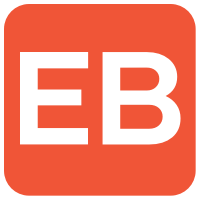
ballerinax/eventbrite Ballerina library
Overview
This is a generated connector for Eventbrite API v3 OpenAPI specification. Eventbrite is an Event Management System with an API which allows creating and managing events including venue management, marketing, selling tickets, and financial management.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an Eventbrite account
- Obtain tokens by following this guide
Quickstart
To use the Eventbrite connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/eventbrite
module into the Ballerina project.
import ballerinax/eventbrite;
Step 2: Create a new connector instance
Create a eventbrite:ClientConfig
with the Bearer_Token
obtained, and initialize the connector with it.
eventbrite:ClientConfig clientConfig = { auth: { token: <Bearer_Token> } }; eventbrite:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list events by organization ID using the connector.
List Events by Organization ID.
public function main() returns error? { eventbrite:EventsByOrganization response = check baseClient->listEventsByOrganization(<organizationId>); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
eventbrite: Client
This is a generated connector for Eventbrite API v3 OpenAPI specification. Eventbrite is an Event Management System with an API which allows creating and managing events including venue management, marketing, selling tickets, and financial management.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Eventbrite account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://www.eventbriteapi.com/v3" - URL of the target service
listOrganizations
function listOrganizations() returns Organizations|error
Returns a list of the organizations to which you are a member. Returns a paginated response.
Return Type
- Organizations|error - An object with a single property organizations which is an array of Organization objects.
listOrganizationsByUser
function listOrganizationsByUser(string userId) returns Organizations|error
Returns the list organizations by user ID. Returns a paginated response.
Parameters
- userId string - ID of User. Example: 12345.
Return Type
- Organizations|error - An object with a single property organizations which is an array of Organization objects.
listEventsByOrganization
function listEventsByOrganization(string organizationId, string? nameFilter, string? currencyFilter, string? orderBy, boolean? seriesFilter, boolean? showSeriesParent, string? status, string? eventGroupId, string? collectionId, decimal? pageSize, string? timeFilter, string? inventoryTypeFilter, string? eventIdsToExclude, string? collectionIdsToExclude) returns EventsByOrganization|error
List Events by Organization ID. Returns a paginated response.
Parameters
- organizationId string - Organization ID Example: 12345.
- nameFilter string? (default ()) - Filter Organization's Events by specified name.
- currencyFilter string? (default ()) - Filter Organization's Events by specified currency.
- orderBy string? (default ()) - Sort order for the list of Events.
- seriesFilter boolean? (default ()) - Filter based on whether an event is not a series, a series, child series or parent series. This filter has higher precedence than show series parent filter. Default will use show series parent filter behavior.
- showSeriesParent boolean? (default ()) - false (Default) = In the list, show the series children and not series parent. true = In the list, show the series parent instead of series.
- status string? (default ()) - Filter Organization's Events by status. Specify multiple status values as a comma delimited
- eventGroupId string? (default ()) - Filter Organization's Events by event_group_id.
- collectionId string? (default ()) - Filter Organization's Events by collection_id.
- pageSize decimal? (default ()) - Number of records to display on each page of the list.
- timeFilter string? (default ()) - Limits the list results to either past or current and future Events.
- inventoryTypeFilter string? (default ()) - Filter Organization's Events by Inventory Type.
- eventIdsToExclude string? (default ()) - IDs of events to exclude from the Organization's Events list.
- collectionIdsToExclude string? (default ()) - IDs of collections to exclude from the Organization's Events list. This will have precedence over event_group_id filter and collection_id filter.
Return Type
- EventsByOrganization|error - An object with a property events which is an array of Event objects.
createEvent
function createEvent(string organizationId, CreateEventRequest payload) returns CreatedEvent|error
Create a new Event.
Parameters
- organizationId string - ID of the Organization that owns the Event. Example: 12345.
- payload CreateEventRequest - An object with a single property event which must be an Event object.
Return Type
- CreatedEvent|error - Created new Event
publishEvent
function publishEvent(string eventId) returns PublishedEvent|error
Publish an Event.
Parameters
- eventId string - Event ID. Example: 12345.
Return Type
- PublishedEvent|error - Published event status
listDiscounts
function listDiscounts(string organizationId, string? scope, string? codeFilter, string? code, decimal? pageSize, string? 'type, string? ticketGroupId, string? eventId, string? orderBy, string? holdIds) returns Discounts|error
List Discounts by Organization ID. Returns a paginated response.
Parameters
- organizationId string - Organization ID. Example: 12345.
- scope string? (default ()) - Discount scope. Example: event
- codeFilter string? (default ()) - Filter Discounts by approximate match code or name
- code string? (default ()) - Filter Discount by exact match code or name. Example: abcde
- pageSize decimal? (default ()) - Number of records to display on each returned page.
- 'type string? (default ()) - Discount type
- ticketGroupId string? (default ()) - Filter Discounts by Ticket Group ID. Example: 12345
- eventId string? (default ()) - Filter Discounts by Event ID. Required for the event scope. Example: 12345
- orderBy string? (default ()) - Response order.
- holdIds string? (default ()) - Filter discounts to only those that apply to specified hold IDs. IDs are in composite id format for either hold class (H123) or hold inventory tier (I123)
Return Type
createDiscount
function createDiscount(string organizationId, CreateDiscountRequest payload) returns CreatedDiscount|error
Create a new Discount.
Parameters
- organizationId string - ID of the Organization that owns the Event. Example: 12345.
- payload CreateDiscountRequest - An object with a single property discount which must be an Discount object.
Return Type
- CreatedDiscount|error - Created new Discount
createTicketClass
function createTicketClass(string eventId, CreateTicketClassRequest payload) returns CreatedTicketClass|error
Create a new Ticket Class.
Parameters
- eventId string - Event ID. Example: 12345.
- payload CreateTicketClassRequest - An object with a single property ticket_class which must be an TicketClass object.
Return Type
- CreatedTicketClass|error - Created ticket class
listCategories
function listCategories() returns Categories|error
Returns a list of Category as categories, including subcategories nested. Returns a paginated response.
Return Type
- Categories|error - An object with a single property categories which is an array of Category objects.
listEventsByVenue
function listEventsByVenue(string venueId, string? status, string? orderBy, string? startDate, boolean? onlyPublic) returns EventsByVenue|error
List Events by Venue ID. Returns a paginated response.
Parameters
- venueId string - Venue ID. Example: 12345.
- status string? (default ()) - Filter Events at the Venue by status. Specify multiple status values as a comma delimited string. Example: live.
- orderBy string? (default ()) - Sort order of list of Events at the Venue. Example: start_asc.
- startDate string? (default ()) - Filter Events at the Venue by a specified date range.
- onlyPublic boolean? (default ()) - True = Filter public Events at the Venue. Example: true
Return Type
- EventsByVenue|error - An object with a property events which is an array of Event objects.
getUserDetails
function getUserDetails(string userId) returns UserObject|error
Retrieve Information about a User Account.
Parameters
- userId string - User ID. Example: 12345.
Return Type
- UserObject|error - An object with a property user which is a User object.
listOrdersByOrganization
function listOrdersByOrganization(string organizationId, string? status, string? changedSince, string? onlyEmails, boolean? excludeEmails) returns OrdersByOrganization|error
Returns a paginated response of orders, under the key orders, of orders placed against any of the events the organization owns (events that would be returned from /organizations/:id/events/).
Parameters
- organizationId string - Organization ID. Example: 12345.
- status string? (default ()) - Filter Event's Order by status. Example: active
- changedSince string? (default ()) - Only return orders changed on or after the time given.
- onlyEmails string? (default ()) - Only include orders placed by one of these emails.
- excludeEmails boolean? (default ()) - Don't include orders placed by any of these emails
Return Type
- OrdersByOrganization|error - An object with a property orders which is an array of Order objects.
listOrdersByEvent
function listOrdersByEvent(string eventId, string? status, string? changedSince, string? lastItemSeen, boolean? onlyEmails, string? excludeEmails, string? refundRequestStatuses) returns OrdersByEvent|error
List Orders by Event ID. Returns a paginated response.
Parameters
- eventId string - Event ID. Example: 12345.
- status string? (default ()) - Filter Event's Order by status. Example: active
- changedSince string? (default ()) - Orders changed on or after this time.
- lastItemSeen string? (default ()) - Orders changed on or after this time, and with an ID larger than last item seen.
- onlyEmails boolean? (default ()) - Orders placed by this email address
- excludeEmails string? (default ()) - Orders placed by this email address are not included in return
- refundRequestStatuses string? (default ()) - Only Orders with specified refund request status are included in return.
Return Type
- OrdersByEvent|error - An object with a property orders which is an array of Order objects.
listOrdersByUser
function listOrdersByUser(string userId, string? changedSince, string? timeFilter) returns OrdersByOrganization|error
List Orders by User ID. Returns a paginated response.
Parameters
- userId string - User ID. Example: 12345.
- changedSince string? (default ()) - Orders changed on or after this time.
- timeFilter string? (default ()) - Filter the list to only, or all, past or current and future Orders.
Return Type
- OrdersByOrganization|error - An object with a property orders which is an array of Order objects.
listAttendees
function listAttendees(string organizationId, string? status, string? changedSince) returns AttendeesByOrganization|error
List Attendees of an Organization's Events by Organization ID. Returns a paginated response.
Parameters
- organizationId string - Organization ID. Example: 12345.
- status string? (default ()) - Filter Attendees by status of either attending or not_attending
- changedSince string? (default ()) - Filter Attendees by resource changed on or after the time given.
Return Type
- AttendeesByOrganization|error - An object with a property attendees which is an array of Attendee objects.
listAttendeesByEvent
function listAttendeesByEvent(string eventId, string? status, string? changedSince, string? lastItemSeen, boolean? attendeeIds) returns AttendeesByEvent|error
List Attendees by Event ID. Returns a paginated response.
Parameters
- eventId string - Event ID. Example: 12345.
- status string? (default ()) - Filter Attendees by status of either attending, not attending or unpaid
- changedSince string? (default ()) - Filter Attendees changed on or after the specified time.
- lastItemSeen string? (default ()) - When passed in conjunction with changed_since, filter Attendees changed on or after the specified time and with an ID later than the value of thelast_item_seen field.
- attendeeIds boolean? (default ()) - Filter Attendees with the specified IDs
Return Type
- AttendeesByEvent|error - An object with a property attendees which is an array of Attendee objects.
getAttendeeDetails
Retrieve an Attendee by Attendee ID.
Parameters
- eventId string - Event ID. Example: 12345.
- attendeeId string - Attendee ID. Example: 12345.
listVenues
List Venues by Organization ID. Returns a paginated response.
Parameters
- organizationId string - Organization ID. Example: 12345.
createVenue
function createVenue(string organizationId, CreateVenueRequest payload) returns CreatedVenue|error
Create new Venue under an Organization.
Parameters
- organizationId string - ID of the Organization that owns the Event. Example: 12345.
- payload CreateVenueRequest - An object with a single property venue which must be a Venue object.
Return Type
- CreatedVenue|error - Created Venue
listTeams
Returns a list of teams for the event. Returns a paginated response.
Parameters
- eventId string - Event ID. Example: 12345.
listAttendeesByTeam
Returns attendee for a single team. Returns a paginated response.
Return Type
getTeamDetails
Returns information for a single team.
getEventDetails
function getEventDetails(string eventId) returns EventObject|error
Retrieve an Event by Event ID.
Parameters
- eventId string - Event ID. Example: 12345.
Return Type
- EventObject|error - An object which is an Event object.
Records
eventbrite: ActualCost
The total cost for this ticket class less the fee
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: ActualFee
The fee for this ticket class
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: Address
The address of the venue
Fields
- address_1 string? - The street/location address (part 1)
- address_2 string? - The street/location address (part 2)
- city string? - City
- region string? - ISO 3166-2 2- or 3-character region code for the state, province, region, or district
- postal_code string? - Postal code
- country string? - ISO 3166-1 2-character international code for the country
- latitude string? - Latitude portion of the address coordinates
- longitude string? - Longitude portion of the address coordinates
eventbrite: Attendee
An object which is an Attendee object.
Fields
- id string? - Attendee ID
- created string? - When the attendee was created (order placed)
- changed string? - When the attendee was last changed
- ticket_class_id string? - The ticket_class that the attendee registered with
- ticket_class_name string? - The name of the ticket_class at the time of registration
- affiliate string? - The attendee's affiliate code
- checked_in boolean? - If the attendee is checked in
- cancelled boolean? - If the attendee is cancelled
- refunded boolean? - If the attendee is refunded
- status string? - The status of the attendee (scheduled to be deprecated)
- event_id string? - The event id that this attendee is attending
- order_id string? - The order id this attendee is part of
- guestlist_id string? - The guestlist id for this attendee. If this is null it means that this is not a guest.
- invited_by string? - The name of the person that invited the attendee. If this is null it means that this is not a guest.
- resource_uri string? - The resource URI
eventbrite: Attendees
An object with a property attendees which is an array of Attendee objects.
Fields
- pagination Pagination? - Pagination
- venues Attendee[]? - An array of Attendee objects.
eventbrite: AttendeesByEvent
An object with a property attendees which is an array of Attendee objects.
Fields
- pagination Pagination? - Pagination
- attendees Attendee[]? - An array of attendee objects.
eventbrite: AttendeesByOrganization
An object with a property attendees which is an array of Attendee objects.
Fields
- pagination Pagination? - Pagination
- attendees Attendee[]? - An array of attendee objects.
eventbrite: BasePrice
The organizer ticket revenue.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: BookmarkInfo
The bookmark information on the event, requires the bookmark_info expansion
Fields
- bookmarked boolean? - User saved the event or not
eventbrite: Categories
An object with a property categories which is an array of Category objects.
Fields
- locale string? - Locale
- pagination Pagination? - Pagination
- opportunities Category[]? - An array of Category objects.
eventbrite: Category
Full details of the event category (requires the category expansion)
Fields
- id string? - Category ID
- resource_uri string? - Resource URI
- name string? - Category name
- name_localized string? - Translated category name
- short_name string? - Shorter category name for display in sidebars and other small spaces
- short_name_localized string? - Translated short category name
- subcategories Subcategory[]? - List of subcategories (only shown on some endpoints)
eventbrite: CheckoutSettings
Additional data about the checkout settings of the Event.
Fields
- created string? - When the checkout settings object was created
- changed string? - When the checkout settings object was last changed
- country_code string? - The ISO 3166 alpha-2 code of the country within which these checkout settings can apply.
- currency_codes string? - The ISO 4217 3-character code of the currency for which these checkout settings can apply.
- checkout_method string? - Checkout method
- offline_settings OfflineSettings[]? - A container for representing additional offline payment method checkout settings.
- user_instrument_vault_id string? - The merchant account user instrument ID for the checkout method. Only specify this value for PayPal and Authorize.net checkout settings.
eventbrite: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
eventbrite: ConfirmationMessage
Order confirmation message from event settings.
Fields
- text string - Description of the confirmation message in text format
- html string - Description of the confirmation message in html format
eventbrite: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
eventbrite: ContactListPreferences
Marketing communication preferences for the email address associated with this Order.
Fields
- _type string? - order_contact_list_preferences
- has_contact_list boolean? - Email address is in at least one contact list for Organization that owns this Event.
- has_opted_in boolean? - Email address is opt-in to receive marketing communication from the Organization that owns this Event.
eventbrite: Cost
Cost of the ticket (currently currency must match event currency) e.g. $45 would be "USD,4500"
Fields
- actual_cost ActualCost? - The total cost for this ticket class less the fee
- actual_fee ActualFee? - The fee for this ticket class
- cost TicketCost? - The display cost for the ticket
- fee Fee? - The fee that should be included in the price (0 if include_fee is false).
- tax Tax? - The ticket's base or discounted tax amount
eventbrite: Costs
Cost breakdown for this order
Fields
- base_price BasePrice? - The organizer ticket revenue.
- display_price DisplayPrice? - The item price with discounts applied.
- display_fee DisplayFee? - The total amount of fees and taxes to be displayed.
- gross Gross? - The total amount the buyer was charged.
- eventbrite_fee EventbriteFee? - Fees taken by Eventbrite as a service fee.
- payment_fee PaymentFee? - Fees taken by Eventbrite as a payment processing fee.
- tax CostsTax? - The tax collected by the organizer.
- display_tax DisplayTax? - The total amount of taxes to be displayed.
- price_before_discount PriceBeforeDiscount? - The item price before a discount code is applied. If no discount is applied it's the display_price.
- discount_amount DiscountAmount? - The total discount to be displayed.
- discount_type string? - Type of discount applied.
- fee_components FeeComponent[]? - List of price costs components that belong to the fee display group.
- tax_components TaxComponent[]? - List of price costs components that belong to the tax display group.
- shipping_components ShippingComponent[]? - List
- has_gts_tax boolean? - Indicates if any of the tax_components is a gts tax.
- tax_name string? - The name of the Organizer-to-Attendee tax that applies, if any.
eventbrite: CostsTax
The tax collected by the organizer.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: CreatedDiscount
The Discount object represents a discount that an Order owner can use when purchasing tickets to an Event.
Fields
- 'type string? - Type
- code string? - Code used to activate the discount
- amount_off string? - Fixed reduction amount. When creating access codes the default value is Null.
- percent_off string? - Percentage reduction. When creating access codes the default value is Null.
- event_id string? - ID of the event. Only used for single event discounts
- ticket_class_ids string[]? - IDs of the ticket classes to limit discount to
- quantity_available decimal? - Number of times the discount can be used
- start_date string? - Allow use from this date. A datetime represented as a string in Naive Local ISO8601 date and time format, in the timezone of the event.
- start_date_relative decimal? - Allow use from this number of seconds before the event starts. Greater than 59 and multiple of 60.
- end_date string? - Allow use until this date. A datetime represented as a string in Naive Local ISO8601 date and time format, in the timezone of the event.
- end_date_relative decimal? - Allow use until this number of seconds before the event starts. Greater than 59 and multiple of 60.
- ticket_group_id string? - ID of the ticket group
- hold_ids string[]? - List of hold IDs this discount can unlock. Null if this discount does not unlock a hold.
- id string? - Discount ID
- quantity_sold decimal? - Number of discounts used during ticket sales
eventbrite: CreatedEvent
The Event object represents an Eventbrite Event. An Event is owned by one Organization.
Fields
- id string? - Event ID
- name Name? - Event name. Value cannot be empty nor whitespace.
- summary string? - Event summary. This is a plaintext field and will have any supplied HTML removed from it. Maximum of 140 characters, mutually exclusive with description.
- description Description? - Event description (contents of the event page). May be long and have significant formatting. Please refer to the event description tutorial to learn about the new way to create an event description.
- 'start Start? - Start date/time of the event.
- end End? - End date/time of the event.
- url string? - The URL to the event page for this event on Eventbrite
- vanity_url string? - The vanity URL to the event page for this event on Eventbrite
- created string - When the event was created
- changed string - When the event was last changed
- published string? - When the event was first published
- status string? - Status of the event
- currency string? - The ISO 4217 currency code for this event
- online_event boolean? - true = Specifies that the Event is online only (i.e. the Event does not have a Venue).
- organization_id string? - Organization owning the event
- organizer_id string? - ID of the event organizer
- organizer Organizer? - Full details of the event organizer (requires the organizer expansion)
- logo_id string? - Image ID of the event logo
- logo Logo? - Full image details for organizer logo
- venue_id string? - Event venue ID
- venue Venue? - Full venue details for venue_id (requires the venue expansion)
- format_id string? - Event format
- format Format? - Full details of the event format (requires the format expansion)
- category_id string? - Event category
- category Category? - Full details of the event category (requires the category expansion)
- subcategory_id string? - Event subcategory (Expansion is subcategory)
- subcategory Subcategory? - Full details of the event subcategory (requires the subcategory expansion)
- music_properties MusicProperties? - This is an object of properties that detail dimensions of music events.
- bookmark_info BookmarkInfo? - The bookmark information on the event, requires the bookmark_info expansion
- refund_policy string? - Refund policy
- ticket_availability TicketAvailability? - Additional data about general tickets information (optional).
- listed boolean? - Is this event publicly searchable on Eventbrite?. Default value is true.
- shareable boolean? - Can this event show social sharing buttons?
- invite_only boolean? - Can only people with invites see the event page?
- show_remaining boolean? - If the remaining number of tickets is publicly visible on the event page
- password string? - Password needed to see the event in unlisted mode
- capacity decimal? - Set specific capacity (if omitted, sums ticket capacities)
- capacity_is_custom boolean? - If True, the value of capacity is a custom-set value; if False, it's a calculated value of the total of all ticket capacities.
- tx_time_limit string? - Maximum duration (in seconds) of a transaction
- hide_start_date boolean? - If true, the event's start date should never be displayed to attendees.
- hide_end_date boolean? - If true, the event's end date should never be displayed to attendees.
- locale string? - Indicates event language on Event's listing page. (Default is en_US)
- is_locked boolean? - Is locked
- privacy_setting string? - Privacy setting
- is_externally_ticketed boolean? - true, if the Event is externally ticketed
- external_ticketing ExternalTicketing? - External ticketing
- is_series boolean? - If the event is part of a series. Specifying this attribute as True during event creation will always designate the event as a series parent, never as a series occurrence. Series occurrences must be created through the schedules API and cannot be created using the events API.
- is_series_parent boolean? - If the event is part of a series and is the series parent
- series_id string? - If the event is part of a series, this is the event id of the series parent. If the event is not part of a series, this field is omitted.
- is_reserved_seating boolean? - If the event is reserved seating
- show_pick_a_seat boolean? - For reserved seating event, if attendees can pick their seats.
- show_seatmap_thumbnail boolean? - For reserved seating event, if venue map thumbnail visible on the event page.
- show_colors_in_seatmap_thumbnail boolean? - For reserved seating event, if venue map thumbnail should have colors on the event page.
- is_free boolean? - Allows to set a free event
- 'source string? - Source of the event (defaults to API)
- 'version string? - Version
- resource_uri string? - Is an absolute URL to the API endpoint that will return you the canonical representation of the event.
- event_sales_status EventSalesStatus? - Additional data about the sales status of the event (optional).
- checkout_settings CheckoutSettings? - Additional data about the checkout settings of the Event.
eventbrite: CreateDiscountRequest
An object with a single property discount which must be an Discount object.
Fields
- event DiscountRequest? - The Discount object represents a discount that an Order owner can use when purchasing tickets to an Event.
eventbrite: CreatedTicketClass
The Ticket Class object represents a possible ticket class (i.e. ticket type) for an Event.
Fields
- description string? - Description of the ticket
- donation boolean? - Is this a donation? (user-supplied cost)
- free boolean? - Is this a free ticket?
- minimum_quantity decimal? - Minimum number per order
- maximum_quantity decimal? - Maximum number per order
- delivery_methods string[]? - A list of the available delivery methods for this ticket class
- cost Cost? - Cost of the ticket (currently currency must match event currency) e.g. $45 would be "USD,4500"
- resource_uri string? - Is an absolute URL to the API endpoint that will return you ticket class.
- image_id string? - Image ID for this ticket class. null if no image is set.
- name string? - Name of this ticket type
- display_name string? - Pretty long name of this ticket class. For tiered inventory tickets, this includes the tier name.
- sorting decimal? - Unsigned integer in the order ticket classes are sorted by.
- capacity decimal? - Total available number of this ticket, required for non-donation and non-tiered ticket classes. For normal ticket, null or 0 is not allowed. For donation ticket, null or 0 means unlimited. For tiered inventory ticket, null or 0 means capacity is only limited by tier capacity and/or event capacity.
- quantity_total decimal? - Deprecated, use capacity instead.
- quantity_sold decimal? - The number of sold tickets.
- sales_start string? - When the ticket is available for sale (leave empty for ‘when event published’)
- sales_end string? - When the ticket stops being on sale (leave empty for 'one hour before event start'). Cannot be set on series parent tickets.
- hidden boolean? - Hide this ticket
- include_fee boolean? - Absorb the fee into the displayed cost
- split_fee boolean? - Absorb the payment fee, but show the eventbrite fee
- hide_description boolean? - Hide the ticket description on the event page
- hide_sale_dates boolean? - Hide the sale dates on event landing and ticket selection page
- auto_hide boolean? - Hide this ticket when it is not on sale
- auto_hide_before string? - Override reveal date for auto-hide
- auto_hide_after string? - Override re-hide date for auto-hide
- sales_start_after string? - The ID of another ticket class - when it sells out, this class will go on sale.
- order_confirmation_message string? - Order message per ticket type
- sales_channels string[]? - A list of all supported sales channels
- inventory_tier_id string? - Optional ID of Inventory Tier with which to associate the ticket class
- secondary_assignment_enabled boolean? - Has secondary barcode assignment enabled (for ex/ RFID)
- category string? - Ticket class category to which a ticket class belongs.
eventbrite: CreatedVenue
The Venue object represents the location of an Event (i.e. where an Event takes place). Venues are grouped together by the Organization object.
Fields
- name string - The name of the venue
- age_restriction string? - The age restrictions for the venue
- capacity decimal? - Set specific capacity (if omitted, sums ticket capacities)
- address Address? - The address of the venue
- resource_uri string? - Resource uri
- id string? - Venue ID
- latitude string? - Latitude portion of the address coordinates of the venue
- longitude string? - Longitude portion of the address coordinates of the venue
eventbrite: CreateEventRequest
An object with a single property event which must be an Event object.
Fields
- event EventRequest? - The Event object represents an Eventbrite Event. An Event is owned by one Organization.
eventbrite: CreateTicketClassRequest
An object with a single property ticket_class which must be a Ticket Class object.
Fields
- ticket_class TicketClass? - The Ticket Class object represents a possible ticket class (i.e. ticket type) for an Event.
eventbrite: CreateVenueRequest
An object with a single property venue which must be a Venue object.
Fields
- event VenueRequest? - The Venue object represents the location of an Event (i.e. where an Event takes place). Venues are grouped together by the Organization object.
eventbrite: Creator
Creator object
Fields
- id string? - Team's creator ID
- name string? - Team's creator name (or email if name is null)
- emails CreatorEmail[]? - Team creator email's information
eventbrite: CreatorEmail
Team creator email's information
Fields
- verified boolean? - If the email is verified
- primary boolean? - If the email is the primary one
- email string? - The creator's email address
eventbrite: CropMask
The crop mask applied to the original image
Fields
- top_left TopLeft? - Coordinate for top-left corner of crop mask
- width decimal? - Crop mask width
- height decimal? - Crop mask height
eventbrite: Description
Event description (contents of the event page). May be long and have significant formatting. Please refer to the event description tutorial to learn about the new way to create an event description.
Fields
- html string? - Event description in html format. <p>Some text</p>.
eventbrite: Discount
The Discount object represents a discount that an Order owner can use when purchasing tickets to an Event.
Fields
- 'type string? - Type
- code string? - Code used to activate the discount
- amount_off string? - Fixed reduction amount. When creating access codes the default value is Null.
- percent_off string? - Percentage reduction. When creating access codes the default value is Null.
- event_id string? - ID of the event. Only used for single event discounts
- ticket_class_ids string[]? - IDs of the ticket classes to limit discount to
- quantity_available decimal? - Number of times the discount can be used
- start_date string? - Allow use from this date. A datetime represented as a string in Naive Local ISO8601 date and time format, in the timezone of the event.
- start_date_relative decimal? - Allow use from this number of seconds before the event starts. Greater than 59 and multiple of 60.
- end_date string? - Allow use until this date. A datetime represented as a string in Naive Local ISO8601 date and time format, in the timezone of the event.
- end_date_relative decimal? - Allow use until this number of seconds before the event starts. Greater than 59 and multiple of 60.
- ticket_group_id string? - ID of the ticket group
- hold_ids string[]? - List of hold IDs this discount can unlock. Null if this discount does not unlock a hold.
- id string? - Discount ID
- quantity_sold decimal? - Number of discounts used during ticket sales
eventbrite: DiscountAmount
The total discount to be displayed.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: DiscountObject
The total discount to be displayed to a specific cost component and the reason of the discount.
Fields
- amount DiscountAmount? - The total discount to be displayed.
- reason string? - The reason why a discount is applied to a specific cost component.
eventbrite: DiscountRequest
The Discount object represents a discount that an Order owner can use when purchasing tickets to an Event.
Fields
- 'type string? - Type
- code string? - Code used to activate the discount
- amount_off string? - Fixed reduction amount. When creating access codes the default value is Null.
- percent_off string? - Percentage reduction. When creating access codes the default value is Null.
- event_id string? - ID of the event. Only used for single event discounts
- ticket_class_ids string[]? - IDs of the ticket classes to limit discount to
- quantity_available decimal? - Number of times the discount can be used
- start_date string? - Allow use from this date. A datetime represented as a string in Naive Local ISO8601 date and time format, in the timezone of the event.
- start_date_relative decimal? - Allow use from this number of seconds before the event starts. Greater than 59 and multiple of 60.
- end_date string? - Allow use until this date. A datetime represented as a string in Naive Local ISO8601 date and time format, in the timezone of the event.
- end_date_relative decimal? - Allow use until this number of seconds before the event starts. Greater than 59 and multiple of 60.
- ticket_group_id string? - ID of the ticket group
- hold_ids string[]? - List of hold IDs this discount can unlock. Null if this discount does not unlock a hold.
eventbrite: Discounts
An object with a property discounts which is an array of Discount objects.
Fields
- discounts Discount[]? - An array of discount objects.
eventbrite: DisplayFee
The total amount of fees and taxes to be displayed.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: DisplayPrice
The item price with discounts applied.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: DisplayTax
The total amount of taxes to be displayed.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: Email
User email object
Fields
- email string? - Email address
- verified boolean? - Has this email address been verified to belong to the user?
eventbrite: End
End date/time of the event.
Fields
- timezone string - The timezone
- utc string - The time relative to UTC
eventbrite: Event
The Event object represents an Eventbrite Event. An Event is owned by one Organization.
Fields
- id string? - Event ID
- name Name - Event name. Value cannot be empty nor whitespace.
- summary string? - Event summary. This is a plaintext field and will have any supplied HTML removed from it. Maximum of 140 characters, mutually exclusive with description.
- description Description? - Event description (contents of the event page). May be long and have significant formatting. Please refer to the event description tutorial to learn about the new way to create an event description.
- 'start Start - Start date/time of the event.
- end End - End date/time of the event.
- hide_start_date boolean? - If true, the event's start date should never be displayed to attendees.
- hide_end_date boolean? - If true, the event's end date should never be displayed to attendees.
- currency string - The ISO 4217 currency code for this event
- online_event boolean? - true = Specifies that the Event is online only (i.e. the Event does not have a Venue).
- organizer_id string? - ID of the event organizer
- logo_id string? - Image ID of the event logo
- venue_id string? - Event venue ID
- format_id string? - Event format
- category_id string? - Event category
- subcategory_id string? - Event subcategory
- listed boolean? - Is this event publicly searchable on Eventbrite?. Default value is true.
- shareable boolean? - Can this event show social sharing buttons?
- invite_only boolean? - Can only people with invites see the event page?
- show_remaining boolean? - If the remaining number of tickets is publicly visible on the event page
- password string? - Password needed to see the event in unlisted mode
- capacity decimal? - Set specific capacity (if omitted, sums ticket capacities)
- is_reserved_seating boolean? - If the event is reserved seating
- is_series boolean? - If the event is part of a series. Specifying this attribute as True during event creation will always designate the event as a series parent, never as a series occurrence. Series occurrences must be created through the schedules API and cannot be created using the events API.
- show_pick_a_seat boolean? - For reserved seating event, if attendees can pick their seats.
- show_seatmap_thumbnail boolean? - For reserved seating event, if venue map thumbnail visible on the event page.
- show_colors_in_seatmap_thumbnail boolean? - For reserved seating event, if venue map thumbnail should have colors on the event page.
- 'source string? - Source of the event (defaults to API)
- locale string? - Indicates event language on Event's listing page. (Default is en_US)
eventbrite: EventbriteFee
Fees taken by Eventbrite as a service fee.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: EventObject
The Event object represents an Eventbrite Event. An Event is owned by one Organization.
Fields
- id string? - Event ID
- name Name? - Event name. Value cannot be empty nor whitespace.
- summary string? - Event summary. This is a plaintext field and will have any supplied HTML removed from it. Maximum of 140 characters, mutually exclusive with description.
- description Description? - Event description (contents of the event page). May be long and have significant formatting. Please refer to the event description tutorial to learn about the new way to create an event description.
- 'start Start? - Start date/time of the event.
- end End? - End date/time of the event.
- url string? - The URL to the event page for this event on Eventbrite
- vanity_url string? - The vanity URL to the event page for this event on Eventbrite
- created string - When the event was created
- changed string - When the event was last changed
- published string? - When the event was first published
- status string? - Status of the event
- currency string? - The ISO 4217 currency code for this event
- online_event boolean? - true = Specifies that the Event is online only (i.e. the Event does not have a Venue).
- organization_id string? - Organization owning the event
- organizer_id string? - ID of the event organizer
- organizer Organizer? - Full details of the event organizer (requires the organizer expansion)
- logo_id string? - Image ID of the event logo
- logo Logo? - Full image details for organizer logo
- venue_id string? - Event venue ID
- venue Venue? - Full venue details for venue_id (requires the venue expansion)
- format_id string? - Event format
- format Format? - Full details of the event format (requires the format expansion)
- category_id string? - Event category
- category Category? - Full details of the event category (requires the category expansion)
- subcategory_id string? - Event subcategory (Expansion is subcategory)
- subcategory Subcategory? - Full details of the event subcategory (requires the subcategory expansion)
- music_properties MusicProperties? - This is an object of properties that detail dimensions of music events.
- bookmark_info BookmarkInfo? - The bookmark information on the event, requires the bookmark_info expansion
- refund_policy string? - Refund policy
- ticket_availability TicketAvailability? - Additional data about general tickets information (optional).
- listed boolean? - Is this event publicly searchable on Eventbrite?. Default value is true.
- shareable boolean? - Can this event show social sharing buttons?
- invite_only boolean? - Can only people with invites see the event page?
- show_remaining boolean? - If the remaining number of tickets is publicly visible on the event page
- password string? - Password needed to see the event in unlisted mode
- capacity decimal? - Set specific capacity (if omitted, sums ticket capacities)
- capacity_is_custom boolean? - If True, the value of capacity is a custom-set value; if False, it's a calculated value of the total of all ticket capacities.
- tx_time_limit string? - Maximum duration (in seconds) of a transaction
- hide_start_date boolean? - If true, the event's start date should never be displayed to attendees.
- hide_end_date boolean? - If true, the event's end date should never be displayed to attendees.
- locale string? - Indicates event language on Event's listing page. (Default is en_US)
- is_locked boolean? - Is locked
- privacy_setting string? - Privacy setting
- is_externally_ticketed boolean? - true, if the Event is externally ticketed
- external_ticketing ExternalTicketing? - External ticketing
- is_series boolean? - If the event is part of a series. Specifying this attribute as True during event creation will always designate the event as a series parent, never as a series occurrence. Series occurrences must be created through the schedules API and cannot be created using the events API.
- is_series_parent boolean? - If the event is part of a series and is the series parent
- series_id string? - If the event is part of a series, this is the event id of the series parent. If the event is not part of a series, this field is omitted.
- is_reserved_seating boolean? - If the event is reserved seating
- show_pick_a_seat boolean? - For reserved seating event, if attendees can pick their seats.
- show_seatmap_thumbnail boolean? - For reserved seating event, if venue map thumbnail visible on the event page.
- show_colors_in_seatmap_thumbnail boolean? - For reserved seating event, if venue map thumbnail should have colors on the event page.
- is_free boolean? - Allows to set a free event
- 'source string? - Source of the event (defaults to API)
- 'version string? - Version
- resource_uri string? - Is an absolute URL to the API endpoint that will return you the canonical representation of the event.
- event_sales_status EventSalesStatus? - Additional data about the sales status of the event (optional).
- checkout_settings CheckoutSettings? - Additional data about the checkout settings of the Event.
eventbrite: EventRequest
The Event object represents an Eventbrite Event. An Event is owned by one Organization.
Fields
- name Name - Event name. Value cannot be empty nor whitespace.
- summary string? - Event summary. This is a plaintext field and will have any supplied HTML removed from it. Maximum of 140 characters, mutually exclusive with description.
- description Description? - Event description (contents of the event page). May be long and have significant formatting. Please refer to the event description tutorial to learn about the new way to create an event description.
- 'start Start - Start date/time of the event.
- end End - End date/time of the event.
- hide_start_date boolean? - If true, the event's start date should never be displayed to attendees.
- hide_end_date boolean? - If true, the event's end date should never be displayed to attendees.
- currency string - The ISO 4217 currency code for this event
- online_event boolean? - true = Specifies that the Event is online only (i.e. the Event does not have a Venue).
- organizer_id string? - ID of the event organizer
- logo_id string? - Image ID of the event logo
- venue_id string? - Event venue ID
- format_id string? - Event format
- category_id string? - Event category
- subcategory_id string? - Event subcategory
- listed boolean? - Is this event publicly searchable on Eventbrite?. Default value is true.
- shareable boolean? - Can this event show social sharing buttons?
- invite_only boolean? - Can only people with invites see the event page?
- show_remaining boolean? - If the remaining number of tickets is publicly visible on the event page
- password string? - Password needed to see the event in unlisted mode
- capacity decimal? - Set specific capacity (if omitted, sums ticket capacities)
- is_reserved_seating boolean? - If the event is reserved seating
- is_series boolean? - If the event is part of a series. Specifying this attribute as True during event creation will always designate the event as a series parent, never as a series occurrence. Series occurrences must be created through the schedules API and cannot be created using the events API.
- show_pick_a_seat boolean? - For reserved seating event, if attendees can pick their seats.
- show_seatmap_thumbnail boolean? - For reserved seating event, if venue map thumbnail visible on the event page.
- show_colors_in_seatmap_thumbnail boolean? - For reserved seating event, if venue map thumbnail should have colors on the event page.
- 'source string? - Source of the event (defaults to API)
- locale string? - Indicates event language on Event's listing page. (Default is en_US)
eventbrite: EventSalesStatus
Additional data about the sales status of the event (optional).
Fields
- sales_status string? - Current sales status of the event.
- start_sales_date StartSalesDate? - The earliest start time when a visible ticket is or will be available
- message string? - Custom message associated with the current event sales status.
- message_type string? - Message type
- message_code string? - Message code
eventbrite: EventsByOrganization
An object with a property events which is an array of Event objects.
Fields
- pagination Pagination? - Pagination
- events Event[]? - An array of Event objects.
eventbrite: EventsByVenue
An object with a property events which is an array of Event objects.
Fields
- pagination Pagination? - Pagination
- events Event[]? - An array of Event objects.
eventbrite: ExternalTicketing
External ticketing
Fields
- external_url string - The URL clients can follow to purchase tickets.
- ticketing_provider_name string - The name of the ticketing provider.
- is_free boolean - Whether this is a free event. Mutually exclusive with ticket price range.
- minimum_ticket_price MinimumTicketPrice - A dictionary with some data of the available ticket with the minimums
- maximum_ticket_price MaximumTicketPrice - A dictionary with some data of the available ticket with the maximum
- sales_start string? - When sales start.
- sales_end string? - When sales end.
eventbrite: Fee
The fee that should be included in the price (0 if include_fee is false).
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: FeeComponent
Price costs component that belong to the fee display group.
Fields
- intermediate boolean? - Indicates whether this is a price component that affects the final item price (if False), or just intermediate metadata (if True).
- name string? - Name of the fee.
- internal_name string? - Name of the fee within the group (organizer facing).
- group_name string? - Display name of the fee group (attendee facing).
- value decimal? - The amount of the component represented in minor units. E.g. 725 means 7.25.
- discount DiscountObject? - The total discount to be displayed to a specific cost component and the reason of the discount.
- rule Rule? - Rate rule that applies to this cost component, if any.
- base string? - Name of the base used to calculate the fee.
- bucket string? - The display group the cost component belongs in.
- recipient string? - Who keeps the amount of the cost component.
- payer string? - Who is paying the fee, used to determine if the fee is being passed on or absorbed into the item price.
eventbrite: Format
Full details of the event format (requires the format expansion)
Fields
- id string? - Format ID
- name string? - Format name
- name_localized string? - Localized format name
- short_name string? - Short name for a format
- short_name_localized string? - Localized short name for a format
- resource_uri string? - Is an absolute URL to the API endpoint that will return you the canonical representation of the format.
eventbrite: Gross
The total amount the buyer was charged.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: Instructions
Instructions for the ticket buyer.
Fields
- text string - Instructions for the ticket buyer in text format
- html string - Instructions for the ticket buyer in html format
eventbrite: Logo
Full image details for organizer logo
Fields
- id string - The image’s ID
- url string - The URL of the image
- crop_mask CropMask? - The crop mask applied to the original image
- original Original? - The original image
- aspect_ratio string? - The aspect ratio of the cropped image
- edge_color string? - The edge color of the image in hexadecimal representation
- edge_color_set boolean? - True if the edge color has been set
eventbrite: MaximumTicketPrice
A dictionary with some data of the available ticket with the maximum
Fields
- currency string - The ISO 4217 3-character code of a currency
- value string - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: MinimumTicketPrice
A dictionary with some data of the available ticket with the minimums
Fields
- currency string - The ISO 4217 3-character code of a currency
- value string - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: MusicProperties
This is an object of properties that detail dimensions of music events.
Fields
- age_restriction string? - Minimum age requirement of event attendees.
- presented_by string? - Main music event sponsor.
- door_time string? - Time relative to UTC that the doors are opened to allow people in the day of the event. When not set the event won't have any door time set.
eventbrite: Name
Event name. Value cannot be empty nor whitespace.
Fields
- html string? - Event name in html format. <p>Some text</p>.
eventbrite: OfflineSettings
A container for representing additional offline payment method checkout settings.
Fields
- payment_method string? - Set of possible values are [CASH, CHECK, INVOICE]
- instructions string? - Instructions
eventbrite: Order
The Order object represents an order made against Eventbrite for one or more Ticket Classes. In other words, a single Order can be made up of multiple tickets. The object contains an Order's financial and transactional information; use the Attendee object to return information on Attendees.
Fields
- id string - The Id of the order
- created string? - When the attendee was created (order placed)
- changed string? - When the attendee was last changed
- name string? - The ticket buyer’s name. Use this in preference to first_name/last_name if possible for forward compatibility with non-Western names.
- first_name string? - The ticket buyer’s first name
- last_name string? - The ticket buyer’s last name
- email string? - The ticket buyer’s email address
- costs Costs? - Cost breakdown for this order
- event_id string? - The event id this order is against
- event EventObject? - The Event object represents an Eventbrite Event. An Event is owned by one Organization.
- attendees Attendee[]? - The list of attendees that belong to this order (requires the attendees expansion)
- time_remaining decimal? - The time remaining to complete this order (in seconds)
- resource_uri string? - Is an absolute URL to the API endpoint that will return you the canonical representation of the order.
- status string? - The status of the order
- ticket_buyer_settings TicketBuyerSettings? - Ticket settings relevant to the purchaser after the order has been processed. Included only when called with the expansion ticket_buyer_settings. Order must be in PLACED or UNPAID state for the ticket_buyer_settings to return any information, otherwise will be an empty object.
- contact_list_preferences ContactListPreferences? - Marketing communication preferences for the email address associated with this Order.
eventbrite: OrdersByEvent
An object with a property orders which is an array of Order objects.
Fields
- pagination Pagination? - Pagination
- orders Order[]? - An array of order objects.
eventbrite: OrdersByOrganization
An object with a property orders which is an array of Order objects.
Fields
- pagination Pagination? - Pagination
- orders Order[]? - An array of order objects.
eventbrite: OrdersByUser
An object with a property orders which is an array of Order objects.
Fields
- pagination Pagination? - Pagination
- orders Order[]? - An array of order objects.
eventbrite: Organization
Full details of the organization
Fields
- name string? - The organization name
- vertical string? - The organization vertical
- image_id string? - The organization image id
- id string? - The organization id
eventbrite: Organizations
An object with a property organizations which is an array of Organization objects.
Fields
- pagination Pagination? - Pagination
- organizations Organization[]? - An array of Organization objects.
eventbrite: Organizer
Full details of the event organizer (requires the organizer expansion)
Fields
- name string? - Organizer name.
- description OrganizerDescription? - Description of the Organizer (may be very long and contain significant formatting).
- long_description OrganizerLongDescription? - Long description of the Organizer.
- logo_id string? - Image ID of the organizer logo
- logo Logo? - Full image details for organizer logo
- resource_uri string? - Is an absolute URL to the API endpoint that will return you the organizer.
- id string? - Id of the organizer
- url string? - URL to the organizer's page on Eventbrite
- num_past_events decimal? - Number of past events the organizer has
- num_future_events decimal? - Number of upcoming events the organizer has
- twitter string? - Organizer's twitter handle
- facebook string? - Organizer's facebook page
eventbrite: OrganizerDescription
Description of the Organizer (may be very long and contain significant formatting).
Fields
- text string - Description of the Organizer in text format
- html string - Description of the Organizer in html format
eventbrite: OrganizerLongDescription
Long description of the Organizer.
Fields
- text string - Description of the Organizer in text format
- html string - Description of the Organizer in html format
eventbrite: Original
The original image
Fields
- url string? - The URL of the image
- width decimal? - The width of the image in pixels
- height decimal? - The height of the image in pixels
eventbrite: Pagination
Pagination
Fields
- object_count decimal? - The total number of objects across all pages (optional)
- page_number decimal? - The current page number (starts at 1)
- page_size decimal? - The number of objects on each page (roughly)
- page_count decimal? - The total number of pages (starting at 1) (optional)
- continuation string? - A token to return to the server to get the next set of results (see “Continuated Responses” below)
- has_more_items boolean? - Has more items
eventbrite: PaymentFee
Fees taken by Eventbrite as a payment processing fee.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: PriceBeforeDiscount
The item price before a discount code is applied. If no discount is applied it's the display_price.
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
eventbrite: PublishedEvent
Published event status.
Fields
- published boolean? - Published status
eventbrite: Rule
Rate rule that applies to this cost component, if any.
Fields
- id string? - Fee Rule ID of a specific cost component.
eventbrite: SalesEndRelative
Relative values used to calculate ticket sales_end. Can only be used for series parent tickets.
Fields
- relative_to_event string - Relative to event
- offset decimal - The amount of time in seconds that the ticket sales are offset before the event start or end. Nonnegative number.
eventbrite: ShippingComponent
List of price costs components that belong to the shipping display group.
Fields
- intermediate boolean? - Indicates whether this is a price component that affects the final item price (if False), or just intermediate metadata (if True).
- name string? - Name of the fee.
- internal_name string? - Name of the fee within the group (organizer facing).
- group_name string? - Display name of the fee group (attendee facing).
- value decimal? - The amount of the component represented in minor units. E.g. 725 means 7.25.
- discount DiscountObject? - The total discount to be displayed to a specific cost component and the reason of the discount.
- rule Rule? - Rate rule that applies to this cost component, if any.
- base string? - Name of the base used to calculate the fee.
- bucket string? - The display group the cost component belongs in.
- recipient string? - Who keeps the amount of the cost component.
- payer string? - Who is paying the fee, used to determine if the fee is being passed on or absorbed into the item price.
eventbrite: Start
Start date/time of the event.
Fields
- timezone string - The timezone
- utc string - The time relative to UTC
eventbrite: StartSalesDate
The earliest start time when a visible ticket is or will be available
Fields
- timezone string - The timezone
- utc string - The time relative to UTC
- local string - The time in the timezone of the event
eventbrite: Subcategory
Full details of the event subcategory (requires the subcategory expansion)
Fields
- id string? - Subcategory ID
- resource_uri string? - Resource URI
- name string? - Subcategory name
- parent_category Category? - Full details of the event category (requires the category expansion)
eventbrite: Tax
The ticket's base or discounted tax amount
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: TaxComponent
Price costs component that belong to the tax display group.
Fields
- intermediate boolean? - Indicates whether this is a price component that affects the final item price (if False), or just intermediate metadata (if True).
- name string? - Name of the fee.
- internal_name string? - Name of the fee within the group (organizer facing).
- group_name string? - Display name of the fee group (attendee facing).
- value decimal? - The amount of the component represented in minor units. E.g. 725 means 7.25.
- discount DiscountObject? - The total discount to be displayed to a specific cost component and the reason of the discount.
- rule Rule? - Rate rule that applies to this cost component, if any.
- base string? - Name of the base used to calculate the fee.
- bucket string? - The display group the cost component belongs in.
- recipient string? - Who keeps the amount of the cost component.
- payer string? - Who is paying the fee, used to determine if the fee is being passed on or absorbed into the item price.
eventbrite: Team
Work with the team information of an event
Fields
- id string? - Team ID
- name string? - Team name
- creator Creator? - Creator object
- changed string? - Date when the team information was last changed
- created string? - Date when the team was created
- attendee_count decimal? - How many attendees the team have
- token string? - Token
- event_id string? - The ID of the event this team is part of
- date_joined string? - When the attendee joined the team
eventbrite: Teams
An object with a property teams which is an array of Team objects.
Fields
- pagination Pagination? - Pagination
- venues Team[]? - An array of Team objects.
eventbrite: TicketAvailability
Additional data about general tickets information (optional).
Fields
- has_available_tickets boolean? - Whether this event has tickets available to purchase
- minimum_ticket_price MinimumTicketPrice? - A dictionary with some data of the available ticket with the minimums
- maximum_ticket_price MaximumTicketPrice? - A dictionary with some data of the available ticket with the maximum
- is_sold_out boolean? - Whether there is at least one ticket with availability
- start_sales_date StartSalesDate? - The earliest start time when a visible ticket is or will be available
- waitlist_available boolean? - Waitlist available
eventbrite: TicketBuyerSettings
Ticket settings relevant to the purchaser after the order has been processed. Included only when called with the expansion ticket_buyer_settings. Order must be in PLACED or UNPAID state for the ticket_buyer_settings to return any information, otherwise will be an empty object.
Fields
- confirmation_message ConfirmationMessage? - Order confirmation message from event settings.
- instructions Instructions? - Instructions for the ticket buyer.
- event_id string? - Public id of the event these ticket buyer settings come from.
- refund_request_enabled boolean? - Are refund requests enabled for this event.
- redirect_url string? - URL to redirect the ticket buyer to for further purchase information.
- ticket_class_confirmation_settings TicketClassConfirmationSettings[]? - List of confirmation messages per unique ticket class in the order.
eventbrite: TicketClass
The Ticket Class object represents a possible ticket class (i.e. ticket type) for an Event.
Fields
- description string? - Description of the ticket
- donation boolean? - Is this a donation? (user-supplied cost)
- free boolean? - Is this a free ticket?
- minimum_quantity decimal? - Minimum number per order
- maximum_quantity decimal? - Maximum number per order
- delivery_methods string? - A list of the available delivery methods for this ticket class
- cost string? - Cost of the ticket (currently currency must match event currency) e.g. $45 would be "USD,4500"
- resource_uri string? - Is an absolute URL to the API endpoint that will return you ticket class.
- image_id string? - Image ID for this ticket class. null if no image is set.
- name string? - Name of this ticket type
- display_name string? - Pretty long name of this ticket class. For tiered inventory tickets, this includes the tier name.
- sorting decimal? - Unsigned integer in the order ticket classes are sorted by.
- capacity decimal? - Total available number of this ticket, required for non-donation and non-tiered ticket classes. For normal ticket, null or 0 is not allowed. For donation ticket, null or 0 means unlimited. For tiered inventory ticket, null or 0 means capacity is only limited by tier capacity and/or event capacity.
- quantity_total decimal? - Deprecated, use capacity instead.
- quantity_sold decimal? - The number of sold tickets.
- sales_start string? - When the ticket is available for sale (leave empty for ‘when event published’)
- sales_end string? - When the ticket stops being on sale (leave empty for 'one hour before event start'). Cannot be set on series parent tickets.
- hidden boolean? - Hide this ticket
- include_fee boolean? - Absorb the fee into the displayed cost
- split_fee boolean? - Absorb the payment fee, but show the eventbrite fee
- hide_description boolean? - Hide the ticket description on the event page
- hide_sale_dates boolean? - Hide the sale dates on event landing and ticket selection page
- auto_hide boolean? - Hide this ticket when it is not on sale
- auto_hide_before string? - Override reveal date for auto-hide
- auto_hide_after string? - Override re-hide date for auto-hide
- sales_start_after string? - The ID of another ticket class - when it sells out, this class will go on sale.
- order_confirmation_message string? - Order message per ticket type
- sales_channels string[]? - A list of all supported sales channels
- inventory_tier_id string? - Optional ID of Inventory Tier with which to associate the ticket class
- secondary_assignment_enabled boolean? - Has secondary barcode assignment enabled (for ex/ RFID)
- sales_end_relative SalesEndRelative? - Relative values used to calculate ticket sales_end. Can only be used for series parent tickets.
- has_pdf_ticket boolean? - Whether to include pdf ticket or not
eventbrite: TicketClassConfirmationSettings
List of confirmation messages per unique ticket class in the order.
Fields
- ticket_class_id string? - ID of the ticket class the confirmation settings apply to.
- event_id string? - ID of the event this ticket class belongs to.
- confirmation_message TicketClassConfirmationSettingsConfirmationMessage? - Confirmation message for customers who have purchased one or more of this ticket class.
eventbrite: TicketClassConfirmationSettingsConfirmationMessage
Confirmation message for customers who have purchased one or more of this ticket class.
Fields
- text string - Description of the confirmation message for customers who have purchased one or more of this ticket class in text format
- html string - Description of the confirmation message for customers who have purchased one or more of this ticket class in html format
eventbrite: TicketCost
The display cost for the ticket
Fields
- currency string - The ISO 4217 3-character code of a currency
- value decimal - The integer value of units of the minor unit of the currency (e.g. cents for US dollars)
- major_value string - You can get a value in the currency's major unit - for example, dollars or pound sterling - by taking the integer value provided and shifting the decimal point left by the exponent value for that currency as defined in ISO 4217
- display string - Provided for your convenience; its formatting may change depending on the locale you query the API with (for example, commas for decimal separators in European locales).
eventbrite: TopLeft
Coordinate for top-left corner of crop mask
Fields
- y decimal? - Y coordinate for top-left corner of crop mask
- x decimal? - X coordinate for top-left corner of crop mask
eventbrite: User
User is an object representing an Eventbrite account. Users are Members of an Organization.
Fields
- name string? - Full name. Use this in preference to first_name/last_name if possible for forward compatibility with non-Western names.
- first_name string? - First name
- last_name string? - Last name
- is_public boolean? - Is this user's profile public?
- image_id string? - The organization image id
- emails Email[]? - List of User Email objects
eventbrite: UserObject
An object with a single property user which must be a User object.
Fields
- user User? - User is an object representing an Eventbrite account. Users are Members of an Organization.
eventbrite: Venue
Full venue details for venue_id (requires the venue expansion)
Fields
- name string - Venue name
- age_restriction string? - Age restriction of the venue
- capacity decimal? - Venue capacity
- address Address? - The address of the venue
- resource_uri string? - Resource uri
- id string? - Venue ID
- latitude string? - Latitude portion of the address coordinates of the venue
- longitude string? - Longitude portion of the address coordinates of the venue
eventbrite: VenueRequest
The Venue object represents the location of an Event (i.e. where an Event takes place). Venues are grouped together by the Organization object.
Fields
- name string - The name of the venue
- age_restriction string? - The age restrictions for the venue
- capacity decimal? - Set specific capacity (if omitted, sums ticket capacities)
- google_place_id string? - The google place id for the venue
- organizer_id string? - The organizer this venue belongs to (optional - leave this off to use the default organizer)
- address Address? - The address of the venue
eventbrite: Venues
An object with a property venues which is an array of Venue objects.
Fields
- pagination Pagination? - Pagination
- venues Venue[]? - An array of venue objects.
Import
import ballerinax/eventbrite;
Metadata
Released date: about 2 years ago
Version: 1.6.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1822
Current verison: 24
Weekly downloads
Keywords
Marketing/Event Management
Cost/Freemium
Contributors
Dependencies