elasticsearch
Module elasticsearch
API
Definitions
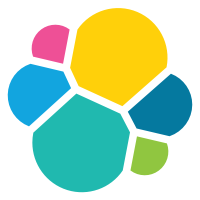
ballerinax/elasticsearch Ballerina library
Overview
This is a generated connector for Elasticsearch OpenAPI specification.
Elasticsearch API allows you to store, search, and analyze data with ease at scale.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Elasticsearch Account.
- Obtain tokens by following this guide.
Quickstart
To use the Elasticsearch in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/elasticsearch
module into the Ballerina project.
import ballerinax/elasticsearch;
Step 2: Create a new connector instance
Create a elasticsearch:ApiKeysConfig
with the authorization
key obtained, and initialize the connector with it.
elasticsearch:ApiKeysConfig apiKeysConfig = { authorization : "<AUTHORIZATION_TOKEN>" }; elasticsearch:Client baseClient = check new (apiKeysConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to obtain information about existing Elastic Stack versions, using the connector.
Get Elastic Stack versions.
public function main() returns error? { elasticsearch:StackVersionConfigs response = check baseClient->getVersionStacks(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
elasticsearch: Client
This is a generated connector for Elastic Cloud API OpenAPI Specification.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Please create a Elastic account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.elastic-cloud.com/api/v1/" - URL of the target service
shutdownDeploymentEsResource
function shutdownDeploymentEsResource(string deploymentId, string refId, boolean? hide, boolean skipSnapshot) returns DeploymentResourceCommandResponse|error
Shutdown Deployment Elasticsearch Resource
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
- hide boolean? (default ()) - Hide cluster on shutdown. Hidden clusters are not listed by default. Only applicable for Platform administrators.
- skipSnapshot boolean (default false) - If true, will skip taking a snapshot of the cluster before shutting the cluster down (if even possible).
Return Type
- DeploymentResourceCommandResponse|error - Standard response.
startDeploymentResourceInstancesAll
function startDeploymentResourceInstancesAll(string deploymentId, string resourceKind, string refId) returns DeploymentResourceCommandResponse|error
Start all instances
Parameters
- deploymentId string - Identifier for the Deployment.
- resourceKind string - The kind of resource (one of elasticsearch, kibana, apm, or integrations_server).
- refId string - User-specified RefId for the Resource.
Return Type
- DeploymentResourceCommandResponse|error - The start command was issued successfully.
getVersionStacks
function getVersionStacks(boolean showDeleted, boolean showUnusable) returns StackVersionConfigs|error
Get stack versions
Parameters
- showDeleted boolean (default false) - Whether to show deleted stack versions or not
- showUnusable boolean (default false) - Whether to show versions that are unusable by the authenticated user
Return Type
- StackVersionConfigs|error - The list of all available Elastic Stack versions are retrieved, including the template version and structure.
restoreDeploymentResource
function restoreDeploymentResource(string deploymentId, string resourceKind, string refId, boolean restoreSnapshot) returns DeploymentResourceCrudResponse|error
Restores a shutdown resource
Parameters
- deploymentId string - Identifier for the Deployment
- resourceKind string - The kind of resource
- refId string - User-specified RefId for the Resource
- restoreSnapshot boolean (default false) - Whether or not to restore a snapshot for those resources that allow it.
Return Type
- DeploymentResourceCrudResponse|error - Standard Deployment Resource Crud Response
restoreDeployment
function restoreDeployment(string deploymentId, boolean restoreSnapshot) returns DeploymentRestoreResponse|error
Restores a shutdown Deployment
Parameters
- deploymentId string - Identifier for the Deployment
- restoreSnapshot boolean (default false) - Whether or not to restore a snapshot for those resources that allow it.
Return Type
- DeploymentRestoreResponse|error - The request was valid and the resources of the deployment were restored.
getDeploymentTemplatesV2
function getDeploymentTemplatesV2(string region, string? metadata, boolean showInstanceConfigurations, boolean showMaxZones, string? stackVersion) returns DeploymentTemplateInfoV2[]|error
Get deployment templates
Parameters
- region string - Region of the deployment templates
- metadata string? (default ()) - An optional key/value pair in the form of (key:value) that will act as a filter and exclude any templates that do not have a matching metadata item associated.
- showInstanceConfigurations boolean (default true) - If true, will return details for each instance configuration referenced by the template.
- showMaxZones boolean (default false) - If true, will populate the max_zones field in the instance configurations. Only relevant if show_instance_configurations=true.
- stackVersion string? (default ()) - If present, it will cause the returned deployment templates to be adapted to return only the elements allowed in that version.
Return Type
- DeploymentTemplateInfoV2[]|error - The deployment templates were returned successfully.
getApiKey
function getApiKey(string apiKeyId) returns ApiKeyResponse|error
Get API key
Parameters
- apiKeyId string - The API Key ID.
Return Type
- ApiKeyResponse|error - The API key metadata is retrieved.
deleteApiKey
function deleteApiKey(string apiKeyId) returns EmptyResponse|error
Delete API key
Parameters
- apiKeyId string - The API Key ID.
Return Type
- EmptyResponse|error - The API key is deleted.
getCurrentAccount
function getCurrentAccount() returns AccountResponse|error
Fetch current account information
Return Type
- AccountResponse|error - Account fetched successfully
updateCurrentAccount
function updateCurrentAccount(AccountUpdateRequest payload) returns AccountResponse|error
Updates the current account
Parameters
- payload AccountUpdateRequest - the current account
Return Type
- AccountResponse|error - Account updated successfully
patchCurrentAccount
function patchCurrentAccount(string payload) returns AccountResponse|error
Updates the current account
Parameters
- payload string - All changes in the specified object are applied to the current account according to the JSON Merge Patch processing rules. Omitting existing fields causes the same values to be reapplied. Specifying a
null
value reverts the field to the default value, or removes the field when no default value exists.
Return Type
- AccountResponse|error - Account updated successfully
getDeploymentEsResourceRemoteClusters
function getDeploymentEsResourceRemoteClusters(string deploymentId, string refId) returns RemoteResources|error
Get remote clusters
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
Return Type
- RemoteResources|error - List of remote clusters for the resource
setDeploymentEsResourceRemoteClusters
function setDeploymentEsResourceRemoteClusters(string deploymentId, string refId, RemoteResources payload) returns EmptyResponse|error
Set remote clusters
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
- payload RemoteResources - List of remote clusters for the resource
Return Type
- EmptyResponse|error - The Remote Clusters were updated
getDeploymentTemplateV2
function getDeploymentTemplateV2(string templateId, string region, boolean showInstanceConfigurations, boolean showMaxZones, string? stackVersion) returns DeploymentTemplateInfoV2|error
Get deployment template
Parameters
- templateId string - The identifier for the deployment template.
- region string - Region of the deployment template
- showInstanceConfigurations boolean (default true) - If true, will return details for each instance configuration referenced by the template.
- showMaxZones boolean (default false) - If true, will populate the max_zones field in the instance configurations. Only relevant if show_instance_configurations=true.
- stackVersion string? (default ()) - If present, it will cause the returned deployment template to be adapted to return only the elements allowed in that version.
Return Type
- DeploymentTemplateInfoV2|error - The deployment template was found and returned successfully.
deploymentApmResetSecretToken
function deploymentApmResetSecretToken(string deploymentId, string refId) returns ApmCrudResponse|error
Reset the secret token for an APM resource.
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
Return Type
- ApmCrudResponse|error - Response containing the new secret token, plan to apply it starts
getDeploymentEnterpriseSearchResourceInfo
function getDeploymentEnterpriseSearchResourceInfo(string deploymentId, string refId, boolean showMetadata, boolean showPlans, boolean showPlanLogs, boolean showPlanHistory, boolean showPlanDefaults, boolean showSettings) returns EnterpriseSearchResourceInfo|error
Get Deployment Enterprise Search Resource Info
Parameters
- deploymentId string - Identifier for the Deployment
- refId string - User-specified RefId for the Resource
- showMetadata boolean (default false) - Whether to include the full cluster metadata in the response - can be large per cluster and also include credentials.
- showPlans boolean (default true) - Whether to include the full current and pending plan information in the response - can be large per cluster.
- showPlanLogs boolean (default false) - Whether to include with the current and pending plan information the attempt log - can be very large per cluster.
- showPlanHistory boolean (default false) - Whether to include with the current and pending plan information the plan history- can be very large per cluster.
- showPlanDefaults boolean (default false) - If showing plans, whether to show values that are left at their default value (less readable but more informative).
- showSettings boolean (default false) - Whether to show cluster settings in the response.
Return Type
- EnterpriseSearchResourceInfo|error - Standard response.
getDeploymentKibResourceInfo
function getDeploymentKibResourceInfo(string deploymentId, string refId, boolean showMetadata, boolean showPlans, boolean showPlanLogs, boolean showPlanHistory, boolean showPlanDefaults, boolean convertLegacyPlans, boolean showSettings) returns KibanaResourceInfo|error
Get Deployment Kibana Resource Info
Parameters
- deploymentId string - Identifier for the Deployment
- refId string - User-specified RefId for the Resource
- showMetadata boolean (default false) - Whether to include the full cluster metadata in the response - can be large per cluster and also include credentials.
- showPlans boolean (default true) - Whether to include the full current and pending plan information in the response - can be large per cluster.
- showPlanLogs boolean (default false) - Whether to include with the current and pending plan information the attempt log - can be very large per cluster.
- showPlanHistory boolean (default false) - Whether to include with the current and pending plan information the plan history- can be very large per cluster.
- showPlanDefaults boolean (default false) - If showing plans, whether to show values that are left at their default value (less readable but more informative).
- convertLegacyPlans boolean (default false) - If showing plans, whether to leave pre-2.0.0 plans in their legacy format (the default), or whether to update them to 2.0.x+ format (if 'true').
- showSettings boolean (default false) - Whether to show cluster settings in the response.
Return Type
- KibanaResourceInfo|error - Standard response.
getAppsearchReadOnlyMode
function getAppsearchReadOnlyMode(string deploymentId, string refId) returns ReadOnlyResponse|error
Set AppSearch read-only status
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
Return Type
- ReadOnlyResponse|error - Standard response
setAppsearchReadOnlyMode
function setAppsearchReadOnlyMode(string deploymentId, string refId, ReadOnlyRequest payload) returns ReadOnlyResponse|error
Set AppSearch read-only status
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
- payload ReadOnlyRequest - read-only request body
Return Type
- ReadOnlyResponse|error - Standard response
restartDeploymentStatelessResource
function restartDeploymentStatelessResource(string deploymentId, string statelessResourceKind, string refId, boolean cancelPending) returns DeploymentResourceCommandResponse|error
Restart Deployment Stateless Resource
Parameters
- deploymentId string - Identifier for the Deployment.
- statelessResourceKind string - The kind of stateless resource
- refId string - User-specified RefId for the Resource.
- cancelPending boolean (default false) - If true, cancels any pending plans before restarting. If false and there are pending plans, returns an error.
Return Type
- DeploymentResourceCommandResponse|error - The restart command was issued successfully
shutdownDeployment
function shutdownDeployment(string deploymentId, boolean? hide, boolean skipSnapshot) returns DeploymentShutdownResponse|error
Shuts down Deployment
Parameters
- deploymentId string - Identifier for the Deployment
- hide boolean? (default ()) - Whether or not to hide the deployment and its resources.Only applicable for Platform administrators.
- skipSnapshot boolean (default false) - Whether or not to skip snapshots before shutting down the resources
Return Type
- DeploymentShutdownResponse|error - The request was valid and the resources of the deployment were shutdown.
enableDeploymentResourceSlm
function enableDeploymentResourceSlm(string deploymentId, string refId, boolean validateOnly) returns DeploymentResourceCommandResponse|error
Migrate Elasticsearch resource to use SLM
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
- validateOnly boolean (default false) - When
true
, does not enable SLM but returns warnings if any applications may lose availability during SLM migration.
Return Type
- DeploymentResourceCommandResponse|error - Standard response
deleteTrafficFilterRulesetAssociation
function deleteTrafficFilterRulesetAssociation(string rulesetId, string associationType, string associatedEntityId) returns EmptyResponse|error
Delete ruleset association
Parameters
- rulesetId string - The mandatory ruleset ID.
- associationType string - Association type
- associatedEntityId string - Associated entity ID
Return Type
- EmptyResponse|error - Delete association request was valid and the association has been deleted
restartDeploymentEsResource
function restartDeploymentEsResource(string deploymentId, string refId, boolean restoreSnapshot, boolean skipSnapshot, boolean cancelPending, string groupAttribute, int shardInitWaitTime) returns DeploymentResourceCommandResponse|error
Restart Deployment Elasticsearch Resource
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
- restoreSnapshot boolean (default true) - When set to true and restoring from shutdown, then will restore the cluster from the last snapshot (if available).
- skipSnapshot boolean (default true) - If true, will not take a snapshot of the cluster before restarting.
- cancelPending boolean (default false) - If true, cancels any pending plans before restarting. If false and there are pending plans, returns an error.
- groupAttribute string (default "__zone__") - Indicates the property or properties used to divide the list of instances to restart in groups. Valid options are: '__all__' (restart all at once), '__zone__' by logical zone, '__name__' one instance at a time, or a comma-separated list of attributes of the instances
- shardInitWaitTime int (default 600) - The time, in seconds, to wait for shards that show no progress of initializing, before rolling the next group (default: 10 minutes)
Return Type
- DeploymentResourceCommandResponse|error - The restart command was issued successfully.
getCostsOverview
function getCostsOverview(string organizationId, string? 'from, string? to) returns CostsOverview|error
Get costs overview for the organization
Parameters
- organizationId string - Identifier for the organization
- 'from string? (default ()) - A datetime for the beginning of the desired range for which to fetch costs. Defaults to start of current month.
- to string? (default ()) - A datetime for the end of the desired range for which to fetch costs. Defaults to the current date.
Return Type
- CostsOverview|error - Top-level cost overview for the organization
startDeploymentResourceInstancesAllMaintenanceMode
function startDeploymentResourceInstancesAllMaintenanceMode(string deploymentId, string resourceKind, string refId) returns DeploymentResourceCommandResponse|error
Start maintenance mode (all instances)
Parameters
- deploymentId string - Identifier for the Deployment.
- resourceKind string - The kind of resource (one of elasticsearch, kibana, apm, or integrations_server).
- refId string - User-specified RefId for the Resource.
Return Type
- DeploymentResourceCommandResponse|error - The start maintenance command was issued successfully.
getCostsDeployments
function getCostsDeployments(string organizationId, string? 'from, string? to) returns DeploymentsCosts|error
Get deployments costs for the organization
Parameters
- organizationId string - Identifier for the organization
- 'from string? (default ()) - A datetime for the beginning of the desired range for which to fetch activity. Defaults to start of current month.
- to string? (default ()) - A datetime for the end of the desired range for which to fetch activity. Defaults to the current date.
Return Type
- DeploymentsCosts|error - The costs associated to a set of products
listExtensions
function listExtensions(boolean includeDeployments) returns Extensions|error
List Extensions
Parameters
- includeDeployments boolean (default false) - Include deployments referencing this extension. DEPRECATED: To get the list of deployments that reference an extension, use the Get Extension API.
Return Type
- Extensions|error - The extensions that are available
createExtension
function createExtension(CreateExtensionRequest payload) returns Extension|error
Create an extension
Parameters
- payload CreateExtensionRequest - the data that creates the extension
getCostsItems
function getCostsItems(string organizationId, string? 'from, string? to) returns ItemsCosts|error
Get itemized costs for the organization
Parameters
- organizationId string - Identifier for the organization
- 'from string? (default ()) - A datetime for the beginning of the desired range for which to fetch costs. Defaults to start of current month.
- to string? (default ()) - A datetime for the end of the desired range for which to fetch costs. Defaults to the current date.
Return Type
- ItemsCosts|error - The costs associated to a set of items
getTrafficFilterRulesetDeploymentAssociations
function getTrafficFilterRulesetDeploymentAssociations(string rulesetId) returns RulesetAssociations|error
Get associated deployments
Parameters
- rulesetId string - The mandatory ruleset ID.
Return Type
- RulesetAssociations|error - Associations referred by traffic filter rulesets were successfully returned
createTrafficFilterRulesetAssociation
function createTrafficFilterRulesetAssociation(string rulesetId, FilterAssociation payload) returns EmptyResponse|error
Create ruleset association
Parameters
- rulesetId string - The mandatory ruleset ID.
- payload FilterAssociation - Mandatory ruleset association description
Return Type
- EmptyResponse|error - Create association request was valid and the association already exists
resetElasticsearchUserPassword
function resetElasticsearchUserPassword(string deploymentId, string refId) returns ElasticsearchElasticUserPasswordResetResponse|error
Reset 'elastic' user password
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
Return Type
- ElasticsearchElasticUserPasswordResetResponse|error - The password reset was out carried successfully
getDeploymentUpgradeAssistantStatus
function getDeploymentUpgradeAssistantStatus(string deploymentId) returns DeploymentUpgradeAssistantStatusResponse|error
Get Deployment upgade assistant status
Parameters
- deploymentId string - Identifier for the Deployment
Return Type
- DeploymentUpgradeAssistantStatusResponse|error - The Upgrade Assistant returned the status successfully
stopDeploymentResourceInstancesAll
function stopDeploymentResourceInstancesAll(string deploymentId, string resourceKind, string refId) returns DeploymentResourceCommandResponse|error
Stop all instances
Parameters
- deploymentId string - Identifier for the Deployment.
- resourceKind string - The kind of resource (one of elasticsearch, kibana, apm, or integrations_server).
- refId string - User-specified RefId for the Resource.
Return Type
- DeploymentResourceCommandResponse|error - The stop command was issued successfully.
getApiKeys
function getApiKeys() returns ApiKeysResponse|error
Get all API keys
Return Type
- ApiKeysResponse|error - The metadata for the API keys is retrieved.
createApiKey
function createApiKey(CreateApiKeyRequest payload) returns ApiKeyResponse|error
Create API key
Parameters
- payload CreateApiKeyRequest - The request to create the API key
Return Type
- ApiKeyResponse|error - The API key is created and returned in the body of the response.
enableDeploymentResourceCcr
function enableDeploymentResourceCcr(string deploymentId, string refId, boolean validateOnly) returns DeploymentResourceCommandResponse|error
Migrate Elasticsearch and associated Kibana resources to enable CCR
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
- validateOnly boolean (default false) - When
true
, will not enable CCR but returns warnings if any elements may lose availability during CCR enablement
Return Type
- DeploymentResourceCommandResponse|error - Standard response
getDeploymentEsResourceKeystore
function getDeploymentEsResourceKeystore(string deploymentId, string refId) returns KeystoreContents|error
Get the items in the Elasticsearch resource keystore
Parameters
- deploymentId string - Identifier for the Deployment
- refId string - User-specified RefId for the Resource
Return Type
- KeystoreContents|error - The contents of the Elasticsearch keystore
setDeploymentEsResourceKeystore
function setDeploymentEsResourceKeystore(string deploymentId, string refId, KeystoreContents payload) returns KeystoreContents|error
Add or remove items from the Elasticsearch resource keystore
Parameters
- deploymentId string - Identifier for the Deployment
- refId string - User-specified RefId for the Resource
- payload KeystoreContents - The new settings that will be applied to the keystore of the Elasticsearch resource.
Return Type
- KeystoreContents|error - The new contents of the Elasticsearch keystore
getTrafficFilterRulesets
function getTrafficFilterRulesets(boolean includeAssociations, string? region) returns TrafficFilterRulesets|error
List traffic filter rulesets
Parameters
- includeAssociations boolean (default false) - Retrieves a list of resources that are associated to the specified ruleset.
- region string? (default ()) - If provided limits the rulesets to that region only.
Return Type
- TrafficFilterRulesets|error - The collection of traffic filter routes
createTrafficFilterRuleset
function createTrafficFilterRuleset(TrafficFilterRulesetRequest payload) returns TrafficFilterRulesetResponse|error
Create a ruleset
Parameters
- payload TrafficFilterRulesetRequest - The specification for traffic filter ruleset.
Return Type
- TrafficFilterRulesetResponse|error - The ruleset definition is valid and the creation has started.
searchDeployments
function searchDeployments(SearchRequest payload) returns DeploymentsSearchResponse|error
Search Deployments
Parameters
- payload SearchRequest - (Optional) The search query to run. When not specified, all of the deployments are matched.
Return Type
- DeploymentsSearchResponse|error - The list of deployments that match the specified query and belong to the authenticated user.
getCostsItemsByDeployment
function getCostsItemsByDeployment(string organizationId, string deploymentId, string? 'from, string? to) returns ItemsCosts|error
Get itemized costs by deployments
Parameters
- organizationId string - Identifier for the organization
- deploymentId string - Id of a Deployment
- 'from string? (default ()) - A datetime for the beginning of the desired range for which to fetch costs. Defaults to start of current month.
- to string? (default ()) - A datetime for the end of the desired range for which to fetch costs. Defaults to the current date.
Return Type
- ItemsCosts|error - The costs associated to a set items billed for a single deployment.
getDeploymentEsResourceEligibleRemoteClusters
function getDeploymentEsResourceEligibleRemoteClusters(string deploymentId, string refId, SearchRequest payload) returns DeploymentsSearchResponse|error
Get eligible remote clusters
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
- payload SearchRequest - (Optional) The search query to run against all deployments containing eligible remote clusters. When not specified, all the eligible deployments are matched.
Return Type
- DeploymentsSearchResponse|error - List of deployments which contains eligible remote clusters for the resource
getDeployment
function getDeployment(string deploymentId, boolean showSecurity, boolean showMetadata, boolean showPlans, boolean showPlanLogs, boolean showPlanHistory, boolean showPlanDefaults, boolean convertLegacyPlans, int showSystemAlerts, boolean showSettings, boolean enrichWithTemplate) returns DeploymentGetResponse|error
Get Deployment
Parameters
- deploymentId string - Identifier for the Deployment
- showSecurity boolean (default false) - Whether to include the Elasticsearch 2.x security information in the response - can be large per cluster and also include credentials
- showMetadata boolean (default false) - Whether to include the full cluster metadata in the response - can be large per cluster and also include credentials
- showPlans boolean (default true) - Whether to include the full current and pending plan information in the response - can be large per cluster
- showPlanLogs boolean (default false) - Whether to include with the current and pending plan information the attempt log - can be very large per cluster
- showPlanHistory boolean (default false) - Whether to include with the current and pending plan information the plan history- can be very large per cluster
- showPlanDefaults boolean (default false) - If showing plans, whether to show values that are left at their default value (less readable but more informative)
- convertLegacyPlans boolean (default false) - If showing plans, whether to leave pre-2.0.0 plans in their legacy format (the default), or whether to update them to 2.0.x+ format (if 'true')
- showSystemAlerts int (default 0) - Number of system alerts (such as forced restarts due to memory limits) to be included in the response - can be large per cluster. Negative numbers or 0 will not return field.
- showSettings boolean (default false) - Whether to show cluster settings in the response.
- enrichWithTemplate boolean (default true) - If showing plans, whether to enrich the plan by including the missing elements from the deployment template it is based on
Return Type
- DeploymentGetResponse|error - The Deployment info response
updateDeployment
function updateDeployment(string deploymentId, DeploymentUpdateRequest payload, boolean hidePrunedOrphans, boolean skipSnapshot, boolean validateOnly, string? 'version) returns DeploymentUpdateResponse|error
Update Deployment
Parameters
- deploymentId string - Identifier for the Deployment
- payload DeploymentUpdateRequest - The deployment definition
- hidePrunedOrphans boolean (default false) - Whether or not to hide orphaned resources that were shut down (relevant if prune on the request is true)
- skipSnapshot boolean (default false) - Whether or not to skip snapshots before shutting down orphaned resources (relevant if prune on the request is true)
- validateOnly boolean (default false) - If true, will just validate the Deployment definition but will not perform the update
- 'version string? (default ()) - If specified then checks for conflicts against the version stored in the persistent store (returned in 'x-cloud-resource-version' of the GET request)
Return Type
- DeploymentUpdateResponse|error - The request was valid and the deployment was updated.
getDeploymentEsResourceInfo
function getDeploymentEsResourceInfo(string deploymentId, string refId, boolean showSecurity, boolean showMetadata, boolean showPlans, boolean showPlanLogs, boolean showPlanHistory, boolean showPlanDefaults, boolean convertLegacyPlans, int showSystemAlerts, boolean showSettings, boolean enrichWithTemplate) returns ElasticsearchResourceInfo|error
Get Deployment Elasticsearch Resource Info
Parameters
- deploymentId string - Identifier for the Deployment
- refId string - User-specified RefId for the Resource
- showSecurity boolean (default false) - Whether to include the Elasticsearch 2.x security information in the response - can be large per cluster and also include credentials.
- showMetadata boolean (default false) - Whether to include the full cluster metadata in the response - can be large per cluster and also include credentials.
- showPlans boolean (default true) - Whether to include the full current and pending plan information in the response - can be large per cluster.
- showPlanLogs boolean (default false) - Whether to include with the current and pending plan information the attempt log - can be very large per cluster.
- showPlanHistory boolean (default false) - Whether to include with the current and pending plan information the plan history- can be very large per cluster.
- showPlanDefaults boolean (default false) - If showing plans, whether to show values that are left at their default value (less readable but more informative).
- convertLegacyPlans boolean (default false) - If showing plans, whether to leave pre-2.0.0 plans in their legacy format (the default), or whether to update them to 2.0.x+ format (if 'true').
- showSystemAlerts int (default 0) - Number of system alerts (such as forced restarts due to memory limits) to be included in the response - can be large per cluster. Negative numbers or 0 will not return field.
- showSettings boolean (default false) - Whether to show cluster settings in the response.
- enrichWithTemplate boolean (default true) - If showing plans, whether to enrich the plan by including the missing elements from the deployment template it is based on.
Return Type
- ElasticsearchResourceInfo|error - Standard response.
stopDeploymentResourceInstancesAllMaintenanceMode
function stopDeploymentResourceInstancesAllMaintenanceMode(string deploymentId, string resourceKind, string refId) returns DeploymentResourceCommandResponse|error
Stop maintenance mode (all instances)
Parameters
- deploymentId string - Identifier for the Deployment.
- resourceKind string - The kind of resource (one of elasticsearch, kibana, apm, or integrations_server).
- refId string - User-specified RefId for the Resource.
Return Type
- DeploymentResourceCommandResponse|error - The stop maintenance mode command was issued successfully.
enableDeploymentResourceIlm
function enableDeploymentResourceIlm(string deploymentId, string refId, EnableIlmRequest payload, boolean validateOnly) returns DeploymentResourceCommandResponse|error
Migrate Elasticsearch resource to use ILM
Parameters
- deploymentId string - Identifier for the Deployment.
- refId string - User-specified RefId for the Resource.
- payload EnableIlmRequest - Information to build the ILM policies that will be created
- validateOnly boolean (default false) - When
true
, does not enable ILM but returns warnings if any applications may lose availability during ILM migration.
Return Type
- DeploymentResourceCommandResponse|error - Standard response
getExtension
Get Extension
Parameters
- extensionId string - Id of an extension
- includeDeployments boolean (default false) - Include deployments referencing this extension. Up to only 10000 deployments will be included.
uploadExtension
function uploadExtension(string extensionId, ExtensionsExtensionIdBody payload) returns Extension|error
Uploads the Extension
updateExtension
function updateExtension(string extensionId, UpdateExtensionRequest payload) returns Extension|error
Update Extension
Parameters
- extensionId string - Id of an extension
- payload UpdateExtensionRequest - The extension update data.
deleteExtension
function deleteExtension(string extensionId) returns EmptyResponse|error
Delete Extension
Parameters
- extensionId string - Id of an extension
Return Type
- EmptyResponse|error - Extension deleted successfully.
shutdownDeploymentStatelessResource
function shutdownDeploymentStatelessResource(string deploymentId, string statelessResourceKind, string refId, boolean? hide, boolean skipSnapshot) returns DeploymentResourceCommandResponse|error
Shutdown Deployment Stateless Resource
Parameters
- deploymentId string - Identifier for the Deployment.
- statelessResourceKind string - The kind of stateless resource
- refId string - User-specified RefId for the Resource.
- hide boolean? (default ()) - Hide cluster on shutdown. Hidden clusters are not listed by default. Only applicable for Platform administrators.
- skipSnapshot boolean (default false) - If true, will skip taking a snapshot of the cluster before shutting the cluster down (if even possible)
Return Type
- DeploymentResourceCommandResponse|error - Standard response
getDeploymentApmResourceInfo
function getDeploymentApmResourceInfo(string deploymentId, string refId, boolean showMetadata, boolean showPlans, boolean showPlanLogs, boolean showPlanHistory, boolean showPlanDefaults, boolean showSettings) returns ApmResourceInfo|error
Get Deployment APM Resource Info
Parameters
- deploymentId string - Identifier for the Deployment
- refId string - User-specified RefId for the Resource
- showMetadata boolean (default false) - Whether to include the full cluster metadata in the response - can be large per cluster and also include credentials.
- showPlans boolean (default true) - Whether to include the full current and pending plan information in the response - can be large per cluster.
- showPlanLogs boolean (default false) - Whether to include with the current and pending plan information the attempt log - can be very large per cluster.
- showPlanHistory boolean (default false) - Whether to include with the current and pending plan information the plan history- can be very large per cluster.
- showPlanDefaults boolean (default false) - If showing plans, whether to show values that are left at their default value (less readable but more informative).
- showSettings boolean (default false) - Whether to show cluster settings in the response.
Return Type
- ApmResourceInfo|error - Standard response.
cancelDeploymentResourcePendingPlan
function cancelDeploymentResourcePendingPlan(string deploymentId, string resourceKind, string refId, boolean forceDelete, boolean ignoreMissing) returns DeploymentResourceCrudResponse|error
Cancel resource pending plan
Parameters
- deploymentId string - Identifier for the Deployment
- resourceKind string - The kind of resource
- refId string - User-specified RefId for the Resource
- forceDelete boolean (default false) - When
true
, deletes the pending plan instead of attempting a graceful cancellation. The default isfalse
.
- ignoreMissing boolean (default false) - When
true
, returns successfully, even when plans are missing. The default isfalse
.
Return Type
- DeploymentResourceCrudResponse|error - Standard Deployment Resource Crud Response
getTrafficFilterDeploymentRulesetAssociations
function getTrafficFilterDeploymentRulesetAssociations(string associationType, string associatedEntityId) returns TrafficFilterSettings|error
Get associated rulesets
Return Type
- TrafficFilterSettings|error - Rulesets in the deployment were successfully returned
getDeploymentAppsearchResourceInfo
function getDeploymentAppsearchResourceInfo(string deploymentId, string refId, boolean showMetadata, boolean showPlans, boolean showPlanLogs, boolean showPlanHistory, boolean showPlanDefaults, boolean showSettings) returns AppSearchResourceInfo|error
Get Deployment App Search Resource Info
Parameters
- deploymentId string - Identifier for the Deployment
- refId string - User-specified RefId for the Resource
- showMetadata boolean (default false) - Whether to include the full cluster metadata in the response - can be large per cluster and also include credentials.
- showPlans boolean (default true) - Whether to include the full current and pending plan information in the response - can be large per cluster.
- showPlanLogs boolean (default false) - Whether to include with the current and pending plan information the attempt log - can be very large per cluster.
- showPlanHistory boolean (default false) - Whether to include with the current and pending plan information the plan history- can be very large per cluster.
- showPlanDefaults boolean (default false) - If showing plans, whether to show values that are left at their default value (less readable but more informative).
- showSettings boolean (default false) - Whether to show cluster settings in the response.
Return Type
- AppSearchResourceInfo|error - Standard response.
searchEligibleRemoteClusters
function searchEligibleRemoteClusters(string 'version, SearchRequest payload) returns DeploymentsSearchResponse|error
Get eligible remote clusters
Parameters
- 'version string - The version of the Elasticsearch cluster cluster that will potentially be configured to have remote clusters.
- payload SearchRequest - (Optional) The search query to run against all deployments containing eligible remote clusters. When not specified, all the eligible deployments are matched.
Return Type
- DeploymentsSearchResponse|error - List of deployments which contains eligible remote clusters for a specific version
getTrafficFilterRuleset
function getTrafficFilterRuleset(string rulesetId, boolean includeAssociations) returns TrafficFilterRulesetInfo|error
Retrieves the ruleset by ID.
Parameters
- rulesetId string - The mandatory ruleset ID.
- includeAssociations boolean (default false) - Retrieves a list of resources that are associated to the specified ruleset.
Return Type
- TrafficFilterRulesetInfo|error - The container for a set of traffic filter rules.
updateTrafficFilterRuleset
function updateTrafficFilterRuleset(string rulesetId, TrafficFilterRulesetRequest payload) returns TrafficFilterRulesetResponse|error
Updates a ruleset
Parameters
- rulesetId string - The mandatory ruleset ID.
- payload TrafficFilterRulesetRequest - The specification for traffic filter ruleset.
Return Type
- TrafficFilterRulesetResponse|error - The ruleset definition was valid and the update has started.
deleteTrafficFilterRuleset
function deleteTrafficFilterRuleset(string rulesetId, boolean ignoreAssociations) returns EmptyResponse|error
Delete a ruleset
Parameters
- rulesetId string - The mandatory ruleset ID.
- ignoreAssociations boolean (default false) - When true, ignores the associations and deletes the ruleset. When false, recognizes the associations, which prevents the deletion of the rule set.
Return Type
- EmptyResponse|error - The traffic filter ruleset was successfully deleted.
listDeployments
function listDeployments() returns DeploymentsListResponse|error
List Deployments
Return Type
- DeploymentsListResponse|error - The list of deployments that belong to the authenticated user.
createDeployment
function createDeployment(DeploymentCreateRequest payload, string? requestId, boolean validateOnly) returns DeploymentCreateResponse|error
Create Deployment
Parameters
- payload DeploymentCreateRequest - The deployment definition
- requestId string? (default ()) - An optional idempotency token - if two create requests share the same request_id token (min size 32 characters, max 128) then only one deployment will be created, the second request will return the info of that deployment (in the same format described below, but with blanks for auth-related fields)
- validateOnly boolean (default false) - If true, will just validate the Deployment definition but will not perform the creation
Return Type
- DeploymentCreateResponse|error - The request was valid (used when validate_only is true).
upgradeDeploymentStatelessResource
function upgradeDeploymentStatelessResource(string deploymentId, string statelessResourceKind, string refId, boolean validateOnly) returns DeploymentResourceUpgradeResponse|error
Upgrade Kibana, APM, Integrations Server, AppSearch, Enterprise Search inside Deployment
Parameters
- deploymentId string - Identifier for the Deployment.
- statelessResourceKind string - The kind of stateless resource
- refId string - User-specified RefId for the Resource.
- validateOnly boolean (default false) - When
true
, returns the update version without performing the upgrade
Return Type
- DeploymentResourceUpgradeResponse|error - The upgrade command was issued successfully, use the "GET" command on the /{cluster_id} resource to monitor progress
Records
elasticsearch: AbsoluteRefId
A reference to a specific resource of a deployment
Fields
- deployment_id string - The deployment id
- ref_id string - The reference id of the resource in the given deployment
elasticsearch: AccountResponse
An account is the entity that owns deployments, and it is accessed by users. Accounts are isolated from each other.
Fields
- trust AccountTrustSettings? - Settings related to the level of trust of the clusters in this account
- id string - The account's identifier
elasticsearch: AccountTrustRelationship
The trust relationship with the clusters of one account.
Fields
- trust_allowlist string[]? - The list of clusters to trust. Only used when
trust_all
is false.
- account_id string - the ID of the Account
- trust_all boolean - If true, all clusters in this account will by default be trusted and the
trust_allowlist
is ignored.
elasticsearch: AccountTrustSettings
Settings related to the level of trust of the clusters in this account
Fields
- trust_all boolean - If true, all clusters in this account will by default trust all other clusters in the same account
elasticsearch: AccountUpdateRequest
A request to update an account
Fields
- trust AccountTrustSettings? - Settings related to the level of trust of the clusters in this account
elasticsearch: AllocatorMoveRequest
As part of the upgrade plan, identifies the move requests for the Kibana instances or APM Servers on the allocators.
Fields
- to string[]? - An optional list of allocator ids to which the instance(s) should be moved. If not specified then any available allocator can be used (including the current one if it is healthy)
- 'from string - The allocator id off which all instances in the cluster should be moved
- allocator_down boolean? - Tells the infrastructure that all instances on the allocator should be considered as permanently down when deciding how to migrate data to new nodes. If left blank then the system will auto-decide (currently: will treat the allocator as up)
elasticsearch: ApiKeyResponse
The response model for an API key.
Fields
- creation_date string - The date/time for when the API key is created.
- user_id string? - The user ID.
- id string - The API key ID.
- 'key string? - The API key. TIP: Since the API key is returned only once, save it in a safe place.
- description string - The API key description. TIP: Useful when you have multiple API keys.
elasticsearch: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- authorization string - Represents API Key
Authorization
elasticsearch: ApiKeysResponse
The response model for the API keys.
Fields
- keys ApiKeyResponse[] - The list of API keys.
elasticsearch: Apm
Holds diagnostics for an APM resource
Fields
- elasticsearch_cluster_ref_id string - The user-specified id of the Elasticsearch Cluster that this will link to
- backend_plan record {} - The backend plan as JSON
- display_name string - The human readable name (defaults to the generated cluster id if not specified)
- ref_id string - A locally-unique user-specified id
elasticsearch: ApmConfiguration
The configuration options for the APM Server.
Fields
- user_settings_override_json record {}? - An arbitrary JSON object allowing ECE admins owners to set clusters' parameters (only one of this and 'user_settings_override_yaml' is allowed), ie in addition to the documented 'system_settings'. (This field together with 'system_settings' and 'user_settings*' defines the total set of Apm settings)
- user_settings_yaml string? - An arbitrary YAML object allowing (non-admin) cluster owners to set their parameters (only one of this and 'user_settings_json' is allowed), provided the parameters are on the allowlist and not on the denylist. (These field together with 'user_settings_override*' and 'system_settings' defines the total set of Apm settings)
- 'version string? - The version of the Apm cluster (must be one of the ECE supported versions, and won't work unless it matches the APM version. Leave blank to auto-detect version.)
- user_settings_json record {}? - An arbitrary JSON object allowing (non-admin) cluster owners to set their parameters (only one of this and 'user_settings_yaml' is allowed), provided the parameters are on the allowlist and not on the denylist. (This field together with 'user_settings_override*' and 'system_settings' defines the total set of Apm settings)
- system_settings ApmSystemSettings? - A structure that defines a curated subset of the APM Server settings.
TIP: To define the complete set of APM Server setting, use
ApmSystemSettings
withuser_settings_override_
anduser_settings_
.
- user_settings_override_yaml string? - An arbitrary YAML object allowing ECE admins owners to set clusters' parameters (only one of this and 'user_settings_override_json' is allowed), ie in addition to the documented 'system_settings'. (This field together with 'system_settings' and 'user_settings*' defines the total set of Apm settings)
- docker_image string? - A docker URI that allows overriding of the default docker image specified for this version
elasticsearch: ApmCrudResponse
The response to an APM CRUD (create/update-plan) request.
Fields
- apm_id string? - For an operation creating or updating an APM server, the Id of that server
- secret_token string - The secret token for accessing the server
- diagnostics record {}? - If the endpoint is called with URL param 'validate_only=true', then this contains advanced debug info (the internal plan representation)
elasticsearch: ApmInfo
The overview information for the APM Server.
Fields
- status string - APM status
- apm_server_mode string? - The mode APM is operating in.
- name string - The name of the APM
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
- elasticsearch_cluster TargetElasticsearchCluster - Information about the specified Elasticsearch cluster.
- healthy boolean - Whether the APM is healthy or not (one or more of the info subsections will have healthy: false)
- region string? - The region that this APM belongs to. Only populated in SaaS or federated ECE.
- settings ApmSettings? - The settings for the APM Server.
- plan_info ApmPlansInfo - Information about current, pending, and past APM Server plans.
- external_links ExternalHyperlink[] - External resources related to the APM
- deployment_id string? - The id of the deployment that this APM Server belongs to.
- topology ClusterTopologyInfo - The topology for Elasticsearch clusters, multiple Kibana instances, or multiple APM Servers. The
ClusterTopologyInfo
also includes the instances and containers, and where they are located.
- id string - The id of the APM
- metadata ClusterMetadataInfo? - Information about the public and internal state, and the configuration settings of an Elasticsearch cluster.
elasticsearch: ApmPayload
An APM creation request paired with the alias of the Elasticsearch cluster it should be paired with
Fields
- elasticsearch_cluster_ref_id string - Alias to the Elasticsearch Cluster to attach APM to
- display_name string? - The human readable name for the APM cluster (default: takes the name of its Elasticsearch cluster)
- settings ApmSettings? - The settings for the APM Server.
- region string - The region where this resource exists
- ref_id string - A locally-unique user-specified id for APM
- plan ApmPlan - The plan for the APM Server.
elasticsearch: ApmPlan
The plan for the APM Server.
Fields
- cluster_topology ApmTopologyElement[]? -
- transient TransientApmPlanConfiguration? - Defines the configuration parameters that control how the plan is applied. For example, the Elasticsearch cluster topology and APM Server settings.
- apm ApmConfiguration - The configuration options for the APM Server.
elasticsearch: ApmPlanControlConfiguration
The plan control configuration options for the APM Server.
Fields
- cluster_reboot string? - Set to 'forced' to force a reboot as part of the upgrade plan
- extended_maintenance boolean? - If true (default false), does not clear the maintenance flag (which prevents its API from being accessed except by the constructor) on new instances added until after a snapshot has been restored, otherwise, the maintenance flag is cleared once the new instances successfully join the new cluster
- calm_wait_time int? - This timeout determines how long to give a cluster after it responds to API calls before performing actual operations on it. It defaults to 5s
- timeout int? - The total timeout in seconds after which the plan is cancelled even if it is not complete. Defaults to 4x the max memory capacity per node (in MB)
elasticsearch: ApmPlanInfo
Information about the APM Server plan.
Fields
- attempt_end_time string? - If this plan completed or failed (ie is not pending), when the attempt ended (ISO format in UTC)
- plan_attempt_id string? - A UUID for each plan attempt
- warnings ClusterPlanWarning[] -
- healthy boolean - Either the plan ended successfully, or is not yet completed (and no errors have occurred)
- plan_end_time string? - If this plan is not current or pending, when the plan was no longer active (ISO format in UTC)
- 'source ChangeSourceInfo? - A container for information about the source of a change.
- plan_attempt_log ClusterPlanStepInfo[] -
- plan ApmPlan? - The plan for the APM Server.
- plan_attempt_name string? - A human readable name for each plan attempt, only populated when retrieving plan histories
- attempt_start_time string? - When this plan attempt (ie to apply the plan to the APM) started (ISO format in UTC)
elasticsearch: ApmPlansInfo
Information about current, pending, and past APM Server plans.
Fields
- healthy boolean - Whether the plan situation is healthy (if unhealthy, means the last plan attempt failed)
- current ApmPlanInfo? - Information about the APM Server plan.
- pending ApmPlanInfo? - Information about the APM Server plan.
- history ApmPlanInfo[] -
elasticsearch: ApmResourceInfo
Describes an APM resource belonging to a Deployment
Fields
- elasticsearch_cluster_ref_id string - The Elasticsearch cluster that this resource depends on.
- info ApmInfo - The overview information for the APM Server.
- region string - The region where this resource exists
- id string - The randomly-generated id of a Resource
- ref_id string - The locally-unique user-specified id of a Resource
elasticsearch: ApmSettings
The settings for the APM Server.
Fields
- metadata ClusterMetadataSettings? - The top-level configuration settings for the Elasticsearch cluster.
elasticsearch: ApmSubInfo
Information about the APM Servers associated with the Elasticsearch cluster.
Fields
- apm_id string - The APM cluster Id
- enabled boolean - Whether the associated APM cluster is currently available
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
elasticsearch: ApmSystemSettings
A structure that defines a curated subset of the APM Server settings.
TIP: To define the complete set of APM Server setting, use ApmSystemSettings
with user_settings_override_
and user_settings_
.
Fields
- elasticsearch_url string? - Optionally override the URL to which to send data (for advanced users only, if unspecified the system selects an internal URL)
- secret_token string? - Optionally override the secret token within APM - defaults to the previously existing secretToken
- elasticsearch_password string? - Optionally override the account within APM - defaults to a system account that always exists (if specified, the username must also be specified). Note that this field is never returned from the API, it is write only.
- debug_enabled boolean? - Optionally enable debug mode for APM servers - defaults false
- kibana_url string? - Optionally override the URL to which to send data (for advanced users only, if unspecified the system selects an internal URL)
- elasticsearch_username string? - Optionally override the account within APM - defaults to a system account that always exists (if specified, the password must also be specified). Note that this field is never returned from the API, it is write only.
elasticsearch: ApmTopologyElement
Defines the topology of the APM Server nodes. For example, the number or capacity of the nodes, and where you can allocate the nodes.
Fields
- apm ApmConfiguration? - The configuration options for the APM Server.
- instance_configuration_id string? - Controls the allocation of this topology element as well as allowed sizes and node_types. It needs to match the id of an existing instance configuration.
- zone_count int? - number of zones in which nodes will be placed
- size TopologySize? - Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
elasticsearch: AppSearch
Holds diagnostics for an AppSearch resource
Fields
- elasticsearch_cluster_ref_id string - The user-specified id of the Elasticsearch Cluster that this will link to
- backend_plan record {} - The backend plan as JSON
- display_name string - The human readable name (defaults to the generated cluster id if not specified)
- ref_id string - A locally-unique user-specified id
elasticsearch: AppSearchConfiguration
Fields
- user_settings_override_json record {}? - An arbitrary JSON object allowing ECE admins owners to set clusters' parameters (only one of this and 'user_settings_override_yaml' is allowed), ie in addition to the documented 'system_settings'. (This field together with 'system_settings' and 'user_settings*' defines the total set of AppSearch settings)
- user_settings_yaml string? - An arbitrary YAML object allowing (non-admin) cluster owners to set their parameters (only one of this and 'user_settings_json' is allowed), provided the parameters are on the allowlist and not on the denylist. (These field together with 'user_settings_override*' and 'system_settings' defines the total set of AppSearch settings)
- 'version string? - The version of the AppSearch cluster (must be one of the ECE supported versions, and won't work unless it matches the Elasticsearch version. Leave blank to auto-detect version.)
- user_settings_json record {}? - An arbitrary JSON object allowing (non-admin) cluster owners to set their parameters (only one of this and 'user_settings_yaml' is allowed), provided the parameters are on the allowlist and not on the denylist. (This field together with 'user_settings_override*' and 'system_settings' defines the total set of AppSearch settings)
- system_settings AppSearchSystemSettings? - This structure defines a curated subset of the AppSearch settings. (This field together with 'user_settings_override*' and 'user_settings*' defines the total set of AppSearch settings)
- user_settings_override_yaml string? - An arbitrary YAML object allowing ECE admins owners to set clusters' parameters (only one of this and 'user_settings_override_json' is allowed), ie in addition to the documented 'system_settings'. (This field together with 'system_settings' and 'user_settings*' defines the total set of AppSearch settings)
- docker_image string? - A docker URI that allows overriding of the default docker image specified for this version
elasticsearch: AppSearchInfo
The overview information for the App Search Server.
Fields
- status string - App Search status
- name string - The name of the App Search
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
- elasticsearch_cluster TargetElasticsearchCluster - Information about the specified Elasticsearch cluster.
- healthy boolean - Whether the App Search is healthy or not (one or more of the info subsections will have healthy: false)
- region string? - The region that this App Search belongs to. Only populated in SaaS or federated ECE.
- settings AppSearchSettings? - The settings for the App Search.
- plan_info AppSearchPlansInfo - Information about current, pending, and past App Search Server plans.
- external_links ExternalHyperlink[] - External resources related to the App Search
- deployment_id string? - The id of the deployment that this App Search belongs to.
- topology ClusterTopologyInfo - The topology for Elasticsearch clusters, multiple Kibana instances, or multiple APM Servers. The
ClusterTopologyInfo
also includes the instances and containers, and where they are located.
- id string - The id of the App Search
- metadata ClusterMetadataInfo? - Information about the public and internal state, and the configuration settings of an Elasticsearch cluster.
elasticsearch: AppSearchNodeTypes
Node types to enable for an AppSearch instance
Fields
- appserver boolean - Defines whether this instance should run as Application/API server
- 'worker boolean - Defines whether this instance should run as background worker
elasticsearch: AppSearchPayload
An AppSearch creation request paired with the alias of the Elasticsearch cluster it should be paired with
Fields
- elasticsearch_cluster_ref_id string - Alias to the Elasticsearch Cluster to attach AppSearch to
- display_name string? - The human readable name for the AppSearch cluster (default: takes the name of its Elasticsearch cluster)
- settings AppSearchSettings? - The settings for the App Search.
- region string - The region where this resource exists
- ref_id string - A locally-unique user-specified id for AppSearch
- plan AppSearchPlan - The plan for the App Search cluster.
elasticsearch: AppSearchPlan
The plan for the App Search cluster.
Fields
- appsearch AppSearchConfiguration -
- cluster_topology AppSearchTopologyElement[]? -
- transient TransientAppSearchPlanConfiguration? - Defines configuration parameters that control how the plan (ie consisting of the cluster topology and AppSearch settings) is applied
elasticsearch: AppSearchPlanControlConfiguration
Fields
- cluster_reboot string? - Set to 'forced' to force a reboot as part of the upgrade plan
- move_allocators AllocatorMoveRequest[]? -
- reallocate_instances boolean? - If true (default: false) does not allow re-using any existing instances currently in the cluster, ie even unchanged instances will be re-created
- preferred_allocators string[]? - List of allocators on which instances are placed if possible (if not possible/not specified then any available allocator with space is used)
- calm_wait_time int? - This timeout determines how long to give a cluster after it responds to API calls before performing actual operations on it. It defaults to 5s
- timeout int? - The total timeout in seconds after which the plan is cancelled even if it is not complete. Defaults to 4x the max memory capacity per node (in MB)
- extended_maintenance boolean? - If true (default false), does not clear the maintenance flag (which prevents its API from being accessed except by the constructor) on new instances added until after a snapshot has been restored, otherwise, the maintenance flag is cleared once the new instances successfully join the new cluster
- move_instances InstanceMoveRequest[]? -
elasticsearch: AppSearchPlanInfo
Information about the App Search Server plan.
Fields
- attempt_end_time string? - If this plan completed or failed (ie is not pending), when the attempt ended (ISO format in UTC)
- plan_attempt_id string? - A UUID for each plan attempt
- warnings ClusterPlanWarning[] -
- healthy boolean - Either the plan ended successfully, or is not yet completed (and no errors have occurred)
- plan_end_time string? - If this plan is not current or pending, when the plan was no longer active (ISO format in UTC)
- 'source ChangeSourceInfo? - A container for information about the source of a change.
- plan_attempt_log ClusterPlanStepInfo[] -
- plan AppSearchPlan? - The plan for the App Search cluster.
- plan_attempt_name string? - A human readable name for each plan attempt, only populated when retrieving plan histories
- attempt_start_time string? - When this plan attempt (ie to apply the plan to the App Search) started (ISO format in UTC)
elasticsearch: AppSearchPlansInfo
Information about current, pending, and past App Search Server plans.
Fields
- healthy boolean - Whether the plan situation is healthy (if unhealthy, means the last plan attempt failed)
- current AppSearchPlanInfo? - Information about the App Search Server plan.
- pending AppSearchPlanInfo? - Information about the App Search Server plan.
- history AppSearchPlanInfo[] -
elasticsearch: AppSearchResourceInfo
Describes an App Search resource belonging to a Deployment
Fields
- elasticsearch_cluster_ref_id string - The Elasticsearch cluster that this resource depends on.
- info AppSearchInfo - The overview information for the App Search Server.
- region string - The region where this resource exists
- id string - The randomly-generated id of a Resource
- ref_id string - The locally-unique user-specified id of a Resource
elasticsearch: AppSearchSettings
The settings for the App Search.
Fields
- metadata ClusterMetadataSettings? - The top-level configuration settings for the Elasticsearch cluster.
elasticsearch: AppSearchSubInfo
Information about the APM Servers associated with the Elasticsearch cluster.
Fields
- enabled boolean - Whether the associated App Search is currently available
- app_search_id string - The App Search Id
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
elasticsearch: AppSearchSystemSettings
This structure defines a curated subset of the AppSearch settings. (This field together with 'user_settings_override*' and 'user_settings*' defines the total set of AppSearch settings)
Fields
- elasticsearch_password string? - Optionally override the account within App Search - defaults to a system account that always exists (if specified, the username must also be specified). Note that this field is never returned from the API, it is write only.
- elasticsearch_username string? - Optionally override the account within App Search - defaults to a system account that always exists (if specified, the password must also be specified). Note that this field is never returned from the API, it is write only.
- secret_session_key string? - Optionally override the secret session key within App Search - defaults to the previously existing secretSession. Note that this field is never returned from the API, it is write only.
- elasticsearch_url string? - Optionally override the URL to which to send data (for advanced users only, if unspecified the system selects an internal URL)
elasticsearch: AppSearchTopologyElement
Defines the topology of the AppSearch nodes (eg number/capacity of nodes, and where they can be allocated)
Fields
- appsearch AppSearchConfiguration? -
- node_type AppSearchNodeTypes? - Node types to enable for an AppSearch instance
- instance_configuration_id string? - Controls the allocation of this topology element as well as allowed sizes and node_types. It needs to match the id of an existing instance configuration.
- zone_count int? - number of zones in which nodes will be placed
- size TopologySize? - Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
elasticsearch: AutodetectStrategyConfig
A strategy that lets constructor choose the most optimal way to execute the plan.
elasticsearch: Balance
The available balance for an organization
Fields
- available decimal - Available balance
- line_items SimplifiedLineItem[] - A collection of order line items for for an organization
- remaining decimal - Remaining balance
elasticsearch: BasicFailedReply
Fields
- errors BasicFailedReplyElement[] - A list of errors that occurred in the failing request
elasticsearch: BasicFailedReplyElement
Fields
- fields string[]? - If the error can be tied to a specific field or fields in the user request, this lists those fields
- message string - A human readable message describing the error that occurred
- code string - A structured code representing the error type that occurred
elasticsearch: BoolQuery
A query for documents that match boolean combinations of other queries.
Fields
- filter QueryContainer[]? -
- minimum_should_match int? - The minimum number of optional should clauses to match.
- should QueryContainer[]? -
- must_not QueryContainer[]? -
- must QueryContainer[]? -
elasticsearch: ChangeSourceInfo
A container for information about the source of a change.
Fields
- user_id string? - The user that requested the change
- remote_addresses string[]? - The host addresses of the user that originated the change
- facilitator string - The service where the change originated from
- admin_id string? - The admin user that requested the change
- date string - The time the change was initiated
- action string - The type of plan change that was initiated
elasticsearch: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
elasticsearch: ClusterCredentials
The username and password for the new Elasticsearch cluster, which is returned from the Elasticsearch cluster create
command.
Fields
- username string - The username of the newly created cluster
- password string - The password of the newly created cluster
elasticsearch: ClusterCurationSettings
The index curation settings for an Elasticsearch cluster.
Fields
- specs ClusterCurationSpec[] - Specifications for curation
elasticsearch: ClusterCurationSpec
Specifies the conditions to trigger an Elasticsearch cluster curation.
Fields
- index_pattern string - Index matching pattern
- trigger_interval_seconds int - Number of seconds after index creation to trigger this spec
elasticsearch: ClusterInstanceConfigurationInfo
Information about a configuration that creates a Kibana instance or APM Server.
Fields
- 'resource string - The resource type of the instance configuration
- id string - The id of the configuration used to create the instance
- name string - The name of the configuration used to create the instance
elasticsearch: ClusterInstanceDiskInfo
Information about the use and storage capacity of a Kibana instance or APM Server.
Fields
- disk_space_available int? - If known, the amount of total disk space available to the container in MB
- storage_multiplier decimal - The storage multiplier originally defined to calculate disk space.
- disk_space_used int - The amount of disk space being used by the service in MB
elasticsearch: ClusterInstanceInfo
Information about each Kibana instance and APM Server in the Elasticsearch cluster.
Fields
- service_roles string[]? - List of roles assigned to the service running in the instance. Currently only populated for Elasticsearch, with possible values: master,data,ingest,ml
- zone string? - The zone in which this instance is being allocated
- container_started boolean - Whether the container has started (does not tell you anything about the service -ie Elasticsearch- running inside the container)
- service_version string? - The version of the service that the instance is running (eg Elasticsearch or Kibana), if available
- healthy boolean - Whether the instance is healthy (ie started and running)
- maintenance_mode boolean - Whether the service is is maintenance mode (meaning that the proxy is not routing external traffic to it)
- instance_name string - Whether the instance is healthy (ie started and running)
- instance_configuration ClusterInstanceConfigurationInfo? - Information about a configuration that creates a Kibana instance or APM Server.
- memory ClusterInstanceMemoryInfo? - Information about the memory capacity and use of the Kibana instance or APM Server.
- service_id string? - The service-specific (eg Elasticsearch) id of the node, if available
- disk ClusterInstanceDiskInfo? - Information about the use and storage capacity of a Kibana instance or APM Server.
- node_roles string[]? - A list of the node roles assigned to the service running in the instance. Currently populated only for Elasticsearch.
- allocator_id string? - The id of the allocator on which this instance is running (if the container is started or starting)
- service_running boolean - Whether the service launched inside the container -ie Elasticsearch- is actually running
elasticsearch: ClusterInstanceMemoryInfo
Information about the memory capacity and use of the Kibana instance or APM Server.
Fields
- instance_capacity int - The memory capacity in MB of the instance
- memory_pressure int? - The % memory pressure of the service if available (60-75% consider increasing capacity, >75% can incur significant performance and stability issues)
- instance_capacity_planned int? - The planned memory capacity in MB of the instance (only shown when an override is present)
- native_memory_pressure int? - The % native memory pressure of the service if available
elasticsearch: ClusterMetadataInfo
Information about the public and internal state, and the configuration settings of an Elasticsearch cluster.
Fields
- endpoint string? - The DNS name of the cluster endpoint, if available
- cloud_id string? - The cloud ID, an encoded string that provides other Elastic services with the necessary information to connect to this Elasticsearch and Kibana (only present if both exist)
- last_modified string - The most recent time the cluster metadata was changed (ISO format in UTC)
- aliased_endpoint string? - The DNS name of the cluster endpoint derived from the deployment alias, if available
- raw record {}? - An unstructured JSON representation of the public and internal state (can be filtered out via URL parameter). The contents and structure of the
raw
field can change at any time.
- 'version int - The resource version number of the cluster metadata
- service_url string? - The full URL to access this deployment resource
- aliased_url string? - The full aliased URL to access this deployment resource
- services_urls ServiceUrl[]? - A list of the URLs to access services that the resource provides at this time. Note that if the service is not running or has not started yet, the URL to access it won't be available
- ports ClusterMetadataPortInfo? - Information about the ports that allow communication between the Elasticsearch cluster and various protocols.
elasticsearch: ClusterMetadataPortInfo
Information about the ports that allow communication between the Elasticsearch cluster and various protocols.
Fields
- transport_passthrough int - Port where the cluster listens for transport traffic using TLS
- http int - Port where the cluster listens for HTTP traffic
- https int - Port where the cluster listens for HTTPS traffic
elasticsearch: ClusterMetadataSettings
The top-level configuration settings for the Elasticsearch cluster.
Fields
- name string? - The display name of the cluster
elasticsearch: ClusterPlanStepInfo
Information about a step in a plan.
Fields
- status string - The status of the step (success, warning, error - warning means something didn't go as expected but it was not serious enough to abort the plan)
- started string - When the step started (ISO format in UTC)
- duration_in_millis int? - The duration of the step in MS
- completed string? - When the step completed (ISO format in UTC)
- step_id string - ID of current step
- info_log ClusterPlanStepLogMessageInfo[] - Human readable summaries of the step, including messages for each stage of the step
- stage string - Current stage that the step is in
elasticsearch: ClusterPlanStepLogMessageInfo
The log message from a specified stage of an executed step in a plan.
Fields
- timestamp string - Timestamp marking on info log of step
- delta_in_millis int? - Time in milliseconds since previous log message
- details record {} - A map with details for the log about what happened during the step execution. Keys and values for are always both strings, representing the name of the detail and its value, respectively.
- failure_type string? - The failure type, in case the step failed
- message string - Human readable log message
- stage string - Stage that info log message takes place in
elasticsearch: ClusterPlanWarning
Information about a warning from a plan.
Fields
- step_id string? - The ID of the step which produced a warning, if any
- message string - A description of the warning
- code string - A unique warning code
elasticsearch: ClusterSnapshotRetention
Information about the Elasticsearch cluster snapshot retention.
Fields
- max_age string? - Total retention period for all snapshots, with the format 'length unit' (space is optional), where unit can be one of: d (day), h (hour), min (minute)
- snapshots int? - Number of snapshots to retain
elasticsearch: ClusterSnapshotSettings
The snapshot configuration settings for an Elasticsearch cluster.
Fields
- slm boolean? - When set to true, the deployment will have SLM enabled. Default value is true.
- cron_expression string? - Cron expression indicating when should snapshots be taken. This can be enabled only if SLM is enabled for the deployment and 'interval' is not present
- interval string? - Interval between snapshots, with the format 'length unit' (space is optional), where unit can be one of: d (day), h (hour), min (minute). Default is 30 minutes
- retention ClusterSnapshotRetention? - Information about the Elasticsearch cluster snapshot retention.
elasticsearch: ClusterSystemAlert
Information about a system alert on an Elasticsearch cluster.
Fields
- instance_name string - Instance that caused the system alert
- timestamp string - Timestamp marking the system alert
- alert_type string - Type of system alert
- url string? - The URL related to the event. Only applicable for alert_type: heap_dump
- exit_code int? - The exit_code related to the event. Only applicable for alert_type: slain
elasticsearch: ClusterTopologyInfo
The topology for Elasticsearch clusters, multiple Kibana instances, or multiple APM Servers. The ClusterTopologyInfo
also includes the instances and containers, and where they are located.
Fields
- healthy boolean - Whether the cluster topology is healthy (ie all instances are started and the services they run - ie elasticsearch - are available
- instances ClusterInstanceInfo[] -
elasticsearch: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
elasticsearch: Costs
Costs overview for an organization. All of the costs, credits, trials are expressed in Elastic Consumption Unit (ECU).
Fields
- total decimal - Total costs
- resources decimal - Total costs associated to the Elastic Cloud resources
- data_transfer_and_storage decimal - Total costs associated to Data Transfer and Storage (DTS)
elasticsearch: CostsOverview
The top level costs overview for an organization. All of the costs, credits, trials are expressed in Elastic Consumption Unit (ECU).
Fields
- trials decimal - Trial costs for the organization
- costs Costs - Costs overview for an organization. All of the costs, credits, trials are expressed in Elastic Consumption Unit (ECU).
- balance Balance? - The available balance for an organization
- hourly_rate decimal - Hourly rate applied.
elasticsearch: CreateApiKeyRequest
The request payload that creates the API keys.
Fields
- description string - API key description. Useful if there are multiple keys
- authentication_token string? - Deprecated. The security token from reauthenticate API
elasticsearch: CreateExtensionRequest
The body of a request to create a new extension
Fields
- 'version string - The Elasticsearch version.
- extension_type string - The extension type.
- name string - The extension name. Only ASCII alphanumeric and [_.-] characters allowed
- download_url string? - The URL to download the extension archive.
- description string? - The extension description.
elasticsearch: Creates
Holds diagnostics for resources that will be created
Fields
- appsearch AppSearch[]? - Diagnostics for AppSearches
- elasticsearch Elasticsearch[]? - Diagnostics for Elasticsearch clusters
- enterprise_search EnterpriseSearch[]? - Diagnostics for Enterprise Search resources
- kibana Kibana[]? - Diagnostics for Kibanas
- apm Apm[]? - Diagnostics for APMs
elasticsearch: DeleteApiKeysRequest
The request payload that deletes the API keys.
Fields
- keys string[] - The list of API key IDs.
elasticsearch: DeploymentCosts
Detailed costs for a deployment for an organization. All of the costs are expressed in Elastic Consumption Unit (ECU).
Fields
- deployment_id string - Elasticsearch deployment id
- costs Costs - Costs overview for an organization. All of the costs, credits, trials are expressed in Elastic Consumption Unit (ECU).
- period Period? - Period
- deployment_name string - Elasticsearch deployment name
- hourly_rate decimal - Price per hour
elasticsearch: DeploymentCreateMetadata
Additional information about the new deployment object.
Fields
- tags MetadataItem[]? - Arbitrary user-defined metadata associated with this deployment
elasticsearch: DeploymentCreateRequest
A request for creating a new Deployment consisting of multiple clusters
Fields
- alias string? - A user-defined alias to use in place of Cluster IDs for user-friendly URLs
- metadata DeploymentCreateMetadata? - Additional information about the new deployment object.
- name string? - A name for the deployment; otherwise this will be the generated deployment id
- resources DeploymentCreateResources - Describes the resources that will belong to a Deployment
- settings DeploymentCreateSettings? - Additional configuration for the new deployment object.
elasticsearch: DeploymentCreateResources
Describes the resources that will belong to a Deployment
Fields
- appsearch AppSearchPayload[]? - A list of payloads for AppSearch updates. AppSearch has been replaced by Enterprise Search in the Elastic Stack 7.7 and higher.
- elasticsearch ElasticsearchPayload[]? - A list of payloads for Elasticsearch cluster creation.
- enterprise_search EnterpriseSearchPayload[]? - A list of payloads for Enterprise Search creation.
- kibana KibanaPayload[]? - A list of payloads for Kibana creation.
- apm ApmPayload[]? - A list of payloads for APM creation.
elasticsearch: DeploymentCreateResponse
A response returned from the Deployment create endpoint
Fields
- name string - The name of the deployment
- created boolean - Whether or not the deployment was freshly created
- alias string? - A user-defined deployment alias for user-friendly resource URLs
- diagnostics DeploymentDiagnostics? - Describes the diagnostics for a given Deployment-modifying payload
- id string - The id of the deployment
- resources DeploymentResource[] - List of created resources.
elasticsearch: DeploymentCreateSettings
Additional configuration for the new deployment object.
Fields
- ip_filtering_settings IpFilteringSettings? - The configuration settings for IP filtering.
- observability DeploymentObservabilitySettings? - The observability settings for a deployment
- traffic_filter_settings TrafficFilterSettings? - The configuration settings for the traffic filter.
elasticsearch: DeploymentDiagnostics
Describes the diagnostics for a given Deployment-modifying payload
Fields
- creates Creates? - Holds diagnostics for resources that will be created
- updates Updates? - Holds diagnostics for existing resources that might be updated
elasticsearch: DeploymentGetResponse
Describes a given Deployment
Fields
- name string - The name of this deployment
- settings DeploymentSettings? - Additional configuration about the current deployment object.
- healthy boolean - Whether the deployment is overall healthy or not (one or more of the resource info subsections will have healthy: false)
- alias string? - A user-defined deployment alias for user-friendly resource URLs
- observability DeploymentObservability? - Observability information for a deployment
- id string - A randomly-generated id of this Deployment
- resources DeploymentResources - Describes a resource belonging to a Deployment
- metadata DeploymentMetadata? - Additional information about the current deployment object.
elasticsearch: DeploymentLogging
Logging information for a deployment
Fields
- healthy boolean - Whether the deployment logging is healthy or not
- issues ObservabilityIssue[]? - Logging health issues for the deployment
- urls record {}? - The URLs to view this deployment's logs in Kibana
elasticsearch: DeploymentLoggingSettings
The logging settings for a deployment
Fields
- destination AbsoluteRefId - A reference to a specific resource of a deployment
elasticsearch: DeploymentMetadata
Additional information about the current deployment object.
Fields
- tags MetadataItem[]? - Arbitrary user-defined metadata associated with this deployment
elasticsearch: DeploymentMetrics
Metrics information for a deployment
Fields
- healthy boolean - Whether the deployment metrics are healthy or not
- issues ObservabilityIssue[]? - Metrics health issues for the deployment
- urls record {}? - The URLs to view this deployment's metrics in Kibana
elasticsearch: DeploymentMetricsSettings
The metrics settings for a deployment
Fields
- destination AbsoluteRefId - A reference to a specific resource of a deployment
elasticsearch: DeploymentObservability
Observability information for a deployment
Fields
- healthy boolean - Whether the deployment observability is healthy or not (one or more of the subsections will have healthy: false)
- metrics DeploymentMetrics? - Metrics information for a deployment
- logging DeploymentLogging? - Logging information for a deployment
- issues ObservabilityIssue[]? - General observability health issues for the deployment
elasticsearch: DeploymentObservabilitySettings
The observability settings for a deployment
Fields
- metrics DeploymentMetricsSettings? - The metrics settings for a deployment
- logging DeploymentLoggingSettings? - The logging settings for a deployment
elasticsearch: DeploymentResource
Data for a deployment resource
Fields
- elasticsearch_cluster_ref_id string? - The Elasticsearch cluster that this resource depends on.
- kind string - The kind of resource
- cloud_id string? - An encoded string that provides other Elastic services with the necessary information to connect to this Elasticsearch and Kibana
- secret_token string? - Secret token for using a created resource. Only provided on initial create and absent otherwise.
- warnings ReplyWarning[]? - List of warnings generated from validating resource updates
- region string - Identifier of the region in which this resource runs.
- ref_id string - A locally-unique friendly alias for this Elasticsearch cluster
- credentials ClusterCredentials? - The username and password for the new Elasticsearch cluster, which is returned from the Elasticsearch cluster
create
command.
- id string - A system-unique id for the created resource
elasticsearch: DeploymentResourceCommandResponse
Response returned when a command is successfully issued against a given Deployment resource
Fields
- warnings ReplyWarning[]? - List of warnings generated from validating command
elasticsearch: DeploymentResourceCrudResponse
A response returned from the Deployment Resource endpoints
Fields
- kind string - The kind of the stateless resource
- id string - The id of the deployment
- ref_id string - The reference id of the resource
elasticsearch: DeploymentResources
Describes a resource belonging to a Deployment
Fields
- appsearch AppSearchResourceInfo[] - List of App Search resources in your Deployment
- elasticsearch ElasticsearchResourceInfo[] - List of Elasticsearch resources in your Deployment
- enterprise_search EnterpriseSearchResourceInfo[] - List of Enterprise Search resources in your Deployment
- kibana KibanaResourceInfo[] - List of Kibana resources in your Deployment
- apm ApmResourceInfo[] - List of Apm resources in your Deployment
elasticsearch: DeploymentResourceUpgradeResponse
Fields
- stack_version string -
- resource_id string -
elasticsearch: DeploymentRestoreResponse
A response returned from the Deployment restore endpoint
Fields
- id string - The id of the deployment
elasticsearch: DeploymentsCosts
Costs associated to a set of deployments for an organization. All of the costs are expressed in Elastic Consumption Unit (ECU).
Fields
- total_cost decimal - Total cost for all deployments
- deployments DeploymentCosts[] - Costs of the list of deployments
elasticsearch: DeploymentSearchResponse
Describes a searched Deployment
Fields
- name string - The name of this deployment
- settings DeploymentSettings? - Additional configuration about the current deployment object.
- healthy boolean - Whether the deployment is overall healthy or not (one or more of the resource info subsections will have healthy: false)
- alias string? - A user-defined deployment alias for user-friendly resource URLs
- id string - A randomly-generated id of this Deployment
- resources DeploymentResources - Describes a resource belonging to a Deployment
- metadata DeploymentMetadata? - Additional information about the current deployment object.
elasticsearch: DeploymentSettings
Additional configuration about the current deployment object.
Fields
- ip_filtering_settings IpFilteringSettings? - The configuration settings for IP filtering.
- observability DeploymentObservabilitySettings? - The observability settings for a deployment
- traffic_filter_settings TrafficFilterSettings? - The configuration settings for the traffic filter.
elasticsearch: DeploymentShutdownResponse
A response returned from the Deployment shutdown endpoint
Fields
- id string - The id of the deployment
- orphaned Orphaned? - Details about orphaned resources.
- name string - The name of the deployment
elasticsearch: DeploymentsListingData
Fields
- id string - The id of this deployment
- resources DeploymentResource[] - List of resources in this deployment
- name string - The name of this deployment
elasticsearch: DeploymentsListResponse
Contains a list of deployments
Fields
- deployments DeploymentsListingData[] - The deployments
elasticsearch: DeploymentsSearchResponse
Contains a list of Deployments as result of a search request.
Fields
- match_count int? - If a query is supplied, then the total number of deployments that matched
- return_count int - The number of deployments actually returned
- deployments DeploymentSearchResponse[] -
elasticsearch: DeploymentTemplateInfoV2
Deployment template detailed information
Fields
- instance_configurations InstanceConfigurationInfo[] - List of instance configurations used in the cluster template.
- description string? - An optional description for the template.
- template_category_id string? - Provider and version agnostic template identifier used for grouping related template types.
- min_version string? - Minimum stack version required by this template, if any.
- kibana_deeplink KibanaDeeplink[]? - The Kibana Deeplink for this type of deployment.
- id string - The unique identifier for the template.
- 'order int? - Determines the order in which this template should be returned when listed. Templates are returned in ascending order. If not specified, then the template willbe appended to the end of the list.
- 'source ChangeSourceInfo? - A container for information about the source of a change.
- deployment_template DeploymentCreateRequest - A request for creating a new Deployment consisting of multiple clusters
- metadata MetadataItem[]? - Optional arbitrary metadata to associate with this template.
- system_owned boolean? - Whether or not if this is system owned template.
- name string - A human readable name for the template.
elasticsearch: DeploymentTemplateReference
Specifies the deployment template used to create the plan.
Fields
- 'version string? - A version identifier to disambiguate multiple revisions of the same template
- id string - The unique identifier of the deployment template
elasticsearch: DeploymentUpdateMetadata
Additional information about the current deployment object.
Fields
- tags MetadataItem[]? - Arbitrary user-defined metadata associated with this deployment
elasticsearch: DeploymentUpdateRequest
A request for updating a Deployment consisting of multiple resources
Fields
- name string? - A new name for the deployment, otherwise stays the same.
- settings DeploymentUpdateSettings? - Additional configuration for the new deployment object.
- prune_orphans boolean - Whether or not to prune orphan resources that are no longer mentioned in this request. Note that resourcesare tracked by ref_id, and if a resource's ref_id is changed, any previous running resources created with that previousref_id are considered to be orphaned as well.
- alias string? - A user-defined alias to use in place of Cluster IDs for user-friendly URLs
- resources DeploymentUpdateResources? - Describes the Deployment resource updates
- metadata DeploymentUpdateMetadata? - Additional information about the current deployment object.
elasticsearch: DeploymentUpdateResources
Describes the Deployment resource updates
Fields
- appsearch AppSearchPayload[]? - A list of payloads for AppSearch updates. AppSearch has been replaced by Enterprise Search in the Elastic Stack 7.7 and higher
- elasticsearch ElasticsearchPayload[]? - A list of payloads for Elasticsearch cluster updates
- enterprise_search EnterpriseSearchPayload[]? - A list of payloads for Enterprise Search updates
- kibana KibanaPayload[]? - A list of payloads for Kibana updates
- apm ApmPayload[]? - A list of payloads for APM updates
elasticsearch: DeploymentUpdateResponse
A response returned from the Deployment update endpoint
Fields
- name string - The name of the deployment
- alias string? - A user-defined alias to use in place of ResourceIds for user-friendly resource URLs
- diagnostics DeploymentDiagnostics? - Describes the diagnostics for a given Deployment-modifying payload
- shutdown_resources Orphaned? - Details about orphaned resources.
- id string - The id of the deployment
- resources DeploymentResource[] - List of resources that are part of the deployment after the update operation.
elasticsearch: DeploymentUpdateSettings
Additional configuration for the new deployment object.
Fields
- observability DeploymentObservabilitySettings? - The observability settings for a deployment
elasticsearch: DeploymentUpgradeAssistantStatusResponse
The status of your cluster and its readiness to be upgraded
Fields
- ready_for_upgrade boolean - A boolean indicating whether or not the cluster is ready to be upgraded
- details string - Message with information about the number of Elasticsearch and Kibana deprecations
elasticsearch: DiscreteSizes
Instance sizes that are supported by the Elasticsearch instance, Kibana instance, or APM Server configuration.
Fields
- default_size int - The default size
- 'resource string - The unit that each size represents
- sizes int[] - List of supported sizes
elasticsearch: DtsDimensionCosts
The costs associated to a Data Transfer and Storage (DTS) dimension for an organization. All of the costs, credits, and trials are expressed in Elastic Consumption Units (ECU).
Fields
- sku string - DTS dimension Stock Keeping Unit (SKU)
- name string - DTS dimension name
- rate DtsRate - DTS Rate
- cost decimal - Costs associated to the Data Transfer and Storage (DTS) dimensions for an organization
- 'type string - Type of the DTS dimension usage
- quantity DtsQuantity - DTS quantity
elasticsearch: DtsQuantity
DTS quantity
Fields
- formatted_value string - Quantity in human readable format
- value int - Raw quantity
elasticsearch: DtsRate
DTS Rate
Fields
- formatted_value string - Rate in human readable format
- value decimal - Raw rate
elasticsearch: Elasticsearch
Holds diagnostics for an Elasticsearch cluster
Fields
- backend_plan record {} - The backend plan as JSON
- display_name string - The human readable name for the cluster (defaults to the generated cluster id if not specified)
- ref_id string - A locally-unique user-specified id
elasticsearch: ElasticsearchClusterBlockingIssueElement
Information about an issue and the Elasticsearch instance it affects.
Fields
- instances string[] - A list of instances that are affected by the issue
- description string - Description of the issue
elasticsearch: ElasticsearchClusterBlockingIssues
Issues that prevent the Elasticsearch cluster or index from correctly operating.
Fields
- healthy boolean - Whether the cluster has issues (false) or not (true)
- cluster_level ElasticsearchClusterBlockingIssueElement[] - A list of issues that affect availability of entire cluster
- index_level ElasticsearchClusterBlockingIssueElement[] - A list of issues that affect availability of the cluster's indices
elasticsearch: ElasticsearchClusterInfo
The information for an Elasticsearch cluster.
Fields
- status string - Cluster status
- associated_apm_clusters ApmSubInfo[] -
- associated_kibana_clusters KibanaSubClusterInfo[] -
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
- associated_enterprise_search_clusters EnterpriseSearchSubInfo[] -
- settings ElasticsearchClusterSettings? - The settings for an Elasticsearch cluster.
- healthy boolean - Whether the cluster is healthy or not (one or more of the info subsections will have healthy: false)
- associated_appsearch_clusters AppSearchSubInfo[] -
- region string? - The region that this cluster belongs to. Only populated in SaaS or federated ECE.
- elasticsearch_monitoring_info ElasticsearchMonitoringInfo? - Information about the monitoring status for the Elasticsearch cluster.
- snapshots SnapshotStatusInfo - Information about the snapshot status for the Elasticsearch cluster. For example, the health status.
- cluster_name string - The name of the cluster
- plan_info ElasticsearchClusterPlansInfo - Information about the current, pending, and past Elasticsearch cluster plans.
- cluster_id string - The id of the cluster
- external_links ExternalHyperlink[] - External resources related to the cluster
- system_alerts ClusterSystemAlert[]? - List of cluster system alerts
- elasticsearch ElasticsearchInfo - Information about the Elasticsearch cluster.
- deployment_id string? - The id of the deployment that this Elasticsearch belongs to.
- topology ClusterTopologyInfo - The topology for Elasticsearch clusters, multiple Kibana instances, or multiple APM Servers. The
ClusterTopologyInfo
also includes the instances and containers, and where they are located.
- security ElasticsearchClusterSecurityInfo? - For 2.x Elasticsearch clusters, specifies the information about the users and roles. For 5.x Elasticsearch clusters, use the Kibana management UI.
- metadata ClusterMetadataInfo - Information about the public and internal state, and the configuration settings of an Elasticsearch cluster.
elasticsearch: ElasticsearchClusterPlan
The plan for the Elasticsearch cluster.
Fields
- autoscaling_enabled boolean? - Enable autoscaling for this Elasticsearch cluster.
- cluster_topology ElasticsearchClusterTopologyElement[] -
- transient TransientElasticsearchPlanConfiguration? - Defines the configuration parameters that control how the plan is applied. For example, the Elasticsearch cluster topology and Elasticsearch settings.
- elasticsearch ElasticsearchConfiguration - The Elasticsearch cluster settings. When specified at the top level, provides a field-by-field default. When specified at the topology level, provides the override settings.
- deployment_template DeploymentTemplateReference? - Specifies the deployment template used to create the plan.
elasticsearch: ElasticsearchClusterPlanInfo
Information about the Elasticsearch cluster plan.
Fields
- attempt_end_time string? - If this plan completed or failed (ie is not pending), when the attempt ended (ISO format in UTC)
- plan_attempt_id string? - A UUID for each plan attempt
- warnings ClusterPlanWarning[] -
- healthy boolean - Either the plan ended successfully, or is not yet completed (and no errors have occurred)
- plan_end_time string? - If this plan is not current or pending, when the plan was no longer active (ISO format in UTC)
- 'source ChangeSourceInfo? - A container for information about the source of a change.
- plan_attempt_log ClusterPlanStepInfo[] -
- plan ElasticsearchClusterPlan? - The plan for the Elasticsearch cluster.
- plan_attempt_name string? - A human readable name for each plan attempt, only populated when retrieving plan histories
- attempt_start_time string? - When this plan attempt (ie to apply the plan to the cluster) started (ISO format in UTC)
elasticsearch: ElasticsearchClusterPlansInfo
Information about the current, pending, and past Elasticsearch cluster plans.
Fields
- healthy boolean - Whether the plan situation is healthy (if unhealthy, means the last plan attempt failed)
- current ElasticsearchClusterPlanInfo? - Information about the Elasticsearch cluster plan.
- pending ElasticsearchClusterPlanInfo? - Information about the Elasticsearch cluster plan.
- history ElasticsearchClusterPlanInfo[] -
elasticsearch: ElasticsearchClusterRole
The authorization information for an Elasticsearch cluster user.
Fields
- username string - The username
- roles string[] - The list of roles for this user
elasticsearch: ElasticsearchClusterSecurityInfo
For 2.x Elasticsearch clusters, specifies the information about the users and roles. For 5.x Elasticsearch clusters, use the Kibana management UI.
Fields
- users_roles ElasticsearchClusterRole[] -
- roles record {} - An arbitrarily nested JSON object mapping roles to sets of resources and permissions - see the Elasticsearch security documentation for more details on roles
- 'version int - The resource version number of the security settings
- users ElasticsearchClusterUser[] -
- last_modified string - The most recent time the security settings were changed (ISO format in UTC)
elasticsearch: ElasticsearchClusterSettings
The settings for an Elasticsearch cluster.
Fields
- monitoring ManagedMonitoringSettings? - The settings for sending monitoring information to another cluster.
- curation ClusterCurationSettings? - The index curation settings for an Elasticsearch cluster.
- ip_filtering IpFilteringSettings? - The configuration settings for IP filtering.
- snapshot ClusterSnapshotSettings? - The snapshot configuration settings for an Elasticsearch cluster.
- traffic_filter TrafficFilterSettings? - The configuration settings for the traffic filter.
- trust ElasticsearchClusterTrustSettings? - Configuration of trust with other clusters.
- dedicated_masters_threshold int? - Threshold starting from which the number of instances in the cluster results in the introduction of dedicated masters. If the cluster is downscaled to a number of nodes below this one, dedicated masters will be removed. Limit is inclusive.
- metadata ClusterMetadataSettings? - The top-level configuration settings for the Elasticsearch cluster.
elasticsearch: ElasticsearchClusterTopologyElement
The topology of the Elasticsearch nodes, including the number, capacity, and type of nodes, and where they can be allocated.
Fields
- autoscaling_policy_override_json record {}? - An arbitrary JSON object overriding the default autoscaling policy. Don't set unless you really know what you are doing.
- zone_count int? - The default number of zones in which data nodes will be placed
- topology_element_control TopologyElementControl? - Controls for the topology element. Only used as part of the deployment template. Ignored if included as part of a deployment.
- autoscaling_min TopologySize? - Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
- node_type ElasticsearchNodeType? - Controls the combinations of Elasticsearch node types. TIP: By default, the Elasticsearch node is master eligible, can hold data, and run ingest pipelines. WARNING: Do not set for tiebreaker topologies.
- elasticsearch ElasticsearchConfiguration? - The Elasticsearch cluster settings. When specified at the top level, provides a field-by-field default. When specified at the topology level, provides the override settings.
- autoscaling_max TopologySize? - Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
- instance_configuration_id string? - Controls the allocation of this topology element as well as allowed sizes and node_types. It needs to match the id of an existing instance configuration.
- node_roles string[]? - The list of node roles for this topology element (ES version >= 7.10). Allowable values are: master, ingest, ml, data_hot, data_content, data_warm, data_cold, data_frozen, remote_cluster_client, transform
- id string? - Unique identifier of this topology element
- size TopologySize? - Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
elasticsearch: ElasticsearchClusterTrustSettings
Configuration of trust with other clusters.
Fields
- accounts AccountTrustRelationship[]? - The list of trust relationships with different accounts
- 'external ExternalTrustRelationship[]? - The list of trust relationships with external entities
elasticsearch: ElasticsearchClusterUser
The information about an Elasticsearch cluster user.
Fields
- username string - The username
- password_hash string - The hashed password
elasticsearch: ElasticsearchConfiguration
The Elasticsearch cluster settings. When specified at the top level, provides a field-by-field default. When specified at the topology level, provides the override settings.
Fields
- user_settings_override_json record {}? - An arbitrary JSON object allowing ECE admins owners to set clusters' parameters (only one of this and 'user_settings_override_yaml' is allowed), ie in addition to the documented 'system_settings'. NOTES: (This field together with 'system_settings' and 'user_settings*' defines the total set of Elasticsearch settings)
- enabled_built_in_plugins string[]? - A list of plugin names from the Elastic-supported subset that are bundled with the version images. NOTES: (Users should consult the Elastic stack objects to see what plugins are available, this is currently only available from the UI)
- user_plugins ElasticsearchUserPlugin[]? - A list of admin-uploaded plugin objects that are available for this user.
- user_settings_yaml string? - An arbitrary YAML object allowing cluster owners to set their parameters (only one of this and 'user_settings_json' is allowed), provided the parameters arey are on the allowlist and not on the denylist. NOTES: (This field together with 'user_settings_override*' and 'system_settings' defines the total set of Elasticsearch settings)
- user_bundles ElasticsearchUserBundle[]? - A list of admin-uploaded bundle objects (eg scripts, synonym files) that are available for this user.
- 'version string? - The version of the Elasticsearch cluster (must be one of the ECE supported versions). Currently cannot be different across the topology (and is generally specified in the globals). Defaults to the latest version if not specified.
- user_settings_json record {}? - An arbitrary JSON object allowing cluster owners to set their parameters (only one of this and 'user_settings_yaml' is allowed), provided the parameters arey are on the allowlist and not on the denylist. NOTES: (This field together with 'user_settings_override*' and 'system_settings' defines the total set of Elasticsearch settings)
- curation ElasticsearchCuration? - The structure that defines the routing settings for index curation.
- system_settings ElasticsearchSystemSettings? - A subset of Elasticsearch settings. TIP: To define the complete set of Elasticsearch settings, use
ElasticsearchSystemSettings
withuser_settings_override*
anduser_settings*
.
- user_settings_override_yaml string? - An arbitrary YAML object allowing ECE admins owners to set clusters' parameters (only one of this and 'user_settings_override_json' is allowed), ie in addition to the documented 'system_settings'. NOTES: (This field together with 'system_settings' and 'user_settings*' defines the total set of Elasticsearch settings)
- docker_image string? - A docker URI that allows overriding of the default docker image specified for this version
- node_attributes record {}? - Defines the Elasticsearch node attributes for the instances in the topology
elasticsearch: ElasticsearchCuration
The structure that defines the routing settings for index curation.
Fields
- to_instance_configuration_id string - The destination instance configuration
- from_instance_configuration_id string - The source instance configuration
elasticsearch: ElasticsearchDependant
Details about an orphaned Elasticsearch-dependent resources.
Fields
- kind string - The kind of resource
- id string - The id of the orphaned resource
elasticsearch: ElasticsearchElasticUserPasswordResetResponse
Envelope holding the newly-reset password for a cluster's user
Fields
- username string - The username for the newly-reset password for the given Elasticsearch cluster
- password string - The newly-reset password for the given Elasticsearch cluster
elasticsearch: ElasticsearchInfo
Information about the Elasticsearch cluster.
Fields
- healthy boolean - Whether the Elasticsearch cluster is healthy (check the sub-objects for more details if not)
- shard_info ElasticsearchShardsInfo - Information about the shards and replicas that comprise the Elasticsearch indices.
- master_info ElasticsearchMasterInfo - Information about the master nodes in the Elasticsearch cluster.
- blocking_issues ElasticsearchClusterBlockingIssues - Issues that prevent the Elasticsearch cluster or index from correctly operating.
elasticsearch: ElasticsearchMasterElement
Information about the Elasticsearch instances. For split-brain cases, this also includes sub-clusters.
Fields
- instances string[] - The names of the instance/container hosting the node belong to the cluster with the given master
- master_node_id string - The Elasticsearch node id of a master node
- master_instance_name string? - The corresponding instance name of the container hosting the Elasticsearch master node, if available
elasticsearch: ElasticsearchMasterInfo
Information about the master nodes in the Elasticsearch cluster.
Fields
- healthy boolean - Whether the master situation in the cluster is healthy (ie is the number of masters != 1), or do any instances have no master
- instances_with_no_master string[] - A list of any instances with no master
- masters ElasticsearchMasterElement[] -
elasticsearch: ElasticsearchMonitoringInfo
Information about the monitoring status for the Elasticsearch cluster.
Fields
- healthy boolean - Whether the Monitoring configuration has been successfully applied
- last_update_status string - The status message from the last update (successful or not)
- destination_cluster_ids string[] - The list of clusters Ids to which this cluster is currently sending monitoring data
- last_modified string - The time the monitoring configuration was last changed
- source_cluster_ids string[] - The list of clusters Ids from which this cluster is currently receiving monitoring data
elasticsearch: ElasticsearchNodeType
Controls the combinations of Elasticsearch node types. TIP: By default, the Elasticsearch node is master eligible, can hold data, and run ingest pipelines. WARNING: Do not set for tiebreaker topologies.
Fields
- data boolean? - Defines whether this node can hold data (default: false)
- master boolean? - Defines whether this node can be elected master (default: false)
- ingest boolean? - Defines whether this node can run an ingest pipeline (default: false)
- ml boolean? - Defines whether this node can run ml jobs, valid only for versions 5.4.0 or greater (default: false)
elasticsearch: ElasticsearchPayload
An alias for an Elasticsearch Cluster paired with a request for creating one
Fields
- region string - The region where this resource exists
- settings ElasticsearchClusterSettings? - The settings for an Elasticsearch cluster.
- display_name string? - The human readable name for the cluster (defaults to the generated cluster id if not specified)
- plan ElasticsearchClusterPlan - The plan for the Elasticsearch cluster.
- ref_id string - A locally-unique user-specified id for this Elasticsearch cluster
elasticsearch: ElasticsearchPlanControlConfiguration
The configuration settings for the timeout and fallback parameters.
Fields
- cluster_reboot string? - Set to 'forced' to force a reboot as part of the upgrade plan. NOTES: (ie taking an existing plan and leaving it alone except for setting 'transient.plan_configuration.cluster_reboot': 'forced' will reboot the cluster)
- calm_wait_time int? - This timeout determines how long to give a cluster after it responds to API calls before performing actual operations on it. It defaults to 5s
- skip_snapshot boolean? - If true (default: false), does not take (or require) a successful snapshot to be taken before performing any potentially destructive changes to this cluster
- max_snapshot_age int? - When you take a snapshot and 'skip_snapshots' is false, specifies the maximum age in seconds of the most recent snapshot before a new snapshot is created. Default is 300
- timeout int? - The total timeout in seconds after which the plan is cancelled even if it is not complete. Defaults to 4x the max memory capacity per node (in MB). NOTES: A 3 zone cluster with 2 nodes of 2048 each would have a timeout of 4*2048=8192 seconds. Timeout does not include time required to run rollback actions.
- extended_maintenance boolean? - If true (default false), does not clear the maintenance flag (which prevents its API from being accessed except by the constructor) on new instances added until after a snapshot has been restored, otherwise, the maintenance flag is cleared once the new instances successfully join the new cluster
- max_snapshot_attempts int? - If taking a snapshot (ie unless 'skip_snapshots': true) then will retry on failure at most this number of times (default: 5)
elasticsearch: ElasticsearchReplicaElement
Information about the unavailable replicas. NOTE: Unlike shards, unavailable replicas indicate a loss of redundancy rather than a loss of availability.
Fields
- instance_name string - The Elastic Cloud name/id of the instance (container)
- replica_count int - The number of unavailable replicas on this instance
elasticsearch: ElasticsearchResourceInfo
Describes an Elasticsearch resource belonging to a Deployment
Fields
- info ElasticsearchClusterInfo - The information for an Elasticsearch cluster.
- region string - The region where this resource exists
- id string - The randomly-generated id of a Resource
- ref_id string - The locally-unique user-specified id of a Resource
elasticsearch: ElasticsearchScriptingUserSettings
Controls the languages supported by the Elasticsearch cluster, such as Painless, Mustache, and Expressions. Controls how the languages are used, such as file, index, and inline. TIP: For complex configurations, leave these blank and configure these settings in the user YAML or JSON.
Fields
- expressions_enabled boolean? - (5.x+ only) If enabled (the default) then the expressions scripting engine is allowed as a sandboxed language. Sandboxed languages are the only ones allowed if 'sandbox_mode' is set to true. NOTES: (Corresponds to the parameters 'script.engine.expression.[file|stored|inline]')
- stored ElasticsearchScriptTypeSettings? - Enables scripting for the specified type and controls other parameters. Store scripts in indexes (
stored
), upload in file bundles (file
), or use in API requests (inline
).
- painless_enabled boolean? - (5.x+ only) If enabled (the default) then the painless scripting engine is allowed as a sandboxed language. Sandboxed languages are the only ones allowed if 'sandbox_mode' is set to true. NOTES: (Corresponds to the parameters 'script.engine.painless.[file|stored|inline]')
- file ElasticsearchScriptTypeSettings? - Enables scripting for the specified type and controls other parameters. Store scripts in indexes (
stored
), upload in file bundles (file
), or use in API requests (inline
).
- inline ElasticsearchScriptTypeSettings? - Enables scripting for the specified type and controls other parameters. Store scripts in indexes (
stored
), upload in file bundles (file
), or use in API requests (inline
).
- mustache_enabled boolean? - (5.x+ only) If enabled (the default) then the mustache scripting engine is allowed as a sandboxed language. Sandboxed languages are the only ones allowed if 'sandbox_mode' is set to true. NOTES: (Corresponds to the parameters 'script.engine.mustache.[file|stored|inline]')
elasticsearch: ElasticsearchScriptTypeSettings
Enables scripting for the specified type and controls other parameters. Store scripts in indexes (stored
), upload in file bundles (file
), or use in API requests (inline
).
Fields
- enabled boolean? - If enabled (default: true) then scripts are enabled, either for sandboxing languages (by default), or for all installed languages if 'sandbox_mode' is disabled (or for 6.x). NOTES: (Corresponds to the parameter 'script.file|stored/indexed|inline')
- sandbox_mode boolean? - If enabled (default: true) and this script type is enabled, then only the sandbox languages are allowed. By default the sandbox languages are painless, expressions and mustache, but this can be restricted via the 'painless_enabled', 'mustache_enabled' 'expression_enabled' settings.NOTES: Not supported in 6.x. (Corresponds to the parameters 'script.engine.[painless|mustache|expressions].[file|stored|inline]')
elasticsearch: ElasticsearchShardElement
Information about the shards for each Elasticsearch instance container that hosts an Elasticsearch node. TIP: When the shard is unavailable, the cluster is unable to serve all of the data.
Fields
- instance_name string - The Elastic Cloud name/id of the instance (container)
- shard_count int - The number of shards of the given type (available/unavailable) on this instance
elasticsearch: ElasticsearchShardsInfo
Information about the shards and replicas that comprise the Elasticsearch indices.
Fields
- healthy boolean - Whether the shard situation is healthy (any unavailable shards is unhealthy)
- unavailable_shards ElasticsearchShardElement[] -
- unavailable_replicas ElasticsearchReplicaElement[] -
- available_shards ElasticsearchShardElement[] -
elasticsearch: ElasticsearchSystemSettings
A subset of Elasticsearch settings. TIP: To define the complete set of Elasticsearch settings, use ElasticsearchSystemSettings
with user_settings_override*
and user_settings*
.
Fields
- reindex_whitelist string[]? - Limits remote Elasticsearch clusters that can be used as the source for '_reindex' API commands
- default_shards_per_index int? - (2.x only - to get the same result in 5.x template mappings must be used) Sets the default number of shards per index, defaulting to 1 if not specified. (Corresponds to the parameter 'index.number_of_shards' in 2.x, not supported in 5.x)
- monitoring_history_duration string? - The duration for which monitoring history is stored (format '(NUMBER)d' eg '3d' for 3 days). NOTES: ('Corresponds to the parameter xpack.monitoring.history.duration' in 5.x, defaults to '7d')
- monitoring_collection_interval int? - The default interval at which monitoring information from the cluster if collected, if monitoring is enabled. NOTES: (Corresponds to the parameter 'marvel.agent.interval' in 2.x and 'xpack.monitoring.collection.interval' in 5.x)
- destructive_requires_name boolean? - If true (default is false) then the index deletion API will not support wildcards or '_all'. NOTES: (Corresponds to the parameter 'action.destructive_requires_name')
- auto_create_index boolean? - If true (the default), then any write operation on an index that does not currently exist will create it. NOTES: (Corresponds to the parameter 'action.auto_create_index')
- watcher_trigger_engine string? - The trigger engine for Watcher, defaults to 'scheduler' - see the xpack documentation for more information. NOTES: (Corresponds to the parameter '(xpack.)watcher.trigger.schedule.engine', depending on version. Ignored from 6.x onwards.)
- use_disk_threshold boolean? - Whether to factor in the available disk space on a node before deciding whether to allocate new shards to that node or actively relocate shards away from the node (default: true). NOTES: (Corresponds to the parameter 'cluster.routing.allocation.disk.threshold_enabled')
- scripting ElasticsearchScriptingUserSettings? - Controls the languages supported by the Elasticsearch cluster, such as Painless, Mustache, and Expressions. Controls how the languages are used, such as file, index, and inline. TIP: For complex configurations, leave these blank and configure these settings in the user YAML or JSON.
- enable_close_index boolean? - Defaults to false on versions <= 7.2.0, true otherwise. If false, then the API commands to close indices are disabled. This is important because Elasticsearch does not snapshot or migrate close indices on versions under 7.2.0, therefore standard Elastic Cloud configuration operations will cause irretrievable loss of indices' data. NOTES: (Corresponds to the parameter 'cluster.indices.close.enable')
elasticsearch: ElasticsearchUserBundle
A list of admin-uploaded bundle objects, such as scripts and synonym files.
Fields
- url string - The URL of the bundle, which must be accessible from the ECE infrastructure. This URL could be cached by platform, make sure to change it when updating the bundle
- name string - The name of the bundle
- elasticsearch_version string - The supported Elasticsearch version (must match the version in the plan)
elasticsearch: ElasticsearchUserPlugin
A list of admin-uploaded plugin objects.
Fields
- url string - The URL of the plugin (must be accessible from the ECE infrastructure)
- name string - The name of the plugin
- elasticsearch_version string - The supported Elasticsearch version (must match the version in the plan)
elasticsearch: EmptyResponse
elasticsearch: EnableIlmRequest
Request sent to enable ILM on a deployment.
Fields
- index_patterns IndexPattern[] - A locally-unique user-specified id for Kibana
elasticsearch: EnterpriseSearch
Holds diagnostics for an Enterprise Search resource
Fields
- elasticsearch_cluster_ref_id string - The user-specified id of the Elasticsearch Cluster that this will link to
- backend_plan record {} - The backend plan as JSON
- display_name string - The human readable name (defaults to the generated cluster id if not specified)
- ref_id string - A locally-unique user-specified id
elasticsearch: EnterpriseSearchConfiguration
Fields
- user_settings_override_json record {}? - An arbitrary JSON object allowing ECE admins to set clusters' parameters (only one of this and 'user_settings_override_yaml' is allowed), i.e. in addition to the documented 'system_settings'. (This field together with 'system_settings' and 'user_settings*' defines the total set of Enterprise Search settings)
- user_settings_yaml string? - An arbitrary YAML object allowing (non-admin) cluster owners to set their parameters (only one of this and 'user_settings_json' is allowed), provided the parameters are on the allowlist and not on the denylist. (This field together with 'user_settings_override*' and 'system_settings' defines the total set of Enterprise Search settings)
- 'version string? - The version of the Enterprise Search cluster (must be one of the ECE supported versions, and won't work unless it matches the Elasticsearch version. Leave blank to auto-detect version.)
- user_settings_json record {}? - An arbitrary JSON object allowing (non-admin) cluster owners to set their parameters (only one of this and 'user_settings_yaml' is allowed), provided the parameters are on the allowlist and not on the denylist. (This field together with 'user_settings_override*' and 'system_settings' defines the total set of Enterprise Search settings)
- system_settings EnterpriseSearchSystemSettings? - This structure defines a curated subset of the Enterprise Search settings. (This field together with 'user_settings_override*' and 'user_settings*' defines the total set of Enterprise Search settings)
- user_settings_override_yaml string? - An arbitrary YAML object allowing ECE admins to set clusters' parameters (only one of this and 'user_settings_override_json' is allowed), i.e. in addition to the documented 'system_settings'. (This field together with 'system_settings' and 'user_settings*' defines the total set of Enterprise Search settings)
- docker_image string? - A docker URI that allows overriding of the default docker image specified for this version
elasticsearch: EnterpriseSearchInfo
The overview information for the Enterprise Search Server.
Fields
- status string - Enterprise Search status
- name string - The name of the Enterprise Search
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
- elasticsearch_cluster TargetElasticsearchCluster - Information about the specified Elasticsearch cluster.
- healthy boolean - Whether the Enterprise Search is healthy or not (one or more of the info subsections will have healthy: false)
- region string? - The region that this Enterprise Search belongs to. Only populated in SaaS or federated ECE.
- settings EnterpriseSearchSettings? - The settings for the Enterprise Search.
- plan_info EnterpriseSearchPlansInfo - Information about current, pending, and past Enterprise Search Server plans.
- external_links ExternalHyperlink[] - External resources related to the Enterprise Search
- deployment_id string? - The id of the deployment that this Enterprise Search belongs to.
- topology ClusterTopologyInfo - The topology for Elasticsearch clusters, multiple Kibana instances, or multiple APM Servers. The
ClusterTopologyInfo
also includes the instances and containers, and where they are located.
- id string - The id of the Enterprise Search
- metadata ClusterMetadataInfo? - Information about the public and internal state, and the configuration settings of an Elasticsearch cluster.
elasticsearch: EnterpriseSearchNodeTypes
Node types to enable for an Enterprise Search instance
Fields
- connector boolean - Defines whether this instance should run as Connector
- appserver boolean - Defines whether this instance should run as Application/API server
- 'worker boolean - Defines whether this instance should run as background worker
elasticsearch: EnterpriseSearchPayload
An Enterprise Search creation request paired with the alias of the Elasticsearch cluster it should be paired with
Fields
- elasticsearch_cluster_ref_id string - Alias to the Elasticsearch Cluster to attach Enterprise Search to
- display_name string? - The human readable name for the Enterprise Search cluster (default: takes the name of its Elasticsearch cluster)
- settings EnterpriseSearchSettings? - The settings for the Enterprise Search.
- region string - The region where this resource exists
- ref_id string - A locally-unique user-specified id for Enterprise Search
- plan EnterpriseSearchPlan - The plan for the Enterprise Search cluster.
elasticsearch: EnterpriseSearchPlan
The plan for the Enterprise Search cluster.
Fields
- cluster_topology EnterpriseSearchTopologyElement[]? -
- transient TransientEnterpriseSearchPlanConfiguration? - Defines configuration parameters that control how the plan (i.e. consisting of the cluster topology and Enterprise Search settings) is applied
- enterprise_search EnterpriseSearchConfiguration -
elasticsearch: EnterpriseSearchPlanControlConfiguration
Fields
- cluster_reboot string? - Set to 'forced' to force a reboot as part of the upgrade plan
- move_allocators AllocatorMoveRequest[]? -
- reallocate_instances boolean? - If true (default: false) does not allow re-using any existing instances currently in the cluster, i.e. even unchanged instances will be re-created
- preferred_allocators string[]? - List of allocators on which instances are placed if possible (if not possible/not specified then any available allocator with space is used)
- calm_wait_time int? - This timeout determines how long to give a cluster after it responds to API calls before performing actual operations on it. It defaults to 5s
- timeout int? - The total timeout in seconds after which the plan is cancelled even if it is not complete. Defaults to 4x the max memory capacity per node (in MB)
- extended_maintenance boolean? - If true (default false), does not clear the maintenance flag (which prevents its API from being accessed except by the constructor) on new instances added until after a snapshot has been restored, otherwise, the maintenance flag is cleared once the new instances successfully join the new cluster
- move_instances InstanceMoveRequest[]? -
elasticsearch: EnterpriseSearchPlanInfo
Information about the Enterprise Search Server plan.
Fields
- attempt_end_time string? - If this plan completed or failed (ie is not pending), when the attempt ended (ISO format in UTC)
- plan_attempt_id string? - A UUID for each plan attempt
- warnings ClusterPlanWarning[] -
- healthy boolean - Either the plan ended successfully, or is not yet completed (and no errors have occurred)
- plan_end_time string? - If this plan is not current or pending, when the plan was no longer active (ISO format in UTC)
- 'source ChangeSourceInfo? - A container for information about the source of a change.
- plan_attempt_log ClusterPlanStepInfo[] -
- plan EnterpriseSearchPlan? - The plan for the Enterprise Search cluster.
- plan_attempt_name string? - A human readable name for each plan attempt, only populated when retrieving plan histories
- attempt_start_time string? - When this plan attempt (ie to apply the plan to the Enterprise Search) started (ISO format in UTC)
elasticsearch: EnterpriseSearchPlansInfo
Information about current, pending, and past Enterprise Search Server plans.
Fields
- healthy boolean - Whether the plan situation is healthy (if unhealthy, means the last plan attempt failed)
- current EnterpriseSearchPlanInfo? - Information about the Enterprise Search Server plan.
- pending EnterpriseSearchPlanInfo? - Information about the Enterprise Search Server plan.
- history EnterpriseSearchPlanInfo[] -
elasticsearch: EnterpriseSearchResourceInfo
Describes an Enterprise Search resource belonging to a Deployment
Fields
- elasticsearch_cluster_ref_id string - The Elasticsearch cluster that this resource depends on.
- info EnterpriseSearchInfo - The overview information for the Enterprise Search Server.
- region string - The region where this resource exists
- id string - The randomly-generated id of a Resource
- ref_id string - The locally-unique user-specified id of a Resource
elasticsearch: EnterpriseSearchSettings
The settings for the Enterprise Search.
Fields
- metadata ClusterMetadataSettings? - The top-level configuration settings for the Elasticsearch cluster.
elasticsearch: EnterpriseSearchSubInfo
Information about the APM Servers associated with the Elasticsearch cluster.
Fields
- enterprise_search_id string - The Enterprise Search Id
- enabled boolean - Whether the associated Enterprise Search is currently available
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
elasticsearch: EnterpriseSearchSystemSettings
This structure defines a curated subset of the Enterprise Search settings. (This field together with 'user_settings_override*' and 'user_settings*' defines the total set of Enterprise Search settings)
Fields
- elasticsearch_password string? - Optionally override the account within Enterprise Search - defaults to a system account that always exists (if specified, the username must also be specified). Note that this field is never returned from the API, it is write only.
- elasticsearch_username string? - Optionally override the account within Enterprise Search - defaults to a system account that always exists (if specified, the password must also be specified). Note that this field is never returned from the API, it is write only.
- secret_session_key string? - Optionally override the secret session key within Enterprise Search - defaults to the previously existing secretSession. Note that this field is never returned from the API, it is write only.
- elasticsearch_url string? - Optionally override the URL to which to send data (for advanced users only, if unspecified the system selects an internal URL)
elasticsearch: EnterpriseSearchTopologyElement
Defines the topology of the Enterprise Search nodes (e.g. number/capacity of nodes, and where they can be allocated)
Fields
- node_count_per_zone record {}? -
- zone_count int? - number of zones in which nodes will be placed
- node_configuration string? -
- memory_per_node record {}? -
- enterprise_search EnterpriseSearchConfiguration? -
- node_type EnterpriseSearchNodeTypes? - Node types to enable for an Enterprise Search instance
- allocator_filter record {}? -
- instance_configuration_id string? - Controls the allocation of this topology element as well as allowed sizes and node_types. It needs to match the id of an existing instance configuration.
- size TopologySize? - Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
elasticsearch: ExistsQuery
Matches documents that have at least one non-null
value in the original field.
Fields
- 'field string - The field to check for non-null values in.
elasticsearch: Extension
An API extension. It represents clusters' plugins and bundles
Fields
- description string? - The extension description.
- url string - The extension URL to be used in the plan.
- extension_type string - The extension type.
- download_url string? - The download URL specified during extension creation.
- deployments string[]? - List of deployments using this extension. Up to only 10000 deployments will be included in the list.
- 'version string - The Elasticsearch version.
- file_metadata ExtensionFileMetadata? -
- id string - The extension ID
- name string - The extension name.
elasticsearch: ExtensionFileMetadata
Fields
- url string? - The temporary URL to download the extension file. Usable for verification.
- last_modified_date string? - The date and time the extension was last modified.
- size int? - The extension file size in bytes.
elasticsearch: Extensions
A collection of extensions
Fields
- extensions Extension[] - The list of extensions.
elasticsearch: ExtensionsExtensionIdBody
Fields
- file string - Zip file that contains the extension
elasticsearch: ExternalHyperlink
Fields
- uri string? - hyperlink to an external resource
- id string - Identifier of the external link
- label string - Human-readable description of the external link
elasticsearch: ExternalTrustRelationship
The trust relationship with external entities (remote environments, remote accounts...).
Fields
- trust_allowlist string[]? - The list of clusters to trust. Only used when
trust_all
is false.
- trust_relationship_id string - the ID of the external trust relationship
- trust_all boolean - If true, all clusters in this external entity will be trusted and the
trust_allowlist
is ignored.
elasticsearch: FilterAssociation
The association with a ruleset or user auth token for a deployment, template, or product.
Fields
- id string - ID of the entity, such as the deployment ID or Elasticsearch cluster ID.
- entity_type string - Type of the traffic filter ruleset association, such as 'deployment', 'cluster'
elasticsearch: GrowShrinkStrategyConfig
A strategy that creates instances with the new plan, migrates data from the old instances, then shuts down the old instances. GrowShrinkStrategyConfig
is safer than 'rolling' and ensures single node availability during a plan change, but can be a lot slower on larger clusters.
elasticsearch: Hyperlink
Fields
- need_elevated_permissions boolean? - Whether the operation requires elevated permissions (when the field is present, elevated permissions are required).
elasticsearch: IndexPattern
An index pattern described indicating how it has to be migrated to ILM.
Fields
- index_pattern string - Index pattern to which the ILM policy will be applied.
- node_attributes record {}? - Defines the Elasticsearch node attributes for the warm element of the topology
- policy_name string - Name of the policy to create
elasticsearch: InstanceConfigurationInfo
The configuration template for Elasticsearch instances, Kibana instances, and APM Servers.
Fields
- discrete_sizes DiscreteSizes - Instance sizes that are supported by the Elasticsearch instance, Kibana instance, or APM Server configuration.
- description string? - Optional description for the instance configuration
- node_types string[]? - Node types (master, data) for the instance
- storage_multiplier decimal? - Settings for the instance storage multiplier
- instance_type string - The type of instance (elasticsearch, kibana)
- cpu_multiplier decimal? - Settings for the instance CPU multiplier
- max_zones int? - The maximum number of availability zones in which this instance configuration has allocators. This field will be missing unless explicitly requested with the show_max_zones parameter.
- id string? - Unique identifier for the instance configuration
- name string - Display name for the instance configuration.
elasticsearch: InstanceMoveRequest
The request that specifies the Elasticsearch instances, Kibana instances, and APM Servers to move to allocators as part of the upgrade plan.
Fields
- to string[]? - An optional list of allocator ids to which the instance should be moved. If not specified then any available allocator can be used (including the current one if it is healthy)
- 'from string - The instance id that is going to be moved
- instance_down boolean? - Tells the infrastructure that the instance should be considered as permanently down when deciding how to migrate data to new nodes. If left blank then the system will automatically decide (currently: will treat the instances as up)
elasticsearch: IpFilteringSettings
The configuration settings for IP filtering.
Fields
- rulesets string[] - IDs of the IP filter rulesets
elasticsearch: ItemCosts
Detailed costs associated to an Elastic Cloud Resource for an organization. All of the costs are expressed in Elastic Consumption Unit (ECU).
Fields
- sku string - Stock Keeping Unit (SKU)
- kind string - Kind of resource
- name string - Resource name
- price decimal - Resource price
- period Period - Period
- instance_count int - Number of instances
- hours int - Resource usage in hours
- price_per_hour decimal - Price per hour
elasticsearch: ItemsCosts
Costs associated to a set of Elastic Cloud resources for an organization. All of the costs are expressed in Elastic Consumption Unit (ECU).
Fields
- costs Costs - Costs overview for an organization. All of the costs, credits, trials are expressed in Elastic Consumption Unit (ECU).
- resources ItemCosts[] - Costs of the list of resources
- data_transfer_and_storage DtsDimensionCosts[] - List of the detailed costs associated to the Data Transfer and Storage (DTS) dimensions
elasticsearch: KeystoreContents
The contents of the Elasticsearch keystore.
Fields
- secrets record {} - List of secrets
elasticsearch: KeystoreSecret
The value that you configure for the Elasticsearch keystore secret.
Fields
- as_file boolean? - Stores the keystore secret as a file. The default is false, which stores the keystore secret as string when value is a plain string, or true when value is an object.
- value record {}? - Value of this setting. This can either be a string or a JSON object that is stored as a JSON string in the keystore. NOTE: When the keystore secret is unspecified, it is removed.
elasticsearch: Kibana
Holds diagnostics for a Kibana resource
Fields
- elasticsearch_cluster_ref_id string - The user-specified id of the Elasticsearch Cluster that this will link to
- backend_plan record {} - The backend plan as JSON
- display_name string - The human readable name (defaults to the generated cluster id if not specified)
- ref_id string - A locally-unique user-specified id
elasticsearch: KibanaClusterInfo
The top-level object information for a Kibana instance.
Fields
- status string - Cluster status
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
- elasticsearch_cluster TargetElasticsearchCluster - Information about the specified Elasticsearch cluster.
- healthy boolean - Whether the cluster is healthy or not (one or more of the info subsections will have healthy: false)
- region string? - The region that this cluster belongs to. Only populated in SaaS or federated ECE.
- settings KibanaClusterSettings? - The settings for multiple Kibana instances.
- cluster_name string - The name of the cluster
- plan_info KibanaClusterPlansInfo - Information about the current, pending, or past Kibana instance plans.
- cluster_id string - The id of the cluster
- external_links ExternalHyperlink[] - External resources related to the cluster
- deployment_id string? - The id of the deployment to which this Kibana Server belongs to.
- topology ClusterTopologyInfo - The topology for Elasticsearch clusters, multiple Kibana instances, or multiple APM Servers. The
ClusterTopologyInfo
also includes the instances and containers, and where they are located.
- metadata ClusterMetadataInfo - Information about the public and internal state, and the configuration settings of an Elasticsearch cluster.
elasticsearch: KibanaClusterPlan
The plan for the Kibana instance.
Fields
- cluster_topology KibanaClusterTopologyElement[]? -
- transient TransientKibanaPlanConfiguration? - Defines the configuration parameters that control how the plan is applied. For example, the Elasticsearch cluster topology and Kibana instance settings.
- kibana KibanaConfiguration - The Kibana instance settings. When specified at the top level, provides a field-by-field default. When specified at the topology level, provides the override settings.
elasticsearch: KibanaClusterPlanInfo
Information about the current, pending, or past Kibana instance plan.
Fields
- attempt_end_time string? - If this plan completed or failed (ie is not pending), when the attempt ended (ISO format in UTC)
- plan_attempt_id string? - A UUID for each plan attempt
- warnings ClusterPlanWarning[] -
- healthy boolean - Either the plan ended successfully, or is not yet completed (and no errors have occurred)
- plan_end_time string? - If this plan is not current or pending, when the plan was no longer active (ISO format in UTC)
- 'source ChangeSourceInfo? - A container for information about the source of a change.
- plan_attempt_log ClusterPlanStepInfo[] -
- plan KibanaClusterPlan? - The plan for the Kibana instance.
- plan_attempt_name string? - A human readable name for each plan attempt, only populated when retrieving plan histories
- attempt_start_time string? - When this plan attempt (ie to apply the plan to the cluster) started (ISO format in UTC)
elasticsearch: KibanaClusterPlansInfo
Information about the current, pending, or past Kibana instance plans.
Fields
- healthy boolean - Whether the plan situation is healthy (if unhealthy, means the last plan attempt failed)
- current KibanaClusterPlanInfo? - Information about the current, pending, or past Kibana instance plan.
- pending KibanaClusterPlanInfo? - Information about the current, pending, or past Kibana instance plan.
- history KibanaClusterPlanInfo[] -
elasticsearch: KibanaClusterSettings
The settings for multiple Kibana instances.
Fields
- metadata ClusterMetadataSettings? - The top-level configuration settings for the Elasticsearch cluster.
elasticsearch: KibanaClusterTopologyElement
The topology of the Kibana nodes, including the number, capacity, and type of nodes, and where they can be allocated.
Fields
- instance_configuration_id string? - Controls the allocation of this topology element as well as allowed sizes and node_types. It needs to match the id of an existing instance configuration.
- zone_count int? - number of zones in which nodes will be placed
- kibana KibanaConfiguration? - The Kibana instance settings. When specified at the top level, provides a field-by-field default. When specified at the topology level, provides the override settings.
- size TopologySize? - Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
elasticsearch: KibanaConfiguration
The Kibana instance settings. When specified at the top level, provides a field-by-field default. When specified at the topology level, provides the override settings.
Fields
- user_settings_override_json record {}? - An arbitrary JSON object allowing ECE admins owners to set clusters' parameters (only one of this and 'user_settings_override_yaml' is allowed), ie in addition to the documented 'system_settings'. (This field together with 'system_settings' and 'user_settings*' defines the total set of Kibana settings)
- user_settings_yaml string? - An arbitrary YAML object allowing (non-admin) cluster owners to set their parameters (only one of this and 'user_settings_json' is allowed), provided the parameters are on the allowlist and not on the denylist. (These field together with 'user_settings_override*' and 'system_settings' defines the total set of Kibana settings)
- 'version string? - The version of the Kibana cluster (must be one of the ECE supported versions, and won't work unless it matches the Elasticsearch version. Leave blank to auto-detect version.)
- user_settings_json record {}? - An arbitrary JSON object allowing (non-admin) cluster owners to set their parameters (only one of this and 'user_settings_yaml' is allowed), provided the parameters are on the allowlist and not on the denylist. (This field together with 'user_settings_override*' and 'system_settings' defines the total set of Kibana settings)
- system_settings KibanaSystemSettings? - A subset of Kibana settings. TIP: To define the complete set of Elasticsearch settings, use
KibanaSystemSettings
withuser_settings_override_
anduser_settings_
.
- user_settings_override_yaml string? - An arbitrary YAML object allowing ECE admins owners to set clusters' parameters (only one of this and 'user_settings_override_json' is allowed), ie in addition to the documented 'system_settings'. (This field together with 'system_settings' and 'user_settings*' defines the total set of Kibana settings)
- docker_image string? - A docker URI that allows overriding of the default docker image specified for this version
elasticsearch: KibanaDeeplink
Embedded object that contains information for linking into a specific Kibana page configured via the template.
Fields
- semver string - Semver condition when to apply the URI.
- uri string - URI to which the user should be directed.
elasticsearch: KibanaPayload
A Kibana creation request paired with the alias of the Elasticsearch cluster it should be paired with
Fields
- elasticsearch_cluster_ref_id string - Alias to the Elasticsearch Cluster to attach Kibana to
- display_name string? - The human readable name for the Kibana cluster (default: takes the name of its Elasticsearch cluster)
- settings KibanaClusterSettings? - The settings for multiple Kibana instances.
- region string - The region where this resource exists
- ref_id string - A locally-unique user-specified id for Kibana
- plan KibanaClusterPlan - The plan for the Kibana instance.
elasticsearch: KibanaPlanControlConfiguration
The configuration settings for the timeout and fallback parameters.
Fields
- cluster_reboot string? - Set to 'forced' to force a reboot as part of the upgrade plan
- extended_maintenance boolean? - If true (default false), does not clear the maintenance flag (which prevents its API from being accessed except by the constructor) on new instances added until after a snapshot has been restored, otherwise, the maintenance flag is cleared once the new instances successfully join the new cluster
- calm_wait_time int? - This timeout determines how long to give a cluster after it responds to API calls before performing actual operations on it. It defaults to 5s
- timeout int? - The total timeout in seconds after which the plan is cancelled even if it is not complete. Defaults to 4x the max memory capacity per node (in MB)
elasticsearch: KibanaResourceInfo
Describes an Kibana resource belonging to a Deployment
Fields
- elasticsearch_cluster_ref_id string - The Elasticsearch cluster that this resource depends on.
- info KibanaClusterInfo - The top-level object information for a Kibana instance.
- region string - The region where this resource exists
- id string - The randomly-generated id of a Resource
- ref_id string - The locally-unique user-specified id of a Resource
elasticsearch: KibanaSubClusterInfo
Information about the Kibana instances associated with the Elasticsearch cluster.
Fields
- kibana_id string - The Kibana cluster Id
- enabled boolean - Whether the associated Kibana cluster is currently available
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
elasticsearch: KibanaSystemSettings
A subset of Kibana settings. TIP: To define the complete set of Elasticsearch settings, use KibanaSystemSettings
with user_settings_override_
and user_settings_
.
Fields
- elasticsearch_password string? - Optionally override the account within Elasticsearch - defaults to a system account that always exists (if specified, the username must also be specified). Note that this field is never returned from the API, it is write only.
- elasticsearch_username string? - Optionally override the account within Elasticsearch - defaults to a system account that always exists (if specified, the password must also be specified). Note that this field is never returned from the API, it is write only.
- elasticsearch_url string? - Optionally override the URL to which to send data (for advanced users only, if unspecified the system selects an internal URL)
elasticsearch: ManagedMonitoringSettings
The settings for sending monitoring information to another cluster.
Fields
- target_cluster_id string - The Id of the target cluster to which to send monitoring information
elasticsearch: MatchAllQuery
A query that matches all documents.
elasticsearch: MatchNoneQuery
A query that doesn't match any documents.
elasticsearch: MatchQuery
Consumes and analyzes text, numbers, and dates, then constructs a query.
Fields
- operator string? - The operator flag can be set to or or and to control the boolean clauses (defaults to or).
- query string - The text/numeric/date to query for.
- minimum_should_match int? - The minimum number of optional should clauses to match.
- analyzer string? - The analyzer that will be used to perform the analysis process on the text. Defaults to the analyzer that was used to index the field.
elasticsearch: MetadataItem
The key-value pair.
Fields
- value string - The metadata value
- 'key string - The metadata field name
elasticsearch: NestedQuery
A query that matches nested objects.
Fields
- query QueryContainer - The container for all of the allowed Elasticsearch queries. Specify only one property each time.
- score_mode string? - Allows to specify how inner children matching affects score of the parent. Refer to the Elasticsearch documentation for details.
- path string - The path to the nested object.
elasticsearch: ObservabilityIssue
Observability health issue
Fields
- description string - A user-friendly description of the observability health issue
- severity string - Severity of the health issue
elasticsearch: Orphaned
Details about orphaned resources.
Fields
- appsearch string[] - List of orphaned AppSearch resource ids
- elasticsearch OrphanedElasticsearch[] - List of orphaned Elasticsearch resources
- enterprise_search string[] - List of orphaned Enterprise Search resource ids
- kibana string[] - List of orphaned Kibana resource ids
- apm string[] - List of orphaned APM resource ids
elasticsearch: OrphanedElasticsearch
Details about an orphaned Elasticsearch resources.
Fields
- dependents ElasticsearchDependant[] - List of orphaned dependent resources
- id string - The id of the orphaned resource
elasticsearch: Period
Period
Fields
- 'start string - Start
- end string - End
elasticsearch: PlanStrategy
The options for performing a plan change. Specify only one property each time. The default is grow_and_shrink
.
Fields
- rolling RollingStrategyConfig? - Performs inline, rolling configuration changes that mutate existing containers. TIP: This is the fastest way to update a plan, but can fail for complex plan changes, such as topology changes. Also, this is less safe for configuration changes that leave a cluster in a non running state. NOTE: When you perform a major version upgrade, and 'group_by' is set to 'pass:macros[all]';, rolling is required.
- rolling_grow_and_shrink RollingGrowShrinkStrategyConfig? - A strategy that creates new Elasticsearch instances, Kibana instances, and APM Servers with the new plan, then migrates the node data to minimize the amount of spare capacity.
- grow_and_shrink GrowShrinkStrategyConfig? - A strategy that creates instances with the new plan, migrates data from the old instances, then shuts down the old instances.
GrowShrinkStrategyConfig
is safer than 'rolling' and ensures single node availability during a plan change, but can be a lot slower on larger clusters.
- autodetect AutodetectStrategyConfig? - A strategy that lets constructor choose the most optimal way to execute the plan.
elasticsearch: PrefixQuery
The query that matches documents with fields that contain terms with a specified, not analyzed, prefix.
Fields
- boost float? - An optional boost value to apply to the query.
- value string - The prefix to search for.
elasticsearch: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
elasticsearch: QueryContainer
The container for all of the allowed Elasticsearch queries. Specify only one property each time.
Fields
- match_all MatchAllQuery? - A query that matches all documents.
- exists ExistsQuery? - Matches documents that have at least one non-
null
value in the original field.
- term record {}? -
- nested NestedQuery? - A query that matches nested objects.
- prefix record {}? -
- bool BoolQuery? - A query for documents that match boolean combinations of other queries.
- query_string QueryStringQuery? - A query that uses the
SimpleQueryParser
for parsing.
- range record {}? -
- match_none MatchNoneQuery? - A query that doesn't match any documents.
- 'match record {}? -
elasticsearch: QueryStringQuery
A query that uses the SimpleQueryParser
for parsing.
Fields
- query string - The actual query to be parsed.
- default_operator string? - The default operator used if no explicit operator is specified.
- default_field string? - The default field for query terms if no prefix field is specified.
- allow_leading_wildcard boolean? - When set, * or ? are allowed as the first character. Defaults to false.
- analyzer string? - The analyzer used to analyze each term of the query when creating composite queries.
elasticsearch: RangeQuery
The query that matches documents with fields that contain terms within a specified range.
Fields
- gt record {}? - Greater-than
- gte record {}? - Greater-than or equal to
- format string? - Formatted dates will be parsed using the format specified on the date field by default, but it can be overridden by passing the format parameter.
- time_zone string? - Dates can be converted from another timezone to UTC either by specifying the time zone in the date value itself (if the format accepts it), or it can be specified as the time_zone parameter.
- lt record {}? - Less-than
- lte record {}? - Less-than or equal to.
- boost float? - An optional boost value to apply to the query.
elasticsearch: ReadOnlyRequest
Read-only mode request payload
Fields
- enabled boolean - Enabled or disabled read-only mode
elasticsearch: ReadOnlyResponse
Read-only mode response
Fields
- enabled boolean - Whether read-only mode is enabled or disabled
elasticsearch: RemoteResourceInfo
Information about a Remote Cluster.
Fields
- healthy boolean - Whether or not the remote cluster is healthy
- compatible boolean - Whether or not the remote cluster version is compatible with this cluster version.
- connected boolean - Whether or not there is at least one connection to the remote cluster.
- trusted boolean - Whether or not the remote cluster is trusted by this cluster.
- trusted_back boolean - Whether or not the remote cluster trusts this cluster back.
elasticsearch: RemoteResourceRef
The Elasticsearch resource used as a Remote Cluster.
Fields
- info RemoteResourceInfo? - Information about a Remote Cluster.
- elasticsearch_ref_id string - The locally-unique user-specified id of an Elasticsearch Resource
- deployment_id string - The id of the deployment
- skip_unavailable boolean? - If true, skip this cluster during search if it is disconnected. Default: false
- alias string - The alias for this remote cluster. Aliases must only contain letters, digits, dashes and underscores
elasticsearch: RemoteResources
The list of resources used as remote clusters
Fields
- resources RemoteResourceRef[] - The remote resources
elasticsearch: ReplyWarning
Fields
- message string? - A human readable message describing the warning that occurred
- code string - A structured code representing the error type that occurred
elasticsearch: RestoreSnapshotApiConfiguration
The configuration for the restore command, such as which indices you want to restore.
Fields
- indices string[]? - The list of indices to restore (supports +ve and -ve selection and wildcarding - see the default Elasticsearch index format documentation)
- raw_settings record {}? - This JSON object (merged with the 'indices' field (if present) is passed untouched into the restore command - see the Elasticsearch '_snapshot' documentation for more details on supported formats
elasticsearch: RestoreSnapshotConfiguration
Restores a snapshot from a local or remote repository.
Fields
- repository_config RestoreSnapshotRepoConfiguration? - Configures the location of a remote repository. The default is the system repository.
- repository_name string? - If specified, contains the name of the snapshot repository - else will default to the Elastic Cloud system repo ('found-snapshots')
- restore_payload RestoreSnapshotApiConfiguration? - The configuration for the restore command, such as which indices you want to restore.
- strategy string? - The restore strategy to use. Defaults to a full restore. Partial restore will attempt to restore unavailable indices only
- source_cluster_id string? - If specified, contains the name of the source cluster id. Do not send this if you are sending repository_config
- snapshot_name string - The name of the snapshot to restore. Use '__latest_success__' to get the most recent snapshot from the specified repository
elasticsearch: RestoreSnapshotRepoConfiguration
Configures the location of a remote repository. The default is the system repository.
Fields
- raw_settings record {}? - The remote snapshot settings raw JSON - see the Elasticsearch '_snapshot' documentation for more details on supported formats
elasticsearch: RollingGrowShrinkStrategyConfig
A strategy that creates new Elasticsearch instances, Kibana instances, and APM Servers with the new plan, then migrates the node data to minimize the amount of spare capacity.
elasticsearch: RollingStrategyConfig
Performs inline, rolling configuration changes that mutate existing containers. TIP: This is the fastest way to update a plan, but can fail for complex plan changes, such as topology changes. Also, this is less safe for configuration changes that leave a cluster in a non running state. NOTE: When you perform a major version upgrade, and 'group_by' is set to 'pass:macros[all]';, rolling is required.
Fields
- skip_synced_flush boolean? - Whether to skip attempting to do a synced flush on the filesystem of the container (default: false), which is less safe but may be required if the container is unhealthy
- allow_inline_resize boolean? - Whether we allow changing the capacity of instances (default false). This is currently implemented by stopping, re-creating then starting the affected instance on its associated allocator when performing the changes. NOTES: This requires a round-trip through the allocation infrastructure of the active constructor, as it has to reserve the target capacity without over-committing
- group_by string? - Specifies the grouping attribute to use when rolling several instances. Instances that share the same value for the provided attribute key are rolled together as a unit. Examples that make sense to use are '__all__' (roll all instances as a single unit), 'logical_zone_name' (roll instances by zone), '__name__' (roll one instance at a time, the default if not specified). Note that '__all__' is required when performing a major version upgrade
- shard_init_wait_time int? - The time, in seconds, to wait for shards that show no progress of initializing before rolling the next group (default: 10 minutes)
elasticsearch: RulesetAssociations
The configuration settings for the traffic filter.
Fields
- associations FilterAssociation[] - List of associations
- total_associations int - Total number of associations. This includes associations the user does not have permission to view.
elasticsearch: SearchRequest
An Elasticsearch search request with a subset of options.
Fields
- sort record {}[]? - An array of fields to sort the search results by.
- query QueryContainer? - The container for all of the allowed Elasticsearch queries. Specify only one property each time.
- 'from int? -
- size int? - The maximum number of search results to return.
elasticsearch: ServiceUrl
A URL to access the service of a resource
Fields
- url string - The full URL to access the service
- 'service string - Name of the service
elasticsearch: SimplifiedLineItem
Line Item
Fields
- 'start string - Start of the line item's validity
- ecu_balance decimal - Elastic Consumption Unit (ECU) Balance
- end string - Expiration of the line item
- id string - Line Item ID
- ecu_quantity decimal - Original Elastic Consumption Unit (ECU) quantity
elasticsearch: SnapshotStatusInfo
Information about the snapshot status for the Elasticsearch cluster. For example, the health status.
Fields
- count int - Number of snapshots stored for this cluster
- scheduled_time string? - Scheduled time of next snapshot attempt
- latest_end_time string? - The end time of the most recently attempted snapshot
- healthy boolean - Health status of snapshots for this cluster
- latest_successful boolean? - Latest snapshot status
- recent_success boolean - Indicates whether the cluster has a relatively recent successful snapshot.
- latest_successful_end_time string? - The end time of the most recently successful snapshot
- latest_status string? - Status of the latest snapshot attempt, if any exist.
elasticsearch: StackVersionApmConfig
The APM Server configuration for an Elastic Stack version.
Fields
- settings record {}? - Settings that are applied to all nodes of this type
- blacklist string[] - List of configuration options that cannot be overridden by user settings
- 'version string? - Version of APM
- capacity_constraints StackVersionInstanceCapacityConstraint? - The Elasticsearch instance, Kibana instance, APM Server capacity constraints for an Elastic Stack node type.
- compatible_node_types string[]? - Node types that are compatible with this one
- docker_image string - Docker image for the APM
elasticsearch: StackVersionAppSearchConfig
AppSearch related configuration of an Elastic Stack version
Fields
- settings record {}? - Settings that are applied to all nodes of this type
- node_types StackVersionNodeType[]? - Node types that are supported by this stack version
- blacklist string[] - List of configuration options that cannot be overridden by user settings
- 'version string? - Version of AppSearch
- capacity_constraints StackVersionInstanceCapacityConstraint? - The Elasticsearch instance, Kibana instance, APM Server capacity constraints for an Elastic Stack node type.
- compatible_node_types string[]? - Node types that are compatible with this one
- docker_image string - Docker image for the AppSearch
elasticsearch: StackVersionConfig
The details for an Elastic Stack configuration.
Fields
- accessible boolean? - Whether or not this version is accessible by the calling user. This is only relevant in EC (SaaS) and is not sent in ECE.
- min_upgradable_from string? - The minimum version recommended to upgrade this version.
- deleted boolean? - Identifies that the Elastic Stack version is marked for deletion
- whitelisted boolean? - Whether or not this version is whitelisted. This is only relevant in EC (SaaS) and is not sent in ECE.
- kibana StackVersionKibanaConfig - The Kibana configuration for an Elastic Stack version.
- upgradable_to string[] - Stack Versions that this version can upgrade to
- 'version string? - Stack version
- elasticsearch StackVersionElasticsearchConfig - The Elasticsearch configuration for an Elastic Stack version.
- template StackVersionTemplateInfo - The template information for an Elastic Stack version.
- apm StackVersionApmConfig? - The APM Server configuration for an Elastic Stack version.
- appsearch StackVersionAppSearchConfig? - AppSearch related configuration of an Elastic Stack version
- metadata StackVersionMetadata? - The metadata for the Elastic Stack.
elasticsearch: StackVersionConfigs
The details for multiple Elastic Stack configurations.
Fields
- stacks StackVersionConfig[] -
elasticsearch: StackVersionElasticsearchConfig
The Elasticsearch configuration for an Elastic Stack version.
Fields
- settings record {}? - Settings that are applied to all nodes of this type
- node_types StackVersionNodeType[]? - Node types that are supported by this stack version
- blacklist string[] - List of configuration options that cannot be overridden by user settings
- plugins string[] - List of available plugins
- capacity_constraints StackVersionInstanceCapacityConstraint? - The Elasticsearch instance, Kibana instance, APM Server capacity constraints for an Elastic Stack node type.
- compatible_node_types string[]? - Node types that are compatible with this one
- default_plugins string[] - List of default plugins
- docker_image string - Docker image for the Elasticsearch
elasticsearch: StackVersionInstanceCapacityConstraint
The Elasticsearch instance, Kibana instance, APM Server capacity constraints for an Elastic Stack node type.
Fields
- max int - Max capacity of the instances
- min int - Min capacity of the instances
elasticsearch: StackVersionKibanaConfig
The Kibana configuration for an Elastic Stack version.
Fields
- settings record {}? - Settings that are applied to all nodes of this type
- blacklist string[] - List of configuration options that cannot be overridden by user settings
- 'version string? - Version of Kibana
- capacity_constraints StackVersionInstanceCapacityConstraint? - The Elasticsearch instance, Kibana instance, APM Server capacity constraints for an Elastic Stack node type.
- compatible_node_types string[]? - Node types that are compatible with this one
- docker_image string - Docker image for the kibana
elasticsearch: StackVersionMetadata
The metadata for the Elastic Stack.
Fields
- pre_release boolean? - Indicates that the stack pack version is not GA and is not supposed to be used in production
- min_wire_compatibility_version string? - The minimum version required for performing a rolling upgrade to this stack version.
- schema_version int? - The schema version of the stack pack version
- min_index_compatibility_version string? - The minimum version required for performing a full cluster restart upgrade to this stack version.
- min_platform_version string? - The minimum version of the platform that the stack pack version is compatible with
- notes string? - Notes for administrator
elasticsearch: StackVersionNodeType
The configuration for an Elastic Stack node type.
Fields
- mandatory boolean? - Flag to specify a node type is mandatory in a cluster's plan
- name string - Name of the node type
- settings record {}? - Settings that are applied to all nodes of this type
- node_type string - Type of the node (master, data, ...)
- capacity_constraints StackVersionInstanceCapacityConstraint? - The Elasticsearch instance, Kibana instance, APM Server capacity constraints for an Elastic Stack node type.
- compatible_node_types string[]? - Node types that are compatible with this one
- description string - Description of the node type
elasticsearch: StackVersionTemplateFileHash
The template file hash for an Elastic Stack version.
Fields
- path string - File path relative to template's root
- hash string - SHA-256 hash of a file
elasticsearch: StackVersionTemplateInfo
The template information for an Elastic Stack version.
Fields
- hashes StackVersionTemplateFileHash[]? - Relative paths of files with SHA-256 hashes that contains the template
- template_version string? - Template version
elasticsearch: TargetElasticsearchCluster
Information about the specified Elasticsearch cluster.
Fields
- elasticsearch_id string - The Elasticsearch cluster Id
- links record {}? - A map of application-specific operations (which map to 'operationId's in the Swagger API) to metadata about that operation
elasticsearch: TermQuery
A query for documents that contain the specified term in the inverted index.
Fields
- value record {} - The exact value to query for.
elasticsearch: TopologyElementControl
Controls for the topology element. Only used as part of the deployment template. Ignored if included as part of a deployment.
Fields
- min TopologySize - Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
elasticsearch: TopologySize
Measured by the amount of a resource. The final cluster size is calculated using multipliers from the topology instance configuration.
Fields
- 'resource string - Type of resource
- value int - Amount of resource
elasticsearch: TrafficFilterEgressRule
The rule detail for a traffic filter egress rule.
Fields
- protocol string - The target protocol for an egress rule
- target string - Allowed traffic filter egress target: IP address or CIDR mask
- ports int[]? - A list of target ports for an egress rule
elasticsearch: TrafficFilterRule
The container for a traffic filter rule.
Fields
- azure_endpoint_name string? - Name of the Azure Private Endpoint to allow connections from
- egress_rule TrafficFilterEgressRule? - The rule detail for a traffic filter egress rule.
- description string? - Description of the rule
- 'source string? - Allowed traffic filter source: IP address, CIDR mask, or VPC endpoint ID
- azure_endpoint_guid string? - Resource GUID of the Azure Private Endpoint to allow connections from
- id string? - The rule ID
elasticsearch: TrafficFilterRulesetInfo
The container for a set of traffic filter rules.
Fields
- associations FilterAssociation[]? - List of associations. Returned only when include_associations query parameter is true
- description string? - Description of the ruleset
- rules TrafficFilterRule[] - List of rules
- region string - The ruleset can be attached only to deployments in the specific region
- include_by_default boolean - Should the ruleset be automatically included in the new deployments
- total_associations int? - Total number of associations. This includes associations the user does not have permission to view.Returned only when include_associations query parameter is true
- 'type string - Type of the ruleset
- id string - The ruleset ID
- name string - Name of the ruleset
elasticsearch: TrafficFilterRulesetRequest
The specification for traffic filter ruleset.
Fields
- name string - Name of the ruleset
- rules TrafficFilterRule[] - List of rules
- region string - The ruleset can be attached only to deployments in the specific region
- include_by_default boolean - Should the ruleset be automatically included in the new deployments
- 'type string - Type of the ruleset
- description string? - Description of the ruleset
elasticsearch: TrafficFilterRulesetResponse
The response after you create a new ruleset.
Fields
- id string - The new ruleset ID
elasticsearch: TrafficFilterRulesets
The container for a set of traffic filter rulesets.
Fields
- rulesets TrafficFilterRulesetInfo[] - List of traffic filter rules
elasticsearch: TrafficFilterSettings
The configuration settings for the traffic filter.
Fields
- rulesets string[] - IDs of the traffic filter rulesets
elasticsearch: TransientApmPlanConfiguration
Defines the configuration parameters that control how the plan is applied. For example, the Elasticsearch cluster topology and APM Server settings.
Fields
- plan_configuration ApmPlanControlConfiguration? - The plan control configuration options for the APM Server.
- strategy PlanStrategy? - The options for performing a plan change. Specify only one property each time. The default is
grow_and_shrink
.
elasticsearch: TransientAppSearchPlanConfiguration
Defines configuration parameters that control how the plan (ie consisting of the cluster topology and AppSearch settings) is applied
Fields
- plan_configuration AppSearchPlanControlConfiguration? -
- strategy PlanStrategy? - The options for performing a plan change. Specify only one property each time. The default is
grow_and_shrink
.
elasticsearch: TransientElasticsearchPlanConfiguration
Defines the configuration parameters that control how the plan is applied. For example, the Elasticsearch cluster topology and Elasticsearch settings.
Fields
- plan_configuration ElasticsearchPlanControlConfiguration? - The configuration settings for the timeout and fallback parameters.
- cluster_settings_json record {}? - If specified, contains transient settings to be applied to an Elasticsearch cluster during changes, default values shown below applied.
These can be overridden by specifying them in the map (or null to unset). Additional settings can also be set. Settings will be cleared after the plan has finished. If not specified, no settings will be applied.
NOTE: These settings are only explicitly cleared for 5.x+ clusters, they must be hand-reset to their defaults in 2.x- (or a cluster reboot will clear them).
- indices.store.throttle.max_bytes_per_sec: 150Mb
- indices.recovery.max_bytes_per_sec: 150Mb
- cluster.routing.allocation.cluster_concurrent_rebalance: 10
- cluster.routing.allocation.node_initial_primaries_recoveries: 8
- cluster.routing.allocation.node_concurrent_incoming_recoveries: 8
- remote_clusters RemoteResources? - The list of resources used as remote clusters
- restore_snapshot RestoreSnapshotConfiguration? - Restores a snapshot from a local or remote repository.
- strategy PlanStrategy? - The options for performing a plan change. Specify only one property each time. The default is
grow_and_shrink
.
elasticsearch: TransientEnterpriseSearchPlanConfiguration
Defines configuration parameters that control how the plan (i.e. consisting of the cluster topology and Enterprise Search settings) is applied
Fields
- plan_configuration EnterpriseSearchPlanControlConfiguration? -
- strategy PlanStrategy? - The options for performing a plan change. Specify only one property each time. The default is
grow_and_shrink
.
elasticsearch: TransientKibanaPlanConfiguration
Defines the configuration parameters that control how the plan is applied. For example, the Elasticsearch cluster topology and Kibana instance settings.
Fields
- plan_configuration KibanaPlanControlConfiguration? - The configuration settings for the timeout and fallback parameters.
- strategy PlanStrategy? - The options for performing a plan change. Specify only one property each time. The default is
grow_and_shrink
.
elasticsearch: UpdateExtensionRequest
The body of a request to update an extension
Fields
- 'version string - The Elasticsearch version.
- extension_type string - The extension type.
- name string - The extension name.
- download_url string? - The URL to download the extension archive.
- description string? - The extension description.
elasticsearch: Updates
Holds diagnostics for existing resources that might be updated
Fields
- appsearch AppSearch[]? - Diagnostics for AppSearches
- elasticsearch Elasticsearch[]? - Diagnostics for Elasticsearch clusters
- enterprise_search EnterpriseSearch[]? - Diagnostics for Enterprise Search resources
- kibana Kibana[]? - Diagnostics for Kibanas
- apm Apm[]? - Diagnostics for APMs
Import
import ballerinax/elasticsearch;
Metadata
Released date: about 1 year ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 2
Weekly downloads
Keywords
Business Intelligence/Analytics
Cost/Paid
Contributors