docusign.rooms
Module docusign.rooms
API
Definitions
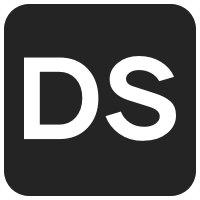
ballerinax/docusign.rooms Ballerina library
Overview
This is a generated connector for DocuSign Rooms API OpenAPI specification.
DocuSign Rooms streamlines real estate and mortgage workflows by connecting all parties in a digital, secure, and central workspace. With the DocuSign Rooms API, it’s easy to integrate Rooms functionality into your own solutions.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a DocuSign account.
- Obtain tokens by following this guide.
Quickstart
To use the DocuSign Rooms connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/docusign.rooms
module into the Ballerina project.
import ballerinax/docusign.rooms;
Step 2 - Create a new connector instance
You can now make the connection configuration using the <ACCESS_TOKEN>.
rooms:ClientConfig clientConfig = { auth : { token: `<ACCESS_TOKEN>` } }; rooms:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to get account information using the connector.
public function main() { string accountID = "1234"; rooms:AccountSummary|error result = baseClient->getAccountInformation(accountID); if (result is rooms:AccountSummary) { log:printInfo(result.toString()); } else { log:printInfo(result.toString()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
docusign.rooms: Client
This is a generated connector for DocuSign Rooms API OpenAPI specification. DocuSign Rooms streamlines real estate and mortgage workflows by connecting all parties in a digital, secure, and central workspace. With the DocuSign Rooms API, it’s easy to integrate Rooms functionality into your own solutions.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a DocuSign account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://rooms.docusign.com/restapi" - URL of the target service
getAccountInformation
function getAccountInformation(string accountId) returns AccountSummary|error
Gets account information.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
Return Type
- AccountSummary|error - Account information successfully retrieved
getDocument
function getDocument(int documentId, string accountId, boolean includeContents) returns Document|error
Gets information about or the contents of a document.
Parameters
- documentId int - (Required) The id of the document.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- includeContents boolean (default false) - (Optional) When set to true, includes the contents of the document in the
base64Contents
property of the response. The default value is false.
deleteDocument
Deletes a specified document.
Parameters
- documentId int - The ID of the document.
- accountId string - The globally unique identifier (GUID) for the account.
createUserAccessToDocument
function createUserAccessToDocument(int documentId, string accountId, DocumentUserForCreate payload) returns DocumentUser|error
Grants a user access to a document.
Parameters
- documentId int - The ID of the document.
- accountId string - The globally unique identifier (GUID) for the account.
- payload DocumentUserForCreate - User information to grant access
Return Type
- DocumentUser|error - User successfully granted access to document.
getEsignPermissionProfiles
function getEsignPermissionProfiles(string accountId) returns ESignPermissionProfileList|error
Gets eSignature Permission Profiles.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
Return Type
- ESignPermissionProfileList|error - Permission profiles successfully retrieved.
createExternalFormFillSession
function createExternalFormFillSession(string accountId, ExternalFormFillSessionForCreate payload) returns ExternalFormFillSession|error
Creates an external form fill session.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload ExternalFormFillSessionForCreate - Details required to create a form fill session.
Return Type
- ExternalFormFillSession|error - Success
getFieldSet
function getFieldSet(string fieldSetId, string accountId, string[]? fieldsCustomDataFilters) returns FieldSet|error
Gets a field set.
Parameters
- fieldSetId string - (Required) The id of the field set. Example:
4aef602b-xxxx-xxxx-xxxx-08d76696f678
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- fieldsCustomDataFilters string[]? (default ()) - (Optional) An comma-separated list that limits the fields to return: -
IsRequiredOnCreate
: include fields that are required in room creation. -IsRequiredOnSubmit
: include fields that are required when submitting a room for review.
getFormGroups
function getFormGroups(string accountId, int count, int startPosition) returns FormGroupSummaryList|error
Gets form groups.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
- startPosition int (default 0) - (Optional) The starting zero-based index position of the results set. The default value is
0
.
Return Type
- FormGroupSummaryList|error - Successfully retrieved Form Groups.
createFormGroup
function createFormGroup(string accountId, FormGroupForCreate payload) returns FormGroup|error
Creates a form group.
Parameters
- accountId string - The globally unique identifier (GUID) for the account.
- payload FormGroupForCreate - Request object for FormGroup::CreateFormGroup.
getFormGroup
Gets a form group.
Parameters
- formGroupId string - The ID of the form group. Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- accountId string - The globally unique identifier (GUID) for the account.
renameFormGroup
function renameFormGroup(string formGroupId, string accountId, FormGroupForUpdate payload) returns FormGroup|error
Renames a form group.
Parameters
- formGroupId string - The ID of the form group. Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- accountId string - The globally unique identifier (GUID) for the account.
- payload FormGroupForUpdate - Details about a form group
deleteFormgroup
Deletes a form group.
Parameters
- formGroupId string - The ID of the form group. Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- accountId string - The globally unique identifier (GUID) for the account.
removeFormInFormGroup
function removeFormInFormGroup(string formGroupId, string formId, string accountId) returns Response|error
Removes a form from a form group.
Parameters
- formGroupId string - The ID of the form group. Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- formId string - The id of the form. Example:
5be324eb-xxxx-xxxx-xxxx-208065181be9
- accountId string - The globally unique identifier (GUID) for the account.
assignFormToFormGroup
function assignFormToFormGroup(string formGroupId, string accountId, FormGroupFormToAssign payload) returns FormGroupFormToAssign|error
Assigns a form to a form group.
Parameters
- formGroupId string - The ID of the form group. Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- accountId string - The globally unique identifier (GUID) for the account.
- payload FormGroupFormToAssign - Group information to assign form
Return Type
- FormGroupFormToAssign|error - Successfully assigned form to form group.
grantOfficeAccessToFormGroup
function grantOfficeAccessToFormGroup(string formGroupId, int officeId, string accountId) returns Response|error
Grants an office access to a form group.
Parameters
- formGroupId string - The ID of the form group. Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- officeId int - The id of the office. This is the id that the system generated when you created the office.
- accountId string - The globally unique identifier (GUID) for the account.
revokeOfficeAccessFromFormGroup
function revokeOfficeAccessFromFormGroup(string formGroupId, int officeId, string accountId) returns Response|error
Revoke an office's access to a form group.
Parameters
- formGroupId string - The ID of the form group. Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- officeId int - The id of the office. This is the id that the system generated when you created the office.
- accountId string - The globally unique identifier (GUID) for the account.
getFormLibraries
function getFormLibraries(string accountId, int count, int startPosition) returns FormLibrarySummaryList|error
Gets form libraries.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
- startPosition int (default 0) - (Optional) The starting zero-based index position of the results set. The default value is
0
.
Return Type
- FormLibrarySummaryList|error - Successfully retrieved Form Libraries.
getFormsInFormLibraryForms
function getFormsInFormLibraryForms(string formLibraryId, string accountId, int count, int startPosition) returns FormSummaryList|error
Gets the forms in a form library.
Parameters
- formLibraryId string - (Required) The id of the form library. Example:
402c6e2f-xxxx-xxxx-xxxx-ff3f249f6da9
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
- startPosition int (default 0) - (Optional) The starting zero-based index position of the results set. The default value is
0
.
Return Type
- FormSummaryList|error - Successfully retrieved library
getFormDetails
function getFormDetails(string formId, string accountId) returns FormDetails|error
Gets form details.
Parameters
- formId string - (Required) The id of the form.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
Return Type
- FormDetails|error - Form based on FormId
getCountries
function getCountries() returns GlobalCountries|error
Gets countries.
Return Type
- GlobalCountries|error - Request was successful.
getClosingStatuses
function getClosingStatuses() returns GlobalClosingStatuses|error
Gets closing statuses.
Return Type
- GlobalClosingStatuses|error - Request was successful.
getContactSides
function getContactSides() returns GlobalContactSides|error
Gets contact sides.
Return Type
- GlobalContactSides|error - Request was successful.
getFinancingTypes
function getFinancingTypes() returns GlobalFinancingTypes|error
Gets financing types.
Return Type
- GlobalFinancingTypes|error - Request was successful.
getOriginsOfLeads
function getOriginsOfLeads() returns GlobalOriginsOfLeads|error
Gets origins of leads.
Return Type
- GlobalOriginsOfLeads|error - Request was successful.
getPropertyTypes
function getPropertyTypes() returns GlobalPropertyTypes|error
Gets property types.
Return Type
- GlobalPropertyTypes|error - Request was successful.
getRoomContactTypes
function getRoomContactTypes() returns GlobalRoomContactTypes|error
Gets room contact types.
Return Type
- GlobalRoomContactTypes|error - Request was successful.
getSellerDecisionTypes
function getSellerDecisionTypes() returns GlobalSellerDecisionTypes|error
Gets seller decision types.
Return Type
- GlobalSellerDecisionTypes|error - Request was successful.
getSpecialCircumstanceTypes
function getSpecialCircumstanceTypes() returns GlobalSpecialCircumstanceTypes|error
Gets special circumstance types.
Return Type
- GlobalSpecialCircumstanceTypes|error - Request was successful.
getTaskDateTypes
function getTaskDateTypes() returns GlobalTaskDateTypes|error
Gets task date types.
Return Type
- GlobalTaskDateTypes|error - Request was successful.
getTaskResponsibilityTypes
function getTaskResponsibilityTypes() returns GlobalTaskResponsibilityTypes|error
Gets task responsibility types.
Return Type
- GlobalTaskResponsibilityTypes|error - Request was successful.
getTaskStatuses
function getTaskStatuses() returns GlobalTaskStatuses|error
Retrieves the list of valid task statuses.
Return Type
- GlobalTaskStatuses|error - Request was successful.
getTransactionSides
function getTransactionSides() returns GlobalTransactionSides|error
Gets transaction sides.
Return Type
- GlobalTransactionSides|error - Request was successful.
getStates
function getStates() returns GlobalStates|error
Gets states.
Return Type
- GlobalStates|error - Request was successful.
getCurrencies
function getCurrencies() returns GlobalCurrencies|error
Gets valid currencies.
Return Type
- GlobalCurrencies|error - Request was successful.
getTimeZones
function getTimeZones() returns GlobalTimeZones|error
Gets time zones.
Return Type
- GlobalTimeZones|error - Request was successful.
getOffices
function getOffices(string accountId, int count, int startPosition, boolean onlyAccessible, string? search) returns OfficeSummaryList|error
Gets offices.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
- startPosition int (default 0) - (Optional) The starting zero-based index position of the results set from which to begin returning values. The default value is
0
.
- onlyAccessible boolean (default false) - (Optional) When set to true, the response only includes the offices that are accessible to the current user.
- search string? (default ()) - (Optional) Filters returned records by the specified string. The response only includes records containing this string in the office
name
field.
Return Type
- OfficeSummaryList|error - Offices successfully retrieved.
createOffice
function createOffice(string accountId, OfficeForCreate payload) returns Office|error
Creates an office.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload OfficeForCreate - Details about the office that you want to create.
getOffice
Gets information about an office.
Parameters
- officeId int - (Required) The id of the office.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
deleteOffice
Deletes an office.
Parameters
- officeId int - (Required) The id of the office.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
getReferenceCounts
function getReferenceCounts(int officeId, string accountId) returns OfficeReferenceCountList|error
Retrieves the number and type of objects that reference an office.
Parameters
- officeId int - (Required) The id of the office.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
Return Type
- OfficeReferenceCountList|error - Reference counts successfully retrieved.
getRegions
function getRegions(string accountId, int count, int startPosition, boolean managedOnly) returns RegionSummaryList|error
Gets regions.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
- startPosition int (default 0) - (Optional) The starting zero-based index position of the results set from which to begin returning values. The default value is
0
.
- managedOnly boolean (default false) - (Optional) When set to true, only the regions that the current user manages are returned. The default value is false.
Return Type
- RegionSummaryList|error - Regions successfully retrieved.
createRegion
Creates a region.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload Region - Information about a region.
getRegion
Gets information about a region.
Parameters
- regionId int - (Required) The id of the region.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
deleteRegion
Deletes a region.
Parameters
- regionId int - (Required) The id of the region.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
getRegionReferenceCounts
function getRegionReferenceCounts(int regionId, string accountId) returns RegionReferenceCountList|error
Retrieves the number and type of objects that reference a region.
Parameters
- regionId int - (Required) The id of the region.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
Return Type
- RegionReferenceCountList|error - Reference counts successfully retrieved.
getRoles
function getRoles(string accountId, boolean onlyAssignable, string? filter, int startPosition, int count) returns RoleSummaryList|error
Gets roles.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- onlyAssignable boolean (default false) - (Optional) When set to true, returns only the roles that the current user can assign to someone else. The default value is false.
- filter string? (default ()) - (Optional) A search filter that returns roles by the beginning of the role name. You can enter the beginning of the role name only to return all of the roles that begin with the text that you entered. For example, if your company has set up roles such as Manager Beginner, Manager Pro, Agent Expert, and Agent Superstar, you could enter
Manager
to return all of the Manager roles (Manager Beginner and Manager Pro). Note: You do not enter a wildcard (*) at the end of the name fragment.
- startPosition int (default 0) - (Optional) The starting zero-based index position of the result set. The default value is 0.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
Return Type
- RoleSummaryList|error - Roles successfully retrieved.
createRole
function createRole(string accountId, RoleForCreate payload) returns Role|error
Creates a role.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload RoleForCreate - Details about the role that you want to create.
getRole
Gets a role.
Parameters
- roleId int - (Required) The id of the role.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- includeIsAssigned boolean (default false) - (Optional) When set to true, the response includes the
isAssigned
property, which specifies whether the role is currently assigned to any users. The default is false.
updateRole
function updateRole(int roleId, string accountId, RoleForUpdate payload) returns Role|error
Updates a role.
Parameters
- roleId int - (Required) The id of the role to update.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload RoleForUpdate - The details to use for the update.
deleteRole
Deletes a role.
Parameters
- roleId int - (Required) The id of the role to delete.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
getRoomFieldData
Gets a room's field data.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
updateRoomFieldData
function updateRoomFieldData(int roomId, string accountId, FieldDataForUpdate payload) returns FieldData|error
Updates a room's field data.
Parameters
- roomId int - The id of the room.
- accountId string - The globally unique identifier (GUID) for the account.
- payload FieldDataForUpdate - The field data to update. When updating field data, specify only the fields being updated.
getRoomFolders
function getRoomFolders(int roomId, string accountId, int startPosition, int count) returns RoomFolderList|error
Gets a list of room folders accessible to the current user.
Parameters
- roomId int - The room id from which to retrieve folders.
- accountId string - The globally unique identifier (GUID) for the account.
- startPosition int (default 0) - Position of the first item in the total results. Defaults to 0.
- count int (default 100) - Number of room folders to return. Defaults to the maximum which is 100.
Return Type
- RoomFolderList|error - Room folders successfully retrieved.
addFormToRoom
function addFormToRoom(int roomId, string accountId, FormForAdd payload) returns RoomDocument|error
Adds a form to a room.
Parameters
- roomId int - (Required) The id of the room to which you want to add the form.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload FormForAdd - Details about the form that you want to add.
Return Type
- RoomDocument|error - Success
getRoomUsers
function getRoomUsers(int roomId, string accountId, int count, int startPosition, string? filter, string? sort) returns RoomUsersResult|error
Gets a room's users.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
- startPosition int (default 0) - (Optional) The index position within the total result set from which to start returning values. The default value is
0
.
- filter string? (default ()) - (Optional) A search filter that returns users by the beginning of the user's first name, last name, or email address. You can enter the beginning of the name or email only to return all of the users whose names or email addresses begin with the text that you entered. Note: You do not enter a wildcard (*) at the end of the name or email fragment.
- sort string? (default ()) - (Optional) The order in which to return results. Valid values are: -
firstNameAsc
: Sort on first name in ascending order. -firstNameDesc
: Sort on first name in descending order. -lastNameAsc
: Sort on last name in ascending order. -lastNameDesc
: Sort on last name in descending order. This is the default value.
Return Type
- RoomUsersResult|error - The room user was successfully retrieved.
inviteUserToRoom
function inviteUserToRoom(int roomId, string accountId, RoomInvite payload) returns RoomInviteResponse|error
Invites a user to a room.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload RoomInvite - The information to use for the invitation.
Return Type
- RoomInviteResponse|error - Success
putRoomUser
function putRoomUser(int roomId, int userId, string accountId, RoomUserForUpdate payload) returns RoomUser|error
Updates a room user.
Parameters
- roomId int - (Required) The id of the room.
- userId int - (Required) The id of the user to update.
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- payload RoomUserForUpdate - This request object contains the information that you want to update for the room user.
revokeRoomUserAccess
function revokeRoomUserAccess(int roomId, int userId, string accountId, RoomUserRemovalDetail payload) returns Response|error
Revokes the specified user's access to the room.
Parameters
- roomId int - The room Id to revoke access from
- userId int - The user Id getting revoked from the room
- accountId string - The globally unique identifier (GUID) for the account.
- payload RoomUserRemovalDetail - Details for removal.
restoreRoomUserAccess
Restores the specified user's access to the room.
Parameters
- roomId int - The room Id to restore access
- userId int - The user Id getting restored to the room
- accountId string - The globally unique identifier (GUID) for the account.
getRooms
function getRooms(string accountId, int count, int startPosition, string? roomStatus, int? officeId, string? fieldDataChangedStartDate, string? fieldDataChangedEndDate, string? roomClosedStartDate, string? roomClosedEndDate) returns RoomSummaryList|error
Returns a list of rooms.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- count int (default 100) - (Optional) The number of results. When this property is used as a request parameter specifying the number of results to return, the value must be a number between 1 and 100 (default).
- startPosition int (default 0) - (Optional) The index position within the total result set from which to start returning values. The default value is
0
.
- roomStatus string? (default ()) - (Optional) The status of the room. Valid values are: -
active
: This is the default value. -pending
-open
: Includes bothactive
andpending
statuses. -closed
To return rooms with multiple statuses, enter a comma-separated list of statuses. Example:closed,open
- officeId int? (default ()) - (Optional) The id of the office.
- fieldDataChangedStartDate string? (default ()) - (Optional) Starting date and time to filter rooms whose field data has changed after this date. Date and time is always given as UTC. If the time (
hh:mm:ss
) is omitted, it defaults to00:00:00
. Valid formats: -yyyy-mm-dd hh:mm:ss
-yyyy/mm/dd hh:mm:ss
The default start date is the beginning of time.
- fieldDataChangedEndDate string? (default ()) - (Optional) Ending date and time to filter rooms whose field data has changed before this date. Date and time is always given as UTC. If the time (
hh:mm:ss
) is omitted, it defaults to00:00:00
. Valid formats: -yyyy-mm-dd hh:mm:ss
-yyyy/mm/dd hh:mm:ss
If this query parameter is omitted, the default end date is now.
- roomClosedStartDate string? (default ()) - (Optional) Starting date and time to filter rooms that were closed this date. Date and time is always given as UTC. If the time (
hh:mm:ss
) is omitted, it defaults to00:00:00
. Valid formats: -yyyy-mm-dd hh:mm:ss
-yyyy/mm/dd hh:mm:ss
The default start date is the beginning of time.
- roomClosedEndDate string? (default ()) - (Optional) Ending date and time to filter rooms that were closed before this date. Date and time is always given as UTC. If the time (
hh:mm:ss
) is omitted, it defaults to00:00:00
. Valid formats: -yyyy-mm-dd hh:mm:ss
-yyyy/mm/dd hh:mm:ss
If this query parameter is omitted, the default end date is now.
Return Type
- RoomSummaryList|error - Rooms successfully retrieved.
createRoom
function createRoom(string accountId, RoomForCreate payload) returns Room|error
Creates a room.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload RoomForCreate - Details about the new room.
getRoom
Gets a room.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- includeFieldData boolean (default false) - (Optional) When set to true, the response includes the field data from the room. This is the information that appears on the room's Details tab.
deleteRoom
Deletes a room.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
roomsGetAssignableRoles
function roomsGetAssignableRoles(int roomId, string accountId, string? assigneeEmail, string? filter, int startPosition, int count) returns AssignableRoles|error
Gets assignable room-level roles in v6.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- assigneeEmail string? (default ()) - (Optional) The email address of a specific member. Using this parameter returns only the roles that the current user can assign to the member with that email address.
- filter string? (default ()) - (Optional) A search filter that returns assignable roles by the beginning of the role name. Note: You do not enter a wildcard (*) at the end of the name fragment.
- startPosition int (default 0) - (Optional) The index position within the total result set from which to start returning values. The default value is
0
.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
Return Type
- AssignableRoles|error - Assignable roles successfully retrieved.
roomsGetDocuments
function roomsGetDocuments(int roomId, string accountId, int count, int startPosition) returns RoomDocumentList|error
Gets a list of documents in a room.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
- startPosition int (default 0) - (Optional) The index position within the total result set from which to start returning values. The default value is
0
.
Return Type
- RoomDocumentList|error - Documents successfully retrieved.
addDocumentToroom
function addDocumentToroom(int roomId, string accountId, Document payload) returns RoomDocument|error
Adds a document to a room.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload Document - Contains information about a document.
Return Type
- RoomDocument|error - Document successfully added.
addDocumentToRoomViaFileUpload
function addDocumentToRoomViaFileUpload(int roomId, string accountId, DocumentsContentsBody payload) returns RoomDocument|error
Uploads the contents of a file as a document to a room.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload DocumentsContentsBody - Document Content
Return Type
- RoomDocument|error - Document successfully added.
updatePicture
function updatePicture(int roomId, string accountId, RoomidPictureBody payload) returns RoomPicture|error
Updates the picture for a room.
Parameters
- roomId int - (Required) The id of the room for which you are updating the picture.
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- payload RoomidPictureBody - Image content
Return Type
- RoomPicture|error - Picture successfully updated.
getRoomFieldSet
Gets the field set for a room.
Parameters
- roomId int - (Required) The globally unique identifier (GUID) for the account.
- accountId string - (Required) The id of the account.
getTaskLists
function getTaskLists(int roomId, string accountId) returns TaskListSummaryList|error
Gets task lists for a room.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The id of the account.
Return Type
- TaskListSummaryList|error - Task lists successfully retrieved.
createTaskList
function createTaskList(int roomId, string accountId, TaskListForCreate payload) returns TaskList|error
Applies a task list to a room.
Parameters
- roomId int - (Required) The id of the room.
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- payload TaskListForCreate - Information about the task list template to use to create the new task list.
getRoomTemplates
function getRoomTemplates(string accountId, int? officeId, boolean onlyAssignable, boolean onlyEnabled, int count, int startPosition) returns RoomTemplatesSummaryList|error
Gets room templates.
Parameters
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- officeId int? (default ()) - (Optional) The ID of the office for which the user wants to create a room. When you pass in a value for this parameter, only room templates that are valid for that office appear in the results. For users who are not Admins, the default is the id of the user's default office. However, you can specify a value if the user belongs to multiple offices. If the user is an Admin, set the
forAdmin
search parameter to true instead and omit theofficeId
parameter.
- onlyAssignable boolean (default false) - (Optional) When set to true, returns only the roles that the current user can assign to someone else. The default value is false.
- onlyEnabled boolean (default true) - When set to true, only returns room templates that are not disabled.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
- startPosition int (default 0) - (Optional) The index position within the total result set from which to start returning values. The default value is
0
.
Return Type
- RoomTemplatesSummaryList|error - Successfully retrieved room templates for the caller
deleteTaskList
Deletes a task list from a room.
Parameters
- taskListId int - The id of the task list.
- accountId string - The globally unique identifier (GUID) for the account.
getTaskListTemplates
function getTaskListTemplates(string accountId, int startPosition, int count) returns TaskListTemplateList|error
Gets task list templates.
Parameters
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- startPosition int (default 0) - (Optional) The starting zero-based index position from which to start returning values. The default is
0
.
- count int (default 100) - (Optional) The number of results to return. This value must be a number between
1
and100
(default).
Return Type
- TaskListTemplateList|error - Successfully returned list of task list
getUsers
function getUsers(string accountId, string? filter, string? sort, int? defaultOfficeId, string? accessLevel, int? titleId, int? roleId, string? status, boolean? lockedOnly, int startPosition, int count) returns UserSummaryList|error
Gets a list of users.
Parameters
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- filter string? (default ()) - (Optional) Filters results by name and email address. This is a "starts with" filter, which means that you can enter only the beginning of a name or email address. Note: You do not use a wildcard with this filter.
- sort string? (default ()) - (Optional) Specifies how to sort the results. Valid values are: -
FirstNameAsc
-LastNameAsc
-EmailAsc
-FirstNameDesc
-LastNameDesc
-EmailDesc
- defaultOfficeId int? (default ()) - (Optional) Filters for users who have this office id as their default office id.
- accessLevel string? (default ()) - (Optional) Filters for users who have the specified access level. A user's access level and role determine the types of resources and actions that are available to them. Valid values are: - Company: Users with this access level can administer resources across the company. - Region: Users with this access level can administer offices and other resources within their regions. - Office: Users with this access level can administer resources within their offices. - Contributor: Users with this access level can only administer their own resources. Note: In requests, the values that you may use for this property depend on your permissions and whether you can add users at your access level or lower. This property applies only to Rooms Version 6.
- titleId int? (default ()) - (Optional) For Rooms Version 5 only, filters for users whose managers have the specified
titleId
.
- roleId int? (default ()) - (Optional) For Rooms Version 6 only, filters for users who have the specified
roleId
.
- status string? (default ()) - (Optional) Filters for users who have the specified
status
. Valid values are: -Active
: The user is active. -Pending
: The user has been invited but has not yet accepted the invitation.
- lockedOnly boolean? (default ()) - (Optional) When set to true, filters for users whose accounts are locked.
- startPosition int (default 0) - (Optional) The starting zero-based index position within the result set from which to begin the response. The default is
0
.
- count int (default 100) - (Optional) The maximum number of users to return in the response. This value must be a number between
1
and100
(default).
Return Type
- UserSummaryList|error - Users information successfully retrieved.
getUser
Gets a user.
Parameters
- userId int - (Required) The id of the user.
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
updateUser
function updateUser(int userId, string accountId, UserForUpdate payload) returns User|error
Updates a user's default office.
Parameters
- userId int - (Required) The id of the user.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload UserForUpdate - This request object contains the information to use to update a user's default office.
removeUser
Removes a user from a company account.
Parameters
- userId int - (Required) The id of the user.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
inviteUserToAccount
function inviteUserToAccount(string accountId, UserToInvite payload) returns User|error
Invites a user to a v6 company account.
Parameters
- accountId string - (Required) The id of the account.
- payload UserToInvite - Information about the user that you are inviting.
inviteClassicAdmin
function inviteClassicAdmin(string accountId, ClassicAdminToInvite payload) returns User|error
Invites a user to a v5 company account as an Admin.
Parameters
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- payload ClassicAdminToInvite - Details about the person who you want to invite.
inviteclassicManager
function inviteclassicManager(string accountId, ClassicManagerToInvite payload) returns User|error
Invites a user to a v5 company account as a Manager.
Parameters
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- payload ClassicManagerToInvite - Details about the person who you want to invite.
inviteClassicAgent
function inviteClassicAgent(string accountId, ClassicAgentToInvite payload) returns User|error
Invites a user to a v5 company account as an Agent.
Parameters
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- payload ClassicAgentToInvite - Details about the person who you want to invite.
reinviteUser
Reinvites a user to join a company account.
Parameters
- userId int - (Required) The id of the user.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
addUserToOffice
function addUserToOffice(int userId, string accountId, DesignatedOffice payload) returns Response|error
Adds a user to an office.
Parameters
- userId int - (Required) The id of the user.
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- payload DesignatedOffice - Information about the office that you want to add a member to or remove a member from.
removeUserFromOffice
function removeUserFromOffice(int userId, string accountId, DesignatedOffice payload) returns Response|error
Removes a user from an office.
Parameters
- userId int - (Required) The id of the user.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload DesignatedOffice - Information about the office that you want to add a member to or remove a member from.
addUserToRegion
function addUserToRegion(int userId, string accountId, DesignatedRegion payload) returns Response|error
Adds a user to a region.
Parameters
- userId int - (Required) The id of the user.
- accountId string - (Required) The globally-unique identifier (GUID) for the account.
- payload DesignatedRegion - Information about the region associated with the member.
removeUserFromRegion
function removeUserFromRegion(int userId, string accountId, DesignatedRegion payload) returns Response|error
Removes a user from a region.
Parameters
- userId int - (Required) The id of the user.
- accountId string - (Required) The globally unique identifier (GUID) for the account.
- payload DesignatedRegion - Information about the region associated with the member.
lockUser
function lockUser(int userId, string accountId, LockedOutDetails payload) returns Response|error
Locks a user's account.
Parameters
- userId int - User Id of the user attempting to be locked.
- accountId string - The globally unique identifier (GUID) for the account.
- payload LockedOutDetails - Details about a locked account.
unlockUser
Unlocks a user's account.
Records
docusign.rooms: Accounts
Information about accounts.
Fields
- companyId int? - The id of the company.
- name string? - The name of the office.
- companyVersion ProductVersion? - Product version
- docuSignAccountGuid string? - The globally-unique identifier (GUID) for the DocuSign Rooms account.
- defaultFieldSetId string? - The id of the company's default field set. A field set is a set of data fields and the information about those data fields that the system uses to configure rooms. It corresponds to the Admin > Company > Room Details area in the console.
- requireOfficeLibraryAssignments boolean? - Require office library assignments
docusign.rooms: AccountSummary
Contains details about a company account.
Fields
- companyId int? - The id of the company.
- name string? - The name of the company.
- companyVersion ProductVersion? - Product version
- docuSignAccountGuid string? - The globally-unique identifier (GUID) for the DocuSign Rooms account.
- defaultFieldSetId string? - The id of the company's default field set. A field set is a set of data fields and the information about those data fields that the system uses to configure rooms. It corresponds to the Admin > Company > Room Details area in the console.
- requireOfficeLibraryAssignments boolean? - Require office library assignments
docusign.rooms: ActivityType
Details about an activity type.
Fields
- activityTypeId string? - The id of the activity type.
Example:
movefrominbx
- name string? - The name of the activity type.
Example:
Document Moved from Inbox
docusign.rooms: ApiError
This object describes errors that occur. It is valid only for responses and ignored in requests.
Fields
- errorCode string? - The code associated with the error condition.
- message string? - A brief message describing the error condition.
docusign.rooms: AssignableRoles
This complex type contains information about assignable roles.
Fields
- currentRoleId int? - The id of the invitee's company-level role. This property lets the requester know what room-level role will give the user the same permissions that they have at the company level. A value is returned only when both the requester and the invitee are internal to the company.
- roles RoleSummary[]? - An array of
role
objects.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: ClassicAdminToInvite
This request object contains details about the person who you want to invite.
Fields
- firstName string - (Required) The user's first name.
- lastName string - (Required) The user's last name.
- email string - (Required) The user's email address.
docusign.rooms: ClassicAgentToInvite
This request object contains details about the person who you want to invite.
Fields
- firstName string - (Required) The user's first name.
- lastName string - (Required) The user's last name.
- email string - (Required) The user's email address.
- officeId int - (Required) The id of the office. This is the id that the system generated when you created the office.
- companyTypeId string? - Company type ID
- eSignPermissionProfileId string? - (Required) When an administrator or authorized member invites a new user to become an account member, the system also creates an eSignature account for the invitee at the same time. The
eSignPermissionProfileId
is the id of the eSignature permission set to assign to the member.
docusign.rooms: ClassicManagerPermissions
This object contains details about user permissions. These permissions are associated only with Rooms v5.
Fields
- isVisibleInTransactionRooms boolean? - When set to true, the user is visible in company rooms. Note: Inherited managers are automatically added to rooms and are visible in those rooms unless this setting is set to false. Inherited managers are users who oversee others and have the auto-access to rooms of members the user manages permission enabled.
- canDeleteCompanyRooms boolean? - When set to true, the user can delete company rooms.
- canDeleteCompanyDocuments boolean? - When set to true, the user can delete company documents.
- canManageCompanyRooms boolean? - When set to true, the user can manage company rooms.
- canManageCompanyAccount boolean? - When set to true, the user can manage the company's account.
- canManageCompanySharedLibrary boolean? - When set to true, the user can manage the company's shared library.
- canManageCompanyMembers boolean? - When set to true, the user can manage other users on the company's account.
- canCloseCompanyRooms boolean? - When set to true, the user can close company rooms.
- canApproveCompanyChecklists boolean? - When set to true, the user can approve company checklists.
- isCompanySystemAdmin boolean? - When set to true, the user is a system administrator for the company.
- isRegionManager boolean? - When set to true, the user is a region manager.
- isOfficeManager boolean? - When set to true, the user is an office manager.
- autoAccessToCompanyRooms boolean? - When set to true, the user is automatically added to new company rooms and is visible in those rooms.
docusign.rooms: ClassicManagerToInvite
This request object contains details about the person who you want to invite.
Fields
- firstName string - (Required) The user's first name.
- lastName string - (Required) The user's last name.
- email string - (Required) The user's email address.
- defaultOfficeId int - (Required) The id of the user's default office.
- titleId int - (Required) In Rooms v5, this is the id of the custom job title for a Manager role within your company. For example, your company might have the custom job titles "Transaction Coordinator" and "Office Manager". Note: If you are using Rooms v6, use the Users::InviteUser method with the roleId property instead.
- accessLevel AccessLevel - Access level
- permissions ClassicManagerPermissions - This object contains details about user permissions. These permissions are associated only with Rooms v5.
- offices int[]? - An array of office ids for the offices to which the user belongs.
Note: You only specify the
offices
property when the user'saccessLevel
isoffice
.
- regions int[]? - An array of region ids for the regions to which the user belongs.
Note: You only specify the
regions
property when the user'saccessLevel
isregion
.
- eSignPermissionProfileId string? - (Required) When an administrator or authorized member invites a new user to become an account member, the system also creates an eSignature account for the invitee at the same time. The eSignPermissionProfileId is the id of the eSignature permission set to assign to the member.
docusign.rooms: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
docusign.rooms: ClosingStatus
Contains information about a closing status, or reason for closing a room.
Fields
- closingStatusId string? - The id of the closing status.
Example:
exp
- name string? - The name of the closing status.
Example:
Listing Expired
docusign.rooms: ClosingStatuses
This resource provides a method that enables you retrieve a list of closing statuses, or valid reasons for closing a room.
Fields
- closingStatusId string? - The id of the closing status.
Example:
exp
- name string? - The name of the office.
docusign.rooms: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
docusign.rooms: ContactSide
Details about a contact side.
Fields
- contactSideId string? - The id of the contact side.
Example:
L
- name string? - The name of the contact side.
Example:
Listing
docusign.rooms: ContactSides
The ContactSides
resource provides a method that enables you to retrieve a list of valid values for transaction contact sides.
Fields
- contactSideId string? - The id of the contact side.
Example:
L
- name string? - The name of the office.
docusign.rooms: Countries
The Countries
resource provide a method that enables you to retrieve a list of countries in which you can create an office.
Fields
- countryId string? - The two-letter country code of the office address (for example, "UK" for United Kingdom).
- name string? - The name of the office.
docusign.rooms: Country
Contains details about a supported country.
Fields
- countryId string? - The two-letter code for the country.
Example:
NZ
- name string? - The name of the country.
Example:
New Zealand
docusign.rooms: Currencies
The Currencies
method provides a resource that enables you to retrieve a list currencies that you can use for listing, offer, and loan amounts.
Fields
- currencyId string? - The three-letter code for the currency.
Example:
CAD
- name string? - The name of the office.
docusign.rooms: Currency
Contains details about a supported currency.
Fields
- currencyId string? - The three-letter code for the currency.
Example:
CAD
- name string? - The name of the currency.
Example:
Canadian Dollar
docusign.rooms: CustomData
Contains information about whether the field is required when a room is created or submitted for review.
Fields
- isRequiredOnCreate boolean? - When set to true, the field is required when a new room is created.
- isRequiredOnSubmit boolean? - When set to true, the field is required when a room is submitted for review.
docusign.rooms: DependsOn
Contains information about a parent field on which this field depends.
Fields
- actionType string? - The child field action that depends on the parent field.
Example:
visibility
For example, in the console, you must select a country for a list of states to become visible.
- parentApiName string? - The name that the Rooms API uses for the parent field.
Example:
country
docusign.rooms: DesignatedOffice
This object contains information about the office that you want to add a member to or remove a member from.
Fields
- officeId int - (Required) The id of the office. This is the id that the system generated when you created the office.
docusign.rooms: DesignatedRegion
This object contains information about the region associated with the member.
Fields
- regionId int - (Required) The id of the region. This is the id that the system generated when you created the region.
docusign.rooms: Document
Contains information about a document.
Fields
- documentId int? - The ID of the document.
- name string - The file name associated with the document.
- roomId int? - The id of the room associated with the document.
- ownerId int? - The id of the user who owns the document.
- size int? - The size of the document in bytes.
- folderId int? - The id of the folder that holds the document.
- createdDate string? - The date and time when the document was created. This is a read-only value that the service assigns.
Example:
2019-11-11T17:15:14.82
- isSigned boolean? - When set to true, indicates that the document is signed.
- contentType string? - Content type
- base64Contents string - The base64-encoded contents of the document. This property is only included in the response when you use the
includeContents
query parameter and set it to true.
docusign.rooms: DocumentsContentsBody
Fields
- file string? - Document
docusign.rooms: DocumentUser
Fields
- userId int? - The id of the user.
- documentId int? - The ID of the document.
- name string? - The file name associated with the document.
- hasAccess boolean? - True if the user
userId
has access to this document.
- canApproveTask boolean? - True if the user
userId
has can approve a task for this document.
- canAssignToTaskList boolean? - True if the user
userId
can assign this document to a task list.
- canCopy boolean? - True if the user
userId
can make a copy of this document.
- canDelete boolean? - True if the user
userId
can delete this document.
- canRemoveFromTaskList boolean? - True if the user
userId
can remove this document from a task list.
- canRemoveApproval boolean? - True if the user
userId
can remove approval for this document.
- canRename boolean? - True if the user
userId
can rename this document.
- canShare boolean? - True if the user
userId
can share this document.
- canViewActivity boolean? - True if the user
userId
can view activity on this document.
docusign.rooms: DocumentUserForCreate
User information to grant access
Fields
- userId int - The id of the user.
docusign.rooms: ESignAccountRoleSettings
This object contains details about the permissions associated with a eSignature permission profile.
Fields
- allowAccountManagement boolean? - A Boolean specifying whether users with this eSignature permission profile can manage the Rooms account. This property is read only and has the following values:
DS Admin
permission profile: trueDS Sender
permission profile: falseDS Viewer
permission profile: false
docusign.rooms: ESignPermissionProfile
When an administrator or authorized member invites a new user to become an account member, the system also creates an eSignature account for the invitee at the same time. This object contains information about the eSignature permission profile, which controls member access to the eSignature account.
Fields
- eSignPermissionProfileId string? - When an administrator or authorized member invites a new user to become an account member, the system also creates an eSignature account for the invitee at the same time. The
eSignPermissionProfileId
is the id of the eSignature permission set to assign to the member.
- name string? - The name of the eSignature permission profile. Valid values are:
- settings ESignAccountRoleSettings? - This object contains details about the permissions associated with a eSignature permission profile.
docusign.rooms: ESignPermissionProfileList
This object contains a list of eSignature permission profiles.
Fields
- permissionProfiles ESignPermissionProfile[]? - An array of eSignature
permissionProfile
objects.
docusign.rooms: ESignPermissionProfiles
When you create or invite a new member in Rooms, the system creates an eSignature account for the member at the same time.
Fields
- eSignPermissionProfileId string? - When an administrator or authorized member invites a new user to become an account member, the system also creates an eSignature account for the invitee at the same time. The
eSignPermissionProfileId
is the id of the eSignature permission set to assign to the member.
- name string? - The name of the eSignature permission profile.
- settings ESignAccountRoleSettings? - This object contains details about the permissions associated with a eSignature permission profile.
docusign.rooms: ExternalFormFillSession
Contains the URL for the new form fill session.
Fields
- url string? - The URL for the new form fill session.
docusign.rooms: ExternalFormFillSessionForCreate
Contains the details required to create a form fill session.
Fields
- formId string - (Required) The id of the form.
Example:
5be324eb-xxxx-xxxx-xxxx-208065181be9
- roomId int - (Required) The id of the room.
- xFrameAllowedUrl string? - (Optional) This property specifies the origin on which the page is allowed to display in a frame.
docusign.rooms: ExternalFormFillSessions
This resource provides a method that returns a URL for a new external form fill session, based on the roomId
and formId
that you specify in the formFillSessionForCreate
request body.
Fields
- url string? - URL for a new external form fill session
docusign.rooms: Field
Contains details about a field in a field set.
Fields
- fieldId string? - An id that uniquely identifies the instance of a
fieldDefinition
within a field set.
- fieldDefinitionId string? - The id of the DocuSign field definition from which this field derives. When an Admin user configures a field set by using the API, this is the id that they use to add this field definition to the field set. The original field definition associated with this id contains more information about the field, such as the default title, default API name, and configurations such as the maximum length or the maximum value allowed.
- title string? - The human-readable title or name of the field.
Example:
Company contact name
- apiName string? - The name that the Rooms API uses for the field.
Example:
companyContactName
Note: When you create a new room, you use theapiName
values for fields to specify the details that you want to appear on the room's Details tab.
- 'type string? - The type of field. The valid values are:
Date
Text
Checkbox
Currency
Numeric
SelectList
TextArea
Percentage
Integer
- fields Field[]? - An array of subfields.
- configuration FieldConfiguration? - Contains details about how a field is configured.
- customData CustomData? - Contains information about whether the field is required when a room is created or submitted for review.
docusign.rooms: FieldConfiguration
Contains details about how a field is configured.
Fields
- maxValue decimal? - The maximum value allowed for the field.
- minValue decimal? - The minimum value allowed for the field.
- multipleOf decimal? - This property is used for validation. When you set a number value for this property, the value for the field must be a multiple of it.
- maxLength int? - The maximum length of the field.
- minLength int? - The minimum length of the field.
- pattern string? - The regular expression pattern to use to validate the value of the field.
- isPublisher boolean? - When set to true, the field is a parent field on which one or more child fields depend.
- dependsOn DependsOn[]? - This property applies to child fields. It contains information about the parent field that must have a value set in order for this field to have a value. For example, you must specify a country before you can select a state.
- options SelectListFieldOption[]? - An array of options in a list.
docusign.rooms: FieldData
The field data associated with a room. See Rooms::GetRoomFieldData.
Fields
- data record {}? - Field data is a collection of name/value pairs where the names correspond to the fields in the room's Details tab. The value of name/value object can be a field data collection itself. These collections are implemented as JSON objects.
For example, the field data for fields named "Tax annual amount" and "buyer1" might look like this:
{ "data": { "taxAnnualAmount": 3389.12, "buyer1": { "email": "lizzy@example.com", "country": "England", "businessPhone": null, "name": "Elizabeth Bennet", "homePhone": null, "city": "Meryton", "address2": null, "postalCode": null, "state": "Hertfordshire", "company": null, "cellPhone": null, "address1": "Longbourn Estate" } } }
docusign.rooms: FieldDataForCreate
Contains key-value pairs that specify the properties of the room and their values.
Fields
- data record {}? - Field data is a collection of name/value pairs where the names correspond to the fields in the room's Details tab. The value of name/value object can be a field data collection itself. These collections are implemented as JSON objects.
For example, the field data for fields named "Tax annual amount" and "buyer1" might look like this:
{ "data": { "taxAnnualAmount": 3389.12, "buyer1": { "email": "lizzy@example.com", "country": "England", "businessPhone": null, "name": "Elizabeth Bennet", "homePhone": null, "city": "Meryton", "address2": null, "postalCode": null, "state": "Hertfordshire", "company": null, "cellPhone": null, "address1": "Longbourn Estate" } } }
docusign.rooms: FieldDataForUpdate
The field data to update. When updating field data, specify only the fields being updated.
Fields
- data record {}? - Field data is a collection of name/value pairs where the names correspond to the fields in the room's Details tab. The value of name/value object can be a field data collection itself. These collections are implemented as JSON objects.
For example, the field data for fields named "Tax annual amount" and "buyer1" might look like this:
{ "data": { "taxAnnualAmount": 3389.12, "buyer1": { "email": "lizzy@example.com", "country": "England", "businessPhone": null, "name": "Elizabeth Bennet", "homePhone": null, "city": "Meryton", "address2": null, "postalCode": null, "state": "Hertfordshire", "company": null, "cellPhone": null, "address1": "Longbourn Estate" } } }
docusign.rooms: Fields
The fields resource provides a method that enables you to retrieve a specific field set. This is a set of fields that can appear on a room's Details tab.
Fields
- fieldId string? - The id of the field.
Example:
10318d28-xxxx-xxxx-xxxx-d3df664f602c
- fieldDefinitionId string? - The id of the DocuSign field definition from which this field derives. When an Admin user configures a field set by using the API, this is the id that they use to add this field definition to the field set. The original field definition associated with this id contains more information about the field, such as the default title, default API name, and configurations such as the maximum length or the maximum value allowed.
- title string? - Title of the fields
- apiName string? - The name that the Rooms API uses for the field.
Example:
companyContactName
Note: When you create a new room, you use theapiName
values for fields to specify the details that you want to appear on the room's Details tab.
- 'type string? - Type of the fields
- fields Field[]? - An array of fields.
- configuration FieldConfiguration? - Contains details about how a field is configured.
- customData CustomData? - Contains information about whether the field is required when a room is created or submitted for review.
docusign.rooms: FieldSet
Contains details about a field set.
Fields
- fieldSetId string? - The id of the field set.
Example:
4aef602b-xxxx-xxxx-xxxx-08d76696f678
- title string? - The title or name of the field set.
- fields Field[]? - An array of fields.
docusign.rooms: FinancingType
Contains information about a financing type.
Fields
- financingTypeId string? - The id of the financing type.
Example:
conv
(forConventional
)
- name string? - The name of the financing type. Possible values are:
Cash
Conventional
FHA
VA
USDA
Bitcoin
Other
docusign.rooms: FinancingTypes
This resource provides a method that enables you to retrieve a list of financing types.
Fields
- financingTypeId string? - The id of the financing type.
Example:
conv
(forConventional
)
- name string? - The name of the office.
docusign.rooms: FormDetails
This resource contains details about a form, such as the date it was created and last updated, the number of pages, the form owner, and other information.
Fields
- formId string? - The id of the form.
Example:
5be324eb-xxxx-xxxx-xxxx-208065181be9
- name string? - The name of the form.
Example:
MORe Private Network Addendum
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
- lastUpdatedDate string? - The UTC date and time when the item was last updated. This is a read-only value that the service assigns. Example: 2019-07-17T17:45:42.783Z
- availableOnDate string? - The UTC DateTime when the form was made available or published. (It is possible for a form to be published but not yet available.)
- ownerName string? - The name of the organization that owns the form.
Example:
Mainstreet organization of Realtors
- 'version string? - The version of the form.
Example:
1
- numberOfPages int? - The number of pages in the form.
Example:
2
docusign.rooms: FormForAdd
Contains details about the form that you want to add.
Fields
- formId string - (Required) The id of the form.
docusign.rooms: FormGroup
Result from getting a form group.
Fields
- formGroupId string? - The ID of the form group.
Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- name string? - The name of the office.
- officeIds int[]? - An array of office IDs.
- forms GroupForm[]? - A list of forms.
docusign.rooms: FormGroupForCreate
Request object for FormGroup::CreateFormGroup.
Fields
- name string - The name of the group.
docusign.rooms: FormGroupFormToAssign
Group information to assign form
Fields
- formId string - The id of the form.
Example:
5be324eb-xxxx-xxxx-xxxx-208065181be9
- isRequired boolean? - True if the form is required.
docusign.rooms: FormGroupForUpdate
Fields
- name string - The name of the office.
docusign.rooms: FormGroups
The FormGroups
resource enables you to create and manage custom groups of association forms.
Fields
- formGroupId string? - The ID of the form group.
Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- name string? - The name of the office.
- formCount int? - The number of forms in the form library.
Example:
50
docusign.rooms: FormGroupSummary
Contains details about a form group.
Fields
- formGroupId string? - The ID of the form group.
Example:
7b879c89-xxxx-xxxx-xxxx-819d6a85e0a1
- name string? - The name of the form group.
Example:
Apartment Rental
- formCount int? - The number of forms in the form group.
Example:
10
docusign.rooms: FormGroupSummaryList
Contains a list of form groups.
Fields
- formGroups FormGroupSummary[]? - A list of form groups.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: FormLibraries
The FormLibraries
resource enables you to access standard real estate industry association forms and add them to rooms.
Fields
- formsLibraryId string? - The id of the form library.
Example:
402c6e2f-xxxx-xxxx-xxxx-ff3f249f6da9
- name string? - The name of the office.
- formCount int? - The number of forms in the form library.
Example:
50
docusign.rooms: FormLibrarySummary
Contains details about a specific form library.
Fields
- formsLibraryId string? - The id of the form library.
Example:
402c6e2f-xxxx-xxxx-xxxx-ff3f249f6da9
- name string? - The name of the form library.
Example:
MOR - Mainstreet Organization of Realtors
- formCount int? - The number of forms in the form library.
Example:
50
docusign.rooms: FormLibrarySummaryList
Contains a list of forms libraries.
Fields
- formsLibrarySummaries FormLibrarySummary[]? - A list of form libraries.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: FormSummary
Contains details about a form in a form library.
Fields
- libraryFormId string? - The id of the form.
Example:
301f560d-xxxx-xxxx-xxxx-063a47cc12c2
- name string? - The name of the form.
Example:
Short Sale Supplement to Marketing Agreement
- lastUpdatedDate string? - The date and time when the form was last updated.
Example:
2017-08-11T19:58:36.18
docusign.rooms: FormSummaryList
Contains a list of forms in a form library.
Fields
- forms FormSummary[]? - A list of forms.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: GlobalActivityTypes
Contains a list of activity types.
Fields
- activityTypes ActivityType[]? - A list of activity types.
docusign.rooms: GlobalClosingStatuses
Contains a list of valid closing statuses, or reasons for closing a room.
Fields
- closingStatuses ClosingStatus[]? - A list of closing statuses (reasons for closing a room).
docusign.rooms: GlobalContactSides
Contains a list of contact sides.
Fields
- contactSides ContactSide[]? - A list of contact sides.
docusign.rooms: GlobalCountries
Contains a list of supported countries.
Fields
- countries Country[]? - An array of country objects.
docusign.rooms: GlobalCurrencies
Contains a list of supported currencies.
Fields
- currencies Currency[]? - A list of currency objects.
docusign.rooms: GlobalFinancingTypes
Contains a list of financing types.
Fields
- financingTypes FinancingType[]? - A list of financing types.
docusign.rooms: GlobalOriginsOfLeads
Contains a list of origins of leads.
Fields
- originsOfLeads OriginOfLead[]? - A list of origins of leads.
docusign.rooms: GlobalPropertyTypes
Contains a list of property types.
Fields
- propertyTypes PropertyType[]? - A list of property types.
docusign.rooms: GlobalRoomContactTypes
Contains a list of room contact types.
Fields
- roomContactTypes RoomContactType[]? - A list of room contact types.
docusign.rooms: GlobalSellerDecisionTypes
Contains a list of seller decision types.
Fields
- sellerDecisionTypes SellerDecisionType[]? - A list of seller decision types.
docusign.rooms: GlobalSpecialCircumstanceTypes
Contains a list of special circumstance types.
Fields
- specialCircumstanceTypes SpecialCircumstanceType[]? - A list of special circumstance types.
docusign.rooms: GlobalStates
Contains a list of supported states.
Fields
- states State[]? - A list of states.
docusign.rooms: GlobalTaskDateTypes
Contains a list of task date types.
Fields
- taskDateTypes TaskDateType[]? - A list of task date types.
docusign.rooms: GlobalTaskResponsibilityTypes
Contains a list of task responsibility types.
Fields
- taskResponsibilityTypes TaskResponsibilityType[]? - A list of task responsibility types.
docusign.rooms: GlobalTaskStatuses
Fields
- taskStatuses TaskStatus[]? -
docusign.rooms: GlobalTimeZones
Contains a list of time zones.
Fields
- timeZones TimeZone[]? - A list of time zones.
docusign.rooms: GlobalTransactionSides
Contains a list of transaction sides.
Fields
- transactionSides TransactionSide[]? - A list of transaction sides.
docusign.rooms: GroupForm
Description of a single form in a group.
Fields
- formId string? - The id of the form.
Example:
5be324eb-xxxx-xxxx-xxxx-208065181be9
- name string? - The name of the office.
- isRequired boolean? - True if the form is required.
- lastUpdatedDate string? - The UTC date and time when the item was last updated. This is a read-only value that the service assigns. Example: 2019-07-17T17:45:42.783Z
docusign.rooms: LockedOutDetails
Details about a locked account.
Fields
- reason string - The reason the account was locked.
docusign.rooms: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://account-d.docusign.com/oauth/token") - Refresh URL
docusign.rooms: Office
Contains information about an office.
Fields
- officeId int? - The id of the office. This is the id that the system generated when you created the office.
- name string - The name of the office.
- regionId int? - The id of the region. This is the id that the system generated when you created the region.
- address1 string? - First line of the office street address.
- address2 string? - Second line of the office street address.
- city string? - City name or metropolitan area of the office address.
- stateId string? - A concatenation of the two-letter country code with the state/province/region of the office address.
Example:
US-OH
(for Ohio)
- postalCode string? - Postal code or ZIP code of the office address.
- countryId string? - The two-letter country code of the office address (for example, "UK" for United Kingdom).
- timeZoneId string? - The id of the time zone for the office address.
Example:
eastern
(for the Eastern US Time Zone)
- phone string? - Phone number of the office.
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
docusign.rooms: OfficeForCreate
Contains details about the office that you want to create.
Fields
- name string - The name of the office.
- regionId int? - The id of the region. This is the id that the system generated when you created the region.
- address1 string? - First line of the office street address.
- address2 string? - Second line of the office street address.
- city string? - City name or metropolitan area of the office address.
- stateId string? - A concatenation of the two-letter country code with the state/province/region of the office address.
Example:
US-OH
(for Ohio)
- postalCode string? - Postal code or ZIP code of the office address.
- countryId string? - The two-letter country code of the office address (for example, "UK" for United Kingdom).
- timeZoneId string? - The id of the time zone for the office address.
Example:
eastern
(for the Eastern US Time Zone)
- phone string? - Phone number of the office.
docusign.rooms: OfficeReferenceCount
A complex element containing the number and type of each object referencing the office.
Fields
- referenceType string? - The type of object referencing the office.
- referencedCount int? - The number of objects of this type referencing the office.
docusign.rooms: OfficeReferenceCountList
Contains a list of each type of object and the number of objects of that type referencing the office.
Fields
- referencesCounts OfficeReferenceCount[]? - A list of each type of object and the number of objects of that type referencing the office.
docusign.rooms: Offices
Object that contains information about an office in the Rooms account.
Fields
- officeId int? - The id of the office. This is the id that the system generated when you created the office.
- name string - The name of the office.
- regionId int? - The id of the region. This is the id that the system generated when you created the region.
- address1 string? - First line of the office street address.
- address2 string? - Second line of the office street address.
- city string? - City name or metropolitan area of the office address.
- stateId string? - A concatenation of the two-letter country code with the state/province/region of the office address.
Example:
US-OH
(for Ohio)
- postalCode string? - Postal code or ZIP code of the office address.
- countryId string? - The two-letter country code of the office address (for example, "UK" for United Kingdom).
- timeZoneId string? - The id of the time zone for the office address.
Example:
eastern
(for the Eastern US Time Zone)
- phone string? - Phone number of the office.
- createdDate string? - The UTC DateTime when the office was created.
Example:
2019-07-17T17:45:42.783Z
Note: This value is read-only.
docusign.rooms: OfficeSummary
A complex element containing summary information on an office; the elements of this object are identical to those of the Offices
object.
Fields
- officeId int? - The id of the office. This is the id that the system generated when you created the office.
- name string? - The name of the office.
- regionId int? - The id of the region. This is the id that the system generated when you created the region.
- address1 string? - First line of the office street address.
- address2 string? - Second line of the office street address.
- city string? - City name or metropolitan area of the office address.
- stateId string? - A concatenation of the two-letter country code with the state/province/region of the office address.
Example:
US-OH
(for Ohio)
- postalCode string? - Postal code or ZIP code of the office address.
- countryId string? - The two-letter country code of the office address (for example, "UK" for United Kingdom).
- timeZoneId string? - The id of the time zone for the office address.
Example:
eastern
(for the Eastern US Time Zone)
- phone string? - Phone number of the office.
- createdDate string? - UTC datetime that the office was created (for example, "2019-07-17T17:45:42.783"). Note that the service assigns this value, so it is read-only.
docusign.rooms: OfficeSummaryList
Object that contains a summary of information about a requested group of offices in the Rooms account.
Fields
- officeSummaries OfficeSummary[]? - A list of
OfficeSummary
objects containing summary information about an office.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The index position within the total result set from which returned values start.
- endPosition int? - The index position within the total result set at which returned values end.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: OriginOfLead
Contains information about an origin of lead.
Fields
- originOfLeadId string? - The id of the origin of lead.
Example:
tru
(forTrulia
)
- name string? - The name of the origin of lead. Possible values are:
Realtor.com
Trulia
Zillow
Company Website
Agent Website
Other Online
Mobile App
Social Media
Personal Referral
Company Referral
Repeat Client
Corporate Relocation
Print Marketing
Prospecting
Other
REO (Real Estate Owned)
docusign.rooms: OriginsOfLeads
The OriginsOfLeads
resource enables you to get a list of origins of leads (such as Trulia or Zillow) that you can specify for rooms.
Fields
- originOfLeadId string? - The id of the origin of lead.
Example:
tru
(forTrulia
)
- name string? - The name of the office.
docusign.rooms: Permissions
Contains details about permissions.
Fields
- canAddUsersToRooms boolean? - When set to true, users can see the Invite button on the room's People tab and can invite people into a room.
- canCreateRooms boolean? - When set to true, users can see the New button on the Rooms tab and can create a room.
- canSubmitRoomsForReview boolean? - When set to true, users can submit rooms for review that are owned by them or someone they manage.
- canCloseRooms boolean? - When set to true, users can review and close rooms that are owned by them or someone they manage.
- canReopenRooms boolean? - When set to true, users can reopen rooms that are owned by them or someone they manage.
- canDeleteOwnedRooms boolean? - When set to true, the user can delete rooms that are owned by them or someone they manage.
- autoAccessToRooms boolean? - When set to true, users are automatically added to new rooms when someone with an internal role in their office or region creates or is invited to a room.
- canExportRoomActivityDetailsPeople boolean? - When set to true, users can export the details, people, and history of a room to a PDF or CSV file.
- isVisibleInRooms boolean? - When set to true, users who also have the
autoAccessToRooms
permission enabled are visible in those rooms.
- canCopyRoomDetails boolean? - When set to true, users see a Copy room option in the room Actions menu, which copies the room's detail information to populate a new room.
- canEditAnyRoomRole boolean? - When set to true, users see a Edit room option in the room Actions menu, which edits the room's role information.
- canEditInvitedRoomRole boolean? - When set to true, users see a Edit room option in the room Actions menu, which edits the invited room's role information.
- canEditRoomSide boolean? - When set to true, users see a Edit room option in the room Actions menu, which edits the room's side information.
- canManageAnyUserRoomAccess boolean? - When set to true, users see a Manage room option in the room Actions menu, which edits the room's access information.
- canManageInvitedUserRoomAccess boolean? - When set to true, users see a Manage room option in the room Actions menu, which edits the invited room's access information.
- isHiddenInRoom boolean? - When set to true, shows if he is hidden in room.
- canManageRoomOwners boolean? - When set to true, users see a Manage room option.
- canDeleteRooms boolean? - When set to true, users see a Delete room option.
- canConnectToMortgageCadence boolean? - When set to true, users see a ConnectToMortgageCadence room option.
- canViewRoomDetails boolean? - When set to true, users can view all room detail fields that the company Admin has set to Use.
- canViewAndEditRoomDetails boolean? - When set to true, users can view and make edits to any room detail fields.
- canSendRoomDetailsToLoneWolf boolean? - When set to true, users see a SendRoomDetailsToLoneWolf room option.
- canAddDocuments boolean? - When set to true, users can add documents to rooms and share the documents that they own with other people in the room.
- canAddDocumentsFromFormGroups boolean? - When set to true, users can add documents from form groups to rooms.
- canAddDocumentsFromFormLibraries boolean? - When set to true, users can add documents from form libraries to rooms.
- documentsViewableByOthersInRoomFromOffice boolean? - When a user for whom this permission is set to true adds a document, the document is automatically shared with other room users that are in the user's office.
- documentsAutoOwnedByPeers boolean? - When a user for whom this permission is set to true adds a document, the document is automatically seen and owned by those users' peers. Peers are others in the same office or region who have the same access level as the user.
- canDeleteOwnedDocuments boolean? - When set to true, users can delete documents that they own from rooms.
- canManageSharedDocs boolean? - When set to true, users can manage all documents, including ones that another user has shared with them.
- canManageFormGroups boolean? - When set to true, users have access to Admin > Forms and can manage form groups and form libraries for the company.
- canShareDocsNotOwned boolean? - When set to true, users can share documents that they do not own (documents that another user has shared with them).
- canAddTasksToAnyTaskLists boolean? - When set to true, users can add tasks to any task list, including lists that they do not own.
- canEditEditableTasks boolean? - When set to true, users can edit editable tasks.
- canEditAnyTasks boolean? - When set to true, users can edit tasks in rooms, even if the task owner has not marked the task as editable.
- canDeleteDeletableTasks boolean? - When set to true, users can delete deletable tasks.
- canDeleteAnyTasks boolean? - When set to true, users can delete tasks, even if the task owner has not marked the task as deletable.
- canApplyTaskList boolean? - When set to true, users see the Attach Task List option in the room's Actions menu and can apply task lists to rooms.
- canRemoveAnyTaskList boolean? - When set to true, users can use the Remove Task List option in the room's Actions menu to remove task lists owned by others. Note: Users can already remove task lists that they own.
- canSubmitTaskList boolean? - When set to true, users can use the Submit Task List option in the room's Actions menu to submit task lists for review.
- canAutoSubmitTaskList boolean? - When set to true, users can use the Submit Task List option in the room's Actions menu to submit task lists for review.
- canReviewTaskList boolean? - When set to true, users can approve or decline a task list. Declining a task list sends it back to open status for the assignee to complete. The assignee also receives a notification.
- canAutoApproveTaskList boolean? - When set to true and a room is approved, the task lists associated with the room auto-approve if all of the tasks are approved.
- canManageTaskTemplatesForAllRegionsAllOffices boolean? - When set to true, users have access to the Admin > Company > Task List Templates menu so that they can create, edit, and delete task list templates for all regions and offices.
- canApplyRoomTemplates boolean? - When set to true, users can apply a room template when they create a room.
- canAddTasksToRooms boolean? - When set to true, users can add tasks to rooms.
- canReviewAnyTask boolean? - When set to true, users can review tasks of rooms.
- canManageDocsOnAnyTask boolean? - When set to true, users can add manage docs on any task of rooms.
- canAddMemberAndSetRoleLowerAccessLevel boolean? - When set to true, users can add other users with a lower access level than their own to offices or regions that they oversee and set those users' roles.
- canAddMemberAndSetRoleSameAccessLevel boolean? - When set to true, users can add other users with the same access level as their own to offices or regions that they oversee and set those users' roles.
- canChangeMemberRoleLowerAccessLevel boolean? - When set to true, users can edit the roles of other users who have a lower access level than their own and that belong to offices or regions that they oversee.
- canChangeMemberRoleSameAccessLevel boolean? - When set to true, users can edit the roles of other users who have the same access level as their own and that belong to offices or regions that they oversee.
- canManageMemberLowerAccessLevel boolean? - When set to true, users can change the access level, office, region, and eSignature permission set of other users who have a lower access level than their own.
- canManageMemberSameAccessLevel boolean? - When set to true, users can change the access level, office, region, and eSignature permission set of other users who have the same access level as their own.
- canRemoveCompanyMemberLowerAccessLevel boolean? - When set to true, users can remove other users who have a lower access level than their own and that belong to offices or regions that they oversee from the company account.
- canRemoveCompanyMemberSameAccessLevel boolean? - When set to true, users can remove other users who have the same access level as their own and that belong to offices or regions that they oversee from the company account.
- canManageAccount boolean? - When set to true, users can access the Company Settings tab under Rooms > Admin > Company to manage company account settings and change the company name, contact information, currency, offices, and regions.
- canManageLogo boolean? - When set to true, users can access the Company Logo section in Company Settings to add or change the company logo.
- canManageRolesAndPermissions boolean? - When set to true, users can add manage roles and permissions of rooms.
- canManageRoomDetails boolean? - When set to true, users see the Room Details tab under Rooms > Admin > Company and can use it to configure room details. They can also add additional contact fields.
- canManageRoomTemplates boolean? - When set to true, users see the Room Templates option in the Rooms > Admin menu, which enables them to add, edit, and delete room templates.
- canManageIntegrationSettings boolean? - When set to true, users can add manage integration settings of rooms.
docusign.rooms: PropertyType
Contains information about a property type.
Fields
- propertyTypeId string? - The id of the property type.
Example:
resd
(forResidential Detached
)
- name string? - The name of the property type. Possible values are:
Residential Detached
Residential Attached
New Construction
Residential Developed Lots
Land/Farm
Rental
Commercial
Condominium
Mobile Home
docusign.rooms: PropertyTypes
The OriginsOfLeads
resource enables you to get a list of property types (such as ????) that you can specify for rooms.
Fields
- propertyTypeId string? - The id of the property type.
Example:
resd
(forResidential Detached
)
- name string? - The name of the office.
docusign.rooms: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
docusign.rooms: Region
Contains information about a region.
Fields
- regionId int? - The id of the region. This is the id that the system generated when you created the region.
- name string - The name of the office.
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
docusign.rooms: RegionReferenceCount
A complex element containing the number and type of each object referencing the region.
Fields
- referenceType string? - The type of object referencing the region.
- referenceCount int? - The number of objects of this type referencing the region.
docusign.rooms: RegionReferenceCountList
Contains a list of each type of object and the number of objects of that type referencing the region.
Fields
- referenceCounts RegionReferenceCount[]? - A list of each type of object and the number of objects of that type referencing the region.
docusign.rooms: Regions
Fields
- regionId int? - The id of the region. This is the id that the system generated when you created the region.
- name string - String that specifies the region name.
- createdDate string? - UTC datetime that the region was created (for example "2019-06-27T19:32:46.943"). Note that the service assigns this value, so it is read-only.
docusign.rooms: RegionSummary
A complex element containing summary information on a region; the elements of this object are identical to those of the Regions
object.
Fields
- regionId int? - The id of the region. This is the id that the system generated when you created the region.
- name string? - String that specifies the region name.
- createdDate string? - UTC datetime that the region was created (for example "2019-06-27T19:32:46.943"). Note that the service assigns this value, so it is read-only.
docusign.rooms: RegionSummaryList
Object that contains a summary of information about a requested group of regions in the Rooms account.
Fields
- regionSummaries RegionSummary[]? - A list of
RegionSummary
objects containing summary information on a region; the elements of this object are identical to those of theRegions
object.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: Role
Contains details about a company role.
Fields
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
- legacyRoleId string? - The legacy name of the role in Rooms Version 5.
- name string? - The name of the role.
- isDefaultForAdmin boolean? - When true, the role is the default for account administrators.
- isExternal boolean? - When set to true, the role is an external role. You assign external roles to people from outside your company when you invite them into a room.
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
- isAssigned boolean? - When set to true, indicates that this role is currently assigned to a user.
- permissions Permissions? - Contains details about permissions.
docusign.rooms: RoleForCreate
Contains details about the role that you want to create.
Fields
- name string? - The name of the role.
- isExternal boolean? - When set to true, the role is an external role. You assign external roles to people from outside your company when you invite them into a room.
- permissions Permissions? - Contains details about permissions.
docusign.rooms: RoleForUpdate
This request object contains the details to use for the update.
Fields
- name string? - The name of the role.
- isExternal boolean? - When set to true, the role is an external role. You assign external roles to people from outside your company when you invite them into a room.
- permissions Permissions? - Contains details about permissions.
docusign.rooms: Roles
This object contains information about a role.
Fields
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
You can assign external roles to users who aren't a part of your organization.
Note: If you are using Rooms v6, you must enter a
roleId
in requests. If you are using Rooms v5, you must enter a value for thetitleId
property instead.
- legacyRoleId string? - The legacy name of the role in Rooms Version 5.
- name string? - The name of the role.
Examples:
Agent
Default Admin
- isDefaultForAdmin boolean? - When true, the role is the default for account administrators.
- isExternal boolean? - When set to true, the role is an external role. You assign external roles to people from outside your company when you invite them into a room.
- createdDate string? - The UTC DateTime when the role was created.
- isAssigned boolean? - When set to true, indicates that this role is currently assigned to a user.
- permissions Permissions? - Contains details about permissions.
docusign.rooms: RoleSummary
Contains details about a role.
Fields
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
- legacyRoleId string? - The legacy name of the role in Rooms Version 5.
- name string? - The name of the role.
- isDefaultForAdmin boolean? - When true, the role is the default for account administrators.
- isExternal boolean? - When set to true, the role is an external role. You assign external roles to people from outside your company when you invite them into a room.
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
docusign.rooms: RoleSummaryList
This complex type contains details about the roles that are associated with an account.
Fields
- roles RoleSummary[]? - An array of
role
objects.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: Room
Contains details about a room.
Fields
- roomId int? - The id of the room.
- companyId int? - The id of the company.
- name string? - The name of the room.
- officeId int? - The id of the office. This is the id that the system generated when you created the office.
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
- submittedForReviewDate string? - The UTC DateTime when the room was submitted for review. Note: In Rooms v5, this is when an agent submitted the room to a manager. In Rooms v6, this is when a member with a role for which the Submit rooms for review permission is set to true submitted the room to a member with a role for which the Review and close rooms permission is set to true.
- closedDate string? - The UTC date and time when the room was closed.
- rejectedDate string? - The date on which the reviewer rejected the room. For example, a reviewer might reject closing a room if documentation is missing or the details are inaccurate.
- createdByUserId int? - The id of the user who created the room.
- rejectedByUserId int? - The id of the user who rejected the room.
- closedStatusId string? - The reason why a room was closed. Possible values are:
sold
: Property sold.dup
: Duplicate room.escrcncl
: Escrow canceled.inspctn
: Inspection issues.exp
: Listing expired.lostbuy
: Buyer withdrew.list
: Listing withdrawn.newlist
: New listing.offrrjct
: Offer not accepted.pend
: Pending. An agent might use this status to temporarily hide a room from their Active rooms view if they are blocked on a task. When they are ready to reopen the room, they can quickly find it by filtering for rooms inpending
status.lstcanc
: Listing canceled.lstleave
: Listing released.sellwtdw
: Seller withdrew.nofin
: Buyer unable to finance.disciss
: Property disclosure issue.appiss
: Appraisal issues.mtgiss
: Mortgage issues. Use when details about why the buyer wasn't able to obtain financing are unknown.zoniss
: Zoning issues.attiss
: Attorney review issues.proplsd
: Property leased. Use for the list side of the transaction.tenlease
: Tenant signed lease. Use when an agent helps renters find a to lease.
- fieldData FieldData? - The field data associated with a room. See Rooms::GetRoomFieldData.
docusign.rooms: RoomContactType
Contains information about a room contact type.
Fields
- id string? - The id of the room contact type.
Example:
lisagent
(forListing Agent
)
- name string? - The name of the room contact type. Possible values are:
Seller
Listing Agent
Buyer
Buyer Agent
Service Provider
Transaction Coordinator
Mortgage Provider
Title Provider
Insurance Provider
Home Warranty Provider
Survey Provider
Escrow Provider
Buyer Broker
Listing Broker
docusign.rooms: RoomContactTypes
The RoomContactTypes
resource provides a method that enables you to retrieve a list of room contact types, such as Buyer, Seller, and Listing Agent.
Fields
- id string? - The id of the room contact type.
Example:
lisagent
(forListing Agent
)
- name string? - The name of the office.
docusign.rooms: RoomDocument
An individual document in a room.
Fields
- documentId int? - The ID of the document.
- name string? - The file name of the document.
Example:
Short Sale Supplement to Marketing Agreement.pdf
- ownerId int? - The id of the user who owns the document.
- size int? - The size of the document in bytes.
- folderId int? - The id of the folder the document is in.
- createdDate string? - The UTC date and time that the document was created or uploaded.
Example:
2019-07-25T22:18:56.95Z
- isSigned boolean? - When set to true, this property indicates that the document is signed.
- docuSignFormId string? - The id of the corresponding DocuSign form.
- isArchived boolean? - True if the document is archived.
- isVirtual boolean? - True if the document is virtual.
- owner RoomDocumentOwner? - Room document owner
docusign.rooms: RoomDocumentList
A list of documents in a room.
Fields
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
- documents RoomDocument[]? - An array of room documents.
docusign.rooms: RoomDocumentOwner
Room document owner
Fields
- userId int? - The id of the user.
- firstName string? - The user's first name.
- lastName string? - The user's last name.
- companyName string? - The company name.
- imageSrc string? - The source of the user's profile picture.
docusign.rooms: RoomFolder
Information about a room folder.
Fields
- roomFolderId int? - The ID of the folder.
- name string? - The name of the folder.
- isDefault boolean? - When true, this is the default folder.
docusign.rooms: RoomFolderList
A list of room folder results.
Fields
- folders RoomFolder[]? - An array of room folders.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: RoomFolders
Fields
- roomFolderId int? -
- name string? - The name of the office.
- isDefault boolean? -
docusign.rooms: RoomForCreate
This request object contains the details about the new room.
Fields
- name string - (Required) The name of the room.
- roleId int - (Required) The id of the role that the owner has in the room.
- transactionSideId string? - The id of the transaction side. Valid values are:
buy
sell
listbuy
refi
- ownerId int? - The id of the user who owns the room.
- templateId int? - (Optional) The id of the template to use to create the room.
- officeId int? - (Optional) The id of the office associated with the room. Required when creating a room on behalf of someone else or a Manager-owned room.
- fieldData FieldDataForCreate? - Contains key-value pairs that specify the properties of the room and their values.
docusign.rooms: RoomidPictureBody
Fields
- file string? - Image
docusign.rooms: RoomInvite
The information to use for the invitation.
Fields
- email string - The user's email address.
- firstName string - The user's first name.
- lastName string - The user's last name.
- roleId int - In Rooms Version 6, this is the id of the company role assigned to the user.
You can assign external roles to users who are not part of your organization.
Note: If you are using Rooms Version 6, you must enter a
roleId
in requests. If you are using Rooms Version 5, you must use one of the Users::InviteClassic methods with thetitleId
property instead.
- transactionSideId string? - Required for a real estate company; otherwise ignored.
docusign.rooms: RoomInviteResponse
Information about the sent invitation.
Fields
- userId int? - The id of the user.
- roomId int? - The id of the room.
- email string? - The user's email address.
- firstName string? - The user's first name.
- lastName string? - The user's last name.
- transactionSideId string? - The id of the transaction side. Valid values are:
buy
sell
listbuy
refi
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
You can assign external roles to users who aren't a part of your organization.
Note: If you are using Rooms v6, you must enter a
roleId
in requests. If you are using Rooms v5, you must enter a value for thetitleId
property instead.
docusign.rooms: RoomPicture
This response object contains the URL for the uploaded picture.
Fields
- url string? - The URL for the uploaded picture.
docusign.rooms: Rooms
The Rooms resource provides methods that enable you to create and manage rooms. In Rooms for Real Estate, a room is a collaborative digital space corresponding to a specific property. In Rooms for Mortgages, a room corresponds to a specific loan.
Fields
- roomId int? - The id of the room.
- companyId int? - The id of the company.
- name string? - The name of the room. Maximum Length: 100 characters.
- officeId int? - The id of the office. This is the id that the system generated when you created the office.
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
- submittedForReviewDate string? - The UTC DateTime when the room was submitted for review. Note: In Rooms v5, this is when an agent submitted the room to a manager. In Rooms v6, this is when a member with a role for which the Submit rooms for review permission is set to true submitted the room to a member with a role for which the Review and close rooms permission is set to true.
- closedDate string? - The UTC date and time when the room was closed.
- rejectedDate string? - The date on which the reviewer rejected the room. For example, a reviewer might reject closing a room if documentation is missing or the details are inaccurate.
- createdByUserId int? - The id of the user who created the room.
- rejectedByUserId int? - The id of the user who rejected the room.
- closedStatusId string? - The reason why a room was closed. Possible values are:
sold
: Property sold.dup
: Duplicate room.escrcncl
: Escrow canceled.inspctn
: Inspection issues.exp
: Listing expired.lostbuy
: Buyer withdrew.list
: Listing withdrawn.newlist
: New listing.offrrjct
: Offer not accepted.pend
: Pending. An agent might use this status to temporarily hide a room from their Active rooms view if they are blocked on a task. When they are ready to reopen the room, they can quickly find it by filtering for rooms inpending
status.lstcanc
: Listing canceled.lstleave
: Listing released.sellwtdw
: Seller withdrew.nofin
: Buyer unable to finance.disciss
: Property disclosure issue.appiss
: Appraisal issues.mtgiss
: Mortgage issues. Use when details about why the buyer wasn't able to obtain financing are unknown.zoniss
: Zoning issues.attiss
: Attorney review issues.proplsd
: Property leased. Use for the list side of the transaction.tenlease
: Tenant signed lease. Use when an agent helps renters find a to lease.
- fieldData FieldData? - The field data associated with a room. See Rooms::GetRoomFieldData.
docusign.rooms: RoomSummary
This object contains details about a room.
Fields
- roomId int? - The id of the room.
- name string? - The name of the room.
- officeId int? - The id of the office. This is the id that the system generated when you created the office.
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
- submittedForReviewDate string? - The UTC DateTime when the room was submitted for review. Note: In Rooms v5, this is when an agent submitted the room to a manager. In Rooms v6, this is when a member with a role for which the Submit rooms for review permission is set to true submitted the room to a member with a role for which the Review and close rooms permission is set to true.
- closedDate string? - The UTC date and time when the room was closed.
- rejectedDate string? - The date on which the reviewer rejected the room. For example, a reviewer might reject closing a room if documentation is missing or the details are inaccurate.
- createdByUserId int? - The id of the user who created the room.
- rejectedByUserId int? - The id of the user who rejected the room.
- closedStatusId string? - The reason why a room was closed. Possible values are:
sold
: Property sold.dup
: Duplicate room.escrcncl
: Escrow canceled.inspctn
: Inspection issues.exp
: Listing expired.lostbuy
: Buyer withdrew.list
: Listing withdrawn.newlist
: New listing.offrrjct
: Offer not accepted.pend
: Pending. An agent might use this status to temporarily hide a room from their Active rooms view if they are blocked on a task. When they are ready to reopen the room, they can quickly find it by filtering for rooms inpending
status.lstcanc
: Listing canceled.lstleave
: Listing released.sellwtdw
: Seller withdrew.nofin
: Buyer unable to finance.disciss
: Property disclosure issue.appiss
: Appraisal issues.mtgiss
: Mortgage issues. Use when details about why the buyer wasn't able to obtain financing are unknown.zoniss
: Zoning issues.attiss
: Attorney review issues.proplsd
: Property leased. Use for the list side of the transaction.tenlease
: Tenant signed lease. Use when an agent helps renters find a to lease.
- fieldDataLastUpdatedDate string? - Field data last updated date
docusign.rooms: RoomSummaryList
This complex type contains details about rooms.
Fields
- rooms RoomSummary[]? - An array of
roomSummary
objects.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: RoomTemplate
Contains details about a room template.
Fields
- roomTemplateId int? - The id of the room template.
- name string? - The name of the office.
- taskTemplateCount int? - The total number of task templates that the room template uses.
docusign.rooms: RoomTemplates
The room template resources provides a method that enables you to retrieve the room templates associated with an account.
Fields
- roomTemplateId int? - The id of the room template.
- name string? - The name of the room template.
- taskTemplateCount int? - The total number of task templates that the room template uses.
docusign.rooms: RoomTemplatesSummaryList
This complex type contains information about room templates.
Fields
- roomTemplates RoomTemplate[]? - An array of
roomTemplate
objects.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: RoomUser
This object contains details about a specific room member.
Fields
- userId int? - The id of the user.
- email string? - The user's email address.
- firstName string? - The user's first name.
- lastName string? - The user's last name.
- transactionSideId string? - The id of the transaction side. Valid values are:
buy
sell
listbuy
refi
- roleId int? - The id of the user's role.
- isRevoked boolean? - When set to true, indicates that the user's access to the room has been revoked.
- invitedByUserId int? - The
userId
of the person who invited the room user to the room.
docusign.rooms: RoomUserForUpdate
This request object contains the information that you want to update for the room user.
Fields
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
You can assign external roles to users who aren't a part of your organization.
Note: If you are using Rooms v6, you must enter a
roleId
in requests. If you are using Rooms v5, you must enter a value for thetitleId
property instead.
- transactionSideId string? - The id of the transaction side. Valid values are:
buy
sell
listbuy
refi
docusign.rooms: RoomUserRemovalDetail
Details for removal.
Fields
- revocationDate string? - The date on which the users room access should be revoked
in ISO 8601 fomat:
1973-12-31T07:54Z
.
docusign.rooms: RoomUsersResult
This complex type contains details about the users associated with a room.
Fields
- users RoomUserSummary[]? - An array of
RoomUser
objects.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: RoomUserSummary
Contains details about a room user.
Fields
- userId int? - The id of the user.
- email string? - The user's email address.
- firstName string? - The user's first name.
- lastName string? - The user's last name.
- transactionSideId string? - The id of the transaction side. Valid values are:
buy
sell
listbuy
refi
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
You can assign external roles to users who aren't a part of your organization.
Note: If you are using Rooms v6, you must enter a
roleId
in requests. If you are using Rooms v5, you must enter a value for thetitleId
property instead.
- titleId int? - In Rooms Version 5, this is the id of the custom job title for a Manager role within your company. For example, your company might have the custom job titles "Transaction Coordinator" and "Office Manager".
Note: If you are using Rooms Version 5, you must enter a
titleId
when using the Users::InviteClassicManager method. (ThetitleId
property is empty for Agent users on Rooms Version 5.) If you are using Rooms Version 6, use the Users::InviteUser method with theroleId
property instead.
- companyName string? - The company name.
- roleName string? - Role name
docusign.rooms: SelectListFieldOption
Contains details about an option in a list.
Fields
- id record {}? - The id of the list option.
Example:
AU
- title string? - The title or name of the list option.
Example:
Australia
- 'order int? - The order of the list option in the list.
Example:
3
docusign.rooms: SellerDecisionType
Contains information about a seller decision type.
Fields
- sellerDecisionTypeId string? - The id of the seller decision type.
Example:
appr
(forApproved
)
- name string? - The name of the seller decision type. Possible values are:
Pending
Approved
Countered
Rejected
Pending Rejection
docusign.rooms: SellerDecisionTypes
Contains information about a seller decision type.
Fields
- sellerDecisionTypeId string? - The id of the seller decision type.
Example:
appr
(forApproved
)
- name string? - The name of the seller decision type.
docusign.rooms: SpecialCircumstanceType
Contains information about a special circumstance type.
Fields
- specialCircumstanceTypeId string? - The id of the special circumstance type.
Example:
ss
(forShort Sale
)
- name string? - The name of the special circumstance type. Possible values are:
Short Sale
Foreclosure
Corporate Owned
Historical
Investor Owned
HUD
Estate Sale
Relocation
Contingency
docusign.rooms: SpecialCircumstanceTypes
The SpecialCircumstanceTypes
resource provides a method that enables you to retrieve a list of special circumstance types, such as Short Sale
and Foreclosure
. These are the values that you can select for the Special circumstances field that appears on the Room Details page.
Fields
- specialCircumstanceTypeId string? - The id of the special circumstance type.
Example:
ss
(forShort Sale
)
- name string? - The name of the office.
docusign.rooms: State
Contains information about a state.
Fields
- stateId string? - A concatenation of the two-letter country code with the state/province/region of the office address.
Example:
US-OH
(for Ohio)
- name string? - The name of the state. Possible values are:
Alberta
Auckland
New South Wales
Alabama
Alaska
Bay of Plenty
British Columbia
Queensland
South Australia
Manitoba
Canterbury
Arizona
Arkansas
New Brunswick
Tasmania
Hawke's Bay
Manawatu-Wanganui
Victoria
Newfoundland and Labrador
California
Colorado
Western Australia
Northwest Territories
Northland
Otago
Australian Capital Territory
Nova Scotia
Connecticut
Delaware
Nunavut
Northern Territory
Southland
Taranaki
Ontario
District of Columbia
Florida
Prince Edward Island
Waikato
Wellington
Quebec
Georgia
Guam
Saskatchewan
West Coast
Gisborne District
Yukon
Hawaii
Idaho
Marlborough District
Nelson City
Illinois
Indiana
Tasman District
Chatham Islands Territory
Iowa
Kansas
Kentucky
Louisiana
Maine
Maryland
Massachusetts
Michigan
Minnesota
Mississippi
Missouri
Montana
Nebraska
Nevada
New Hampshire
New Jersey
New Mexico
New York
North Carolina
North Dakota
Ohio
Oklahoma
Oregon
Pennsylvania
Puerto Rico
Rhode Island
South Carolina
South Dakota
Tennessee
Texas
US Virgin Islands
Utah
Vermont
Virginia
Washington
West Virginia
Wisconsin
Wyoming
docusign.rooms: States
The States
resource provides a method that enables you to retrieve a list of states and state ids that the Rooms API uses.
Fields
- stateId string? - A concatenation of the two-letter country code with the state/province/region of the office address.
Example:
US-OH
(for Ohio)
- name string? - The name of the office.
docusign.rooms: TaskDateType
Contains information about a task date type.
Fields
- taskDateTypeId string? - The id of the task date type.
Example:
tdd
(forTask Due Date
)
- name string? - The name of the task date type.
Possible values are:
Specific Calendar Date
Task Due Date
Actual Close Date
Binding Date
Contingency Removal Date
Contract Date
Expected Closing Date
Listing Date
Listing Expiration Date
Offer Date
docusign.rooms: TaskDateTypes
This resource provides a method that returns a list of date types that you can use with tasks, such as Actual Close Date
and Task Due Date
.
Fields
- taskDateTypeId string? - The id of the task date type.
Example:
tdd
(forTask Due Date
)
- name string? - The name of the office.
docusign.rooms: TaskList
This response object contains details about the new task list.
Fields
- taskListId int? - The id of the task list.
- name string? - The name of the task list.
- taskListTemplateId int? - The id of the task list template used to create the task list.
- submittedForReviewDate string? - The UTC DateTime when the task list was submitted for review.
- approvalDate string? - The UTC DateTime when the task list was approved.
- rejectedDate string? - The UTC DateTime when the reviewer rejected the task list.
- createdDate string? - The UTC date and time when the task list was created. This is a read-only value that the service assigns. Example: 2019-07-17T17:45:42.783Z
- approvedByUserId int? - The id of the user who approved the task list.
- rejectedByUserId int? - The id of the user who rejected the task list.
- comment string? - Contains a comment about the task list.
- tasks TaskSummary[]? - A list of tasks in the task list.
docusign.rooms: TaskListForCreate
Contains information about the task list template to use to create the new task list.
Fields
- taskListTemplateId int? - (Required) The id of the task list template.
docusign.rooms: TaskLists
The TaskLists
resource helps you keep track of the documents and activities you must complete before you can close a room.
Fields
- taskListId int? - The id of the task list.
- name string? - The name of the office.
- taskListTemplateId int? - The id of the task list template.
- submittedForReviewDate string? - The UTC DateTime when the room was submitted for review. Note: In Rooms v5, this is when an agent submitted the room to a manager. In Rooms v6, this is when a member with a role for which the Submit rooms for review permission is set to true submitted the room to a member with a role for which the Review and close rooms permission is set to true.
- approvalDate string? - Approval date
- rejectedDate string? - The date on which the reviewer rejected the room. For example, a reviewer might reject closing a room if documentation is missing or the details are inaccurate.
- createdDate string? - The UTC date and time when the item was created. This is a read-only value that the service assigns.
Example:
2019-07-17T17:45:42.783Z
- approvedByUserId int? - Approved by user ID
- rejectedByUserId int? - The id of the user who rejected the room.
- comment string? - Task comments
- tasks TaskSummary[]? - A list of tasks in the task list.
docusign.rooms: TaskListSummary
Contains information about a task list.
Fields
- taskListId int? - The id of the task list.
- name string? - The name of the task list.
- taskListTemplateId int? - The id of the task list template.
- submittedForReviewDate string? - The UTC DateTime when the task list was submitted for review.
- approvalDate string? - The UTC DateTime when the task list was approved.
- rejectedDate string? - The date on which the reviewer rejected the task list.
- createdDate string? - The UTC date and time when the task list was created. This is a read-only value that the service assigns. Example: 2019-07-17T17:45:42.783Z
- approvedByUserId int? - The id of the user who approved the task list.
- rejectedByUserId int? - The id of the user who rejected the task list.
- comment string? - Contains a user comment about the task list.
docusign.rooms: TaskListSummaryList
Contains a list of task list summaries.
Fields
- taskListSummaries TaskListSummary[]? - A list of task list summaries.
docusign.rooms: TaskListTemplate
Contains details about a task list template.
Fields
- taskListTemplateId int? - The id of the task list template.
- name string? - The name of the task list template.
- taskCount int? - The total number of tasks in the task list template.
- tasksWithDocumentsCount int? - The number of tasks in the task list template that have documents associated with them.
docusign.rooms: TaskListTemplateList
Contains a list of task list templates.
Fields
- taskListTemplates TaskListTemplate[]? - A list of task list templates.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: TaskListTemplates
A task list template is a custom task list that can be added to rooms.
Fields
- taskListTemplateId int? - The id of the task list template.
- name string? - The name of the office.
- taskCount int? - The total number of tasks in the task list template.
- tasksWithDocumentsCount int? - The number of tasks in the task list template that have documents associated with them.
docusign.rooms: TaskResponsibilityType
Contains information about a task responsibility type.
Fields
- taskResponsibilityTypeId string? - The id of the task responsibility type.
- name string? - The name of the task responsibility type. The possible values are:
Assignee
Watcher
Reviewer
docusign.rooms: TaskResponsibilityTypes
The TaskResponsibilityTypes
resource enables you to return a list of responsibility types that you can assign to users when you add them to a task.
Fields
- taskResponsibilityTypeId string? - The id of the task responsibility type.
- name string? - The name of the office.
docusign.rooms: TaskStatus
Fields
- taskStatusId string? -
- name string? - The name of the office.
docusign.rooms: TaskStatuses
Fields
- taskStatusId string? -
- name string? - The name of the office.
docusign.rooms: TaskSummary
Contains information about a task list.
Fields
- taskId int? - The id of the task list.
- name string? - The name of the task list.
- requiresApproval boolean? - When set to true, the task must be completed and reviewed before it can be closed.
- dueDateTypeId string? - The id of the due date type (such as Actual Close Date or Contract Date).
- dueDateOffset int? - The number of days before or after the due date (specified by the
dueDateTypeId
) within which the task must be completed. A negative number indicates that the task must be completed within a certain number of days before the due date. A positive number indicates that the task must be completed within a certain number of days after the due date.
- fixedDueDate string? - A specific calendar due date for the task. In the API, this value is a UTC DateTime that does not actually include a time. Example: 2019-07-17T00:00:00.000Z
- ownerUserId int? - The id of the user who owns the task.
- completionDate string? - The UTC DateTime when the task was completed. Example: 2019-07-17T17:45:42.783Z
- approvalDate string? - The UTC DateTime when the task was approved. Example: 2019-07-17T17:45:42.783Z
- rejectedDate string? - The date on which the reviewer rejected the task.
- createdDate string? - The UTC date and time when the task was created. This is a read-only value that the service assigns. Example: 2019-07-17T17:45:42.783Z
- isDocumentTask boolean? - When set to true, the task is associated with a document.
- requiresReview boolean? - When set to true, the task is optional. If the task is completed (if a document is added or the task is marked complete), it must be reviewed before it can be closed.
docusign.rooms: TimeZone
Contains information about a time zone.
Fields
- timeZoneId string? - The id of the time zone.
Example:
brisbane
- name string? - The name of the time zone.
Example:
Eastern Australia (Brisbane)
docusign.rooms: TimeZones
The TimeZones
resource enables you to list the time zones that you can assign to an office.
Fields
- timeZoneId string? - The id of the time zone for the office address.
Example:
eastern
(for the Eastern US Time Zone)
- name string? - The name of the office.
docusign.rooms: TransactionSide
Contains information about a real estate transaction side.
Fields
- transactionSideId string? - The id of the transaction side. Valid values are:
buy
sell
listbuy
refi
- name string? - The name of the transaction side. Valid values are:
List Side
Buy Side
List & Buy Side
Refinance
docusign.rooms: TransactionSides
The TransactionSides
resource provides a method that enables you to list possible real estate transaction sides.
Fields
- transactionSideId string? - The id of the transaction side. Valid values are:
buy
sell
listbuy
refi
- name string? - The name of the office.
docusign.rooms: User
Contains details about a user.
Fields
- userId int? - The id of the user.
- email string? - The user's email address.
- firstName string? - The user's first name.
- lastName string? - The user's last name.
- isLockedOut boolean? - When set to true, an administrator has locked the user's account. For example, an administrator might want to lock an agent's account after they leave the brokerage until they determine how to transfer the agent's rooms and data to another active user.
- status string? - The user's status. Read only. Possible values are:
Active
: The user is active.Pending
: The user has been invited but has not yet accepted the invitation.
- accessLevel AccessLevel? - Access level
- defaultOfficeId int? - The id of the user's default office.
- titleId int? - In Rooms Version 5, this is the id of the custom job title for a Manager role within your company. For example, your company might have the custom job titles "Transaction Coordinator" and "Office Manager".
Note: If you are using Rooms Version 5, you must enter a
titleId
when using the Users::InviteClassicManager method. (ThetitleId
property is empty for Agent users on Rooms Version 5.) If you are using Rooms Version 6, use the Users::InviteUser method with theroleId
property instead.
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
You can assign external roles to users who aren't a part of your organization.
Note: If you are using Rooms v6, you must enter a
roleId
in requests. If you are using Rooms v5, you must enter a value for thetitleId
property instead.
- profileImageUrl string? - The URL for the user's profile image.
- offices int[]? - An array of office ids for the offices in which a user with an
Office
orContributor
accessLevel
has been granted the ability to participate.
- regions int[]? - An array of region ids for the regions in which a user with the
Region accessLevel
has been granted the ability to participate.
- permissions ClassicManagerPermissions? - This object contains details about user permissions. These permissions are associated only with Rooms v5.
docusign.rooms: UserForUpdate
This request object contains the information to use to update a user's default office.
Fields
- defaultOfficeId int - (Required) The id of the user's default office.
docusign.rooms: Users
The Users resource provides methods that enable you to add, update, delete, and manage users. A user is a person who is either added to a room (as a participant), or who is a member of a company.
Fields
- userId int? - The id of the user.
- email string? - The user's email address.
- firstName string? - The user's first name.
- lastName string? - The user's last name.
- isLockedOut boolean? - When set to true, an administrator has locked the user's account. For example, an administrator might want to lock an agent's account after they leave the brokerage until they determine how to transfer the agent's rooms and data to another active user.
- status string? - Valid values are 'Active', 'Pending'
- accessLevel AccessLevel? - Access level
- defaultOfficeId int? - The id of the user's default office.
- titleId int? - In Rooms Version 5, this is the id of the custom job title for a Manager role within your company. For example, your company might have the custom job titles "Transaction Coordinator" and "Office Manager".
Note: If you are using Rooms Version 5, you must enter a
titleId
when using the Users::InviteClassicManager method. (ThetitleId
property is empty for Agent users on Rooms Version 5.) If you are using Rooms Version 6, use the Users::InviteUser method with theroleId
property instead.
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
You can assign external roles to users who aren't a part of your organization.
Note: If you are using Rooms v6, you must enter a
roleId
in requests. If you are using Rooms v5, you must enter a value for thetitleId
property instead.
- profileImageUrl string? - The URL for the user's profile image.
- offices int[]? - An array of office ids for the offices in which a user with an
Office
orContributor
accessLevel
has been granted the ability to participate.
- regions int[]? - An array of region ids for the regions in which a user with the
Region accessLevel
has been granted the ability to participate.
- permissions ClassicManagerPermissions? - This object contains details about user permissions. These permissions are associated only with Rooms v5.
docusign.rooms: UserSummary
Contains details about a user.
Fields
- userId int? - The id of the user.
- email string? - The user's email address.
- firstName string? - The user's first name.
- lastName string? - The user's last name.
- isLockedOut boolean? - When set to true, an administrator has locked the user's account. For example, an administrator might want to lock an agent's account after they leave the brokerage until they determine how to transfer the agent's rooms and data to another active user.
- status string? - The user's status. Possible values are:
Active
Pending
- accessLevel AccessLevel? - Access level
- defaultOfficeId int? - The id of the user's default office.
- titleId int? - In Rooms Version 5, this is the id of the custom job title for a Manager role within your company. For example, your company might have the custom job titles "Transaction Coordinator" and "Office Manager".
Note: If you are using Rooms Version 5, you must enter a
titleId
when using the Users::InviteClassicManager method. (ThetitleId
property is empty for Agent users on Rooms Version 5.) If you are using Rooms Version 6, use the Users::InviteUser method with theroleId
property instead.
- roleId int? - In Rooms v6, this is the id of the company role assigned to the user.
You can assign external roles to users who aren't a part of your organization.
Note: If you are using Rooms v6, you must enter a
roleId
in requests. If you are using Rooms v5, you must enter a value for thetitleId
property instead.
- profileImageUrl string? - The URL for the user's profile image.
docusign.rooms: UserSummaryList
Contains a list of users.
Fields
- userSummaries UserSummary[]? - A list of users.
- resultSetSize int? - The number of results returned in this response.
- startPosition int? - The starting zero-based index position of the results set. When this property is used as a query parameter, the default value is
0
.
- endPosition int? - The last zero-based index position in the result set.
- nextUri string? - The URI for the next chunk of records based on the search request. This property is
null
for the last set of search results.
- priorUri string? - The URI for the previous chunk of records based on the search request. This property is
null
for the first set of search results.
- totalRowCount int? - Total row count
docusign.rooms: UserToInvite
This request object contains information about the user that you are inviting.
Fields
- firstName string - (Required) The user's first name.
- lastName string - (Required) The user's last name.
- email string - (Required) The user's email address.
- roleId int - (Required) In Rooms v6, this is the id of the company role assigned to the user. You can assign external roles to users who are not part of your organization.
- accessLevel AccessLevel - Access level
- defaultOfficeId int - (Required) The id of the user's default office.
- regions int[]? - An array of region ids for the regions in which a user with the
Region accessLevel
has been granted the ability to participate. If the value foraccessLevel
isRegion
, this property is required.
- offices int[]? - An array of office ids for the offices in which a user with an
Office
orContributor
accessLevel
has been granted the ability to participate. If the value foraccessLevel
isOffice
, this property is required.
- eSignPermissionProfileId string - (Required) When an administrator or authorized member invites a new user to become an account member, the system also creates an eSignature account for the invitee at the same time. The eSignPermissionProfileId is the id of the eSignature permission set to assign to the member.
- redirectUrl string? - URL to redirect to after inviting.
String types
docusign.rooms: AccessLevel
AccessLevel
Access level
docusign.rooms: AccountStatus
AccountStatus
docusign.rooms: FieldsCustomDataFilterType
FieldsCustomDataFilterType
docusign.rooms: MemberSortingOption
MemberSortingOption
docusign.rooms: ProductVersion
ProductVersion
Product version
docusign.rooms: RoomStatus
RoomStatus
docusign.rooms: RoomUserSortingOption
RoomUserSortingOption
Import
import ballerinax/docusign.rooms;
Metadata
Released date: about 1 year ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 4
Current verison: 2
Weekly downloads
Keywords
Content & Files/Documents
Cost/Freemium
Contributors