docusign.admin
Module docusign.admin
API
Definitions
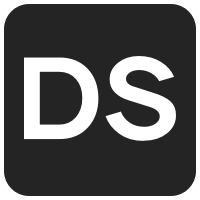
ballerinax/docusign.admin Ballerina library
Overview
This is a generated connector for DocuSign Admin API OpenAPI specification.
DocuSign Admin API is for an organization administrator to manage organizations, accounts and users which enables you to automate user management with your existing systems while ensuring governance and compliance.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a DocuSign account.
- Obtain tokens by following this guide.
Quickstart
To use the DocuSign Admin connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/docusign.admin
module into the Ballerina project.
import ballerinax/docusign.admin;
Step 2 - Create a new connector instance
You can now make the connection configuration using the <ACCESS_TOKEN>.
admin:ClientConfig clientConfig = { auth : { token: `<ACCESS_TOKEN>` } }; admin:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to list all clickwraps using the connector.
public function main() { admin:OrganizationsResponse|error result = baseClient->getOrganizationList(); if (result is admin:OrganizationsResponse) { log:printInfo(result.toString()); } else { log:printInfo(result.toString()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
docusign.admin: Client
This is a generated connector for DocuSign Admin API OpenAPI specification. An API for an organization administrator to manage organizations, accounts and users which enables you to automate user management with your existing systems while ensuring governance and compliance.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a DocuSign account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.docusign.net/Management" - URL of the target service
getOrganizationList
function getOrganizationList(string? mode) returns OrganizationsResponse|error
Returns a list of organizations that the authenticated user belongs to.
Parameters
- mode string? (default ()) - Specifies how to select the organizations. Valid values: -
org_admin
: Returns organizations for which the authenticated user is an admin. -account_membership
: Returns organizations that contain an account of which the authenticated user is a member Default value:org_admin
Return Type
getAccountsPermissionProfiles
function getAccountsPermissionProfiles(string organizationId, string accountId) returns PermissionsResponse|error
Returns the list of permission profiles in an account.
Return Type
- PermissionsResponse|error - OK
getGroupsInAccount
function getGroupsInAccount(string organizationId, string accountId, int? 'start, int? take, int? end) returns MemberGroupsResponse|error
Returns the list of groups in an account.
Parameters
- organizationId string - The organization ID Guid
- accountId string - The account ID Guid
- 'start int? (default ()) - Index of first item to include in the response. The default value is 0.
- take int? (default ()) - Page size of the response. The default value is 20.
- end int? (default ()) - Index of the last item to include in the response. Ignored if
take
parameter is specified.
Return Type
getOrganizationExportRequests
function getOrganizationExportRequests(string organizationId) returns OrganizationExportsResponse|error
Returns a list of pending and completed export requests.
Parameters
- organizationId string - The organization ID Guid
Return Type
createUserListExportRequest
function createUserListExportRequest(string organizationId, OrganizationExportRequest payload) returns OrganizationExportResponse|error
Creates a user list export request.
Parameters
- organizationId string - The organization ID Guid
- payload OrganizationExportRequest - Enables you to specify the kind of export request.
Return Type
getAccountSettingExportRequests
function getAccountSettingExportRequests(string organizationId) returns OrganizationExportsResponse|error
Returns a list of pending and completed account settings export request.
Parameters
- organizationId string - The organization ID Guid
Return Type
createAccountSettingsExportRequest
function createAccountSettingsExportRequest(string organizationId, OrganizationAccountsRequest payload) returns OrganizationExportResponse|error
Creates a new account settings export request.
Parameters
- organizationId string - The organization ID Guid
- payload OrganizationAccountsRequest - Organization account request.
Return Type
getUserListExportRequestByExportId
function getUserListExportRequestByExportId(string organizationId, string exportId) returns OrganizationExportResponse|error
Returns the results for single user list export request.
Parameters
- organizationId string - The organization ID Guid
- exportId string - The export ID GUID for the request.
Return Type
deleteUserListExpotByExportId
Deletes a single user list export request.
Parameters
- organizationId string - The organization ID Guid
- exportId string - The export ID GUID for the request.
Return Type
- json|error - OK
getAccountSettingsExportByExportId
function getAccountSettingsExportByExportId(string organizationId, string exportId) returns OrganizationExportResponse|error
Returns the results for a single account settings export request.
Parameters
- organizationId string - The organization ID Guid
- exportId string - The export ID GUID for the request.
Return Type
deleteAccountSettingsExportByExportId
function deleteAccountSettingsExportByExportId(string organizationId, string exportId) returns json|error
Deletes a single account settings export request.
Parameters
- organizationId string - The organization ID Guid
- exportId string - The export ID GUID for the request.
Return Type
- json|error - OK
getOrganizationImportAccountSettingsRequests
function getOrganizationImportAccountSettingsRequests(string organizationId) returns OrganizationAccountSettingsImportResponse[]|error
Returns the details and metadata for Bulk Account Settings Import requests in the organization.
Parameters
- organizationId string - The organization ID Guid
Return Type
createImportAccountSettingsRequest
function createImportAccountSettingsRequest(string organizationId, ImportsAccountSettingsBody payload) returns OrganizationAccountSettingsImportResponse|error
Creates a new account settings import request.
Parameters
- organizationId string - The organization ID GUID.
- payload ImportsAccountSettingsBody - CSV file containing the account settings
Return Type
getBulkAccountSettingsImportRequestById
function getBulkAccountSettingsImportRequestById(string organizationId, string importId) returns OrganizationAccountSettingsImportResponse|error
Returns the details/metadata for a Bulk Account Settings Import request.
Parameters
- organizationId string - The organization ID Guid
- importId string - The import ID GUID for the request.
Return Type
deleteAccountSettingsImportRequestById
function deleteAccountSettingsImportRequestById(string organizationId, string importId) returns json|error
Deletes a Bulk Account Settings Import request.
Parameters
- organizationId string - The organization ID Guid
- importId string - The import ID GUID for the request.
Return Type
- json|error - OK
createImportUsersRequest
function createImportUsersRequest(string organizationId, BulkUsersAddBody payload) returns OrganizationImportResponse|error
Creates a request to import new users into an account.
Parameters
- organizationId string - The organization ID Guid
- payload BulkUsersAddBody - The bulk user data as a CSV content
Return Type
createImportSingleAccountUsersInsertRequest
function createImportSingleAccountUsersInsertRequest(string organizationId, string accountId, BulkUsersAddBody1 payload) returns OrganizationImportResponse|error
Import request for adding user to a single account within the organization.
Parameters
- organizationId string - The organization ID Guid
- accountId string - The account ID Guid
- payload BulkUsersAddBody1 - The bulk user data as a CSV content
Return Type
updateImportedUsers
function updateImportedUsers(string organizationId, BulkUsersUpdateBody payload) returns OrganizationImportResponse|error
Bulk updates information for existing users.
Parameters
- organizationId string - The organization ID Guid
- payload BulkUsersUpdateBody - The bulk user data as a CSV content
Return Type
updateSingleAccountUsersInOrganization
function updateSingleAccountUsersInOrganization(string organizationId, string accountId, BulkUsersUpdateBody1 payload) returns OrganizationImportResponse|error
Import request for updating users for a single account within the organization.
Parameters
- organizationId string - The organization ID Guid
- accountId string - The account ID Guid
- payload BulkUsersUpdateBody1 - The bulk user data as a CSV content
Return Type
createImportUsersAccountCloseRequest
function createImportUsersAccountCloseRequest(string organizationId) returns OrganizationImportResponse|error
Creates a request to close the accounts of existing users.
Parameters
- organizationId string - The organization ID Guid
Return Type
closeExtenalMemberships
function closeExtenalMemberships(string organizationId) returns OrganizationImportResponse|error
Closes external memberships.
Parameters
- organizationId string - The organization ID Guid
Return Type
getImportUsersRequests
function getImportUsersRequests(string organizationId) returns OrganizationImportsResponse|error
Gets a list of all of the user import requests.
Parameters
- organizationId string - The organization ID Guid
Return Type
getImportUserRequestById
function getImportUserRequestById(string organizationId, string importId) returns OrganizationImportResponse|error
Returns the details of a single user import request.
Parameters
- organizationId string - The organization ID Guid
- importId string - The import ID GUID for the request.
Return Type
deleteImportUserRequestById
Deletes a specific user import request.
Parameters
- organizationId string - The organization ID Guid
- importId string - The import ID GUID for the request.
Return Type
- json|error - OK
getCsvResultsOfUserImportRequest
function getCsvResultsOfUserImportRequest(string organizationId, string importId) returns json|error
Given the ID of a user import request, return the CSV file that was imported.
Parameters
- organizationId string - The organization ID Guid
- importId string - The import ID GUID for the request.
Return Type
- json|error - OK
getIdentityProvidersOfOrganization
function getIdentityProvidersOfOrganization(string organizationId) returns IdentityProvidersResponse|error
Returns the list of identity providers for an organization.
Parameters
- organizationId string - The organization ID Guid
Return Type
getReservedDomainsOfOrganization
function getReservedDomainsOfOrganization(string organizationId) returns DomainsResponse|error
Returns the list of reserved domains for the organization.
Parameters
- organizationId string - The organization ID Guid
Return Type
- DomainsResponse|error - OK
updateUser
function updateUser(string organizationId, UpdateUsersRequest payload) returns UsersUpdateResponse|error
Updates a user's information.
Parameters
- organizationId string - The organization ID Guid
- payload UpdateUsersRequest - A list of users whose information you want to change.
Return Type
- UsersUpdateResponse|error - OK
upateUserEmailAddress
function upateUserEmailAddress(string organizationId, UpdateUsersEmailRequest payload) returns UsersUpdateResponse|error
Updates a user's email address.
Parameters
- organizationId string - The organization ID Guid
- payload UpdateUsersEmailRequest - A change email request.
Return Type
- UsersUpdateResponse|error - OK
closeUserMemberships
function closeUserMemberships(string organizationId, string userId) returns DeleteMembershipsResponse|error
Closes a user's memberships.
Return Type
getUsersInOrganization
function getUsersInOrganization(string organizationId, int? 'start, int? take, int? end, string? email, string? emailUserNameLike, string? status, string? membershipStatus, string? accountId, string? organizationReservedDomainId, string? lastModifiedSince) returns OrganizationUsersResponse|error
Returns information about the users in an organization.
Parameters
- organizationId string - The organization ID Guid
- 'start int? (default ()) - Index of first item to include in the response. The default value is 0.
- take int? (default ()) - Page size of the response. The default value is 20.
- end int? (default ()) - Index of the last item to include in the response. Ignored if
take
parameter is specified.
- email string? (default ()) - Email address of the desired user. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- emailUserNameLike string? (default ()) - Selects users by pattern matching on the user's email address
- status string? (default ()) - Status.
- membershipStatus string? (default ()) - The user's membership status. One of: -
activation_required
-activation_sent
-active
-closed
-disabled
- accountId string? (default ()) - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- organizationReservedDomainId string? (default ()) - Select users that are in the specified domain. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- lastModifiedSince string? (default ()) - Select users whose data have been modified since the date specified.
account_id
ororganization_reserved_domain_id
must be specified.
Return Type
createUser
function createUser(string organizationId, NewUserRequest payload) returns NewUserResponse|error
Creates a new user.
Parameters
- organizationId string - The organization ID Guid
- payload NewUserRequest - Information about a new user.
Return Type
- NewUserResponse|error - OK
activateUserMembership
function activateUserMembership(string organizationId, string userId, string membershipId, ForceActivateMembershipRequest payload) returns UpdateResponse|error
Activates user memberships.
Parameters
- organizationId string - The organization ID Guid
- userId string - The user ID Guid
- membershipId string - The membership ID GUID.
- payload ForceActivateMembershipRequest - Data to ctivate user memership
Return Type
- UpdateResponse|error - OK
getRecentlyModifiedUsers
function getRecentlyModifiedUsers(string organizationId, string? email) returns UsersDrilldownResponse|error
Returns information about recently modified users.
Parameters
- organizationId string - The organization ID Guid
- email string? (default ()) - The email address associated with the users you want to retrieve.
Return Type
deleteUserIdentities
function deleteUserIdentities(string organizationId, string userId) returns DeleteResponse|error
Deletes user identities.
Return Type
- DeleteResponse|error - OK
createAccountUsers
function createAccountUsers(string organizationId, string accountId, NewAccountUserRequest payload) returns NewUserResponse|error
Adds users to an account.
Parameters
- organizationId string - The organization ID Guid
- accountId string - The account ID Guid
- payload NewAccountUserRequest - A new user request.
Return Type
- NewUserResponse|error - OK
getDsGroups
function getDsGroups(string organizationId, string accountId, int? page, int? pageSize) returns DSGroupListResponse|error
Returns a list of DSGroups.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- page int? (default ()) - Start page of DSGroups.
- pageSize int? (default ()) - Page size of DSGroups.
Return Type
- DSGroupListResponse|error - OK
createDsGroup
function createDsGroup(string organizationId, string accountId, DSGroupAddRequest payload) returns DSGroupResponse|error
Creates a new DSGroup.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- payload DSGroupAddRequest - DS Group add data
Return Type
- DSGroupResponse|error - OK
getDsGroup
function getDsGroup(string organizationId, string accountId, string dsGroupId) returns DSGroupResponse|error
Returns details about a single DSGroup.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- dsGroupId string - The DSGroup's ID GUID
Return Type
- DSGroupResponse|error - OK
deleteDsGroup
function deleteDsGroup(string organizationId, string accountId, string dsGroupId) returns Response|error
Deletes a DSGroup.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- dsGroupId string - The DSGroup's GUID.
getDsGroupUsers
function getDsGroupUsers(string organizationId, string accountId, string dsGroupId, int? page, int? pageSize) returns DSGroupAndUsersResponse|error
Gets a list of users in a DSGroup.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- dsGroupId string - The DSGroup's GUID.
- page int? (default ()) - Start page of DSGroups.
- pageSize int? (default ()) - Page size of DSGroups.
Return Type
addDsGroupUsers
function addDsGroupUsers(string organizationId, string accountId, string dsGroupId, DSGroupUsersAddRequest payload) returns AddDSGroupAndUsersResponse|error
Adds a list of users to a DSGroup.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- dsGroupId string - The DSGroup's GUID.
- payload DSGroupUsersAddRequest - DG Group user data
Return Type
removeDsGroupUsers
function removeDsGroupUsers(string organizationId, string accountId, string dsGroupId) returns RemoveDSGroupUsersResponse|error
Removes a list of users from a DSGroup.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- dsGroupId string - The DSGroup's GUID.
Return Type
getProductPermissionProfiles
function getProductPermissionProfiles(string organizationId, string accountId) returns ProductPermissionProfilesResponse|error
Gets products associated with the account and the available permission profiles.
Return Type
getUserProductPermissionProfilesOfProduct
function getUserProductPermissionProfilesOfProduct(string organizationId, string accountId, string userId) returns ProductPermissionProfilesResponse|error
Retrieves a list of user's permission profiles for each account's product.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- userId string - The user ID GUID.
Return Type
assignUserToProductPermissionProfiles
function assignUserToProductPermissionProfiles(string organizationId, string accountId, string userId, ProductPermissionProfilesRequest payload) returns UserProductPermissionProfilesResponse|error
Assigns user to permission profiles for one or more products.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- userId string - The user ID GUID.
- payload ProductPermissionProfilesRequest - Product permission profile data
Return Type
createOrUpdateMultiProductUsers
function createOrUpdateMultiProductUsers(string organizationId, string accountId, NewMultiProductUserAddRequest payload) returns AddUserResponse|error
Creates and updates a multi-product user.
Parameters
- organizationId string - The organization's GUID.
- accountId string - The account ID GUID.
- payload NewMultiProductUserAddRequest - Multi product user data
Return Type
- AddUserResponse|error - OK
Records
docusign.admin: AccountSettingsExport
Methods and objects to get account information.
docusign.admin: AccountSettingsImport
Methods and objects to update account settings.
docusign.admin: AddDSGroupAndUsersResponse
Fields
- group DSGroupResponse? -
- group_users AddDSGroupUsersResponse? -
docusign.admin: AddDSGroupUsersResponse
Fields
- is_success boolean? -
- TotalCount int? -
- users DSGroupUserResponse[]? - A list of users.
docusign.admin: AddUserResponse
Fields
- id string? -
- site_id int? -
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- email string? - The email address.
- language_culture string? - The language and culture of the user.
- Chinese Simplified:
zh_CN
- Chinese Traditional:
zh_TW
- Dutch:
nl
- English:
en
- French:
fr
- German:
de
- Italian:
it
- Japanese:
ja
- Korean:
ko
- Portuguese:
pt
- Portuguese Brazil:
pt_BR
- Russian:
ru
- Spanish:
es
- Chinese Simplified:
- federated_status string? - The user's federated status. One of:
RemoveStatus
FedAuthRequired
FedAuthBypass
Evicted
- accounts AddUserResponseAccountProperties[]? -
docusign.admin: AddUserResponseAccountProperties
Fields
- id string? -
- site_id int? -
- product_permission_profiles ProductPermissionProfileResponse[]? -
- ds_groups DSGroupResponse[]? -
- company_name string? - The user's company name.
- job_title string? - The user's job title.
docusign.admin: BulkUsersAddBody
Fields
- 'file\.csv string - CSV file.
docusign.admin: BulkUsersAddBody1
Fields
- 'file\.csv string - CSV file.
docusign.admin: BulkUsersUpdateBody
Fields
- 'file\.csv string - CSV file.
docusign.admin: BulkUsersUpdateBody1
Fields
- 'file\.csv string - CSV file.
docusign.admin: CertificateResponse
Information about a single certificate.
Fields
- id string? - The unique ID of the certificate.
- issuer string? - The certificate issuer.
- thumbprint string? - The thumbprint of the certificate.
- expiration_date string? - The date when the certificate expires.
- is_valid boolean? - If true, the certificate is valid.
- links LinkResponse[]? - A list of useful links.
docusign.admin: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
docusign.admin: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
docusign.admin: DeleteMembershipRequest
Fields
- id string - The ID of a user's account you want to close.
docusign.admin: DeleteMembershipResponse
Results of closing accounts.
Fields
- id string? - The ID of an account that could not be closed.
- error_details ErrorDetails? - Errors.
docusign.admin: DeleteMembershipsRequest
A list of accounts to close for a user.
Fields
- accounts DeleteMembershipRequest[] - A list of accounts to close for a user.
docusign.admin: DeleteMembershipsResponse
The results of closing a user's account.
Fields
- success boolean? - If true, the request to close the accounts succeeded.
- accounts DeleteMembershipResponse[]? - A list of accounts that were closed.
docusign.admin: DeleteResponse
Results of deleting identities.
Fields
- success boolean? - If true, the request succeeded.
- identities UserIdentityResponse[]? - A list of identities to delete.
docusign.admin: DeleteUserIdentityRequest
Request to delete a user's identities,
Fields
- identities UserIdentityRequest[] - A list of identities.
docusign.admin: DomainResponse
Information about a reserved domain.
Fields
- id string? - The ID of the reserved domain.
- status string? - The status of the request. One of:
unknown
pending
active
deactivated
rejected
- host_name string? - The host name of the reserved domain.
- txt_token string? - A token in form of text of the reserved domain.
- identity_provider_id string? - The identity provider ID of the reserved domain.
- settings SettingResponse[]? - A list of settings for the reserved domain.
- links LinkResponse[]? - A list of useful links.
docusign.admin: DomainsResponse
A response about reserved domains.
Fields
- reserved_domains DomainResponse[]? - Information about reserved domains.
docusign.admin: DSGroupAddRequest
DS Group add data
Fields
- group_name string - Group name
- description string? - Description
docusign.admin: DSGroupAndUsersResponse
Fields
- group DSGroupResponse? -
- group_users DSGroupUsersResponse? -
docusign.admin: DSGroupListResponse
Fields
- page int? - The page number.
- page_size int? - The number of items per page.
- total_count int? -
- account_id string? - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- ds_groups DSGroupResponse[]? -
docusign.admin: DSGroupRequest
Fields
- ds_group_id string -
docusign.admin: DSGroupResponse
Fields
- ds_group_id string? -
- account_id string? - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- source_product_name string? -
- group_id string? -
- group_name string? -
- description string? -
- is_admin boolean? -
- last_modified_on string? -
- user_count int? -
- external_account_id int? -
- account_name string? -
docusign.admin: DSGroupUserResponse
Fields
- user_id string? -
- account_id string? - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- middle_name string? -
- status string? - Status.
- error_details ErrorDetails? - Errors.
docusign.admin: DSGroupUsersAddRequest
DG Group user data
Fields
- user_ids string[] - User IDs
docusign.admin: DSGroupUsersRemoveRequest
Fields
- user_ids string[] -
docusign.admin: DSGroupUsersResponse
Fields
- page int? - The page number.
- page_size int? - The number of items per page.
- total_count int? -
- users DSGroupUserResponse[]? - A list of users.
docusign.admin: ErrorDetails
Errors.
Fields
- 'error string? - The code for the error.
- error_description string? - A description of the error.
docusign.admin: ESignUserManagement
Methods to manage eSignature users in an account.
docusign.admin: ForceActivateMembershipRequest
Data to ctivate user memership
Fields
- site_id int - Site ID
docusign.admin: GroupRequest
A group for a user to belong to.
Fields
- id int - The ID of the group.
- name string? - The name of the group.
- 'type string? - The type of group. One of:
invalid
admin_group
everyone_group
custom_group
docusign.admin: IdentityProviderResponse
Information about a single identity provider.
Fields
- id string? - The unique ID of the identity provider.
- friendly_name string? - The human-readable name of the identity provider.
- auto_provision_users boolean? - If true, users who use this identity provider are automatically provisioned.
- 'type string? - The type of the identity provider. One of:
none
saml_20
saml_11
saml_10
ws_federation
open_id_connect
- saml_20 Saml2IdentityProviderResponse? - Information about a SAML 2.0 identity provider.
- links LinkResponse[]? - A list of useful URLs.
docusign.admin: IdentityProviders
Methods to get a list of identity providers.
docusign.admin: IdentityProvidersResponse
Fields
- identity_providers IdentityProviderResponse[]? -
docusign.admin: ImportsAccountSettingsBody
Fields
- 'file\.csv string - CSV file.
docusign.admin: LinkResponse
A link to a useful URL.
Fields
- rel string? - The kind of linked item.
- href string? - The URL of the linked item.
docusign.admin: MemberGroupResponse
And individual group responses,.
Fields
- id int? - The unique ID of the group.
- name string? - The name of the group.
- 'type string? - The type of group. One of:
invalid
admin_group
everyone_group
custom_group
docusign.admin: MemberGroupsResponse
A response about member groups. It contains the groups and paging information.
Fields
- groups MemberGroupResponse[]? - A list of the responses.
- paging PagingResponseProperties? - Contains information about paging through the results.
docusign.admin: MembershipResponse
Information about group membership.
Fields
- email string? - The email address.
- account_id string? - The ID of the account.
- external_account_id string? - The external account ID.
- account_name string? - The name of the account.
- is_external_account boolean? - If true, this is an external account.
- status string? - The status of the user's membership.
- permission_profile PermissionProfileResponse? - This object is an individual permission profile response.
- created_on string? - The date the user's account was created.
- groups MemberGroupResponse[]? - A list of groups the user is a member of in this account,
- is_admin boolean? - If true, the user has administration privileges on the account.
docusign.admin: NewAccountUserRequest
A new user request.
Fields
- permission_profile PermissionProfileRequest? - A permission profile.
- groups GroupRequest[]? - The new user's requested groups.
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- email string - The email address of the user.
- default_account_id string? - The account ID of the user's default account.
- language_culture string? - The language and culture of the user.
- Chinese Simplified:
zh_CN
- Chinese Traditional:
zh_TW
- Dutch:
nl
- English:
en
- French:
fr
- German:
de
- Italian:
it
- Japanese:
ja
- Korean:
ko
- Portuguese:
pt
- Portuguese Brazil:
pt_BR
- Russian:
ru
- Spanish:
es
- Chinese Simplified:
- selected_languages string? - Selected languages
- access_code string? - The access code that the user needs to activate an account.
- federated_status string? - The user's federated status. One of:
RemoveStatus
FedAuthRequired
FedAuthBypass
Evicted
- auto_activate_memberships boolean? - If true, the user's account is activated automatically.
docusign.admin: NewMultiProductUserAddRequest
Multi product user data
Fields
- product_permission_profiles ProductPermissionProfileRequest[] - Product permission profiles
- ds_groups DSGroupRequest[]? - DS groups
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- email string - The email address.
- default_account_id string? - Default account ID
- language_culture string? - The language and culture of the user.
- Chinese Simplified:
zh_CN
- Chinese Traditional:
zh_TW
- Dutch:
nl
- English:
en
- French:
fr
- German:
de
- Italian:
it
- Japanese:
ja
- Korean:
ko
- Portuguese:
pt
- Portuguese Brazil:
pt_BR
- Russian:
ru
- Spanish:
es
- Chinese Simplified:
- access_code string? - The access code that the user needs to activate an account.
- federated_status string? - The user's federated status. One of:
RemoveStatus
FedAuthRequired
FedAuthBypass
Evicted
- auto_activate_memberships boolean? - If true, the user's account is activated automatically.
docusign.admin: NewUserRequest
Information about a new user.
Fields
- accounts NewUserRequestAccountProperties[] - A list of accounts the user will belong to.
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- email string - The email address.
- default_account_id string? - The account ID of the user's default account.
- language_culture string? - The language and culture of the user.
- Chinese Simplified:
zh_CN
- Chinese Traditional:
zh_TW
- Dutch:
nl
- English:
en
- French:
fr
- German:
de
- Italian:
it
- Japanese:
ja
- Korean:
ko
- Portuguese:
pt
- Portuguese Brazil:
pt_BR
- Russian:
ru
- Spanish:
es
- Chinese Simplified:
- selected_languages string? - Selected languages
- access_code string? - The access code that the user needs to activate an account.
- federated_status string? - The user's federated status. One of:
RemoveStatus
FedAuthRequired
FedAuthBypass
Evicted
- auto_activate_memberships boolean? - If true, the user's account is activated automatically.
docusign.admin: NewUserRequestAccountProperties
An individual new account user.
Fields
- id string - The account ID.
- permission_profile PermissionProfileRequest? - A permission profile.
- groups GroupRequest[]? - The new user's requested groups.
- company_name string? - The user's company name.
- job_title string? - The user's job title.
docusign.admin: NewUserResponse
Information about a newly created user.
Fields
- id string? - The ID of the added user
- site_id int? - The site ID of the added user.
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- email string? - The primary email address of the user.
- language_culture string? - The language and culture of the user.
- Chinese Simplified:
zh_CN
- Chinese Traditional:
zh_TW
- Dutch:
nl
- English:
en
- French:
fr
- German:
de
- Italian:
it
- Japanese:
ja
- Korean:
ko
- Portuguese:
pt
- Portuguese Brazil:
pt_BR
- Russian:
ru
- Spanish:
es
- Chinese Simplified:
- federated_status string? - The user's federated status. One of:
RemoveStatus
FedAuthRequired
FedAuthBypass
Evicted
- accounts NewUserResponseAccountProperties[]? - A list of accounts the user belongs to.
docusign.admin: NewUserResponseAccountProperties
Information about a newly created user.
Fields
- id string? - The user's unique ID.
- site_id int? - The site ID of the account.
- permission_profile PermissionProfileResponse? - This object is an individual permission profile response.
- groups MemberGroupResponse[]? - A list of groups that the user belongs to.
- company_name string? - The user's company name.
- job_title string? - The job title of the user.
docusign.admin: OasirrErrordetails
Fields
- 'error string? - The error number.
- error_description string? - A longer description of the error.
docusign.admin: OasirrOrganizationaccountsettingserrordataresponse
Fields
- account_id string? - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- account_name string? -
- 'error string? - The error number.
- error_key string? -
- setting_key string? -
docusign.admin: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://account-d.docusign.com/oauth/token") - Refresh URL
docusign.admin: OetrErrordetails
Fields
- 'error string? - The error number.
- error_description string? - A longer description of the error.
docusign.admin: Organization
Methods for working with organizations.
docusign.admin: OrganizationAccountRequest
Fields
- account_id string - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
docusign.admin: OrganizationAccountResponse
Information about an account.
Fields
- id string? - The unique ID of the account.
- name string? - The name of the account.
- external_account_id int? - The external account ID.
- site_id int? - The site ID.
docusign.admin: OrganizationAccountSettingsImportRequestorResponse
Fields
- id string? -
- 'type string? -
- name string? -
- email string? - The email address.
docusign.admin: OrganizationAccountSettingsImportResponse
Fields
- created string? -
- last_modified string? -
- completed string? -
- expires string? -
- percent_completed int? -
- number_processed_accounts int? -
- number_unprocessed_accounts int? -
- results OrganizationAccountSettingsImportResultResponse[]? -
- success boolean? -
- skipped_settings_by_account record {}? -
- id string? -
- organization_id string? -
- status string? - Status.
- 'type string? -
- requestor OrganizationAccountSettingsImportRequestorResponse? -
docusign.admin: OrganizationAccountSettingsImportResultResponse
Fields
- id string? -
- site_id int? -
- url string? -
- number_processed_accounts int? -
- error_details OasirrErrordetails? -
- processing_issues_by_account OasirrOrganizationaccountsettingserrordataresponse[]? -
- number_unprocessed_accounts int? -
docusign.admin: OrganizationAccountsRequest
Fields
- accounts OrganizationAccountRequest[]? -
docusign.admin: OrganizationExportAccount
Fields
- account_id string? - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
docusign.admin: OrganizationExportDomain
Fields
- domain string? -
docusign.admin: OrganizationExportRequest
Enables you to specify the kind of export request.
Fields
- 'type string? - The type of export requested. One of:
organization_domain_users_export
: All users of the reserved domains.organization_external_memberships_export
: Users whose email address domain is not linked to the organization.organization_memberships_export
: Every user in every account in the organization. Users in multiple accounts will appear more than once.organization_account_settings_export
: This value only applies to requests to export account settings.
- accounts OrganizationExportAccount[]? - Organization export accounts
- domains OrganizationExportDomain[]? - Organization export domains
docusign.admin: OrganizationExportRequestorResponse
Fields
- name string? -
- id string? -
- 'type string? -
- email string? - The email address.
docusign.admin: OrganizationExportResponse
Fields
- id string? -
- 'type string? -
- requestor OrganizationExportRequestorResponse? -
- created string? -
- last_modified string? -
- completed string? -
- expires string? -
- status string? - Status.
- selected_accounts OrgExportSelectedAccount[]? -
- selected_domains OrgExportSelectedDomain[]? -
- metadata_url string? -
- percent_completed int? -
- number_rows int? -
- size_bytes int? -
- results OrganizationExportTaskResponse[]? -
- success boolean? -
docusign.admin: OrganizationExportsResponse
Fields
- exports OrganizationExportResponse[]? -
docusign.admin: OrganizationExportTaskResponse
Fields
- id string? -
- site_id int? -
- url string? -
- number_rows int? -
- size_bytes int? -
- error_details OetrErrordetails? -
docusign.admin: OrganizationImportResponse
Fields
- id string? -
- 'type string? -
- requestor OrganizationImportResponseRequestor? -
- created string? -
- last_modified string? -
- status string? - Status.
- user_count int? -
- processed_user_count int? -
- added_user_count int? -
- updated_user_count int? -
- closed_user_count int? -
- no_action_required_user_count int? -
- error_count int? -
- warning_count int? -
- invalid_column_headers string? -
- imports_not_found_or_not_available_for_accounts string? -
- imports_failed_for_accounts string? -
- imports_timed_out_for_accounts string? -
- imports_not_found_or_not_available_for_sites string? -
- imports_failed_for_sites string? -
- imports_timed_out_for_sites string? -
- file_level_error_rollups OrganizationImportResponseErrorRollup[]? -
- user_level_error_rollups OrganizationImportResponseErrorRollup[]? -
- user_level_warning_rollups OrganizationImportResponseWarningRollup[]? -
- has_csv_results boolean? -
- results_uri string? -
docusign.admin: OrganizationImportResponseErrorRollup
Fields
- error_type string? -
- count int? -
docusign.admin: OrganizationImportResponseRequestor
Fields
- name string? -
- id string? -
- 'type string? -
- email string? - The email address.
docusign.admin: OrganizationImportResponseWarningRollup
Fields
- warning_type string? -
- count int? -
docusign.admin: OrganizationImportsResponse
Fields
- imports OrganizationImportResponse[]? -
docusign.admin: OrganizationResponse
Information about an individual organization.
Fields
- id string? - The ID of the organization.
- name string? - The name of the organization.
- description string? - A description of the organization.
- default_account_id string? - The default account ID of the organization.
- default_permission_profile_id int? - The default permission profile ID of the organization.
- created_on string? - The date the organization's account was created.
- created_by string? - The user who created the organization account.
- last_modified_on string? - The date the organization's account was last updated.
- last_modified_by string? - The user who last updated the organization's account.
- accounts OrganizationAccountResponse[]? - A list of organization accounts.
- users OrganizationSimpleIdObject[]? - A list of the organization accounts users.
- reserved_domains DomainResponse[]? - A list of reserved domains for the organization.
- identity_providers IdentityProvidersResponse[]? - A list of identity providers for the organization.
- links LinkResponse[]? - A list of links for the organization.
docusign.admin: OrganizationSalesforceAccountManagersResponse
Fields
- account_id string? - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- account_name string? -
- account_type string? -
- account_owner OsamrContact? -
- account_manager OsamrContact? -
- parent_account OrganizationSalesforceAccountManagersResponse? -
docusign.admin: OrganizationSimpleIdObject
An ID object.
Fields
- id string? - The ID.
docusign.admin: OrganizationsResponse
Organization list.
Fields
- organizations OrganizationResponse[]? - A list of organizations of which the authenticated user is a member.
docusign.admin: OrganizationUserResponse
Information about a user.
Fields
- id string? - The user's unique ID.
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- user_status string? - The user's status. One of:
active
created
closed
- membership_status string? - The user's membership status. One of:
activation_required
activation_sent
active
closed
disabled
- email string? - The email address.
- created_on string? - The date the user's account was created.
- membership_created_on string? - The date on which the user became a member of the organization.
- ds_groups DSGroupResponse[]? - DS group response
- membership_id string? - Membership ID
docusign.admin: OrganizationUsersResponse
A response containing information about users.
Fields
- users OrganizationUserResponse[]? - A list of users.
- paging PagingResponseProperties? - Contains information about paging through the results.
docusign.admin: OrgExportSelectedAccount
Fields
- account_id string? - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
docusign.admin: OrgExportSelectedDomain
Fields
- domain string? -
docusign.admin: OrgReportConfigurationResponse
Fields
- is_account_limit_disabled boolean? -
- custom_dates_enabled boolean? -
- enabled_report_types int[]? -
docusign.admin: OrgReportCreateResponse
Fields
- report_correlation_id string? -
docusign.admin: OrgReportListResponse
Fields
- reports OrgreportlistresponseOrgreport[]? -
docusign.admin: OrgreportlistresponseOrgreport
Fields
- complete boolean? -
- report_correlation_id string? -
- site_id int? -
- report_id string? -
- requestor OrgreportlistresponseRequestor? -
- created_on string? -
- account_export_count int? -
- url string? -
- report_type_id string? -
- report_date_range string? -
- custom_start_date string? -
- custom_end_date string? -
docusign.admin: OrgreportlistresponseRequestor
Fields
- id string? -
- name string? -
docusign.admin: OrgReportRequest
Fields
- report_type string? -
- report_date_range string? -
- account_ids string[]? -
- custom_start_date string? -
- custom_end_date string? -
docusign.admin: OsamrContact
Fields
- name string? -
- email string? - The email address.
- title string? -
docusign.admin: PagingResponseProperties
Contains information about paging through the results.
Fields
- result_set_size int? - The number of items in a result set (page)
- result_set_start_position int? - The index position of the first result in this set
- result_set_end_position int? - The index position of the last result in this set
- total_set_size int? - The total number of results
- next string? - A URL to the next set of results
- previous string? - A URL to the previous set of results
docusign.admin: PermissionProfileRequest
A permission profile.
Fields
- id int - The ID of the permission profile.
- name string? - The name of the permission profile.
docusign.admin: PermissionProfileResponse
This object is an individual permission profile response.
Fields
- id int? - The ID of the permission profile.
- name string? - The name of the permission profile.
Example:
DocuSign Sender
docusign.admin: Permissionprofileresponse21
Fields
- permission_profile_id string? -
- permission_profile_name string? -
docusign.admin: PermissionsResponse
A list of permission profiles for a given account.
Fields
- permissions PermissionProfileResponse[]? - An array of permission profile responses.
docusign.admin: ProductPermissionProfileRequest
Fields
- product_id string -
- permission_profile_id string -
docusign.admin: ProductPermissionProfileResponse
Fields
- product_id string? -
- product_name string? -
- permission_profiles Permissionprofileresponse21[]? -
- error_message string? -
docusign.admin: ProductPermissionProfilesRequest
Product permission profile data
Fields
- product_permission_profiles ProductPermissionProfileRequest[] - Prouct permission profiles
docusign.admin: ProductPermissionProfilesResponse
Fields
- product_permission_profiles ProductPermissionProfileResponse[]? -
docusign.admin: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
docusign.admin: RemoveDSGroupUsersResponse
Fields
- is_success boolean? -
- failed_users DSGroupUserResponse[]? -
docusign.admin: RequiredAttributeMappingResponse
A single attribute mapping response.
Fields
- required_attribute_id int? - The unique ID of the attribute.
- required_attribute_name string? - The name of the attribute.
- required_attribute_friendly_name string? - The human-readable name of the attribute.
- substitute_attribute_name string? - The name of the substitute attribute.
docusign.admin: ReservedDomains
Methods to get a list of reserved domains.
docusign.admin: Saml2IdentityProviderResponse
Information about a SAML 2.0 identity provider.
Fields
- issuer string? - The name of the certificate issuer.
- settings SettingResponse[]? - A list of settings.
- certificates CertificateResponse[]? - A list of certificates responses.
- attribute_mappings RequiredAttributeMappingResponse[]? - A list of attribute mappings.
docusign.admin: SettingResponse
A key/value list of settings.
Fields
- 'key string? - The key of the setting.
- value record {}? - The value of the setting.
- 'type string? - The type of the setting. One of:
unknown
guid
text
integer
boolean
datetime
enumeration
docusign.admin: UpdateMembershipRequest
A request to update group membership.
Fields
- account_id string - The user's unique ID.
- permission_profile PermissionProfileRequest? - A permission profile.
- groups GroupRequest[]? - The user's requested groups.
- company_name string? - The user's company name.
- job_title string? - The user's job title.
- send_activation boolean? - If true, send an activation request after the update.
- access_code string? - The access code that the user needs to activate an account.
docusign.admin: UpdateResponse
A response.
Fields
- status string? - The status of the request.
docusign.admin: UpdateUserEmailRequest
And individual change of email.
Fields
- id string - The ID of the users whose email address you want to change.
- site_id int - The site ID.
- email string - The new email address.
docusign.admin: UpdateUserRequest
Request to change a user's information.
Fields
- id string - The user's unique ID.
- site_id int - The site ID.
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- email string? - The email address.
- default_account_id string? - The account ID of the user's default account.
- language_culture string? - The language and culture of the user.
- Chinese Simplified:
zh_CN
- Chinese Traditional:
zh_TW
- Dutch:
nl
- English:
en
- French:
fr
- German:
de
- Italian:
it
- Japanese:
ja
- Korean:
ko
- Portuguese:
pt
- Portuguese Brazil:
pt_BR
- Russian:
ru
- Spanish:
es
- Chinese Simplified:
- selected_languages string? - Selected languages
- federated_status string? - The user's federated status. One of:
RemoveStatus
FedAuthRequired
FedAuthBypass
Evicted
- force_password_change boolean? - If true, the user will be required to change the account password.
- memberships UpdateMembershipRequest[]? - A list of group membership requests.
- device_verification_enabled boolean? - Device verification enabled
docusign.admin: UpdateUsersEmailRequest
A change email request.
Fields
- users UpdateUserEmailRequest[]? - A list of users whose email address to change.
docusign.admin: UpdateUsersRequest
A list of users whose information you want to change.
Fields
- users UpdateUserRequest[]? - A list of users whose information you want to change.
docusign.admin: UserDrilldownResponse
Information about a user.
Fields
- id string? - The user's unique ID.
- site_id int? - The site ID of the organization.
- site_name string? - The site name of the account.
- user_name string? - The full name of the user.
- first_name string? - The user's first name.
- last_name string? - The user's last name.
- user_status string? - The user's status. One of:
active
created
closed
- default_account_id string? - The ID of the user's default account.
- default_account_name string? - The name of the user's default account.
- language_culture string? - The language and culture of the user.
- Chinese Simplified:
zh_CN
- Chinese Traditional:
zh_TW
- Dutch:
nl
- English:
en
- French:
fr
- German:
de
- Italian:
it
- Japanese:
ja
- Korean:
ko
- Portuguese:
pt
- Portuguese Brazil:
pt_BR
- Russian:
ru
- Spanish:
es
- Chinese Simplified:
- selected_languages string? - Selected language
- federated_status string? - The user's federated status. One of:
RemoveStatus
FedAuthRequired
FedAuthBypass
Evicted
- is_organization_admin boolean? - If true, the user has organization administration privileges.
- created_on string? - The date the user's account was created.
- last_login string? - The last time the user logged in.
- memberships MembershipResponse[]? - A list of organizations that have groups that the user is a member of.
- identities UserIdentityResponse[]? - A list of identities associated with the user.
- device_verification_enabled boolean? - Device verification enabled
docusign.admin: UserExport
Methods for exporting a user list.
docusign.admin: UserIdentityRequest
User identity,
Fields
- id string? - The ID of the identity.
docusign.admin: UserIdentityResponse
Results of deleting a user identity.
Fields
- id string? - The ID of the result.
- provider_id string? - The ID of the identity provider.
- user_id string? - The user ID.
- immutable_id string? - A unique ID to identify the removed user.
- error_details ErrorDetails? - Errors.
docusign.admin: UserImport
Methods to import users.
docusign.admin: UserProductPermissionProfilesResponse
Fields
- user_id string? -
- account_id string? - Select users that are members of the specified account. At least one of
email
,account_id
ororganization_reserved_domain_id
must be specified.
- product_permission_profiles ProductPermissionProfileResponse[]? -
docusign.admin: Users
Methods to manage users in an account.
docusign.admin: UsersDrilldownResponse
Information about a list of users.
Fields
- users UserDrilldownResponse[]? - A list of users.
docusign.admin: UsersUpdateResponse
The results of changing a user's information.
Fields
- success boolean? - If true, the request to change user information succeeded.
- users UserUpdateResponse[]? - A list of users whose email addresses have been updated.
docusign.admin: UserUpdateResponse
Error result of attempting to change a user's email address.
Fields
- id string? - The ID of the user whose email address has been updated.
- site_id int? - The site ID.
- email string? - The email address.
- error_details ErrorDetails? - Errors.
Import
import ballerinax/docusign.admin;
Metadata
Released date: over 1 year ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 3
Current verison: 2
Weekly downloads
Keywords
Content & Files/Documents
Cost/Freemium
Contributors