disqus
Module disqus
API
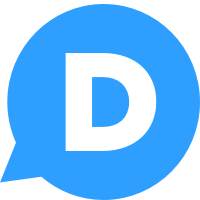
ballerinax/disqus Ballerina library
Overview
This is a generated connector for Disqus API v3 OpenAPI specification. Disqus is a service for web comments and discussions. Disqus makes commenting easier and more interactive, helping publishers power online discussions.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Disqus account
- Obtain tokens by following this guide
Quickstart
To use the SupportBee connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/disqus
module into the Ballerina project.
import ballerinax/disqus;
Step 2: Create a new connector instance
Create a disqus:ApiKeysConfig
with the Access token, API key & API secret obtained, and initialize the connector with it.
disqus:ApiKeysConfig config = { accessToken: "<ACCESS_TOKEN>", apiKey: "<API_KEY>", apiSecret: "<API_SECRET>" } disqus:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get a list of categories within a forum using the connector.
Returns a list of categories within a forum.
public function main() returns error? { disqus:Categories response = check baseClient->listCategories("forum"); log:printInfo(response.toJsonString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
disqus: Client
This is a generated connector for Disqus API v1 OpenAPI specification. Disqus is a service for web comments and discussions. Disqus makes commenting easier and more interactive, helping publishers power online discussions.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Disqus account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://disqus.com/api/3.0" - URL of the target service
listCategories
function listCategories(string forum, string? sinceId, string? cursor, string? 'limit, string? 'order) returns Categories|error
Returns a list of categories within a forum.
Parameters
- forum string - Looks up a forum by ID (aka short name)
- sinceId string? (default ()) - since_id. Defaults to null.
- cursor string? (default ()) - cursor. Defaults to null.
- 'limit string? (default ()) - limit. Defaults to 25. Maximum value of 100.
- 'order string? (default ()) - order. Defaults to 'asc'. Choices: asc, desc.
Return Type
- Categories|error - An object with a single property response which is an array of Category objects.
getCategoryDetails
Returns category details.
Parameters
- category string - Looks up a category by ID.
getForumDetails
Returns forum details.
Parameters
- forum string - Looks up a forum by ID (aka short name)
- attach string? (default ()) - Choices: followsForum, forumCanDisableAds, forumForumCategory, counters, forumDaysAlive, forumFeatures, forumIntegration, forumNewPolicy, forumPermissions. Defaults to [].
- related string? (default ()) - You may specify relations to include with your response. Choices: author. Defaults to [].
listPosts
function listPosts(string? category, string? end, string? sortType, string? thread, string? forum, string? 'start, string? since, string? related, string? cursor, int? 'limit, string? filters, string? query, string? include, string? 'order) returns Posts|error
Returns a list of posts ordered by the date created.
Parameters
- category string? (default ()) - Looks up a category by ID. Defaults to null.
- end string? (default ()) - Unix timestamp (or ISO datetime standard). Defaults to null.
- sortType string? (default ()) - Choices: date, priority. Defaults to 'date'.
- thread string? (default ()) - Looks up a thread by ID. Defaults to null.
- forum string? (default ()) - Defaults to all forums you moderate. Use :all to retrieve all forums. Defaults to null.
- 'start string? (default ()) - Unix timestamp (or ISO datetime standard). Defaults to null.
- since string? (default ()) - Unix timestamp (or ISO datetime standard). Defaults to null.
- related string? (default ()) - You may specify relations to include with your response. Choices: forum, thread. Defaults to [].
- cursor string? (default ()) - Cursor. Defaults to null.
- 'limit int? (default ()) - Limit. Maximum value of 100. Defaults to 25.
- filters string? (default ()) - Filters. Defaults to []. Valid values are Is_Anonymous, Has_Link, Has_Low_Rep_Author, Has_Bad_Word, Is_Flagged, No_Issue, Is_Toxic, Modified_By_Rule, Shadow_Banned, Has_Media, Is_At_Flag_Limit, Shadow_Banned_Global.
- query string? (default ()) - Query. Defaults to null.
- include string? (default ()) - Choices: unapproved, approved, spam, deleted, flagged, highlighted. Defaults to [ 'approved' ].
- 'order string? (default ()) - Choices: asc, desc. Defaults to 'desc'
Return Type
getPostDetails
Returns information about a post.
Parameters
- post string - Looks up a post by ID.
- related string? (default ()) - You may specify relations to include with your response. Choices: forum, thread. Defaults to [].
listThreads
function listThreads(string? category, string? forum, string? thread, string? author, string? since, string? related, string? cursor, string? attach, int? 'limit, string? include, string? 'order) returns Threads|error
Returns a list of threads sorted by the date created.
Parameters
- category string? (default ()) - Looks up a category by ID. Defaults to null.
- forum string? (default ()) - Looks up a forum by ID (aka short name). Defaults to null.
- thread string? (default ()) - Looks up a thread by ID. You may pass us the 'ident' query type instead of an ID by including 'forum'. You may pass us the 'link' query type to filter by URL. You must pass the 'forum' if you do not have the Pro API Access addon. Defaults to null.
- author string? (default ()) - Looks up a user by ID. You may look up a user by username using the 'username' query type. Defaults to null.
- since string? (default ()) - Unix timestamp (or ISO datetime standard). Defaults to null.
- related string? (default ()) - You may specify relations to include with your response. Choices: forum, author, category. Defaults to [].
- cursor string? (default ()) - Cursor. Defaults to null.
- attach string? (default ()) - Attach. Defaults to [].
- 'limit int? (default ()) - Limit. Maximum value of 100. Defaults to 25.
- include string? (default ()) - Choices: open, closed, killed. Defaults to [ 'open', 'closed' ]
- 'order string? (default ()) - Choices: asc, desc. Defaults to 'desc'.
Return Type
getThreadDetails
function getThreadDetails(string thread, string? forum, string? related, string? attach) returns DisqusThread|error
Returns thread details.
Parameters
- thread string - Looks up a thread by ID. You may pass us the 'ident' or 'link' query types instead of an ID by including 'forum'.
- forum string? (default ()) - Looks up a forum by ID (aka short name). Defaults to null.
- related string? (default ()) - You may specify relations to include with your response. Choices: forum, author, category. Defaults to [].
- attach string? (default ()) - Choices: topics. Defaults to [].
Return Type
- DisqusThread|error - An object with a single property response which is a Thread object.
Records
disqus: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- accessToken string - Represents API Key
access_token
- apiKey string - Represents API Key
api_key
- apiSecret string - Represents API Key
api_secret
disqus: Author
Author
Fields
- username string? - Username
- about string? - About
- name string? - Name
- url string? - Url
- isFollowing boolean? - Is Following
- isFollowedBy boolean? - Is Followed By
- profileUrl string? - Profile Url
- avatar Avatar? - Avatar
- id string? - Author ID
- isAnonymous boolean? - Is Anonymous
- email string? - Email
disqus: Avatar
Avatar
Fields
- permalink string? - Permalink
- cache string? - Cache
disqus: Categories
An object with a single property response which is an array of Category objects.
Fields
- cursor Cursor? - Cursor
- code decimal? - Code
- response CategoryObject[]? - An array of Category objects.
disqus: Category
An object with a single property response which is a Category object.
Fields
- code decimal? - Code
- response CategoryObject? - Category
disqus: CategoryObject
Category
Fields
- id string? - Category ID
- forum string? - Forum
- 'order decimal? - Order
- isDefault boolean? - Is Default
- title string? - Title
disqus: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
disqus: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
disqus: Cursor
Cursor
Fields
- prev string? - Prev
- hasNext boolean? - Has next
- next string? - Next
- hasPrev boolean? - Has prev
- total string? - Total
- id string? - ID
- more boolean? - More
disqus: DisqusThread
An object with a single property response which is a Thread object.
Fields
- code decimal? - Code
- response ThreadObject? - Thread
disqus: Favicon
Favicon
Fields
- permalink string? - Permalink
- cache string? - Cache
disqus: Forum
An object with a single property response which is a Forum object.
Fields
- code decimal? - Code
- response ForumObject? - Forum
disqus: ForumObject
Forum
Fields
- id string? - Forum ID
- name string? - Forum name
- founder string? - Founder
- favicon Favicon? - Favicon
disqus: Post
An object with a single property response which is a Post object.
Fields
- code decimal? - Code
- response PostObject? - Category
disqus: PostObject
Category
Fields
- isJuliaFlagged boolean? - Is Julia Flagged
- isFlagged boolean? - Is Flagged
- forum string? - Forum
- parent decimal? - Is Default
- author Author? - Author
- isApproved boolean? - Is Approved
- dislikes decimal? - Dislikes
- raw_message string? - Raw message
- id string? - Post ID
- thread string? - Thread
- points decimal? - Points
- createdAt string? - Created At
- isEdited boolean? - Is Edited
- message string? - Message
- isHighlighted boolean? - Is Highlighted
- ipAddress string? - IP Address
- isSpam boolean? - Is Spam
- isDeleted boolean? - Is Deleted
- likes decimal? - Likes
disqus: Posts
An object with a single property response which is an array of Post objects.
Fields
- cursor Cursor? - Cursor
- code decimal? - Code
- response PostObject[]? - An array of Post objects.
disqus: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
disqus: ThreadObject
Thread
Fields
- category string? - Category ID
- reactions decimal? - Reactions
- forum string? - Forum
- title string? - Title
- dislikes decimal? - Dislikes
- isDeleted boolean? - Is Deleted
- author string? - Author
- userScore decimal? - UserScore
- id string? - Thread ID
- isClosed boolean? - Is Closed
- posts decimal? - Posts
- link string? - Link
- likes decimal? - Likes
- message string? - Message
- ipAddress string? - IP Address
- slug string? - Slug
- createdAt string? - Created At
disqus: Threads
An object with a single property response which is an array of Thread objects.
Fields
- cursor Cursor? - Cursor
- code decimal? - Code
- response ThreadObject[]? - An array of Thread objects.
Import
import ballerinax/disqus;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 2
Weekly downloads
Keywords
Website & App Building/Website Builders
Cost/Freemium
Contributors
Dependencies