discord
Module discord
API
Definitions
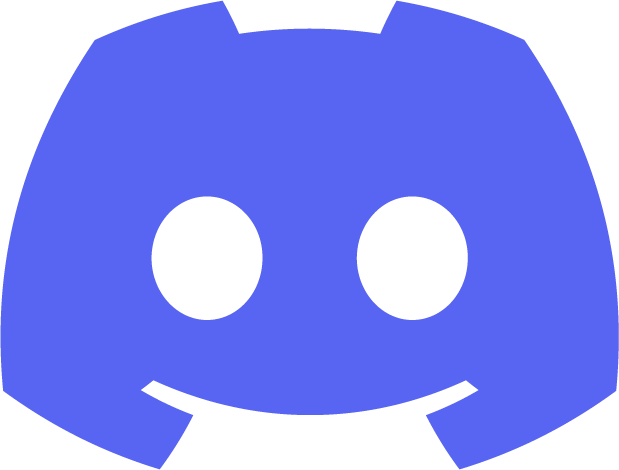
ballerinax/discord Ballerina library
Overview
Discord is a popular communication platform designed for creating communities and facilitating real-time messaging, voice, and video interactions over the internet.
The Ballerina Discord connector offers APIs to connect and interact with the Discord REST API v10.
Setup guide
Follow these steps to create a Discord developer account.
Step 1: Login to Discord developer page
-
Visit Discord developer portal by logging into your Discord account.
-
If you do not have a Discord account already, create a new discord account by clicking on the
Register
hyperlink below theLog In
button when opening the Discord developer page. -
Complete the account creation process by including the relevant information in the given fields.
Step 2: Make a new Discord application
-
Once in the Discord developer portal is open, click on the
New Application
button as displayed above to start the process.
Step 3: Name the Discord Application
-
Proceed by giving the Discord Application a name and click on the terms of service.
-
Finally complete the naming process by clicking on the
next
button.
Step 4: Obtain the Client ID and Client Secret
-
Under the
OAuth2
section found on the left-sided list, locate the Client's Information as shown on the screen. To implement the functionalities provided by Discord's API, you will need the Client ID and Client Secret.
Quickstart
To use the discord
connector in your Ballerina application, modify the .bal
file as follows:
Step 1: Import the module
Import the discord
module.
import ballerinax/discord;
Step 2: Instantiate a new connector
Create a discord:ConnectionConfig
with the obtained OAuth2.0 Client Credentials and initialize the connector with it.
Apps must receive approval from users installing them to perform actions within Discord. To enable these functions, specific scopes must be defined. These scopes are outlined in the OAuth2 Scopes documentation.
configurable string clientId = ?; configurable string clientSecret = ?; configurable string[] scopes = ?; discord:Client discord = check new({ auth: { clientId, clientSecret, scopes } });
Step 3: Invoke the connector operation
Now, utilize the available connector operations.
Return linked third-party accounts of the user
public function main() returns error? { ConnectedAccountResponse[] connectedAccounts = check discord->/users/\@me/connections(); }
Step 4: Run the Ballerina application
bal run
Examples
The Discord
connector provides practical examples illustrating usage in various scenarios. Explore these examples, covering the following use cases:
- Automated Event Reminders - This use case illustrates how the Discord API can be leveraged to create a scheduled event in a Discord server and automate daily reminders about this event across all channels within the server.
- Automated Role Assignment Based on Reactions - This use case illustrates the utilization of the Discord API to assign roles to members based on their interests, enabling them to gain roles by reacting to designated messages.
Clients
discord: Client
Preview of the Discord v10 HTTP API specification. See https://discord.com/developers/docs for more details.
Constructor
Gets invoked to initialize the connector
.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://discord.com/api/v10" - URL of the target service
delete applications/[string application_id]/commands/[string command_id]
function delete applications/[string application_id]/commands/[string command_id](map<string|string[]> headers) returns Response|error
delete_application_command
delete applications/[string application_id]/entitlements/[string entitlement_id]
function delete applications/[string application_id]/entitlements/[string entitlement_id](map<string|string[]> headers) returns Response|error
delete_entitlement
delete applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id]
function delete applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id](map<string|string[]> headers) returns Response|error
delete_guild_application_command
delete channels/[string channel_id]
function delete channels/[string channel_id](map<string|string[]> headers) returns inline_response_200_6|error
delete_channel
Return Type
- inline_response_200_6|error - 200 response for delete_channel
delete channels/[string channel_id]/messages/[string message_id]
function delete channels/[string channel_id]/messages/[string message_id](map<string|string[]> headers) returns Response|error
delete_message
delete channels/[string channel_id]/messages/[string message_id]/reactions
function delete channels/[string channel_id]/messages/[string message_id]/reactions(map<string|string[]> headers) returns Response|error
delete_all_message_reactions
delete channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name]
function delete channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name](map<string|string[]> headers) returns Response|error
delete_all_message_reactions_by_emoji
delete channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name]/[string user_id]
function delete channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name]/[string user_id](map<string|string[]> headers) returns Response|error
delete_user_message_reaction
delete channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name]/@me
function delete channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name]/\@me(map<string|string[]> headers) returns Response|error
delete channels/[string channel_id]/permissions/[string overwrite_id]
function delete channels/[string channel_id]/permissions/[string overwrite_id](map<string|string[]> headers) returns Response|error
delete_channel_permission_overwrite
delete channels/[string channel_id]/pins/[string message_id]
function delete channels/[string channel_id]/pins/[string message_id](map<string|string[]> headers) returns Response|error
unpin_message
delete channels/[string channel_id]/recipients/[string user_id]
function delete channels/[string channel_id]/recipients/[string user_id](map<string|string[]> headers) returns Response|error
delete_group_dm_user
delete channels/[string channel_id]/thread-members/[string user_id]
function delete channels/[string channel_id]/thread\-members/[string user_id](map<string|string[]> headers) returns Response|error
delete_thread_member
delete channels/[string channel_id]/thread-members/@me
function delete channels/[string channel_id]/thread\-members/\@me(map<string|string[]> headers) returns Response|error
delete guilds/[string guild_id]
delete_guild
delete guilds/[string guild_id]/auto-moderation/rules/[string rule_id]
function delete guilds/[string guild_id]/auto\-moderation/rules/[string rule_id](map<string|string[]> headers) returns Response|error
delete_auto_moderation_rule
delete guilds/[string guild_id]/bans/[string user_id]
function delete guilds/[string guild_id]/bans/[string user_id](map<string|string[]> headers) returns Response|error
unban_user_from_guild
delete guilds/[string guild_id]/emojis/[string emoji_id]
function delete guilds/[string guild_id]/emojis/[string emoji_id](map<string|string[]> headers) returns Response|error
delete_guild_emoji
delete guilds/[string guild_id]/integrations/[string integration_id]
function delete guilds/[string guild_id]/integrations/[string integration_id](map<string|string[]> headers) returns Response|error
delete_guild_integration
delete guilds/[string guild_id]/members/[string user_id]
function delete guilds/[string guild_id]/members/[string user_id](map<string|string[]> headers) returns Response|error
delete_guild_member
delete guilds/[string guild_id]/members/[string user_id]/roles/[string role_id]
function delete guilds/[string guild_id]/members/[string user_id]/roles/[string role_id](map<string|string[]> headers) returns Response|error
delete_guild_member_role
delete guilds/[string guild_id]/roles/[string role_id]
function delete guilds/[string guild_id]/roles/[string role_id](map<string|string[]> headers) returns Response|error
delete_guild_role
delete guilds/[string guild_id]/scheduled-events/[string guild_scheduled_event_id]
function delete guilds/[string guild_id]/scheduled\-events/[string guild_scheduled_event_id](map<string|string[]> headers) returns Response|error
delete_guild_scheduled_event
delete guilds/[string guild_id]/stickers/[string sticker_id]
function delete guilds/[string guild_id]/stickers/[string sticker_id](map<string|string[]> headers) returns Response|error
delete_guild_sticker
delete guilds/[string guild_id]/templates/[string code]
function delete guilds/[string guild_id]/templates/[string code](map<string|string[]> headers) returns GuildTemplateResponse|error
delete_guild_template
Return Type
- GuildTemplateResponse|error - 200 response for delete_guild_template
delete invites/[string code]
function delete invites/[string code](map<string|string[]> headers) returns inline_response_200_2|error
invite_revoke
Return Type
- inline_response_200_2|error - 200 response for invite_revoke
delete stage-instances/[string channel_id]
function delete stage\-instances/[string channel_id](map<string|string[]> headers) returns Response|error
delete users/@me/guilds/[string guild_id]
function delete users/\@me/guilds/[string guild_id](map<string|string[]> headers) returns Response|error
leave_guild
delete webhooks/[string webhook_id]
delete_webhook
delete webhooks/[string webhook_id]/[string webhook_token]
function delete webhooks/[string webhook_id]/[string webhook_token](map<string|string[]> headers) returns Response|error
delete_webhook_by_token
delete webhooks/[string webhook_id]/[string webhook_token]/messages/[string message_id]
function delete webhooks/[string webhook_id]/[string webhook_token]/messages/[string message_id](map<string|string[]> headers, *Delete_webhook_messageQueries queries) returns Response|error
delete_webhook_message
Parameters
- queries *Delete_webhook_messageQueries - Queries to be sent with the request
delete webhooks/[string webhook_id]/[string webhook_token]/messages/@original
function delete webhooks/[string webhook_id]/[string webhook_token]/messages/\@original(map<string|string[]> headers, *Delete_original_webhook_messageQueries queries) returns Response|error
Parameters
- queries *Delete_original_webhook_messageQueries -
get applications/[string application_id]
function get applications/[string application_id](map<string|string[]> headers) returns PrivateApplicationResponse|error
get_application
Return Type
- PrivateApplicationResponse|error - 200 response for get_application
get applications/[string application_id]/commands
function get applications/[string application_id]/commands(map<string|string[]> headers, *List_application_commandsQueries queries) returns ApplicationCommandResponse[]|error
list_application_commands
Parameters
- queries *List_application_commandsQueries - Queries to be sent with the request
Return Type
- ApplicationCommandResponse[]|error - 200 response for list_application_commands
get applications/[string application_id]/commands/[string command_id]
function get applications/[string application_id]/commands/[string command_id](map<string|string[]> headers) returns ApplicationCommandResponse|error
get_application_command
Return Type
- ApplicationCommandResponse|error - 200 response for get_application_command
get applications/[string application_id]/entitlements
function get applications/[string application_id]/entitlements(map<string|string[]> headers, *Get_entitlementsQueries queries) returns EntitlementResponse[]|error
get_entitlements
Parameters
- queries *Get_entitlementsQueries - Queries to be sent with the request
Return Type
- EntitlementResponse[]|error - 200 response for get_entitlements
get applications/[string application_id]/entitlements/[string entitlement_id]
function get applications/[string application_id]/entitlements/[string entitlement_id](map<string|string[]> headers) returns EntitlementResponse|error
get_entitlement
Return Type
- EntitlementResponse|error - 200 response for get_entitlement
get applications/[string application_id]/guilds/[string guild_id]/commands
function get applications/[string application_id]/guilds/[string guild_id]/commands(map<string|string[]> headers, *List_guild_application_commandsQueries queries) returns ApplicationCommandResponse[]|error
list_guild_application_commands
Parameters
- queries *List_guild_application_commandsQueries - Queries to be sent with the request
Return Type
- ApplicationCommandResponse[]|error - 200 response for list_guild_application_commands
get applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id]
function get applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id](map<string|string[]> headers) returns ApplicationCommandResponse|error
get_guild_application_command
Return Type
- ApplicationCommandResponse|error - 200 response for get_guild_application_command
get applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id]/permissions
function get applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id]/permissions(map<string|string[]> headers) returns CommandPermissionsResponse|error
get_guild_application_command_permissions
Return Type
- CommandPermissionsResponse|error - 200 response for get_guild_application_command_permissions
get applications/[string application_id]/guilds/[string guild_id]/commands/permissions
function get applications/[string application_id]/guilds/[string guild_id]/commands/permissions(map<string|string[]> headers) returns CommandPermissionsResponse[]|error
list_guild_application_command_permissions
Return Type
- CommandPermissionsResponse[]|error - 200 response for list_guild_application_command_permissions
get applications/[string application_id]/role-connections/metadata
function get applications/[string application_id]/role\-connections/metadata(map<string|string[]> headers) returns ApplicationRoleConnectionsMetadataItemResponse[]|error
get_application_role_connections_metadata
Return Type
- ApplicationRoleConnectionsMetadataItemResponse[]|error - 200 response for get_application_role_connections_metadata
get applications/@me
function get applications/\@me(map<string|string[]> headers) returns PrivateApplicationResponse|error
get channels/[string channel_id]
function get channels/[string channel_id](map<string|string[]> headers) returns inline_response_200_6|error
get_channel
Return Type
- inline_response_200_6|error - 200 response for get_channel
get channels/[string channel_id]/invites
function get channels/[string channel_id]/invites(map<string|string[]> headers) returns anydata[]|error
list_channel_invites
Return Type
- anydata[]|error - 200 response for list_channel_invites
get channels/[string channel_id]/messages
function get channels/[string channel_id]/messages(map<string|string[]> headers, *List_messagesQueries queries) returns MessageResponse[]|error
list_messages
Parameters
- queries *List_messagesQueries - Queries to be sent with the request
Return Type
- MessageResponse[]|error - 200 response for list_messages
get channels/[string channel_id]/messages/[string message_id]
function get channels/[string channel_id]/messages/[string message_id](map<string|string[]> headers) returns MessageResponse|error
get_message
Return Type
- MessageResponse|error - 200 response for get_message
get channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name]
function get channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name](map<string|string[]> headers, *List_message_reactions_by_emojiQueries queries) returns UserResponse[]|error
list_message_reactions_by_emoji
Parameters
- queries *List_message_reactions_by_emojiQueries - Queries to be sent with the request
Return Type
- UserResponse[]|error - 200 response for list_message_reactions_by_emoji
get channels/[string channel_id]/pins
function get channels/[string channel_id]/pins(map<string|string[]> headers) returns MessageResponse[]|error
list_pinned_messages
Return Type
- MessageResponse[]|error - 200 response for list_pinned_messages
get channels/[string channel_id]/thread-members
function get channels/[string channel_id]/thread\-members(map<string|string[]> headers, *List_thread_membersQueries queries) returns ThreadMemberResponse[]|error
get channels/[string channel_id]/thread-members/[string user_id]
function get channels/[string channel_id]/thread\-members/[string user_id](map<string|string[]> headers, *Get_thread_memberQueries queries) returns ThreadMemberResponse|error
get_thread_member
Parameters
- queries *Get_thread_memberQueries - Queries to be sent with the request
Return Type
- ThreadMemberResponse|error - 200 response for get_thread_member
get channels/[string channel_id]/threads/archived/'private
function get channels/[string channel_id]/threads/archived/'private(map<string|string[]> headers, *List_private_archived_threadsQueries queries) returns ThreadsResponse|error
Parameters
- queries *List_private_archived_threadsQueries -
get channels/[string channel_id]/threads/archived/'public
function get channels/[string channel_id]/threads/archived/'public(map<string|string[]> headers, *List_public_archived_threadsQueries queries) returns ThreadsResponse|error
Parameters
- queries *List_public_archived_threadsQueries -
get channels/[string channel_id]/users/@me/threads/archived/'private
function get channels/[string channel_id]/users/\@me/threads/archived/'private(map<string|string[]> headers, *List_my_private_archived_threadsQueries queries) returns ThreadsResponse|error
Parameters
- queries *List_my_private_archived_threadsQueries -
get channels/[string channel_id]/webhooks
function get channels/[string channel_id]/webhooks(map<string|string[]> headers) returns anydata[]|error
list_channel_webhooks
Return Type
- anydata[]|error - 200 response for list_channel_webhooks
get gateway
function get gateway(map<string|string[]> headers) returns GatewayResponse|error
get_gateway
Return Type
- GatewayResponse|error - 200 response for get_gateway
get gateway/bot
function get gateway/bot(map<string|string[]> headers) returns GatewayBotResponse|error
get_bot_gateway
Return Type
- GatewayBotResponse|error - 200 response for get_bot_gateway
get guilds/[string guild_id]
function get guilds/[string guild_id](map<string|string[]> headers, *Get_guildQueries queries) returns GuildWithCountsResponse|error
get_guild
Parameters
- queries *Get_guildQueries - Queries to be sent with the request
Return Type
- GuildWithCountsResponse|error - 200 response for get_guild
get guilds/[string guild_id]/audit-logs
function get guilds/[string guild_id]/audit\-logs(map<string|string[]> headers, *List_guild_audit_log_entriesQueries queries) returns GuildAuditLogResponse|error
Parameters
- queries *List_guild_audit_log_entriesQueries -
get guilds/[string guild_id]/auto-moderation/rules
function get guilds/[string guild_id]/auto\-moderation/rules(map<string|string[]> headers) returns anydata[]|error
list_auto_moderation_rules
Return Type
- anydata[]|error - 200 response for list_auto_moderation_rules
get guilds/[string guild_id]/auto-moderation/rules/[string rule_id]
function get guilds/[string guild_id]/auto\-moderation/rules/[string rule_id](map<string|string[]> headers) returns inline_response_200_1|error
get_auto_moderation_rule
Return Type
- inline_response_200_1|error - 200 response for get_auto_moderation_rule
get guilds/[string guild_id]/bans
function get guilds/[string guild_id]/bans(map<string|string[]> headers, *List_guild_bansQueries queries) returns GuildBanResponse[]|error
list_guild_bans
Parameters
- queries *List_guild_bansQueries - Queries to be sent with the request
Return Type
- GuildBanResponse[]|error - 200 response for list_guild_bans
get guilds/[string guild_id]/bans/[string user_id]
function get guilds/[string guild_id]/bans/[string user_id](map<string|string[]> headers) returns GuildBanResponse|error
get_guild_ban
Return Type
- GuildBanResponse|error - 200 response for get_guild_ban
get guilds/[string guild_id]/channels
function get guilds/[string guild_id]/channels(map<string|string[]> headers) returns anydata[]|error
list_guild_channels
Return Type
- anydata[]|error - 200 response for list_guild_channels
get guilds/[string guild_id]/emojis
function get guilds/[string guild_id]/emojis(map<string|string[]> headers) returns EmojiResponse[]|error
list_guild_emojis
Return Type
- EmojiResponse[]|error - 200 response for list_guild_emojis
get guilds/[string guild_id]/emojis/[string emoji_id]
function get guilds/[string guild_id]/emojis/[string emoji_id](map<string|string[]> headers) returns EmojiResponse|error
get_guild_emoji
Return Type
- EmojiResponse|error - 200 response for get_guild_emoji
get guilds/[string guild_id]/integrations
function get guilds/[string guild_id]/integrations(map<string|string[]> headers) returns anydata[]|error
list_guild_integrations
Return Type
- anydata[]|error - 200 response for list_guild_integrations
get guilds/[string guild_id]/invites
list_guild_invites
Return Type
- anydata[]|error - 200 response for list_guild_invites
get guilds/[string guild_id]/members
function get guilds/[string guild_id]/members(map<string|string[]> headers, *List_guild_membersQueries queries) returns GuildMemberResponse[]|error
list_guild_members
Parameters
- queries *List_guild_membersQueries - Queries to be sent with the request
Return Type
- GuildMemberResponse[]|error - 200 response for list_guild_members
get guilds/[string guild_id]/members/[string user_id]
function get guilds/[string guild_id]/members/[string user_id](map<string|string[]> headers) returns GuildMemberResponse|error
get_guild_member
Return Type
- GuildMemberResponse|error - 200 response for get_guild_member
get guilds/[string guild_id]/members/search
function get guilds/[string guild_id]/members/search(map<string|string[]> headers, *Search_guild_membersQueries queries) returns GuildMemberResponse[]|error
search_guild_members
Parameters
- queries *Search_guild_membersQueries - Queries to be sent with the request
Return Type
- GuildMemberResponse[]|error - 200 response for search_guild_members
get guilds/[string guild_id]/new-member-welcome
function get guilds/[string guild_id]/new\-member\-welcome(map<string|string[]> headers) returns GuildHomeSettingsResponse|error
get guilds/[string guild_id]/onboarding
function get guilds/[string guild_id]/onboarding(map<string|string[]> headers) returns UserGuildOnboardingResponse|error
get_guilds_onboarding
Return Type
- UserGuildOnboardingResponse|error - 200 response for get_guilds_onboarding
get guilds/[string guild_id]/preview
function get guilds/[string guild_id]/preview(map<string|string[]> headers) returns GuildPreviewResponse|error
get_guild_preview
Return Type
- GuildPreviewResponse|error - 200 response for get_guild_preview
get guilds/[string guild_id]/prune
function get guilds/[string guild_id]/prune(map<string|string[]> headers, *Preview_prune_guildQueries queries) returns GuildPruneResponse|error
preview_prune_guild
Parameters
- queries *Preview_prune_guildQueries - Queries to be sent with the request
Return Type
- GuildPruneResponse|error - 200 response for preview_prune_guild
get guilds/[string guild_id]/regions
function get guilds/[string guild_id]/regions(map<string|string[]> headers) returns VoiceRegionResponse[]|error
list_guild_voice_regions
Return Type
- VoiceRegionResponse[]|error - 200 response for list_guild_voice_regions
get guilds/[string guild_id]/roles
function get guilds/[string guild_id]/roles(map<string|string[]> headers) returns GuildRoleResponse[]|error
list_guild_roles
Return Type
- GuildRoleResponse[]|error - 200 response for list_guild_roles
get guilds/[string guild_id]/scheduled-events
function get guilds/[string guild_id]/scheduled\-events(map<string|string[]> headers, *List_guild_scheduled_eventsQueries queries) returns anydata[]|error
get guilds/[string guild_id]/scheduled-events/[string guild_scheduled_event_id]
function get guilds/[string guild_id]/scheduled\-events/[string guild_scheduled_event_id](map<string|string[]> headers, *Get_guild_scheduled_eventQueries queries) returns inline_response_200_3|error
get_guild_scheduled_event
Parameters
- queries *Get_guild_scheduled_eventQueries - Queries to be sent with the request
Return Type
- inline_response_200_3|error - 200 response for get_guild_scheduled_event
get guilds/[string guild_id]/scheduled-events/[string guild_scheduled_event_id]/users
function get guilds/[string guild_id]/scheduled\-events/[string guild_scheduled_event_id]/users(map<string|string[]> headers, *List_guild_scheduled_event_usersQueries queries) returns ScheduledEventUserResponse[]|error
list_guild_scheduled_event_users
Parameters
- queries *List_guild_scheduled_event_usersQueries - Queries to be sent with the request
Return Type
- ScheduledEventUserResponse[]|error - 200 response for list_guild_scheduled_event_users
get guilds/[string guild_id]/stickers
function get guilds/[string guild_id]/stickers(map<string|string[]> headers) returns GuildStickerResponse[]|error
list_guild_stickers
Return Type
- GuildStickerResponse[]|error - 200 response for list_guild_stickers
get guilds/[string guild_id]/stickers/[string sticker_id]
function get guilds/[string guild_id]/stickers/[string sticker_id](map<string|string[]> headers) returns GuildStickerResponse|error
get_guild_sticker
Return Type
- GuildStickerResponse|error - 200 response for get_guild_sticker
get guilds/[string guild_id]/templates
function get guilds/[string guild_id]/templates(map<string|string[]> headers) returns GuildTemplateResponse[]|error
list_guild_templates
Return Type
- GuildTemplateResponse[]|error - 200 response for list_guild_templates
get guilds/[string guild_id]/threads/active
function get guilds/[string guild_id]/threads/active(map<string|string[]> headers) returns ThreadsResponse|error
get_active_guild_threads
Return Type
- ThreadsResponse|error - 200 response for get_active_guild_threads
get guilds/[string guild_id]/vanity-url
function get guilds/[string guild_id]/vanity\-url(map<string|string[]> headers) returns VanityURLResponse|error
get guilds/[string guild_id]/webhooks
function get guilds/[string guild_id]/webhooks(map<string|string[]> headers) returns anydata[]|error
get_guild_webhooks
Return Type
- anydata[]|error - 200 response for get_guild_webhooks
get guilds/[string guild_id]/welcome-screen
function get guilds/[string guild_id]/welcome\-screen(map<string|string[]> headers) returns GuildWelcomeScreenResponse|error
get guilds/[string guild_id]/widget
function get guilds/[string guild_id]/widget(map<string|string[]> headers) returns WidgetSettingsResponse|error
get_guild_widget_settings
Return Type
- WidgetSettingsResponse|error - 200 response for get_guild_widget_settings
get guilds/[string guild_id]/widget.json
function get guilds/[string guild_id]/widget\.json(map<string|string[]> headers) returns WidgetResponse|error
get guilds/[string guild_id]/widget.png
function get guilds/[string guild_id]/widget\.png(map<string|string[]> headers, *Get_guild_widget_pngQueries queries) returns byte[]|error
get guilds/templates/[string code]
function get guilds/templates/[string code](map<string|string[]> headers) returns GuildTemplateResponse|error
get_guild_template
Return Type
- GuildTemplateResponse|error - 200 response for get_guild_template
get invites/[string code]
function get invites/[string code](map<string|string[]> headers, *Invite_resolveQueries queries) returns inline_response_200_2|error
invite_resolve
Parameters
- queries *Invite_resolveQueries - Queries to be sent with the request
Return Type
- inline_response_200_2|error - 200 response for invite_resolve
get oauth2/@me
function get oauth2/\@me(map<string|string[]> headers) returns OAuth2GetAuthorizationResponse|error
get oauth2/applications/@me
function get oauth2/applications/\@me(map<string|string[]> headers) returns PrivateApplicationResponse|error
get oauth2/keys
function get oauth2/keys(map<string|string[]> headers) returns OAuth2GetKeys|error
get_public_keys
Return Type
- OAuth2GetKeys|error - 200 response for get_public_keys
get stage-instances/[string channel_id]
function get stage\-instances/[string channel_id](map<string|string[]> headers) returns StageInstanceResponse|error
get sticker-packs
function get sticker\-packs(map<string|string[]> headers) returns StickerPackCollectionResponse|error
get stickers/[string sticker_id]
function get stickers/[string sticker_id](map<string|string[]> headers) returns inline_response_200_5|error
get_sticker
Return Type
- inline_response_200_5|error - 200 response for get_sticker
get users/[string user_id]
function get users/[string user_id](map<string|string[]> headers) returns UserResponse|error
get_user
Return Type
- UserResponse|error - 200 response for get_user
get users/@me
function get users/\@me(map<string|string[]> headers) returns UserPIIResponse|error
get users/@me/applications/[string application_id]/role-connection
function get users/\@me/applications/[string application_id]/role\-connection(map<string|string[]> headers) returns ApplicationUserRoleConnectionResponse|error
get users/@me/connections
function get users/\@me/connections(map<string|string[]> headers) returns ConnectedAccountResponse[]|error
list_my_connections
Return Type
- ConnectedAccountResponse[]|error - 200 response for list_my_connections
get users/@me/guilds
function get users/\@me/guilds(map<string|string[]> headers, *List_my_guildsQueries queries) returns MyGuildResponse[]|error
list_my_guilds
Parameters
- queries *List_my_guildsQueries - Queries to be sent with the request
Return Type
- MyGuildResponse[]|error - 200 response for list_my_guilds
get users/@me/guilds/[string guild_id]/member
function get users/\@me/guilds/[string guild_id]/member(map<string|string[]> headers) returns PrivateGuildMemberResponse|error
get_my_guild_member
Return Type
- PrivateGuildMemberResponse|error - 200 response for get_my_guild_member
get voice/regions
function get voice/regions(map<string|string[]> headers) returns VoiceRegionResponse[]|error
list_voice_regions
Return Type
- VoiceRegionResponse[]|error - 200 response for list_voice_regions
get webhooks/[string webhook_id]
function get webhooks/[string webhook_id](map<string|string[]> headers) returns inline_response_200_4|error
get_webhook
Return Type
- inline_response_200_4|error - 200 response for get_webhook
get webhooks/[string webhook_id]/[string webhook_token]
function get webhooks/[string webhook_id]/[string webhook_token](map<string|string[]> headers) returns inline_response_200_4|error
get_webhook_by_token
Return Type
- inline_response_200_4|error - 200 response for get_webhook_by_token
get webhooks/[string webhook_id]/[string webhook_token]/messages/[string message_id]
function get webhooks/[string webhook_id]/[string webhook_token]/messages/[string message_id](map<string|string[]> headers, *Get_webhook_messageQueries queries) returns MessageResponse|error
get_webhook_message
Parameters
- queries *Get_webhook_messageQueries - Queries to be sent with the request
Return Type
- MessageResponse|error - 200 response for get_webhook_message
get webhooks/[string webhook_id]/[string webhook_token]/messages/@original
function get webhooks/[string webhook_id]/[string webhook_token]/messages/\@original(map<string|string[]> headers, *Get_original_webhook_messageQueries queries) returns MessageResponse|error
Parameters
- queries *Get_original_webhook_messageQueries -
patch applications/[string application_id]
function patch applications/[string application_id](ApplicationFormPartial payload, map<string|string[]> headers) returns PrivateApplicationResponse|error
update_application
Parameters
- payload ApplicationFormPartial -
Return Type
- PrivateApplicationResponse|error - 200 response for update_application
patch applications/[string application_id]/commands/[string command_id]
function patch applications/[string application_id]/commands/[string command_id](ApplicationCommandPatchRequestPartial payload, map<string|string[]> headers) returns ApplicationCommandResponse|error
update_application_command
Parameters
- payload ApplicationCommandPatchRequestPartial -
Return Type
- ApplicationCommandResponse|error - 200 response for update_application_command
patch applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id]
function patch applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id](ApplicationCommandPatchRequestPartial payload, map<string|string[]> headers) returns ApplicationCommandResponse|error
update_guild_application_command
Parameters
- payload ApplicationCommandPatchRequestPartial -
Return Type
- ApplicationCommandResponse|error - 200 response for update_guild_application_command
patch applications/@me
function patch applications/\@me(ApplicationFormPartial payload, map<string|string[]> headers) returns PrivateApplicationResponse|error
patch channels/[string channel_id]
function patch channels/[string channel_id](channels_channel_id_body payload, map<string|string[]> headers) returns inline_response_200_6|error
update_channel
Parameters
- payload channels_channel_id_body -
Return Type
- inline_response_200_6|error - 200 response for update_channel
patch channels/[string channel_id]/messages/[string message_id]
function patch channels/[string channel_id]/messages/[string message_id](Update_messageHeaders headers, messages_message_id_body payload) returns MessageResponse|error
update_message
Parameters
- headers Update_messageHeaders - Headers to be sent with the request
- payload messages_message_id_body -
Return Type
- MessageResponse|error - 200 response for update_message
patch guilds/[string guild_id]
function patch guilds/[string guild_id](GuildPatchRequestPartial payload, map<string|string[]> headers) returns GuildResponse|error
update_guild
Parameters
- payload GuildPatchRequestPartial -
Return Type
- GuildResponse|error - 200 response for update_guild
patch guilds/[string guild_id]/auto-moderation/rules/[string rule_id]
function patch guilds/[string guild_id]/auto\-moderation/rules/[string rule_id](rules_rule_id_body payload, map<string|string[]> headers) returns inline_response_200_1|error
update_auto_moderation_rule
Parameters
- payload rules_rule_id_body -
Return Type
- inline_response_200_1|error - 200 response for update_auto_moderation_rule
patch guilds/[string guild_id]/channels
function patch guilds/[string guild_id]/channels(GuildsChannelsRequest[] payload, map<string|string[]> headers) returns Response|error
bulk_update_guild_channels
Parameters
- payload GuildsChannelsRequest[] -
patch guilds/[string guild_id]/emojis/[string emoji_id]
function patch guilds/[string guild_id]/emojis/[string emoji_id](GuildsEmojisRequest payload, map<string|string[]> headers) returns EmojiResponse|error
update_guild_emoji
Parameters
- payload GuildsEmojisRequest -
Return Type
- EmojiResponse|error - 200 response for update_guild_emoji
patch guilds/[string guild_id]/members/[string user_id]
function patch guilds/[string guild_id]/members/[string user_id](GuildsMembersRequest1 payload, map<string|string[]> headers) returns GuildMemberResponse|error
update_guild_member
Parameters
- payload GuildsMembersRequest1 -
Return Type
- GuildMemberResponse|error - 200 response for update_guild_member
patch guilds/[string guild_id]/members/@me
function patch guilds/[string guild_id]/members/\@me(GuildsMembersMeRequest payload, map<string|string[]> headers) returns PrivateGuildMemberResponse|error
patch guilds/[string guild_id]/roles
function patch guilds/[string guild_id]/roles(GuildsRolesRequest2[] payload, map<string|string[]> headers) returns GuildRoleResponse[]|error
bulk_update_guild_roles
Parameters
- payload GuildsRolesRequest2[] -
Return Type
- GuildRoleResponse[]|error - 200 response for bulk_update_guild_roles
patch guilds/[string guild_id]/roles/[string role_id]
function patch guilds/[string guild_id]/roles/[string role_id](GuildsRolesRequest payload, map<string|string[]> headers) returns GuildRoleResponse|error
update_guild_role
Parameters
- payload GuildsRolesRequest -
Return Type
- GuildRoleResponse|error - 200 response for update_guild_role
patch guilds/[string guild_id]/scheduled-events/[string guild_scheduled_event_id]
function patch guilds/[string guild_id]/scheduled\-events/[string guild_scheduled_event_id](scheduledevents_guild_scheduled_event_id_body payload, map<string|string[]> headers) returns inline_response_200_3|error
update_voice_state
Parameters
Return Type
- inline_response_200_3|error - 204 response for update_voice_state
patch guilds/[string guild_id]/stickers/[string sticker_id]
function patch guilds/[string guild_id]/stickers/[string sticker_id](GuildsStickersRequest payload, map<string|string[]> headers) returns GuildStickerResponse|error
update_guild_sticker
Parameters
- payload GuildsStickersRequest -
Return Type
- GuildStickerResponse|error - 200 response for update_guild_sticker
patch guilds/[string guild_id]/templates/[string code]
function patch guilds/[string guild_id]/templates/[string code](GuildsTemplatesRequest1 payload, map<string|string[]> headers) returns GuildTemplateResponse|error
update_guild_template
Parameters
- payload GuildsTemplatesRequest1 -
Return Type
- GuildTemplateResponse|error - 200 response for update_guild_template
patch guilds/[string guild_id]/voice-states/[string user_id]
function patch guilds/[string guild_id]/voice\-states/[string user_id](GuildsVoiceStatesRequest payload, map<string|string[]> headers) returns Response|error
update_voice_state
Parameters
- payload GuildsVoiceStatesRequest -
patch guilds/[string guild_id]/voice-states/@me
function patch guilds/[string guild_id]/voice\-states/\@me(GuildsVoiceStatesMeRequest payload, map<string|string[]> headers) returns Response|error
patch guilds/[string guild_id]/welcome-screen
function patch guilds/[string guild_id]/welcome\-screen(WelcomeScreenPatchRequestPartial payload, map<string|string[]> headers) returns GuildWelcomeScreenResponse|error
patch guilds/[string guild_id]/widget
function patch guilds/[string guild_id]/widget(GuildsWidgetRequest payload, map<string|string[]> headers) returns WidgetSettingsResponse|error
update_guild_widget_settings
Parameters
- payload GuildsWidgetRequest -
Return Type
- WidgetSettingsResponse|error - 200 response for update_guild_widget_settings
patch stage-instances/[string channel_id]
function patch stage\-instances/[string channel_id](StageInstancesRequest1 payload, map<string|string[]> headers) returns StageInstanceResponse|error
patch users/@me
function patch users/\@me(BotAccountPatchRequest payload, map<string|string[]> headers) returns UserPIIResponse|error
patch webhooks/[string webhook_id]
function patch webhooks/[string webhook_id](WebhooksRequest2 payload, map<string|string[]> headers) returns inline_response_200_4|error
update_webhook
Parameters
- payload WebhooksRequest2 -
Return Type
- inline_response_200_4|error - 200 response for update_webhook
patch webhooks/[string webhook_id]/[string webhook_token]
function patch webhooks/[string webhook_id]/[string webhook_token](WebhooksRequest1 payload, map<string|string[]> headers) returns inline_response_200_4|error
update_webhook_by_token
Parameters
- payload WebhooksRequest1 -
Return Type
- inline_response_200_4|error - 200 response for update_webhook_by_token
patch webhooks/[string webhook_id]/[string webhook_token]/messages/[string message_id]
function patch webhooks/[string webhook_id]/[string webhook_token]/messages/[string message_id](Update_webhook_messageHeaders headers, messages_message_id_body_1 payload, *Update_webhook_messageQueries queries) returns MessageResponse|error
update_webhook_message
Parameters
- headers Update_webhook_messageHeaders - Headers to be sent with the request
- payload messages_message_id_body_1 -
- queries *Update_webhook_messageQueries - Queries to be sent with the request
Return Type
- MessageResponse|error - 200 response for update_webhook_message
patch webhooks/[string webhook_id]/[string webhook_token]/messages/@original
function patch webhooks/[string webhook_id]/[string webhook_token]/messages/\@original(messages_original_body payload, Update_original_webhook_messageHeaders headers, *Update_original_webhook_messageQueries queries) returns MessageResponse|error
Parameters
- payload messages_original_body -
- headers Update_original_webhook_messageHeaders -
- queries *Update_original_webhook_messageQueries -
post applications/[string application_id]/commands
function post applications/[string application_id]/commands(ApplicationCommandCreateRequest payload, map<string|string[]> headers) returns ApplicationCommandResponse|error
create_application_command
Parameters
- payload ApplicationCommandCreateRequest -
Return Type
- ApplicationCommandResponse|error - 200 response for create_application_command
post applications/[string application_id]/entitlements
function post applications/[string application_id]/entitlements(CreateEntitlementRequestData payload, map<string|string[]> headers) returns EntitlementResponse|error
create_entitlement
Parameters
- payload CreateEntitlementRequestData -
Return Type
- EntitlementResponse|error - 200 response for create_entitlement
post applications/[string application_id]/entitlements/[string entitlement_id]/consume
function post applications/[string application_id]/entitlements/[string entitlement_id]/consume(map<string|string[]> headers) returns Response|error
consume_entitlement
post applications/[string application_id]/guilds/[string guild_id]/commands
function post applications/[string application_id]/guilds/[string guild_id]/commands(ApplicationCommandCreateRequest payload, map<string|string[]> headers) returns ApplicationCommandResponse|error
create_guild_application_command
Parameters
- payload ApplicationCommandCreateRequest -
Return Type
- ApplicationCommandResponse|error - 200 response for create_guild_application_command
post channels/[string channel_id]/followers
function post channels/[string channel_id]/followers(ChannelsFollowersRequest payload, map<string|string[]> headers) returns ChannelFollowerResponse|error
follow_channel
Parameters
- payload ChannelsFollowersRequest -
Return Type
- ChannelFollowerResponse|error - 200 response for follow_channel
post channels/[string channel_id]/invites
function post channels/[string channel_id]/invites(channel_id_invites_body payload, map<string|string[]> headers) returns inline_response_200_2|error
create_channel_invite
Parameters
- payload channel_id_invites_body -
Return Type
- inline_response_200_2|error - 200 response for create_channel_invite
post channels/[string channel_id]/messages
function post channels/[string channel_id]/messages(Create_messageHeaders headers, channel_id_messages_body payload) returns MessageResponse|error
create_message
Parameters
- headers Create_messageHeaders - Headers to be sent with the request
- payload channel_id_messages_body -
Return Type
- MessageResponse|error - 200 response for create_message
post channels/[string channel_id]/messages/[string message_id]/crosspost
function post channels/[string channel_id]/messages/[string message_id]/crosspost(map<string|string[]> headers) returns MessageResponse|error
crosspost_message
Return Type
- MessageResponse|error - 200 response for crosspost_message
post channels/[string channel_id]/messages/[string message_id]/threads
function post channels/[string channel_id]/messages/[string message_id]/threads(CreateTextThreadWithMessageRequest payload, map<string|string[]> headers) returns ThreadResponse|error
create_thread_from_message
Parameters
- payload CreateTextThreadWithMessageRequest -
Return Type
- ThreadResponse|error - 201 response for create_thread_from_message
post channels/[string channel_id]/messages/bulk-delete
function post channels/[string channel_id]/messages/bulk\-delete(ChannelsMessagesBulkDeleteRequest payload, map<string|string[]> headers) returns Response|error
post channels/[string channel_id]/threads
function post channels/[string channel_id]/threads(channel_id_threads_body payload, map<string|string[]> headers) returns CreatedThreadResponse|error
create_thread
Parameters
- payload channel_id_threads_body -
Return Type
- CreatedThreadResponse|error - 201 response for create_thread
post channels/[string channel_id]/typing
function post channels/[string channel_id]/typing(map<string|string[]> headers) returns record {}|error
trigger_typing_indicator
Return Type
- record {}|error - 200 response for trigger_typing_indicator
post channels/[string channel_id]/webhooks
function post channels/[string channel_id]/webhooks(ChannelsWebhooksRequest payload, map<string|string[]> headers) returns GuildIncomingWebhookResponse|error
create_webhook
Parameters
- payload ChannelsWebhooksRequest -
Return Type
- GuildIncomingWebhookResponse|error - 200 response for create_webhook
post guilds
function post guilds(GuildCreateRequest payload, map<string|string[]> headers) returns GuildResponse|error
create_guild
Parameters
- payload GuildCreateRequest -
Return Type
- GuildResponse|error - 201 response for create_guild
post guilds/[string guild_id]/auto-moderation/rules
function post guilds/[string guild_id]/auto\-moderation/rules(automoderation_rules_body payload, map<string|string[]> headers) returns inline_response_200_1|error
create_auto_moderation_rule
Parameters
- payload automoderation_rules_body -
Return Type
- inline_response_200_1|error - 200 response for create_auto_moderation_rule
post guilds/[string guild_id]/bulk-ban
function post guilds/[string guild_id]/bulk\-ban(GuildsBulkBanRequest payload, map<string|string[]> headers) returns BulkBanUsersResponse|error
post guilds/[string guild_id]/channels
function post guilds/[string guild_id]/channels(CreateGuildChannelRequest payload, map<string|string[]> headers) returns GuildChannelResponse|error
create_guild_channel
Parameters
- payload CreateGuildChannelRequest -
Return Type
- GuildChannelResponse|error - 201 response for create_guild_channel
post guilds/[string guild_id]/emojis
function post guilds/[string guild_id]/emojis(GuildsEmojisRequest1 payload, map<string|string[]> headers) returns EmojiResponse|error
create_guild_emoji
Parameters
- payload GuildsEmojisRequest1 -
Return Type
- EmojiResponse|error - 201 response for create_guild_emoji
post guilds/[string guild_id]/mfa
function post guilds/[string guild_id]/mfa(GuildMFALevelResponse payload, map<string|string[]> headers) returns GuildMFALevelResponse|error
set_guild_mfa_level
Parameters
- payload GuildMFALevelResponse -
Return Type
- GuildMFALevelResponse|error - 200 response for set_guild_mfa_level
post guilds/[string guild_id]/prune
function post guilds/[string guild_id]/prune(GuildsPruneRequest payload, map<string|string[]> headers) returns GuildPruneResponse|error
prune_guild
Parameters
- payload GuildsPruneRequest -
Return Type
- GuildPruneResponse|error - 200 response for prune_guild
post guilds/[string guild_id]/roles
function post guilds/[string guild_id]/roles(GuildsRolesRequest payload, map<string|string[]> headers) returns GuildRoleResponse|error
create_guild_role
Parameters
- payload GuildsRolesRequest -
Return Type
- GuildRoleResponse|error - 200 response for create_guild_role
post guilds/[string guild_id]/scheduled-events
function post guilds/[string guild_id]/scheduled\-events(guild_id_scheduledevents_body payload, map<string|string[]> headers) returns inline_response_200_3|error
post guilds/[string guild_id]/stickers
function post guilds/[string guild_id]/stickers(guild_id_stickers_body payload, map<string|string[]> headers) returns GuildStickerResponse|error
create_guild_sticker
Parameters
- payload guild_id_stickers_body -
Return Type
- GuildStickerResponse|error - 201 response for create_guild_sticker
post guilds/[string guild_id]/templates
function post guilds/[string guild_id]/templates(GuildsTemplatesRequest2 payload, map<string|string[]> headers) returns GuildTemplateResponse|error
create_guild_template
Parameters
- payload GuildsTemplatesRequest2 -
Return Type
- GuildTemplateResponse|error - 200 response for create_guild_template
post guilds/templates/[string code]
function post guilds/templates/[string code](GuildsTemplatesRequest payload, map<string|string[]> headers) returns GuildResponse|error
create_guild_from_template
Parameters
- payload GuildsTemplatesRequest -
Return Type
- GuildResponse|error - 201 response for create_guild_from_template
post interactions/[string interaction_id]/[string interaction_token]/callback
function post interactions/[string interaction_id]/[string interaction_token]/callback(interaction_token_callback_body payload, map<string|string[]> headers) returns Response|error
create_interaction_response
Parameters
- payload interaction_token_callback_body -
post stage-instances
function post stage\-instances(StageInstancesRequest payload, map<string|string[]> headers) returns StageInstanceResponse|error
post users/@me/channels
function post users/\@me/channels(CreatePrivateChannelRequest payload, map<string|string[]> headers) returns inline_response_200|error
create_dm
Parameters
- payload CreatePrivateChannelRequest -
Return Type
- inline_response_200|error - 200 response for create_dm
post webhooks/[string webhook_id]/[string webhook_token]
function post webhooks/[string webhook_id]/[string webhook_token](webhook_id_webhook_token_body payload, map<string|string[]> headers, *Execute_webhookQueries queries) returns MessageResponse|error
execute_webhook
Parameters
- payload webhook_id_webhook_token_body -
- queries *Execute_webhookQueries - Queries to be sent with the request
Return Type
- MessageResponse|error - 200 response for execute_webhook
post webhooks/[string webhook_id]/[string webhook_token]/github
function post webhooks/[string webhook_id]/[string webhook_token]/github(GithubWebhook payload, map<string|string[]> headers, *Execute_github_compatible_webhookQueries queries) returns Response|error
execute_github_compatible_webhook
Parameters
- payload GithubWebhook -
- queries *Execute_github_compatible_webhookQueries - Queries to be sent with the request
post webhooks/[string webhook_id]/[string webhook_token]/slack
function post webhooks/[string webhook_id]/[string webhook_token]/slack(Execute_slack_compatible_webhookHeaders headers, webhook_token_slack_body payload, *Execute_slack_compatible_webhookQueries queries) returns string?|error
execute_slack_compatible_webhook
Parameters
- headers Execute_slack_compatible_webhookHeaders - Headers to be sent with the request
- payload webhook_token_slack_body -
- queries *Execute_slack_compatible_webhookQueries - Queries to be sent with the request
put applications/[string application_id]/commands
function put applications/[string application_id]/commands(ApplicationCommandUpdateRequest[] payload, map<string|string[]> headers) returns ApplicationCommandResponse[]|error
bulk_set_application_commands
Parameters
- payload ApplicationCommandUpdateRequest[] -
Return Type
- ApplicationCommandResponse[]|error - 200 response for bulk_set_application_commands
put applications/[string application_id]/guilds/[string guild_id]/commands
function put applications/[string application_id]/guilds/[string guild_id]/commands(ApplicationCommandUpdateRequest[] payload, map<string|string[]> headers) returns ApplicationCommandResponse[]|error
bulk_set_guild_application_commands
Parameters
- payload ApplicationCommandUpdateRequest[] -
Return Type
- ApplicationCommandResponse[]|error - 200 response for bulk_set_guild_application_commands
put applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id]/permissions
function put applications/[string application_id]/guilds/[string guild_id]/commands/[string command_id]/permissions(ApplicationsGuildsCommandsCommandIdPermissionsRequest payload, map<string|string[]> headers) returns CommandPermissionsResponse|error
set_guild_application_command_permissions
Parameters
Return Type
- CommandPermissionsResponse|error - 200 response for set_guild_application_command_permissions
put applications/[string application_id]/role-connections/metadata
function put applications/[string application_id]/role\-connections/metadata(ApplicationRoleConnectionsMetadataItemRequest[] payload, map<string|string[]> headers) returns ApplicationRoleConnectionsMetadataItemResponse[]|error
update_application_role_connections_metadata
Parameters
- payload ApplicationRoleConnectionsMetadataItemRequest[] -
Return Type
- ApplicationRoleConnectionsMetadataItemResponse[]|error - 200 response for update_application_role_connections_metadata
put channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name]/@me
function put channels/[string channel_id]/messages/[string message_id]/reactions/[string emoji_name]/\@me(map<string|string[]> headers) returns Response|error
put channels/[string channel_id]/permissions/[string overwrite_id]
function put channels/[string channel_id]/permissions/[string overwrite_id](ChannelsPermissionsRequest payload, map<string|string[]> headers) returns Response|error
set_channel_permission_overwrite
Parameters
- payload ChannelsPermissionsRequest -
put channels/[string channel_id]/pins/[string message_id]
function put channels/[string channel_id]/pins/[string message_id](map<string|string[]> headers) returns Response|error
pin_message
put channels/[string channel_id]/recipients/[string user_id]
function put channels/[string channel_id]/recipients/[string user_id](ChannelsRecipientsRequest payload, map<string|string[]> headers) returns inline_response_200|error
add_group_dm_user
Parameters
- payload ChannelsRecipientsRequest -
Return Type
- inline_response_200|error - 201 response for add_group_dm_user
put channels/[string channel_id]/thread-members/[string user_id]
function put channels/[string channel_id]/thread\-members/[string user_id](map<string|string[]> headers) returns Response|error
add_thread_member
put channels/[string channel_id]/thread-members/@me
function put channels/[string channel_id]/thread\-members/\@me(map<string|string[]> headers) returns Response|error
put guilds/[string guild_id]/bans/[string user_id]
function put guilds/[string guild_id]/bans/[string user_id](GuildsBansRequest payload, map<string|string[]> headers) returns Response|error
ban_user_from_guild
Parameters
- payload GuildsBansRequest -
put guilds/[string guild_id]/members/[string user_id]
function put guilds/[string guild_id]/members/[string user_id](GuildsMembersRequest payload, map<string|string[]> headers) returns GuildMemberResponse|error
add_guild_member
Parameters
- payload GuildsMembersRequest -
Return Type
- GuildMemberResponse|error - 201 response for add_guild_member
put guilds/[string guild_id]/members/[string user_id]/roles/[string role_id]
function put guilds/[string guild_id]/members/[string user_id]/roles/[string role_id](map<string|string[]> headers) returns Response|error
add_guild_member_role
put guilds/[string guild_id]/onboarding
function put guilds/[string guild_id]/onboarding(UpdateGuildOnboardingRequest payload, map<string|string[]> headers) returns GuildOnboardingResponse|error
put_guilds_onboarding
Parameters
- payload UpdateGuildOnboardingRequest -
Return Type
- GuildOnboardingResponse|error - 200 response for put_guilds_onboarding
put guilds/[string guild_id]/templates/[string code]
function put guilds/[string guild_id]/templates/[string code](map<string|string[]> headers) returns GuildTemplateResponse|error
sync_guild_template
Return Type
- GuildTemplateResponse|error - 200 response for sync_guild_template
put users/@me/applications/[string application_id]/role-connection
function put users/\@me/applications/[string application_id]/role\-connection(UsersMeApplicationsRoleConnectionRequest payload, map<string|string[]> headers) returns ApplicationUserRoleConnectionResponse|error
Parameters
- payload UsersMeApplicationsRoleConnectionRequest -
Records
discord: AccountResponse
Fields
- id string -
- name string? -
discord: ActionRow
Fields
- 'type 1 -
- components (Button|ChannelSelect|InputText|MentionableSelect|RoleSelect|StringSelect|UserSelect)[] -
discord: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- Authorization string - Discord bot token
discord: ApplicationCommandAttachmentOption
Fields
- 'type 11 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandAttachmentOptionResponse
Fields
- 'type 11 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandAutocompleteCallbackRequest
Fields
- 'type 8 -
discord: ApplicationCommandBooleanOption
Fields
- 'type 5 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandBooleanOptionResponse
Fields
- 'type 5 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandChannelOption
Fields
- 'type 7 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
- channel_types ChannelTypes[]? -
discord: ApplicationCommandChannelOptionResponse
Fields
- 'type 7 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
- channel_types ChannelTypes[]? -
discord: ApplicationCommandCreateRequest
Fields
- name string -
- name_localizations record { string... }? -
- description string? -
- description_localizations record { string... }? -
- options (anydata)[]? -
- default_member_permissions Signed32? -
- dm_permission boolean? -
- 'type anydata? -
discord: ApplicationCommandIntegerOption
Fields
- 'type 4 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
- autocomplete boolean? -
- choices ApplicationCommandOptionIntegerChoice[]? -
- min_value anydata? -
- max_value anydata? -
discord: ApplicationCommandIntegerOptionResponse
Fields
- 'type 4 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
- autocomplete boolean? -
- choices ApplicationCommandOptionIntegerChoiceResponse[]? -
- min_value anydata? -
- max_value anydata? -
discord: ApplicationCommandMentionableOption
Fields
- 'type 9 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandMentionableOptionResponse
Fields
- 'type 9 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandNumberOption
Fields
- 'type 10 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
- autocomplete boolean? -
- choices ApplicationCommandOptionNumberChoice[]? -
- min_value decimal? -
- max_value decimal? -
discord: ApplicationCommandNumberOptionResponse
Fields
- 'type 10 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
- autocomplete boolean? -
- choices ApplicationCommandOptionNumberChoiceResponse[]? -
- min_value decimal? -
- max_value decimal? -
discord: ApplicationCommandOptionIntegerChoice
Fields
- name string -
- name_localizations record { string... }? -
- value int -
discord: ApplicationCommandOptionIntegerChoiceResponse
Fields
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- value int -
discord: ApplicationCommandOptionNumberChoice
Fields
- name string -
- name_localizations record { string... }? -
- value decimal -
discord: ApplicationCommandOptionNumberChoiceResponse
Fields
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- value decimal -
discord: ApplicationCommandOptionStringChoice
Fields
- name string -
- name_localizations record { string... }? -
- value string -
discord: ApplicationCommandOptionStringChoiceResponse
Fields
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- value string -
discord: ApplicationCommandPatchRequestPartial
Fields
- name string? -
- name_localizations record { string... }? -
- description string? -
- description_localizations record { string... }? -
- options (anydata)[]? -
- default_member_permissions Signed32? -
- dm_permission boolean? -
discord: ApplicationCommandPermission
Fields
- id string -
- 'type ApplicationCommandPermissionType -
- permission boolean -
discord: ApplicationCommandResponse
Fields
- id string -
- application_id string -
- version string -
- default_member_permissions string? -
- 'type ApplicationCommandType -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- guild_id anydata? -
- dm_permission boolean? -
- options (anydata)[]? -
- nsfw boolean? -
discord: ApplicationCommandRoleOption
Fields
- 'type 8 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandRoleOptionResponse
Fields
- 'type 8 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandStringOption
Fields
- 'type 3 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
- autocomplete boolean? -
- min_length Signed32? -
- max_length Signed32? -
- choices ApplicationCommandOptionStringChoice[]? -
discord: ApplicationCommandStringOptionResponse
Fields
- 'type 3 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
- autocomplete boolean? -
- choices ApplicationCommandOptionStringChoiceResponse[]? -
- min_length Signed32? -
- max_length Signed32? -
discord: ApplicationCommandSubcommandGroupOption
Fields
- 'type 2 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
- options ApplicationCommandSubcommandOption[]? -
discord: ApplicationCommandSubcommandGroupOptionResponse
Fields
- 'type 2 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
- options ApplicationCommandSubcommandOptionResponse[]? -
discord: ApplicationCommandSubcommandOption
Fields
- 'type 1 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
- options (anydata)[]? -
discord: ApplicationCommandSubcommandOptionResponse
Fields
- 'type 1 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
- options (anydata)[]? -
discord: ApplicationCommandUpdateRequest
Fields
- name string -
- name_localizations record { string... }? -
- description string? -
- description_localizations record { string... }? -
- options (anydata)[]? -
- default_member_permissions Signed32? -
- dm_permission boolean? -
- 'type anydata? -
- id anydata? -
discord: ApplicationCommandUserOption
Fields
- 'type 6 -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationCommandUserOptionResponse
Fields
- 'type 6 -
- name string -
- name_localized string? -
- name_localizations record { string... }? -
- description string -
- description_localized string? -
- description_localizations record { string... }? -
- required boolean? -
discord: ApplicationFormPartial
Fields
- description anydata? -
- icon record { fileContent byte[], fileName string }? -
- cover_image record { fileContent byte[], fileName string }? -
- team_id anydata? -
- flags Signed32? -
- interactions_endpoint_url string? -
- max_participants Signed32? -
- 'type anydata? -
- tags ApplicationFormPartialTagsItemsString[]? -
- custom_install_url string? -
- install_params anydata? -
- role_connections_verification_url string? -
discord: ApplicationIncomingWebhookResponse
Fields
- application_id anydata? -
- avatar string? -
- channel_id anydata? -
- guild_id anydata? -
- id string -
- name string -
- 'type 3 -
- user anydata? -
discord: ApplicationOAuth2InstallParams
Fields
- scopes OAuth2Scopes[]? -
- permissions Signed32? -
discord: ApplicationOAuth2InstallParamsResponse
Fields
- scopes OAuth2Scopes[] -
- permissions string -
discord: ApplicationResponse
Fields
- id string -
- name string -
- icon string? -
- description string -
- 'type anydata? -
- cover_image string? -
- primary_sku_id anydata? -
- bot anydata? -
- slug string? -
- guild_id anydata? -
- rpc_origins string[]? -
- bot_public boolean? -
- bot_require_code_grant boolean? -
- terms_of_service_url string? -
- privacy_policy_url string? -
- custom_install_url string? -
- install_params anydata? -
- verify_key string -
- flags Signed32 -
- max_participants Signed32? -
- tags string[]? -
discord: ApplicationRoleConnectionsMetadataItemRequest
Fields
- 'type MetadataItemTypes -
- 'key string -
- name string -
- name_localizations record { string?... }? -
- description string -
- description_localizations record { string?... }? -
discord: ApplicationRoleConnectionsMetadataItemResponse
Fields
- 'type MetadataItemTypes -
- 'key string -
- name string -
- name_localizations record { string... }? -
- description string -
- description_localizations record { string... }? -
discord: ApplicationsGuildsCommandsCommandIdPermissionsRequest
Fields
- permissions ApplicationCommandPermission[]? -
discord: ApplicationUserRoleConnectionResponse
Fields
- platform_name string? -
- platform_username string? -
- metadata record { string... }? -
discord: AuditLogEntryResponse
Fields
- id string -
- action_type AuditLogActionTypes -
- user_id anydata? -
- target_id anydata? -
- changes AuditLogObjectChangeResponse[]? -
- options record { string... }? -
- reason string? -
discord: AuditLogObjectChangeResponse
Fields
- 'key string? -
- new_value anydata? -
- old_value anydata? -
discord: BaseCreateMessageCreateRequest
Fields
- content string? -
- embeds RichEmbed[]? -
- allowed_mentions anydata? -
- sticker_ids BaseCreateMessageCreateRequestStickeridsItemsString[]? -
- components ActionRow[]? -
- flags Signed32? -
- attachments MessageAttachmentRequest[]? -
discord: BasicApplicationResponse
Fields
- id string -
- name string -
- icon string? -
- description string -
- 'type anydata? -
- cover_image string? -
- primary_sku_id anydata? -
- bot anydata? -
discord: BasicMessageResponse
Fields
- 'type MessageType -
- channel_id string -
- content string -
- attachments MessageAttachmentResponse[] -
- embeds MessageEmbedResponse[] -
- timestamp string -
- edited_timestamp string? -
- flags Signed32 -
- components (MessageComponentActionRowResponse|MessageComponentButtonResponse|MessageComponentChannelSelectResponse|MessageComponentInputTextResponse|MessageComponentMentionableSelectResponse|MessageComponentRoleSelectResponse|MessageComponentStringSelectResponse|MessageComponentUserSelectResponse)[] -
- resolved anydata? -
- id string -
- author UserResponse -
- mentions UserResponse[] -
- mention_roles BasicMessageResponseMentionrolesItemsString[] -
- pinned boolean -
- mention_everyone boolean -
- tts boolean -
- call anydata? -
- activity anydata? -
- application anydata? -
- application_id anydata? -
- interaction anydata? -
- nonce anydata? -
- webhook_id anydata? -
- message_reference anydata? -
- thread anydata? -
- mention_channels (anydata)[]? -
- stickers (anydata)[]? -
- sticker_items MessageStickerItemResponse[]? -
- role_subscription_data anydata? -
- purchase_notification anydata? -
- position Signed32? -
discord: BlockMessageAction
Fields
- 'type 1 -
- metadata anydata? -
discord: BlockMessageActionMetadata
Fields
- custom_message string? -
discord: BlockMessageActionMetadataResponse
Fields
- custom_message string? -
discord: BlockMessageActionResponse
Fields
- 'type 1 -
- metadata BlockMessageActionMetadataResponse -
discord: BotAccountPatchRequest
Fields
- username string -
- avatar record { fileContent byte[], fileName string }? -
- banner record { fileContent byte[], fileName string }? -
discord: BulkBanUsersResponse
Fields
- banned_users BulkBanUsersResponseBannedusersItemsString[] -
- failed_users BulkBanUsersResponseFailedusersItemsString[] -
discord: Button
Fields
- 'type 2 -
- custom_id string? -
- style ButtonStyleTypes -
- label string? -
- disabled boolean? -
- emoji anydata? -
- url string? -
- sku_id anydata? -
discord: channel_id_messages_body
Fields
- content string? -
- embeds RichEmbed[]? -
- allowed_mentions anydata? -
- sticker_ids channel_id_messages_bodyStickeridsItemsString[]? -
- components ActionRow[]? -
- flags Signed32? -
- attachments MessageAttachmentRequest[]? -
- message_reference anydata? -
- nonce anydata? -
- tts boolean? -
discord: ChannelFollowerResponse
Fields
- channel_id string -
- webhook_id string -
discord: ChannelFollowerWebhookResponse
Fields
- application_id anydata? -
- avatar string? -
- channel_id anydata? -
- guild_id anydata? -
- id string -
- name string -
- 'type 2 -
- user anydata? -
- source_guild anydata? -
- source_channel anydata? -
discord: ChannelPermissionOverwriteRequest
Fields
- id string -
- 'type anydata? -
- allow Signed32? -
- deny Signed32? -
discord: ChannelPermissionOverwriteResponse
Fields
- id string -
- 'type ChannelPermissionOverwrites -
- allow string -
- deny string -
discord: ChannelSelect
Fields
- 'type 8 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
- default_values ChannelSelectDefaultValue[]? -
- channel_types ChannelTypes[]? -
discord: ChannelSelectDefaultValue
Fields
- 'type "channel" -
- id string -
discord: ChannelsFollowersRequest
Fields
- webhook_channel_id string -
discord: ChannelsMessagesBulkDeleteRequest
Fields
- messages ChannelsMessagesBulkDeleteRequestMessagesItemsString[] -
discord: ChannelsPermissionsRequest
Fields
- 'type anydata? -
- allow Signed32? -
- deny Signed32? -
discord: ChannelsRecipientsRequest
Fields
- access_token string? -
- nick string? -
discord: ChannelsWebhooksRequest
Fields
- name string -
- avatar record { fileContent byte[], fileName string }? -
discord: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
discord: CommandPermissionResponse
Fields
- id string -
- 'type ApplicationCommandPermissionType -
- permission boolean -
discord: CommandPermissionsResponse
Fields
- id string -
- application_id string -
- guild_id string -
- permissions CommandPermissionResponse[] -
discord: ConnectedAccountGuildResponse
Fields
- id string -
- name string -
- icon string? -
discord: ConnectedAccountIntegrationResponse
Fields
- id string -
- 'type IntegrationTypes -
- account AccountResponse -
- guild ConnectedAccountGuildResponse -
discord: ConnectedAccountResponse
Fields
- id string -
- name string? -
- 'type ConnectedAccountProviders -
- friend_sync boolean -
- integrations ConnectedAccountIntegrationResponse[]? -
- show_activity boolean -
- two_way_link boolean -
- verified boolean -
- visibility ConnectedAccountVisibility -
- revoked boolean? -
discord: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth OAuth2ClientCredentialsGrantConfig|BearerTokenConfig|OAuth2RefreshTokenGrantConfig|ApiKeysConfig - Provides Auth configurations needed when communicating with a remote HTTP endpoint.
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
discord: Create_messageHeaders
Represents the Headers record for the operation: create_message
Fields
- Content\-Type "application/x-www-form-urlencoded" -
discord: CreatedThreadResponse
Fields
- id string -
- 'type ChannelTypes -
- last_message_id anydata? -
- flags Signed32 -
- last_pin_timestamp string? -
- guild_id string -
- name string -
- parent_id anydata? -
- rate_limit_per_user Signed32? -
- bitrate Signed32? -
- user_limit Signed32? -
- rtc_region string? -
- video_quality_mode anydata? -
- permissions string? -
- owner_id string -
- thread_metadata anydata? -
- message_count Signed32 -
- member_count Signed32 -
- total_message_sent Signed32 -
- applied_tags CreatedThreadResponseAppliedtagsItemsString[]? -
- member anydata? -
discord: CreateEntitlementRequestData
Fields
- sku_id string -
- owner_id string -
- owner_type Signed32 -
discord: CreateForumThreadRequest
Fields
- name string -
- auto_archive_duration anydata? -
- rate_limit_per_user Signed32? -
- applied_tags CreateForumThreadRequestAppliedtagsItemsString[]? -
- message BaseCreateMessageCreateRequest -
discord: CreateGroupDMInviteRequest
Fields
- max_age Signed32? -
discord: CreateGuildChannelRequest
Fields
- 'type anydata? -
- name string -
- position Signed32? -
- topic string? -
- bitrate Signed32? -
- user_limit Signed32? -
- nsfw boolean? -
- rate_limit_per_user Signed32? -
- parent_id anydata? -
- permission_overwrites ChannelPermissionOverwriteRequest[]? -
- rtc_region string? -
- video_quality_mode anydata? -
- default_auto_archive_duration anydata? -
- default_reaction_emoji anydata? -
- default_thread_rate_limit_per_user Signed32? -
- default_sort_order anydata? -
- default_forum_layout anydata? -
- available_tags (anydata)[]? -
discord: CreateGuildInviteRequest
Fields
- max_age Signed32? -
- temporary boolean? -
- max_uses Signed32? -
- unique boolean? -
- target_user_id anydata? -
- target_application_id anydata? -
- target_type anydata? -
discord: CreateGuildRequestChannelItem
Fields
- 'type anydata? -
- name string -
- position Signed32? -
- topic string? -
- bitrate Signed32? -
- user_limit Signed32? -
- nsfw boolean? -
- rate_limit_per_user Signed32? -
- parent_id anydata? -
- permission_overwrites ChannelPermissionOverwriteRequest[]? -
- rtc_region string? -
- video_quality_mode anydata? -
- default_auto_archive_duration anydata? -
- default_reaction_emoji anydata? -
- default_thread_rate_limit_per_user Signed32? -
- default_sort_order anydata? -
- default_forum_layout anydata? -
- id anydata? -
- available_tags CreateOrUpdateThreadTagRequest[]? -
discord: CreateGuildRequestRoleItem
Fields
- id Signed32 -
- name string? -
- permissions Signed32? -
- color Signed32? -
- hoist boolean? -
- mentionable boolean? -
- unicode_emoji string? -
discord: CreateMessageInteractionCallbackRequest
Fields
- 'type InteractionCallbackTypes -
- data anydata? -
discord: CreateOrUpdateThreadTagRequest
Fields
- name string -
- emoji_id anydata? -
- emoji_name string? -
- moderated boolean? -
discord: CreatePrivateChannelRequest
Fields
- recipient_id anydata? -
- access_tokens CreatePrivateChannelRequestAccesstokensItemsString[]? -
- nicks record { string?... }? -
discord: CreateTextThreadWithMessageRequest
Fields
- name string -
- auto_archive_duration anydata? -
- rate_limit_per_user Signed32? -
discord: CreateTextThreadWithoutMessageRequest
Fields
- name string -
- auto_archive_duration anydata? -
- rate_limit_per_user Signed32? -
- 'type anydata? -
- invitable boolean? -
discord: DefaultKeywordListTriggerMetadata
Fields
- allow_list DefaultKeywordListTriggerMetadataAllowlistItemsString[]? -
- presets AutomodKeywordPresetType[]? -
discord: DefaultKeywordListTriggerMetadataResponse
Fields
- allow_list string[] -
- presets AutomodKeywordPresetType[] -
discord: DefaultKeywordListUpsertRequest
Fields
- name string -
- event_type AutomodEventType -
- actions (anydata)[]? -
- enabled boolean? -
- exempt_roles DefaultKeywordListUpsertRequestExemptrolesItemsString[]? -
- exempt_channels DefaultKeywordListUpsertRequestExemptchannelsItemsString[]? -
- trigger_type 4 -
- trigger_metadata DefaultKeywordListTriggerMetadata -
discord: DefaultKeywordListUpsertRequestPartial
Fields
- name string? -
- event_type AutomodEventType? -
- actions (anydata)[]? -
- enabled boolean? -
- exempt_roles DefaultKeywordListUpsertRequestPartialExemptrolesItemsString[]? -
- exempt_channels DefaultKeywordListUpsertRequestPartialExemptchannelsItemsString[]? -
- trigger_type AutomodTriggerType? -
- trigger_metadata DefaultKeywordListTriggerMetadata? -
discord: DefaultKeywordRuleResponse
Fields
- id string -
- guild_id string -
- creator_id string -
- name string -
- event_type AutomodEventType -
- trigger_type 4 -
- enabled boolean? -
- exempt_roles DefaultKeywordRuleResponseExemptrolesItemsString[]? -
- exempt_channels DefaultKeywordRuleResponseExemptchannelsItemsString[]? -
- trigger_metadata DefaultKeywordListTriggerMetadataResponse -
discord: DefaultReactionEmojiResponse
Fields
- emoji_id anydata? -
- emoji_name string? -
discord: Delete_original_webhook_messageQueries
Represents the Queries record for the operation: delete_original_webhook_message
Fields
- thread_id string? -
discord: Delete_webhook_messageQueries
Represents the Queries record for the operation: delete_webhook_message
Fields
- thread_id string? -
discord: DiscordIntegrationResponse
Fields
- 'type "discord" -
- name string? -
- account anydata? -
- enabled boolean? -
- id string -
- application IntegrationApplicationResponse -
- scopes OAuth2Scopes[] -
- user anydata? -
discord: Emoji
Fields
- id anydata? -
- name string -
- animated boolean? -
discord: EmojiResponse
Fields
- id string -
- name string -
- user anydata? -
- roles EmojiResponseRolesItemsString[] -
- require_colons boolean -
- managed boolean -
- animated boolean -
- available boolean -
discord: EntitlementResponse
Fields
- id string -
- sku_id string -
- application_id string -
- user_id string -
- guild_id anydata? -
- deleted boolean -
- starts_at string? -
- ends_at string? -
- 'type EntitlementTypes -
- fulfilled_at string? -
- fulfillment_status anydata? -
- consumed boolean? -
discord: EntityMetadataExternal
Fields
- location string -
discord: EntityMetadataExternalResponse
Fields
- location string -
discord: Execute_github_compatible_webhookQueries
Represents the Queries record for the operation: execute_github_compatible_webhook
Fields
- 'wait boolean? -
- thread_id string? -
discord: Execute_slack_compatible_webhookHeaders
Represents the Headers record for the operation: execute_slack_compatible_webhook
Fields
- Content\-Type "application/x-www-form-urlencoded" -
discord: Execute_slack_compatible_webhookQueries
Represents the Queries record for the operation: execute_slack_compatible_webhook
Fields
- 'wait boolean? -
- thread_id string? -
discord: Execute_webhookQueries
Represents the Queries record for the operation: execute_webhook
Fields
- 'wait boolean? -
- thread_id string? -
discord: ExternalConnectionIntegrationResponse
Fields
- 'type IntegrationTypes -
- name string? -
- account anydata? -
- enabled boolean? -
- id string -
- user UserResponse -
- revoked boolean? -
- expire_behavior anydata? -
- expire_grace_period anydata? -
- subscriber_count Signed32? -
- synced_at string? -
- role_id anydata? -
- syncing boolean? -
- enable_emoticons boolean? -
discord: ExternalScheduledEventCreateRequest
Fields
- name string -
- description string? -
- image record { fileContent byte[], fileName string }? -
- scheduled_start_time string -
- scheduled_end_time string? -
- privacy_level GuildScheduledEventPrivacyLevels -
- entity_type 3 -
- channel_id anydata? -
- entity_metadata EntityMetadataExternal -
discord: ExternalScheduledEventPatchRequestPartial
Fields
- status anydata? -
- name string? -
- description string? -
- image record { fileContent byte[], fileName string }? -
- scheduled_start_time string? -
- scheduled_end_time string? -
- entity_type anydata? -
- privacy_level GuildScheduledEventPrivacyLevels? -
- channel_id anydata? -
- entity_metadata EntityMetadataExternal? -
discord: ExternalScheduledEventResponse
Fields
- id string -
- guild_id string -
- name string -
- description string? -
- channel_id anydata? -
- creator_id anydata? -
- creator anydata? -
- image string? -
- scheduled_start_time string -
- scheduled_end_time string? -
- status GuildScheduledEventStatuses -
- entity_type 3 -
- entity_id anydata? -
- user_count Signed32? -
- privacy_level GuildScheduledEventPrivacyLevels -
- user_rsvp anydata? -
- entity_metadata EntityMetadataExternalResponse -
discord: FlagToChannelAction
Fields
- 'type 2 -
- metadata FlagToChannelActionMetadata -
discord: FlagToChannelActionMetadata
Fields
- channel_id string -
discord: FlagToChannelActionMetadataResponse
Fields
- channel_id string -
discord: FlagToChannelActionResponse
Fields
- 'type 2 -
- metadata FlagToChannelActionMetadataResponse -
discord: ForumTagResponse
Fields
- id string -
- name string -
- moderated boolean -
- emoji_id anydata? -
- emoji_name string? -
discord: FriendInviteResponse
Fields
- 'type anydata? -
- code string -
- inviter anydata? -
- max_age Signed32? -
- created_at string? -
- expires_at string? -
- friends_count Signed32? -
- channel anydata? -
- is_contact boolean? -
- uses Signed32? -
- max_uses Signed32? -
- flags Signed32? -
discord: GatewayBotResponse
Fields
- url string -
- session_start_limit GatewayBotSessionStartLimitResponse -
- shards Signed32 -
discord: GatewayBotSessionStartLimitResponse
Fields
- max_concurrency Signed32 -
- remaining Signed32 -
- reset_after Signed32 -
- total Signed32 -
discord: GatewayResponse
Fields
- url string -
discord: Get_entitlementsQueries
Represents the Queries record for the operation: get_entitlements
Fields
- exclude_ended boolean? -
- user_id string? -
- before string? -
- sku_ids sku_ids -
- guild_id string? -
- 'limit Signed32? -
- after string? -
- only_active boolean? -
discord: Get_guild_scheduled_eventQueries
Represents the Queries record for the operation: get_guild_scheduled_event
Fields
- with_user_count boolean? -
discord: Get_guild_widget_pngQueries
Represents the Queries record for the operation: get_guild_widget_png
Fields
- style WidgetImageStyles? -
discord: Get_guildQueries
Represents the Queries record for the operation: get_guild
Fields
- with_counts boolean? -
discord: Get_original_webhook_messageQueries
Represents the Queries record for the operation: get_original_webhook_message
Fields
- thread_id string? -
discord: Get_thread_memberQueries
Represents the Queries record for the operation: get_thread_member
Fields
- with_member boolean? -
discord: Get_webhook_messageQueries
Represents the Queries record for the operation: get_webhook_message
Fields
- thread_id string? -
discord: GithubAuthor
Fields
- username string? -
- name string -
discord: GithubCheckApp
Fields
- name string -
discord: GithubCheckPullRequest
Fields
- number Signed32 -
discord: GithubCheckRun
Fields
- conclusion string? -
- name string -
- html_url string -
- check_suite GithubCheckSuite -
- details_url string? -
- output anydata? -
- pull_requests GithubCheckPullRequest[]? -
discord: GithubCheckRunOutput
Fields
- title string? -
- summary string? -
discord: GithubCheckSuite
Fields
- conclusion string? -
- head_branch string? -
- head_sha string -
- pull_requests GithubCheckPullRequest[]? -
- app GithubCheckApp -
discord: GithubComment
Fields
- id Signed32 -
- html_url string -
- user GithubUser -
- commit_id string? -
- body string -
discord: GithubCommit
Fields
- id string -
- url string -
- message string -
- author GithubAuthor -
discord: GithubDiscussion
Fields
- title string -
- number Signed32 -
- html_url string -
- answer_html_url string? -
- body string? -
- user GithubUser -
discord: GithubIssue
Fields
- id Signed32 -
- number Signed32 -
- html_url string -
- user GithubUser -
- title string -
- body string? -
- pull_request anydata? -
discord: GithubRelease
Fields
- id Signed32 -
- tag_name string -
- html_url string -
- author GithubUser -
discord: GithubRepository
Fields
- id Signed32 -
- html_url string -
- name string -
- full_name string -
discord: GithubReview
Fields
- user GithubUser -
- body string? -
- html_url string -
- state string -
discord: GithubUser
Fields
- id Signed32 -
- login string -
- html_url string -
- avatar_url string -
discord: GithubWebhook
Fields
- action string? -
- ref string? -
- ref_type string? -
- comment anydata? -
- issue anydata? -
- pull_request anydata? -
- repository anydata? -
- forkee anydata? -
- sender GithubUser -
- member anydata? -
- release anydata? -
- head_commit anydata? -
- commits GithubCommit[]? -
- forced boolean? -
- compare string? -
- review anydata? -
- check_run anydata? -
- check_suite anydata? -
- discussion anydata? -
- answer anydata? -
discord: GroupDMInviteResponse
Fields
- 'type anydata? -
- code string -
- inviter anydata? -
- max_age Signed32? -
- created_at string? -
- expires_at string? -
- channel anydata? -
- approximate_member_count Signed32? -
discord: guild_id_stickers_body
Fields
- name string -
- tags string -
- description string? -
- file record { fileContent byte[], fileName string } -
discord: GuildAuditLogResponse
Fields
- audit_log_entries AuditLogEntryResponse[] -
- users UserResponse[] -
- guild_scheduled_events (ExternalScheduledEventResponse|StageScheduledEventResponse|VoiceScheduledEventResponse)[] -
- threads ThreadResponse[] -
- application_commands ApplicationCommandResponse[] -
- auto_moderation_rules (DefaultKeywordRuleResponse|KeywordRuleResponse|MLSpamRuleResponse|MentionSpamRuleResponse|SpamLinkRuleResponse)[] -
discord: GuildBanResponse
Fields
- user UserResponse -
- reason string? -
discord: GuildChannelResponse
Fields
- id string -
- 'type ChannelTypes -
- last_message_id anydata? -
- flags Signed32 -
- last_pin_timestamp string? -
- guild_id string -
- name string -
- parent_id anydata? -
- rate_limit_per_user Signed32? -
- bitrate Signed32? -
- user_limit Signed32? -
- rtc_region string? -
- video_quality_mode anydata? -
- permissions string? -
- topic string? -
- default_auto_archive_duration anydata? -
- default_thread_rate_limit_per_user Signed32? -
- position Signed32 -
- permission_overwrites ChannelPermissionOverwriteResponse[]? -
- nsfw boolean? -
- available_tags ForumTagResponse[]? -
- default_reaction_emoji anydata? -
- default_sort_order anydata? -
- default_forum_layout anydata? -
discord: GuildCreateRequest
Fields
- description string? -
- name string -
- region string? -
- icon record { fileContent byte[], fileName string }? -
- verification_level anydata? -
- default_message_notifications anydata? -
- explicit_content_filter anydata? -
- preferred_locale anydata? -
- afk_timeout anydata? -
- roles CreateGuildRequestRoleItem[]? -
- channels CreateGuildRequestChannelItem[]? -
- afk_channel_id anydata? -
- system_channel_id anydata? -
- system_channel_flags Signed32? -
discord: GuildHomeSettingsResponse
Fields
- guild_id string -
- enabled boolean -
- welcome_message anydata? -
- new_member_actions (anydata)[]? -
- resource_channels (anydata)[]? -
discord: GuildIncomingWebhookResponse
Fields
- application_id anydata? -
- avatar string? -
- channel_id anydata? -
- guild_id anydata? -
- id string -
- name string -
- 'type 1 -
- user anydata? -
- token string? -
- url string? -
discord: GuildInviteResponse
Fields
- 'type anydata? -
- code string -
- inviter anydata? -
- max_age Signed32? -
- created_at string? -
- expires_at string? -
- is_contact boolean? -
- flags Signed32? -
- guild anydata? -
- guild_id anydata? -
- channel anydata? -
- stage_instance anydata? -
- target_type anydata? -
- target_user anydata? -
- target_application anydata? -
- guild_scheduled_event anydata? -
- uses Signed32? -
- max_uses Signed32? -
- temporary boolean? -
- approximate_member_count Signed32? -
- approximate_presence_count Signed32? -
discord: GuildMemberResponse
Fields
- avatar string? -
- avatar_decoration_data anydata? -
- communication_disabled_until string? -
- flags Signed32 -
- joined_at string -
- nick string? -
- pending boolean -
- premium_since string? -
- roles GuildMemberResponseRolesItemsString[] -
- user UserResponse -
- mute boolean -
- deaf boolean -
discord: GuildMFALevelResponse
Fields
- level GuildMFALevel -
discord: GuildOnboardingResponse
Fields
- guild_id string -
- prompts OnboardingPromptResponse[] -
- default_channel_ids GuildOnboardingResponseDefaultchannelidsItemsString[] -
- enabled boolean -
discord: GuildPatchRequestPartial
Fields
- name string? -
- description string? -
- region string? -
- icon record { fileContent byte[], fileName string }? -
- verification_level anydata? -
- default_message_notifications anydata? -
- explicit_content_filter anydata? -
- preferred_locale anydata? -
- afk_timeout anydata? -
- afk_channel_id anydata? -
- system_channel_id anydata? -
- owner_id string? -
- splash record { fileContent byte[], fileName string }? -
- banner record { fileContent byte[], fileName string }? -
- system_channel_flags Signed32? -
- features GuildPatchRequestPartialFeaturesItemsString[]? -
- discovery_splash record { fileContent byte[], fileName string }? -
- home_header record { fileContent byte[], fileName string }? -
- rules_channel_id anydata? -
- safety_alerts_channel_id anydata? -
- public_updates_channel_id anydata? -
- premium_progress_bar_enabled boolean? -
discord: GuildPreviewResponse
Fields
- id string -
- name string -
- icon string? -
- description string? -
- home_header string? -
- splash string? -
- discovery_splash string? -
- features GuildFeatures[] -
- approximate_member_count Signed32 -
- approximate_presence_count Signed32 -
- emojis EmojiResponse[] -
- stickers GuildStickerResponse[] -
discord: GuildProductPurchaseResponse
Fields
- listing_id string -
- product_name string -
discord: GuildPruneResponse
Fields
- pruned Signed32? -
discord: GuildResponse
Fields
- id string -
- name string -
- icon string? -
- description string? -
- home_header string? -
- splash string? -
- discovery_splash string? -
- features GuildFeatures[] -
- banner string? -
- owner_id string -
- application_id anydata? -
- region string -
- afk_channel_id anydata? -
- afk_timeout AfkTimeouts -
- system_channel_id anydata? -
- system_channel_flags Signed32 -
- widget_enabled boolean -
- widget_channel_id anydata? -
- verification_level VerificationLevels -
- roles GuildRoleResponse[] -
- default_message_notifications UserNotificationSettings -
- mfa_level GuildMFALevel -
- explicit_content_filter GuildExplicitContentFilterTypes -
- max_presences Signed32? -
- max_members Signed32? -
- max_stage_video_channel_users Signed32? -
- max_video_channel_users Signed32? -
- vanity_url_code string? -
- premium_tier PremiumGuildTiers -
- premium_subscription_count Signed32 -
- preferred_locale AvailableLocalesEnum -
- rules_channel_id anydata? -
- safety_alerts_channel_id anydata? -
- public_updates_channel_id anydata? -
- premium_progress_bar_enabled boolean -
- nsfw boolean -
- nsfw_level GuildNSFWContentLevel -
- emojis EmojiResponse[] -
- stickers GuildStickerResponse[] -
discord: GuildRoleResponse
Fields
- id string -
- name string -
- description string? -
- permissions string -
- position Signed32 -
- color Signed32 -
- hoist boolean -
- managed boolean -
- mentionable boolean -
- icon string? -
- unicode_emoji string? -
- tags anydata? -
discord: GuildRoleTagsResponse
Fields
- premium_subscriber string? -
- bot_id anydata? -
- integration_id anydata? -
- subscription_listing_id anydata? -
- available_for_purchase string? -
- guild_connections string? -
discord: GuildsBansRequest
Fields
- delete_message_seconds Signed32? -
- delete_message_days Signed32? -
discord: GuildsBulkBanRequest
Fields
- user_ids GuildsBulkBanRequestUseridsItemsString[] -
- delete_message_seconds Signed32? -
discord: GuildsChannelsRequest
Fields
- id string? -
- position Signed32? -
- parent_id anydata? -
- lock_permissions boolean? -
discord: GuildsEmojisRequest
Fields
- name string? -
- roles (anydata)[]? -
discord: GuildsEmojisRequest1
Fields
- name string -
- image record { fileContent byte[], fileName string } -
- roles (anydata)[]? -
discord: GuildsMembersMeRequest
Fields
- nick string? -
discord: GuildsMembersRequest
Fields
- nick string? -
- roles (anydata)[]? -
- mute boolean? -
- deaf boolean? -
- access_token string -
- flags Signed32? -
discord: GuildsMembersRequest1
Fields
- nick string? -
- roles (anydata)[]? -
- mute boolean? -
- deaf boolean? -
- channel_id anydata? -
- communication_disabled_until string? -
- flags Signed32? -
discord: GuildsPruneRequest
Fields
- days Signed32? -
- compute_prune_count boolean? -
- include_roles anydata? -
discord: GuildsRolesRequest
Fields
- name string? -
- permissions Signed32? -
- color Signed32? -
- hoist boolean? -
- mentionable boolean? -
- icon record { fileContent byte[], fileName string }? -
- unicode_emoji string? -
discord: GuildsRolesRequest2
Fields
- id anydata? -
- position Signed32? -
discord: GuildsStickersRequest
Fields
- name string? -
- tags string? -
- description string? -
discord: GuildsTemplatesRequest
Fields
- name string -
- icon record { fileContent byte[], fileName string }? -
discord: GuildsTemplatesRequest1
Fields
- name string? -
- description string? -
discord: GuildsTemplatesRequest2
Fields
- name string -
- description string? -
discord: GuildStickerResponse
Fields
- id string -
- name string -
- tags string -
- 'type 2 -
- format_type anydata? -
- description string? -
- available boolean -
- guild_id string -
- user anydata? -
discord: GuildSubscriptionIntegrationResponse
Fields
- 'type "guild_subscription" -
- name string? -
- account anydata? -
- enabled boolean? -
- id string -
discord: GuildsVoiceStatesMeRequest
Fields
- request_to_speak_timestamp string? -
- suppress boolean? -
- channel_id anydata? -
discord: GuildsVoiceStatesRequest
Fields
- suppress boolean? -
- channel_id anydata? -
discord: GuildsWidgetRequest
Fields
- channel_id anydata? -
- enabled boolean? -
discord: GuildTemplateChannelResponse
Fields
- id Signed32? -
- 'type ChannelTypes -
- name string? -
- position Signed32? -
- topic string? -
- bitrate Signed32 -
- user_limit Signed32 -
- nsfw boolean -
- rate_limit_per_user Signed32 -
- parent_id anydata? -
- default_auto_archive_duration anydata? -
- permission_overwrites (ChannelPermissionOverwriteResponse)[] -
- available_tags GuildTemplateChannelTags[]? -
- template string -
- default_reaction_emoji anydata? -
- default_thread_rate_limit_per_user Signed32? -
- default_sort_order anydata? -
- default_forum_layout anydata? -
- icon_emoji anydata? -
- theme_color Signed32? -
discord: GuildTemplateChannelTags
Fields
- name string -
- emoji_id anydata? -
- emoji_name string? -
- moderated boolean? -
discord: GuildTemplateResponse
Fields
- code string -
- name string -
- description string? -
- usage_count Signed32 -
- creator_id string -
- creator anydata? -
- created_at string -
- updated_at string -
- source_guild_id string -
- serialized_source_guild GuildTemplateSnapshotResponse -
- is_dirty boolean? -
discord: GuildTemplateRoleResponse
Fields
- id Signed32 -
- name string -
- permissions string -
- color Signed32 -
- hoist boolean -
- mentionable boolean -
- icon string? -
- unicode_emoji string? -
discord: GuildTemplateSnapshotResponse
Fields
- name string -
- description string? -
- region string? -
- verification_level VerificationLevels -
- default_message_notifications UserNotificationSettings -
- explicit_content_filter GuildExplicitContentFilterTypes -
- preferred_locale AvailableLocalesEnum -
- afk_channel_id anydata? -
- afk_timeout AfkTimeouts -
- system_channel_id anydata? -
- system_channel_flags Signed32 -
- roles GuildTemplateRoleResponse[] -
- channels GuildTemplateChannelResponse[] -
discord: GuildWelcomeChannel
Fields
- channel_id string -
- description string -
- emoji_id anydata? -
- emoji_name string? -
discord: GuildWelcomeScreenChannelResponse
Fields
- channel_id string -
- description string -
- emoji_id anydata? -
- emoji_name string? -
discord: GuildWelcomeScreenResponse
Fields
- description string? -
- welcome_channels GuildWelcomeScreenChannelResponse[] -
discord: GuildWithCountsResponse
Fields
- id string -
- name string -
- icon string? -
- description string? -
- home_header string? -
- splash string? -
- discovery_splash string? -
- features GuildFeatures[] -
- banner string? -
- owner_id string -
- application_id anydata? -
- region string -
- afk_channel_id anydata? -
- afk_timeout AfkTimeouts -
- system_channel_id anydata? -
- system_channel_flags Signed32 -
- widget_enabled boolean -
- widget_channel_id anydata? -
- verification_level VerificationLevels -
- roles GuildRoleResponse[] -
- default_message_notifications UserNotificationSettings -
- mfa_level GuildMFALevel -
- explicit_content_filter GuildExplicitContentFilterTypes -
- max_presences Signed32? -
- max_members Signed32? -
- max_stage_video_channel_users Signed32? -
- max_video_channel_users Signed32? -
- vanity_url_code string? -
- premium_tier PremiumGuildTiers -
- premium_subscription_count Signed32 -
- preferred_locale AvailableLocalesEnum -
- rules_channel_id anydata? -
- safety_alerts_channel_id anydata? -
- public_updates_channel_id anydata? -
- premium_progress_bar_enabled boolean -
- nsfw boolean -
- nsfw_level GuildNSFWContentLevel -
- emojis EmojiResponse[] -
- stickers GuildStickerResponse[] -
- approximate_member_count Signed32? -
- approximate_presence_count Signed32? -
discord: IncomingWebhookInteractionRequest
Fields
- content string? -
- embeds RichEmbed[]? -
- allowed_mentions anydata? -
- components ActionRow[]? -
- attachments MessageAttachmentRequest[]? -
- tts boolean? -
- flags Signed32? -
discord: IncomingWebhookRequestPartial
Fields
- content string? -
- embeds RichEmbed[]? -
- allowed_mentions anydata? -
- components ActionRow[]? -
- attachments MessageAttachmentRequest[]? -
- tts boolean? -
- flags Signed32? -
- username string? -
- avatar_url string? -
- thread_name string? -
- applied_tags IncomingWebhookRequestPartialAppliedtagsItemsString[]? -
discord: IncomingWebhookUpdateForInteractionCallbackRequestPartial
Fields
- content string? -
- embeds RichEmbed[]? -
- allowed_mentions anydata? -
- components ActionRow[]? -
- attachments MessageAttachmentRequest[]? -
- flags Signed32? -
discord: IncomingWebhookUpdateRequestPartial
Fields
- content string? -
- embeds RichEmbed[]? -
- allowed_mentions anydata? -
- components ActionRow[]? -
- attachments MessageAttachmentRequest[]? -
- flags Signed32? -
discord: InputText
Fields
- 'type 4 -
- custom_id string -
- style TextStyleTypes -
- label string -
- value string? -
- placeholder string? -
- required boolean? -
- min_length Signed32? -
- max_length Signed32? -
discord: IntegrationApplicationResponse
Fields
- id string -
- name string -
- icon string? -
- description string -
- 'type anydata? -
- cover_image string? -
- primary_sku_id anydata? -
- bot anydata? -
discord: InteractionApplicationCommandAutocompleteCallbackIntegerData
Fields
- choices (anydata)[]? -
discord: InteractionApplicationCommandAutocompleteCallbackNumberData
Fields
- choices (anydata)[]? -
discord: InteractionApplicationCommandAutocompleteCallbackStringData
Fields
- choices (anydata)[]? -
discord: Invite_resolveQueries
Represents the Queries record for the operation: invite_resolve
Fields
- with_counts boolean? -
- guild_scheduled_event_id string? -
discord: InviteApplicationResponse
Fields
- id string -
- name string -
- icon string? -
- description string -
- 'type anydata? -
- cover_image string? -
- primary_sku_id anydata? -
- bot anydata? -
- slug string? -
- guild_id anydata? -
- rpc_origins string[]? -
- bot_public boolean? -
- bot_require_code_grant boolean? -
- terms_of_service_url string? -
- privacy_policy_url string? -
- custom_install_url string? -
- install_params anydata? -
- verify_key string -
- flags Signed32 -
- max_participants Signed32? -
- tags string[]? -
discord: InviteChannelRecipientResponse
Fields
- username string -
discord: InviteChannelResponse
Fields
- id string -
- 'type ChannelTypes -
- name string? -
- icon string? -
- recipients InviteChannelRecipientResponse[]? -
discord: InviteGuildResponse
Fields
- id string -
- name string -
- splash string? -
- banner string? -
- description string? -
- icon string? -
- features GuildFeatures[] -
- verification_level anydata? -
- vanity_url_code string? -
- nsfw_level anydata? -
- nsfw boolean? -
- premium_subscription_count Signed32? -
discord: InviteStageInstanceResponse
Fields
- topic string -
- participant_count Signed32? -
- speaker_count Signed32? -
- members GuildMemberResponse[]? -
discord: KeywordRuleResponse
Fields
- id string -
- guild_id string -
- creator_id string -
- name string -
- event_type AutomodEventType -
- trigger_type 1 -
- enabled boolean? -
- exempt_roles KeywordRuleResponseExemptrolesItemsString[]? -
- exempt_channels KeywordRuleResponseExemptchannelsItemsString[]? -
- trigger_metadata KeywordTriggerMetadataResponse -
discord: KeywordTriggerMetadata
Fields
- keyword_filter KeywordTriggerMetadataKeywordfilterItemsString[]? -
- regex_patterns KeywordTriggerMetadataRegexpatternsItemsString[]? -
- allow_list KeywordTriggerMetadataAllowlistItemsString[]? -
discord: KeywordTriggerMetadataResponse
Fields
- keyword_filter string[] -
- regex_patterns string[] -
- allow_list string[] -
discord: KeywordUpsertRequest
Fields
- name string -
- event_type AutomodEventType -
- actions (anydata)[]? -
- enabled boolean? -
- exempt_roles KeywordUpsertRequestExemptrolesItemsString[]? -
- exempt_channels KeywordUpsertRequestExemptchannelsItemsString[]? -
- trigger_type 1 -
- trigger_metadata anydata? -
discord: KeywordUpsertRequestPartial
Fields
- name string? -
- event_type AutomodEventType? -
- actions (anydata)[]? -
- enabled boolean? -
- exempt_roles KeywordUpsertRequestPartialExemptrolesItemsString[]? -
- exempt_channels KeywordUpsertRequestPartialExemptchannelsItemsString[]? -
- trigger_type AutomodTriggerType? -
- trigger_metadata anydata? -
discord: List_application_commandsQueries
Represents the Queries record for the operation: list_application_commands
Fields
- with_localizations boolean? -
discord: List_guild_application_commandsQueries
Represents the Queries record for the operation: list_guild_application_commands
Fields
- with_localizations boolean? -
discord: List_guild_audit_log_entriesQueries
Represents the Queries record for the operation: list_guild_audit_log_entries
Fields
- user_id string? -
- action_type Signed32? -
- before string? -
- 'limit Signed32? -
- target_id string? -
- after string? -
discord: List_guild_bansQueries
Represents the Queries record for the operation: list_guild_bans
Fields
- before string? -
- 'limit Signed32? -
- after string? -
discord: List_guild_membersQueries
Represents the Queries record for the operation: list_guild_members
Fields
- 'limit Signed32? -
- after Signed32? -
discord: List_guild_scheduled_event_usersQueries
Represents the Queries record for the operation: list_guild_scheduled_event_users
Fields
- before string? -
- 'limit Signed32? -
- after string? -
- with_member boolean? -
discord: List_guild_scheduled_eventsQueries
Represents the Queries record for the operation: list_guild_scheduled_events
Fields
- with_user_count boolean? -
discord: List_message_reactions_by_emojiQueries
Represents the Queries record for the operation: list_message_reactions_by_emoji
Fields
- 'limit Signed32? -
- after string? -
discord: List_messagesQueries
Represents the Queries record for the operation: list_messages
Fields
- before string? -
- 'limit Signed32? -
- after string? -
- around string? -
discord: List_my_guildsQueries
Represents the Queries record for the operation: list_my_guilds
Fields
- with_counts boolean? -
- before string? -
- 'limit Signed32? -
- after string? -
discord: List_my_private_archived_threadsQueries
Represents the Queries record for the operation: list_my_private_archived_threads
Fields
- before string? -
- 'limit Signed32? -
discord: List_private_archived_threadsQueries
Represents the Queries record for the operation: list_private_archived_threads
Fields
- before string? -
- 'limit Signed32? -
discord: List_public_archived_threadsQueries
Represents the Queries record for the operation: list_public_archived_threads
Fields
- before string? -
- 'limit Signed32? -
discord: List_thread_membersQueries
Represents the Queries record for the operation: list_thread_members
Fields
- 'limit Signed32? -
- after string? -
- with_member boolean? -
discord: MentionableSelect
Fields
- 'type 7 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
- default_values (anydata)[]? -
discord: MentionSpamRuleResponse
Fields
- id string -
- guild_id string -
- creator_id string -
- name string -
- event_type AutomodEventType -
- trigger_type 5 -
- enabled boolean? -
- exempt_roles MentionSpamRuleResponseExemptrolesItemsString[]? -
- exempt_channels MentionSpamRuleResponseExemptchannelsItemsString[]? -
- trigger_metadata MentionSpamTriggerMetadataResponse -
discord: MentionSpamTriggerMetadata
Fields
- mention_total_limit Signed32 -
- mention_raid_protection_enabled boolean? -
discord: MentionSpamTriggerMetadataResponse
Fields
- mention_total_limit Signed32 -
discord: MentionSpamUpsertRequest
Fields
- name string -
- event_type AutomodEventType -
- actions (anydata)[]? -
- enabled boolean? -
- exempt_roles MentionSpamUpsertRequestExemptrolesItemsString[]? -
- exempt_channels MentionSpamUpsertRequestExemptchannelsItemsString[]? -
- trigger_type 5 -
- trigger_metadata anydata? -
discord: MentionSpamUpsertRequestPartial
Fields
- name string? -
- event_type AutomodEventType? -
- actions (anydata)[]? -
- enabled boolean? -
- exempt_roles MentionSpamUpsertRequestPartialExemptrolesItemsString[]? -
- exempt_channels MentionSpamUpsertRequestPartialExemptchannelsItemsString[]? -
- trigger_type AutomodTriggerType? -
- trigger_metadata anydata? -
discord: MessageAllowedMentionsRequest
Fields
- parse AllowedMentionTypes[]? -
- users (anydata)[]? -
- roles (anydata)[]? -
- replied_user boolean? -
discord: MessageAttachmentRequest
Fields
- id string -
- filename string? -
- description string? -
- is_remix boolean? -
discord: MessageAttachmentResponse
Fields
- id string -
- filename string -
- size Signed32 -
- url string -
- proxy_url string -
- width Signed32? -
- height Signed32? -
- duration_secs decimal? -
- waveform string? -
- description string? -
- content_type string? -
- ephemeral boolean? -
- title string? -
- application anydata? -
- clip_created_at string? -
- clip_participants UserResponse[]? -
discord: MessageCallResponse
Fields
- ended_timestamp string? -
- participants MessageCallResponseParticipantsItemsString[] -
discord: MessageComponentActionRowResponse
Fields
- 'type 1 -
- id Signed32 -
- components (anydata)[]? -
discord: MessageComponentButtonResponse
Fields
- 'type 2 -
- id Signed32 -
- custom_id string? -
- style ButtonStyleTypes -
- label string? -
- disabled boolean? -
- emoji anydata? -
- url string? -
- sku_id anydata? -
discord: MessageComponentChannelSelectResponse
Fields
- 'type 8 -
- id Signed32 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
- channel_types ChannelTypes[]? -
discord: MessageComponentEmojiResponse
Fields
- id anydata? -
- name string -
- animated boolean? -
discord: MessageComponentInputTextResponse
Fields
- 'type 4 -
- id Signed32 -
- custom_id string -
- style TextStyleTypes -
- label string? -
- value string? -
- placeholder string? -
- required boolean? -
- min_length Signed32? -
- max_length Signed32? -
discord: MessageComponentMentionableSelectResponse
Fields
- 'type 7 -
- id Signed32 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
discord: MessageComponentRoleSelectResponse
Fields
- 'type 6 -
- id Signed32 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
discord: MessageComponentStringSelectResponse
Fields
- 'type 3 -
- id Signed32 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
- options (anydata)[]? -
discord: MessageComponentUserSelectResponse
Fields
- 'type 5 -
- id Signed32 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
discord: MessageEmbedAuthorResponse
Fields
- name string -
- url string? -
- icon_url string? -
- proxy_icon_url string? -
discord: MessageEmbedFieldResponse
Fields
- name string -
- value string -
- inline boolean -
discord: MessageEmbedFooterResponse
Fields
- text string -
- icon_url string? -
- proxy_icon_url string? -
discord: MessageEmbedImageResponse
Fields
- url string? -
- proxy_url string? -
- width anydata? -
- height anydata? -
- placeholder string? -
- placeholder_version anydata? -
discord: MessageEmbedProviderResponse
Fields
- name string -
- url string? -
discord: MessageEmbedResponse
Fields
- 'type string -
- url string? -
- title string? -
- description string? -
- color Signed32? -
- timestamp string? -
- fields MessageEmbedFieldResponse[]? -
- author anydata? -
- provider anydata? -
- image anydata? -
- thumbnail anydata? -
- video anydata? -
- footer anydata? -
discord: MessageEmbedVideoResponse
Fields
- url string? -
- proxy_url string? -
- width anydata? -
- height anydata? -
- placeholder string? -
- placeholder_version anydata? -
discord: MessageInteractionResponse
Fields
- id string -
- 'type InteractionTypes -
- name string -
- user anydata? -
- name_localized string? -
discord: MessageMentionChannelResponse
Fields
- id string -
- name string -
- 'type ChannelTypes -
- guild_id string -
discord: MessageReactionCountDetailsResponse
Fields
- burst Signed32 -
- normal Signed32 -
discord: MessageReactionEmojiResponse
Fields
- id anydata? -
- name string? -
- animated boolean? -
discord: MessageReactionResponse
Fields
- emoji MessageReactionEmojiResponse -
- count Signed32 -
- count_details MessageReactionCountDetailsResponse -
- burst_colors string[] -
- me_burst boolean -
- me boolean -
discord: MessageReferenceRequest
Fields
- guild_id anydata? -
- channel_id anydata? -
- message_id string -
- fail_if_not_exists boolean? -
- 'type anydata? -
discord: MessageReferenceResponse
Fields
- 'type anydata? -
- channel_id string -
- message_id anydata? -
- guild_id anydata? -
discord: MessageResponse
Fields
- 'type MessageType -
- channel_id string -
- content string -
- attachments MessageAttachmentResponse[] -
- embeds MessageEmbedResponse[] -
- timestamp string -
- edited_timestamp string? -
- flags Signed32 -
- components (MessageComponentActionRowResponse|MessageComponentButtonResponse|MessageComponentChannelSelectResponse|MessageComponentInputTextResponse|MessageComponentMentionableSelectResponse|MessageComponentRoleSelectResponse|MessageComponentStringSelectResponse|MessageComponentUserSelectResponse)[] -
- resolved anydata? -
- id string -
- author UserResponse -
- mentions UserResponse[] -
- mention_roles MessageResponseMentionrolesItemsString[] -
- pinned boolean -
- mention_everyone boolean -
- tts boolean -
- call anydata? -
- activity anydata? -
- application anydata? -
- application_id anydata? -
- interaction anydata? -
- nonce anydata? -
- webhook_id anydata? -
- message_reference anydata? -
- thread anydata? -
- mention_channels (anydata)[]? -
- stickers (anydata)[]? -
- sticker_items MessageStickerItemResponse[]? -
- role_subscription_data anydata? -
- purchase_notification anydata? -
- position Signed32? -
- reactions MessageReactionResponse[]? -
- referenced_message anydata? -
discord: MessageRoleSubscriptionDataResponse
Fields
- role_subscription_listing_id string -
- tier_name string -
- total_months_subscribed Signed32 -
- is_renewal boolean -
discord: messages_message_id_body
Fields
- content string? -
- embeds RichEmbed[]? -
- flags Signed32? -
- allowed_mentions anydata? -
- sticker_ids messages_message_id_bodyStickeridsItemsString[]? -
- components ActionRow[]? -
- attachments MessageAttachmentRequest[]? -
discord: messages_message_id_body_1
Fields
- content string? -
- embeds RichEmbed[]? -
- allowed_mentions anydata? -
- components ActionRow[]? -
- attachments MessageAttachmentRequest[]? -
- flags Signed32? -
discord: messages_original_body
Fields
- content string? -
- embeds RichEmbed[]? -
- allowed_mentions anydata? -
- components ActionRow[]? -
- attachments MessageAttachmentRequest[]? -
- flags Signed32? -
discord: MessageStickerItemResponse
Fields
- id string -
- name string -
- format_type StickerFormatTypes -
discord: MLSpamRuleResponse
Fields
- id string -
- guild_id string -
- creator_id string -
- name string -
- event_type AutomodEventType -
- trigger_type 3 -
- enabled boolean? -
- exempt_roles MLSpamRuleResponseExemptrolesItemsString[]? -
- exempt_channels MLSpamRuleResponseExemptchannelsItemsString[]? -
- trigger_metadata record {} -
discord: MLSpamUpsertRequest
Fields
- name string -
- event_type AutomodEventType -
- actions (anydata)[]? -
- enabled boolean? -
- exempt_roles MLSpamUpsertRequestExemptrolesItemsString[]? -
- exempt_channels MLSpamUpsertRequestExemptchannelsItemsString[]? -
- trigger_type 3 -
- trigger_metadata anydata? -
discord: MLSpamUpsertRequestPartial
Fields
- name string? -
- event_type AutomodEventType? -
- actions (anydata)[]? -
- enabled boolean? -
- exempt_roles MLSpamUpsertRequestPartialExemptrolesItemsString[]? -
- exempt_channels MLSpamUpsertRequestPartialExemptchannelsItemsString[]? -
- trigger_type AutomodTriggerType? -
- trigger_metadata anydata? -
discord: ModalInteractionCallbackData
Fields
- custom_id string -
- title string -
- components ActionRow[] -
discord: ModalInteractionCallbackRequest
Fields
- 'type 9 -
- data ModalInteractionCallbackData -
discord: MyGuildResponse
Fields
- id string -
- name string -
- icon string? -
- owner boolean -
- permissions string -
- features GuildFeatures[] -
- approximate_member_count Signed32? -
- approximate_presence_count Signed32? -
discord: NewMemberActionResponse
Fields
- channel_id string -
- action_type NewMemberActionType -
- title string -
- description string -
- emoji anydata? -
- icon string? -
discord: OAuth2ClientCredentialsGrantConfig
OAuth2 Client Credentials Grant Configs
Fields
- Fields Included from *OAuth2ClientCredentialsGrantConfig
- tokenUrl string(default "https://discord.com/api/oauth2/token") - Token URL
discord: OAuth2GetAuthorizationResponse
Fields
- application ApplicationResponse -
- expires string -
- scopes OAuth2Scopes[] -
- user anydata? -
discord: OAuth2GetKeys
Fields
- keys OAuth2Key[] -
discord: OAuth2Key
Fields
- kty string -
- use string -
- kid string -
- n string -
- e string -
- alg string -
discord: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://discord.com/api/oauth2/token") - Refresh URL
discord: OnboardingPromptOptionRequest
Fields
- id anydata? -
- title string -
- description string? -
- emoji_id anydata? -
- emoji_name string? -
- emoji_animated boolean? -
- role_ids OnboardingPromptOptionRequestRoleidsItemsString[]? -
- channel_ids OnboardingPromptOptionRequestChannelidsItemsString[]? -
discord: OnboardingPromptOptionResponse
Fields
- id string -
- title string -
- description string -
- emoji SettingsEmojiResponse -
- role_ids OnboardingPromptOptionResponseRoleidsItemsString[] -
- channel_ids OnboardingPromptOptionResponseChannelidsItemsString[] -
discord: OnboardingPromptResponse
Fields
- id string -
- title string -
- options OnboardingPromptOptionResponse[] -
- single_select boolean -
- required boolean -
- in_onboarding boolean -
- 'type OnboardingPromptType -
discord: PartialDiscordIntegrationResponse
Fields
- id string -
- 'type "discord" -
- name string? -
- account anydata? -
- application_id string -
discord: PartialExternalConnectionIntegrationResponse
Fields
- id string -
- 'type IntegrationTypes -
- name string? -
- account anydata? -
discord: PartialGuildSubscriptionIntegrationResponse
Fields
- id string -
- 'type "guild_subscription" -
- name string? -
- account anydata? -
discord: PongInteractionCallbackRequest
Fields
- 'type 1 -
discord: Preview_prune_guildQueries
Represents the Queries record for the operation: preview_prune_guild
Fields
- days Signed32? -
- include_roles include_roles? -
discord: PrivateApplicationResponse
Fields
- id string -
- name string -
- icon string? -
- description string -
- 'type anydata? -
- cover_image string? -
- primary_sku_id anydata? -
- bot anydata? -
- slug string? -
- guild_id anydata? -
- rpc_origins string[]? -
- bot_public boolean? -
- bot_require_code_grant boolean? -
- terms_of_service_url string? -
- privacy_policy_url string? -
- custom_install_url string? -
- install_params anydata? -
- verify_key string -
- flags Signed32 -
- max_participants Signed32? -
- tags string[]? -
- redirect_uris string[] -
- interactions_endpoint_url string? -
- role_connections_verification_url string? -
- owner UserResponse -
- approximate_guild_count Signed32? -
- team anydata? -
discord: PrivateChannelRequestPartial
Fields
- name string? -
- icon record { fileContent byte[], fileName string }? -
discord: PrivateChannelResponse
Fields
- id string -
- 'type 1 -
- last_message_id anydata? -
- flags Signed32 -
- last_pin_timestamp string? -
- recipients UserResponse[] -
discord: PrivateGroupChannelResponse
Fields
- id string -
- 'type 3 -
- last_message_id anydata? -
- flags Signed32 -
- last_pin_timestamp string? -
- recipients UserResponse[] -
- name string? -
- icon string? -
- owner_id anydata? -
- managed boolean? -
- application_id anydata? -
discord: PrivateGuildMemberResponse
Fields
- avatar string? -
- avatar_decoration_data anydata? -
- communication_disabled_until string? -
- flags Signed32 -
- joined_at string -
- nick string? -
- pending boolean -
- premium_since string? -
- user UserResponse -
- mute boolean -
- deaf boolean -
- banner string? -
discord: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
discord: PurchaseNotificationResponse
Fields
- 'type PurchaseType -
- guild_product_purchase anydata? -
discord: QuarantineUserAction
Fields
- 'type 4 -
- metadata anydata? -
discord: QuarantineUserActionResponse
Fields
- 'type 4 -
- metadata record {} -
discord: ResolvedObjectsResponse
Fields
- users record { UserResponse... } -
- members record { GuildMemberResponse... } -
- channels record {} -
- roles record { GuildRoleResponse... } -
discord: ResourceChannelResponse
Fields
- channel_id string -
- title string -
- emoji anydata? -
- icon string? -
- description string -
discord: RichEmbed
Fields
- 'type string? -
- url string? -
- title string? -
- color Signed32? -
- timestamp string? -
- description string? -
- author anydata? -
- image anydata? -
- thumbnail anydata? -
- footer anydata? -
- fields RichEmbedField[]? -
- provider anydata? -
- video anydata? -
discord: RichEmbedAuthor
Fields
- name string? -
- url string? -
- icon_url string? -
discord: RichEmbedField
Fields
- name string -
- value string -
- inline boolean? -
discord: RichEmbedFooter
Fields
- text string? -
- icon_url string? -
discord: RichEmbedImage
Fields
- url string? -
- width Signed32? -
- height Signed32? -
- placeholder string? -
- placeholder_version Signed32? -
discord: RichEmbedProvider
Fields
- name string? -
- url string? -
discord: RichEmbedThumbnail
Fields
- url string? -
- width Signed32? -
- height Signed32? -
- placeholder string? -
- placeholder_version Signed32? -
discord: RichEmbedVideo
Fields
- url string? -
- width Signed32? -
- height Signed32? -
- placeholder string? -
- placeholder_version Signed32? -
discord: RoleSelect
Fields
- 'type 6 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
- default_values RoleSelectDefaultValue[]? -
discord: RoleSelectDefaultValue
Fields
- 'type "role" -
- id string -
discord: ScheduledEventResponse
Fields
- id string -
- guild_id string -
- name string -
- description string? -
- channel_id anydata? -
- creator_id anydata? -
- creator anydata? -
- image string? -
- scheduled_start_time string -
- scheduled_end_time string? -
- status GuildScheduledEventStatuses -
- entity_type GuildScheduledEventEntityTypes -
- entity_id anydata? -
- user_count Signed32? -
- privacy_level GuildScheduledEventPrivacyLevels -
- user_rsvp anydata? -
discord: ScheduledEventUserResponse
Fields
- guild_scheduled_event_id string -
- user_id string -
- user anydata? -
- member anydata? -
discord: Search_guild_membersQueries
Represents the Queries record for the operation: search_guild_members
Fields
- query string -
- 'limit Signed32 -
discord: SelectOption
Fields
- label string -
- value string -
- description string? -
- emoji anydata? -
- default boolean? -
discord: SelectOptionResponse
Fields
- label string -
- value string -
- description string? -
- emoji anydata? -
- default boolean? -
discord: SettingsEmojiResponse
Fields
- id anydata? -
- name string? -
- animated boolean? -
discord: SpamLinkRuleResponse
Fields
- id string -
- guild_id string -
- creator_id string -
- name string -
- event_type AutomodEventType -
- trigger_type 2 -
- enabled boolean? -
- exempt_roles SpamLinkRuleResponseExemptrolesItemsString[]? -
- exempt_channels SpamLinkRuleResponseExemptchannelsItemsString[]? -
- trigger_metadata record {} -
discord: StageInstanceResponse
Fields
- guild_id string -
- channel_id string -
- topic string -
- privacy_level StageInstancesPrivacyLevels -
- id string -
- discoverable_disabled boolean? -
- guild_scheduled_event_id anydata? -
discord: StageInstancesRequest
Fields
- topic string -
- channel_id string -
- privacy_level anydata? -
- guild_scheduled_event_id anydata? -
- send_start_notification boolean? -
discord: StageInstancesRequest1
Fields
- topic string? -
- privacy_level StageInstancesPrivacyLevels? -
discord: StageScheduledEventCreateRequest
Fields
- name string -
- description string? -
- image record { fileContent byte[], fileName string }? -
- scheduled_start_time string -
- scheduled_end_time string? -
- privacy_level GuildScheduledEventPrivacyLevels -
- entity_type 1 -
- channel_id anydata? -
- entity_metadata anydata? -
discord: StageScheduledEventPatchRequestPartial
Fields
- status anydata? -
- name string? -
- description string? -
- image record { fileContent byte[], fileName string }? -
- scheduled_start_time string? -
- scheduled_end_time string? -
- entity_type anydata? -
- privacy_level GuildScheduledEventPrivacyLevels? -
- channel_id anydata? -
- entity_metadata anydata? -
discord: StageScheduledEventResponse
Fields
- id string -
- guild_id string -
- name string -
- description string? -
- channel_id anydata? -
- creator_id anydata? -
- creator anydata? -
- image string? -
- scheduled_start_time string -
- scheduled_end_time string? -
- status GuildScheduledEventStatuses -
- entity_type 1 -
- entity_id anydata? -
- user_count Signed32? -
- privacy_level GuildScheduledEventPrivacyLevels -
- user_rsvp anydata? -
- entity_metadata anydata? -
discord: StandardStickerResponse
Fields
- id string -
- name string -
- tags string -
- 'type 1 -
- format_type anydata? -
- description string? -
- pack_id string -
- sort_value Signed32 -
discord: StickerPackCollectionResponse
Fields
- sticker_packs StickerPackResponse[] -
discord: StickerPackResponse
Fields
- id string -
- sku_id string -
- name string -
- description string? -
- stickers StandardStickerResponse[] -
- cover_sticker_id anydata? -
- banner_asset_id anydata? -
discord: StringSelect
Fields
- 'type 3 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
- options SelectOption[] -
discord: TeamMemberResponse
Fields
- user UserResponse -
- team_id string -
- membership_state TeamMembershipStates -
discord: TeamResponse
Fields
- id string -
- icon string? -
- name string -
- owner_user_id string -
- members TeamMemberResponse[] -
discord: ThreadMemberResponse
Fields
- id string -
- user_id string -
- join_timestamp string -
- flags Signed32 -
- member anydata? -
discord: ThreadMetadataResponse
Fields
- archived boolean -
- archive_timestamp string? -
- auto_archive_duration ThreadAutoArchiveDuration -
- locked boolean -
- create_timestamp string? -
- invitable boolean? -
discord: ThreadResponse
Fields
- id string -
- 'type ChannelTypes -
- last_message_id anydata? -
- flags Signed32 -
- last_pin_timestamp string? -
- guild_id string -
- name string -
- parent_id anydata? -
- rate_limit_per_user Signed32? -
- bitrate Signed32? -
- user_limit Signed32? -
- rtc_region string? -
- video_quality_mode anydata? -
- permissions string? -
- owner_id string -
- thread_metadata anydata? -
- message_count Signed32 -
- member_count Signed32 -
- total_message_sent Signed32 -
- applied_tags ThreadResponseAppliedtagsItemsString[]? -
- member anydata? -
discord: ThreadsResponse
Fields
- threads ThreadResponse[] -
- members ThreadMemberResponse[] -
- has_more boolean? -
discord: Update_messageHeaders
Represents the Headers record for the operation: update_message
Fields
- Content\-Type "application/x-www-form-urlencoded" -
discord: Update_original_webhook_messageHeaders
Represents the Headers record for the operation: update_original_webhook_message
Fields
- Content\-Type "application/x-www-form-urlencoded" -
discord: Update_original_webhook_messageQueries
Represents the Queries record for the operation: update_original_webhook_message
Fields
- thread_id string? -
discord: Update_webhook_messageHeaders
Represents the Headers record for the operation: update_webhook_message
Fields
- Content\-Type "application/x-www-form-urlencoded" -
discord: Update_webhook_messageQueries
Represents the Queries record for the operation: update_webhook_message
Fields
- thread_id string? -
discord: UpdateDefaultReactionEmojiRequest
Fields
- emoji_id anydata? -
- emoji_name string? -
discord: UpdateGuildChannelRequestPartial
Fields
- 'type anydata? -
- name string? -
- position Signed32? -
- topic string? -
- bitrate Signed32? -
- user_limit Signed32? -
- nsfw boolean? -
- rate_limit_per_user Signed32? -
- parent_id anydata? -
- permission_overwrites ChannelPermissionOverwriteRequest[]? -
- rtc_region string? -
- video_quality_mode anydata? -
- default_auto_archive_duration anydata? -
- default_reaction_emoji anydata? -
- default_thread_rate_limit_per_user Signed32? -
- default_sort_order anydata? -
- default_forum_layout anydata? -
- flags Signed32? -
- available_tags UpdateThreadTagRequest[]? -
discord: UpdateGuildOnboardingRequest
Fields
- prompts UpdateOnboardingPromptRequest[]? -
- enabled boolean? -
- default_channel_ids UpdateGuildOnboardingRequestDefaultchannelidsItemsString[]? -
- mode anydata? -
discord: UpdateMessageInteractionCallbackRequest
Fields
- 'type InteractionCallbackTypes -
- data anydata? -
discord: UpdateOnboardingPromptRequest
Fields
- title string -
- options OnboardingPromptOptionRequest[] -
- single_select boolean? -
- required boolean? -
- in_onboarding boolean? -
- 'type anydata? -
- id string -
discord: UpdateThreadRequestPartial
Fields
- name string? -
- archived boolean? -
- locked boolean? -
- invitable boolean? -
- auto_archive_duration anydata? -
- rate_limit_per_user Signed32? -
- flags Signed32? -
- applied_tags UpdateThreadRequestPartialAppliedtagsItemsString[]? -
- bitrate Signed32? -
- user_limit Signed32? -
- rtc_region string? -
- video_quality_mode anydata? -
discord: UpdateThreadTagRequest
Fields
- name string -
- emoji_id anydata? -
- emoji_name string? -
- moderated boolean? -
- id anydata? -
discord: UserCommunicationDisabledAction
Fields
- 'type 3 -
- metadata UserCommunicationDisabledActionMetadata -
discord: UserCommunicationDisabledActionMetadata
Fields
- duration_seconds Signed32? -
discord: UserCommunicationDisabledActionMetadataResponse
Fields
- duration_seconds Signed32 -
discord: UserCommunicationDisabledActionResponse
Fields
- 'type 3 -
discord: UserGuildOnboardingResponse
Fields
- guild_id string -
- prompts OnboardingPromptResponse[] -
- default_channel_ids UserGuildOnboardingResponseDefaultchannelidsItemsString[] -
- enabled boolean -
discord: UserPIIResponse
Fields
- id string -
- username string -
- avatar string? -
- discriminator string -
- public_flags Signed32 -
- flags int -
- bot boolean? -
- system boolean? -
- banner string? -
- accent_color Signed32? -
- global_name string? -
- mfa_enabled boolean -
- locale AvailableLocalesEnum -
- premium_type anydata? -
- email string? -
- verified boolean? -
discord: UserResponse
Fields
- id string -
- username string -
- avatar string? -
- discriminator string -
- public_flags Signed32 -
- flags int -
- bot boolean? -
- system boolean? -
- banner string? -
- accent_color Signed32? -
- global_name string? -
discord: UserSelect
Fields
- 'type 5 -
- custom_id string -
- placeholder string? -
- min_values Signed32? -
- max_values Signed32? -
- disabled boolean? -
- default_values UserSelectDefaultValue[]? -
discord: UserSelectDefaultValue
Fields
- 'type "user" -
- id string -
discord: UsersMeApplicationsRoleConnectionRequest
Fields
- platform_name string? -
- platform_username string? -
- metadata record { string... }? -
discord: VanityURLErrorResponse
Fields
- message string -
- code Signed32 -
discord: VanityURLResponse
Fields
- code string? -
- uses Signed32 -
- 'error anydata? -
discord: VoiceRegionResponse
Fields
- id string -
- name string -
- custom boolean -
- deprecated boolean -
- optimal boolean -
discord: VoiceScheduledEventCreateRequest
Fields
- name string -
- description string? -
- image record { fileContent byte[], fileName string }? -
- scheduled_start_time string -
- scheduled_end_time string? -
- privacy_level GuildScheduledEventPrivacyLevels -
- entity_type 2 -
- channel_id anydata? -
- entity_metadata anydata? -
discord: VoiceScheduledEventPatchRequestPartial
Fields
- status anydata? -
- name string? -
- description string? -
- image record { fileContent byte[], fileName string }? -
- scheduled_start_time string? -
- scheduled_end_time string? -
- entity_type anydata? -
- privacy_level GuildScheduledEventPrivacyLevels? -
- channel_id anydata? -
- entity_metadata anydata? -
discord: VoiceScheduledEventResponse
Fields
- id string -
- guild_id string -
- name string -
- description string? -
- channel_id anydata? -
- creator_id anydata? -
- creator anydata? -
- image string? -
- scheduled_start_time string -
- scheduled_end_time string? -
- status GuildScheduledEventStatuses -
- entity_type 2 -
- entity_id anydata? -
- user_count Signed32? -
- privacy_level GuildScheduledEventPrivacyLevels -
- user_rsvp anydata? -
- entity_metadata anydata? -
discord: webhook_token_slack_body
Fields
- text string? -
- username string? -
- icon_url string? -
- attachments WebhookSlackEmbed[]? -
discord: WebhookSlackEmbed
Fields
- title string? -
- title_link string? -
- text string? -
- color string? -
- ts Signed32? -
- pretext string? -
- footer string? -
- footer_icon string? -
- author_name string? -
- author_link string? -
- author_icon string? -
- image_url string? -
- thumb_url string? -
- fields WebhookSlackEmbedField[]? -
discord: WebhookSlackEmbedField
Fields
- name string? -
- value string? -
- inline boolean? -
discord: WebhookSourceChannelResponse
Fields
- id string -
- name string -
discord: WebhookSourceGuildResponse
Fields
- id string -
- icon string? -
- name string -
discord: WebhooksRequest1
Fields
- name string? -
- avatar record { fileContent byte[], fileName string }? -
discord: WebhooksRequest2
Fields
- name string? -
- avatar record { fileContent byte[], fileName string }? -
- channel_id anydata? -
discord: WelcomeMessageResponse
Fields
- author_ids WelcomeMessageResponseAuthoridsItemsString[] -
- message string -
discord: WelcomeScreenPatchRequestPartial
Fields
- description string? -
- welcome_channels GuildWelcomeChannel[]? -
- enabled boolean? -
discord: WidgetActivity
Fields
- name string -
discord: WidgetChannel
Fields
- id string -
- name string -
- position Signed32 -
discord: WidgetMember
Fields
- id string -
- username string -
- discriminator WidgetUserDiscriminator -
- avatar string? -
- status string -
- avatar_url string -
- activity anydata? -
- deaf boolean? -
- mute boolean? -
- self_deaf boolean? -
- self_mute boolean? -
- suppress boolean? -
- channel_id anydata? -
discord: WidgetResponse
Fields
- id string -
- name string -
- instant_invite string? -
- channels WidgetChannel[] -
- members WidgetMember[] -
- presence_count Signed32 -
discord: WidgetSettingsResponse
Fields
- enabled boolean -
- channel_id anydata? -
Union types
discord: interaction_token_callback_body
interaction_token_callback_body
discord: GuildScheduledEventEntityTypes
GuildScheduledEventEntityTypes
discord: MessageType
MessageType
discord: MetadataItemTypes
MetadataItemTypes
discord: automoderation_rules_body
automoderation_rules_body
discord: AutomodEventType
AutomodEventType
discord: AutomodTriggerType
AutomodTriggerType
discord: GuildFeatures
GuildFeatures
discord: rules_rule_id_body
rules_rule_id_body
discord: AfkTimeouts
AfkTimeouts
discord: channel_id_threads_body
channel_id_threads_body
discord: InteractionTypes
InteractionTypes
discord: UserNotificationSettings
UserNotificationSettings
discord: IntegrationTypes
IntegrationTypes
discord: OnboardingPromptType
OnboardingPromptType
discord: InteractionCallbackTypes
InteractionCallbackTypes
discord: AvailableLocalesEnum
AvailableLocalesEnum
discord: inline_response_200
inline_response_200
discord: channels_channel_id_body
channels_channel_id_body
discord: guild_id_scheduledevents_body
guild_id_scheduledevents_body
discord: scheduledevents_guild_scheduled_event_id_body
scheduledevents_guild_scheduled_event_id_body
discord: ConnectedAccountProviders
ConnectedAccountProviders
discord: ChannelTypes
ChannelTypes
discord: AuditLogActionTypes
AuditLogActionTypes
discord: inline_response_200_5
inline_response_200_5
discord: inline_response_200_6
inline_response_200_6
discord: inline_response_200_1
inline_response_200_1
discord: inline_response_200_2
inline_response_200_2
discord: inline_response_200_3
inline_response_200_3
discord: inline_response_200_4
inline_response_200_4
discord: EntitlementTypes
EntitlementTypes
discord: OAuth2Scopes
OAuth2Scopes
discord: webhook_id_webhook_token_body
webhook_id_webhook_token_body
discord: GuildNSFWContentLevel
GuildNSFWContentLevel
discord: channel_id_invites_body
channel_id_invites_body
discord: StageInstancesPrivacyLevels
StageInstancesPrivacyLevels
discord: AutomodKeywordPresetType
AutomodKeywordPresetType
discord: GuildExplicitContentFilterTypes
GuildExplicitContentFilterTypes
Anydata types
discord: MessageAllowedMentionsRequestRolesItemsnull
MessageAllowedMentionsRequestRolesItemsnull
discord: GuildsMembersRequestRolesItemsnull
GuildsMembersRequestRolesItemsnull
discord: GuildsEmojisRequest1RolesItemsnull
GuildsEmojisRequest1RolesItemsnull
discord: GuildsEmojisRequestRolesItemsnull
GuildsEmojisRequestRolesItemsnull
discord: MessageAllowedMentionsRequestUsersItemsnull
MessageAllowedMentionsRequestUsersItemsnull
discord: GuildsMembersRequest1RolesItemsnull
GuildsMembersRequest1RolesItemsnull
String types
discord: KeywordUpsertRequestPartialExemptrolesItemsString
KeywordUpsertRequestPartialExemptrolesItemsString
discord: WelcomeMessageResponseAuthoridsItemsString
WelcomeMessageResponseAuthoridsItemsString
discord: CreatePrivateChannelRequestAccesstokensItemsString
CreatePrivateChannelRequestAccesstokensItemsString
discord: UpdateThreadRequestPartialAppliedtagsItemsString
UpdateThreadRequestPartialAppliedtagsItemsString
discord: PrivateGuildMemberResponseRolesItemsString
PrivateGuildMemberResponseRolesItemsString
discord: KeywordRuleResponseExemptchannelsItemsString
KeywordRuleResponseExemptchannelsItemsString
discord: OnboardingPromptOptionRequestChannelidsItemsString
OnboardingPromptOptionRequestChannelidsItemsString
discord: MLSpamUpsertRequestPartialExemptchannelsItemsString
MLSpamUpsertRequestPartialExemptchannelsItemsString
discord: ChannelsMessagesBulkDeleteRequestMessagesItemsString
ChannelsMessagesBulkDeleteRequestMessagesItemsString
discord: MLSpamUpsertRequestExemptchannelsItemsString
MLSpamUpsertRequestExemptchannelsItemsString
discord: CreateForumThreadRequestAppliedtagsItemsString
CreateForumThreadRequestAppliedtagsItemsString
discord: MLSpamRuleResponseExemptchannelsItemsString
MLSpamRuleResponseExemptchannelsItemsString
discord: MLSpamRuleResponseExemptrolesItemsString
MLSpamRuleResponseExemptrolesItemsString
discord: ThreadResponseAppliedtagsItemsString
ThreadResponseAppliedtagsItemsString
discord: MentionSpamUpsertRequestExemptrolesItemsString
MentionSpamUpsertRequestExemptrolesItemsString
discord: messages_message_id_bodyStickeridsItemsString
messages_message_id_bodyStickeridsItemsString
discord: BaseCreateMessageCreateRequestStickeridsItemsString
BaseCreateMessageCreateRequestStickeridsItemsString
discord: UpdateGuildOnboardingRequestDefaultchannelidsItemsString
UpdateGuildOnboardingRequestDefaultchannelidsItemsString
discord: GuildsBulkBanRequestUseridsItemsString
GuildsBulkBanRequestUseridsItemsString
discord: DefaultKeywordRuleResponseExemptchannelsItemsString
DefaultKeywordRuleResponseExemptchannelsItemsString
discord: MLSpamUpsertRequestExemptrolesItemsString
MLSpamUpsertRequestExemptrolesItemsString
discord: GuildMemberResponseRolesItemsString
GuildMemberResponseRolesItemsString
discord: DefaultKeywordListUpsertRequestPartialExemptchannelsItemsString
DefaultKeywordListUpsertRequestPartialExemptchannelsItemsString
discord: MentionSpamRuleResponseExemptchannelsItemsString
MentionSpamRuleResponseExemptchannelsItemsString
discord: IncomingWebhookRequestPartialAppliedtagsItemsString
IncomingWebhookRequestPartialAppliedtagsItemsString
discord: EmojiResponseRolesItemsString
EmojiResponseRolesItemsString
discord: DefaultKeywordListUpsertRequestPartialExemptrolesItemsString
DefaultKeywordListUpsertRequestPartialExemptrolesItemsString
discord: UserGuildOnboardingResponseDefaultchannelidsItemsString
UserGuildOnboardingResponseDefaultchannelidsItemsString
discord: GuildOnboardingResponseDefaultchannelidsItemsString
GuildOnboardingResponseDefaultchannelidsItemsString
discord: SkuidsItemsnull
SkuidsItemsnull
discord: MentionSpamRuleResponseExemptrolesItemsString
MentionSpamRuleResponseExemptrolesItemsString
discord: MLSpamUpsertRequestPartialExemptrolesItemsString
MLSpamUpsertRequestPartialExemptrolesItemsString
discord: DefaultKeywordListTriggerMetadataAllowlistItemsString
DefaultKeywordListTriggerMetadataAllowlistItemsString
discord: MessageResponseMentionrolesItemsString
MessageResponseMentionrolesItemsString
discord: MentionSpamUpsertRequestPartialExemptchannelsItemsString
MentionSpamUpsertRequestPartialExemptchannelsItemsString
discord: ApplicationFormPartialTagsItemsString
ApplicationFormPartialTagsItemsString
discord: BasicMessageResponseMentionrolesItemsString
BasicMessageResponseMentionrolesItemsString
discord: KeywordUpsertRequestPartialExemptchannelsItemsString
KeywordUpsertRequestPartialExemptchannelsItemsString
discord: KeywordTriggerMetadataAllowlistItemsString
KeywordTriggerMetadataAllowlistItemsString
discord: KeywordTriggerMetadataRegexpatternsItemsString
KeywordTriggerMetadataRegexpatternsItemsString
discord: SpamLinkRuleResponseExemptrolesItemsString
SpamLinkRuleResponseExemptrolesItemsString
discord: BulkBanUsersResponseFailedusersItemsString
BulkBanUsersResponseFailedusersItemsString
discord: BulkBanUsersResponseBannedusersItemsString
BulkBanUsersResponseBannedusersItemsString
discord: OnboardingPromptOptionResponseRoleidsItemsString
OnboardingPromptOptionResponseRoleidsItemsString
discord: OnboardingPromptOptionResponseChannelidsItemsString
OnboardingPromptOptionResponseChannelidsItemsString
discord: OnboardingPromptOptionRequestRoleidsItemsString
OnboardingPromptOptionRequestRoleidsItemsString
discord: channel_id_messages_bodyStickeridsItemsString
channel_id_messages_bodyStickeridsItemsString
discord: KeywordUpsertRequestExemptrolesItemsString
KeywordUpsertRequestExemptrolesItemsString
discord: CreatedThreadResponseAppliedtagsItemsString
CreatedThreadResponseAppliedtagsItemsString
discord: DefaultKeywordListUpsertRequestExemptrolesItemsString
DefaultKeywordListUpsertRequestExemptrolesItemsString
discord: MentionSpamUpsertRequestExemptchannelsItemsString
MentionSpamUpsertRequestExemptchannelsItemsString
discord: KeywordRuleResponseExemptrolesItemsString
KeywordRuleResponseExemptrolesItemsString
discord: KeywordTriggerMetadataKeywordfilterItemsString
KeywordTriggerMetadataKeywordfilterItemsString
discord: DefaultKeywordRuleResponseExemptrolesItemsString
DefaultKeywordRuleResponseExemptrolesItemsString
discord: DefaultKeywordListUpsertRequestExemptchannelsItemsString
DefaultKeywordListUpsertRequestExemptchannelsItemsString
discord: MentionSpamUpsertRequestPartialExemptrolesItemsString
MentionSpamUpsertRequestPartialExemptrolesItemsString
discord: KeywordUpsertRequestExemptchannelsItemsString
KeywordUpsertRequestExemptchannelsItemsString
discord: MessageCallResponseParticipantsItemsString
MessageCallResponseParticipantsItemsString
discord: SpamLinkRuleResponseExemptchannelsItemsString
SpamLinkRuleResponseExemptchannelsItemsString
discord: IncluderolesItemsnull
IncluderolesItemsnull
Simple name reference types
discord: PurchaseType
PurchaseType
discord: GuildScheduledEventPrivacyLevels
GuildScheduledEventPrivacyLevels
discord: WidgetUserDiscriminator
WidgetUserDiscriminator
Import
import ballerinax/discord;
Other versions
1.0.0
Metadata
Released date: 12 months ago
Version: 1.0.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.9.3
GraalVM compatible: Yes
Pull count
Total: 19
Current verison: 19
Weekly downloads
Keywords
Communication/discord
Cost/Free
Contributors