dayforce
Module dayforce
API
Definitions
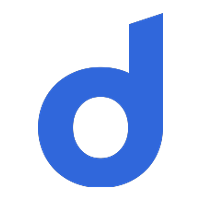
ballerinax/dayforce Ballerina library
Overview
Dayforce is a comprehensive human capital management system that covers the entire employee lifecycle including HR, payroll, benefits, talent management, workforce management, and services. The entire system resides on cloud that takes the burden of managing and replicating data on-premise.
The Dayforce connector allows you to access the REST API of Dayforce HCM specifically based on Rest API version v1.
This connector has been tested exclusively in the Dayforce developer sample environment. If you encounter any technical issues, please reach out to us using the links provided in the Useful links section.
Setup guide
Step 1: Create a Dayforce account
-
Navigate to the Dayforce website and register. Follow the instructions to create an account. If your company has already purchased a namespace in Dayforce, use that to sign up. Otherwise, you can still use their
sample
environment by selectingsample
option. -
Once you have registered, you will receive an email with a link to activate your account.
-
Click on the link sent in the email to complete the registration process.
-
After confirming your registration, you'll get a success message. Click on the "Sign In" button to log in to your account.
-
Enter your email address and password to log in.
Step 2: Obtain the user credentials to access the Dayforce API
To get the credentials for the sample
environment, follow the instructions below:
- Navigate to API Explorer -> Employee -> GET Employees.
- Locate the basic authentication credentials, including the
username
andpassword
, for the sample environment.
Quickstart
To use the dayforce
connector in your Ballerina project, modify the .bal
file as follows:
Step 1: Import the module
Import the ballerinax/dayforce
module into your Ballerina project.
import ballerinax/dayforce;
Step 2: Instantiate a new connector
Instantiate a new dayforce:Client
giving the auth details.
dayforce:Client dayforce = check new ( { auth: { username: "<username>", password: "<password>" } }, "https://www.dayforcehcm.com/Api/ddn/V1/");
Step 3: Invoke the connector operation
Now, utilize the available connector operations.
public function main() returns error? { dayforce:Payload_Employee employee = check dayforce->/ddn/V1/Employees/'42199; }
Step 4: Run the Ballerina application
Use the following command to compile and run the Ballerina program.
bal run
Useful links
- Chat live with us via our Discord server.
- Post all technical questions on Stack Overflow with the #ballerina tag.
Clients
dayforce: Client
Constructor
Gets invoked to initialize the connector
.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://ustest241-services.dayforcehcm.com/Api" - URL of the target service
post [string clientNamespace]/v1/GetEmployeeBulkAPI
function post [string clientNamespace]/v1/GetEmployeeBulkAPI(true isValidateOnly, EmployeeExportParams payload) returns json|error
Add new HR Bulk Export details into Job Queue tables.
Parameters
- isValidateOnly true - This parameter used to run a test case without fail
- payload EmployeeExportParams - The JSON-formatted content containing the data entities and elements to be processed in POST. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A HR Bulk Export is created with an empty response body.
get [string clientNamespace]/v1/GetEmployeeBulkAPI/Status/[int:Signed32 backgroundJobQueueItemId]
function get [string clientNamespace]/v1/GetEmployeeBulkAPI/Status/[int:Signed32 backgroundJobQueueItemId]() returns Payload_Object|error
Get the status of Job Queue tables.
Return Type
- Payload_Object|error - Job Status and Access API URL
get [string clientNamespace]/v1/GetEmployeeBulkAPI/Data/[string jobId]
function get [string clientNamespace]/v1/GetEmployeeBulkAPI/Data/[string jobId](Paging? pagination) returns PaginatedPayload_IEnumerable_Employee|error?
Get bulk employee of data as a string in json format
PaginatedPayload_IEnumerable_Employee? employeeDetails = check dayforce->/[NAMESPACE]/v1/GetEmployeeBulkAPI/Data/["12810"]; if employees is PaginatedPayload_IEnumerable_Employee { employeeDetails = check dayforce->/[NAMESPACE]/v1/GetEmployeeBulkAPI/Data/["12810"](employeeDetails.Paging); }
Parameters
- pagination Paging? (default ()) - The pagination information to be used for the request if there is any. You can obtain the Paging record from the initial request's response.
Return Type
- PaginatedPayload_IEnumerable_Employee|error? - Returns a page of employee data,
nil
if the given paginated data does not have a url(marking the end of the pages) or else an error if the request fails.
get [string clientNamespace]/V1/Analytics/Reports
function get [string clientNamespace]/V1/Analytics/Reports() returns Payload_IEnumerable_AnalyticsReportDefinitions|Payload_IEnumerable_Object|error
Get Reports
Return Type
- Payload_IEnumerable_AnalyticsReportDefinitions|Payload_IEnumerable_Object|error - A collection of Reports
get [string clientNamespace]/V1/Analytics/Datasets/[string datasetId]
function get [string clientNamespace]/V1/Analytics/Datasets/[string datasetId](string? page, string? datasetPageSize) returns Payload_AnalyticsReportDataset|error
Get Dataset
Return Type
- Payload_AnalyticsReportDataset|error - Contents of a dataset
post [string clientNamespace]/V1/Analytics/Datasets/[string datasetId]
function post [string clientNamespace]/V1/Analytics/Datasets/[string datasetId](true isValidateOnly, AnalyticsReportMetadata payload) returns Payload_AnalyticsDatasetMetadataResponse|json|error
Refresh Dataset
Parameters
- isValidateOnly true - This parameter is applied here for testing purposes. Please remember to remove it when utilizing this endpoint to actually refresh the dataset
- payload AnalyticsReportMetadata -
Return Type
- Payload_AnalyticsDatasetMetadataResponse|json|error - Status of a dataset refresh and its metadata
post [string clientNamespace]/V1/Analytics/Reports/[int:Signed32 reportId]
function post [string clientNamespace]/V1/Analytics/Reports/[int:Signed32 reportId](true isValidateOnly, AnalyticsReportMetadata payload) returns Payload_Object|Payload_AnalyticsDatasetMetadata|error
Create Dataset
Parameters
- isValidateOnly true - This parameter is applied here for testing purposes. Please remember to remove it when utilizing this endpoint to actually post the report
- payload AnalyticsReportMetadata -
Return Type
- Payload_Object|Payload_AnalyticsDatasetMetadata|error - Metadata of a dataset
get [string clientNamespace]/V1/Analytics/Datasets/[string datasetId]/Metadata
function get [string clientNamespace]/V1/Analytics/Datasets/[string datasetId]/Metadata() returns Payload_Object|Payload_AnalyticsDatasetMetadata|error
Get Dataset Metadata
Return Type
- Payload_Object|Payload_AnalyticsDatasetMetadata|error - Metadata of a dataset
get [string clientNamespace]/V1/Analytics/Reports/[int:Signed32 reportId]/Metadata
function get [string clientNamespace]/V1/Analytics/Reports/[int:Signed32 reportId]/Metadata() returns Payload_IEnumerable_AnalyticsReportMetadata|Payload_AnalyticsReportMetadata|error
Get Report Metadata
Return Type
- Payload_IEnumerable_AnalyticsReportMetadata|Payload_AnalyticsReportMetadata|error - Metadata of the report
get [string clientNamespace]/V1/Employees/[string xRefCode]/SSOAccounts
function get [string clientNamespace]/V1/Employees/[string xRefCode]/SSOAccounts(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeSSOAccount|error
GET AppUserSSO Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeSSOAccount|error - The AppUserSSO with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/SSOAccounts
function post [string clientNamespace]/V1/Employees/[string xRefCode]/SSOAccounts(true isValidateOnly, EmployeeSSOAccount payload) returns json|error
POST a AppUserSSO
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeSSOAccount - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/SSOAccounts
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/SSOAccounts(true isValidateOnly, EmployeeSSOAccount payload) returns json|error
PATCH a AppUserSSO
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeSSOAccount - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The AppUserSSO is updated, no response body.
delete [string clientNamespace]/V1/Employees/[string employeeXRefCode]/SSOAccounts/[string loginName]
function delete [string clientNamespace]/V1/Employees/[string employeeXRefCode]/SSOAccounts/[string loginName](true isValidateOnly) returns Payload_SubordinateEntityReferences|error
Delete an SSOAccount entry that has matching {loginName}
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, this operation is validated without applying updates to the database.
Return Type
- Payload_SubordinateEntityReferences|error - An SSO Account is deleted for this Employee
get [string clientNamespace]/V1/Employees/[string xRefCode]/Availability
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Availability(string filterAvailabilityStartDate, string filterAvailabilityEndDate) returns Payload_IEnumerable_EmployeeAvailability|error
GET List of Employee Availability
Parameters
- filterAvailabilityStartDate string - Inclusive period start date to determine which employee availability data to retrieve . Example: 2017-01-01T00:00:00
- filterAvailabilityEndDate string - Inclusive period end date to determine which employee availability data to retrieve . Example: 2017-01-01T00:00:00
Return Type
- Payload_IEnumerable_EmployeeAvailability|error - A collection of employee availability meeting the search criteria.
post [string clientNamespace]/V1/[string employeeXRefCode]/EmployeeAvailability
function post [string clientNamespace]/V1/[string employeeXRefCode]/EmployeeAvailability(true isValidateOnly, EmployeeAvailabilityPostAPIRequestDTO payload) returns Payload_IEnumerable_ProcessResult|error
Post Employee Availablity
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validates the request without applying updates to the database.
- payload EmployeeAvailabilityPostAPIRequestDTO -
Return Type
- Payload_IEnumerable_ProcessResult|error - OK response
get [string clientNamespace]/V1/BackgroundJobLogs
function get [string clientNamespace]/V1/BackgroundJobLogs(Signed32? pageSize, string? codeName, string? status, boolean? hasItemLevelErrors, string? queueTimeUtcStart, string? queueTimeUtcEnd, boolean? wasScheduled, string? submittedBy, string? filterUpdateTimeUtcStart, string? filterUpdateTimeUtcEnd, boolean? includeSuppressedTaskTypes) returns PaginatedPayload_IEnumerable_BackgroundJobLog|error
GET List of Background Job Logs
Parameters
- pageSize Signed32? (default ()) - Use to specify the number of records returned per page in the paginated response. This value will be constrained to be between 1 and 10,000 (default is 1,000).
- codeName string? (default ()) - Use to search for background job logs using a comma separated list of code names. If omitted, all job code names are included.
- status string? (default ()) - Use to search for background job logs by job status. If omitted, all job statuses are included. Accepted values: Queued, In Progress, Paused, Completed, Cancelled, Error.
- hasItemLevelErrors boolean? (default ()) - Use to search for background job logs with item-level errors. If omitted, both logs with and without item level errors are included. Accepted values: True, False
- queueTimeUtcStart string? (default ()) - The beginning date/time used when searching for logs that were queued in a specified time frame. When a value is provided for this parameter, a queueTimeUtcEnd value must also be provided. Example: 2017-01-01T13:24:56
- queueTimeUtcEnd string? (default ()) - The ending date/time used when searching for logs that were queued in a specified time frame. When a value is provided for this parameter, a queueTimeUtcStart value must also be provided. Example: 2017-01-01T13:24:56
- wasScheduled boolean? (default ()) - Use to search for background job logs that were scheduled or ran on-demand. If omitted, logs for both scheduled and on-demand background jobs are included. Accepted values: True, False
- submittedBy string? (default ()) - Use to search for background job logs that were manually submitted by a specified user. If omitted, all job submitters including the System are included.
- filterUpdateTimeUtcStart string? (default ()) - The beginning date/time used when searching for background job logs with updates in a specified time frame. When a value is provided for this parameter, a filterUpdateTimeUtcEnd value must also be provided. Example: 2017-01-01T13:24:56
- filterUpdateTimeUtcEnd string? (default ()) - The ending date/time used when searching for background job logs with updates in a specified time frame. When a value is provided for this parameter, a filterUpdateTimeUtcStart value must also be provided. Example: 2017-01-01T13:24:56
- includeSuppressedTaskTypes boolean? (default ()) - Used to specify whether commonly suppressed background jobs should be included in the filtered results. Accepted values: True, False. Defaults to False.
Return Type
- PaginatedPayload_IEnumerable_BackgroundJobLog|error - A collection of background job logs meeting the search criteria. Parameters, JobInformation, ErrorInformation, DebugInformation and FileList fields are not retrieved with this call.
get [string clientNamespace]/V1/BackgroundJobLogs/[string backgroundJobLogId]
function get [string clientNamespace]/V1/BackgroundJobLogs/[string backgroundJobLogId]() returns Payload_BackgroundJobLog|error
GET Details of a Background Job Log based on backgroundLogId
Return Type
- Payload_BackgroundJobLog|error - A background job log that meets the search criteria.
patch [string clientNamespace]/V1/BackgroundScreening/Status
function patch [string clientNamespace]/V1/BackgroundScreening/Status(true isValidateOnly, BackgroundScreeningStatus payload) returns BackgroundScreeningStatus|error
PATCH (Update) a Background Screening Status for a specific candidate.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload BackgroundScreeningStatus - The Json payload containing an event and the new status.
Return Type
- BackgroundScreeningStatus|error - The Background Screening request is updated, no response body.
patch [string clientNamespace]/V1/BackgroundScreening/AdjudicationStatus
function patch [string clientNamespace]/V1/BackgroundScreening/AdjudicationStatus(true isValidateOnly, BackgroundScreeningAdjudicationStatus payload) returns BackgroundScreeningAdjudicationStatus|error
PATCH (Update) a Background Screening Adjudication Status for a specific candidate.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload BackgroundScreeningAdjudicationStatus - The Json payload containing an event and the new adjudication status.
Return Type
- BackgroundScreeningAdjudicationStatus|error - The Background Screening request is updated, no response body.
post [string clientNamespace]/V1/BackgroundScreening/Package
function post [string clientNamespace]/V1/BackgroundScreening/Package(true isValidateOnly, BackgroundScreeningPackage payload) returns BackgroundScreeningPackage[]|error
POST Background Screening Packages.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload BackgroundScreeningPackage -
Return Type
- BackgroundScreeningPackage[]|error - The Background Screening Packages are added, no response body.
post [string clientNamespace]/V1/BackgroundScreening/BillingCode
function post [string clientNamespace]/V1/BackgroundScreening/BillingCode(true isValidateOnly, BackgroundScreeningBillingCode payload) returns BackgroundScreeningBillingCode[]|error
POST Background Screening Billing Codes.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload BackgroundScreeningBillingCode -
Return Type
- BackgroundScreeningBillingCode[]|error - The Background Screening Billing Codes are added, no response body.
post [string clientNamespace]/V1/BackgroundScreening/ProviderStatuses
function post [string clientNamespace]/V1/BackgroundScreening/ProviderStatuses(true isValidateOnly, BackgroundScreeningProviderStatuses payload) returns BackgroundScreeningProviderStatuses|error
POST Background Screening Provider Statuses.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload BackgroundScreeningProviderStatuses -
Return Type
- BackgroundScreeningProviderStatuses|error - Custom Provider Statuses are added, no response body.
patch [string clientNamespace]/V1/BackgroundScreening
function patch [string clientNamespace]/V1/BackgroundScreening(true isValidateOnly, BackgroundScreeningStatusUrlReport payload) returns BackgroundScreeningStatusUrlReport[]|error
PATCH Background Screening Status, URL and Report containing PII
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload BackgroundScreeningStatusUrlReport - The Json payload containing Status, Url and Report containing PII.
Return Type
- BackgroundScreeningStatusUrlReport[]|error - The Background Screening Status, Url and Report containing PII are added, no response body.
patch [string clientNamespace]/V1/BackgroundScreening/PersonalIdentifiableInformation
function patch [string clientNamespace]/V1/BackgroundScreening/PersonalIdentifiableInformation(true isValidateOnly, BackgroundScreeningReport payload) returns BackgroundScreeningReport[]|error
PATCH Background Screening Report containing Personal Identifiable Informations
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload BackgroundScreeningReport - The Json payload with a Report containing Personal Identifiable Informations.
Return Type
- BackgroundScreeningReport[]|error - The Background Screening Report containing Personal Identifiable Informations are added, no response body.
get [string clientNamespace]/V1/BenefitsCarrierFeedOngoingExports
function get [string clientNamespace]/V1/BenefitsCarrierFeedOngoingExports(string carrierXRefCode, string planStartDate, string planEndDate, string? payrollEarningAccumulationXRefCodes, string? blackoutPeriodStartDate, Signed32? lookBackDays, Signed32? lookForwardDays) returns Payload_CarrierFeeds|error
GET Benefits ongoing carrier feed export in JSON format
Parameters
- carrierXRefCode string - A reference code that maps to a given carrier.
- planStartDate string - The plan start date.
- planEndDate string - The plan end date.
- payrollEarningAccumulationXRefCodes string? (default ()) - A comma delimited list of reference codes, mapping to a list of individual payroll earning accumulations.
- blackoutPeriodStartDate string? (default ()) - If applicable, the blackout period start date.
- lookBackDays Signed32? (default ()) - The number of days the export will look back from execution date to find employee elections.
- lookForwardDays Signed32? (default ()) - The number of days the export will look forward from execution date to find employee elections.
Return Type
- Payload_CarrierFeeds|error - The CarrierFeeds model in json format.
get [string clientNamespace]/V1/BenefitsCarrierFeedOpenEnrollmentExports
function get [string clientNamespace]/V1/BenefitsCarrierFeedOpenEnrollmentExports(string carrierXRefCode, string planStartDate, string? planEndDate, string? payrollEarningAccumulationXRefCodes) returns Payload_CarrierFeeds|error
GET Benefits open enrollment carrier feed export in JSON format
Parameters
- carrierXRefCode string - A reference code that maps to a given carrier.
- planStartDate string - The plan start date.
- planEndDate string? (default ()) - The plan end date.
- payrollEarningAccumulationXRefCodes string? (default ()) - A comma delimited list of reference codes, mapping to a list of individual payroll earning accumulations.
Return Type
- Payload_CarrierFeeds|error - The CarrierFeeds model in json format.
get [string clientNamespace]/V1/BenefitsCarrierFeedMultiCarrierOngoingExports
function get [string clientNamespace]/V1/BenefitsCarrierFeedMultiCarrierOngoingExports(string carrierXRefCodes, string planStartDate, string planEndDate, string? payrollEarningAccumulationXRefCodes, string? blackoutPeriodStartDate, Signed32? lookBackDays, Signed32? lookForwardDays) returns Payload_CarrierFeeds|error
GET Benefits multi carrier ongoing carrier feed export in JSON format
Parameters
- carrierXRefCodes string - A comma delimited list of reference codes, mapping to a list of individual carriers.
- planStartDate string - The plan start date.
- planEndDate string - The plan end date.
- payrollEarningAccumulationXRefCodes string? (default ()) - A comma delimited list of reference codes, mapping to a list of individual payroll earning accumulations.
- blackoutPeriodStartDate string? (default ()) - If applicable, the blackout period start date.
- lookBackDays Signed32? (default ()) - The number of days the export will look back from execution date to find employee elections.
- lookForwardDays Signed32? (default ()) - The number of days the export will look forward from execution date to find employee elections.
Return Type
- Payload_CarrierFeeds|error - The CarrierFeeds model in json format.
get [string clientNamespace]/V1/BenefitsCarrierFeedMultiCarrierOpenEnrollmentExports
function get [string clientNamespace]/V1/BenefitsCarrierFeedMultiCarrierOpenEnrollmentExports(string carrierXRefCodes, string planStartDate, string? planEndDate, string? payrollEarningAccumulationXRefCodes) returns Payload_CarrierFeeds|error
GET Benefits multi carrier open enrollment carrier feed export in JSON format
Parameters
- carrierXRefCodes string - A comma delimited list of reference codes, mapping to a list of individual carriers.
- planStartDate string - The plan start date.
- planEndDate string? (default ()) - The plan end date.
- payrollEarningAccumulationXRefCodes string? (default ()) - A comma delimited list of reference codes, mapping to a list of individual payroll earning accumulations.
Return Type
- Payload_CarrierFeeds|error - The CarrierFeeds model in json format.
post [string clientNamespace]/V1/CandidateSourcing
function post [string clientNamespace]/V1/CandidateSourcing(true isValidateOnly, JobPostingApplicantModel payload) returns Response|error
POST a Candidate Application and/or a Candidate Profile
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, this will validate the request without applying updates to the database.
- payload JobPostingApplicantModel - The Json payload containing candidate and application details.
get [string clientNamespace]/V1/JobPostingQuestionnaires
function get [string clientNamespace]/V1/JobPostingQuestionnaires(Signed32 jobPostingId, Signed32 cultureId) returns Payload_IEnumerable_QuestionModel|error
GET Job Posting Questionnaires
Parameters
- jobPostingId Signed32 - The job posting id
- cultureId Signed32 - The culture id to determine the language screening questions
Return Type
- Payload_IEnumerable_QuestionModel|error - A collection of questionnaires for a particular job posting. Each questionnaire section is separated by a page break object.
get [string clientNamespace]/V1/CandidateApplicationStatuses
function get [string clientNamespace]/V1/CandidateApplicationStatuses() returns Payload_CandidateApplicationStatusUpdatesPaginationModel|error
GET Candidate Application Statuses
Return Type
- Payload_CandidateApplicationStatusUpdatesPaginationModel|error - A collection of candidate application statuses for all job applications sent by the provider calling this endpoint. Once the latest unsent application statuses are provided via this endpoint, no further data will be provided on subsequent calls unless further application status updates have been made. This endpoint will return application statuses in tranches of up to 1000 statuses per call and will continue to do so for subsequent calls until all unsent statuses have been provided.
get [string clientNamespace]/V1/JobPostings
function get [string clientNamespace]/V1/JobPostings(string? companyName, string? parentCompanyName, string? internalJobBoardCode, boolean? includeActivePostingOnly, string? lastUpdateTimeFrom, string? lastUpdateTimeTo, string? datePostedFrom, string? datePostedTo, boolean? htmlDescription) returns Payload_IEnumerable_JobFeed|error
GET Job Postings
Parameters
- companyName string? (default ()) - Company name. Example: XYZ Co.
- parentCompanyName string? (default ()) - Parent Company name. Example: Ceridian
- internalJobBoardCode string? (default ()) - XRefCode of Job Board. Example: CANDIDATEPORTAL
- includeActivePostingOnly boolean? (default ()) - If true, then exclude inactive postings from the result. If False, then the 'Last Update Time From' and 'Last Update Time To' parameters are required and the range specified between the 'Last Update Time From' and 'Last Update Time To' parameters must not be larger than 1 month. Example: True
- lastUpdateTimeFrom string? (default ()) - A starting timestamp of last updated job posting. Example: 2017-01-01T13:24:56
- lastUpdateTimeTo string? (default ()) - An ending timestamp of last updated job posting. Example: 2017-02-01T13:24:56
- datePostedFrom string? (default ()) - A starting timestamp of job posting date. Example: 2017-01-01T13:24:56
- datePostedTo string? (default ()) - An ending timestamp of job posting date. Example: 2017-02-01T13:24:56
- htmlDescription boolean? (default ()) - A flag to feed the jobs over with html formatting or plain text description
Return Type
- Payload_IEnumerable_JobFeed|error - A collection of all active external job postings (i.e. public facing job postings) based on specified filter parameters. Each job posting included in the response also includes URLs for Candidate Sourcing and Apply endpoints. Users with access to the Candidate Sourcing and Apply feature should use this endpoint for obtaining job postings rather than GET JobFeeds.
get [string clientNamespace]/V1/Certifications
function get [string clientNamespace]/V1/Certifications(Signed32? pageSize, string? xRefCode) returns PaginatedPayload_IEnumerable_LMSCertification|error
GET the list of all certifications
Parameters
- pageSize Signed32? (default ()) - The page size for the pagination (Default is 1000)
- xRefCode string? (default ()) - The xrefcode filter for the certifications
Return Type
- PaginatedPayload_IEnumerable_LMSCertification|error - A collection of certifications
post [string clientNamespace]/V1/Certifications
function post [string clientNamespace]/V1/Certifications(true isValidateOnly, LMSCertification payload) returns json|error
POST one certification
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validate the request without applying the changes to the database.
- payload LMSCertification - The certification payload that will be used for certification creation
Return Type
- json|error - A certification is created, no response body
patch [string clientNamespace]/V1/Certifications
function patch [string clientNamespace]/V1/Certifications(string xRefCode, true isValidateOnly, LMSCertification payload) returns json|error
PATCH one certification
Parameters
- xRefCode string -
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validate the request without applying the changes to the database.
- payload LMSCertification - The certification payload that will be used for certification modification
Return Type
- json|error - A certification is updated, no response body
get [string clientNamespace]/V1/ClientMetadata
function get [string clientNamespace]/V1/ClientMetadata() returns ClientMetadata|error
GET Client Metadata
Return Type
- ClientMetadata|error - Returns client metadata.
get [string clientNamespace]/V1/ClientPayrollCountry
function get [string clientNamespace]/V1/ClientPayrollCountry(string? countryCodes, boolean? hcmPayroll, boolean? connectedPay, boolean? payGroup) returns Payload_ClientPayrollCountry|error
GET client payroll countries.
Parameters
- countryCodes string? (default ()) - Comma separated list of country codes to filter the results.
- hcmPayroll boolean? (default ()) - Flag to filter countries for HCM Payroll.
- connectedPay boolean? (default ()) - Flag to filter countries for Connected Pay.
- payGroup boolean? (default ()) - Flag to filter countries having pay group(s).
Return Type
- Payload_ClientPayrollCountry|error - Client Payroll Countries.
post [string clientNamespace]/V1/ClientPayrollCountry
function post [string clientNamespace]/V1/ClientPayrollCountry(true isValidateOnly, ClientPayrollCountryUpdate[] payload) returns json|error
POST Client Payroll Country.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload ClientPayrollCountryUpdate[] - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The ClientPayrollCountry has been added successfully, no response body.
patch [string clientNamespace]/V1/ClientPayrollCountry
function patch [string clientNamespace]/V1/ClientPayrollCountry(true isValidateOnly, ClientPayrollCountryUpdate[] payload) returns json|error
PATCH Client Payroll Country.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload ClientPayrollCountryUpdate[] - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - ClientPayrollCountry has been updated successfully, no response body.
get [string clientNamespace]/V1/ContactInformationTypes
function get [string clientNamespace]/V1/ContactInformationTypes() returns Payload_IEnumerable_ContactInformationType|error
GET a List of ContactInformationTypes
Return Type
- Payload_IEnumerable_ContactInformationType|error - A collection of ContactInformationType XRefCodes.
get [string clientNamespace]/V1/ContactInformationTypes/[string xrefCode]
function get [string clientNamespace]/V1/ContactInformationTypes/[string xrefCode]() returns Payload_ContactInformationType|error
GET a ContactInformationType with the requested XRefCode.
Return Type
- Payload_ContactInformationType|error - The ContactInformationType with the requested XRefCode.
get [string clientNamespace]/V1/Courses
function get [string clientNamespace]/V1/Courses(Signed32? pageSize, string? courseType, string? courseProvider, string? xRefCode) returns PaginatedPayload_IEnumerable_Course|error
GET the list of all courses
Parameters
- pageSize Signed32? (default ()) - The page size for the pagination (Default is 1000)
- courseType string? (default ()) - The course type filter for the courses
- courseProvider string? (default ()) - The course provider filter for the courses
- xRefCode string? (default ()) - The xrefcode filter for the courses
Return Type
- PaginatedPayload_IEnumerable_Course|error - A collection of courses
post [string clientNamespace]/V1/Courses
function post [string clientNamespace]/V1/Courses(true isValidateOnly, Course payload) returns json|error
POST one course
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validate the request without applying the changes to the database.
- payload Course - The course payload that will be used for course creation
Return Type
- json|error - A course is created, no response body
patch [string clientNamespace]/V1/Courses
function patch [string clientNamespace]/V1/Courses(string xRefCode, true isValidateOnly, Course payload) returns json|error
PATCH one course
Parameters
- xRefCode string - The course payload that will be used for course creation
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validate the request without applying the changes to the database.
- payload Course - The course payload that will be used for course creation
Return Type
- json|error - A course is updated, no response body
get [string clientNamespace]/V1/Payroll/DataEntry/[string payGroupXRefCode]/[string entryType]
function get [string clientNamespace]/V1/Payroll/DataEntry/[string payGroupXRefCode]/[string entryType](string? periodStartDate, string? periodEndDate, string? payDate, string? ppn, string? orgUnitXRefCode, string? codeType, string? codeXRefCode, string? employeeXRefCode, string? projectXRefCodes, string? sinceLastModifiedTimestamp, string? 'source, Signed32? pageSize) returns PaginatedPayload_IEnumerable_DataEntry|error
GET List of Data Entries.
Parameters
- periodStartDate string? (default ()) - The pay run period start date.
- periodEndDate string? (default ()) - The pay run period end date.
- payDate string? (default ()) - The pay run pay date.
- ppn string? (default ()) - The pay run's pay period number (format: ##-##).
- orgUnitXRefCode string? (default ()) - The user org unit reference code to load data entries from (in org unit hierarchy).
- codeType string? (default ()) - The type of the data entry codes.
- codeXRefCode string? (default ()) - The data entry code reference code.
- employeeXRefCode string? (default ()) - The employee reference code.
- projectXRefCodes string? (default ()) - The comma separated project reference codes.
- sinceLastModifiedTimestamp string? (default ()) - Last modified timestamp to load entries since.
- 'source string? (default ()) -
- pageSize Signed32? (default ()) - Number of records to be loaded.
Return Type
- PaginatedPayload_IEnumerable_DataEntry|error - Payroll data entries.
get [string clientNamespace]/V1/DataPrivacy/PersonManagementHistory
function get [string clientNamespace]/V1/DataPrivacy/PersonManagementHistory(Signed32? pageSize, string? policy, string? personType, string? contextDateRangeFrom, string? contextDateRangeTo) returns PaginatedPayload_PersonManagementHistory|error
Get a list of Person Management History
Parameters
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
- policy string? (default ()) - Person Management Policy Types such as HR, WFM, etc
- personType string? (default ()) - Can be either Employee or Candidate
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which Person Management History data to search when records have specific start and end dates.The service defaults to null if the requester does not specify a value.Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which Person Management History data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- PaginatedPayload_PersonManagementHistory|error - A collection of Person Management History.
get [string clientNamespace]/V1/DataPrivacy/PersonManagementExemptions
function get [string clientNamespace]/V1/DataPrivacy/PersonManagementExemptions(Signed32? pageSize, string? number, string? personType) returns PaginatedPayload_PersonManagementExemption|error
Get a list of Person Management Exemption
Parameters
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
- number string? (default ()) - Represent the Employee number
- personType string? (default ()) - Can be either Employee or Candidate
Return Type
- PaginatedPayload_PersonManagementExemption|error - A collection of Person Management Exemption.
get [string clientNamespace]/V1/DataPrivacy/PersonManagementEraseHistory
function get [string clientNamespace]/V1/DataPrivacy/PersonManagementEraseHistory(Signed32? pageSize, string? personType, string? contextDateRangeFrom, string? contextDateRangeTo, Signed32? requestId) returns PaginatedPayload_PersonManagementEraseHistory|error
Get a list of Person Management Erase History
Parameters
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
- personType string? (default ()) - Can be either Employee or Candidate
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which user data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which user data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- requestId Signed32? (default ()) - The right to be forgotten request identifier. The value provided must be exact match; otherwise, a bad request (400) error will be returned.
Return Type
- PaginatedPayload_PersonManagementEraseHistory|error - A collection of Person Management Erase History.
get [string clientNamespace]/V1/DataPrivacy/PolicyAssociations
function get [string clientNamespace]/V1/DataPrivacy/PolicyAssociations(string? countryCodes, Signed32? pageSize, string? personType, string? xRefCode) returns PaginatedPayload_IEnumerable_PolicyAssociation|error
Get a List of Policy Associations
Parameters
- countryCodes string? (default ()) - The country codes to search policies for, if ommited all countries will be returned
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
- personType string? (default ()) - Can be either Employee or Candidate
- xRefCode string? (default ()) - The unique identifier (external reference code) of the employee whose data will be retrieved. The value provided must be the exact match for an employee; otherwise, a bad request (400) error will be returned.
Return Type
- PaginatedPayload_IEnumerable_PolicyAssociation|error - A collection of PolicyAssociation data meeting the search criteria.
get [string clientNamespace]/V1/DeductionDefinition
function get [string clientNamespace]/V1/DeductionDefinition(string? countryCodes, string? deductionXRefCodes, string? taxComplianceXRefCodes, string? taxTypeCodes, boolean? systemDeduction) returns Payload_IEnumerable_DeductionDefinition|error
Get List of Deduction Definitions.
Parameters
- countryCodes string? (default ()) - >List of country codes with comma separator to filter the result base on country.
- deductionXRefCodes string? (default ()) - List of Deduction Reference Codes with comma separator to filter the result.
- taxComplianceXRefCodes string? (default ()) - List of Tax Compliance Reference Codes (DeductionCodeXRefCodes) with comma separator to filter the result.
- taxTypeCodes string? (default ()) - List of Tax Type Codes with comma separator to filter the result.
- systemDeduction boolean? (default ()) - Set to True to get only System Defined Deduction, or set to False to get only User Defined Deductions.
Return Type
- Payload_IEnumerable_DeductionDefinition|error - List of Deduction Definitions and associated payees.
get [string clientNamespace]/V1/Departments
function get [string clientNamespace]/V1/Departments() returns Payload_IEnumerable_Department|error
Return Type
- Payload_IEnumerable_Department|error - A collection of Department XRefCodes.
post [string clientNamespace]/V1/Departments
function post [string clientNamespace]/V1/Departments(true isValidateOnly, Department payload) returns json|error
Return Type
- json|error - A Department is created, no response body.
get [string clientNamespace]/V1/Departments/[string xRefCode]
function get [string clientNamespace]/V1/Departments/[string xRefCode](true isValidateOnly) returns Payload_Department|error
Parameters
- isValidateOnly true -
Return Type
- Payload_Department|error - A Department XRefCode meets the search criteria.
patch [string clientNamespace]/V1/Departments/[string xRefCode]
function patch [string clientNamespace]/V1/Departments/[string xRefCode](true isValidateOnly, Department payload) returns json|error
Return Type
- json|error - A Department is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/DocumentManagementSecurityGroups
function get [string clientNamespace]/V1/Employees/[string xRefCode]/DocumentManagementSecurityGroups() returns Payload_IEnumerable_EmployeeDocumentManagementSecurityGroup|error
GET DocMgmtSecurityGroupUserMap Details
Return Type
- Payload_IEnumerable_EmployeeDocumentManagementSecurityGroup|error - The DocMgmtSecurityGroupUserMap with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/DocumentManagementSecurityGroups
function post [string clientNamespace]/V1/Employees/[string xRefCode]/DocumentManagementSecurityGroups(true isValidateOnly, EmployeeDocumentManagementSecurityGroup payload) returns json|error
POST a DocMgmtSecurityGroupUserMap
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeDocumentManagementSecurityGroup - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The DocMgmtSecurityGroupUserMap is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/DocumentManagementSecurityGroups
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/DocumentManagementSecurityGroups(true isValidateOnly, EmployeeDocumentManagementSecurityGroup payload) returns json|error
PATCH a DocMgmtSecurityGroupUserMap
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeDocumentManagementSecurityGroup - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The DocMgmtSecurityGroupUserMap is updated, no response body.
get [string clientNamespace]/V1/Documents
function get [string clientNamespace]/V1/Documents(string? employeeXRefCode, string? lastModifiedTimestampStart, string? lastModifiedTimestampEnd, string? entity, string? entityTypeXRefCode, string? documentTypeXRefCode) returns Payload_IEnumerable_Document|error
GET a List of Documents
Parameters
- employeeXRefCode string? (default ()) - Filter for documents attached to the uniquely identified employee specified. Partial search is not supported, so provide the full value. Otherwise, a 400 error will be returned.
- lastModifiedTimestampStart string? (default ()) - Filter for documents starting from this date time value. The lastModifiedTimestampStart and lastModifiedTimestampEnd criteria require additional criteria to be specified.
- lastModifiedTimestampEnd string? (default ()) - Filter for documents until this date time value. The lastModifiedTimestampStart and lastModifiedTimestampEnd criteria require additional criteria to be specified.
- entity string? (default ()) - Filter documents attached to this specified entity. The entity and entityTypeXRefCode must both be provided to apply this filter criteria.
- entityTypeXRefCode string? (default ()) - Filter for documents associated with this specified Entity Type. The current Entity Types supported for filtering are: "BENEFIT_PLAN", "BENEFIT_PLAN_OPTION", "HR_POLICY", "PAY_RUN", "PAY_GROUP_CALENDAR", "EMPLOYEE_FILE". The entity and entityTypeXRefCode must both be provided to apply this filter criteria.
- documentTypeXRefCode string? (default ()) - Filter for documents associated with this specified Document Type
Return Type
- Payload_IEnumerable_Document|error - Returns documents associated with the given criteria.
get [string clientNamespace]/V1/documents/[string documentGuid]
function get [string clientNamespace]/V1/documents/[string documentGuid]() returns Payload_ArchiveDocument|error
GET Document Details
Return Type
- Payload_ArchiveDocument|error - Returns the document identified by the documentGuid
post [string clientNamespace]/V1/Documents/Import/Queue
function post [string clientNamespace]/V1/Documents/Import/Queue(true isValidateOnly, DocumentImportQueueRequest[] payload) returns Payload_DocumentImportQueueResponse|error
POST (Create) Queue Document Import task
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validates the request without applying updates to the database.
- payload DocumentImportQueueRequest[] - The JSON-formatted content containing the metadata entities and elements of document import to be processed in POST (queue task) operation.
Return Type
- Payload_DocumentImportQueueResponse|error - Document import queued.
post [string clientNamespace]/V1/Documents/Upload
function post [string clientNamespace]/V1/Documents/Upload(true isValidateOnly) returns DocMgmtUploadResponse|error
Upload one or more documents using a multipart request.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validates the request without applying updates to the database.
Return Type
- DocMgmtUploadResponse|error - Document(s) processed.
get [string clientNamespace]/V1/EarningDefinition
function get [string clientNamespace]/V1/EarningDefinition(string? countryCodes, string? earningXRefCodes, string? taxComplianceXRefCodes, string? earningTypeXRefCodes) returns Payload_IEnumerable_EarningDefinition|error
GET List of Earning Definitions.
Parameters
- countryCodes string? (default ()) - List of country codes with comma separator to filter the result base on country.
- earningXRefCodes string? (default ()) - List of Earning Reference Codes with comma separator to filter the result.
- taxComplianceXRefCodes string? (default ()) - List of Tax Compliance Reference Codes (EarningCodeXRefCodes) with comma separator to filter the result.
- earningTypeXRefCodes string? (default ()) - List of Earning Type Reference Codes with comma separator to filter the result.
Return Type
- Payload_IEnumerable_EarningDefinition|error - List of Earning Definitions and associated payees.
get [string clientNamespace]/V1/EarningStatementHeader
function get [string clientNamespace]/V1/EarningStatementHeader(Signed32? pageSize, string? payGroupXRefCode, string? payDate, string? payPeriodStartDate, string? payPeriodEndDate, string? ppn, string? employeeXRefCodes, string? employeeLastNames, string? employeePayDateBegin, string? employeePayDateEnd) returns PaginatedPayload_EarningStatementHeader|error
GET Earning Statement Headers
Parameters
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
- payGroupXRefCode string? (default ()) - The pay group's reference code.
- payDate string? (default ()) - The pay run's pay date (format: yyyy-mm-dd).
- payPeriodStartDate string? (default ()) - The pay run's period start date (format: yyyy-mm-dd).
- payPeriodEndDate string? (default ()) - The pay run's period end date (format: yyyy-mm-dd).
- ppn string? (default ()) - The PayPeriodNumber(format: ##-##).
- employeeXRefCodes string? (default ()) - Comma delimited list of XRef codes for employees
- employeeLastNames string? (default ()) - Comma delimited list of last names for employees
- employeePayDateBegin string? (default ()) - The employee's pay start date(format: yyyy-mm-dd).
- employeePayDateEnd string? (default ()) - The employee's pay end date(format: yyyy-mm-dd).
Return Type
- PaginatedPayload_EarningStatementHeader|error - An encrypted string which contains list of earning statement headers.
get [string clientNamespace]/V1/EarningStatement/[string earningStatementXRefCode]
function get [string clientNamespace]/V1/EarningStatement/[string earningStatementXRefCode](string employeeXRefCode) returns Payload_List_EarningStatementDocument|error
GET Earning Statement Pdf
Parameters
- employeeXRefCode string - Employee XRefCode.
Return Type
- Payload_List_EarningStatementDocument|error - Earning statement document.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmergencyContacts
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmergencyContacts(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeEmergencyContact|error
GET EmergencyContact Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeEmergencyContact|error - The emergency contacts of employee with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmergencyContacts
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmergencyContacts(true isValidateOnly, EmployeeEmergencyContact payload) returns json|error
POST an EmergencyContact
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEmergencyContact - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmergencyContact is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmergencyContacts
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmergencyContacts(true isValidateOnly, EmployeeEmergencyContact payload) returns json|error
PATCH an EmergencyContact
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEmergencyContact - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmergencyContact is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAccidentInsurance/AccidentInsurance
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAccidentInsurance/AccidentInsurance() returns Payload_IEnumerable_DEUEmployeeAccidentInsurance|error
GET DEUEmployeeAccidentInsurance Details
Return Type
- Payload_IEnumerable_DEUEmployeeAccidentInsurance|error - The DEUEmployeeAccidentInsurance with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAccidentInsurance/AccidentInsurance
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAccidentInsurance/AccidentInsurance(true isValidateOnly, DEUEmployeeAccidentInsurance payload) returns json|error
POST A dEUEmployeeAccidentInsurance
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload DEUEmployeeAccidentInsurance - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A DEUEmployeeAccidentInsurance is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAccidentInsurance/AccidentInsurance
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAccidentInsurance/AccidentInsurance(true isValidateOnly, DEUEmployeeAccidentInsurance payload) returns json|error
PATCH an DEUEmployeeAccidentInsurance
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload DEUEmployeeAccidentInsurance - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The dEUEmployeeAccidentInsurance is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAssignedSexAndGenderIdentity
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAssignedSexAndGenderIdentity(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeAssignedSexAndGenderIdentity|error
GET EmployeeAssignedSexAndGenderIdentity Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeAssignedSexAndGenderIdentity|error - The EmployeeAssignedSexAndGenderIdentity with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAssignedSexAndGenderIdentity
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAssignedSexAndGenderIdentity(true isValidateOnly, EmployeeAssignedSexAndGenderIdentity payload) returns json|error
POST a EmployeeAssignedSexAndGenderIdentity
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeAssignedSexAndGenderIdentity -
Return Type
- json|error - The EmployeeAssignedSexAndGenderIdentity is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAssignedSexAndGenderIdentity
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeAssignedSexAndGenderIdentity(true isValidateOnly, EmployeeAssignedSexAndGenderIdentity payload) returns json|error
PATCH a EmployeeAssignedSexAndGenderIdentity
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeAssignedSexAndGenderIdentity -
Return Type
- json|error - The EmployeeAssignedSexAndGenderIdentity is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/AUSFederalTaxes
function get [string clientNamespace]/V1/Employees/[string xRefCode]/AUSFederalTaxes(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeAUSFederalTax|error
GET EmployeeAUSFederalTax Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeAUSFederalTax|error - The EmployeeAUSFederalTax with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/AUSFederalTaxes
function post [string clientNamespace]/V1/Employees/[string xRefCode]/AUSFederalTaxes(true isValidateOnly, EmployeeAUSFederalTax payload) returns json|error
POST a EmployeeAUSFederalTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeAUSFederalTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeAUSFederalTax is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/AUSFederalTaxes
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/AUSFederalTaxes(true isValidateOnly, EmployeeAUSFederalTax payload) returns json|error
PATCH a EmployeeAUSFederalTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeAUSFederalTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeAUSFederalTax is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/AUSSuperannuation
function get [string clientNamespace]/V1/Employees/[string xRefCode]/AUSSuperannuation(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeAUSSuperannuation|error
GET Employee superannuation details for Australia
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeAUSSuperannuation|error - The employee superannuation details for Australia by requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/AUSSuperannuationRules
function get [string clientNamespace]/V1/Employees/[string xRefCode]/AUSSuperannuationRules(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeAUSSuperannuationRules|error
GET Employee superannuation rules for Australia
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeAUSSuperannuationRules|error - The employee superannuation rules for Australia by requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/AuthorizationAssignments
function get [string clientNamespace]/V1/Employees/[string xRefCode]/AuthorizationAssignments(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_AuthorizationAssignment|error
GET Employee's Authorization Assignment Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_AuthorizationAssignment|error - The EmployeeAuthorizationAssignments with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/AuthorizationAssignments
function post [string clientNamespace]/V1/Employees/[string xRefCode]/AuthorizationAssignments(true isValidateOnly, AuthorizationAssignment payload) returns json|error
POST an AuthorizationAssignment
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload AuthorizationAssignment -
Return Type
- json|error - An AuthorizationAssignment is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/AuthorizationAssignments
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/AuthorizationAssignments(true isValidateOnly, AuthorizationAssignment payload) returns json|error
PATCH an AuthorizationAssignment
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload AuthorizationAssignment -
Return Type
- json|error - The AuthorizationAssignment is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Badges
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Badges(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeBadge|error
GET EmployeeBadge Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeBadge|error - The EmployeeBadge with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Badges
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Badges(true isValidateOnly, EmployeeBadge payload) returns json|error
POST a EmployeeBadge
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeBadge - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeBadge is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Badges
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Badges(true isValidateOnly, EmployeeBadge payload) returns json|error
PATCH a EmployeeBadge
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeBadge - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeBadge is updated, no response body.
get [string clientNamespace]/V1/EmployeeBalancePeriods
function get [string clientNamespace]/V1/EmployeeBalancePeriods(string employeeXRefCode, string? asOfDate, string? startDate, string? endDate) returns Payload_IEnumerable_EmployeeBalancePeriod|error
Get a list of Employee Balance Periods
Parameters
- employeeXRefCode string - A case-sensitive field that identifies a unique employee.
- asOfDate string? (default ()) - The date as of which to calculate the current values for the employee balance periods returned. If omitted, current values will be calculated as of today. Example: 2020-01-01T00:00:00
- startDate string? (default ()) - Period start date to determine which employee balance periods to retrieve. If omitted, today's date will be used. Example: 2020-01-01T00:00:00
- endDate string? (default ()) - Period end date to determine which employee balance periods to retrieve. If omitted, the period start date will be used. If the period start date is also omitted, today's date will be used. Example: 2020-01-01T00:00:00
Return Type
- Payload_IEnumerable_EmployeeBalancePeriod|error - A collection of employee balance periods meeting the search criteria.
get [string clientNamespace]/V1/EmployeeBalanceTransactions
function get [string clientNamespace]/V1/EmployeeBalanceTransactions(string employeeXRefCode, string balanceXRefCode, string? startDate, string? endDate) returns Payload_IEnumerable_EmployeeBalanceTransactions|error
Get a list of Employee Balance Transactions grouped by their employee balance periods
Parameters
- employeeXRefCode string - A case-sensitive field that identifies a unique employee.
- balanceXRefCode string - A case-sensitive field that identifies a unique balance.
- startDate string? (default ()) - Period start date to determine which employee balance periods to retrieve. If omitted, today's date will be used. Example: 2020-01-01T00:00:00
- endDate string? (default ()) - Period end date to determine which employee balance periods to retrieve. If omitted, the period start date will be used. If the period start date is also omitted, today's date will be used. Example: 2020-01-01T00:00:00
Return Type
- Payload_IEnumerable_EmployeeBalanceTransactions|error - A collection of employee balance transactions grouped by their employee balance periods meeting the search criteria.
post [string clientNamespace]/V1/EmployeeBalanceTransactions
function post [string clientNamespace]/V1/EmployeeBalanceTransactions(true isValidateOnly, EmployeeBalanceTransactionForSubmit payload) returns EmployeeBalanceTransactionPostResponse|error
POST (Create) Employee Balance Transaction
Parameters
- isValidateOnly true -
- payload EmployeeBalanceTransactionForSubmit - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- EmployeeBalanceTransactionPostResponse|error - An employee balance transaction is created
patch [string clientNamespace]/V1/EmployeeBalanceTransactions
function patch [string clientNamespace]/V1/EmployeeBalanceTransactions(string employeeBalanceTransactionId, true isValidateOnly, EmployeeBalanceTransactionForPatch payload) returns EmployeeBalanceTransactionPatchResponse|error
PATCH (Modify) Employee Balance Transaction
Parameters
- employeeBalanceTransactionId string -
- isValidateOnly true -
- payload EmployeeBalanceTransactionForPatch - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- EmployeeBalanceTransactionPatchResponse|error - An employee balance transaction is modified
get [string clientNamespace]/V1/Employees/[string xRefCode]/CANFederalTaxes
function get [string clientNamespace]/V1/Employees/[string xRefCode]/CANFederalTaxes(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeCANFederalTax|error
GET EmployeeCANFederalTax Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeCANFederalTax|error - The EmployeeCANFederalTax with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/CANFederalTaxes
function post [string clientNamespace]/V1/Employees/[string xRefCode]/CANFederalTaxes(true isValidateOnly, EmployeeCANFederalTax payload) returns json|error
POST a EmployeeCANFederalTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeCANFederalTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeCANFederalTax is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/CANFederalTaxes
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/CANFederalTaxes(true isValidateOnly, EmployeeCANFederalTax payload) returns json|error
PATCH a EmployeeCANFederalTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeCANFederalTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeCANFederalTax is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/CANStateTaxes
function get [string clientNamespace]/V1/Employees/[string xRefCode]/CANStateTaxes(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeCANStateTax|error
GET EmployeeCANStateTax Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeCANStateTax|error - The EmployeeCANStateTax with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/CANStateTaxes
function post [string clientNamespace]/V1/Employees/[string xRefCode]/CANStateTaxes(true isValidateOnly, EmployeeCANStateTax payload) returns json|error
POST a EmployeeCANStateTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeCANStateTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A CANStateTaxes is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/CANStateTaxes
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/CANStateTaxes(true isValidateOnly, EmployeeCANStateTax payload) returns json|error
PATCH a EmployeeCANStateTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeCANStateTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The CANStateTaxes is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/CANTaxStatuses
function get [string clientNamespace]/V1/Employees/[string xRefCode]/CANTaxStatuses(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeCANTaxStatus|error
GET EmployeeCANTaxStatus Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeCANTaxStatus|error - The EmployeeCANTaxStatus with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/CANTaxStatuses
function post [string clientNamespace]/V1/Employees/[string xRefCode]/CANTaxStatuses(true isValidateOnly, EmployeeCANTaxStatus payload) returns json|error
POST EmployeeCANTaxStatus Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeCANTaxStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeCANTaxStatus Detail is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/CANTaxStatuses
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/CANTaxStatuses(true isValidateOnly, EmployeeCANTaxStatus payload) returns json|error
PATCH EmployeeCANTaxStatus Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeCANTaxStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeCANTaxStatus Detail is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeCertifications
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeCertifications(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_LMSEmployeeCertification|error
GET EmployeeCertification Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_LMSEmployeeCertification|error - The EmployeeCertification with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeCertifications
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeCertifications(true isValidateOnly, LMSEmployeeCertification payload) returns json|error
POST an Employee Certification
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload LMSEmployeeCertification - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee Certification is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeCertifications
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeCertifications(true isValidateOnly, LMSEmployeeCertification payload) returns json|error
PATCH (Update) an Employee Certification
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload LMSEmployeeCertification - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee Certification is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/ClockDeviceGroups
function get [string clientNamespace]/V1/Employees/[string xRefCode]/ClockDeviceGroups(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeClockDeviceGroup|error
GET EmployeeClockDeviceGroup Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeClockDeviceGroup|error - The EmployeeClockDeviceGroup with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/ClockDeviceGroups
function post [string clientNamespace]/V1/Employees/[string xRefCode]/ClockDeviceGroups(true isValidateOnly, EmployeeClockDeviceGroup payload) returns json|error
POST an EmployeeClockDeviceGroup
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeClockDeviceGroup - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeClockDeviceGroup is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/ClockDeviceGroups
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/ClockDeviceGroups(true isValidateOnly, EmployeeClockDeviceGroup payload) returns json|error
PATCH an EmployeeClockDeviceGroup
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeClockDeviceGroup - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeClockDeviceGroup is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/CompensationSummary
function get [string clientNamespace]/V1/Employees/[string xRefCode]/CompensationSummary(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeCompensation|error
GET EmployeeCompensation Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeCompensation|error - The EmployeeCompensation with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/ConfidentialIdentification
function get [string clientNamespace]/V1/Employees/[string xRefCode]/ConfidentialIdentification(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeConfidentialIdentification|error
GET EmployeeConfidentialIdentification Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeConfidentialIdentification|error - The EmployeeConfidentialIdentification with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/ConfidentialIdentification
function post [string clientNamespace]/V1/Employees/[string xRefCode]/ConfidentialIdentification(true isValidateOnly, EmployeeConfidentialIdentification payload) returns json|error
POST a EmployeeConfidentialIdentification
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeConfidentialIdentification - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeConfidentialIdentification is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/ConfidentialIdentification
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/ConfidentialIdentification(true isValidateOnly, EmployeeConfidentialIdentification payload) returns json|error
PATCH a EmployeeConfidentialIdentification
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeConfidentialIdentification - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeConfidentialIdentification is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Courses
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Courses() returns Payload_IEnumerable_EmployeeCourse|error
GET EmployeeCourse Details
Return Type
- Payload_IEnumerable_EmployeeCourse|error - The EmployeeCourse with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Courses
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Courses(true isValidateOnly, EmployeeCourse payload) returns json|error
POST an Employee Course Enrollment
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validate the request without applying the changes to the database.
- payload EmployeeCourse - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee Course Enrollment is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Courses
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Courses(true isValidateOnly, EmployeeCourse payload) returns json|error
PATCH (Update) an Employee Course Enrollment
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH operation validate the request without applying the changes to the database.
- payload EmployeeCourse - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee Course Enrollment is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/LaborDefaults
function get [string clientNamespace]/V1/Employees/[string xRefCode]/LaborDefaults(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeDefaultLabor|error
GET EmployeeDefaultLabor Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeDefaultLabor|error - The EmployeeDefaultLabor with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/LaborDefaults
function post [string clientNamespace]/V1/Employees/[string xRefCode]/LaborDefaults(true isValidateOnly, EmployeeDefaultLabor payload) returns json|error
POST a EmployeeDefaultLabor
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeDefaultLabor -
Return Type
- json|error - An EmployeeDefaultLabor is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/LaborDefaults
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/LaborDefaults(true isValidateOnly, EmployeeDefaultLabor payload) returns json|error
PATCH a EmployeeDefaultLabor
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeDefaultLabor -
Return Type
- json|error - The EmployeeDefaultLabor is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/DependentsBeneficiaries
function get [string clientNamespace]/V1/Employees/[string xRefCode]/DependentsBeneficiaries(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeDependentBeneficiary|error
GET EmployeeDependentsBeneficiaries Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeDependentBeneficiary|error - The EmployeeDependentsBeneficiaries with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/DEUEmployeeWageTax
function get [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/DEUEmployeeWageTax(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_DEUEmployeeWageTax|error
GET EmployeeWageTax Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_DEUEmployeeWageTax|error - The EmployeeWageTax with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/DEUEmployeeWageTax
function post [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/DEUEmployeeWageTax(true isValidateOnly, DEUEmployeeWageTax payload) returns json|error
POST an Employee Wage Tax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload DEUEmployeeWageTax -
Return Type
- json|error - An Employee Wage Tax is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/DEUEmployeeWageTax
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/DEUEmployeeWageTax(true isValidateOnly, DEUEmployeeWageTax payload) returns json|error
PATCH (Update) an Employee Wage Tax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload DEUEmployeeWageTax -
Return Type
- json|error - An Employee Wage Tax is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/SocialInsurance
function get [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/SocialInsurance(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_DEUTaxSocialInsurance|error
GET Employee Social Insurance Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_DEUTaxSocialInsurance|error - The Employee Social Insurance details with the requested Employee XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/SocialInsurance
function post [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/SocialInsurance(true isValidateOnly, DEUTaxSocialInsurance payload) returns json|error
POST Employee Social Insurance Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload DEUTaxSocialInsurance -
Return Type
- json|error - An Employee Social Insurance is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/SocialInsurance
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/DEUTax/SocialInsurance(true isValidateOnly, DEUTaxSocialInsurance payload) returns json|error
PATCH (Update) aEmployee Social Insurance Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload DEUTaxSocialInsurance -
Return Type
- json|error - An Employee Social Insurance is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/DirectDeposits
function get [string clientNamespace]/V1/Employees/[string xRefCode]/DirectDeposits(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeDirectDeposit|error
GET EmployeeDirectDeposit Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeDirectDeposit|error - The EmployeeDirectDeposit with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Disabilities
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Disabilities(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeDisability|error
GET EmployeeDisability Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeDisability|error - The EmployeeDisabilityController with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Disabilities
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Disabilities(true isValidateOnly, EmployeeDisability payload) returns json|error
POST an EmployeeDisability
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeDisability -
Return Type
- json|error - A EmployeeDisability is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Disabilities
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Disabilities(true isValidateOnly, EmployeeDisability payload) returns json|error
PATCH an EmployeeDisability
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeDisability -
Return Type
- json|error - The EmployeeDisability is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EIRates
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EIRates(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeCANEmploymentInsuranceRate|error
GET EmployeeEIRate Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeCANEmploymentInsuranceRate|error - The EmployeeEIRate with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentAgreements
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentAgreements(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeEmploymentAgreement|error
GET EmployeeEmploymentAgreement Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeEmploymentAgreement|error - The EmployeeEmploymentAgreement with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentAgreements
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentAgreements(true isValidateOnly, EmployeeEmploymentAgreement payload) returns json|error
POST a EmployeeEmploymentAgreement
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEmploymentAgreement - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentAgreements
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentAgreements(true isValidateOnly, EmployeeEmploymentAgreement payload) returns json|error
PATCH a EmployeeEmploymentAgreement
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEmploymentAgreement - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeEmploymentAgreement is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentStatuses
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentStatuses(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeEmploymentStatus|error
GET EmploymentStatus Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeEmploymentStatus|error - The Employment Statuses of employee with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentStatuses
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentStatuses(true isValidateOnly, EmployeeEmploymentStatus payload) returns json|error
POST an EmploymentStatus
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEmploymentStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmploymentStatus is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentStatuses
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentStatuses(true isValidateOnly, EmployeeEmploymentStatus payload) returns json|error
PATCH an EmploymentStatus
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEmploymentStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmploymentStatus is updated, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentStatuses/Replacement
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentStatuses/Replacement(true isValidateOnly, EmployeeEmploymentStatus payload, string? replaceFrom, string? replaceTo) returns json|error
Replace EmploymentStatuses
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, validate the request without applying updates to the database.
- payload EmployeeEmploymentStatus - The new JSON-formatted EmploymentStatus with which to replace the deleted ones.
- replaceFrom string? (default ()) - The date from which all EmploymentStatuses will be replaced.
- replaceTo string? (default ()) - The date up to which all EmploymentStatuses will be replaced.
Return Type
- json|error - The Employee's Employment Statuses are replaced within the given date range; no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentTypes
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentTypes(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeEmploymentType|error
GET EmployeeEmploymentType Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeEmploymentType|error - The EmployeeEmploymentType with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentTypes
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentTypes(true isValidateOnly, EmployeeEmploymentType payload) returns json|error
POST an EmployeeEmploymentType
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEmploymentType - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeEmploymentType is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentTypes
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmploymentTypes(true isValidateOnly, EmployeeEmploymentType payload) returns json|error
PATCH an EmployeeEmploymentType
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEmploymentType - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeEmploymentType is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Ethnicities
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Ethnicities(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeEthnicity|error
GET EmployeeEthnicity Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeEthnicity|error - The EmployeeEthnicity with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Ethnicities
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Ethnicities(true isValidateOnly, EmployeeEthnicity payload) returns json|error
POST EmployeeEthnicity Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEthnicity - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeEthnicity Detail is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Ethnicities
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Ethnicities(true isValidateOnly, EmployeeEthnicity payload) returns json|error
PATCH EmployeeEthnicity Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeEthnicity -
Return Type
- json|error - The EmployeeEthnicity Detail is updated, no response body.
get [string clientNamespace]/v1/EmployeeExportJobs/Status/[int:Signed32 backgroundJobQueueItemId]
function get [string clientNamespace]/v1/EmployeeExportJobs/Status/[int:Signed32 backgroundJobQueueItemId]() returns Payload_Object|error
Get the status of Job Queue tables.
Return Type
- Payload_Object|error - Job Status and Access API URL
get [string clientNamespace]/v1/EmployeeExportJobs/Data/[string jobId]
function get [string clientNamespace]/v1/EmployeeExportJobs/Data/[string jobId]() returns PaginatedPayload_IEnumerable_Employee|error
Get bulk employee of data as a string in json format
Return Type
- PaginatedPayload_IEnumerable_Employee|error - Returns a page of employee data.
post [string clientNamespace]/V1/EmployeeExportJobs
function post [string clientNamespace]/V1/EmployeeExportJobs(true isValidateOnly, EmployeeExportParams payload) returns json|error
Add new HR Bulk Export details into Job Queue tables.
Parameters
- isValidateOnly true - This parameter used to run a test case without fail
- payload EmployeeExportParams - The JSON-formatted content containing the data entities and elements to be processed in POST. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A HR Bulk Export is created with an empty response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeGlobalProperties
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeGlobalProperties(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeGlobalPropertyValue|error
GET EmployeeGlobalPropertyValue Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeGlobalPropertyValue|error - The EmployeeGlobalPropertyValue with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeGlobalProperties
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeGlobalProperties(true isValidateOnly, EmployeeGlobalPropertyValue payload) returns json|error
POST a EmployeeGlobalPropertyValue
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeGlobalPropertyValue - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee Global Property is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeGlobalProperties
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeGlobalProperties(true isValidateOnly, EmployeeGlobalPropertyValue payload) returns json|error
PATCH an EmployeeGlobalPropertyValue
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeGlobalPropertyValue - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeGlobalPropertyValue is updated, no response body.
get [string clientNamespace]/V1/Payroll/EmployeeGLSplits
function get [string clientNamespace]/V1/Payroll/EmployeeGLSplits(string? payGroupXRefCode, string? employeeXRefCodes, string? splitStatus, Signed32? pageSize) returns PaginatedPayload_IEnumerable_EmployeeGLSplits|error
GET the list of employee gl splits.
Parameters
- payGroupXRefCode string? (default ()) - The pay group reference code.
- employeeXRefCodes string? (default ()) - The comma separated employee XRef codes.
- splitStatus string? (default ()) - Filter to fetch all or current/future effective GL splits. Value can be All or Active. Default value is Active.
- pageSize Signed32? (default ()) - Number of records to be loaded.
Return Type
- PaginatedPayload_IEnumerable_EmployeeGLSplits|error - Employee GL Splits.
post [string clientNamespace]/V1/Payroll/EmployeeGLSplits
function post [string clientNamespace]/V1/Payroll/EmployeeGLSplits(true isValidateOnly, EmployeeGLSplitUpsert[] payload) returns json|error
POST a list of employee GLSplitSets.
Parameters
- isValidateOnly true - If TRUE, POST operations validate the request without queue the import. The default value is FALSE. Note, POST operation will only validate data structure.
- payload EmployeeGLSplitUpsert[] - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - OK response
patch [string clientNamespace]/V1/Payroll/EmployeeGLSplits
function patch [string clientNamespace]/V1/Payroll/EmployeeGLSplits(true isValidateOnly, EmployeeGLSplitUpsert[] payload) returns json|error
PATCH a list of employee GLSplitSets.
Parameters
- isValidateOnly true - If TRUE, PATCH operations validate the request without queue the import. The default value is FALSE. Note, PATCH operation will only validate data structure.
- payload EmployeeGLSplitUpsert[] - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - OK response
get [string clientNamespace]/V1/Employees/[string xRefCode]/HighlyCompensatedEmployees
function get [string clientNamespace]/V1/Employees/[string xRefCode]/HighlyCompensatedEmployees(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeHighlyCompensatedEmployeeIndicator|error
GET HighlyCompensatedEmployee Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeHighlyCompensatedEmployeeIndicator|error - The HighlyCompensatedEmployee with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/HighlyCompensatedEmployees
function post [string clientNamespace]/V1/Employees/[string xRefCode]/HighlyCompensatedEmployees(true isValidateOnly, EmployeeHighlyCompensatedEmployeeIndicator payload) returns json|error
POST a HighlyCompensatedEmployee
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeHighlyCompensatedEmployeeIndicator - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A HighlyCompensatedEmployee is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/HighlyCompensatedEmployees
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/HighlyCompensatedEmployees(true isValidateOnly, EmployeeHighlyCompensatedEmployeeIndicator payload) returns json|error
PATCH a HighlyCompensatedEmployee
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeHighlyCompensatedEmployeeIndicator - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The HighlyCompensatedEmployee is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/HealthWellnessDetails
function get [string clientNamespace]/V1/Employees/[string xRefCode]/HealthWellnessDetails(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeHealthWellness|error
GET EmployeeHealthWellness Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeHealthWellness|error - The EmployeeHealthWellness with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/HealthWellnessDetails
function post [string clientNamespace]/V1/Employees/[string xRefCode]/HealthWellnessDetails(true isValidateOnly, EmployeeHealthWellness payload) returns json|error
POST a EmployeeHealthWellness
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeHealthWellness - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeHealthWellness is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/HealthWellnessDetails
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/HealthWellnessDetails(true isValidateOnly, EmployeeHealthWellness payload) returns json|error
PATCH a EmployeeHealthWellness
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeHealthWellness - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeHealthWellness is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/HRIncidents
function get [string clientNamespace]/V1/Employees/[string xRefCode]/HRIncidents() returns Payload_IEnumerable_EmployeeHRIncident|error
GET EmployeeHRIncident Details
Return Type
- Payload_IEnumerable_EmployeeHRIncident|error - The EmployeeHRIncident with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/HRIncidents
function post [string clientNamespace]/V1/Employees/[string xRefCode]/HRIncidents(true isValidateOnly, EmployeeHRIncident payload) returns json|error
POST an EmployeeHRIncident
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeHRIncident -
Return Type
- json|error - An EmployeeHRIncident is created, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/HRPolicies
function get [string clientNamespace]/V1/Employees/[string xRefCode]/HRPolicies() returns Payload_IEnumerable_EmployeeHRPolicy|error
GET EmployeeHRPolicy Details
Return Type
- Payload_IEnumerable_EmployeeHRPolicy|error - The Employee HRPolicy with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/HRPolicies
function post [string clientNamespace]/V1/Employees/[string xRefCode]/HRPolicies(true isValidateOnly, EmployeeHRPolicy payload) returns json|error
POST an EmployeeHRPolicy
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validates the request without applying updates to the database.
- payload EmployeeHRPolicy - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee HRPolicy is created, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/EWSS
function get [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/EWSS() returns Payload_IEnumerable_EmployeeIRLTaxEWSS|error
GET an EmployeeIRLTax
Return Type
- Payload_IEnumerable_EmployeeIRLTaxEWSS|error - The EmployeeIRLTaxEWSS with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/EWSS
function post [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/EWSS(true isValidateOnly, EmployeeIRLTaxEWSS payload) returns json|error
POST an EmployeeIRLTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeIRLTaxEWSS - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeIRLTaxEWSS is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/EWSS
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/EWSS(true isValidateOnly, EmployeeIRLTaxEWSS payload) returns json|error
PATCH an EmployeeIRLTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeIRLTaxEWSS - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeIRLTaxEWSS is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PAYEExclusion
function get [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PAYEExclusion() returns Payload_IEnumerable_EmployeeIRLTaxPAYEExclusion|error
GET EmployeeIRLTaxPAYEExclusion Details
Return Type
- Payload_IEnumerable_EmployeeIRLTaxPAYEExclusion|error - The EmployeeIRLTaxPAYEExclusion with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PAYEExclusion
function post [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PAYEExclusion(true isValidateOnly, EmployeeIRLTaxPAYEExclusion payload) returns json|error
POST an EmployeeIRLTaxPAYEExclusion
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeIRLTaxPAYEExclusion -
Return Type
- json|error - An EmployeeIRLTaxPAYEExclusion is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PAYEExclusion
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PAYEExclusion(true isValidateOnly, EmployeeIRLTaxPAYEExclusion payload) returns json|error
PATCH an EmployeeIRLTaxPAYEExclusion
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeIRLTaxPAYEExclusion -
Return Type
- json|error - The EmployeeIRLTaxPAYEExclusion is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PRSI
function get [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PRSI() returns Payload_IEnumerable_EmployeeIRLTaxPRSI|error
GET EmployeeIRLTax Details
Return Type
- Payload_IEnumerable_EmployeeIRLTaxPRSI|error - The EmployeeIRLTaxPRSI with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PRSI
function post [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PRSI(true isValidateOnly, EmployeeIRLTaxPRSI payload) returns json|error
POST a EmployeeIRLTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeIRLTaxPRSI - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeIRLTaxPRSI is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PRSI
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/PRSI(true isValidateOnly, EmployeeIRLTaxPRSI payload) returns json|error
PATCH a EmployeeIRLTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeIRLTaxPRSI - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeIRLTaxPRSI is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/RPN
function get [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/RPN() returns Payload_IEnumerable_EmployeeIRLTaxRPN|error
GET an EmployeeIRLTax
Return Type
- Payload_IEnumerable_EmployeeIRLTaxRPN|error - The EmployeeIRLTaxRPN with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/RPN
function post [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/RPN(true isValidateOnly, EmployeeIRLTaxRPN payload) returns json|error
POST an EmployeeIRLTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeIRLTaxRPN - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeIRLTaxRPN is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/RPN
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/IRLTax/RPN(true isValidateOnly, EmployeeIRLTaxRPN payload) returns json|error
PATCH an EmployeeIRLTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeIRLTaxRPN -
Return Type
- json|error - The EmployeeIRLTaxRPN is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Locations
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Locations(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeLocation|error
GET EmployeeLocation Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeLocation|error - The EmployeeLocation with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Locations
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Locations(true isValidateOnly, EmployeeLocation payload) returns json|error
POST a EmployeeLocation
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeLocation - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeLocation is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Locations
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Locations(true isValidateOnly, EmployeeLocation payload) returns json|error
PATCH a EmployeeLocation
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeLocation - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeLocation is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeManagers
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeManagers(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeManager|error
GET EmployeeManager Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeManager|error - The EmployeeManager with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/OnboardingPolicies
function get [string clientNamespace]/V1/Employees/[string xRefCode]/OnboardingPolicies(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeOnboardingPolicy|error
GET EmployeeOnboardingPolicy Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeOnboardingPolicy|error - The EmployeeOnboardingPolicy with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/OnboardingPolicies
function post [string clientNamespace]/V1/Employees/[string xRefCode]/OnboardingPolicies(true isValidateOnly, EmployeeOnboardingPolicy payload) returns json|error
POST a EmployeeOnboardingPolicy
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeOnboardingPolicy - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/OnboardingPolicies
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/OnboardingPolicies(true isValidateOnly, EmployeeOnboardingPolicy payload) returns json|error
PATCH a EmployeeOnboardingPolicy
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeOnboardingPolicy - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeOnboardingPolicy is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/OrgUnitInfos
function get [string clientNamespace]/V1/Employees/[string xRefCode]/OrgUnitInfos() returns Payload_IEnumerable_EmployeeOrgUnitInformation|error
GET EmployeeOrgUnitInfo Details
Return Type
- Payload_IEnumerable_EmployeeOrgUnitInformation|error - The EmployeeOrgUnitInfo with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayAdjustCodeGroups
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayAdjustCodeGroups() returns Payload_IEnumerable_EmployeePayAdjustCodeGroup|error
GET EmployeePayAdjCodeGroupList Details
Return Type
- Payload_IEnumerable_EmployeePayAdjustCodeGroup|error - The EmployeePayAdjCodeGroupList with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayAdjustCodeGroups
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayAdjustCodeGroups(true isValidateOnly, EmployeePayAdjustCodeGroup payload) returns json|error
POST a EmployeePayAdjCodeGroupList
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeePayAdjustCodeGroup - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeePayAdjCodeGroupList is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayAdjustCodeGroups
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayAdjustCodeGroups(true isValidateOnly, EmployeePayAdjustCodeGroup payload) returns json|error
PATCH a EmployeePayAdjCodeGroupList
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeePayAdjustCodeGroup - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeePayAdjCodeGroupList is updated, no response body.
get [string clientNamespace]/V1/EmployeePayAdjustments
function get [string clientNamespace]/V1/EmployeePayAdjustments(string filterPayAdjustmentStartDate, string filterPayAdjustmentEndDate, string? filterLastModifiedStartDateUTC, string? filterLastModifiedEndDateUTC, string? orgUnitXRefCode, string? employeeXRefCode, string? payAdjustmentCodeXRefCode, string? projectXRefCode, string? departmentXRefCode, string? jobXRefCode, string? docketXRefCode, string? referenceDate, boolean? managerAuthorized, boolean? employeeAuthorized, boolean? isDeleted, Signed32? pageSize) returns PaginatedPayload_IEnumerable_EmployeePayAdjustment|error
Get a list of Employee Pay Adjustments
Parameters
- filterPayAdjustmentStartDate string - Inclusive period start date in UTC to determine which employee pay adjustment data to retrieve. Example: 2017-01-01T00:00:00
- filterPayAdjustmentEndDate string - Inclusive period end date in UTC to determine which employee pay adjustment data to retrieve. Example: 2017-01-01T00:00:00
- filterLastModifiedStartDateUTC string? (default ()) - Inclusive period last modified start date in UTC to determine which employee pay adjustment data to retrieve. Example: 2017-01-01T00:00:00Z
- filterLastModifiedEndDateUTC string? (default ()) - Inclusive period last modified end date in UTC to determine which employee pay adjustment data to retrieve. Example: 2017-01-01T00:00:00Z
- orgUnitXRefCode string? (default ()) - A case-sensitive field that identifies a unique organization
- employeeXRefCode string? (default ()) - A case-sensitive field that identifies a unique employee
- payAdjustmentCodeXRefCode string? (default ()) - A case-sensitive field that identifies a unique pay adjustment code
- projectXRefCode string? (default ()) - A case-sensitive field that identifies a unique project
- departmentXRefCode string? (default ()) - A case-sensitive field that identifies a unique department
- jobXRefCode string? (default ()) - A case-sensitive field that identifies a unique job
- docketXRefCode string? (default ()) - A case-sensitive field that identifies a unique docket
- referenceDate string? (default ()) - Inclusive period reference date in UTC to determine which employee pay adjustment data to retrieve. Example: 2017-01-01T00:00:00
- managerAuthorized boolean? (default ()) - A flag to determine if a pay adjustment is manager authorized
- employeeAuthorized boolean? (default ()) - A flag to determine if a pay adjustment is employee authorized
- isDeleted boolean? (default ()) - A flag to determine if a pay adjustment is deleted
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
Return Type
- PaginatedPayload_IEnumerable_EmployeePayAdjustment|error - A collection of EmployeePayAdjustment data meeting the search criteria.
post [string clientNamespace]/V1/EmployeePayAdjustments
function post [string clientNamespace]/V1/EmployeePayAdjustments(true isValidateOnly, EmployeePayAdjustmentForSubmit payload, boolean? isValidateLabor) returns EmployeePayAdjustmentPostResponse|error
POST (Create) Employee Pay Adjustment
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operations validate the request without applying updates to the database.
- payload EmployeePayAdjustmentForSubmit - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
- isValidateLabor boolean? (default ()) - When a TRUE value is used in this parameter, POST operations validate against Labor Validation Engine to verify pay adjustment follows rules/qualifiers created
Return Type
- EmployeePayAdjustmentPostResponse|error - An employee pay adjustment is created
patch [string clientNamespace]/V1/EmployeePayAdjustments/[string xRefCode]
function patch [string clientNamespace]/V1/EmployeePayAdjustments/[string xRefCode](true isValidateOnly, EmployeePayAdjustmentForSubmit payload, boolean? isValidateLabor) returns EmployeePayAdjustmentPostResponse|error
PATCH (Update) Employee Pay Adjustment
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH operations validate the request without applying updates to the database.
- payload EmployeePayAdjustmentForSubmit - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
- isValidateLabor boolean? (default ()) - When a TRUE value is used in this parameter, PATCH operations validate against Labor Validation Engine to verify pay adjustment follows rules/qualifiers created
Return Type
- EmployeePayAdjustmentPostResponse|error - An employee pay adjustment is updated
get [string clientNamespace]/V1/Employees/[string xRefCode]/PayGradeRates
function get [string clientNamespace]/V1/Employees/[string xRefCode]/PayGradeRates(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeePayGradeRate|error
GET EmployeePayGradeRate Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeePayGradeRate|error - The EmployeePayGradeRate with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxes
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxes() returns Payload_IEnumerable_EmployeePayrollTax|error
GET List of Taxes for a specific employee.
Return Type
- Payload_IEnumerable_EmployeePayrollTax|error - The EmployeePayrollTax with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParameters
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParameters(string? contextDateRangeFrom, string? contextDateRangeTo, string? taxAuthorityInstance, string? legalEntityXRefCode) returns Payload_IEnumerable_EmployeePayrollTaxParameter|error
GET List of Tax Parameters for a specific employee.
Parameters
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to the current day if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- taxAuthorityInstance string? (default ()) - The Governmental Authority having jurisdiction over the assessment, determination, collection or imposition of any tax. The value provided must be an exact match to an authority assigned to the employee. Example: USA-00000000-001.
- legalEntityXRefCode string? (default ()) - The unique reference code which identifies the organization that the federal tax authorities recognize. The value provided must be an exact match to the Legal Entity reference to which the employee is assigned.
Return Type
- Payload_IEnumerable_EmployeePayrollTaxParameter|error - The EmployeePayrollTax with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParameters
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParameters(true isValidateOnly, EmployeePayrollTaxParameter payload) returns json|error
POST Tax Parameter for an employee.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload EmployeePayrollTaxParameter - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeePayrollTaxParameter is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParameters
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParameters(true isValidateOnly, EmployeePayrollTaxParameter payload) returns json|error
PATCH Tax Parameter for an employee.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload EmployeePayrollTaxParameter - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeePayrollTaxParameter is created, no response body.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParametersList
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParametersList(true isValidateOnly, EmployeePayrollTaxParameter[] payload) returns json|error
POST Tax Parameter for an employee.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload EmployeePayrollTaxParameter[] - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - EmployeePayrollTaxParameter is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParametersList
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeePayrollTaxParametersList(true isValidateOnly, EmployeePayrollTaxParameter[] payload) returns json|error
PATCH Tax Parameter for an employee.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload EmployeePayrollTaxParameter[] - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - EmployeePayrollTaxParameter is created, no response body.
get [string clientNamespace]/V1/EmployeePaySummaries
function get [string clientNamespace]/V1/EmployeePaySummaries(string filterPaySummaryStartDate, string filterPaySummaryEndDate, string? employeeXRefCode, string? locationXRefCode, string? payGroupXRefCode, string? payCategoryXRefCode) returns PaginatedPayload_IEnumerable_EmployeePaySummary|error
GET a List of Employee pay summaries
Parameters
- filterPaySummaryStartDate string - Inclusive period start date in UTC to determine which employee pay summary data to retrieve. Example: 2017-01-01T00:00:00
- filterPaySummaryEndDate string - Inclusive period end date in UTC to determine which employee pay summary data to retrieve. Example: 2017-01-01T00:00:00
- employeeXRefCode string? (default ()) - The unique identifier (external reference code) of the employee to be retrieved. The value provided must be the exact match for an employee
- locationXRefCode string? (default ()) - The unique identifier (external reference code) of the location to be retrieved. The value provided must be the exact match for a location
- payGroupXRefCode string? (default ()) - The unique identifier (external reference code) of the position to be retrieved. The value provided must be the exact match for a position
- payCategoryXRefCode string? (default ()) - The unique identifier (external reference code) of the payCatergory to be retrieved. The value provided must be the exact match for a payCatergory
Return Type
- PaginatedPayload_IEnumerable_EmployeePaySummary|error - A collection of EmployeePaySummary data meeting the search criteria.
get [string clientNamespace]/V1/EmployeePaySummariesRetro
function get [string clientNamespace]/V1/EmployeePaySummariesRetro(string payGroupXRefCode, string? periodStartDate, string? periodEndDate, string? payDate, string? employeeXRefCode, string? locationXRefCode, string? payCategoryXRefCode, boolean? onlyRetros, int? payExportId, Signed32? pageSize) returns PaginatedPayload_IEnumerable_EmployeePaySummaryRetro|error
GET a List of Employee pay summaries retro
Parameters
- payGroupXRefCode string - The unique identifier (external reference code) of the pay group to be retrieved. The value provided must be the exact match for a pay group.
- periodStartDate string? (default ()) - The start date of the pay calendar to be retrieved. The value provided must be the exact match for an effective start of a pay calendar.
- periodEndDate string? (default ()) - The end date of the pay calendar to be retrieved. The value provided must be the exact match for an effective end of a pay calendar.
- payDate string? (default ()) - The pay date of the pay calendar to be retrieved. The value provided must be the exact match for a pay date of a pay calendar.
- employeeXRefCode string? (default ()) - The unique identifier (external reference code) of the employee to be retrieved. The value provided must be the exact match for an employee.
- locationXRefCode string? (default ()) - The unique identifier (external reference code) of the location to be retrieved. The value provided must be the exact match for a location.
- payCategoryXRefCode string? (default ()) - The unique identifier (external reference code) of the payCategory to be retrieved. The value provided must be the exact match for a payCategory.
- onlyRetros boolean? (default ()) - Filters only Retro Employee Pay Summaries (defaults to false).
- payExportId int? (default ()) - The unique identifier of the Pay export data to be retrieved. The value provided must be the exact match for a Pay export.
- pageSize Signed32? (default ()) - Number of records to be loaded (defaults to a 1000 records).
Return Type
- PaginatedPayload_IEnumerable_EmployeePaySummaryRetro|error - A collection of EmployeePaySummary retro data meeting the search criteria.
get [string clientNamespace]/V1/Employees/[string xRefCode]/PerformanceRatings
function get [string clientNamespace]/V1/Employees/[string xRefCode]/PerformanceRatings() returns Payload_IEnumerable_EmployeePerformanceRating|error
GET EmployeePerformanceRating Details
Return Type
- Payload_IEnumerable_EmployeePerformanceRating|error - The EmployeePerformanceRating with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/PerformanceRatings
function post [string clientNamespace]/V1/Employees/[string xRefCode]/PerformanceRatings(true isValidateOnly, EmployeePerformanceRating payload) returns json|error
Return Type
- json|error - An Employee Performance Rating is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/PerformanceRatings
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/PerformanceRatings(true isValidateOnly, EmployeePerformanceRating payload) returns json|error
Return Type
- json|error - An Employee Performance Rating is created, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeProperties
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeProperties(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeePropertyValue|error
GET EmployeePropertyValue Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeePropertyValue|error - The EmployeePropertyValue with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeProperties
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeProperties(true isValidateOnly, EmployeePropertyValue payload) returns json|error
POST a EmployeePropertyValue
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeePropertyValue - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeProperties
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeProperties(true isValidateOnly, EmployeePropertyValue payload) returns json|error
PATCH a EmployeePropertyValue
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeePropertyValue - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeePropertyValue is updated, no response body.
get [string clientNamespace]/V1/EmployeePunches
function get [string clientNamespace]/V1/EmployeePunches(string filterTransactionStartTimeUTC, string filterTransactionEndTimeUTC, string? employeeXRefCode, string? locationXRefCode, string? positionXRefCode, string? departmentXRefCode, string? jobXRefCode, string? docketXRefCode, string? projectXRefCode, string? payAdjustmentXRefCode, string? shiftStatus, string? filterShiftTimeStart, string? filterShiftTimeEnd, string? businessDate, Signed32? pageSize) returns PaginatedPayload_IEnumerable_EmployeePunch|error
GET a List of Employee Punches
Parameters
- filterTransactionStartTimeUTC string - Inclusive transaction period start date in UTC to determine which employee punch data to retrieve. Example: 2017-01-01T00:00:00
- filterTransactionEndTimeUTC string - Inclusive transaction period end date in UTC to determine which employee punch data to retrieve. Example: 2017-01-01T00:00:00
- employeeXRefCode string? (default ()) - The unique identifier (external reference code) of the employee to be retrieved. The value provided must be the exact match for an employee
- locationXRefCode string? (default ()) - A case-sensitive field that identifies a location or organizational units
- positionXRefCode string? (default ()) - A case-sensitive field that identifies one or more Positions
- departmentXRefCode string? (default ()) - A case-sensitive field that identifies one or more Departments
- jobXRefCode string? (default ()) - A case-sensitive field that identifies one or more Jobs
- docketXRefCode string? (default ()) - A case-sensitive field that identifies one or more dockets
- projectXRefCode string? (default ()) - A case-sensitive field that identifies one or more projects
- payAdjustmentXRefCode string? (default ()) - A case-sensitive field that identifies one or more pay adjustment
- shiftStatus string? (default ()) - A case-sensitive field containing shift status groups. Examples: [ACTIVE, COMPLETED, PROBLEM, ALL]
- filterShiftTimeStart string? (default ()) - Use with FilterTransactionStartTimeUTC to search for shifts with a Start and end time in a given timeframe. Example: Used to include or exclude edits made to historical punches
- filterShiftTimeEnd string? (default ()) - Use with FilterTransactionEndTimeUTC to search for shifts with a Start and end time in a given timeframe. Example: Used to include or exclude edits made to historical
- businessDate string? (default ()) - The Business Date value is intended as a “Timesheet View” to return punch data related to a clients Business day parameter configuration. Example: 2017-01-01T00:00:00
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
Return Type
- PaginatedPayload_IEnumerable_EmployeePunch|error - A collection of EmployeePunch data meeting the search criteria.
delete [string clientNamespace]/V1/EmployeePunches
function delete [string clientNamespace]/V1/EmployeePunches(string employeePunchXRefCode) returns Payload_EmployeePunchPatchPostDeleteResponse|error
Parameters
- employeePunchXRefCode string -
Return Type
- Payload_EmployeePunchPatchPostDeleteResponse|error - EmployeePunch details which matches XRefCode were Deleted.
get [string clientNamespace]/V1/EmployeeRawPunches
function get [string clientNamespace]/V1/EmployeeRawPunches(string filterTransactionStartTimeUTC, string filterTransactionEndTimeUTC, string? employeeXRefCode, string? employeeBadge, string? punchState, string? punchTypes, Signed32? pageSize) returns PaginatedPayload_IEnumerable_EmployeeRawPunch|error
GET a List of Employee Raw Punches
Parameters
- filterTransactionStartTimeUTC string - Inclusive transaction period start date in UTC to determine which employee punch data to retrieve. Example: 2017-01-01T00:00:00
- filterTransactionEndTimeUTC string - Inclusive transaction period end date in UTC to determine which employee punch data to retrieve. Example: 2017-01-01T00:00:00
- employeeXRefCode string? (default ()) - The unique identifier (external reference code) of the employee to be retrieved. The value provided must be the exact match for an employee
- employeeBadge string? (default ()) - The badge number of the employee to be retrieved. The value provided must be the exact match for a badge
- punchState string? (default ()) - The state of the punch. Examples: [PROCESSED, REJECTED, ALL]
- punchTypes string? (default ()) - Comma separated values of punch types. Example: [Punch_In, Break_Out, Job_Transfer, ALL, etc]
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
Return Type
- PaginatedPayload_IEnumerable_EmployeeRawPunch|error - A collection of EmployeePunch data meeting the search criteria.
post [string clientNamespace]/V1/EmployeeRawPunches
function post [string clientNamespace]/V1/EmployeeRawPunches(true isValidateOnly, EmployeeRawPunchForSubmit payload) returns PaginatedPayload_IEnumerable_EmployeeRawPunch|error
POST (Create) Employee Raw Punch
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validates the request without applying updates to the database.
- payload EmployeeRawPunchForSubmit -
Return Type
- PaginatedPayload_IEnumerable_EmployeeRawPunch|error - A raw punch is created.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Roles
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Roles(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeRole|error
GET EmployeeRole Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeRole|error - The EmployeeRole with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Roles
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Roles(true isValidateOnly, EmployeeRole payload) returns json|error
POST a EmployeeRole
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeRole - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Roles
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Roles(true isValidateOnly, EmployeeRole payload) returns json|error
PATCH a EmployeeRole
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeRole - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeRole is updated, no response body.
get [string clientNamespace]/V1/Employees
function get [string clientNamespace]/V1/Employees(string? employeeNumber, string? displayName, string? socialSecurityNumber, string? employmentStatusXRefCode, string? orgUnitXRefCode, string? departmentXRefCode, string? jobXRefCode, string? positionXRefCode, string? payClassXRefCode, string? payGroupXRefCode, string? payPolicyXRefCode, string? payTypeXRefCode, string? payrollPolicyXRefCode, string? filterHireStartDate, string? filterHireEndDate, string? filterTerminationStartDate, string? filterTerminationEndDate, string? filterUpdatedStartDate, string? filterUpdatedEndDate, string? filterUpdatedEntities, string? filterOriginalHireStartDate, string? filterOriginalHireEndDate, string? filterSeniorityStartDate, string? filterSeniorityEndDate, decimal? filterBaseSalaryFrom, decimal? filterBaseSalaryTo, decimal? filterBaseRateFrom, decimal? filterBaseRateTo, string? contextDate) returns Payload_IEnumerable_Employee|error
GET a List of Employees
Parameters
- employeeNumber string? (default ()) - Employment identification number assigned to an employee. A partial value can be provided for a wider search.
- displayName string? (default ()) - Employee Name. A partial value can be provided for a wider search.
- socialSecurityNumber string? (default ()) - Social Security Number of the employee. A partial value can be provided for a wider search.
- employmentStatusXRefCode string? (default ()) - Employment status xrefcode, which can be client-specific. Use this to search employees having the given employment status. Use a ContextDate value to search for employees with a given status as of a point in time. Otherwise, the search will use the current date and time.
- orgUnitXRefCode string? (default ()) - Organizational units' xrefcode. Use this to search all levels of the employees’ organization including department, location, region, corporate, etc. Use a ContextDate value to search for employees with a specific value as of a point in time. Otherwise, the search will use the current date and time.
- departmentXRefCode string? (default ()) - Department xrefcode value, which can be client-specific. Use this to search employees' work assignments having the given department.Use a ContextDate value to search for employees with a given department as of a point in time. Otherwise, the search will use the current date and time.
- jobXRefCode string? (default ()) - Job xrefcode value, which can be client-specific. Use this to search employees' work assignments having the given job. Use a ContextDate value to search for employees with a given job as of a point in time. Otherwise, the search will use the current date and time.
- positionXRefCode string? (default ()) - Position xrefcode value, which can be client-specific. Use this to search employees' work assignments having the given position. Use a ContextDate value to search for employees with a given position as of a point in time. Otherwise, the search will use the current date and time.
- payClassXRefCode string? (default ()) - Pay class xrefcode value, which can be client-specific. Use this to search employees having the given pay class. Use a ContextDate value to search for employees with a given pay class as of a point in time. Otherwise, the search will use the current date and time.
- payGroupXRefCode string? (default ()) - Pay group xrefcode value, which can be client-specific. Use this to search employees having the given pay group. Use a ContextDate value to search for employees with a given pay group as of a point in time. Otherwise, the search will use the current date and time.
- payPolicyXRefCode string? (default ()) - Pay policy xrefcode value, which can be client-specific. Use this to search employees having the given pay policy. Use a ContextDate value to search for employees with a given pay policy as of a point in time. Otherwise, the search will use the current date and time.
- payTypeXRefCode string? (default ()) - Pay type xrefcode value, which can be client-specific. Use this to search employees having the given pay type. Use a ContextDate value to search for employees with a given pay type as of a point in time. Otherwise, the search will use the current date and time.
- payrollPolicyXRefCode string? (default ()) - Payroll policy xrefcode value, which can be client-specific. Use this to search employees having the given payroll policy. Use a ContextDate value to search for employees with a given payroll policy as of a point in time. Otherwise, the search will use the current date and time.
- filterHireStartDate string? (default ()) - Use to search for employees whose most recent hire date is greater than or equal to the specified value (e.g. 2017-01-01T13:24:56). Use with filterHireEndDate to search for employees hired or rehired in a given timeframe.
- filterHireEndDate string? (default ()) - Use to search for employees whose most recent hire date is less than or equal to the specified value. Typically this parameter is used in conjunction with FilterHireStartDate to search for employees hired or rehired in a given timeframe. Example: 2017-01-01T13:24:56
- filterTerminationStartDate string? (default ()) - Use to search for employees with termination date values greater than or equal to the specified value. Typically this parameter is used in conjunction with FilterTerminationStartDate to search for employees terminated in a given timeframe. Example: 2017-01-01T13:24:56
- filterTerminationEndDate string? (default ()) - Use to search for employees with termination date values less than or equal to the specified value. Typically this parameter is used in conjunction with filterTerminationStartDate to search for employees terminated in a given timeframe. Example: 2017-01-01T13:24:56
- filterUpdatedStartDate string? (default ()) - The beginning date used when searching for employees with updates (and newly effective records) in a specified timeframe. When a value is provided for this parameter, a filterUpdatedEndDate value must also be provided. Because this search is conducted across all entities in the HR data model regardless of whether the requesting user has access to them, it is possible that the query will return XRefCode of employees with changes in which the consuming application is not interested. Example: 2017-01-01T13:24:56
- filterUpdatedEndDate string? (default ()) - The end date used when searching for employees with updates (and newly effective records) in a specified timeframe. When a value is provided for this parameter, a filterUpdatedStartDate value must also be provided. Example: 2017-01-01T13:24:56
- filterUpdatedEntities string? (default ()) - Use to search employees with changes to specific employee sub-entities. These sub-entity names, based on the employee model, can be provided in a comma-separated value, e.g. filterUpdatedEntities=EmploymentStatuses,WorkAssignments,Addresses. The base Employee is always searched by default. This parameter requires that filterUpdatedStartDate/filterUpdatedEndDate range is provided, otherwise it is ignored and all relevant employee entities are searched.
- filterOriginalHireStartDate string? (default ()) - Use to search for employees with original hire date values greater than or equal to the specified value. Typically this parameter is used in conjunction with filterOriginialHireEndDate to search for employees who were originally hired in a given timeframe. Example: 2017-01-01T13:24:56
- filterOriginalHireEndDate string? (default ()) - Use to search for employees with original hire date values less than or equal to the specified value. Typically this parameter is used in conjunction with filterOriginialHireStartDate to search for employees who were originally hired in a given timeframe. Example: 2017-01-01T13:24:56
- filterSeniorityStartDate string? (default ()) - Use to search for employees with seniority date values greater than or equal to the specified value. Typically this parameter is used in conjunction with filterSeniorityEndDate to search for employees seniority date in a given timeframe. Example: 2017-01-01T13:24:56
- filterSeniorityEndDate string? (default ()) - Use to search for employees with original hire date values less than or equal to the specified value. Typically this parameter is used in conjunction with filterSeniorityStartDate to search for employees seniority date in a given timeframe. Example: 2017-01-01T13:24:56
- filterBaseSalaryFrom decimal? (default ()) - Use to search for employees base salary greater than or equal to the specified value. Typically this parameter is used in conjunction with filterBaseSalaryTo to search for employees base salary in a given range. Example: 20000
- filterBaseSalaryTo decimal? (default ()) - Use to search for employees base salary less than or equal to the specified value. Typically this parameter is used in conjunction with filterBaseSalaryFrom to search for employees base salary in a given range. Example: 40000
- filterBaseRateFrom decimal? (default ()) - Use to search for employees base rate greater than or equal to the specified value. Typically this parameter is used in conjunction with filterBaseRateTo to search for employees base rate in a given range. Example: 10
- filterBaseRateTo decimal? (default ()) - Use to search for employees base rate less than or equal to the specified value. Typically this parameter is used in conjunction with filterBaseRateFrom to search for employees base rate in a given range. Example: 40
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_Employee|error - A collection of Employees XRefCodes meeting the search criteria.
post [string clientNamespace]/V1/Employees
function post [string clientNamespace]/V1/Employees(true isValidateOnly, Employee payload) returns json|error
POST (Hire) an Employee
Parameters
- isValidateOnly true - If true, then the POST data is validated without being submitted.
- payload Employee - The JSON-formatted content containing the data entities and elements to be processed in POST (hire and rehire ) and PATCH (employee update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee is created, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]
function get [string clientNamespace]/V1/Employees/[string xRefCode](string? contextDate, string? expand, string? contextDateRangeFrom, string? contextDateRangeTo, string? amfEntity, string? amfLevel, string? amfLevelValue) returns Payload_Employee|error
GET Employee Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- expand string? (default ()) - This parameter accepts a comma-separated list of top-level entities that contain the data elements needed for downstream processing. When this parameter is not used, only data elements from the employee master record will be included. For more information, please refer to the Introduction to Dayforce Web Services document.
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- amfEntity string? (default ()) - This parameter is to identify the application object for Application Metadata Framework (AMF) request.
- amfLevel string? (default ()) - This parameter is to identify the level for Application Metadata Framework (AMF) request.
- amfLevelValue string? (default ()) - This parameter is to identify context specific to amfLevel for Application Metadata Framework (AMF) request.
Return Type
- Payload_Employee|error - The Employee with the requested XRefCode.
patch [string clientNamespace]/V1/Employees/[string xRefCode]
function patch [string clientNamespace]/V1/Employees/[string xRefCode](true isValidateOnly, Employee payload, string? replaceExisting) returns json|error
PATCH (Update) an Employee
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload Employee - The JSON-formatted content containing the data entities and elements to be processed in POST (hire and rehire ) and PATCH (employee update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
- replaceExisting string? (default ()) - This parameter accepts a comma-separated list of employee-subordinate entities where the respective data provided will replace all existing records. It applies to the following collections (which are not effective dated and thus require this special treatment): <list type="bullet"><item>ClockDeviceGroups, </item><item>DirectDeposits, </item><item>EmployeePayAdjustCodeGroups, </item><item>OrgUnitLocationTypes, </item><item>SSOAccounts, </item><item>UserPayAdjustCodeGroups</item></list>
Return Type
- json|error - The Employee is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Schedules
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Schedules(string filterScheduleStartDate, string filterScheduleEndDate, boolean? isPosted, string? expand) returns Payload_IEnumerable_EmployeeSchedule|error
GET List Employee Schedules
Parameters
- filterScheduleStartDate string - Inclusive period start aligned to the employee business day start date to determine which employee schedule data to retrieve . Example: 2017-01-01T13:24:56
- filterScheduleEndDate string - Exclusive period end aligned to the employee business day start to determine which employee schedule data to retrieve . Example: 2017-01-01T13:24:56
- isPosted boolean? (default ()) - A flag to determine whether to display posted schedules.By default it searches for published schedules
- expand string? (default ()) - This parameter accepts a comma-separated list of top-level entities that contain the data elements needed for downstream processing. When this parameter is not used, only data elements from the master record will be included. For more information, please refer to the Introduction to Dayforce Web Services document.
Return Type
- Payload_IEnumerable_EmployeeSchedule|error - A collection of employee schedule meeting the search criteria.
get [string clientNamespace]/V1/EmployeeSchedules
function get [string clientNamespace]/V1/EmployeeSchedules(string filterScheduleStartDate, string filterScheduleEndDate, string? employeeXRefCode, string? departmentXRefCode, string? jobXRefCode, string? positionXRefCode, string? orgUnitXRefCode, boolean? unfill, string? expand, Signed32? pageSize) returns PaginatedPayload_IEnumerable_EmployeeSchedule|error
GET List of Employee Schedules
Parameters
- filterScheduleStartDate string - Inclusive period start aligned to the employee business day start date to determine which employee schedule data to retrieve . Example: 2017-01-01T13:24:56
- filterScheduleEndDate string - Exclusive period end aligned to the employee business day start to determine which employee schedule data to retrieve . Example: 2017-01-01T13:24:56
- employeeXRefCode string? (default ()) - Only valid when Unfill = FALSE or not provided
- departmentXRefCode string? (default ()) -
- jobXRefCode string? (default ()) -
- positionXRefCode string? (default ()) -
- orgUnitXRefCode string? (default ()) - Not required when Unfill = TRUE, Will send all unfilled shifts for the specified date range
- unfill boolean? (default ()) - When a TRUE value is used in this parameter, the API returns all Unfill shifts. When a FALSE value is used in this parameter, only employee schdules are returned
- expand string? (default ()) - This parameter accepts a comma-separated list of top-level entities that contain the data elements needed for downstream processing. When this parameter is not used, only data elements from the master record will be included. For more information, please refer to the Introduction to Dayforce Web Services document.
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
Return Type
- PaginatedPayload_IEnumerable_EmployeeSchedule|error - A collection of employee schedule meeting the search criteria.
post [string clientNamespace]/V1/EmployeeSchedules
function post [string clientNamespace]/V1/EmployeeSchedules(string orgUnitXRefCode, true isValidateOnly, EmployeeSchedulePostAPIRequestDTO[] payload, 0|1|2|3|4? violationLevel) returns Payload_IEnumerable_EmployeeSchedulePostAPIResponse|error
POST (Create) Employee schedule
Parameters
- orgUnitXRefCode string -
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validates the request without applying updates to the database.
- payload EmployeeSchedulePostAPIRequestDTO[] -
- violationLevel 0|1|2|3|4? (default ()) -
Return Type
- Payload_IEnumerable_EmployeeSchedulePostAPIResponse|error - OK response
patch [string clientNamespace]/V1/EmployeeSchedules
function patch [string clientNamespace]/V1/EmployeeSchedules(string orgUnitXRefCode, true isValidateOnly, EmployeeSchedulePatchAPIRequestDTO[] payload, 0|1|2|3|4? violationLevel) returns Payload_IEnumerable_EmployeeSchedulePostAPIResponse|error
PATCH (Update) Employee schedule
Parameters
- orgUnitXRefCode string -
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH operation validates the request without applying updates to the database.
- payload EmployeeSchedulePatchAPIRequestDTO[] -
- violationLevel 0|1|2|3|4? (default ()) -
Return Type
- Payload_IEnumerable_EmployeeSchedulePostAPIResponse|error - OK response
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeShortTimeWorks
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeShortTimeWorks(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeShortTimeWork|error
GET EmployeeShortTimeWork Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeShortTimeWork|error - The EmployeeShortTimeWorkController with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Skills
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Skills(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeSkill|error
GET EmployeeSkill Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeSkill|error - The EmployeeSkill with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Skills
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Skills(true isValidateOnly, EmployeeSkill payload) returns json|error
POST an Employee Skill
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeSkill - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee Skill is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Skills
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Skills(true isValidateOnly, EmployeeSkill payload) returns json|error
PATCH (Update) an Employee Skill
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeSkill - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An Employee Skill is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/TrainingPrograms
function get [string clientNamespace]/V1/Employees/[string xRefCode]/TrainingPrograms() returns Payload_IEnumerable_EmployeeTrainingProgram|error
GET EmployeeTrainingProgram Details
Return Type
- Payload_IEnumerable_EmployeeTrainingProgram|error - The EmployeeTrainingProgram with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/TrainingPrograms
function post [string clientNamespace]/V1/Employees/[string xRefCode]/TrainingPrograms(true isValidateOnly, EmployeeTrainingProgram payload) returns json|error
POST an EmployeeTrainingProgram
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeTrainingProgram - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeTrainingProgram is created, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/Irregular
function get [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/Irregular() returns Payload_IEnumerable_EmployeeUKIrregularPaymentDetails|error
GET an EmployeeUKIrregularPaymentDetails
Return Type
- Payload_IEnumerable_EmployeeUKIrregularPaymentDetails|error - The EmployeeUKIrregularPaymentDetail with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/Irregular
function post [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/Irregular(true isValidateOnly, EmployeeUKIrregularPaymentDetails payload) returns json|error
POST an EmployeeUKIrregularPaymentDetails
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUKIrregularPaymentDetails - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeUKIrregularPaymentDetails is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/Irregular
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/Irregular(true isValidateOnly, EmployeeUKIrregularPaymentDetails payload) returns json|error
PATCH an EmployeeUKIrregularPaymentDetails
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUKIrregularPaymentDetails -
Return Type
- json|error - The EmployeeUKIrregularPaymentDetails is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/UKNI/NIInfo
function get [string clientNamespace]/V1/Employees/[string xRefCode]/UKNI/NIInfo() returns Payload_IEnumerable_EmployeeUKNIDetails|error
GET Employee NI Details
Return Type
- Payload_IEnumerable_EmployeeUKNIDetails|error - The EmployeeUKNIDetails with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/UKNI/NIInfo
function post [string clientNamespace]/V1/Employees/[string xRefCode]/UKNI/NIInfo(true isValidateOnly, EmployeeUKNIDetails payload) returns Payload_IEnumerable_EmployeeUKNIDetails|error
POST Employee NI Details
Return Type
- Payload_IEnumerable_EmployeeUKNIDetails|error - Created NI Details for Employee with supplied XRefCode.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKNI/NIInfo
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKNI/NIInfo(true isValidateOnly, EmployeeUKNIDetails payload) returns json|error
PATCH Employee NI Details
Return Type
- json|error - The EmployeeUKTaxDetails is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/PostgraduateLoan
function get [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/PostgraduateLoan(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeUKPostgraduateLoan|error
GET EmployeeUKPostgraduateLoan Details
Parameters
- contextDate string? (default ()) -
- contextDateRangeFrom string? (default ()) -
- contextDateRangeTo string? (default ()) -
Return Type
- Payload_IEnumerable_EmployeeUKPostgraduateLoan|error - The EmployeeUKPostgraduateLoan with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/PostgraduateLoan
function post [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/PostgraduateLoan(true isValidateOnly, EmployeeUKPostgraduateLoan payload) returns json|error
POST an EmployeeUKPostgraduateLoan
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUKPostgraduateLoan - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeUKPostgraduateLoan is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/PostgraduateLoan
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/PostgraduateLoan(true isValidateOnly, EmployeeUKPostgraduateLoan payload) returns json|error
PATCH an EmployeeUKPostgraduateLoan
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUKPostgraduateLoan - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeUKPostgraduateLoan is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/StudentLoan
function get [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/StudentLoan(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeUKStudentLoan|error
GET EmployeeUKStudentLoan Details
Parameters
- contextDate string? (default ()) -
- contextDateRangeFrom string? (default ()) -
- contextDateRangeTo string? (default ()) -
Return Type
- Payload_IEnumerable_EmployeeUKStudentLoan|error - The EmployeeUKStudentLoan with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/StudentLoan
function post [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/StudentLoan(true isValidateOnly, EmployeeUKStudentLoan payload) returns json|error
POST an EmployeeUKStudentLoan
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUKStudentLoan - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeUKStudentLoan is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/StudentLoan
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/StudentLoan(true isValidateOnly, EmployeeUKStudentLoan payload) returns json|error
PATCH an EmployeeUKStudentLoan
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUKStudentLoan - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeUKStudentLoan is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/TaxInfo
function get [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/TaxInfo() returns Payload_IEnumerable_EmployeeUKTaxDetails|error
GET EmployeeUKTaxDetails Details
Return Type
- Payload_IEnumerable_EmployeeUKTaxDetails|error - The EmployeeUKTaxDetails with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/TaxInfo
function post [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/TaxInfo(true isValidateOnly, EmployeeUKTaxDetails payload) returns json|error
POST an EmployeeUKTaxDetails
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUKTaxDetails - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeUKTaxDetails is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/TaxInfo
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/UKTax/TaxInfo(true isValidateOnly, EmployeeUKTaxDetails payload) returns json|error
PATCH an EmployeeUKTaxDetails
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUKTaxDetails - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeUKTaxDetails is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/UnionMemberships
function get [string clientNamespace]/V1/Employees/[string xRefCode]/UnionMemberships(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeUnionMembership|error
GET EmployeeUnion Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeUnionMembership|error - The EmployeeUnion with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/UnionMemberships
function post [string clientNamespace]/V1/Employees/[string xRefCode]/UnionMemberships(true isValidateOnly, EmployeeUnionMembership payload) returns json|error
POST an EmployeeUnion
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUnionMembership - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeUnion is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/UnionMemberships
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/UnionMemberships(true isValidateOnly, EmployeeUnionMembership payload) returns json|error
PATCH an EmployeeUnion
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUnionMembership -
Return Type
- json|error - The EmployeeUnion is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/USFederalTaxes
function get [string clientNamespace]/V1/Employees/[string xRefCode]/USFederalTaxes(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeUSFederalTax|error
GET EmployeeUSFederalTax Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeUSFederalTax|error - The EmployeeUSFederalTax with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/USFederalTaxes
function post [string clientNamespace]/V1/Employees/[string xRefCode]/USFederalTaxes(true isValidateOnly, EmployeeUSFederalTax payload) returns json|error
POST a EmployeeUSFederalTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUSFederalTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeUSFederalTax is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/USFederalTaxes
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/USFederalTaxes(true isValidateOnly, EmployeeUSFederalTax payload) returns json|error
PATCH a EmployeeUSFederalTax
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUSFederalTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeUSFederalTax is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/USStateTaxes
function get [string clientNamespace]/V1/Employees/[string xRefCode]/USStateTaxes(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeUSStateTax|error
GET EmployeeUSStateTax Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeUSStateTax|error - The EmployeeUSStateTax with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/USStateTaxes
function post [string clientNamespace]/V1/Employees/[string xRefCode]/USStateTaxes(true isValidateOnly, EmployeeUSStateTax payload) returns json|error
POST EmployeeUSStateTax Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUSStateTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeUSStateTax Detail is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/USStateTaxes
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/USStateTaxes(true isValidateOnly, EmployeeUSStateTax payload) returns json|error
PATCH EmployeeUSStateTax Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUSStateTax - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeUSStateTax Detail is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/USTaxStatuses
function get [string clientNamespace]/V1/Employees/[string xRefCode]/USTaxStatuses(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeUSTaxStatus|error
GET EmployeeUSTaxStatus Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeUSTaxStatus|error - The EmployeeUSTaxStatus with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/USTaxStatuses
function post [string clientNamespace]/V1/Employees/[string xRefCode]/USTaxStatuses(true isValidateOnly, EmployeeUSTaxStatus payload) returns json|error
POST EmployeeUSTaxStatus Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUSTaxStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeUSTaxStatus Detail is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/USTaxStatuses
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/USTaxStatuses(true isValidateOnly, EmployeeUSTaxStatus payload) returns json|error
PATCH EmployeeUSTaxStatus Details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeUSTaxStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeUSTaxStatus Detail is updated, no response body.
get [string clientNamespace]/V1/EmployeeVOE/[string countryCode]/[string employeeXRefCode]
function get [string clientNamespace]/V1/EmployeeVOE/[string countryCode]/[string employeeXRefCode]() returns Payload_EmployeeVOE|error
GET Verification of Employment (VOE) for a specific employee.
Return Type
- Payload_EmployeeVOE|error - The EmployeePayrollTax with the requested XRefCode.
get [string clientNamespace]/V1/EmployeeVOI/[string countryCode]/[string employeeXRefCode]
function get [string clientNamespace]/V1/EmployeeVOI/[string countryCode]/[string employeeXRefCode]() returns Payload_EmployeeVOI|error
GET Verification of Income (VOI) for a specific employee.
Return Type
- Payload_EmployeeVOI|error - The EmployeePayrollTax with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/VeteranStatuses
function get [string clientNamespace]/V1/Employees/[string xRefCode]/VeteranStatuses(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeVeteransStatus|error
GET EmployeeVeteransStatus Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeVeteransStatus|error - The EmployeeVeteransStatusController with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/VeteranStatuses
function post [string clientNamespace]/V1/Employees/[string xRefCode]/VeteranStatuses(true isValidateOnly, EmployeeVeteransStatus payload) returns json|error
POST an EmployeeVeteransStatus
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeVeteransStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeVeteransStatus is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/VeteranStatuses
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/VeteranStatuses(true isValidateOnly, EmployeeVeteransStatus payload) returns json|error
PATCH an EmployeeVeteransStatus
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeVeteransStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeVeteransStatus is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/VolunteerLists
function get [string clientNamespace]/V1/Employees/[string xRefCode]/VolunteerLists(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeVolunteerList|error
GET Employee's Volunteer List Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeVolunteerList|error - The EmployeeVolunteerList for the requested employee XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/VolunteerLists
function post [string clientNamespace]/V1/Employees/[string xRefCode]/VolunteerLists(true isValidateOnly, EmployeeVolunteerList payload) returns json|error
POST an EmployeeVolunteerList
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeVolunteerList - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeVolunteerList is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/VolunteerLists
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/VolunteerLists(true isValidateOnly, EmployeeVolunteerList payload) returns json|error
PATCH an EmployeeVolunteerList
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeVolunteerList - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A EmployeeVolunteerList is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeWorkAssignmentManagers
function get [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeWorkAssignmentManagers(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeWorkAssignmentManager|error
GET EmployeeWorkAssignmentManager Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeWorkAssignmentManager|error - The EmployeeWorkAssignmentManager with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeWorkAssignmentManagers
function post [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeWorkAssignmentManagers(true isValidateOnly, EmployeeWorkAssignmentManager payload) returns json|error
POST a EmployeeWorkAssignmentManager
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeWorkAssignmentManager - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeWorkAssignmentManager is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeWorkAssignmentManagers
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeWorkAssignmentManagers(true isValidateOnly, EmployeeWorkAssignmentManager payload) returns json|error
PATCH a EmployeeWorkAssignmentManager
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeWorkAssignmentManager - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeWorkAssignmentManager is updated, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeWorkAssignmentManagers/Replacement
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/EmployeeWorkAssignmentManagers/Replacement(true isValidateOnly, EmployeeWorkAssignmentManager payload, string? replaceFrom, string? replaceTo) returns json|error
Replace EmployeeWorkAssignmentManagers
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, validate the request without applying updates to the database.
- payload EmployeeWorkAssignmentManager - The new JSON-formatted EmployeeWorkAssignmentManager with which to replace the deleted ones.
- replaceFrom string? (default ()) - The date from which all EmployeeWorkAssignmentManagers will be replaced.
- replaceTo string? (default ()) - The date up to which all EmployeeWorkAssignmentManagers will be replaced.
Return Type
- json|error - The Employee's Work Assignment Managers are replaced within the given date range; no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/WorkAssignments
function get [string clientNamespace]/V1/Employees/[string xRefCode]/WorkAssignments(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeWorkAssignment|error
GET WorkAssignment Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeWorkAssignment|error - The Work Assignments of employee with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/WorkAssignments
function post [string clientNamespace]/V1/Employees/[string xRefCode]/WorkAssignments(true isValidateOnly, EmployeeWorkAssignment payload) returns json|error
POST a WorkAssignment
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeWorkAssignment -
Return Type
- json|error - An EmployeeWorkAssignment is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/WorkAssignments
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/WorkAssignments(true isValidateOnly, EmployeeWorkAssignment payload) returns json|error
PATCH a Work Assignment
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeWorkAssignment - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The Work Assignment is updated, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/WorkAssignments/Replacement
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/WorkAssignments/Replacement(true isValidateOnly, EmployeeWorkAssignment payload, string? replaceFrom, string? replaceTo) returns json|error
Replace WorkAssignments
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, validate the request without applying updates to the database.
- payload EmployeeWorkAssignment - The new JSON-formatted WorkAssignment with which to replace the deleted ones.
- replaceFrom string? (default ()) - The date from which all WorkAssignments will be replaced.
- replaceTo string? (default ()) - The date up to which all WorkAssignments will be replaced.
Return Type
- json|error - The Employee's Work Assignments are replaced within the given date range; no response body.
get [string clientNamespace]/V1/Payroll/WorkLocationOverride
function get [string clientNamespace]/V1/Payroll/WorkLocationOverride(string? workLocationOverrideXRefCodes, string? locationAddressStateCodes, string? legalEntityXRefCode, string? legalEntityOverrideDetails, Signed32? pageSize) returns PaginatedPayload_IEnumerable_EmployeeWorkLocationOverride|error
GET List of work location overrides.
Parameters
- workLocationOverrideXRefCodes string? (default ()) - The comma delimited list of work location overrides XRef codes.
- locationAddressStateCodes string? (default ()) - The comma delimited list of location address state codes.
- legalEntityXRefCode string? (default ()) - The legal entity XRef code.
- legalEntityOverrideDetails string? (default ()) - Filter to fetch all or current/future effective legal entity overrides. Value can be All or Active. Default value is Active.
- pageSize Signed32? (default ()) - Number of records to be loaded.
Return Type
- PaginatedPayload_IEnumerable_EmployeeWorkLocationOverride|error - Work location overrides.
post [string clientNamespace]/v1/Payroll/WorkLocationOverrides
function post [string clientNamespace]/v1/Payroll/WorkLocationOverrides(true isValidateOnly, EmployeeWorkLocationOverride[] payload) returns Payload_IEnumerable_EmployeeWorkLocationOverride|error
POST Employee Elections
Parameters
- isValidateOnly true - If TRUE, POST operations validate the request without queue the import. The default value is FALSE. Note, POST operation will only validate data structure.
- payload EmployeeWorkLocationOverride[] - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- Payload_IEnumerable_EmployeeWorkLocationOverride|error - OK response
patch [string clientNamespace]/v1/Payroll/WorkLocationOverrides
function patch [string clientNamespace]/v1/Payroll/WorkLocationOverrides(true isValidateOnly, EmployeeWorkLocationOverride[] payload) returns Payload_IEnumerable_EmployeeWorkLocationOverride|error
PATCH Employee Elections
Parameters
- isValidateOnly true - If TRUE, PATCH operations validate the request without queue the import. The default value is FALSE. Note, PATCH operation will only validate data structure.
- payload EmployeeWorkLocationOverride[] - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- Payload_IEnumerable_EmployeeWorkLocationOverride|error - OK response
get [string clientNamespace]/V1/EmploymentStatuses
function get [string clientNamespace]/V1/EmploymentStatuses() returns Payload_IEnumerable_EmploymentStatus|error
GET a List of EmploymentStatus XRefCodes
Return Type
- Payload_IEnumerable_EmploymentStatus|error - A collection of EmploymentStatus XRefCodes.
get [string clientNamespace]/V1/EmploymentStatuses/[string xrefCode]
function get [string clientNamespace]/V1/EmploymentStatuses/[string xrefCode]() returns Payload_EmploymentStatus|error
GET a EmploymentStatus with the requested XRefCode.
Return Type
- Payload_EmploymentStatus|error - The EmploymentStatus with the requested XRefCode.
get [string clientNamespace]/V1/EmploymentStatusReasons
function get [string clientNamespace]/V1/EmploymentStatusReasons() returns Payload_IEnumerable_EmploymentStatusReason|error
GET a List of EmploymentStatusReason XRefCodes
Return Type
- Payload_IEnumerable_EmploymentStatusReason|error - A collection of EmploymentStatusReason XRefCodes.
get [string clientNamespace]/V1/EmploymentStatusReasons/[string xrefCode]
function get [string clientNamespace]/V1/EmploymentStatusReasons/[string xrefCode]() returns Payload_EmploymentStatusReason|error
GET a EmploymentStatusReason with the requested XRefCode.
Return Type
- Payload_EmploymentStatusReason|error - The EmploymentStatusReason with the requested XRefCode.
post [string clientNamespace]/V1/ESignatureOrder
POST e-signature order status.
Parameters
- payload record {}[] -
Return Type
get [string clientNamespace]/V1/ExtensibleProperty/[string typeCode]/[string entityType]/[string countryCode]/[string entityXRefCode]
function get [string clientNamespace]/V1/ExtensibleProperty/[string typeCode]/[string entityType]/[string countryCode]/[string entityXRefCode](string elementXRefCode, string activeAll) returns Payload_IEnumerable_ExtensibleProperty|error
GET The list of regional property.
Return Type
- Payload_IEnumerable_ExtensibleProperty|error - The ExtensibleProperty with the requested TypeCode,EntityType,CountryCode,EntityXrefCode.
post [string clientNamespace]/V1/ExtensibleProperty/[string typeCode]/[string entityType]/[string countryCode]/[string entityXRefCode]
function post [string clientNamespace]/V1/ExtensibleProperty/[string typeCode]/[string entityType]/[string countryCode]/[string entityXRefCode](true isValidateOnly, ExtensibleProperty[] payload) returns ExtensibleProperty[]|error
POST (Create) Extensible Property
Return Type
- ExtensibleProperty[]|error - The ExtensibleProperty with the requested TypeCode,EntityType,CountryCode,EntityXrefCode.
post [string clientNamespace]/V1/ExtensibleProperty/Remove/[string typeCode]/[string entityType]/[string countryCode]/[string entityXRefCode]
function post [string clientNamespace]/V1/ExtensibleProperty/Remove/[string typeCode]/[string entityType]/[string countryCode]/[string entityXRefCode](true isValidateOnly) returns ExtensibleProperty|error
Parameters
- isValidateOnly true -
Return Type
- ExtensibleProperty|error - The ExtensibleProperty with the requested EntityXrefCode.
post [string clientNamespace]/V1/GLOBALPayRunImport
function post [string clientNamespace]/V1/GLOBALPayRunImport(true isValidateOnly) returns json|error
POST Global Pay Run/s Details
Parameters
- isValidateOnly true - If TRUE, POST operations validate the request without queue the import. The default value is FALSE. Note, POST operation will only validate data structure.
Return Type
- json|error - A Global Pay Run is created with an empty response body.
patch [string clientNamespace]/V1/I9Orders/[string i9OrderId]
function patch [string clientNamespace]/V1/I9Orders/[string i9OrderId](true isValidateOnly, I9Order payload) returns xml|error
PATCH (Update) an I-9 order
get [string clientNamespace]/V1/JobFeeds
function get [string clientNamespace]/V1/JobFeeds(string? companyName, string? parentCompanyName, string? internalJobBoardCode, boolean? includeActivePostingOnly, string? lastUpdateTimeFrom, string? lastUpdateTimeTo, string? datePostedFrom, string? datePostedTo, boolean? htmlDescription) returns JobFeed[]|error
GET Job Feeds
Parameters
- companyName string? (default ()) - Company name. Example: XYZ Co.
- parentCompanyName string? (default ()) - Parent Company name. Example: Ceridian
- internalJobBoardCode string? (default ()) - XRefCode of Job Board. Example: CANDIDATEPORTAL
- includeActivePostingOnly boolean? (default ()) - If true, then exclude inactive postings from the result. If False, then the 'Last Update Time From' and 'Last Update Time To' parameters are required and the range specified between the 'Last Update Time From' and 'Last Update Time To' parameters must not be larger than 1 month. Example: True
- lastUpdateTimeFrom string? (default ()) - A starting timestamp of last updated job posting. Example: 2017-01-01T13:24:56
- lastUpdateTimeTo string? (default ()) - An ending timestamp of last updated job posting. Example: 2017-02-01T13:24:56
- datePostedFrom string? (default ()) - A starting timestamp of job posting date. Example: 2017-01-01T13:24:56
- datePostedTo string? (default ()) - An ending timestamp of job posting date. Example: 2017-02-01T13:24:56
- htmlDescription boolean? (default ()) - A flag to feed the jobs over with html formatting or plain text description
Return Type
get [string clientNamespace]/V1/Jobs
function get [string clientNamespace]/V1/Jobs(string? contextDate) returns Payload_IEnumerable_Job|error
GET a List of Jobs
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which job data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_Job|error - A collection of Job XRefCodes meeting the search criteria.
post [string clientNamespace]/V1/Jobs
POST (Add) a Job
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH (job update) operations validate the request without applying updates to the database.
- payload Job - The JSON-formatted content containing the data entities and elements to be processed in PATCH (job update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A Job is created, no response body.
get [string clientNamespace]/V1/Jobs/[string xrefCode]
function get [string clientNamespace]/V1/Jobs/[string xrefCode]() returns Payload_Job|error
GET a List of Jobs
Return Type
- Payload_Job|error - The Job with the requested XRefCode.
patch [string clientNamespace]/V1/Jobs/[string xRefCode]
function patch [string clientNamespace]/V1/Jobs/[string xRefCode](true isValidateOnly, Job payload) returns json|error
PATCH (Update) a Job
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH (job update) operations validate the request without applying updates to the database.
- payload Job - The JSON-formatted content containing the data entities and elements to be processed in PATCH (job update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A Job is updated, no response body.
get [string clientNamespace]/V1/KpiData
function get [string clientNamespace]/V1/KpiData(string orgUnitXRefCode, string kpiXRefCode, string kpiDataType, string timePeriod, string filterFrom, string filterTo, string? zoneXRefCode, string? mdseXRefCode) returns Payload_IEnumerable_KpiData|error
Get KPI Data matching search criteria (if neither Zone or MDSE supplied the data is assumed to be organization-wide)
Parameters
- orgUnitXRefCode string - Org unit reference code
- kpiXRefCode string - KPI reference Code
- kpiDataType string - KPI data type - Enter values for either actual, plan or forecast
- timePeriod string - KPI time period - Enter values for either minute, day or week
- filterFrom string - Start date of filter range
- filterTo string - End date of filter range
- zoneXRefCode string? (default ()) - Zone reference code
- mdseXRefCode string? (default ()) - MDSE reference code
Return Type
- Payload_IEnumerable_KpiData|error - KPI Data which matches the supplied parameters
post [string clientNamespace]/V1/KpiData
function post [string clientNamespace]/V1/KpiData(string orgUnitXRefCode, string kpiXRefCode, string kpiDataType, string timePeriod, true isValidateOnly, KpiData[] payload, boolean? aggregateToDay) returns json|error
Post KPI Data for the supplied parameters
Parameters
- orgUnitXRefCode string - Org unit reference code
- kpiXRefCode string - KPI reference code
- kpiDataType string - KPI data type - Enter values for either actual, plan or forecast
- timePeriod string - KPI time period - Enter values for either minute, day or week
- isValidateOnly true -
- payload KpiData[] -
- aggregateToDay boolean? (default ()) - Aggregate to day will be set to true by default. This will aggregate data from minute to day
Return Type
- json|error - The KPI Data has been created
patch [string clientNamespace]/V1/KpiData
function patch [string clientNamespace]/V1/KpiData(string orgUnitXRefCode, string kpiXRefCode, string kpiDataType, string timePeriod, true isValidateOnly, KpiData[] payload, boolean? aggregateToDay) returns json|error
Patch KPI Data for the supplied parameters
Parameters
- orgUnitXRefCode string - Org unit reference code
- kpiXRefCode string - KPI reference code
- kpiDataType string - KPI data type - Enter values for either actual, plan or forecast
- timePeriod string - KPI time period - Enter values for either minute, day or week
- isValidateOnly true -
- payload KpiData[] -
- aggregateToDay boolean? (default ()) - Aggregate to day will be set to true by default. This will aggregate data from minute to day
Return Type
- json|error - The KPI Data has been updated
get [string clientNamespace]/V1/KpiTargetPattern
function get [string clientNamespace]/V1/KpiTargetPattern(string orgUnitXRefCode, string kpiXRefCode, string startDate, string endDate, string? zoneXRefCode) returns Payload_IEnumerable_KpiTargetPattern|error
Get KPI Target Patterns matching search criteria
Parameters
- orgUnitXRefCode string - Org unit reference code
- kpiXRefCode string - KPI reference code
- startDate string - Start date
- endDate string - End date
- zoneXRefCode string? (default ()) - Zone reference code
Return Type
- Payload_IEnumerable_KpiTargetPattern|error - KPI Target Pattern details which matches the supplied parameters
post [string clientNamespace]/V1/KpiTargetPattern
function post [string clientNamespace]/V1/KpiTargetPattern(string orgUnitXRefCode, string kpiXRefCode, true isValidateOnly, KpiTargetPatternPOST[] payload) returns json|error
Post KPI Target Pattern for the supplied parameters
Parameters
- orgUnitXRefCode string - Org unit reference code
- kpiXRefCode string - KPI reference code
- isValidateOnly true - When a TRUE value is used in this parameter, the POST operation validates the request without creating records in the database. The default value is FALSE if parameter is not specified
- payload KpiTargetPatternPOST[] -
Return Type
- json|error - If isValidateOnly was passed in as true, this shows that the validation passed.
patch [string clientNamespace]/V1/KpiTargetPattern
function patch [string clientNamespace]/V1/KpiTargetPattern(string orgUnitXRefCode, string kpiXRefCode, true isValidateOnly, KpiTargetPatternPOST[] payload) returns json|error
Patch KPI Target Pattern for the supplied parameters
Parameters
- orgUnitXRefCode string - Org unit reference code
- kpiXRefCode string - KPI reference code
- isValidateOnly true - When a TRUE value is used in this parameter, the PATCH operation validates the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload KpiTargetPatternPOST[] -
Return Type
- json|error - If isValidateOnly was passed in as true, this shows that the validation passed
get [string clientNamespace]/V1/LaborCosts
function get [string clientNamespace]/V1/LaborCosts(string locationXRefCode, string businessDate) returns Payload_LaborCostResult|error
Return Type
- Payload_LaborCostResult|error - Labor cost result in the segment of 15 minutes.
get [string clientNamespace]/V1/LaborDemands
function get [string clientNamespace]/V1/LaborDemands(string orgUnitXRefCode, string schedulePeriodStart, string? filterFrom, string? filterTo, string? zoneXRefCode, string? activityXRefCode, string? laborMeasureXRefCode, string? axisXRefCode) returns Payload_LaborDemand|error
Get Labor Demand Curve details for Org over period
Parameters
- orgUnitXRefCode string - Org Unit Ref Code
- schedulePeriodStart string - start of schedule period
- filterFrom string? (default ()) - start of range (optional) - defaults to schedulePeriodStart if not supplied
- filterTo string? (default ()) - end of range (optional) - defaults to a week from schedulePeriodStart if not supplied
- zoneXRefCode string? (default ()) - filter by Zone Ref Code (optional)
- activityXRefCode string? (default ()) - filter by Activity Ref Code (optional)
- laborMeasureXRefCode string? (default ()) - filter by Labor Measure Ref Code (optional)
- axisXRefCode string? (default ()) - filter by Axis Ref Code (optional)
Return Type
- Payload_LaborDemand|error - Labor demand details which matches the supplied OrgXrefCode and SchedulePeriod
post [string clientNamespace]/V1/LaborDemands
function post [string clientNamespace]/V1/LaborDemands(true isValidateOnly, LaborDemand payload) returns json|error
Create a set of labor demands for an organization in the defined SchedulePeriod
Return Type
- json|error - The Labor Demands have been created
delete [string clientNamespace]/V1/LaborDemands
function delete [string clientNamespace]/V1/LaborDemands(string orgUnitXRefCode, string schedulePeriodStart, string? filterFrom, string? filterTo, string? zoneXRefCode, string? activityXRefCode, string? laborMeasureXRefCode, string? axisXRefCode) returns json|error
Deletes Labor Demand Curve details for Org over period Optional will only delete demands matching filters
Parameters
- orgUnitXRefCode string - Org Unit Ref Code
- schedulePeriodStart string - start of schedule period
- filterFrom string? (default ()) - start of range (optional) - defaults to schedulePeriodStart if not supplied
- filterTo string? (default ()) - end of range (optional) - defaults to a week from schedulePeriodStart if not supplied
- zoneXRefCode string? (default ()) - filter by Zone Ref Code (optional)
- activityXRefCode string? (default ()) - filter by Activity Ref Code (optional)
- laborMeasureXRefCode string? (default ()) - filter by Labor Measure Ref Code (optional)
- axisXRefCode string? (default ()) - filter by Axis Ref Code (optional)
Return Type
- json|error - The Labor demand details for the supplied OrgXrefCode and SchedulePeriod have been deleted
patch [string clientNamespace]/V1/LaborDemands
function patch [string clientNamespace]/V1/LaborDemands(string dayToPatch, true isValidateOnly, LaborDemand payload, string? zoneXRefCode, string? activityXRefCode, string? laborMeasureXRefCode, string? axisXRefCode) returns json|error
Update a set of labor demands for an organization in the defined SchedulePeriod
Parameters
- dayToPatch string -
- isValidateOnly true -
- payload LaborDemand -
- zoneXRefCode string? (default ()) -
- activityXRefCode string? (default ()) -
- laborMeasureXRefCode string? (default ()) -
- axisXRefCode string? (default ()) -
Return Type
- json|error - Created
get [string clientNamespace]/V1/LaborMetrics/[string xRefCode]
function get [string clientNamespace]/V1/LaborMetrics/[string xRefCode]() returns Payload_LaborMetricCodes|error
Get Labor Metric details
Return Type
- Payload_LaborMetricCodes|error - Labor metric details which matches the XRefCode.
post [string clientNamespace]/V1/LaborMetrics
function post [string clientNamespace]/V1/LaborMetrics(true isValidateOnly, LaborMetricsCodesForSubmit payload) returns LaborMetricsCodePostPatchResponse|error
Post Insert Labor metrics codes
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operations validate the request without applying updates to the database.
- payload LaborMetricsCodesForSubmit - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- LaborMetricsCodePostPatchResponse|error - New Labor metrics code data is inserted into Dayforce
patch [string clientNamespace]/V1/LaborMetrics
function patch [string clientNamespace]/V1/LaborMetrics(true isValidateOnly, LaborMetricsCodesForSubmit payload) returns json|error
Patch (Update) a Labor metrics code
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH operations validate the request without applying updates to the database.
- payload LaborMetricsCodesForSubmit - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - Labor metrics code data is updated
get [string clientNamespace]/V1/LaborMetricTypes/[string xRefCode]
function get [string clientNamespace]/V1/LaborMetricTypes/[string xRefCode]() returns Payload_LaborMetricType|error
Get Labor Metric Type details
Return Type
- Payload_LaborMetricType|error - Labor metric type details which matches the XRefCode.
post [string clientNamespace]/V1/LaborMetricTypes
function post [string clientNamespace]/V1/LaborMetricTypes(true isValidateOnly, LaborMetricTypeForSubmit payload) returns LaborMetricTypePatchPostResponse|error
POST Labor Metric Type details
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operations validate the request without applying updates to the database.
- payload LaborMetricTypeForSubmit - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- LaborMetricTypePatchPostResponse|error - New Labor Metric Type is inserted into Dayforce
patch [string clientNamespace]/V1/LaborMetricTypes
function patch [string clientNamespace]/V1/LaborMetricTypes(string laborMetricTypeXRefCode, true isValidateOnly, LaborMetricTypeForSubmit payload) returns json|error
Patch (Update) Labor Metric Type
Parameters
- laborMetricTypeXRefCode string - Labor Metric Type XRefCode
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH operations validate the request without applying updates to the database.
- payload LaborMetricTypeForSubmit - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - Labor Metric Type data is updated
post [string clientNamespace]/V1/LaborValidationPolicy/Search
function post [string clientNamespace]/V1/LaborValidationPolicy/Search(LaborValidationFilters payload, Signed32? pageSize) returns PaginatedPayload_IEnumerable_LaborValidationRule|error
Return Type
- PaginatedPayload_IEnumerable_LaborValidationRule|error - Search results for Labor Validation Policy rules based on parameters
get [string clientNamespace]/V1/LaborValidationPolicy/Rule/[string laborValidationPolicyRuleXRefCode]
function get [string clientNamespace]/V1/LaborValidationPolicy/Rule/[string laborValidationPolicyRuleXRefCode]() returns Payload_IEnumerable_LaborValidationRule|error
Get Labor Validation Policy Rule Details
Return Type
- Payload_IEnumerable_LaborValidationRule|error - Labor Validation Policy rules which matches the XRefCode
delete [string clientNamespace]/V1/LaborValidationPolicy/Rule/[string laborValidationPolicyRuleXRefCode]
function delete [string clientNamespace]/V1/LaborValidationPolicy/Rule/[string laborValidationPolicyRuleXRefCode]() returns json|error
Return Type
- json|error - Delete labor validation policy rules based on xrefcode
patch [string clientNamespace]/V1/LaborValidationPolicy/Rule/[string laborValidationPolicyRuleXRefCode]
function patch [string clientNamespace]/V1/LaborValidationPolicy/Rule/[string laborValidationPolicyRuleXRefCode](true isValidateOnly, LaborValidationRule payload) returns Payload_Boolean|error
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validates the request without applying updates to the database.
- payload LaborValidationRule -
Return Type
- Payload_Boolean|error - Post Validation Policy Rule
post [string clientNamespace]/V1/LaborValidationPolicy/Rule
function post [string clientNamespace]/V1/LaborValidationPolicy/Rule(LaborValidationRule payload) returns Payload_Boolean|error
Parameters
- payload LaborValidationRule -
Return Type
- Payload_Boolean|error - Post Validation Policy Rule
get [string clientNamespace]/V1/LegacyLaborMetric
function get [string clientNamespace]/V1/LegacyLaborMetric(string legacyLaborMetricType, string legacyLaborMetricXRefCode) returns Payload_LegacyLaborMetricCodes|error
Get Legacy Labor Metric data
Return Type
- Payload_LegacyLaborMetricCodes|error - Legacy labor metric details which matches the Type and XRefCode.
post [string clientNamespace]/V1/LegacyLaborMetric
function post [string clientNamespace]/V1/LegacyLaborMetric(true isValidateOnly, LegacyLaborMetricForSubmit payload) returns LegacyLaborMetricPatchPostDeleteResponse|error
Post Insert Legacy Labor metrics
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operations validate the request without applying updates to the database.
- payload LegacyLaborMetricForSubmit - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- LegacyLaborMetricPatchPostDeleteResponse|error - New Legacy Labor Metric data is inserted into Dayforce
delete [string clientNamespace]/V1/LegacyLaborMetric
function delete [string clientNamespace]/V1/LegacyLaborMetric(string legacyLaborMetricType, string legacyLaborMetricXRefCode) returns json|error
Return Type
- json|error - Legacy labor metric details which matches the Type and XRefCode were Deleted.
patch [string clientNamespace]/V1/LegacyLaborMetric
function patch [string clientNamespace]/V1/LegacyLaborMetric(string legacyLaborMetricXRefCode, true isValidateOnly, LegacyLaborMetricForSubmit payload) returns json|error
Patch (Update) a Legacy Labor Metric
Parameters
- legacyLaborMetricXRefCode string - XRefCode
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH operations validate the request without applying updates to the database.
- payload LegacyLaborMetricForSubmit - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - Legacy Labor Metric data is updated
get [string clientNamespace]/V1/LocationAddresses
function get [string clientNamespace]/V1/LocationAddresses(string? shortName, string? countryCode, string? stateProvinceCode) returns Payload_IEnumerable_LocationAddresses|error
GET List of Location Addresses.
Parameters
- shortName string? (default ()) - Filter the location addresses by their ShortName. The service defaults to NULL if the requester does not specify a value.
- countryCode string? (default ()) -
- stateProvinceCode string? (default ()) -
Return Type
- Payload_IEnumerable_LocationAddresses|error - A collection of Location Addresses meeting the search criteria, if provided.
post [string clientNamespace]/V1/LocationAddresses
function post [string clientNamespace]/V1/LocationAddresses(true isValidateOnly, LocationAddresses payload) returns json|error
POST Location Address.
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload LocationAddresses - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A Location Address is created with an empty response body.
patch [string clientNamespace]/V1/LocationAddresses
function patch [string clientNamespace]/V1/LocationAddresses(string xRefCode, true isValidateOnly, LocationAddresses payload) returns json|error
PATCH Location Address.
Parameters
- xRefCode string - The unique identifier (external reference code) of the position. The value provided must be the exact match for a position; otherwise, a bad request (400) error will be returned.
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload LocationAddresses - The JSON-formatted content containing the data entities and elements to be processed in POST (position add) and PATCH (position update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A Location Address is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/MaritalStatuses
function get [string clientNamespace]/V1/Employees/[string xRefCode]/MaritalStatuses(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeMaritalStatus|error
GET MaritalStatus Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeMaritalStatus|error - The Marital Statuses of employee with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/MaritalStatuses
function post [string clientNamespace]/V1/Employees/[string xRefCode]/MaritalStatuses(true isValidateOnly, EmployeeMaritalStatus payload) returns json|error
POST a MaritalStatus
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeMaritalStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A MaritalStatus is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/MaritalStatuses
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/MaritalStatuses(true isValidateOnly, EmployeeMaritalStatus payload) returns json|error
PATCH a MaritalStatus
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeMaritalStatus - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The MaritalStatus is updated, no response body.
get [string clientNamespace]/V1/OperatingHours/GetOperatingHours
function get [string clientNamespace]/V1/OperatingHours/GetOperatingHours(string startDate, string endDate, string orgUnitXRefCode) returns Payload_OperatingHours|error
Parameters
- startDate string - Date to represent the start of the period for which operating hours should be retrieved. Format yyyy-mm-dd
- endDate string - Date to represent the end of the period for which operating hours should be retrieved. Format yyyy-mm-dd
- orgUnitXRefCode string - The unique identifier (external reference code) of the organization to be retrieved. The value provided must be the exact match for an organization
Return Type
- Payload_OperatingHours|error - Operating Hours details which matches the supplied OrgUnitXRefCode and date range.
post [string clientNamespace]/V1/OperatingHours/PostOperatingHoursException
function post [string clientNamespace]/V1/OperatingHours/PostOperatingHoursException(true isValidateOnly, OperatingHoursExceptionPOST[] payload) returns json|error
Return Type
- json|error - OK
patch [string clientNamespace]/V1/OperatingHours/PatchOperatingHoursException
function patch [string clientNamespace]/V1/OperatingHours/PatchOperatingHoursException(true isValidateOnly, OperatingHoursExceptionPOST[] payload) returns json|error
Return Type
- json|error - OK
get [string clientNamespace]/V1/OrgUnits
function get [string clientNamespace]/V1/OrgUnits() returns Payload_IEnumerable_Location|error
Get a list of OrgUnits
Return Type
- Payload_IEnumerable_Location|error - A collection of OrgUnit XRefCodes meeting the search criteria.
post [string clientNamespace]/V1/OrgUnits
function post [string clientNamespace]/V1/OrgUnits(true isValidateOnly, Location payload, string? calibrateOrg) returns json|error
POST (Add) an OrgUnit
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload Location - The JSON-formatted content containing the data entities and elements to be processed in POST (orgunit add) and PATCH (orgunit update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
- calibrateOrg string? (default ()) - This parameter accepts TRUE or FALSE values to determine whether the Org Recalculation process is to be triggered. The default value is TRUE if parameter is not specified.
Return Type
- json|error - An Org Unit is created, no response body.
get [string clientNamespace]/V1/OrgUnits/[string xrefCode]
function get [string clientNamespace]/V1/OrgUnits/[string xrefCode](string? contextDate, string? expand, boolean? includeChildOrgUnits) returns Payload_Location|error
Get OrgUnit details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which org unit data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2019-01-01T12:34:56
- expand string? (default ()) - This parameter accepts a comma-separated list of top-level entities that contain the data elements needed for downstream processing. When this parameter is not used, only data elements from the orgunit master record will be included. For more information, please refer to the Introduction to Dayforce Web Services document.
- includeChildOrgUnits boolean? (default ()) - When a TRUE value is used in this parameter, the immediate child org units’ information under the org unit being retrieved will be returned as well. The default value is FALSE if parameter is not specified.
Return Type
- Payload_Location|error - The Org Unit with the requested XRefCode.
patch [string clientNamespace]/V1/OrgUnits/[string xRefCode]
function patch [string clientNamespace]/V1/OrgUnits/[string xRefCode](true isValidateOnly, Location payload, string? replaceExisting, string? calibrateOrg) returns json|error
PATCH (Update) an OrgUnit
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload Location - The JSON-formatted content containing the data entities and elements to be processed in POST (orgunit add) and PATCH (orgunit update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
- replaceExisting string? (default ()) - This parameter accepts a comma-separated list of OrgUnit sub-entities where the respective data provided will replace all existing records. This currently applies to OrgUnitLocationTypes sub-entities, which are considered as a list and are not effective dated.
- calibrateOrg string? (default ()) - This parameter accepts TRUE or FALSE values to determine whether the Org Recalculation process is to be triggered. The default value is TRUE if parameter is not specified.
Return Type
- json|error - An Org Unit is updated, no response body.
get [string clientNamespace]/V1/PayClasses
function get [string clientNamespace]/V1/PayClasses(string? contextDate) returns Payload_IEnumerable_PayClass|error
GET a List of PayClasses
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which PayClass data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_PayClass|error - A collection of PayClass XRefCodes meeting the search criteria.
get [string clientNamespace]/V1/PayClasses/[string xrefCode]
function get [string clientNamespace]/V1/PayClasses/[string xrefCode]() returns Payload_PayClass|error
Get PayClass details
Return Type
- Payload_PayClass|error - The PayClass with the requested XRefCode.
get [string clientNamespace]/V1/Payee
function get [string clientNamespace]/V1/Payee(string? payeeStatus, string? payeeXRefCodes, string? payeeCategoryXRefCodes, string? paymentMethodXRefCodes, string? operatingCountries, boolean? earningPayee, boolean? deductionPayee, boolean? systemPayee) returns Payload_IEnumerable_Payee|error
GET List of Third Party Payees.
Parameters
- payeeStatus string? (default ()) - >Can be Active, InActive or All. The default value is Active.
- payeeXRefCodes string? (default ()) - >List of Payee Reference Codes with comma separator to filter the result.
- payeeCategoryXRefCodes string? (default ()) - >List of Payee Category Reference Codes with comma separator to filter the result.
- paymentMethodXRefCodes string? (default ()) - >List of Pay Method Reference Codes with comma separator to filter the result.
- operatingCountries string? (default ()) - >List of country codes with comma separator to filter the result base on country.
- earningPayee boolean? (default ()) - >Set to true to only get Earning Payees.
- deductionPayee boolean? (default ()) - >Set to true to only get Deduction Payees.
- systemPayee boolean? (default ()) - >Set to true to only get System Payees.
Return Type
- Payload_IEnumerable_Payee|error - List of payees.
post [string clientNamespace]/V1/Payee
function post [string clientNamespace]/V1/Payee(true isValidateOnly, Payee[] payload) returns Payload_List_Payee|error
POST Add Third Party Payees
Parameters
- isValidateOnly true - If true, only validate the request. Otherwise, validate and save.
- payload Payee[] -
Return Type
- Payload_List_Payee|error - OK response
patch [string clientNamespace]/V1/Payee
function patch [string clientNamespace]/V1/Payee(true isValidateOnly, Payee[] payload) returns Payload_List_Payee|error
PATCH Update Third Party Payees
Parameters
- isValidateOnly true - If true, only validate the request. Otherwise, validate and save.
- payload Payee[] -
Return Type
- Payload_List_Payee|error - OK response
get [string clientNamespace]/V1/PayGroupCalendar/[string payGroupXRefCode]
function get [string clientNamespace]/V1/PayGroupCalendar/[string payGroupXRefCode](boolean? payrollCommitted, Signed32? payDateYear, string? payDate, string? periodStartDate, string? periodEndDate, Signed32? payPeriod) returns Payload_PayGroupCalendar|error
GET List of Pay Group Calendars.
Parameters
- payrollCommitted boolean? (default ()) -
- payDateYear Signed32? (default ()) - The pay group calen xdar's pay date year.
- payDate string? (default ()) - The pay group calendar's pay date.
- periodStartDate string? (default ()) - The pay group calendar's period start date.
- periodEndDate string? (default ()) - The pay group calendar's period end date.
- payPeriod Signed32? (default ()) - The pay group calendar's pay period number.
Return Type
- Payload_PayGroupCalendar|error - The Pay Group Calendar.
get [string clientNamespace]/V1/Payroll/PayrollElection
function get [string clientNamespace]/V1/Payroll/PayrollElection(string? 'source, string? codeType, string? electionStatus, string? payGroupXRefCode, string? employeeXRefCodes, string? employmentStatusXRefCode, Signed32? pageSize) returns PaginatedPayload_IEnumerable_EmployeePayrollElection|error
GET List of Employee Elections.
Parameters
- 'source string? (default ()) -
- codeType string? (default ()) -
- electionStatus string? (default ()) -
- payGroupXRefCode string? (default ()) -
- employeeXRefCodes string? (default ()) -
- employmentStatusXRefCode string? (default ()) -
- pageSize Signed32? (default ()) -
Return Type
- PaginatedPayload_IEnumerable_EmployeePayrollElection|error - The Employee Payroll Election.
post [string clientNamespace]/v1/Payroll/PayrollElections
function post [string clientNamespace]/v1/Payroll/PayrollElections(true isValidateOnly, EmployeePayrollElection[] payload, boolean? autoUpdateExisting) returns Payload_IEnumerable_EmployeePayrollElection|error
POST Employee Elections
Parameters
- isValidateOnly true - If TRUE, POST operations validate the request without queue the import. The default value is FALSE. Note, POST operation will only validate data structure.
- payload EmployeePayrollElection[] - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
- autoUpdateExisting boolean? (default ()) - If TRUE, all other elections of the same employee will be end-dated. The default value is FALSE.
Return Type
- Payload_IEnumerable_EmployeePayrollElection|error - OK response
patch [string clientNamespace]/v1/Payroll/PayrollElections
function patch [string clientNamespace]/v1/Payroll/PayrollElections(true isValidateOnly, EmployeePayrollElection[] payload, boolean? autoUpdateExisting) returns Payload_IEnumerable_EmployeePayrollElection|error
PATCH Employee Elections
Parameters
- isValidateOnly true - If TRUE, POST operations validate the request without queue the import. The default value is FALSE. Note, POST operation will only validate data structure.
- payload EmployeePayrollElection[] - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
- autoUpdateExisting boolean? (default ()) - If TRUE, all other elections of the same employee will be end-dated. The default value is FALSE.
Return Type
- Payload_IEnumerable_EmployeePayrollElection|error - OK response
patch [string clientNamespace]/v1/Payroll/DeletePayrollElections
function patch [string clientNamespace]/v1/Payroll/DeletePayrollElections(true isValidateOnly, EmployeePayrollElectionDeletionModel[] payload) returns Payload_IEnumerable_EmployeePayrollElectionDeletionModel|error
PATCH Employee Elections
Parameters
- isValidateOnly true -
- payload EmployeePayrollElectionDeletionModel[] - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
post [string clientNamespace]/V1/PayrollGL/Import
function post [string clientNamespace]/V1/PayrollGL/Import(true isValidateOnly, record {} payload) returns json|error
POST Import GL Result
Parameters
- isValidateOnly true - If TRUE, POST operations validate the request without queue the import. The default value is FALSE. Note, POST operation will only validate data structure.
- payload record {} - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - GLResult Import was created successfully, no response body.
post [string clientNamespace]/V1/Payroll/Import/TimeData
function post [string clientNamespace]/V1/Payroll/Import/TimeData(true isValidateOnly, record {} payload) returns json|error
POST Import Time Data
Parameters
- isValidateOnly true - If TRUE, POST operations validate the request without queue the import. The default value is FALSE. Note, POST operation will only validate data structure.
- payload record {} - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - Time Data Import was created successfully, no response body.
patch [string clientNamespace]/V1/Payroll/TimeDataDelete/[string payGroupXRefCode]
function patch [string clientNamespace]/V1/Payroll/TimeDataDelete/[string payGroupXRefCode](true isValidateOnly, ImportSetModel payload, string? 'source, string? periodStartDate, string? periodEndDate, string? payDate, string? ppn, string? orgUnitXRefCode, string? offCyclePayRunXRefCode) returns json|error
DELETE Import Time Data
Parameters
- isValidateOnly true - If TRUE, DELETE operations validate the request and IDs. The default value is FALSE. Note, DELETE operation will only validate data structure.
- payload ImportSetModel - The JSON-formatted content containing the data entities ids for DELETE operation.
- 'source string? (default ()) - The source of the import.
- periodStartDate string? (default ()) - The pay run period start date.
- periodEndDate string? (default ()) - The pay run period end date.
- payDate string? (default ()) - The pay run pay date.
- ppn string? (default ()) - The pay run's pay period number (format: ##-##).
- orgUnitXRefCode string? (default ()) - The user org unit reference code to load data entries from (in org unit hierarchy).
- offCyclePayRunXRefCode string? (default ()) - Offcycle pay run XRef Code.
Return Type
- json|error - Time Data Import was deleted successfully, no response body.
get [string clientNamespace]/V1/Payroll/PayrollPayEntryImportHistory/[string payGroupXRefCode]
function get [string clientNamespace]/V1/Payroll/PayrollPayEntryImportHistory/[string payGroupXRefCode](string? 'source, string? importsFromDate, string? importsToDate, boolean? problemsOnly, string? periodStartDate, string? periodEndDate, string? payDate, string? ppn, string? offCyclePayRunXRefCode, string? importIdentifiers) returns Payload_PayrollPayEntryImportHistory|error
GET the list of Time Data Import Job History with Error info.
Parameters
- 'source string? (default ()) - The time data import source name.
- importsFromDate string? (default ()) - The time data import history from date.
- importsToDate string? (default ()) - The time data import history to date.
- problemsOnly boolean? (default ()) - If true, then return only time data which has errors. If false, then return both error and non error records.
- periodStartDate string? (default ()) - The pay run period start date.
- periodEndDate string? (default ()) - The pay run period end date.
- payDate string? (default ()) - The pay run pay date.
- ppn string? (default ()) - The pay run's pay period number (format: ##-##).
- offCyclePayRunXRefCode string? (default ()) - The off cycle pay run reference code.
- importIdentifiers string? (default ()) - The comma separated list of import identifiers.
Return Type
- Payload_PayrollPayEntryImportHistory|error - Time data import jobs history.
post [string clientNamespace]/V1/Payroll/Import/QuickEntry
function post [string clientNamespace]/V1/Payroll/Import/QuickEntry(true isValidateOnly, record {} payload) returns json|error
POST Quick Entry Items
Parameters
- isValidateOnly true - If TRUE, POST operations validate the request without queue the import. The default value is FALSE. Note, POST operation will only validate data structure.
- payload record {} - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - Quick Entry Import was created successfully, no response body.
patch [string clientNamespace]/V1/Payroll/QuickEntry
function patch [string clientNamespace]/V1/Payroll/QuickEntry(true isValidateOnly, DataEntry[] payload) returns json|error
PATCH Quick Entry Items
Parameters
- isValidateOnly true - If TRUE, PATCH operations validate the request without updating the item. The default value is FALSE. Note, PATCH operation will only validate data structure.
- payload DataEntry[] -
Return Type
- json|error - Quick Entry Import was updated successfully, no response body.
get [string clientNamespace]/V1/TaxAuthorityInstanceDetails
function get [string clientNamespace]/V1/TaxAuthorityInstanceDetails(string? countryCodes) returns Payload_IEnumerable_TaxAuthorityInstanceDetails|error
GET List of IPS Tax Authority Instance Details.
Parameters
- countryCodes string? (default ()) - List of three letter country codes with comma separator to filter the result.
Return Type
- Payload_IEnumerable_TaxAuthorityInstanceDetails|error - The IPS Tax Authority Instance Details.
get [string clientNamespace]/V1/PayRunStatus/[string payGroupXRefCode]
function get [string clientNamespace]/V1/PayRunStatus/[string payGroupXRefCode](string? payDate, string? periodStartDate, string? periodEndDate, string? ppn) returns Payload_PayRunStatus|error
GET Pay Run Status.
Parameters
- payDate string? (default ()) - The pay run's pay date (format: yyyy-mm-dd).
- periodStartDate string? (default ()) - The pay run's period start date (format: yyyy-mm-dd).
- periodEndDate string? (default ()) - The pay run's period end date (format: yyyy-mm-dd).
- ppn string? (default ()) - The PayPeriodNumber(format: ##-##).
Return Type
- Payload_PayRunStatus|error - The pay run status.
get [string clientNamespace]/V1/PayTypes
function get [string clientNamespace]/V1/PayTypes() returns Payload_IEnumerable_PayType|error
GET a List of PayTypes
Return Type
- Payload_IEnumerable_PayType|error - A collection of PayType XRefCodes.
get [string clientNamespace]/V1/PayTypes/[string xrefCode]
function get [string clientNamespace]/V1/PayTypes/[string xrefCode]() returns Payload_PayType|error
GET a PayType with the requested XRefCode.
Return Type
- Payload_PayType|error - The PayType with the requested XRefCode.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Addresses
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Addresses(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_PersonAddress|error
GET PersonAddress Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_PersonAddress|error - The PersonAddress with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Addresses
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Addresses(true isValidateOnly, PersonAddress payload) returns json|error
POST a PersonAddress
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload PersonAddress - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A PersonAddress is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Addresses
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Addresses(true isValidateOnly, PersonAddress payload) returns json|error
PATCH a PersonAddress
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload PersonAddress - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The PersonAddress is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/Contacts
function get [string clientNamespace]/V1/Employees/[string xRefCode]/Contacts(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_PersonContact|error
GET PersonContact Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_PersonContact|error - The PersonContact with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/Contacts
function post [string clientNamespace]/V1/Employees/[string xRefCode]/Contacts(true isValidateOnly, PersonContact payload) returns json|error
POST a PersonContact
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload PersonContact - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A PersonContact is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/Contacts
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/Contacts(true isValidateOnly, PersonContact payload) returns json|error
PATCH a PersonContact
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload PersonContact - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The PersonContact is updated, no response body.
get [string clientNamespace]/V1/PlanTargets
function get [string clientNamespace]/V1/PlanTargets(string? planTargetXRefCode, string? orgUnitXRefCode, string? kpiXRefCode, string? zoneXRefCode, string? axisXRefCode, boolean? isActive) returns Payload_IEnumerable_PlanTarget|error
Get Plan Targets matching search criteria
Parameters
- planTargetXRefCode string? (default ()) - filter by Plan Target Ref Code (optional)
- orgUnitXRefCode string? (default ()) - filter by Org Unit Ref Code
- kpiXRefCode string? (default ()) - filter by KPI Ref Code (optional)
- zoneXRefCode string? (default ()) - filter by Zone Ref Code (optional)
- axisXRefCode string? (default ()) - filter by Axis Ref Code (optional)
- isActive boolean? (default ()) - filter only active Plan Targets
Return Type
- Payload_IEnumerable_PlanTarget|error - Plan Target details which matches the supplied parameters (or all if none supplied)
post [string clientNamespace]/V1/PlanTargets
function post [string clientNamespace]/V1/PlanTargets(true isValidateOnly, PlanTarget payload) returns json|error
Return Type
- json|error - If isValidateOnly was passed in as true, this shows that the validation passed.
delete [string clientNamespace]/V1/PlanTargets
function delete [string clientNamespace]/V1/PlanTargets(string planTargetXRefCode) returns json|error
Deletes a plan target matching the supplied XRefCode
Parameters
- planTargetXRefCode string -
Return Type
- json|error - All matching plan targets were deleted
patch [string clientNamespace]/V1/PlanTargets
function patch [string clientNamespace]/V1/PlanTargets(string planTargetXRefCode, true isValidateOnly, PlanTarget payload) returns json|error
Amend a Plan Method Target record, plus any child table entries.
Parameters
- planTargetXRefCode string -
- isValidateOnly true - When a TRUE value is used in this parameter the PATCH operation validates the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload PlanTarget - The details of the
Return Type
- json|error - If isValidateOnly was passed in as true, this shows that the validation passed.
get [string clientNamespace]/V1/Positions
function get [string clientNamespace]/V1/Positions(string? contextDate) returns Payload_IEnumerable_Position|error
Get a list of Positions
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which Position data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2019-01-01T12:34:56
Return Type
- Payload_IEnumerable_Position|error - A collection of Position XRefCodes meeting the search criteria.
post [string clientNamespace]/V1/Positions
function post [string clientNamespace]/V1/Positions(true isValidateOnly, Position payload) returns json|error
POST (Add) a Position
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload Position - The JSON-formatted content containing the data entities and elements to be processed in POST (position add) and PATCH (position update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A Position is created, no response body.
get [string clientNamespace]/V1/Positions/[string xrefCode]
function get [string clientNamespace]/V1/Positions/[string xrefCode](string? contextDate, string? expand) returns Payload_Position|error
Get Position details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which Position data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2019-01-01T12:34:56
- expand string? (default ()) - This parameter accepts a comma-separated list of top-level entities that contain the data elements needed for downstream processing. When this parameter is not used, only data elements from the position master record will be included. For more information, please refer to the Introduction to Dayforce Web Services document.
Return Type
- Payload_Position|error - The Position with the requested XRefCode.
patch [string clientNamespace]/V1/Positions/[string xRefCode]
function patch [string clientNamespace]/V1/Positions/[string xRefCode](true isValidateOnly, Position payload) returns json|error
PATCH (Update) a Position
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying the updates to the database. The default value is FALSE if parameter is not specified.
- payload Position - The JSON-formatted content containing the data entities and elements to be processed in POST (position add) and PATCH (position update) operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - A Position is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/GLSplits
function get [string clientNamespace]/V1/Employees/[string xRefCode]/GLSplits(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_PRGLSplitSet|error
GET GLSplit Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_PRGLSplitSet|error - The GLSplit with the requested XRefCode.
get [string clientNamespace]/V1/Projects
function get [string clientNamespace]/V1/Projects(string? shortName, string? longName, Signed32? pageSize, string? projectClientXRefCode, string? projectTypeXRefCode, string? projectPhaseXRefCode, string? productGroupXRefCode, string? productModuleXRefCode, string? creationOrgUnitXRefCode, string? lastModifedStartDate, string? lastModifedEndDate, string? filterStartDateFrom, string? filterStartDateTo, string? filterDueDateFrom, string? filterDueDateTo, string? filterCompletedDateFrom, string? filterCompletedDateTo, Signed32? certifiedPayrollProjectNumber, string? parentProjectXRefCode, boolean? isDeleted, string? ledgerCode, string? clockTransferCode, string? accountNum, boolean? iFRSClassification, Signed32? filterProjectPriorityFrom, Signed32? filterProjectPriorityTo, decimal? filterBudgetHoursFrom, decimal? filterBudgetHoursTo, decimal? filterBudgetAmountFrom, decimal? filterBudgetAmountTo, decimal? filterPctCompleteFrom, decimal? filterPctCompleteTo) returns PaginatedPayload_IEnumerable_BaseProject|error
Get a list of client projects meeting the search criteria
Parameters
- shortName string? (default ()) - Project Name
- longName string? (default ()) - Project Description
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
- projectClientXRefCode string? (default ()) - This field identifies a unique Project Client
- projectTypeXRefCode string? (default ()) - This field identifies a unique Project Type
- projectPhaseXRefCode string? (default ()) - This field identifies a unique Project Phase
- productGroupXRefCode string? (default ()) - This field identifies a unique Product Group
- productModuleXRefCode string? (default ()) - This field identifies a unique Product Module
- creationOrgUnitXRefCode string? (default ()) - Use to search for projects based on Organizational units' xrefcode
- lastModifedStartDate string? (default ()) - The Start date used when searching for projects with updates in a specified timeframe
- lastModifedEndDate string? (default ()) - The End date used when searching for projects with updates in a specified timeframe
- filterStartDateFrom string? (default ()) - Use to search for projects with Start date values greater than or equal to the specified value
- filterStartDateTo string? (default ()) - Use to search for projects with Start date values less than or equal to the specified value
- filterDueDateFrom string? (default ()) - Use to search for projects with Due Date values greater than or equal to the specified value
- filterDueDateTo string? (default ()) - Use to search for projects with Due Date values less than or equal to the specified value
- filterCompletedDateFrom string? (default ()) - Use to search for projects with Completed Date values greater than or equal to the specified value
- filterCompletedDateTo string? (default ()) - Use to search for Projects with Completed Date values less than or equal to the specified value
- certifiedPayrollProjectNumber Signed32? (default ()) - Use to search for projects with certifiedPayrollProjectNumber
- parentProjectXRefCode string? (default ()) - Use to search for projects with immediate Parent Project
- isDeleted boolean? (default ()) - This filters the projects based on those are deleted or not
- ledgerCode string? (default ()) -
- clockTransferCode string? (default ()) - Use to search for Projects with Clock Code
- accountNum string? (default ()) -
- iFRSClassification boolean? (default ()) -
- filterProjectPriorityFrom Signed32? (default ()) - Use to search for projects with Project Priority value greater than or equal to the specified value. When a value is provided for this parameter filterProjectPriorityTo value must also be provided.
- filterProjectPriorityTo Signed32? (default ()) - Use to search for Projects with Project Priority values less than or equal to the specified value. When a value is provided for this parameter filterProjectPriorityFrom value must also be provided.
- filterBudgetHoursFrom decimal? (default ()) - Use to search for projects with Budget Hours value greater than or equal to the specified value. When a value is provided for this parameter filterBudgetHoursTo value must also be provided.
- filterBudgetHoursTo decimal? (default ()) - Use to search for Projects with Budget Hours values less than or equal to the specified value. When a value is provided for this parameter filterBudgetHoursFrom value must also be provided.
- filterBudgetAmountFrom decimal? (default ()) - Use to search for projects with Budget Amount value greater than or equal to the specified value. When a value is provided for this parameter filterBudgetAmountTo value must also be provided.
- filterBudgetAmountTo decimal? (default ()) - Use to search for Projects with Budget Amount values less than or equal to the specified value. When a value is provided for this parameter filterBudgetAmountFrom value must also be provided.
- filterPctCompleteFrom decimal? (default ()) - Use to search for projects with Percent Complete value greater than or equal to the specified value. When a value is provided for this parameter filterPctCompleteTo value must also be provided.
- filterPctCompleteTo decimal? (default ()) - Use to search for Projects with Percent Complete values less than or equal to the specified value. When a value is provided for this parameter filterPctCompleteFrom value must also be provided.
Return Type
- PaginatedPayload_IEnumerable_BaseProject|error - A collection of client project data meeting the search criteria.
post [string clientNamespace]/V1/Projects
function post [string clientNamespace]/V1/Projects(true isValidateOnly, ProjectForSubmit payload) returns ProjectsPostResponse|error
POST (Insert) new Projects data into Dayforce
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operations validate the request without applying updates to the database.
- payload ProjectForSubmit -
Return Type
- ProjectsPostResponse|error - New Projects data is inserted into Dayforce
patch [string clientNamespace]/V1/Projects
function patch [string clientNamespace]/V1/Projects(string projectXRefCode, true isValidateOnly, ProjectForSubmit payload) returns json|error
Patch (Update) a project
Parameters
- projectXRefCode string - Project XRefCode
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH operations validate the request without applying updates to the database.
- payload ProjectForSubmit - The JSON-formatted content containing the data entities and elements to be processed in PATCH operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - Project data is updated
get [string clientNamespace]/V1/Projects/[string xRefCode]
function get [string clientNamespace]/V1/Projects/[string xRefCode]() returns Payload_Projects|error
Get project details
Return Type
- Payload_Projects|error - Project details which matches with the XRefCode.
get [string clientNamespace]/V1/ReportMetadata
function get [string clientNamespace]/V1/ReportMetadata() returns Payload_IEnumerable_ReportMetadata|error
GET a List of Report Metadata
Return Type
- Payload_IEnumerable_ReportMetadata|error - Metadata for all Reports
get [string clientNamespace]/V1/ReportMetadata/[string xRefCode]
function get [string clientNamespace]/V1/ReportMetadata/[string xRefCode]() returns Payload_IEnumerable_ReportMetadata|error
GET Report Metadata for the given XRefCode
Return Type
- Payload_IEnumerable_ReportMetadata|error - The Metadata for the Report matching the requested XRefCode
get [string clientNamespace]/V1/Reports/[string xRefCode]
function get [string clientNamespace]/V1/Reports/[string xRefCode](Signed32? pageSize, record {}? reportParameters) returns PaginatedPayload_Report|error
GET Report
Parameters
- pageSize Signed32? (default ()) - The number of records returned per page in the paginated response
- reportParameters record {}? (default ()) - A list of key value pairs for those reports which take as input user supplied parameter values.
Return Type
- PaginatedPayload_Report|error - report that match the given request
get [string clientNamespace]/V1/Employees/[string employeeXRefCode]/UnfilledShiftBids
function get [string clientNamespace]/V1/Employees/[string employeeXRefCode]/UnfilledShiftBids(string orgUnitXRefCode, string scheduleStartDate, string scheduleEndDate) returns Payload_IEnumerable_EmployeeSchedule|error
Get List of Unfilled Shift Bids for Employee
Parameters
- orgUnitXRefCode string - The unique identifier (external reference code) of the organization to be retrieved. The value provided must be the exact match for an organization
- scheduleStartDate string - Date to represent the start of the schedule period for which Shift Bids should be retrieved
- scheduleEndDate string - Date to represent the end of the schedule period for which Shift Bids should be retrieved
Return Type
- Payload_IEnumerable_EmployeeSchedule|error - A Collection of Open Shift Bids.
post [string clientNamespace]/V1/Employees/[string employeeXRefCode]/UnfilledShiftBids
function post [string clientNamespace]/V1/Employees/[string employeeXRefCode]/UnfilledShiftBids(string orgUnitXRefCode, true isValidateOnly, EmployeeShiftBidDTO payload) returns Payload_IEnumerable_ProcessResult|error
POST (Create) Employee Shift Bids. Employee Request or Revoke Shift Bid Request.
Parameters
- orgUnitXRefCode string - The unique identifier (external reference code) of the organization to be retrieved. The value provided must be the exact match for an organization
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validates the request without applying updates to the database.
- payload EmployeeShiftBidDTO -
Return Type
- Payload_IEnumerable_ProcessResult|error - Specifies an employee bid request/revocation was successful.
get [string clientNamespace]/V1/Organization/[string orgUnitXRefCode]/UnfilledShiftBids
function get [string clientNamespace]/V1/Organization/[string orgUnitXRefCode]/UnfilledShiftBids(string scheduleStartDate, string scheduleEndDate, boolean? activeBids) returns Payload_IEnumerable_ManagerShiftBid|error
Get List of Shift Bids
Parameters
- scheduleStartDate string - Date to represent the start of the schedule period for which Shift Bids should be retrieved
- scheduleEndDate string - Date to represent the end of the schedule period for which Shift Bids should be retrieved
- activeBids boolean? (default ()) - Specifies whether to return bids with or without active employee bid requests. When no value is supplied, all shift bids will be returned.
Return Type
- Payload_IEnumerable_ManagerShiftBid|error - A Collection of Shift Bids
post [string clientNamespace]/V1/Organization/[string orgUnitXRefCode]/UnfilledShiftBid
function post [string clientNamespace]/V1/Organization/[string orgUnitXRefCode]/UnfilledShiftBid(true isValidateOnly, ManagerAssignShiftBidDTO[] payload, 0|1|2|3|4? violationLevel) returns Payload_IEnumerable_EmployeeSchedule|error
Manager assignment of shift to an employee who has bid for a specific group schedule. Notifies awarded bidder. Notifies rejected bidder.
Parameters
- isValidateOnly true -
- payload ManagerAssignShiftBidDTO[] -
- violationLevel 0|1|2|3|4? (default ()) -
Return Type
- Payload_IEnumerable_EmployeeSchedule|error - Assigns shift bid to bidding employee
get [string clientNamespace]/V1/Skills
function get [string clientNamespace]/V1/Skills(Signed32? pageSize, string? xRefCode) returns PaginatedPayload_IEnumerable_Skill|error
GET the list of all skills
Parameters
- pageSize Signed32? (default ()) - The page size for the pagination (Default is 1000)
- xRefCode string? (default ()) - The xrefcode filter for the skill
Return Type
- PaginatedPayload_IEnumerable_Skill|error - A collection of skills
post [string clientNamespace]/V1/Skills
function post [string clientNamespace]/V1/Skills(true isValidateOnly, Skill payload) returns Payload_IEnumerable_Skill|error
POST one skill
Return Type
- Payload_IEnumerable_Skill|error - The skill created
patch [string clientNamespace]/V1/Skills
function patch [string clientNamespace]/V1/Skills(string xRefCode, true isValidateOnly, Skill payload) returns Payload_IEnumerable_Skill|error
PATCH one skill
Parameters
- xRefCode string - The xRefCode of the skill to be updated
- isValidateOnly true -
- payload Skill - The skill payload used for skill modification
Return Type
- Payload_IEnumerable_Skill|error - The skill updated
get [string clientNamespace]/V1/Employees/[string xRefCode]/TimeAwayFromWork
function get [string clientNamespace]/V1/Employees/[string xRefCode]/TimeAwayFromWork(string filterTAFWStartDate, string filterTAFWEndDate, string status) returns Payload_IEnumerable_EmployeeTimeAwayFromWork|error
GET List of Employee Time Away From Work
Parameters
- filterTAFWStartDate string - Inclusive period start date to determine which employee time away from work data to retrieve. Format: YYYY-MM-DD
- filterTAFWEndDate string - Inclusive period end date to determine which employee time away from work data to retrieve. Format: YYYY-MM-DD
- status string - A case-sensitive field containing status for time away from work values. Examples: [APPROVED,PENDING,CANCELED,DENIED,CANCELPENDING]
Return Type
- Payload_IEnumerable_EmployeeTimeAwayFromWork|error - A collection of employee time away from work meeting the search criteria.
post [string clientNamespace]/V1/TimeOff
function post [string clientNamespace]/V1/TimeOff(true isValidateOnly, EmployeeTimeAwayFromWorkForSubmit payload) returns Payload_EmployeeTimeAwayFromWorkForSubmit|error
POST (Create) Employee Time Away From Work
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeTimeAwayFromWorkForSubmit - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- Payload_EmployeeTimeAwayFromWorkForSubmit|error - An Employee Time Away From Work is created.
patch [string clientNamespace]/V1/TimeOff/[string xRefCode]
function patch [string clientNamespace]/V1/TimeOff/[string xRefCode](true isValidateOnly, EmployeeTimeAwayFromWorkForSubmit payload) returns Payload_IEnumerable_EmployeeTimeAwayFromWorkForSubmit|error
PATCH (Update) Employee Time Away From Work
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeTimeAwayFromWorkForSubmit - The JSON-formatted content containing the data entities and elements to be processed in POST operation. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- Payload_IEnumerable_EmployeeTimeAwayFromWorkForSubmit|error - An Employee Time Away From Work is updated.
get [string clientNamespace]/V1/TrainingPrograms
function get [string clientNamespace]/V1/TrainingPrograms(Signed32? pageSize, string? xRefCode) returns PaginatedPayload_IEnumerable_TrainingProgram|error
Get a list of or one Training Program detail
Parameters
- pageSize Signed32? (default ()) - The page size for the pagination (Default is 1000)
- xRefCode string? (default ()) - The xrefcode filter for the Training Program
Return Type
- PaginatedPayload_IEnumerable_TrainingProgram|error - A collection of Training Program
post [string clientNamespace]/V1/TrainingPrograms
function post [string clientNamespace]/V1/TrainingPrograms(true isValidateOnly, TrainingProgram payload) returns json|error
POST one training program
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST operation validate the request without applying the changes to the database.
- payload TrainingProgram - The training program payload that will be used for training program creation
Return Type
- json|error - A training program is created, no response body
patch [string clientNamespace]/V1/TrainingPrograms
function patch [string clientNamespace]/V1/TrainingPrograms(string xRefCode, true isValidateOnly, TrainingProgram payload) returns json|error
PATCH one training program
Parameters
- xRefCode string - The course xRefCode to be updated
- isValidateOnly true - When a TRUE value is used in this parameter, PATCH operation validate the request without applying the changes to the database.
- payload TrainingProgram - The training program payload that will be used for training program creation
Return Type
- json|error - A training program is updated, no response body
post [string clientNamespace]/V1/TransmissionService
function post [string clientNamespace]/V1/TransmissionService() returns json|error
Return Type
- json|error - OK
get [string clientNamespace]/V1/Employees/[string xRefCode]/UserPayAdjustCodeGroups
function get [string clientNamespace]/V1/Employees/[string xRefCode]/UserPayAdjustCodeGroups() returns Payload_IEnumerable_UserPayAdjustCodeGroup|error
GET UserPayAdjCodeGroup Details
Return Type
- Payload_IEnumerable_UserPayAdjustCodeGroup|error - The UserPayAdjCodeGroup with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/UserPayAdjustCodeGroups
function post [string clientNamespace]/V1/Employees/[string xRefCode]/UserPayAdjustCodeGroups(true isValidateOnly, UserPayAdjustCodeGroup payload) returns json|error
POST a UserPayAdjCodeGroup
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload UserPayAdjustCodeGroup - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The UserPayAdjCodeGroup is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/UserPayAdjustCodeGroups
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/UserPayAdjustCodeGroups(true isValidateOnly, UserPayAdjustCodeGroup payload) returns json|error
PATCH a UserPayAdjCodeGroup
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload UserPayAdjustCodeGroup - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The UserPayAdjCodeGroup is updated, no response body.
get [string clientNamespace]/V1/Employees/[string xRefCode]/WorkContracts
function get [string clientNamespace]/V1/Employees/[string xRefCode]/WorkContracts(string? contextDate, string? contextDateRangeFrom, string? contextDateRangeTo) returns Payload_IEnumerable_EmployeeWorkContract|error
GET EmployeeWorkContract Details
Parameters
- contextDate string? (default ()) - The Context Date value is an “as-of” date used to determine which employee data to search when records have specific start and end dates. The service defaults to the current datetime if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeFrom string? (default ()) - The Context Date Range From value is the start of the range of dates used to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
- contextDateRangeTo string? (default ()) - The Context Date Range To value is the end of the range of dates to determine which employee data to search when records have specific start and end dates. The service defaults to null if the requester does not specify a value. Example: 2017-01-01T13:24:56
Return Type
- Payload_IEnumerable_EmployeeWorkContract|error - The EmployeeWorkContract with the requested XRefCode.
post [string clientNamespace]/V1/Employees/[string xRefCode]/WorkContracts
function post [string clientNamespace]/V1/Employees/[string xRefCode]/WorkContracts(true isValidateOnly, EmployeeWorkContract payload) returns json|error
POST a EmployeeWorkContract
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeWorkContract - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - An EmployeeWorkContract is created, no response body.
patch [string clientNamespace]/V1/Employees/[string xRefCode]/WorkContracts
function patch [string clientNamespace]/V1/Employees/[string xRefCode]/WorkContracts(true isValidateOnly, EmployeeWorkContract payload) returns json|error
PATCH a EmployeeWorkContract
Parameters
- isValidateOnly true - When a TRUE value is used in this parameter, POST and PATCH operations validate the request without applying updates to the database.
- payload EmployeeWorkContract - The JSON-formatted content containing the data entities and elements to be processed in POST and PATCH operations. For more information and examples, please refer to the Dayforce Web Services RESTful Developer's Guide.
Return Type
- json|error - The EmployeeWorkContract is updated, no response body.
Records
dayforce: AccidentInsuranceHazardCategory
Fields
- PRDEUAccidentInsuranceProvider_BBNR_UV string? -
- CategoryNumber string? -
- Percentage Signed32? -
dayforce: AccumulationType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Activity
Fields
- TimeStart string? -
- TimeEnd string? -
- XRefCode string? -
dayforce: AdditionalTaxType
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: Address
Fields
- Address1 string? -
- Address2 string? -
- Address3 string? -
- Address4 string? -
- Address5 string? -
- Address6 string? -
- City string? -
- PostalCode string? -
- Country Country? -
- State State? -
- LastModifiedTimestamp string? -
dayforce: AmfAuthorize
Fields
- Tags string[]? -
dayforce: AmfCfg
Fields
- Type string? -
- Model string? -
- Module string? -
- AppView string? -
dayforce: AmfEntity
Fields
- Id Signed32? -
- EffectiveStart string? -
- EffectiveEnd string? -
dayforce: AmfEnumFrom
Fields
- Criteria record {}? -
- Model string? -
- Module string? -
- AppView string? -
dayforce: AmfOnCUDActionModel
Fields
- Type string? -
- Action 0|1|2 ? -
- Location string? -
- Value record {}? -
- Params record {}? -
dayforce: AmfProperty
Fields
- Id Signed32? -
- EntityId Signed32? -
- ParentId Signed32? -
- EntityState string? -
- StringValue string? -
- BoolValue boolean? -
- NumberValue decimal? -
- DateTimeValue string? -
dayforce: AmfRuleModel
Fields
- WorkflowName string? -
- FunctionName string? -
- Parameters AmfRuleParameterModel[]? -
dayforce: AmfRuleParameterModel
Fields
- AccessType string? -
- DataType string? -
- Value record {}? -
- InputName string? -
- InnerParameter AmfRuleParameterModel? -
dayforce: AmfValidationModel
Fields
- Type string? -
- Value record {}? -
dayforce: AnalyticsDatasetMetadata
Fields
- DatasetId string? - Dataset Id.
- Status string? -
- RowCount string? - Row count in the dataset.
- ReportId Signed32? - Report Id.
- RefreshStatus string? - RefreshStatus.
- Filters string? - Report Filters
- Parameters string? - Report Parameters
- CreatedDate string? - Created Date of report
- LastModifiedTimestamp string? - Last modified timestamp of report
- StartedTimestamp string? - Started refresh Timestamp
- EndedTimestamp string? - Ended refresh Timestamp
- DifferenceCount Signed32? - Difference between report row counts
- TotalPages Signed32? - Total number of pages for given dataset
dayforce: AnalyticsDatasetMetadataResponse
Fields
- Message string? - Message returned to user
- DatasetId string? - Dataset Id.
- Status string? -
- RowCount string? - Row count in the dataset.
- ReportId Signed32? - Report Id.
- RefreshStatus string? - RefreshStatus.
- Filters string? - Report Filters
- Parameters string? - Report Parameters
- CreatedDate string? - Created Date of report
- LastModifiedTimestamp string? - Last modified timestamp of report
- StartedTimestamp string? - Started refresh Timestamp
- EndedTimestamp string? - Ended refresh Timestamp
- DifferenceCount Signed32? - Difference between report row counts
- TotalPages Signed32? - Total number of pages for given dataset
dayforce: AnalyticsListValue
Fields
- ListValueId Signed32? - The Id of the list item. This value would be passed as the parameter value. Multiple Id's can be passed as a parameter value if comma separated.
- Name string? - The name that can be displayed in a list to the user.
dayforce: AnalyticsReportColumnMetadata
This class encapsulates the report data generated during the execution of the report.
Fields
- Name string? - CodeName is a unique name that can be used with code to refer to this specific column. Its value should be consistent.
- Description string? - This is a name as defined by a user when developing this report. This name would be a human facing name that would be used on a printed report. This field is used as the key to the values dictionary
- DataType string? - This is a string representation of the data type.
dayforce: AnalyticsReportDataset
Fields
- Dataset record {}? -
dayforce: AnalyticsReportDefinitions
Fields
- ReportId string? - Feature Id for the Report.
- Name string? - Name of the Report. The name will be localized, based on the Session Culture.
- Description string? - Description of the Report. The description will be localized, based on the Session Culture.
dayforce: AnalyticsReportFilterMetadata
Report meta data that defines report parameters. Parameter values are supplied by the end-user for execution. All parameters must be supplied during execution. Not all Parameters have default value.
Fields
- Name string? - Name of the parameter.
- Description string? - Description of the Parameter. The description will be localized, based on the Session Culture.
- DataType string? - Data type of the parameter.
- Operator string? - Operator type of the parameter, such as =, <, >=, etc... Not all parameters have operator type. For example, SQL Parameters don't have operators.
- IsRequired boolean? - True if a value needs to be supplied.
- Value string? - Value that will be used. Reference values should contain Ids and be comma separated. E.g "1,2,3"
- Editable boolean? - True if the operator is editable
- Sequence Signed32? - Sequence of the filter
- AvailableValues AnalyticsListValue[]? - List of available values a parameter can have.
dayforce: AnalyticsReportMetadata
Fields
- Name string? - Name of the Report. The name will be localized, based on the Session Culture.
- Description string? - Description of the Report. The description will be localized, based on the Session Culture.
- XRefCode string? - Reference Code for the Report. This is a unique, human readable, code used to identify the Report.
- ReportId Signed32? - FeatureId of the report
- MaxRows Signed32? - Maximum number of rows the Report will return as part of it's ResultSet.
- OnlyIncludeUniqueRecords boolean? -
- Columns AnalyticsReportColumnMetadata[]? - An array of ReportColumnMetadata objects.
- Filters AnalyticsReportFilterMetadata[]? - An array of AnalyticsParameterMetadata objects.
dayforce: ApplicantDetails
Fields
- FullName string? -
- FirstName string? -
- LastName string? -
- Email string? -
- UseEmailForLogin boolean? -
- PhoneNumber string? -
- HomePhoneNumber string? -
- CoverLetter string? -
- Resume ApplicantResume? -
- AdditionalDocuments FileDetails[]? -
dayforce: ApplicantResume
Fields
- Text string? -
- HrXml string? -
- Html string? -
- Json CandidateDetails? -
- File FileDetails? -
dayforce: AppUser
Fields
- AllowNativeAuthentication boolean? -
- Display24HourTime boolean? -
- IsApproved boolean? -
- LastModifiedTimestamp string? -
dayforce: AppUserSSOCollection
Fields
- Items EmployeeSSOAccount[]? -
dayforce: ArchiveDocument
Fields
- DocumentType string? -
- DocumentGroup string? -
- UserId Signed32? -
- SourceReportUniqueId string? -
- PublishDateTime string? -
- Title string? -
- PageCount Signed32? -
- CultureId Signed32? -
- Contents string? -
- Link string? -
- FileName string? -
- SizeBytes int? -
- ReportAlternateTitle string? -
- EmployeeXRefCode string? -
- IsAvailableOnCloud boolean? -
dayforce: AssignedSexComplianceCode
Fields
- ComplianceCode string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: AssignedSexCountryAware
Fields
- AssignedSexComplianceCode AssignedSexComplianceCode? -
- UserDefinedComplianceCode string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: AssociationDetails
Fields
- Title string? -
- StartDateMonth string? -
- StartDateYear string? -
- EndDateMonth string? -
- EndDateYear string? -
- EndCurrent boolean? -
- Description string? -
dayforce: Associations
Fields
- _Total Signed32? -
- Values AssociationDetails[]? -
dayforce: AttendancePolicy
Fields
- TrackingBasedOn Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: AuthorityType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: AuthorizationAssignment
Fields
- AuthorityType AuthorityType? -
- EffectiveStart string? -
- EffectiveEnd string? -
- AuthorizedPersonnelFirstName string? -
- AuthorizedPersonnelLastName string? -
- AuthorizedPersonnelMiddleName string? -
- AuthorizedPersonnelXrefCode string? -
- LastModifiedTimestamp string? -
dayforce: AuthorizationPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: AwardDetails
Fields
- Title string? -
- DateMonth string? -
- DateYear string? -
- Description string? -
dayforce: Awards
Fields
- _Total Signed32? -
- Values AwardDetails[]? -
dayforce: BackgroundJobLog
Fields
- BackgroundJobLogId Signed32? -
- BackgroundJobLogGuid string? -
- Name string? -
- CodeName string? -
- Status string? -
- WasScheduled boolean? -
- HasItemLevelErrors boolean? -
- QueueTimeUtc string? -
- ExecStartTimeUtc string? -
- ExecEndTimeUtc string? -
- SubmittedBy string? -
- Parameters BackgroundJobLogParameters[]? -
- JobInformation string? -
- ErrorInformation string? -
- DebugInformation string? -
- FileList string? -
- LastModifiedTimestampUtc string? -
dayforce: BackgroundJobLogParameters
Fields
- Name string? -
- Value string? -
dayforce: BackgroundScreeningAdjudicationStatus
Fields
- ScreeningRequestId string? -
- AdjudicationStatus string? -
dayforce: BackgroundScreeningAssociatedBillingCode
Fields
- BillingCodeId string? -
- Name string? -
- Description string? -
dayforce: BackgroundScreeningAssociatedPackage
Fields
- PackageId string? -
- Name string? -
- Description string? -
- IncludeRTW boolean? -
dayforce: BackgroundScreeningBillingCode
Fields
- ProviderReference string? -
- BillingCodes BackgroundScreeningProviderBillingCode[]? -
dayforce: BackgroundScreeningPackage
Fields
- ProviderReference string? -
- Packages BackgroundScreeningProviderPackage[]? -
dayforce: BackgroundScreeningProviderBillingCode
Fields
- BillingCodeId string? -
- Name string? -
- Description string? -
- AssociatedPackages BackgroundScreeningAssociatedPackage[]? -
dayforce: BackgroundScreeningProviderPackage
Fields
- PackageId string? -
- Name string? -
- Description string? -
- IncludeRTW boolean? -
- AssociatedBillingCodes BackgroundScreeningAssociatedBillingCode[]? -
dayforce: BackgroundScreeningProviderStatuses
Fields
- ProviderReference string? -
- OrderStatuses string[]? -
- AdjudicationStatuses string[]? -
- ResultStatuses string[]? -
dayforce: BackgroundScreeningReport
Fields
- ScreeningRequestId string? -
- CandidatePII CandidatePersonalIdentifiableInformation? -
dayforce: BackgroundScreeningReportScreeningStatus
Fields
- Status string? -
- AdjudicationStatus string? -
- Result string? -
dayforce: BackgroundScreeningStatus
Fields
- ScreeningRequestId string? -
- ScreeningStatus string? -
- ScreeningResult string? -
dayforce: BackgroundScreeningStatusUrlReport
Fields
- ScreeningRequestId string? -
- Url string? -
- ScreeningStatus BackgroundScreeningReportScreeningStatus? -
- CandidatePII CandidatePersonalIdentifiableInformation? -
- DetailedResults CandidateScreeningDetailedResult[]? -
- CandidateRightToWorkResults CandidateRightToWorkResult? -
dayforce: BankAccountBranchAddress
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: BaseEmployeeSubset
Fields
- XRefCode string? -
- NewXRefCode string? -
- CommonName string? -
- DisplayName string? -
- FirstName string? -
- LastName string? -
- Initials string? -
- MaidenName string? -
- MiddleName string? -
- PreferredLastName string? -
- SecondLastName string? -
- Suffix string? -
- Title string? -
- PreviousLastName string? -
- LastModifiedTimestamp string? -
dayforce: BaseProject
Fields
- ProjectXRefCode string? -
- ShortName string? -
- ParentProjectXRefCode string? -
- StartDate string? -
- DueDate string? -
dayforce: BenefitsDFLinkExportAddress
Fields
- RecordType string? -
- AddressType string? -
- AddressLine1 string? -
- AddressLine2 string? -
- AddressLine3 string? -
- City string? -
- County string? -
- StateCode string? -
- CountryCode string? -
- PostalCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
dayforce: BenefitsDFLinkExportBeneficiaryElectionDetail
Fields
- RecordType string? -
- UniqueBeneficiaryId Signed32? -
- PlanTypeCode string? -
- PlanSubTypeCode string? -
- PlanXrefCode string? -
- PlanCode string? -
- PolicyNumber string? -
- OptionCode string? -
- CoverageStartDate string? -
- CoverageEndDate string? -
- BeneficiaryPercentage decimal? -
- ContingentBeneficiaryIndicator boolean? -
- EffectiveStartDate string? -
- EffectiveEndDate string? -
- PlanStartDate string? -
dayforce: BenefitsDFLinkExportBeneficiaryRecord
Fields
- RecordType string? -
- UniqueBeneficiaryId Signed32? -
- EmployeeNationalId string? -
- NationalId string? -
- NationalIDExpiryDate string? -
- FirstName string? -
- MiddleName string? -
- LastName string? -
- DateOfBirth string? -
- GenderCode Signed32? -
- RelationshipCode string? -
- XRefCode string? -
- HomePhoneNumber string? -
- AddressDetails BenefitsDFLinkExportAddress[]? -
- ContactDetails BenefitsDFLinkExportContact[]? -
- BeneficiaryElectionDetails BenefitsDFLinkExportBeneficiaryElectionDetail[]? -
dayforce: BenefitsDFLinkExportCareProvider
Fields
- RecordType string? -
- ProviderIDQualifier string? -
- ProviderId string? -
- RelationshipCode string? -
- EntityIDCode string? -
dayforce: BenefitsDFLinkExportCarrierInfo
Fields
- RecordType string? -
- FileFormatMajorVersion string? -
- FileFormatMinorVersion string? -
- CTCReceiverId string? -
- CarrierName string? -
- CarrierFEINNumber string? -
- MasterPolicyNumber string? -
- EmployerName string? -
- EmployerFEINNumber string? -
- EmployerDivisionNumber string? -
- PlanStartDate string? -
- CreateDate string? -
- ClientId string? -
- CorrelationId string? -
dayforce: BenefitsDFLinkExportContact
Fields
- RecordType string? -
- ContactInformation string? -
- CountryCode string? -
- ContactInformationCategoryCode Signed32? -
- ContactInformationType string? -
dayforce: BenefitsDFLinkExportDependentElectionDetail
Fields
- RecordType string? -
- EmployeeElectionId Signed32? -
- UniqueDependentId Signed32? -
- PlanTypeCode string? -
- PlanSubTypeCode string? -
- PlanXrefCode string? -
- PlanCode string? -
- PolicyNumber string? -
- OptionCode string? -
- CoverageStartDate string? -
- CoverageEndDate string? -
- EffectiveStartDate string? -
- EffectiveEndDate string? -
- PlanStartDate string? -
- CareProviderDetails BenefitsDFLinkExportCareProvider[]? -
dayforce: BenefitsDFLinkExportDependentRecord
Fields
- RecordType string? -
- UniqueDependentId Signed32? -
- EmployeeNationalId string? -
- NationalId string? -
- NationalIDExpiryDate string? -
- FirstName string? -
- MiddleName string? -
- LastName string? -
- DateOfBirth string? -
- GenderCode Signed32? -
- RelationshipCode string? -
- MedicareStatusCode Signed32? -
- MedicareReasonCode Signed32? -
- StudentIndicator boolean? -
- DependentHandicapIndicator boolean? -
- DeathDate string? -
- EligibilityBeginDate string? -
- EligibilityEndDate string? -
- XRefCode string? -
- TobaccoSubstanceUse boolean? -
- DateLastSmoked string? -
- Height Signed32? -
- Weight Signed32? -
- InitialNoticeFlag boolean? -
- SocialSecurityDisabilityAwardDate string? -
- DependentAge Signed32? -
- AddressDetails BenefitsDFLinkExportAddress[]? -
- ContactDetails BenefitsDFLinkExportContact[]? -
- DependentElectionDetails BenefitsDFLinkExportDependentElectionDetail[]? -
dayforce: BenefitsDFLinkExportEarningGroupingCustomPeriodResults
Fields
- RecordType string? -
- LegalEntityXrefCode string? -
- EarningGroupingXrefCode string? -
- CustomPeriodXrefCode string? -
- Hours decimal? -
dayforce: BenefitsDFLinkExportEarningGroupingResults
Fields
- RecordType string? -
- LegalEntityReferenceCode string? -
- EarningGroupingReferenceCode string? -
- CurrentHours decimal? -
- MTDHours decimal? -
- QTDHours decimal? -
- YTDHours decimal? -
dayforce: BenefitsDFLinkExportEmployeeElectionDetail
Fields
- RecordType string? -
- ElectionId Signed32? -
- PlanTypeCode string? -
- PlanSubTypeCode string? -
- PlanXrefCode string? -
- PlanCode string? -
- PolicyNumber string? -
- OptionCode string? -
- CoverageStartDate string? -
- CoverageEndDate string? -
- EffectiveStartDate string? -
- EffectiveEndDate string? -
- EmployeePerPayCost decimal? -
- EmployeeDeductionScheduleCode string? -
- EmployeeAnnualCost decimal? -
- EmployerPerPayCost decimal? -
- EmployerDeductionScheduleCode string? -
- EmployerAnnualCost decimal? -
- CarrierPerPayCost decimal? -
- CarrierAnnualCost decimal? -
- EmployeeContributionAmount decimal? -
- EmployeeContributionPercentage decimal? -
- EmployerContributionAmount decimal? -
- EmployerContributionPercentage decimal? -
- CoverageAmount decimal? -
- CoverageMultiplier decimal? -
- RequestedCoverageAmount decimal? -
- PreAgeReducedCoverageAmount decimal? -
- EOIStatusCode Signed32? -
- RateXrefCode string? -
- PlanStartDate string? -
- ElectionDate string? -
- PlanEndDate string? -
- CareProviderDetails BenefitsDFLinkExportCareProvider[]? -
dayforce: BenefitsDFLinkExportEmployeeRecord
Fields
- RecordType string? -
- FirstName string? -
- MiddleName string? -
- LastName string? -
- NameSuffix string? -
- NationalId string? -
- NationalIDExpiryDate string? -
- XRefCode string? -
- EmployeeNumber string? -
- CultureCode string? -
- Citizenship string? -
- SeniorityDate string? -
- IsVisibleMinority boolean? -
- IsAboriginal boolean? -
- BadgeNumber string? -
- EmploymentStatus string? -
- EmploymentStatusReasonCode string? -
- EmploymentStatusStartDate string? -
- EmploymentStatusEndDate string? -
- NormalWeeklyHours decimal? -
- NormalSemiMonthlyHoursTop decimal? -
- NormalSemiMonthlyHoursBottom decimal? -
- AverageDailyHours decimal? -
- BaseSalary decimal? -
- BaseRate decimal? -
- PayGroupCode string? -
- EmployeeGroupCode string? -
- PayTypeCode string? -
- PayClassCode string? -
- DateOfBirth string? -
- GenderCode Signed32? -
- MaritalStatusCode string? -
- IncomeFrequency Signed32? -
- TobaccoSubstanceUse boolean? -
- DateLastSmoked string? -
- TobaccoUseEffectiveStart string? -
- TobaccoUseEffectiveEnd string? -
- Height Signed32? -
- Weight Signed32? -
- DisabilityCode boolean? -
- DisabilityBeginDate string? -
- DisabilityEndDate string? -
- DepartmentJobNameCode string? -
- WorkAssignmentEffectiveStart string? -
- WorkAssignmentEffectiveEnd string? -
- EmploymentIndicatorCode string? -
- VirtualIndicator boolean? -
- PositionLedgerCode string? -
- WorkedInState string? -
- ManagerFirstName string? -
- ManagerLastName string? -
- ManagerEmail string? -
- ManagerPhoneNumber string? -
- PayRunCheckDate string? -
- PayPeriodStartDate string? -
- PayPeriodEndDate string? -
- PayFrequencyCode Signed32? -
- Ethnicity string? -
- MedicareStatus Signed32? -
- MedicareReasonCode Signed32? -
- OriginalHireDate string? -
- TerminationDate string? -
- HireDate string? -
- DateOfDeath string? -
- InitialNoticeFlag boolean? -
- GenderIdentity string? -
- Age Signed32? -
dayforce: BenefitsDFLinkExportOrgUnit
Fields
- RecordType string? -
- OrgUnitName string? -
- OrgLevelCode string? -
- OrgUnitCode string? -
- LegalEntity string? -
- OrgUnitWorkSiteZipCode string? -
- OrgUnitLedgerCode string? -
dayforce: BenefitsDFLinkExportProperty
Fields
- RecordType string? -
- PropertyTypeCode string? -
- PropertyValue string? -
- PropertyValueTypeCode Signed32? -
- EffectiveStartDate string? -
- EffectiveEndDate string? -
dayforce: BenefitsDFLinkExportRecord
Fields
- EmployeeDetails BenefitsDFLinkExportEmployeeRecord? -
- AddressDetails BenefitsDFLinkExportAddress[]? -
- PropertyDetails BenefitsDFLinkExportProperty[]? -
- OrgUnitDetails BenefitsDFLinkExportOrgUnit[]? -
- UnionMembershipDetails BenefitsDFLinkExportUnionMembership[]? -
- ContactDetails BenefitsDFLinkExportContact[]? -
- EmployeeElectionDetails BenefitsDFLinkExportEmployeeElectionDetail[]? -
- DependentDetails BenefitsDFLinkExportDependentRecord[]? -
- BeneficiaryDetails BenefitsDFLinkExportBeneficiaryRecord[]? -
- EarningGroupingResults BenefitsDFLinkExportEarningGroupingResults[]? -
- EarningGroupingCustomPeriodResults BenefitsDFLinkExportEarningGroupingCustomPeriodResults[]? -
dayforce: BenefitsDFLinkExportUnionMembership
Fields
- RecordType string? -
- UnionName string? -
- UnionXrefCode string? -
- MembershipEffectiveStartDate string? -
- MembershipDate string? -
dayforce: BidderInfo
Fields
- EmployeeXRefCode string? -
- BaseRate decimal? -
- CurrentWeeklyHours decimal? -
- SeniorityDate string? -
dayforce: BioSensitivityLevel
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Breaks
Fields
- TimeStart string? -
- TimeEnd string? -
- NetHours decimal? -
- Type string? -
dayforce: BusinessUnit
Fields
- BusinessUnitGlobalId string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LedgerCode string? -
- BusinessUnitParents BusinessUnitParentAssignment[]? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: BusinessUnitParentAssignment
Fields
- BusinessUnitGlobalId string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: CalculationType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: CandidateApplicationStatusModel
Fields
- StatusName string? -
- StatusGroupName string? -
- EffectiveStart string? -
- EffectiveEnd string? -
dayforce: CandidateApplicationStatusUpdatesModel
Fields
- Identifier string? -
- ApplicationStatuses CandidateApplicationStatusModel[]? -
dayforce: CandidateApplicationStatusUpdatesPaginationModel
Fields
- HasUnretrievedStatuses string? -
- ApplicationStatusUpdates CandidateApplicationStatusUpdatesModel[]? -
dayforce: CandidateDetails
Fields
- FirstName string? -
- LastName string? -
- Headline string? -
- Summary string? -
- PublicProfileUrl string? -
- AdditionalInfo string? -
- PhoneNumber string? -
- HomePhoneNumber string? -
- Location CandidateLocation? -
- Skills string? -
- Positions Positions? -
- Educations Educations? -
- Links Links? -
- Awards Awards? -
- Certifications Certifications? -
- Associations Associations? -
- Patents Patents? -
- Publications Publications? -
- MilitaryServices MilitaryServices? -
dayforce: CandidateLocation
Fields
- City string? -
- PostalCode string? -
- Address1 string? -
- Address2 string? -
- Address3 string? -
- Country string? -
- StateCode string? -
- StateName string? -
- County string? -
dayforce: CandidatePersonalIdentifiableInformation
Fields
- DateOfBirth string? -
- SocialSecurityNumber string? -
- SocialInsuranceNumber string? -
- DriverLicense string? -
dayforce: CandidateProfileSource
Fields
- ShortName string? -
- CandidateProfileSourceAdditionalInfo string? -
dayforce: CandidateRightToWorkResult
Fields
- CandidateRightToWorkResultId Signed32? -
- WorkRightStatus string? -
- Status string? -
- DateIssued string? -
- PartnerIdentifier Signed32? -
- DateCompleted string? -
- WorkRightEffectiveStart string? -
- WorkRightEffectiveEnd string? -
- RejectionOrCancellationReasons CandidateRTWRejectionReason[]? -
- Documents CandidateRTWDocument[]? -
dayforce: CandidateRTWDocument
Fields
- CandidateRTWDocumentId Signed32? -
- DocumentName string? -
- DocumentExtension string? -
- DateAdded string? -
- DateExpire string? -
- Type string? -
- PathwayCode string? -
- CountryCode string? -
- Sources CandidateRTWDocumentSource? -
- Details CandidateRTWDocumentDetails[]? -
- DocumentErrors CandidateRTWDocumentGenericError[]? -
dayforce: CandidateRTWDocumentDetails
Fields
- Name string? -
- Value string? -
dayforce: CandidateRTWDocumentGenericError
Fields
- Code string? -
- Message string? -
dayforce: CandidateRTWDocumentSource
Fields
- SingleDocumentBase64 string? -
- DocumentFrontBase64 string? -
- DocumentBackBase64 string? -
dayforce: CandidateRTWRejectionReason
Fields
- ReasonCode string? -
- ReasonDescription string? -
dayforce: CandidateScreeningDetailedResult
Fields
- ReportIdentifier string? -
- ScreeningType string? -
- Status string? -
- Result string? -
- CompletionDate string? -
dayforce: CarrierFeeds
Fields
- Header BenefitsDFLinkExportCarrierInfo? -
- EmployeeRecords BenefitsDFLinkExportRecord[]? -
dayforce: CertificationDetails
Fields
- Title string? -
- StartDateMonth string? -
- StartDateYear string? -
- EndDateMonth string? -
- EndDateYear string? -
- EndCurrent boolean? -
- Description string? -
dayforce: Certifications
Fields
- _Total Signed32? -
- Values CertificationDetails[]? -
dayforce: ChildLocation
Fields
- OrgLevel OrgLevel? -
- OrgUnitLegalEntities OrgUnitLegalEntityCollection? -
- OrgUnitParents OrgUnitParentCollection? -
- OrgUnitLocationTypes OrgUnitLocationTypeCollection? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ChildLocationCollection
Fields
- Items ChildLocation[]? -
dayforce: CitizenshipType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: City
Fields
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
dayforce: ClientMetadata
Fields
- ServiceVersion string? -
- ServiceUri string? -
dayforce: ClientPayrollCountry
Fields
- ClientOperatingLocations string? -
- ClientOperatingLocationsLabel string? -
- ConnectPayEnabled boolean? -
- Countries PayrollCountry[]? -
dayforce: ClientPayrollCountryUpdate
Fields
- CountryCode string? -
- DefaultTimeZoneXRefCode string? -
- HCMPayrollCountry boolean? -
- ConnectedPayCountry boolean? -
dayforce: ClockDeviceGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ConfidentialIdentificationType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
dayforce: ContactInformationType
Fields
- ContactInformationTypeGroup ContactInformationTypeGroup? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ContactInformationTypeGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ContextLevel
Fields
- Level string? -
- LevelValue string? -
dayforce: Country
Fields
- Name string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Course
Fields
- CourseCode string? -
- CourseType CourseType? -
- CourseProvider CourseProvider? -
- EffectiveStart string? -
- EffectiveEnd string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: CourseProvider
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: CourseType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Culture
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DataEntry
Fields
- DataEntryXRefCode int? -
- EntryType string? -
- PayGroupXRefCode string? -
- Currency string? -
- PeriodStart string? -
- PeriodEnd string? -
- PPN string? -
- PayDate string? -
- EditSet string? -
- EmployeeXRefCode string? -
- EmployeeName string? -
- EmployeeNumber string? -
- Replace boolean? -
- CodeType string? -
- CodeXRefCode string? -
- CodeName string? -
- TaxAuthorityInstance string? -
- Hours decimal? -
- Rate decimal? -
- Amount decimal? -
- BusinessDate string? -
- JobAssignmentXRefCode string? -
- JobAssignmentName string? -
- WorkLocationXRefCode string? -
- WorkLocation string? -
- LegalEntityXrefCode string? -
- LegalEntityName string? -
- LegalEntityIdNumber string? -
- LaborPercent boolean? -
- DebitArrears boolean? -
- CheckTemplateXRefCode string? -
- CheckTemplateName string? -
- FLSAAdjustStartDate string? -
- FLSAAdjustEndDate string? -
- Message string? -
- ProjectXRefCode string? -
- ProjectName string? -
- DocketXRefCode string? -
- DocketName string? -
- PayPeriodNumber string? -
- OrderedAmountTypeXRefCode string? -
- OrderedAmountType string? -
- OrderPercent decimal? -
- LimitAmount decimal? -
- DisposableEarningAmount decimal? -
- PayPeriodsForTax Signed32? -
- PayOutAccurals boolean? -
- WorkersCompAccountNumber string? -
- WorkersCompAccountName string? -
- WorkersCompCode string? -
- DoNotDisburseToPayee boolean? -
- ImportSet string? -
- ApplyROEMapping boolean? -
- TrailingTaxationPeriodStart string? -
- TrailingTaxationPeriodEnd string? -
- LaborMetrics EmployeeLaborMetrics[]? -
- SavedBy string? -
- SavedAt string? -
- ImportIdentifier string? -
- SourceSystem string? -
- PayrollFrequencyType string? -
- PayrollFrequencyName string? -
- OffCyclePayRunXrefCode string? -
dayforce: DataEntryDeleteModel
Fields
- DataEntryXRefCode int? -
dayforce: Deduction
Fields
- CalculationType CalculationType? -
- DeductionCode DeductionCode? -
- DeductionType DeductionType? -
- DisplayName string? -
- DebitJournalNumber string? -
- CreditJournalNumber string? -
- IsDecliningBalance boolean? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DeductionCode
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DeductionCountry
Fields
- TaxComplianceName string? -
- TaxComplianceXRefCode string? -
- TaxTypeName string? -
- TaxTypeCode string? -
- CountryCode string? -
- CountryName string? -
dayforce: DeductionDefinition
Fields
- SystemDeduction boolean? -
- Active boolean? -
- AllowArrears boolean? -
- WorkersComp boolean? -
- Countries DeductionCountry[]? -
- Payees DeductionPayee[]? -
- Name string? -
- Description string? -
- DisplayName string? -
- XRefCode string? -
- AllowPayee boolean? -
- SourceTypeName string? -
- SourceTypeXRefCode string? -
- PayeeCategoryName string? -
- PayeeCategoryXRefCode string? -
dayforce: DeductionLimit
Fields
- LimitAccessType LimitAccessType? -
- AccumulationType AccumulationType? -
- LimitType LimitType? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DeductionParameter
Fields
- ParameterAccessType ParameterAccessType? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DeductionPayee
Fields
- PayeeParameters DeductionPayeeParameter[]? -
- PayeeName string? -
- PayeeXRefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- Default boolean? -
dayforce: DeductionPayeeParameter
Fields
- PayeeParameterName string? -
- PayeeParameterXRefCode string? -
- Value string? -
dayforce: DeductionType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Department
Fields
- LedgerCode string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DEUEmployeeAccidentInsurance
Fields
- LegalEntityXrefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- IsExempt boolean? -
- LastModifiedTimestamp string? -
- AccidentInsuranceHazardCategory DEUEmployeeAccidentInsuranceHazardCategoryCollection? -
dayforce: DEUEmployeeAccidentInsuranceCollection
Fields
- Items DEUEmployeeAccidentInsurance[]? -
dayforce: DEUEmployeeAccidentInsuranceHazardCategoryCollection
Fields
- Items AccidentInsuranceHazardCategory[]? -
dayforce: DEUEmployeeWageTax
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- ReferenceDate string? -
- EmploymentTypeCodeName string? -
- ElectronicDataTransfer boolean? -
- TaxClass string? -
- Factor decimal? -
- ChildAllowance decimal? -
- DenominationCodeName string? -
- TaxExemption boolean? -
- RequestedAnnualAllowance decimal? -
- AnnualAllowance decimal? -
- MonthlyAllowance decimal? -
- SpouseDenominationCodeName string? -
- OptOutYearEndWageTaxAdjustment boolean? -
- IsManualInput boolean? -
- LastModifiedTimestamp string? -
dayforce: DEUEmployeeWageTaxCollection
Fields
- Items DEUEmployeeWageTax[]? -
dayforce: DEUHoursChangeReason
Fields
- ITSGCode string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DEUSTWParticipationReason
Fields
- DEUSTWParticipationReasonId Signed32? -
- ShortName string? -
- LongName string? -
- XRefCode string? -
- LastModifiedTimestamp string? -
dayforce: DEUTaxSocialInsurance
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- PublicHealthInsuranceXrefCode string? -
- LevyHealthInsuranceProviderXrefCode string? -
- HighestSchoolEducationXrefCode string? -
- HighestProfessionalEducationXrefCode string? -
- RelationToEmployerXrefCode string? -
- IsContractor boolean? -
- MainlySelfEmployed boolean? -
- IsParentLegalGuardian boolean? -
- NumberOfEntitledChildren string? -
- ContractTypeXrefCode string? -
- LevyXrefCode string? -
- IsMidijob boolean? -
- IsSeasonalEmployee boolean? -
- EmployeeGroupXrefCode string? -
- HealthInsuranceXrefCode string? -
- PensionInsuranceXrefCode string? -
- UnemploymentInsuranceXrefCode string? -
- CareInsuranceXrefCode string? -
- CalculationBasisXrefCode string? -
- EntitledToSickBenefit boolean? -
- EmployeeOccupationalPension EmployeeOccupationalPension? -
- EmployeePrivateHealthInsurance EmployeePrivateHealthInsurance? -
- MainEmploymentWithMultipleMiniJobs boolean? -
- LastModifiedTimestamp string? -
dayforce: DFEthnicity
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DFUnion
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DFVeteransStatus
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DisabilityEvidenceIssuingAgency
Fields
- DisabilityEvidenceIssuingAgencyId Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DisabilityEvidenceType
Fields
- DisabilityEvidenceTypeId Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DisabilityGroup
Fields
- DisabilityGroupId Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DisabilityWorkingTime
Fields
- DisabilityWorkingTimeId Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Docket
Fields
- LedgerCode string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DocMgmtFileProcessedResponse
Fields
- Index Signed32? -
- DocumentGUID string? -
- UploadStatus string? -
- Message string? -
dayforce: DocMgmtSecurityGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: DocMgmtSecurityGroupUserMapCollection
Fields
- Items EmployeeDocumentManagementSecurityGroup[]? -
dayforce: DocMgmtUploadResponse
Fields
- Status string? -
- FilesProcessed DocMgmtFileProcessedResponse[]? -
dayforce: Document
Fields
- DocumentGUID string? -
- DocumentName string? -
- DocumentType DocumentType? -
- FileName string? -
- UploadedDate string? -
- UploadedBy UploadedBy? -
dayforce: DocumentImportQueueRequest
Fields
- FileName string? -
- Link string? -
- DocumentTypeXRefCode string? -
- EntityTypeXRefCode string? -
- Entity string? -
- EmployeeNumber string? -
- Tags string[]? -
- Comment string? -
- AdditionalData string? -
- EmployeeXRefCode string? -
dayforce: DocumentImportQueueResponse
Fields
- Status string? -
- JobGuid string? -
- QueueTimestamp string? -
- DocumentsQueued Signed32[]? -
- ProcessResults ProcessResult[]? -
dayforce: DocumentType
Fields
- ShortName string? -
- Description string? -
- XRefCode string? -
dayforce: Earning
Fields
- CalculationType CalculationType? -
- CreditJournalNumber string? -
- DebitJournalNumber string? -
- DisplayName string? -
- EarningCode EarningCode? -
- IsDecliningBalance boolean? -
- IsGenerated boolean? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EarningCode
Fields
- EarningType EarningType? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EarningCountry
Fields
- TaxComplianceName string? -
- TaxComplianceXRefCode string? -
- EarningTypeName string? -
- EarningTypeXRefCode string? -
- CountryCode string? -
- CountryName string? -
dayforce: EarningDefinition
Fields
- PayEntryRates boolean? -
- Generated boolean? -
- GrossedUp boolean? -
- EIRefund boolean? -
- PRPensionPaymentType string? -
- PREarningBenefitCategory string? -
- SeparateCheckName string? -
- SeparateCheckXRefCode string? -
- Countries EarningCountry[]? -
- Payees EarningPayee[]? -
- Name string? -
- Description string? -
- DisplayName string? -
- XRefCode string? -
- AllowPayee boolean? -
- SourceTypeName string? -
- SourceTypeXRefCode string? -
- PayeeCategoryName string? -
- PayeeCategoryXRefCode string? -
dayforce: EarningLimit
Fields
- LimitAccessType LimitAccessType? -
- AccumulationType AccumulationType? -
- LimitType LimitType? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EarningParameter
Fields
- CodeName string? -
- ParameterAccessType ParameterAccessType? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EarningPayee
Fields
- EarningScheduleName string? -
- EarningScheduleXRefCode string? -
- PayeeParameters EarningPayeeParameter[]? -
- PayeeEarningParameters EarningPayeeEarningParameter[]? -
- PayeeEarningLimits EarningPayeeEarningLimit[]? -
- PayeeName string? -
- PayeeXRefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- Default boolean? -
dayforce: EarningPayeeEarningLimit
Fields
- LimitAmount decimal? -
- LimitPercent decimal? -
- LimitTypeXRefCode string? -
- LimitTypeName string? -
- EarningLimitXRefCode string? -
dayforce: EarningPayeeEarningParameter
Fields
- ElementParamName string? -
- ElementParamCodeName string? -
- Value decimal? -
dayforce: EarningPayeeParameter
Fields
- PayeeParameterName string? -
- PayeeParameterXRefCode string? -
- Value string? -
dayforce: EarningStatementDocument
Fields
- Contents string? -
- Title string? -
- Link string? -
- FileName string? -
dayforce: EarningStatementHeader
Fields
- EarningStatementHeaders string? -
dayforce: EarningType
Fields
- CodeName string? -
- IsGLAllocatedDebit boolean? -
- IsGLAllocatedCredit boolean? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EducationDetails
Fields
- Degree string? -
- Field string? -
- School string? -
- Location string? -
- StartDateYear string? -
- EndDateYear string? -
- EndCurrent boolean? -
dayforce: Educations
Fields
- _Total Signed32? -
- Values EducationDetails[]? -
dayforce: ElectionSchedule
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmergencyContactCollection
Fields
- Items EmployeeEmergencyContact[]? -
dayforce: EmergencyContactPersonAddress
Fields
- Address1 string? -
- Address2 string? -
- Address3 string? -
- Address4 string? -
- Address5 string? -
- Address6 string? -
- County string? -
- City string? -
- PostalCode string? -
- Country Country? -
- State State? -
- EffectiveStart string? -
- EffectiveEnd string? -
- ContactInformationType ContactInformationType? -
- IsPayrollMailing boolean? -
- DisplayOnTaxForm boolean? -
- DisplayOnEarningStatement boolean? -
- LastModifiedTimestamp string? -
dayforce: EmergencyContactPersonAddressCollection
Fields
- Items EmergencyContactPersonAddress[]? -
dayforce: EmergencyContactPersonContact
Fields
- ContactNumber string? -
- Country Country? -
- ElectronicAddress string? -
- Extension string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- ContactInformationType ContactInformationType? -
- IsForSystemCommunications boolean? -
- IsPreferredContactMethod boolean? -
- IsUnlistedNumber boolean? -
- FormattedNumber string? -
- IsVerified boolean? -
- IsRejected boolean? -
- ShowRejectedWarning boolean? -
- NumberOfVerificationRequests Signed32? -
- LastModifiedTimestamp string? -
dayforce: EmergencyContactPersonContactCollection
Fields
- Items EmergencyContactPersonContact[]? -
dayforce: Employee
Fields
- AvatarUri string? -
- BadgeNumber string? -
- BioExempt boolean? -
- BirthDate string? -
- BirthCountry string? -
- BirthState string? -
- BirthCity string? -
- ChecksumTimestamp string? -
- ClockSupervisor boolean? -
- COBRANotificationSentDate string? -
- COBRANotificationStatus Signed32? -
- Culture Culture? -
- DateOfDeath string? -
- EligibleForRehire string? -
- EmployeePin string? -
- EntitlementOverrideDate string? -
- EstimatedReturnDate string? -
- ExportDate string? -
- FederatedId string? -
- Gender string? -
- HireDate string? -
- HomePhone string? -
- IsAboriginal string? -
- IsVisibleMinority string? -
- EligibleForOnDemandPay boolean? -
- EligibleForDFWalletPayCard boolean? -
- EmployeeLatestUpdatedTimestamp string? -
- LastPayrollNewHireExportDate string? -
- Nationality string? -
- NewHireApprovalDate string? -
- NewHireApproved boolean? -
- NewHireApprovedBy string? -
- OriginalHireDate string? -
- PhotoExempt boolean? -
- PPACAOverrideDate string? -
- RegisteredDisabled string? -
- RequiresExitInterview boolean? -
- SeniorityDate string? -
- SocialSecurityNumber string? -
- SSNCountryCode string? -
- SSNExpiryDate string? -
- StartDate string? -
- TaxExempt boolean? -
- TaxpayerId string? -
- TerminationDate string? -
- VeteranSeparationDate string? -
- FirstTimeAccessEmailSentCount Signed32? -
- FirstTimeAccessVerificationAttempts Signed32? -
- SendFirstTimeAccessEmail boolean? -
- EmployeeBadge EmployeeBadge? -
- Badges EmployeeBadgeCollection? -
- PreStartDate string? -
- PayrollKey string? -
- LoginId string? -
- HomeOrganization Location? -
- EmployeeNumber string? -
- EmploymentStatuses EmployeeEmploymentStatusCollection? -
- WorkAssignments EmployeeWorkAssignmentCollection? -
- Contacts PersonContactCollection? -
- Addresses PersonAddressCollection? -
- Roles EmployeeRoleCollection? -
- EmployeeManagers EmployeeManagerCollection? -
- EmployeeWorkAssignmentManagers EmployeeWorkAssignmentManagerCollection? -
- CompensationSummary EmployeeCompensationCollection? -
- SSOAccounts AppUserSSOCollection? -
- MaritalStatuses EmployeeMaritalStatusCollection? -
- Locations EmployeeLocationCollection? -
- BioSensitivityLevel BioSensitivityLevel? -
- CitizenshipType CitizenshipType? -
- SchoolYear SchoolYear? -
- HealthWellnessDetails EmployeeHealthWellnessCollection? -
- Courses EmployeeCourseCollection? -
- VolunteerLists EmployeeVolunteerListCollection? -
- HRIncidents EmployeeHRIncidentCollection? -
- Skills EmployeeSkillCollection? -
- TrainingPrograms EmployeeTrainingProgramCollection? -
- UnionMemberships EmployeeUnionCollection? -
- EmploymentTypes EmployeeEmploymentTypeCollection? -
- HighlyCompensatedEmployees HighlyCompensatedEmployeeCollection? -
- PayGradeRates EmployeePayGradeRateCollection? -
- DirectDeposits EmployeeDirectDepositCollection? -
- IRLTaxPAYEExclusionInfo EmployeeIRLTaxPAYEExclusionCollection? -
- IRLTaxPRSIInfo EmployeeIRLTaxPRSICollection? -
- IRLTaxRPNInfo EmployeeIRLTaxRPNCollection? -
- IRLTaxEWSSInfo EmployeeIRLTaxEWSSCollection? -
- CANFederalTaxes EmployeeCANFederalTaxCollection? -
- CANStateTaxes EmployeeCANStateTaxCollection? -
- USFederalTaxes EmployeeUSFederalTaxCollection? -
- MUSTaxes EmployeeMUSTaxDetailsCollection? -
- AUSFederalTaxes EmployeeAUSFederalTaxCollection? -
- EmployeePayrollTaxes EmployeePayrollTaxCollection? -
- EmployeePayrollTaxParameters EmployeePayrollTaxParameterCollection? -
- USStateTaxes EmployeeUSStateTaxCollection? -
- EmergencyContacts EmergencyContactCollection? -
- Ethnicities EmployeeEthnicityCollection? -
- Disabilities EmployeeDisabilityCollection? -
- VeteranStatuses EmployeeVeteransStatusCollection? -
- GLSplits PRGLSplitSetCollection? -
- EmployeeProperties EmployeePropertyValueCollection? -
- GlobalProperties EmployeeGlobalPropertyValueCollection? -
- LaborDefaults EmployeeDefaultLaborCollection? -
- DocumentManagementSecurityGroups DocMgmtSecurityGroupUserMapCollection? -
- ClockDeviceGroups EmployeeClockDeviceGroupCollection? -
- EmployeePayAdjustCodeGroups EmployeePayAdjCodeGroupListCollection? -
- UserPayAdjustCodeGroups UserPayAdjCodeGroupCollection? -
- WorkContracts EmployeeWorkContractCollection? -
- PerformanceRatings EmployeePerformanceRatingCollection? -
- EIRates EmployeeEIRateCollection? -
- USTaxStatuses EmployeeUSTaxStatusCollection? -
- CANTaxStatuses EmployeeCANTaxStatusCollection? -
- OrgUnitInfos EmployeeOrgUnitInfoCollection? -
- ManagedEmployees EmployeeWorkAssignmentManagerCollection? -
- ConfidentialIdentification EmployeeConfidentialIdentificationCollection? -
- EmployeeCertifications LMSEmployeeCertificationCollection? -
- EmploymentAgreements EmployeeEmploymentAgreementCollection? -
- HRPolicies EmployeeHRPolicyCollection? -
- AuthorizationAssignments UserEmployeeAuthorityTypeAssignmentCollection? -
- GenderIdentity GenderIdentity? -
- EmployeeAssignedSexAndGenderIdentities EmployeeAssignedSexAndGenderIdentityCollection? -
- OnboardingPolicies EmployeeOnboardingPolicyCollection? -
- PayPeriodInformation PayPeriodInformationCollection? -
- EarningElections EmployeeEarningsCollection? -
- DeductionElections EmployeeDeductionsCollection? -
- LastActiveManagers EmployeeManagerCollection? -
- DependentsBeneficiaries EmployeeDependentsBeneficiariesCollection? -
- UKTaxDetails EmployeeUKTaxDetailsCollection? -
- UKStudentLoan EmployeeUKStudentLoanCollection? -
- UKPostgraduateLoan EmployeeUKPostgraduateLoanCollection? -
- EmployeeUKIrregularPaymentDetails EmployeeUKIrregularPaymentDetailsCollection? -
- ContinuousEmploymentStartDate string? -
- LSLEligibilityDate string? -
- UKNIDetails EmployeeUKNIDetailsCollection? -
- UserAccount AppUser? -
- LastNamePrefix LastNamePrefix? -
- LastNamePrefixAtBirth LastNamePrefix? -
- NameAffix NameAffix? -
- NameAffixAtBirth NameAffix? -
- ProfessionalTitle string? -
- AUSSuperannuation EmployeeAUSSuperannuationCollection? -
- AUSSuperannuationRules EmployeeAUSSuperannuationRulesCollection? -
- TerminationNoticeDate string? -
- RetirementRequestDate string? -
- EmployeeShortTimeWork EmployeeShortTimeWorkCollection? -
- DEUTaxSocialInsurance EmployeeDEUTaxSocialInsuranceCollection? -
- DEUEmployeeAccidentInsurance DEUEmployeeAccidentInsuranceCollection? -
- DEUEmployeeWageTax DEUEmployeeWageTaxCollection? -
- NZLKiwiSaver EmployeeNZLKiwiSaver[]? -
- UnknownBirthDate boolean? -
- XRefCode string? -
- NewXRefCode string? -
- CommonName string? -
- DisplayName string? -
- FirstName string? -
- LastName string? -
- Initials string? -
- MaidenName string? -
- MiddleName string? -
- PreferredLastName string? -
- SecondLastName string? -
- Suffix string? -
- Title string? -
- PreviousLastName string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeAddress
Fields
- AddressLine1 string? -
- AddressLine2 string? -
- City string? -
- State string? -
- Country string? -
- PostalCode string? -
dayforce: EmployeeAssignedSexAndGenderIdentity
Fields
- Country Country? -
- State State? -
- AssignedSexCountryAware AssignedSexCountryAware? -
- GenderIdentityCountryAware GenderIdentityCountryAware? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeAssignedSexAndGenderIdentityCollection
Fields
- Items EmployeeAssignedSexAndGenderIdentity[]? -
dayforce: EmployeeAUSFederalTax
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- STSLDebt boolean? -
- ClaimTaxFreeThreshold boolean? -
- AmountClaimedforTaxOffset decimal? -
- MedicareLevyReductionClaimed boolean? -
- Spouse boolean? -
- CombineWeeklyTotalIncomelessthanTableA boolean? -
- NumberofDependentChildren Signed32? -
- SeniorAndPensionersTaxOffset boolean? -
- PayBasis string? -
- ClaimingZoneOverseasorCarerOffset boolean? -
- ApplyMedicareForWorkingHolidayMaker boolean? -
- WithholdingVariationAmount decimal? -
- DoesWithholdingVariationIncludeSTSLDebt boolean? -
- IsUpwardVariation boolean? -
- TFNExemptionReason TFNExemptionReason? -
- ResidentCode PRTaxResidentCode? -
- MedicareLevyExemptionType MedicareLevyExemptionType? -
- WithholdingVariationType WithholdingVariationType? -
- WithholdingVariationAmountType WithholdingVariationAmountType? -
- EarningGroup PREarningAccumulator? -
- AdditionalTaxType AdditionalTaxType? -
- FlatComissionerInstalmentRate decimal? -
- IsContractorSuperLiabilityOnly boolean? -
- LastModifiedTimestamp string? -
dayforce: EmployeeAUSFederalTaxCollection
Fields
- Items EmployeeAUSFederalTax[]? -
dayforce: EmployeeAUSSuperannuation
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- MembershipNumber string? -
- SuperannuationContributionCalcValue decimal? -
- PayrollPayee PayrollPayeeInfo? -
- PayrollDeduction PayrollDeduction? -
- SuperannuationContributionType SuperannuationContributionType? -
- SuperannuationType SuperannuationType? -
- SuperannuationContributionCalculationType SuperannuationContributionCalculationType? -
- LastModifiedTimestamp string? -
dayforce: EmployeeAUSSuperannuationCollection
Fields
- Items EmployeeAUSSuperannuation[]? -
dayforce: EmployeeAUSSuperannuationRules
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- ApplyQuarterlyMaxBaseCapForEmployerSuperannuation boolean? -
- OverrideMinBaseForEmployerSuperannuation boolean? -
- MinimumContributionAmount decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeeAUSSuperannuationRulesCollection
Fields
- Items EmployeeAUSSuperannuationRules[]? -
dayforce: EmployeeAvailability
Fields
- DateOfRequest string? -
- UnAvailable boolean? -
- IsDefault boolean? -
- StartTime1 string? -
- EndTime1 string? -
- StartTime2 string? -
- EndTime2 string? -
dayforce: EmployeeAvailabilityDaysPostRequestDTO
Fields
- StartTime1 string? -
- EndTime1 string? -
- StartTime2 string? -
- EndTime2 string? -
- IsAvailable boolean -
dayforce: EmployeeAvailabilityPostAPIRequestDTO
Fields
- IsDefault boolean -
- EffectiveStart string -
- EffectiveEnd string -
- ManagerComments string? -
- Weeks EmployeeAvailabilityWeeksPostRequestDTO[] -
dayforce: EmployeeAvailabilityWeeksPostRequestDTO
Fields
dayforce: EmployeeBadge
Fields
- BadgeNumber string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeBadgeCollection
Fields
- Items EmployeeBadge[]? -
dayforce: EmployeeBalancePeriod
Fields
- EmployeeXRefCode string? -
- BalanceName string? -
- BalanceXRefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- InitialAccruedValue decimal? -
- InitialGrantValue decimal? -
- CurrentAccruedValue decimal? -
- CurrentGrantValue decimal? -
- MinimumValue decimal? -
- MaximumValue decimal? -
dayforce: EmployeeBalanceTransaction
Fields
- TransactionDate string? -
- ExpiryDate string? -
- Delta decimal? -
- GrantDelta decimal? -
- TransactionSource Signed32? -
- TAFWXRefCode string? -
- Comment string? -
- ApprovalStatus string? -
dayforce: EmployeeBalanceTransactionForPatch
Fields
- TransactionDate string? -
- Delta decimal? -
- GrantDelta decimal? -
- Comment string? -
dayforce: EmployeeBalanceTransactionForSubmit
Fields
- BalanceXRefCode string? -
- EmployeeXRefCode string? -
- TransactionDate string? -
- Value decimal? -
- Comment string? -
dayforce: EmployeeBalanceTransactionPatchResponse
Fields
- EmployeeBalanceTransactionXRefCode string? -
dayforce: EmployeeBalanceTransactionPostResponse
Fields
- EmployeeBalanceTransactionXRefCode string? -
dayforce: EmployeeBalanceTransactions
Fields
- EmployeeBalancePeriodIdExternal int? -
- EffectiveStart string? -
- EffectiveEnd string? -
- Transactions EmployeeBalanceTransaction[]? -
dayforce: EmployeeCANEmploymentInsuranceRate
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- LegalEntity LegalEntity? -
- RateGroup string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeCANFederalTax
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- TotalClaimAmount decimal? -
- IsNonResident boolean? -
- MultipleEmployer boolean? -
- IncomeLessThanClaim boolean? -
- DistrictTaxDeduction decimal? -
- AuthorizedTaxCredits decimal? -
- EstimatedExpense decimal? -
- EstimatedRemuneration decimal? -
- AdditionalAmount decimal? -
- PrescribedAreaDeduction decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeeCANFederalTaxCollection
Fields
- Items EmployeeCANFederalTax[]? -
dayforce: EmployeeCANStateTax
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- State State? -
- TotalClaimAmount decimal? -
- PrescribedAreaDeduction decimal? -
- MaintenanceDeduction decimal? -
- AuthorizedTaxCredits decimal? -
- QuebecDevelopmentFunds decimal? -
- EstimatedExpense decimal? -
- EstimatedRemuneration decimal? -
- AdditionalAmount decimal? -
- LabourSponsoredFunds decimal? -
- HasQuebecHealthContributionExemption boolean? -
- IncomeLessThanClaim boolean? -
- LastModifiedTimestamp string? -
dayforce: EmployeeCANStateTaxCollection
Fields
- Items EmployeeCANStateTax[]? -
dayforce: EmployeeCANTaxStatus
Fields
- ProvinceCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- TaxPropertyCollection TaxPropertyCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeCANTaxStatusCollection
Fields
- Items EmployeeCANTaxStatus[]? -
dayforce: EmployeeClockDeviceGroup
Fields
- ClockDeviceGroup ClockDeviceGroup? -
- LastModifiedTimestamp string? -
dayforce: EmployeeClockDeviceGroupCollection
Fields
- Items EmployeeClockDeviceGroup[]? -
dayforce: EmployeeCompensation
Fields
- EmployeeNumber string? -
- EffectiveEnd string? -
- EffectiveStart string? -
- PayGrade PayGrade? -
- PayType PayType? -
- PayGroup PayGroup? -
- PayClass PayClass? -
- EmploymentStatusReason EmploymentStatusReason? -
- AlternateRate decimal? -
- AverageDailyHours decimal? -
- AverageOvertimeRate decimal? -
- BaseRate decimal? -
- BaseRateManuallySet boolean? -
- BaseSalary decimal? -
- DailyRate decimal? -
- NormalWeeklyHours decimal? -
- NormalSemiMonthlyHoursTop decimal? -
- NormalSemiMonthlyHoursBottom decimal? -
- LastPayEditDate string? -
- VacationRate decimal? -
- MinimumRate decimal? -
- ControlRate decimal? -
- MaximumRate decimal? -
- RateMidPoint decimal? -
- MinimumSalary decimal? -
- ControlSalary decimal? -
- MaximumSalary decimal? -
- SalaryMidPoint decimal? -
- CompRatio decimal? -
- ChangePercent decimal? -
- ChangeValue decimal? -
- PreviousBaseSalary decimal? -
- PreviousBaseRate decimal? -
- PayPolicy PayPolicy? -
- RatePolicy JobStepPolicy? -
- LastModifiedTimestamp string? -
dayforce: EmployeeCompensationCollection
Fields
- Items EmployeeCompensation[]? -
dayforce: EmployeeConfidentialIdentification
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- ConfidentialIdentificationType ConfidentialIdentificationType? -
- CountryCode string? -
- ExpiryDate string? -
- IDNumber string? -
- PlaceOfIssue string? -
- IssueDate string? -
- UseForRTW boolean? -
- RTWDocReview string? -
- RTWDocReviewOn string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeConfidentialIdentificationCollection
Fields
- Items EmployeeConfidentialIdentification[]? -
dayforce: EmployeeCourse
Fields
- StartDate string? -
- CompletionDate string? -
- CertificationExpiryDate string? -
- Course Course? -
- EmployeeTrainingProgram EmployeeTrainingProgram? -
- CertificationNumber string? -
- Comment string? -
- Score string? -
- PassFail string? -
- Credits string? -
- Cost decimal? -
- CostCurrencyCode string? -
- EnrollmentStatus LMSEnrollmentStatus? -
- TimeSpent Signed32? -
- LastModifiedTimestamp string? -
dayforce: EmployeeCourseCollection
Fields
- Items EmployeeCourse[]? -
dayforce: EmployeeDeduction
Fields
- Deduction Deduction? -
- EffectiveStart string? -
- EffectiveEnd string? -
- ElectionStartPayPeriodStartDate string? -
- ElectionStartPayGroup PayGroup? -
- ElectionSchedule ElectionSchedule? -
- IsBlocked boolean? -
- Position Position? -
- Location Location? -
- ContinuePaymentOnStatutoryPay boolean? -
- IsOptOut Signed32? -
- EmployeeDeductionParameters EmployeeDeductionParameterCollection? -
- EmployeeDeductionLimits EmployeeDeductionLimitCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDeductionLimit
Fields
- DeductionLimit DeductionLimit? -
- LimitAmount decimal? -
- LimitPercent decimal? -
- PayGroupLimitAmount decimal? -
- PayGroupLimitPercent decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDeductionLimitCollection
Fields
- Items EmployeeDeductionLimit[]? -
dayforce: EmployeeDeductionParameter
Fields
- DeductionParameter DeductionParameter? -
- Value decimal? -
- PayGroupValue decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDeductionParameterCollection
Fields
- Items EmployeeDeductionParameter[]? -
dayforce: EmployeeDeductionPayeeParameter
Fields
- ShortName string? -
- XRefCode string? -
- EmployeeElectedValue string? -
- PayeeDefaultValue string? -
- Comments string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDeductionsCollection
Fields
- Items EmployeeDeduction[]? -
dayforce: EmployeeDefaultLabor
Fields
- Position Position? -
- EffectiveEnd string? -
- EffectiveStart string? -
- Location Location? -
- PayAdjCode PayAdjCode? -
- Project Project? -
- Docket Docket? -
- EmployeeDefaultLaborMetricsCodes EmployeeDefaultLaborMetricsCodeCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDefaultLaborCollection
Fields
- Items EmployeeDefaultLabor[]? -
dayforce: EmployeeDefaultLaborMetricsCode
Fields
- LaborMetricsCode LaborMetricsCode? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDefaultLaborMetricsCodeCollection
Fields
- Items EmployeeDefaultLaborMetricsCode[]? -
dayforce: EmployeeDependentBeneficiary
Fields
- FirstName string? -
- MiddleName string? -
- LastName string? -
- BirthDate string? -
- SocialSecurityNumber string? -
- SSNExpiryDate string? -
- TobaccoUser string? -
- DateLastSmoked string? -
- IsStudent boolean? -
- IsDisabled boolean? -
- SocialSecurityDisabilityAwardDate string? -
- Gender string? -
- IsBeneficiary boolean? -
- IsDependent boolean? -
- IsActiveDependent boolean? -
- IsActiveBeneficiary boolean? -
- Relationship RelationshipType? -
- MaritalStatus MaritalStatus? -
- Addresses EmployeeDependentsBeneficiariesAddressCollection? -
- Contacts EmployeeDependentsBeneficiariesContactCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDependentsBeneficiariesAddress
Fields
- County string? -
- ContactInformationType ContactInformationType? -
- EffectiveStart string? -
- EffectiveEnd string? -
- Address1 string? -
- Address2 string? -
- Address3 string? -
- Address4 string? -
- Address5 string? -
- Address6 string? -
- City string? -
- PostalCode string? -
- Country Country? -
- State State? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDependentsBeneficiariesAddressCollection
Fields
- Items EmployeeDependentsBeneficiariesAddress[]? -
dayforce: EmployeeDependentsBeneficiariesCollection
Fields
- Items EmployeeDependentBeneficiary[]? -
dayforce: EmployeeDependentsBeneficiariesContact
Fields
- ContactNumber string? -
- Extension string? -
- IsUnlistedNumber boolean? -
- EffectiveStart string? -
- EffectiveEnd string? -
- ContactInformationType ContactInformationType? -
- Country Country? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDependentsBeneficiariesContactCollection
Fields
- Items EmployeeDependentsBeneficiariesContact[]? -
dayforce: EmployeeDEUTaxSocialInsuranceCollection
Fields
- Items DEUTaxSocialInsurance[]? -
dayforce: EmployeeDirectDeposit
Fields
- AccountNumber string? -
- BankName string? -
- BankNumber string? -
- BuildingSocietyNumber string? -
- DepositNumber Signed32? -
- DepositValue decimal? -
- EffectiveStart string? -
- EffectiveEnd string? -
- PayMethod PayMethod? -
- IsDeleted boolean? -
- IsPercentage boolean? -
- IsRemainder boolean? -
- IsSpecialDisbursementAccount boolean? -
- IsMultiSourceAccount boolean? -
- NumberOfPreNoteDays Signed32? -
- PrenoteFileRunPayDate string? -
- PrenoteFileSentOn string? -
- RequiresPreNote boolean? -
- RoutingTransitNumber string? -
- PayCardType string? -
- FinancialInstitution FinancialInstitution? -
- AccountHolder string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDirectDepositCollection
Fields
- Items EmployeeDirectDeposit[]? -
dayforce: EmployeeDisability
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- EvidenceNumber string? -
- CountryCode string? -
- DisabilityEvidenceIssuingAgencyLocation string? -
- ValidFrom string? -
- ExpiryDate string? -
- DisabilityEvidenceIssuingAgency DisabilityEvidenceIssuingAgency? -
- DisabilityEvidenceType DisabilityEvidenceType? -
- DisabilityWorkingTime DisabilityWorkingTime? -
- DisabilityGroup DisabilityGroup? -
- LastModifiedTimestamp string? -
dayforce: EmployeeDisabilityCollection
Fields
- Items EmployeeDisability[]? -
dayforce: EmployeeDocumentManagementSecurityGroup
Fields
- DocMgmtSecurityGroup DocMgmtSecurityGroup? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEarning
Fields
- ContinuePaymentOnStatutoryPay boolean? -
- IsOptOut Signed32? -
- Earning Earning? -
- EffectiveEnd string? -
- EffectiveStart string? -
- ElectionSchedule ElectionSchedule? -
- ElectionStartPayGroup PayGroup? -
- ElectionStartPayPeriodStartDate string? -
- IsBlocked boolean? -
- LimitAmount decimal? -
- LimitPercent decimal? -
- Location Location? -
- Position Position? -
- EmployeeEarningParameters EmployeeEarningParameterCollection? -
- EmployeeEarningLimits EmployeeEarningLimitCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEarningLimit
Fields
- EarningLimit EarningLimit? -
- LimitAmount decimal? -
- LimitPercent decimal? -
- PayGroupLimitAmount decimal? -
- PayGroupLimitPercent decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEarningLimitCollection
Fields
- Items EmployeeEarningLimit[]? -
dayforce: EmployeeEarningParameter
Fields
- EarningParameter EarningParameter? -
- Value decimal? -
- PayGroupValue decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEarningParameterCollection
Fields
- Items EmployeeEarningParameter[]? -
dayforce: EmployeeEarningPayeeParameter
Fields
- ShortName string? -
- XRefCode string? -
- EmployeeElectedValue string? -
- PayeeDefaultValue string? -
- Comments string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEarningsCollection
Fields
- Items EmployeeEarning[]? -
dayforce: EmployeeEarningSummary
Fields
- Year Signed32? -
- NationalIdNumber string? -
- FirstName string? -
- LastName string? -
- RateOfPay decimal? -
- EmployeeResidentialAddress EmployeeAddress? -
- GrossEarningYTD decimal? -
- GrossNormalEarningYTD decimal? -
- GrossOverTimeEarningYTD decimal? -
- GrossBonusEarningYTD decimal? -
- GrossCommissionEarningYTD decimal? -
- GrossOtherEarningYTD decimal? -
- EarningGroupingsSumUp boolean? -
- EmploymentStatus string? -
- EmploymentType string? -
- JobAssignment string? -
- HireDate string? -
- TerminationDate string? -
- PayFrequency string? -
- PayType string? -
- LastPayrollPayDate string? -
- LegalEntityName string? -
- FEIN string? -
- LegalEntityAddress LegalEntityAddress? -
dayforce: EmployeeEEO
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEIRateCollection
Fields
- Items EmployeeCANEmploymentInsuranceRate[]? -
dayforce: EmployeeElectionAPIDeletionModel
Fields
- ElectionXrefCode string? -
- SourceTypeXRefCode string? -
dayforce: EmployeeEmergencyContact
Fields
- FirstName string? -
- LastName string? -
- MiddleName string? -
- Comment string? -
- IsPrimary boolean? -
- Relationship RelationshipType? -
- Addresses EmergencyContactPersonAddressCollection? -
- Contacts EmergencyContactPersonContactCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEmploymentAgreement
Fields
- EffectiveEnd string? -
- EffectiveStart string? -
- XRefCode string? -
- EmploymentAgreementType EmploymentAgreementType? -
- EmploymentAgreementPopulation EmploymentAgreementPopulation? -
- EmploymentAgreementDetails EmploymentAgreementDetails? -
- EmploymentAgreementTaxRegime EmploymentAgreementTaxRegime? -
- EmploymentAgreementDuration EmploymentAgreementDuration? -
- EmploymentAgreementSettlement EmploymentAgreementSettlement? -
- GovernmentIdentifier string? -
- AgreementReason EmploymentStatusReason? -
- AgreementEndReason EmploymentStatusReason? -
- Country Country? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEmploymentAgreementCollection
Fields
- Items EmployeeEmploymentAgreement[]? -
dayforce: EmployeeEmploymentStatus
Fields
- EmployeeNumber string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- EmploymentStatus EmploymentStatus? -
- EmploymentStatusGroup EmploymentStatusGroup? -
- PayType PayType? -
- PayGroup PayGroup? -
- PayTypeGroup PayTypeGroup? -
- PayClass PayClass? -
- PunchPolicy PunchPolicy? -
- PayPolicy PayPolicy? -
- PayHolidayGroup PayHolidayGroup? -
- EmployeeGroup EmployeeGroup? -
- EntitlementPolicy EntitlementPolicy? -
- ShiftRotation ShiftRotation? -
- ShiftRotationDayOffset int? -
- ShiftRotationStartDate string? -
- CreateShiftRotationShift boolean? -
- TimeOffPolicy TimeOffPolicy? -
- ShiftTradePolicy ShiftTradePolicy? -
- AttendancePolicy AttendancePolicy? -
- SchedulePolicy EmployeeSchedulePolicy? -
- OvertimeGroup EmployeeOvertimeGroup? -
- PayrollPolicy PayrollPolicy? -
- JobStepPolicy JobStepPolicy? -
- AlternateRate decimal? -
- AverageDailyHours decimal? -
- AverageOvertimeRate decimal? -
- BaseRate decimal? -
- BaseRateManuallySet boolean? -
- BaseSalary decimal? -
- PeriodicSalary decimal? -
- DailyRate decimal? -
- EmploymentStatusReason EmploymentStatusReason? -
- NormalWeeklyHours decimal? -
- DEUHoursChangeReason DEUHoursChangeReason? -
- NormalSemiMonthlyHoursTop decimal? -
- NormalSemiMonthlyHoursBottom decimal? -
- ScheduleChangePolicy ScheduleChangePolicy? -
- AuthorizationPolicy AuthorizationPolicy? -
- WorkContractPremiumPolicy WorkContractPremiumPolicy? -
- LastPayEditDate string? -
- VacationRate decimal? -
- TargetBonus decimal? -
- OriginalEmploymentStatus EmploymentStatus? -
- OriginalEffectiveStart string? -
- OriginalEffectiveEnd string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEmploymentStatusCollection
Fields
- Items EmployeeEmploymentStatus[]? -
dayforce: EmployeeEmploymentType
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- EmploymentType EmploymentTypeConfig? -
- TaxPayerId string? -
- DBAName string? -
- GamingProfitsDistributions boolean? -
- IR35 boolean? -
- PensionType PensionTypeConfiguration? -
- ContractorTaxFormType string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEmploymentTypeCollection
Fields
- Items EmployeeEmploymentType[]? -
dayforce: EmployeeEthnicity
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- Ethnicity DFEthnicity? -
- ManagerEthnicity DFEthnicity? -
- LastModifiedTimestamp string? -
dayforce: EmployeeEthnicityCollection
Fields
- Items EmployeeEthnicity[]? -
dayforce: EmployeeExportParams
Fields
- PayGroupXRefCode string? -
- EmployeeXRefCode string? -
- EmployeeNumber string? -
- Expand string? -
- PageSize Signed32? -
- ContextDate string? -
- ContextDateRangeFrom string? -
- ContextDateRangeTo string? -
- ContextDateOption string? -
- DeltaOption string? -
- DeltaDate string? -
- AmfEntity string? -
- AmfLevel string? -
- AmfLevelValue string? -
- ExportAllEmployeeDetailOnDelta boolean? -
- ExcludeTerminatedEmployeesOlderThanXDays Signed32? -
dayforce: EmployeeGlobalPropertyValue
Fields
- EffectiveEnd string? -
- EffectiveStart string? -
- GlobalProperty GlobalProperty? -
- BitValue boolean? -
- NumberValue decimal? -
- OptionValue GlobalPropertyOption? -
- StringValue string? -
- DateTimeValue string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeGlobalPropertyValueCollection
Fields
- Items EmployeeGlobalPropertyValue[]? -
dayforce: EmployeeGLSplits
Fields
- EmployeeName string? -
- EmployeeNumber string? -
- EmployeeXRefCode string? -
- EmployeeGLSplitSets EmployeeGLSplitSets[]? -
dayforce: EmployeeGLSplitSetDetailLaborMetricCodes
Fields
- LaborMetricsTypeXRefCode string? -
- LaborMetricsTypeName string? -
- LaborMetricsCodeXRefCode string? -
- LaborMetricsCodeName string? -
dayforce: EmployeeGLSplitSetDetails
Fields
- LaborPercentage decimal? -
- IsPrimary boolean? -
- EmployeeGLSplitSetDetailLaborMetricCodeDetails EmployeeGLSplitSetDetailLaborMetricCodes[]? -
dayforce: EmployeeGLSplitSetDetailUpsert
Fields
- LaborPercentage Signed32? -
- IsPrimary boolean? -
- LaborMetricsXRefCodes string[]? -
dayforce: EmployeeGLSplitSets
Fields
- OrgUnitXRefCode string? -
- DeptJobXRefCode string? -
- DeptJobName string? -
- OrgUnitName string? -
- SplitEffectiveTo string? -
- SplitEffectiveFrom string? -
- EmployeeGLSplitSetDetails EmployeeGLSplitSetDetails[]? -
dayforce: EmployeeGLSplitUpsert
Fields
- EmployeeXRefCode string? -
- GLSplitSets GLSplitSetUpsert[]? -
dayforce: EmployeeGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeHealthWellness
Fields
- TobaccoUser string? -
- DateLastSmoked string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeHealthWellnessCollection
Fields
- Items EmployeeHealthWellness[]? -
dayforce: EmployeeHighlyCompensatedEmployeeIndicator
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- IsHCE boolean? -
- LastModifiedTimestamp string? -
dayforce: EmployeeHRIncident
Fields
- EmployeeComplainantXRefCode string? -
- HRIncidentNotes EmployeeHRIncidentNoteCollection? -
- OrgUnit OrgUnitDetailConfiguration? -
- HRIncidentState string? -
- OpenDate string? -
- HRIncidentAction HRIncidentAction? -
- AssignedToUserXRefCode string? -
- HRIncidentType HRIncidentType? -
- ClosedDate string? -
- HRIncidentDate string? -
- HRIncidentBeganWork string? -
- HRIncidentEventTime string? -
- SafetyHealthType SafetyHealthType? -
- HRIncidentInjury HRIncidentInjury? -
- HRIncidentBodyPart HRIncidentBodyPart? -
- Died boolean? -
- HRIncidentArea string? -
- TaskBeingPerformed string? -
- CausedObject string? -
- CausedAction string? -
- PrivacyCase boolean? -
- DoctorName string? -
- EmergencyRoom boolean? -
- HospitalOvernight boolean? -
- Hospital string? -
- HospitalStreet string? -
- HospitalCity string? -
- HospitalStateCode string? -
- HospitalZip string? -
- DateReturnToWork string? -
- DaysLost decimal? -
- DaysRestricted decimal? -
- DateDied string? -
- QuestionableClaim boolean? -
- IsDaysLost boolean? -
- WCBCaseNumber string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeHRIncidentCollection
Fields
- Items EmployeeHRIncident[]? -
dayforce: EmployeeHRIncidentNote
Fields
- DateAdded string? -
- Notes string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeHRIncidentNoteCollection
Fields
- Items EmployeeHRIncidentNote[]? -
dayforce: EmployeeHRPolicy
Fields
- SignOff boolean? -
- SignOffDate string? -
- HRPolicy HRPolicy? -
- LastModifiedTimestamp string? -
dayforce: EmployeeHRPolicyCollection
Fields
- Items EmployeeHRPolicy[]? -
dayforce: EmployeeIRLTaxEWSS
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- IsEWSSEligible boolean? -
- LegalEntityXrefCode string? -
- LegalEntity LegalEntity? -
- LastModifiedTimestamp string? -
dayforce: EmployeeIRLTaxEWSSCollection
Fields
- Items EmployeeIRLTaxEWSS[]? -
dayforce: EmployeeIRLTaxPAYEExclusion
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- ExclusionOrder boolean? -
- LegalEntityXrefCode string? -
- LegalEntity LegalEntity? -
- LastModifiedTimestamp string? -
dayforce: EmployeeIRLTaxPAYEExclusionCollection
Fields
- Items EmployeeIRLTaxPAYEExclusion[]? -
dayforce: EmployeeIRLTaxPRSI
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- PRSIClassXrefCode string? -
- CommunityEmployment boolean? -
- PRSIExempt boolean? -
- PRSIExemptReasonXrefCode string? -
- LegalEntityXrefCode string? -
- LegalEntity LegalEntity? -
- PrsiClass PRSIClass? -
- PrsiExemptReason PRSIExemptReason? -
- CompanyDirector string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeIRLTaxPRSICollection
Fields
- Items EmployeeIRLTaxPRSI[]? -
dayforce: EmployeeIRLTaxRPN
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- EmploymentCessationDate string? -
- TaxYear Signed32? -
- RpnNumber string? -
- EmployeePpsn string? -
- EmploymentID string? -
- RpnIssueDate string? -
- EmployerReference string? -
- FirstName string? -
- FamilyName string? -
- PreviousEmployeePPSN string? -
- IncomeTaxCalculationBasis string? -
- ExclusionOrder boolean? -
- YearlyTaxCredits decimal? -
- PayForIncomeTaxToDate decimal? -
- IncomeTaxDeductedToDate decimal? -
- UscStatus string? -
- PayForUSCToDate decimal? -
- UscDeductedToDate decimal? -
- LptToDeduct decimal? -
- PRSIExempt boolean? -
- PrsiClass string? -
- TaxRatePercent1 decimal? -
- YearlyRateCutOff1 decimal? -
- TaxRatePercent2 decimal? -
- UscRatePercent1 decimal? -
- YearlyUSCRateCutOff1 decimal? -
- UscRatePercent2 decimal? -
- YearlyUSCRateCutOff2 decimal? -
- UscRatePercent3 decimal? -
- YearlyUSCRateCutOff3 decimal? -
- UscRatePercent4 decimal? -
- LegalEntityXrefCode string? -
- LegalEntity LegalEntity? -
- LastModifiedTimestamp string? -
dayforce: EmployeeIRLTaxRPNCollection
Fields
- Items EmployeeIRLTaxRPN[]? -
dayforce: EmployeeLaborMetrics
Fields
- LaborMetricsTypeXRefCode string? -
- LaborMetricsTypeName string? -
- LaborMetricsCodeXRefCode string? -
- LaborMetricsCodeName string? -
dayforce: EmployeeLegalEntity
Fields
- NormalWeeklyHours decimal? -
- WorkLocationAddress WorkLocationAddress? -
- OriginalHireDate string? -
- BaseSalary decimal? -
- HourlyRate decimal? -
- EmploymentStatus string? -
- EmploymentType string? -
- JobAssignment string? -
- HireDate string? -
- TerminationDate string? -
- PayFrequency string? -
- PayType string? -
- LastPayrollPayDate string? -
- LegalEntityName string? -
- FEIN string? -
- LegalEntityAddress LegalEntityAddress? -
dayforce: EmployeeLocation
Fields
- IsPrimary boolean? -
- Location Location? -
- EffectiveStart string? -
- EffectiveEnd string? -
- IsDefault boolean? -
- EmployeeLocationAuthorities EmployeeLocationAuthorityCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeLocationAuthority
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- AuthorityType AuthorityType? -
- LastModifiedTimestamp string? -
dayforce: EmployeeLocationAuthorityCollection
Fields
- Items EmployeeLocationAuthority[]? -
dayforce: EmployeeLocationCollection
Fields
- Items EmployeeLocation[]? -
dayforce: EmployeeManager
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- ManagerXRefCode string? -
- ManagerFirstName string? -
- ManagerMiddleName string? -
- ManagerLastName string? -
- ManagerBadgeNumber string? -
- DerivationMethod Signed32? -
- LastModifiedTimestamp string? -
dayforce: EmployeeManagerCollection
Fields
- Items EmployeeManager[]? -
dayforce: EmployeeMaritalStatus
Fields
- MaritalStatus MaritalStatus? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeMaritalStatusCollection
Fields
- Items EmployeeMaritalStatus[]? -
dayforce: EmployeeMUSTaxDetails
Fields
- LastModifiedTimestamp string? -
dayforce: EmployeeMUSTaxDetailsCollection
Fields
- Items EmployeeMUSTaxDetails[]? -
dayforce: EmployeeNZLKiwiSaver
dayforce: EmployeeOccupationalPension
Fields
- PensionStartDate string? -
- PensionProviderXrefCode string? -
- PaymentCompletedBy string? -
- MembershipNumber string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeOnboardingPolicy
Fields
- OnboardingPolicy OnboardingPolicy? -
- EffectiveStart string? -
- EffectiveEnd string? -
- IsInternalHire boolean? -
- LastModifiedTimestamp string? -
dayforce: EmployeeOnboardingPolicyCollection
Fields
- Items EmployeeOnboardingPolicy[]? -
dayforce: EmployeeOrgUnitInfoCollection
Fields
- Items EmployeeOrgUnitInformation[]? -
dayforce: EmployeeOrgUnitInformation
Fields
- OrgUnitDetail OrgUnitDetailConfiguration? -
- Department Department? -
- LastModifiedTimestamp string? -
dayforce: EmployeeOvertimeGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmployeePayAdjCodeGroupListCollection
Fields
- Items EmployeePayAdjustCodeGroup[]? -
dayforce: EmployeePayAdjustCodeGroup
Fields
- PayAdjCodeGroup PayAdjCodeGroup? -
- LastModifiedTimestamp string? -
dayforce: EmployeePayAdjustment
Fields
- EmployeePayAdjustXRefCode string? -
- AdjustPeriodStartDate string? -
- AdjustPeriodEndDate string? -
- EmployeeXRefCode string? -
- DepartmentXRefCode string? -
- JobXRefCode string? -
- PayAdjustmentCodeXRefCode string? -
- PayCategoryXRefCode string? -
- PayDate string? -
- OrgUnitXRefCode string? -
- IsPremium boolean? -
- IsDeleted boolean? -
- TimeStart string? -
- TimeEnd string? -
- Hours decimal? -
- Rate decimal? -
- Amount decimal? -
- ReferenceDate string? -
- ProjectXRefCode string? -
- DocketXRefCode string? -
- EmployeeComment string? -
- ManagerComment string? -
- EmployeeAuthorized boolean? -
- ManagerAuthorized boolean? -
- LaborMetrics EmployeePayAdjustmentLaborMetrics[]? -
- LastModifiedTimestampUtc string? -
dayforce: EmployeePayAdjustmentForSubmit
Fields
- EmployeePayAdjustXRefCode string? -
- OrgUnitXRefCode string? -
- EmployeeXRefCode string? -
- PayAdjustmentCodeXRefCode string? -
- PayAdjustmentDate string? -
- Hours decimal? -
- Amount decimal? -
- JobXRefCode string? -
- DepartmentXRefCode string? -
- PayCategoryXRefCode string? -
- ProjectXRefCode string? -
- DocketXRefCode string? -
- IsPremium boolean? -
- IsDeleted boolean? -
- Rate decimal? -
- PayDate string? -
- ReferenceDate string? -
- EmployeeComment string? -
- ManagerComment string? -
- ManagerAuthorized boolean? -
- EmployeeAuthorized boolean? -
- AdjustPeriodStartDate string? -
- AdjustPeriodEndDate string? -
- LaborMetrics EmployeePayAdjustmentLaborMetrics[]? -
dayforce: EmployeePayAdjustmentLaborMetrics
Fields
- LaborMetricsTypeXRefCode string? -
- LaborMetricsCodeXRefCode string? -
dayforce: EmployeePayAdjustmentPostResponse
Fields
- EmployeePayAdjustXRefCode string? -
dayforce: EmployeePayGradeRate
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- WorkAssignmentEffectiveStart string? -
- WorkAssignmentEffectiveEnd string? -
- PayGrade PayGrade? -
- MinimumRate decimal? -
- ControlRate decimal? -
- MaximumRate decimal? -
- RateMidPoint decimal? -
- MinimumSalary decimal? -
- ControlSalary decimal? -
- MaximumSalary decimal? -
- SalaryMidPoint decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeePayGradeRateCollection
Fields
- Items EmployeePayGradeRate[]? -
dayforce: EmployeePayrollElection
Fields
- EmployeeName string? -
- EmployeeNumber string? -
- EmployeeXRefCode string? -
- RowNumber int? -
- Elections PayrollElection[]? -
- CurrentPayRunStatus string? -
- CurrentPayPeriodStart string? -
- CurrentPayPeriodEnd string? -
- PreviousPayPeriodStart string? -
- PreviousPayPeriodEnd string? -
dayforce: EmployeePayrollElectionDeletionModel
Fields
- EmployeeXRefCode string? -
- Elections EmployeeElectionAPIDeletionModel[]? -
dayforce: EmployeePayrollTax
Fields
- TaxAuthority string? -
- TaxType string? -
- Name EmployeePayrollTaxName? -
- EmployeeTax boolean? -
- EmployerTax boolean? -
- LegalEntity string? -
- ResidentCode string? -
- ManuallyAddedTax boolean? -
- Addresses string[]? -
- TaxAuthorityInstance string? -
- LegalEntityXrefCode string? -
- LastModifiedTimestamp string? -
dayforce: EmployeePayrollTaxCollection
Fields
- Items EmployeePayrollTax[]? -
dayforce: EmployeePayrollTaxName
Fields
- TaxName string? -
- Description string? -
dayforce: EmployeePayrollTaxParameter
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- ExemptTaxOnlyUpdateWages boolean? -
- ExemptTaxAndTaxableWages boolean? -
- InactivateTax boolean? -
- TaxAuthorityInstance string? -
- LegalEntityXrefCode string? -
- OverrideParameters EmployeePayrollTaxParameterElement[]? -
- LastModifiedTimestamp string? -
dayforce: EmployeePayrollTaxParameterCollection
Fields
- Items EmployeePayrollTaxParameter[]? -
dayforce: EmployeePayrollTaxParameterElement
Fields
- Value string? -
- LastModifiedTimestamp string? -
dayforce: EmployeePayrollTaxParameterElementName
Fields
- ShortName string? -
- LongName string? -
- XRefCode string? -
dayforce: EmployeePaySummary
Fields
- EmployeeXRefCode string? -
- PositionXRefCode string? -
- DepartmentXRefCode string? -
- JobXRefCode string? -
- PayCodeXRefCode string? -
- PayCategoryXRefCode string? -
- PayDate string? -
- BusinessDate string? -
- TimeStart string? -
- TimeEnd string? -
- PunchSegmentStart string? -
- LocationXRefCode string? -
- NetHours decimal? -
- MinuteDuration Signed32? -
- Rate decimal? -
- PayAmount decimal? -
- IsPremium boolean? -
- ProjectXRefCode string? -
- DocketXRefCode string? -
- PieceQuantity decimal? -
- PayExportId int? -
- LaborMetricsCode0XRefCode string? -
- LaborMetricsCode1XRefCode string? -
- LaborMetricsCode2XRefCode string? -
- LaborMetricsCode3XRefCode string? -
- LaborMetricsCode4XRefCode string? -
- LaborMetricsCode5XRefCode string? -
- LaborMetricsCode6XRefCode string? -
- LaborMetricsCode7XRefCode string? -
- LaborMetricsCode8XRefCode string? -
- LaborMetricsCode9XRefCode string? -
dayforce: EmployeePaySummaryRetro
Fields
- IsRetro boolean? -
- EmployeeXRefCode string? -
- PositionXRefCode string? -
- DepartmentXRefCode string? -
- JobXRefCode string? -
- PayCodeXRefCode string? -
- PayCategoryXRefCode string? -
- PayDate string? -
- BusinessDate string? -
- TimeStart string? -
- TimeEnd string? -
- PunchSegmentStart string? -
- LocationXRefCode string? -
- NetHours decimal? -
- MinuteDuration Signed32? -
- Rate decimal? -
- PayAmount decimal? -
- IsPremium boolean? -
- ProjectXRefCode string? -
- DocketXRefCode string? -
- PieceQuantity decimal? -
- PayExportId int? -
- LaborMetricsCode0XRefCode string? -
- LaborMetricsCode1XRefCode string? -
- LaborMetricsCode2XRefCode string? -
- LaborMetricsCode3XRefCode string? -
- LaborMetricsCode4XRefCode string? -
- LaborMetricsCode5XRefCode string? -
- LaborMetricsCode6XRefCode string? -
- LaborMetricsCode7XRefCode string? -
- LaborMetricsCode8XRefCode string? -
- LaborMetricsCode9XRefCode string? -
dayforce: EmployeePerformanceRating
Fields
- Comments string? -
- NextReviewDate string? -
- PerformanceCycle PerformanceCycle? -
- PerformanceRatingScale PFRatingScale? -
- PerformanceRating PerformanceRating? -
- RatingScore decimal? -
- ReviewDate string? -
- Reviewer BaseEmployeeSubset? -
- ReviewPeriodStartDate string? -
- ReviewPeriodEndDate string? -
- LastModifiedTimestamp string? -
dayforce: EmployeePerformanceRatingCollection
Fields
- Items EmployeePerformanceRating[]? -
dayforce: EmployeePrivateHealthInsurance
Fields
- HealthInsuranceStartDate string? -
- PrivateHealthInsuranceXrefCode string? -
- PaymentCompletedByXrefCode string? -
- TotalContributionHealth decimal? -
- TotalContributionCare decimal? -
- BaseContributionHealth decimal? -
- BaseContributionCare decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeeProperty
Fields
- DataType Signed32? -
- EmployeeCardinality Signed32? -
- IsEditable boolean? -
- DataTypeParam string? -
- GenerateHREvent boolean? -
- Sequence Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmployeePropertyOption
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmployeePropertyValue
Fields
- EffectiveEnd string? -
- EffectiveStart string? -
- EmployeeProperty EmployeeProperty? -
- BitValue boolean? -
- NumberValue decimal? -
- OptionValue EmployeePropertyOption? -
- StringValue string? -
- DateTimeValue string? -
- LastModifiedTimestamp string? -
dayforce: EmployeePropertyValueCollection
Fields
- Items EmployeePropertyValue[]? -
dayforce: EmployeePunch
Fields
- PunchXRefCode string? -
- EmployeeXRefCode string? -
- PunchStatus string? -
- TimeStart string? -
- TimeEnd string? -
- TimeStartRaw string? -
- TimeEndRaw string? -
- NetHours decimal? -
- LocationXRefCode string? -
- PositionXRefCode string? -
- DepartmentXRefCode string? -
- JobXRefCode string? -
- DocketXRefCode string? -
- DocketQuantity decimal? -
- ProjectXRefCode string? -
- PayAdjustmentXRefCode string? -
- StartExceptionCode string? -
- EndExceptionCode string? -
- EmployeeComment string? -
- ManagerComment string? -
- BusinessDate string? -
- IsDeleted boolean? -
- IsOnCall boolean? -
- FuturePunch boolean? -
- AssumedTimeStart string? -
- AssumedTimeEnd string? -
- LastModifiedTimestampUtc string? -
- MealBreaks MealBreaks[]? -
- Transfers Transfers[]? -
- LaborMetrics LaborMetrics[]? -
dayforce: EmployeePunchPatchPostDeleteResponse
Fields
- XRefCode string? -
dayforce: EmployeeRawPunch
Fields
- RawPunchXRefCode string? -
- PunchState string? -
- PayDate string? -
- EmployeeXRefCode string? -
- EmployeeBadge string? -
- RawPunchTime string? -
- WasOfflinePunch boolean? -
- ExtraData PunchExtraData? -
- PunchType string? -
- Comment string? -
- PunchDevice string? -
- SupervisorBadge string? -
- IsDuplicate boolean? -
- RejectedReason string? -
- LocationXRefCode string? -
- PositionXRefCode string? -
- DepartmentXRefCode string? -
- JobXRefCode string? -
- IPAddress string? -
- PunchOrigin string? -
- Latitude decimal? -
- Longitude decimal? -
- Accuracy Signed32? -
- PunchXRefCode string? -
dayforce: EmployeeRawPunchForSubmit
Fields
- EmployeeBadge string? -
- RawPunchTime string? -
- PunchType string? -
- PunchDevice string? -
- SupervisorBadge string? -
- LocationXRefCode string? -
- LocationClockTransferCode string? -
- PositionXRefCode string? -
- PositionClockTransferCode string? -
- DocketXRefCode string? -
- DocketClockTransferCode string? -
- ProjectXRefCode string? -
- ProjectClockTransferCode string? -
- LaborMetrics PunchLaborMetric[]? -
- Quantity decimal? -
- MealWaiver string? -
- BreakAttestation boolean? -
dayforce: EmployeeRole
Fields
- IsDefault boolean? -
- Role Role? -
- EffectiveStart string? -
- IsPrestartRole boolean? -
- EffectiveEnd string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeRoleCollection
Fields
- Items EmployeeRole[]? -
dayforce: EmployeeSchedule
Fields
- EmployeeScheduleXRefCode string? -
- EmployeeXRefCode string? -
- TimeStart string? -
- TimeEnd string? -
- NetHours decimal? -
- DepartmentXRefCode string? -
- JobXRefCode string? -
- PositionXRefCode string? -
- OrgUnitXRefCode string? -
- SiteOrgUnitXRefCode string? -
- OrgLocationTypeXRefCode string? -
- PayAdjCodeXRefCode string? -
- DocketXRefCode string? -
- ProjectXRefCode string? -
- Comment string? -
- Published boolean? -
- Breaks Breaks[]? -
- Activities Activity[]? -
- Skills Skills[]? -
- LaborMetrics LaborMetric[]? -
- Segments Segment[]? -
- IsPostedShiftBid boolean? -
dayforce: EmployeeScheduleActivityPostAPIRequestDTO
Fields
- XRefCode string -
- TimeStart string -
- TimeEnd string -
dayforce: EmployeeScheduleLaborMetricPostAPIRequestDTO
Fields
- CodeXRefCode string -
- TypeXRefCode string -
dayforce: EmployeeScheduleMBPostAPIRequestDTO
Fields
- TimeStart string -
- TimeEnd string -
- Type 1|2 -
dayforce: EmployeeSchedulePatchAPIRequestDTO
Fields
- EmployeeScheduleXRefCode string -
- TimeStart string? -
- TimeEnd string? -
- IsDeleted boolean? -
- EmployeeXRefCode string? -
- PostShiftBid boolean? -
- Breaks EmployeeScheduleMBPostAPIRequestDTO[]? -
- Activities EmployeeScheduleActivityPostAPIRequestDTO[]? -
- Segments EmployeeScheduleSegmentAPIRequestDTO[]? -
- PositionXRefCode string? -
- DocketXRefCode string? -
- PayAdjCodeXRefCode string? -
- OrgLocationTypeXRefCode string? -
- ProjectXRefCode string? -
- Comment string? -
- LaborMetrics EmployeeScheduleLaborMetricPostAPIRequestDTO[]? -
dayforce: EmployeeSchedulePolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeSchedulePostAPIRequestDTO
Fields
- TimeStart string -
- TimeEnd string -
- PositionXRefCode string -
- EmployeeXRefCode string? -
- PostShiftBid boolean? -
- Breaks EmployeeScheduleMBPostAPIRequestDTO[]? -
- Activities EmployeeScheduleActivityPostAPIRequestDTO[]? -
- Segments EmployeeScheduleSegmentAPIRequestDTO[]? -
- DocketXRefCode string? -
- PayAdjCodeXRefCode string? -
- OrgLocationTypeXRefCode string? -
- ProjectXRefCode string? -
- Comment string? -
- LaborMetrics EmployeeScheduleLaborMetricPostAPIRequestDTO[]? -
dayforce: EmployeeSchedulePostAPIResponse
Fields
- EmployeeScheduleXRefCode string? -
- EmployeeXRefCode string? -
- TimeStart string? -
- TimeEnd string? -
dayforce: EmployeeScheduleSegmentAPIRequestDTO
Fields
- TimeStart string -
- TimeEnd string -
- PositionXRefCode string -
- DocketXRefCode string? -
- PayAdjCodeXRefCode string? -
- OrgLocationTypeXRefCode string? -
- ProjectXRefCode string? -
- Comment string? -
- LaborMetrics EmployeeScheduleLaborMetricPostAPIRequestDTO[]? -
dayforce: EmployeeScheduleSegmentLaborMetric
Fields
- CodeXRefCode string? -
- TypeXRefCode string? -
dayforce: EmployeeShiftBidDTO
Fields
- GroupXRefCode string -
- BidState string -
dayforce: EmployeeShortTimeWork
Fields
- EmployeeShortTimeWorkId Signed32? -
- EffectiveStart string? -
- EffectiveEnd string? -
- IncreasedRate boolean? -
- Consent boolean? -
- DEUSTWParticipationReason DEUSTWParticipationReason? -
- LegalEntityDEUShortTimeWork LegalEntityDEUShortTimeWork? -
- LastModifiedTimestamp string? -
dayforce: EmployeeShortTimeWorkCollection
Fields
- Items EmployeeShortTimeWork[]? -
dayforce: EmployeeSkill
Fields
- Skill Skill? -
- SkillLevel SkillLevel? -
- EffectiveStart string? -
- EffectiveEnd string? -
- Course Course? -
- TrainingProgram TrainingProgram? -
- LMSAssignmentMethod LMSAssignmentMethod? -
- LastAssignedBy string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeSkillCollection
Fields
- Items EmployeeSkill[]? -
dayforce: EmployeeSSOAccount
Fields
- LoginName string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeTimeAwayFromWork
Fields
- TAFWXRefCode string? -
- DateOfRequest string? -
- TimeStart string? -
- TimeEnd string? -
- NetHours decimal? -
- NetUnits decimal? -
- NetUnitsType Signed32? -
- ManagerComment string? -
- EmployeeComment string? -
- ReasonName string? -
- AllDay boolean? -
- HalfDay boolean? -
- DailyElapsedHours decimal? -
dayforce: EmployeeTimeAwayFromWorkForSubmit
Fields
- EmployeeXRefCode string? -
- TAFWXRefCode string? -
- PayAdjustmentCodeXRefCode string? -
- StatusXRefCode string? -
- TimeStart string? -
- TimeEnd string? -
- NetHours decimal? -
- NetHoursPerDay decimal[]? -
- ManagerComment string? -
- EmployeeComment string? -
- AllDay boolean? -
- HalfDay boolean? -
- Days Signed32? -
dayforce: EmployeeTrainingProgram
Fields
- TrainingProgram TrainingProgram? -
- EnrollmentDate string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeTrainingProgramCollection
Fields
- Items EmployeeTrainingProgram[]? -
dayforce: EmployeeUKIrregularPaymentDetails
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- IrregularPayments boolean? -
- LegalEntityXrefCode string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUKIrregularPaymentDetailsCollection
Fields
- Items EmployeeUKIrregularPaymentDetails[]? -
dayforce: EmployeeUKNIDetails
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- NILetterXrefCode string? -
- DirectorStartDate string? -
- IsDirector boolean? -
- Annual boolean? -
- ChangeReasonXrefCode string? -
- LegalEntityXrefCode string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUKNIDetailsCollection
Fields
- Items EmployeeUKNIDetails[]? -
dayforce: EmployeeUKPostgraduateLoan
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- LegalEntityXrefCode string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUKPostgraduateLoanCollection
Fields
- Items EmployeeUKPostgraduateLoan[]? -
dayforce: EmployeeUKStudentLoan
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- StudentLoanPlanType Signed32? -
- LegalEntityXrefCode string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUKStudentLoanCollection
Fields
- Items EmployeeUKStudentLoan[]? -
dayforce: EmployeeUKTaxDetails
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- TaxCode string? -
- TaxBasis boolean? -
- PreviousTaxableGrossPaidToDate decimal? -
- PreviousTaxPaidToDate decimal? -
- StarterDeclaration string? -
- PreviouslyReportedOnFPS boolean? -
- ChangeReasonXrefCode string? -
- LegalEntityXrefCode string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUKTaxDetailsCollection
Fields
- Items EmployeeUKTaxDetails[]? -
dayforce: EmployeeUnionCollection
Fields
- Items EmployeeUnionMembership[]? -
dayforce: EmployeeUnionMembership
Fields
- UnionMembershipDate string? -
- EffectiveStart string? -
- Union DFUnion? -
- EffectiveEnd string? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUSFederalTax
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- FilingStatus FederalFilingStatus? -
- Allowances Signed32? -
- AdditionalAmount decimal? -
- IsTaxExempt boolean? -
- IsLocked boolean? -
- TwoJobs boolean? -
- DependentTaxCredit decimal? -
- OtherIncome decimal? -
- Deductions decimal? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUSFederalTaxCollection
Fields
- Items EmployeeUSFederalTax[]? -
dayforce: EmployeeUSStateTax
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- State State? -
- FilingStatus StateFilingStatus? -
- StateFilingStatusDisplayName string? -
- Allowances Signed32? -
- AdditionalAmount decimal? -
- DependentAllowances Signed32? -
- PersonalAllowances Signed32? -
- AdditionalAllowances Signed32? -
- IsTaxExempt boolean? -
- AlternateCalculationCode string? -
- AlternateCalculationCodeDisplayName string? -
- ExemptionAmount decimal? -
- AdditionalTaxPercent decimal? -
- AdditionalExemptionAmount decimal? -
- IsLocked boolean? -
- PRYoungEntrepreneurExemptionOptOut boolean? -
- AdoptionDependents Signed32? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUSStateTaxCollection
Fields
- Items EmployeeUSStateTax[]? -
dayforce: EmployeeUSTaxStatus
Fields
- StateCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- TaxPropertyCollection TaxPropertyCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeUSTaxStatusCollection
Fields
- Items EmployeeUSTaxStatus[]? -
dayforce: EmployeeVeteransStatus
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- IsVevraa boolean? -
- VeteransStatus DFVeteransStatus? -
- LastModifiedTimestamp string? -
dayforce: EmployeeVeteransStatusCollection
Fields
- Items EmployeeVeteransStatus[]? -
dayforce: EmployeeVOE
Fields
- Namespace string? -
- LegalEntities EmployeeLegalEntity[]? -
- NationalIdNumber string? -
- EmployeeXRefCode string? -
- FirstName string? -
- MiddleName string? -
- LastName string? -
- BirthDate string? -
- EmployeeResidentialAddress EmployeeAddress? -
dayforce: EmployeeVOI
Fields
- Earnings EmployeeEarningSummary[]? -
- Namespace string? -
- LegalEntities EmployeeLegalEntity[]? -
- NationalIdNumber string? -
- EmployeeXRefCode string? -
- FirstName string? -
- MiddleName string? -
- LastName string? -
- BirthDate string? -
- EmployeeResidentialAddress EmployeeAddress? -
dayforce: EmployeeVolunteerList
Fields
- EffectiveEnd string? -
- EffectiveStart string? -
- VolunteerList VolunteerList? -
- LastModifiedTimestamp string? -
dayforce: EmployeeVolunteerListCollection
Fields
- Items EmployeeVolunteerList[]? -
dayforce: EmployeeWorkAssignment
Fields
- Position Position? -
- Location Location? -
- EmploymentIndicator EmploymentIndicator? -
- EmploymentStatusReason EmploymentStatusReason? -
- EffectiveStart string? -
- EffectiveEnd string? -
- FlatAmount decimal? -
- FTE decimal? -
- IsPAPrimaryWorkSite boolean? -
- IsPrimary boolean? -
- IsStatutory boolean? -
- IsVirtual boolean? -
- BusinessTitle string? -
- JobSetLevel JobSetLevel? -
- LaborPercentage decimal? -
- LastModifiedTimeStamp string? -
- MultiJSalaryAllocationPercent decimal? -
- ParticipateInReciprocalTaxCalculation boolean? -
- TelecommuterPercentage decimal? -
- IsConvenienceOfEmployee boolean? -
- EndSecondaryWorkAssignments boolean? -
- PositionTerm PositionTerm? -
- PRBankAccountBranchAddress BankAccountBranchAddress? -
- WorkLocationOverride WorkLocationOverride? -
- Rank int? -
- JobRate decimal? -
- TipTypeGroup TipTypeGroup? -
- LedgerCode string? -
- PMPositionAssignment PMPositionAssignment? -
- JobClassificationGlobal JobClassificationGlobal? -
dayforce: EmployeeWorkAssignmentCollection
Fields
- Items EmployeeWorkAssignment[]? -
dayforce: EmployeeWorkAssignmentManager
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- TerminationDate string? -
- EmploymentStatusGroupXRefCode string? -
- ManagerXRefCode string? -
- ManagerName string? -
- ActiveEmployeePosition Position? -
- ActiveEmployeeLocation Location? -
- LastModifiedTimestamp string? -
dayforce: EmployeeWorkAssignmentManagerCollection
Fields
- Items EmployeeWorkAssignmentManager[]? -
dayforce: EmployeeWorkContract
Fields
- AverageNumOfDays decimal? -
- BaseHours decimal? -
- BaseComplementaryHours decimal? -
- ContractWorkPercent decimal? -
- CreateShiftOnHolidays boolean? -
- EndDate string? -
- FullTimeHours decimal? -
- StartDate string? -
- WorkContract WorkContract? -
- WorkPatternLengthDays Signed32? -
- WorkPatterns EmployeeWorkPatternCollection? -
- LastModifiedTimestamp string? -
dayforce: EmployeeWorkContractCollection
Fields
- Items EmployeeWorkContract[]? -
dayforce: EmployeeWorkLocationOverride
Fields
- LocationName string? -
- Description string? -
- ReferenceCode string? -
- AllowLegalEntityOverride boolean? -
- LocationAddressXRefCode string? -
- Address string? -
- Address2 string? -
- CityName string? -
- StateName string? -
- StateCode string? -
- PostalCode string? -
- County string? -
- CountryName string? -
- CountryCode string? -
- JeddTaxes string? -
- LegalEntityOverrideDetails LegalEntityOverride[]? -
dayforce: EmployeeWorkPattern
Fields
- NetHours decimal? -
- ShiftTimeBegin string? -
- ShiftTimeEnd string? -
- WorkPatternDayIndex Signed32? -
- Deparment Department? -
- Job Job? -
- ShiftType WorkPatternShiftType? -
- Location Location? -
- OrgLocationType OrgLocationType? -
- LastModifiedTimestamp string? -
dayforce: EmployeeWorkPatternCollection
Fields
- Items EmployeeWorkPattern[]? -
dayforce: EmploymentAgreementDetails
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentAgreementDuration
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentAgreementPopulation
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentAgreementSettlement
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentAgreementTaxRegime
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentAgreementType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentIndicator
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentStatus
Fields
- IsBenefitArrearsEnabled boolean? -
- EffectiveStartingPointOfDay string? -
- EmploymentStatusGroup EmploymentStatusGroup? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentStatusGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentStatusReason
Fields
- IsCompChangeReason boolean? -
- IsLeaveReason boolean? -
- IsPositionChangeReason boolean? -
- IsTerminationReason boolean? -
- IsVoluntaryReason boolean? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EmploymentTypeConfig
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EntitlementPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: EVerify
Fields
- EVerifyCaseNumber string? -
- EVerifyStatus string? -
- EVerifyStatusDate string? -
- EVerifyStatusDesc string? -
dayforce: ExtensibleProperty
Fields
- TypeCode string? -
- EntityType string? -
- CountryCode string? -
- EntityXRefCode string? -
- EntityName string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- Comment string? -
- SavedAt string? -
- SavedBy Signed32? -
- PropertyValues PropertyValue[]? -
dayforce: FederalFilingStatus
Fields
- CountryCode string? -
- FederalFilingStatusCode string? -
- CalculationCode string? -
- PayrollOutput string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: FileDetails
Fields
- ContentType string? -
- Data string? -
- FileName string? -
dayforce: FinancialInstitution
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: FLSAStatus
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: GenderIdentity
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: GenderIdentityCountryAware
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: GlobalProperty
Fields
- DataType Signed32? -
- EmployeeCardinality Signed32? -
- IsEditable boolean? -
- DataTypeParam string? -
- GenerateHREvent boolean? -
- Sequence Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: GlobalPropertyOption
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: GLSplitSetUpsert
Fields
- OrgUnitXRefCode string? -
- DeptJobXRefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- GLSplitSetDetails EmployeeGLSplitSetDetailUpsert[]? -
dayforce: HighlyCompensatedEmployeeCollection
Fields
- Items EmployeeHighlyCompensatedEmployeeIndicator[]? -
dayforce: HRConfigurationEntity
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: HRIncidentAction
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: HRIncidentBodyPart
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: HRIncidentInjury
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: HRIncidentType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: HRPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: I9Order
Fields
- I9OrderId string? -
- OrderStatusXRefCode string? -
- EVerify EVerify? -
dayforce: ImportSetModel
Fields
- ImportSetName string? -
- DataEntries DataEntryDeleteModel[]? -
dayforce: Job
Fields
- EmployeeEEO EmployeeEEO? -
- IsUnionJob boolean? -
- JobQualifications string? -
- JobRank Signed32? -
- JobSOC JobSOC? -
- JobUDFString1 string? -
- JobUDFString2 string? -
- JobUDFString3 string? -
- LedgerCode string? -
- NOC string? -
- JobClassification JobClassification? -
- JobFunction JobFunction? -
- PayGrade PayGrade? -
- Union DFUnion? -
- EffectiveEnd string? -
- EffectiveStart string? -
- FLSAStatus FLSAStatus? -
- JobFamily JobFamily? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: JobClassification
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: JobClassificationGlobal
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: JobFamily
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: JobFeed
Fields
- Title string? -
- Description string? -
- ClientSiteName string? -
- ClientSiteXRefCode string? -
- CompanyName string? -
- ParentCompanyName string? -
- JobDetailsUrl string? -
- ApplyUrl string? -
- AddressLine1 string? -
- AddressLine2 string? -
- City string? -
- State string? -
- Country string? -
- PostalCode string? -
- Education string? -
- JobFamily string? -
- JobFunction string? -
- EmploymentIndicator string? -
- Qualifications string? -
- DatePosted string? -
- LastUpdated string? -
- ReferenceNumber Signed32? -
- CandidatesUrl string? -
- CultureCode string? -
- ParentRequisitionCode Signed32? -
- MinHiringRate decimal? -
- MaxHiringRate decimal? -
- HiringRate decimal? -
- JobType Signed32? -
- TravelPercentage decimal? -
- TravelRequired Signed32? -
- TelecommutePercentage decimal? -
- IsVirtualLocation boolean? -
- QuestionnaireUrl string? -
- CandidateApplicationPostUrl string? -
dayforce: JobFunction
Fields
- Level Signed32? -
- UsableForExternalPosting boolean? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: JobPostingApplicantModel
Fields
- Locale string? -
- AppliedOnMillis int? -
- Job JobPostingResponseModel? -
- Applicant ApplicantDetails? -
- Questions QuestionsInResponse? -
- CandidateSource CandidateProfileSource? -
dayforce: JobPostingResponseModel
Fields
- JobId string? -
- JobTitle string? -
- JobCompany string? -
- JobLocation string? -
- JobUrl string? -
- JobMeta string? -
- JobRequisitionId string? -
dayforce: JobSet
Fields
- Grade Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: JobSetLevel
Fields
- JobSet JobSet? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: JobSOC
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: JobStepPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: KpiData
Fields
- OrgUnitXRefCode string? -
- KpiXRefCode string? -
- Day string? -
- Week string? -
- Minute string? -
- AggregateToDay boolean? -
- Value decimal? -
- AdjustedValue decimal? -
- Forma string? -
- Forecast string? -
- ZoneXRefCode string? -
- MdseXRefCode string? -
dayforce: KpiTargetPattern
Fields
- KpiXRefCode string? -
- OrgUnitXRefCode string? -
- ZoneXRefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- PatternOffset Signed32? -
- PatternLength Signed32? -
- Pattern KpiTargetPatternDetail[]? -
dayforce: KpiTargetPatternAssignment
Fields
- KpiXRefCode string? -
- OrgUnitXRefCode string? -
- ZoneXRefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- PatternOffset Signed32? -
dayforce: KpiTargetPatternDetail
Fields
- PatternIndex Signed32? -
- Value decimal? -
dayforce: KpiTargetPatternPOST
Fields
- Assignment KpiTargetPatternAssignment[]? -
- Pattern KpiTargetPatternDetail[]? -
dayforce: LaborCostResult
Fields
- LocationXRefCode string? -
- CalendarDate string? -
- Currency string? -
- Totals LaborCostTotalResult? -
- Intervals LaborCostTimeInterval[]? -
dayforce: LaborCostTimeInterval
Fields
- IntervalStartTime string? -
- IntervalEndTime string? -
- Totals LaborCostTotalResult? -
dayforce: LaborCostTotalResult
Fields
- LaborCost decimal? -
- LaborHours decimal? -
dayforce: LaborDemand
Fields
- OrgUnitXRefCode string? -
- SchedulePeriodStart string? -
- LaborDemands LaborDemandItem[]? -
dayforce: LaborDemandItem
Fields
- ZoneXRefCode string? -
- LaborMeasureXRefCode string? -
- ActivityXRefCode string? -
- AxisXRefCode string? -
- Day string? -
- Time string? -
- Hours decimal? -
- ServiceHours decimal? -
- NonServiceHours decimal? -
dayforce: LaborMetric
Fields
- CodeXRefCode string? -
- TypeXRefCode string? -
dayforce: LaborMetricCodes
Fields
- Name string? -
- Description string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LaborMetricsCodeXRefCode string? -
- ClockTransferCode string? -
- LedgerCode string? -
- OrgXRefCodes string[]? -
- LaborMetricsTypeXRefCode string? -
dayforce: LaborMetrics
Fields
- LaborMetricsTypeXRefCode string? -
- LaborMetricsCodeXRefCode string? -
dayforce: LaborMetricsCode
Fields
- LaborMetricsType LaborMetricsType? -
- ClockTransferCode string? -
- LedgerCode string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LaborMetricsCodePostPatchResponse
Fields
- LaborMetricsCodePostPatchResponseXRefCode string? -
dayforce: LaborMetricsCodesForSubmit
Fields
- ShortName string? -
- LongName string? -
- EffectiveFrom string? -
- EffectiveEnd string? -
- LaborMetricsCodeXRefCode string? -
- ClockTransferCode string? -
- LedgerCode string? -
- OrgUnitXRefCodes string[]? -
- LaborMetricsTypeXRefCode string? -
- IsDeleted boolean? -
dayforce: LaborMetricsType
Fields
- ClockTransferCode string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LaborMetricType
Fields
- Name string? -
- Description string? -
- ClockTransferCode string? -
- LaborMetricTypeXRefCode string? -
dayforce: LaborMetricTypeForSubmit
Fields
- ShortName string? -
- LongName string? -
- LaborMetricTypeXRefCode string? -
- ClockTransferCode string? -
- IsDeleted boolean? -
dayforce: LaborMetricTypePatchPostResponse
Fields
- LaborMetricTypePatchPostResponseXrefCode string? -
dayforce: LaborValidationFilters
Fields
- LaborValidationPolicyXRefCode string? -
- FilterXRefCodes LaborValidationMetricFilterXRefCodes[]? -
dayforce: LaborValidationMetricFilterXRefCodes
Fields
- Criteria LaborValidationModel[]? -
dayforce: LaborValidationModel
Fields
- CodeName string? -
- XRefCode record {}? -
dayforce: LaborValidationQualifier
Fields
- XRefCode string? -
- Name string? -
- Description string? -
- Active boolean? -
- Sequence Signed32? -
- CodeName string? -
- Models LaborValidationModel[]? -
- Children LaborValidationQualifier[]? -
dayforce: LaborValidationRule
Fields
- PolicyId Signed32? -
- PolicyXRefCode string? -
- XRefCode string? -
- Name string? -
- Description string? -
- EffectiveFrom string? -
- EffectiveTo string? -
- Active boolean? -
- CodeName string? -
- Sequence Signed32? -
- ValidationErrorMessage string? -
- SeverityLevel Signed32? -
- Models LaborValidationModel[]? -
- Children LaborValidationQualifier[]? -
- Qualifiers LaborValidationQualifier[]? -
dayforce: LastNamePrefix
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LegacyLaborMetricCodes
Fields
- Name string? -
- Description string? -
- LegacyLaborMetricType string? -
- LegacyLaborMetricXRefCode string? -
dayforce: LegacyLaborMetricForSubmit
Fields
- ShortName string? -
- LongName string? -
- LegacyLaborMetricType string? -
- LegacyLaborMetricXRefCode string? -
dayforce: LegacyLaborMetricPatchPostDeleteResponse
Fields
- XRefCode string? -
dayforce: LegalEntity
Fields
- Country Country? -
- LegalEntityAddress Address? -
- LegalIdNumber string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LegalEntityAddress
Fields
- AddressLine1 string? -
- AddressLine2 string? -
- City string? -
- State string? -
- Country string? -
- PostalCode string? -
dayforce: LegalEntityDEUShortTimeWork
Fields
- LegalEntityDEUShortTimeWorkID Signed32? -
- StoppageNumber string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LegalEntityMasterBankAccountSetting
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LegalEntityOverride
Fields
- LegalEntityName string? -
- LegalEntityXrefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- BankBranchAddressName string? -
- BankBranchAddressXRefCode string? -
- WorkSiteName string? -
- WorkSiteXRefCode string? -
- WorkSiteNumber string? -
- WorkSiteStateCode string? -
- OverrideCustomerFundingIdentiferName string? -
- OverrideCustomerFundingIdentiferXRefCode string? -
dayforce: LegalEntityWorkSiteState
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LimitAccessType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LimitType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LinkDetails
Fields
- Url string? -
dayforce: Links
Fields
- _Total Signed32? -
- Values LinkDetails[]? -
dayforce: ListValue
Fields
- ListValueId Signed32? - The Id of the list item. This value would be passed as the parameter value. Multiple Id's can be passed as a parameter value if comma separated.
- Name string? - The name that can be displayed in a list to the user.
dayforce: LMSAssignmentMethod
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LMSCertification
Fields
- EffectiveEnd string? -
- ExpirationUnit string? -
- ExpirationValue Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LMSCertificationStatus
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LMSEmployeeCertification
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- LMSCertification LMSCertification? -
- CertificationNumber string? -
- Course Course? -
- TrainingProgram TrainingProgram? -
- LMSAssignmentMethod LMSAssignmentMethod? -
- LMSCertificationStatus LMSCertificationStatus? -
- LastModifiedTimestamp string? -
dayforce: LMSEmployeeCertificationCollection
Fields
- Items LMSEmployeeCertification[]? -
dayforce: LMSEnrollmentStatus
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Location
Fields
- PhysicalLocation boolean? -
- BusinessPhone string? -
- ContactBusinessPhone string? -
- ContactCellPhone string? -
- PostalCode string? -
- CountryCode string? -
- OpeningDate string? -
- ClosingDate string? -
- ComparableLocation HRConfigurationEntity? -
- Department Department? -
- Zone Zone? -
- StartDayOfWeek Signed32? -
- GeoCity City? -
- Timezone TimeZone? -
- County string? -
- IsOrgManaged boolean? -
- IsMobileOrg boolean? -
- PublicName string? -
- ClockTransferCode string? -
- ContactEmail string? -
- ContactName string? -
- LedgerCode string? -
- StateCode string? -
- Address string? -
- Address2 string? -
- LegalEntity LegalEntity? -
- OrgUnitLegalEntities OrgUnitLegalEntityCollection? -
- OrgUnitParents OrgUnitParentCollection? -
- ChildOrgUnits ChildLocationCollection? -
- OrgUnitLocationTypes OrgUnitLocationTypeCollection? -
- OrgLevel OrgLevel? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LocationAddress
Fields
- Address1 string? -
- Address2 string? -
- PostalCode string? -
- State State? -
- Country Country? -
- City string? -
- County string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: LocationAddresses
Fields
- Description string? -
- Address string? -
- AddressLineTwo string? -
- ZipPostalCode string? -
- CountryName string? -
- StateProvince string? -
- City string? -
- County string? -
- IsTaxation boolean? -
- CountryCode string? -
- StateProvinceCode string? -
- LocationInJtEconomicDevDistAreaJedd boolean? -
- JeddTaxes string? -
- TaxAuthorityInstance string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ManagerAssignShiftBidDTO
Fields
- GroupXRefCode string -
- EmployeeXRefCode string -
dayforce: ManagerShiftBid
Fields
- GroupXRefCode string? -
- QualifyingBids int? -
- BiddingEmployees BidderInfo[]? -
- EmployeeScheduleXRefCodes string[]? -
- EmployeeSchedule EmployeeSchedule? -
dayforce: MaritalStatus
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: MealBreaks
Fields
- PunchXRefCode string? -
- Type string? -
- TimeStart string? -
- TimeEnd string? -
- TimeStartRaw string? -
- TimeEndRaw string? -
- NetHours decimal? -
- StartExceptionCode string? -
- EndExceptionCode string? -
- IsAutoInjected boolean? -
- LastModifiedTimestampUtc string? -
dayforce: MedicareLevyExemptionType
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: MetadataField
Fields
- OriginalId string? -
- ParentEntityType string? -
- Label string? -
- DbColumn string? -
- DataType string? -
- Validators AmfValidationModel[]? -
- PredefinedValues PredefinedValue[]? -
- EnableAdditionalAssumePreviousValueIfNullLogic boolean? -
- DfAuthorize AmfAuthorize? -
- Cfg AmfCfg? -
- EnumFrom AmfEnumFrom? -
- IsUniqueField boolean? -
- IsPublicKey boolean? -
- IsIndexKey boolean? -
- Id string? -
- Title string? -
- ShortDescription string? -
- LongDescription string? -
- MetadataId string? -
- DbTable string? -
- Name string? -
- Mappings record { string... }? -
- IsExtended boolean? -
- IsCommon boolean? -
- GeoContext ContextLevel? -
- SchemaName string? -
- ApplicationMapping string? -
- DayforcePropertyName string? -
dayforce: MetadataModel
Fields
- MetadataFields MetadataField[]? -
- MetadataModels MetadataModel[]? -
- MetadataModelCollections MetadataModelCollection[]? -
- Validators AmfValidationModel[]? -
- OnCUDActions AmfOnCUDActionModel[]? -
- CompositeFields string[]? -
- IsEffectiveDatedContiguous boolean? -
- Rules AmfRuleModel[]? -
- DfAuthorize AmfAuthorize? -
- Id string? -
- Title string? -
- ShortDescription string? -
- LongDescription string? -
- MetadataId string? -
- DbTable string? -
- Name string? -
- Mappings record { string... }? -
- IsExtended boolean? -
- IsCommon boolean? -
- GeoContext ContextLevel? -
- SchemaName string? -
- ApplicationMapping string? -
- DayforcePropertyName string? -
dayforce: MetadataModelCollection
Fields
- MetadataModel MetadataModel? -
- Id string? -
- Title string? -
- ShortDescription string? -
- LongDescription string? -
- MetadataId string? -
- DbTable string? -
- Name string? -
- Mappings record { string... }? -
- IsExtended boolean? -
- IsCommon boolean? -
- GeoContext ContextLevel? -
- SchemaName string? -
- ApplicationMapping string? -
- DayforcePropertyName string? -
dayforce: MilitaryServiceDetails
Fields
- ServiceCountry string? -
- Branch string? -
- Rank string? -
- StartDateMonth string? -
- StartDateYear string? -
- EndDateMonth string? -
- EndDateYear string? -
- EndCurrent boolean? -
- Commendations string? -
- Description string? -
dayforce: MilitaryServices
Fields
- _Total Signed32? -
- Values MilitaryServiceDetails[]? -
dayforce: NameAffix
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: OnboardingPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: OperatingHours
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- OrgUnitXRefCode string? -
- OperatingHours OperatingHoursItem[]? -
dayforce: OperatingHoursDayPattern
Fields
- SundayStartTime string? -
- SundayEndTime string? -
- MondayStartTime string? -
- MondayEndTime string? -
- TuesdayStartTime string? -
- TuesdayEndTime string? -
- WednesdayStartTime string? -
- WednesdayEndTime string? -
- ThursdayStartTime string? -
- ThursdayEndTime string? -
- FridayStartTime string? -
- FridayEndTime string? -
- SaturdayStartTime string? -
- SaturdayEndTime string? -
dayforce: OperatingHoursExceptionDay
Fields
- ExceptionName string? -
- ExceptionDescription string? -
- ExceptionStartDateTime string? -
- ExceptionEndDateTime string? -
- IsClosed string? -
- OperatingHoursRecurExceptionDetails OperatingHoursRecurExceptionDetails? -
dayforce: OperatingHoursExceptionPOST
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- OrgUnitXRefCode string? -
- OpHrsPatternAssignment int? -
- OpHrsPatternException int? -
- OperatingHoursExceptionDay OperatingHoursExceptionDay[]? -
- OperatingHoursDayPattern OperatingHoursDayPattern? -
dayforce: OperatingHoursItem
Fields
- Day string? -
- IsClosed Signed32? -
- NoDayPattern Signed32? -
- ShortName string? -
- LongName string? -
- OpenTime string? -
- CloseTime string? -
- IsException Signed32? -
- LastModifiedTimestamp string? -
- Client Signed32? -
dayforce: OperatingHoursRecurExceptionDetails
Fields
- RecurrenceType Signed32? -
- RecurrencePeriod Signed32? -
- RecurrenceValue string? -
- RecurrenceEndDate string? -
dayforce: OrgLevel
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: OrgLocationType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: OrgUnitDetailConfiguration
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- Address Address? -
- TaxLocationAddress LocationAddress? -
- ChildSortOrder Signed32? -
- IsPhysicalLocation boolean? -
- IsPrimary boolean? -
- LedgerCode string? -
- ParentSortOrder Signed32? -
- OrgLevel OrgLevel? -
- Timezone TimeZone? -
- OrgUnitParent OrgUnitParent? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: OrgUnitLegalEntity
Fields
- LegalEntity HRConfigurationEntity? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LegalEntityWorkSiteState LegalEntityWorkSiteState? -
- OverrideCustomerFundingIdentifier LegalEntityMasterBankAccountSetting? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: OrgUnitLegalEntityCollection
Fields
- Items OrgUnitLegalEntity[]? -
dayforce: OrgUnitLocationType
Fields
- LocationType OrgLocationType? -
- LastModifiedTimestamp string? -
dayforce: OrgUnitLocationTypeCollection
Fields
- Items OrgUnitLocationType[]? -
dayforce: OrgUnitParent
Fields
- ParentOrgUnit HRConfigurationEntity? -
- EffectiveStart string? -
- EffectiveEnd string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: OrgUnitParentCollection
Fields
- Items OrgUnitParent[]? -
dayforce: PaginatedPayload_EarningStatementHeader
Fields
- Data EarningStatementHeader? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_BackgroundJobLog
Fields
- Data BackgroundJobLog[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_BaseProject
Fields
- Data BaseProject[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_Course
Fields
- Data Course[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_DataEntry
Fields
- Data DataEntry[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_Employee
Fields
- Data Employee[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeeGLSplits
Fields
- Data EmployeeGLSplits[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeePayAdjustment
Fields
- Data EmployeePayAdjustment[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeePayrollElection
Fields
- Data EmployeePayrollElection[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeePaySummary
Fields
- Data EmployeePaySummary[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeePaySummaryRetro
Fields
- Data EmployeePaySummaryRetro[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeePunch
Fields
- Data EmployeePunch[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeeRawPunch
Fields
- Data EmployeeRawPunch[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeeSchedule
Fields
- Data EmployeeSchedule[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_EmployeeWorkLocationOverride
Fields
- Data EmployeeWorkLocationOverride[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_LaborValidationRule
Fields
- Data LaborValidationRule[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_LMSCertification
Fields
- Data LMSCertification[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_PolicyAssociation
Fields
- Data PolicyAssociation[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_Skill
Fields
- Data Skill[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_IEnumerable_TrainingProgram
Fields
- Data TrainingProgram[]? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_PersonManagementEraseHistory
Fields
- Data PersonManagementEraseHistory? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_PersonManagementExemption
Fields
- Data PersonManagementExemption? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_PersonManagementHistory
Fields
- Data PersonManagementHistory? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_Report
Fields
- Data Report? - This class encapsulates the report data generated during the execution of the report.
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: PaginatedPayload_String
Fields
- Data string? -
- ProcessResults ProcessResult[]? -
- Paging Paging? -
dayforce: Paging
Fields
- Next string? -
dayforce: ParameterAccessType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PatentDetails
Fields
- PatentNumber string? -
- Title string? -
- Url string? -
- DateMonth string? -
- DateYear string? -
- Description string? -
dayforce: Patents
Fields
- _Total Signed32? -
- Values PatentDetails[]? -
dayforce: PayAdjCode
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayAdjCodeGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayClass
Fields
- SortOrder Signed32? -
- DefaultNormalWeeklyHours decimal? -
- LedgerCode string? -
- PayClassGroup PayClassGroup? -
- PayClassFrequency PayClassFrequency? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayClassFrequency
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayClassGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Payee
Fields
- Name string? -
- Description string? -
- XRefCode string? -
- PayeeCategoryName string? -
- PayeeCategoryXRefCode string? -
- OperatingCountryCode string? -
- OperatingCountryName string? -
- Active boolean? -
- EarningPayee boolean? -
- DeductionPayee boolean? -
- SystemPayee boolean? -
- ElectronicServiceAddress string? -
- BusinessNumber string? -
- UniqueSuperIdentifier string? -
- Address PayeeAddress? -
- PaymentMethod PayeePaymentMethod? -
- HealthCareProvider PayeeHealthCareProvider? -
- PayeeParameters PayeeParameter[]? -
- StateCoverage PayeeStateCoverage[]? -
dayforce: PayeeAddress
Fields
- AddressLine3 string? -
- AddressLine1 string? -
- AddressLine2 string? -
- City string? -
- StateProvinceCode string? -
- StateProvinceName string? -
- CountryCode string? -
- CountryName string? -
- PostalCode string? -
dayforce: PayeeHealthCareProvider
Fields
- EffectiveFrom string? -
- EffectiveTo string? -
- HealthInsuranceType Signed32? -
- HealthCareBusinessNumber Signed32? -
- HealthCareSuccessorBusinessNumber Signed32? -
- AdditionalContributionPercentage decimal? -
- U1Percentage decimal? -
- U2Percentage decimal? -
- DataCollectionPoint Signed32? -
- ForwardingOffice Signed32? -
- InstitutionCode Signed32? -
dayforce: PayeeParameter
Fields
- PayeeParameterName string? -
- PayeeParameterDescription string? -
- PayeeParameterXRefCode string? -
- AccessTypeName string? -
- AccessTypeCode string? -
- ParameterTypeName string? -
- ParameterTypeXRefCode string? -
- Required boolean? -
- Pattern string? -
- MaxLength Signed32? -
- DataTypeCode string? -
dayforce: PayeePaymentMethod
Fields
- PaymentMethodXrefCode string? -
- PaymentMethodName string? -
- StatementMessage string? -
- PayableTo string? -
- CombineEmployee boolean? -
- CombineEarningAndDeduction boolean? -
- ShowEmployeeDetail boolean? -
- AllowNegativeAmounts boolean? -
- AccountNumber string? -
- TransitNumber string? -
- AccountTypeName string? -
- AccountTypeCode string? -
- BankName string? -
- BankNumber string? -
- SuppressPrintOfAdviceStatements boolean? -
- PaymentFrequencyName string? -
- PaymentFrequencyXRefCode string? -
- StatementLanguageCode string? -
- StatementLanguageName string? -
- BuildingSocietyNumber string? -
- PayeeCode string? -
- PayeeReferenceNumber string? -
- ScheduleStartDate string? -
- ScheduleEndDate string? -
- SchedulePayDate string? -
dayforce: PayeeStateCoverage
Fields
- StateCode string? -
- StateName string? -
- EffectiveStart string? -
- EffectiveEnd string? -
dayforce: PayFrequency
Fields
- PayFrequencyType string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayGrade
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayGroup
Fields
- PayFrequency PayFrequency? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayGroupBrief
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayGroupCalendar
Fields
- PayGroupXRefCode string? -
- PayGroupName string? -
- Frequency string? -
- PayrollFrequency string? -
- DayStartOfWeek string? -
- Country string? -
- CollectionFrequency string? -
- Calendar PayGroupCalendarDetails[]? -
dayforce: PayGroupCalendarDetails
Fields
- PeriodStartDate string? -
- PeriodEndDate string? -
- TimeCollectionStartDate string? -
- TimeCollectionEndDate string? -
- OffsetPayPeriodStartDate string? -
- OffsetPayPeriodEndDate string? -
- ApproveByDate string? -
- TransmitByDate string? -
- CommitByDate string? -
- PayrollCommitted boolean? -
- DataGateOpen string? -
- DataGateClose string? -
- ImpoundBy string? -
- PayDate string? -
- ContractPayDate string? -
- LedgerCode1 string? -
- LedgerCode2 string? -
- GLAccrualPercent decimal? -
- PayPeriod string? -
- PayPeriodSuffix string? -
- Locked boolean? -
- LockDate string? -
- LockedBy Signed32? -
- AutoLockDate string? -
- SupervisorDate string? -
- Transmitted boolean? -
- TransmittedDate string? -
- TransmittedBy Signed32? -
- Approved boolean? -
- ApprovedDate string? -
- ApprovedBy Signed32? -
- Closed boolean? -
- ClosedDate string? -
- ClosedBy Signed32? -
- ChecklistClosed boolean? -
- ChecklistClosedDate string? -
- ChecklistClosedBy Signed32? -
- ArrearsStart string? -
- ArrearsEnd string? -
- FuturePunchesEnabled boolean? -
dayforce: PayHolidayGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Payload_AnalyticsDatasetMetadata
Fields
- Data AnalyticsDatasetMetadata? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_AnalyticsDatasetMetadataResponse
Fields
- Data AnalyticsDatasetMetadataResponse? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_AnalyticsReportDataset
Fields
- Data AnalyticsReportDataset? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_AnalyticsReportMetadata
Fields
- Data AnalyticsReportMetadata? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_ArchiveDocument
Fields
- Data ArchiveDocument? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_BackgroundJobLog
Fields
- Data BackgroundJobLog? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_Boolean
Fields
- Data boolean? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_CandidateApplicationStatusUpdatesPaginationModel
Fields
- ProcessResults ProcessResult[]? -
dayforce: Payload_CarrierFeeds
Fields
- Data CarrierFeeds? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_ClientPayrollCountry
Fields
- Data ClientPayrollCountry? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_ContactInformationType
Fields
- Data ContactInformationType? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_Department
Fields
- Data Department? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_DocumentImportQueueResponse
Fields
- Data DocumentImportQueueResponse? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_Employee
Fields
- Data Employee? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_EmployeePunchPatchPostDeleteResponse
Fields
- ProcessResults ProcessResult[]? -
dayforce: Payload_EmployeeTimeAwayFromWorkForSubmit
Fields
- ProcessResults ProcessResult[]? -
dayforce: Payload_EmployeeVOE
Fields
- Data EmployeeVOE? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_EmployeeVOI
Fields
- Data EmployeeVOI? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_EmploymentStatus
Fields
- Data EmploymentStatus? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_EmploymentStatusReason
Fields
- Data EmploymentStatusReason? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_AnalyticsReportDefinitions
Fields
- Data AnalyticsReportDefinitions[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_AnalyticsReportMetadata
Fields
- Data AnalyticsReportMetadata[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_AuthorizationAssignment
Fields
- Data AuthorizationAssignment[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_ContactInformationType
Fields
- Data ContactInformationType[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_DeductionDefinition
Fields
- Data DeductionDefinition[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Department
Fields
- Data Department[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_DEUEmployeeAccidentInsurance
Fields
- Data DEUEmployeeAccidentInsurance[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_DEUEmployeeWageTax
Fields
- Data DEUEmployeeWageTax[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_DEUTaxSocialInsurance
Fields
- Data DEUTaxSocialInsurance[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Document
Fields
- Data Document[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EarningDefinition
Fields
- Data EarningDefinition[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Employee
Fields
- Data Employee[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeAssignedSexAndGenderIdentity
Fields
- Data EmployeeAssignedSexAndGenderIdentity[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeAUSFederalTax
Fields
- Data EmployeeAUSFederalTax[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeAUSSuperannuation
Fields
- Data EmployeeAUSSuperannuation[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeAUSSuperannuationRules
Fields
- Data EmployeeAUSSuperannuationRules[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeAvailability
Fields
- Data EmployeeAvailability[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeBadge
Fields
- Data EmployeeBadge[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeBalancePeriod
Fields
- Data EmployeeBalancePeriod[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeBalanceTransactions
Fields
- Data EmployeeBalanceTransactions[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeCANEmploymentInsuranceRate
Fields
- Data EmployeeCANEmploymentInsuranceRate[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeCANFederalTax
Fields
- Data EmployeeCANFederalTax[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeCANStateTax
Fields
- Data EmployeeCANStateTax[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeCANTaxStatus
Fields
- Data EmployeeCANTaxStatus[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeClockDeviceGroup
Fields
- Data EmployeeClockDeviceGroup[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeCompensation
Fields
- Data EmployeeCompensation[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeConfidentialIdentification
Fields
- Data EmployeeConfidentialIdentification[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeCourse
Fields
- Data EmployeeCourse[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeDefaultLabor
Fields
- Data EmployeeDefaultLabor[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeDependentBeneficiary
Fields
- Data EmployeeDependentBeneficiary[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeDirectDeposit
Fields
- Data EmployeeDirectDeposit[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeDisability
Fields
- Data EmployeeDisability[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeDocumentManagementSecurityGroup
Fields
- Data EmployeeDocumentManagementSecurityGroup[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeEmergencyContact
Fields
- Data EmployeeEmergencyContact[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeEmploymentAgreement
Fields
- Data EmployeeEmploymentAgreement[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeEmploymentStatus
Fields
- Data EmployeeEmploymentStatus[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeEmploymentType
Fields
- Data EmployeeEmploymentType[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeEthnicity
Fields
- Data EmployeeEthnicity[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeGlobalPropertyValue
Fields
- Data EmployeeGlobalPropertyValue[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeHealthWellness
Fields
- Data EmployeeHealthWellness[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeHighlyCompensatedEmployeeIndicator
Fields
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeHRIncident
Fields
- Data EmployeeHRIncident[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeHRPolicy
Fields
- Data EmployeeHRPolicy[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeIRLTaxEWSS
Fields
- Data EmployeeIRLTaxEWSS[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeIRLTaxPAYEExclusion
Fields
- Data EmployeeIRLTaxPAYEExclusion[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeIRLTaxPRSI
Fields
- Data EmployeeIRLTaxPRSI[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeIRLTaxRPN
Fields
- Data EmployeeIRLTaxRPN[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeLocation
Fields
- Data EmployeeLocation[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeManager
Fields
- Data EmployeeManager[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeMaritalStatus
Fields
- Data EmployeeMaritalStatus[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeOnboardingPolicy
Fields
- Data EmployeeOnboardingPolicy[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeOrgUnitInformation
Fields
- Data EmployeeOrgUnitInformation[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeePayAdjustCodeGroup
Fields
- Data EmployeePayAdjustCodeGroup[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeePayGradeRate
Fields
- Data EmployeePayGradeRate[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeePayrollElection
Fields
- Data EmployeePayrollElection[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeePayrollElectionDeletionModel
Fields
- Data EmployeePayrollElectionDeletionModel[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeePayrollTax
Fields
- Data EmployeePayrollTax[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeePayrollTaxParameter
Fields
- Data EmployeePayrollTaxParameter[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeePerformanceRating
Fields
- Data EmployeePerformanceRating[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeePropertyValue
Fields
- Data EmployeePropertyValue[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeRole
Fields
- Data EmployeeRole[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeSchedule
Fields
- Data EmployeeSchedule[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeSchedulePostAPIResponse
Fields
- Data EmployeeSchedulePostAPIResponse[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeShortTimeWork
Fields
- Data EmployeeShortTimeWork[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeSkill
Fields
- Data EmployeeSkill[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeSSOAccount
Fields
- Data EmployeeSSOAccount[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeTimeAwayFromWork
Fields
- Data EmployeeTimeAwayFromWork[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeTimeAwayFromWorkForSubmit
Fields
- Data EmployeeTimeAwayFromWorkForSubmit[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeTrainingProgram
Fields
- Data EmployeeTrainingProgram[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUKIrregularPaymentDetails
Fields
- Data EmployeeUKIrregularPaymentDetails[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUKNIDetails
Fields
- Data EmployeeUKNIDetails[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUKPostgraduateLoan
Fields
- Data EmployeeUKPostgraduateLoan[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUKStudentLoan
Fields
- Data EmployeeUKStudentLoan[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUKTaxDetails
Fields
- Data EmployeeUKTaxDetails[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUnionMembership
Fields
- Data EmployeeUnionMembership[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUSFederalTax
Fields
- Data EmployeeUSFederalTax[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUSStateTax
Fields
- Data EmployeeUSStateTax[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeUSTaxStatus
Fields
- Data EmployeeUSTaxStatus[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeVeteransStatus
Fields
- Data EmployeeVeteransStatus[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeVolunteerList
Fields
- Data EmployeeVolunteerList[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeWorkAssignment
Fields
- Data EmployeeWorkAssignment[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeWorkAssignmentManager
Fields
- Data EmployeeWorkAssignmentManager[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeWorkContract
Fields
- Data EmployeeWorkContract[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmployeeWorkLocationOverride
Fields
- Data EmployeeWorkLocationOverride[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmploymentStatus
Fields
- Data EmploymentStatus[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_EmploymentStatusReason
Fields
- Data EmploymentStatusReason[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_ExtensibleProperty
Fields
- Data ExtensibleProperty[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Job
Fields
- Data Job[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_JobFeed
Fields
- Data JobFeed[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_KpiData
Fields
- Data KpiData[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_KpiTargetPattern
Fields
- Data KpiTargetPattern[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_LaborValidationRule
Fields
- Data LaborValidationRule[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_LMSEmployeeCertification
Fields
- Data LMSEmployeeCertification[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Location
Fields
- Data Location[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_LocationAddresses
Fields
- Data LocationAddresses[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_ManagerShiftBid
Fields
- Data ManagerShiftBid[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Object
Fields
- Data record {}[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_PayClass
Fields
- Data PayClass[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Payee
Fields
- Data Payee[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_PayType
Fields
- Data PayType[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_PersonAddress
Fields
- Data PersonAddress[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_PersonContact
Fields
- Data PersonContact[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_PlanTarget
Fields
- Data PlanTarget[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Position
Fields
- Data Position[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_PRGLSplitSet
Fields
- Data PRGLSplitSet[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_ProcessResult
Fields
- Data ProcessResult[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_QuestionModel
Fields
- Data QuestionModel[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_ReportMetadata
Fields
- Data ReportMetadata[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_Skill
Fields
- Data Skill[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_TaxAuthorityInstanceDetails
Fields
- Data TaxAuthorityInstanceDetails[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_IEnumerable_UserPayAdjustCodeGroup
Fields
- Data UserPayAdjustCodeGroup[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_Job
Fields
- Data Job? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_LaborCostResult
Fields
- Data LaborCostResult? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_LaborDemand
Fields
- Data LaborDemand? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_LaborMetricCodes
Fields
- Data LaborMetricCodes? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_LaborMetricType
Fields
- Data LaborMetricType? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_LegacyLaborMetricCodes
Fields
- Data LegacyLaborMetricCodes? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_List_EarningStatementDocument
Fields
- Data EarningStatementDocument[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_List_Employee
Fields
- Data Employee[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_List_Payee
Fields
- Data Payee[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_List_ReportMetadata
Fields
- Data ReportMetadata[]? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_Location
Fields
- Data Location? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_Object
Fields
- Data record {}? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_OperatingHours
Fields
- Data OperatingHours? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_PayClass
Fields
- Data PayClass? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_PayGroupCalendar
Fields
- Data PayGroupCalendar? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_PayrollPayEntryImportHistory
Fields
- Data PayrollPayEntryImportHistory? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_PayRunStatus
Fields
- Data PayRunStatus? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_PayType
Fields
- Data PayType? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_Position
Fields
- Data Position? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_Projects
Fields
- Data Projects? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_String
Fields
- Data string? -
- ProcessResults ProcessResult[]? -
dayforce: Payload_SubordinateEntityReferences
Fields
- Data SubordinateEntityReferences? -
- ProcessResults ProcessResult[]? -
dayforce: PayMethod
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayPeriodInformation
Fields
- PayGroup PayGroupBrief? -
- PayPeriodStartDate string? -
- PayPeriodEndDate string? -
- OffsetPayPeriodStartDate string? -
- OffsetPayPeriodEndDate string? -
- LastModifiedTimestamp string? -
dayforce: PayPeriodInformationCollection
Fields
- Items PayPeriodInformation[]? -
dayforce: PayPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayrollCountry
Fields
- CountryName string? -
- CountryCode string? -
- HCMPayrollCountry boolean? -
- ConnectedPayCountry boolean? -
- IPSEnabled boolean? -
- PayGroup boolean? -
- DefaultTimeZoneName string? -
- DefaultTimeZoneXRefCode string? -
dayforce: PayrollDeduction
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: PayrollElection
Fields
- IsNew boolean? -
- ElectionXrefCode string? -
- Code string? -
- Type string? -
- TypeCode string? -
- EarningDeductionXRefCode string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- CreatedDate string? -
- LastModifiedTimestamp string? -
- Source string? -
- Schedule string? -
- ScheduleXRefCode string? -
- IsBlocked boolean? -
- IsOptOut Signed32? -
- OrgUnitXRefCode string? -
- DeptJobXRefCode string? -
- SourceTypeXRefCode string? -
- PayeePayableTo string? -
- DefaultPayee string? -
- DefaultPayeeXRefCode string? -
- PensionTracingNumber string? -
- IsCreatedByBenefits boolean? -
- ContinuePaymentOnStatutoryPay boolean? -
- ArrearMultipleLimitOption decimal? -
- EmployeeDeductionParams PayrollEmployeeDeductionParameter[]? -
- EmployeeEarningParams PayrollEmployeeEarningParameter[]? -
- EmployeeDeductionLimits PayrollEmployeeDeductionLimit[]? -
- EmployeeEarningLimits PayrollEmployeeEarningLimit[]? -
- EmployeePayeeEarnings PRPayeeEarning[]? -
- EmployeePayeeDeductions PRPayeeDeduction[]? -
- EmployeeDeductionPayeeParameters EmployeeDeductionPayeeParameter[]? -
- EmployeeEarningPayeeParameters EmployeeEarningPayeeParameter[]? -
- ExternalXrefCode string? -
dayforce: PayrollEmployeeDeductionLimit
Fields
- LimitAmount decimal? -
- LimitPercent decimal? -
- LimitTypeCodeName string? -
- ShortNameFormattedAmount string? -
- ShortNameFormattedPercent string? -
- PayGroupDefaultLimitAmount decimal? -
- PayGroupDefaultLimitPercent decimal? -
- PRDeductionLimitXRefCode string? -
- LastModifiedTimestamp string? -
dayforce: PayrollEmployeeDeductionParameter
Fields
- ParamCodeName string? -
- ShortName string? -
- EmployeeElectedValue decimal? -
- PayGroupDefaultValue decimal? -
- LastModifiedTimestamp string? -
dayforce: PayrollEmployeeEarningLimit
Fields
- LimitTypeCodeName string? -
- LimitAmount decimal? -
- LimitPercent decimal? -
- ShortNameFormattedAmount string? -
- ShortNameFormattedPercent string? -
- PayGroupDefaultLimitAmount decimal? -
- PayGroupDefaultLimitPercent decimal? -
- PREarningLimitXRefCode string? -
- LastModifiedTimestamp string? -
dayforce: PayrollEmployeeEarningParameter
Fields
- ParamCodeName string? -
- ShortName string? -
- EmployeeElectedValue decimal? -
- PayGroupDefaultValue decimal? -
- LastModifiedTimestamp string? -
dayforce: PayrollPayeeCategory
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayrollPayeeInfo
Fields
- IsActive boolean? -
- IsDeductionPayee boolean? -
- ABN string? -
- USI string? -
- ESA string? -
- AddressLine1 string? -
- AddressLine2 string? -
- AddressLine3 string? -
- City string? -
- State string? -
- PostalCode string? -
- CountryCode string? -
- Category PayrollPayeeCategory? -
- PaymentMethod PayrollPaymentMethod? -
- AccountNumber string? -
- BSBCode string? -
- CombineEmployees boolean? -
- CombineEarningsandDeductions boolean? -
- ShowEmployeeDetails boolean? -
- AllowNegativeAmounts boolean? -
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: PayrollPayEntryImportHistory
Fields
- PayGroupXRefCode string? -
- Currency string? -
- PeriodStart string? -
- PeriodEnd string? -
- PayDate string? -
- PPN string? -
- Imports PayrollPayEntryImportHistoryDetails[]? -
dayforce: PayrollPayEntryImportHistoryDetails
Fields
- Source string? -
- ImportSetName string? -
- ImportIdentifier string? -
- SourceFile string? -
- UploadedDateUTC string? -
- StartDate string? -
- FinishedDate string? -
- ErrorActionCode Signed32? -
- ErrorOccurActionName string? -
- ImportStatus string? -
- TotalRecordsIncluded Signed32? -
- TotalRecordsImported Signed32? -
- TotalInvalidRecords Signed32? -
- Errors PayrollPayEntryImportHistoryErrorDetails[]? -
dayforce: PayrollPayEntryImportHistoryErrorDetails
Fields
- LineNumber Signed32? -
- LineContent string? -
- ErrorMessage string? -
dayforce: PayrollPaymentMethod
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayrollPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayRunStatus
Fields
- PayGroupXRefCode string? -
- PayGroupName string? -
- PPN string? -
- PayDate string? -
- PeriodStartDate string? -
- PeriodEndDate string? -
- CountryCode string? -
- CountryName string? -
- Currency string? -
- Status string? -
- RejectionReason string? -
- RejectionReasonDescription string? -
- RejectionReasonXRefCode string? -
- RejectionReasonCategory string? -
- RejectionReasonCategoryXRefCode string? -
dayforce: PayType
Fields
- MaximumRate decimal? -
- MaximumSalary decimal? -
- SortOrder Signed32? -
- HidePayOnTimesheet boolean? -
- IsPayrollAutoPay boolean? -
- LedgerCode string? -
- PayTypeGroup PayTypeGroup? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PayTypeGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PensionTypeConfiguration
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PerformanceCycle
Fields
- CycleStartDate string? -
- CycleEndDate string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PerformanceRating
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PersonAddress
Fields
- Address1 string? -
- Address2 string? -
- Address3 string? -
- Address4 string? -
- Address5 string? -
- Address6 string? -
- City string? -
- PostalCode string? -
- Country Country? -
- State State? -
- ContactInformationType ContactInformationType? -
- IsPayrollMailing boolean? -
- DisplayOnTaxForm boolean? -
- DisplayOnEarningStatement boolean? -
- EffectiveStart string? -
- EffectiveEnd string? -
- County string? -
- LastModifiedTimestamp string? -
dayforce: PersonAddressCollection
Fields
- Items PersonAddress[]? -
dayforce: PersonContact
Fields
- ContactInformationType ContactInformationType? -
- ContactNumber string? -
- Country Country? -
- EffectiveEnd string? -
- EffectiveStart string? -
- ElectronicAddress string? -
- Extension string? -
- IsForSystemCommunications boolean? -
- IsPreferredContactMethod boolean? -
- IsUnlistedNumber boolean? -
- FormattedNumber string? -
- IsVerified boolean? -
- IsRejected boolean? -
- ShowRejectedWarning boolean? -
- NumberOfVerificationRequests Signed32? -
- LastModifiedTimestamp string? -
dayforce: PersonContactCollection
Fields
- Items PersonContact[]? -
dayforce: PersonManagementEraseHistory
Fields
- HireDate string? -
- TerminationDate string? -
- LastPayDate string? -
- PersonType string? -
- LastBenefitCoverageDate string? -
- LastDeptJob string? -
- LastOrgUnit string? -
- EmploymentStatusReason string? -
- EligibleForRehire string? -
- DisplayName string? -
- Reason string? -
- PurgeDate string? -
- Originator string? -
- EraseRequestDate string? -
- LastPayGroup string? -
- DayforceCreateDate string? -
- RequestId Signed32? -
dayforce: PersonManagementExemption
Fields
- AppliedByName string? -
- Country string? -
- PersonType string? -
- Name string? -
- Number string? -
- EmploymentStatus string? -
- AppliedOn string? -
dayforce: PersonManagementHistory
Fields
- PurgeDate string? -
- ValidationMessage Validation? -
- Policy string[]? -
- Name string? -
- RemovalAction string? -
- ApprovedBy string? -
- PersonType string? -
- TerminationDate string? -
dayforce: PFRatingScale
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PlanTarget
Fields
- PlanTargetXRefCode string? -
- Name string? -
- Description string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- TargetValue decimal? -
- KpiXRefCode string? -
- ZoneXRefCode string? -
- DaysOfWeek string[]? -
- OrgUnitXRefCodes string[]? -
- CreationOrgUnitXRefCode string? -
dayforce: PMPosition
Fields
- PositionGlobalId string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LedgerCode string? -
- Number string? -
- FTE decimal? -
- BusinessUnit BusinessUnit? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PMPositionAssignment
Fields
- PositionAssignmentGlobalId string? -
- EffectiveStart string? -
- EffectiveEnd string? -
- IsPrimary boolean? -
- PMPosition PMPosition? -
- LastModifiedTimestamp string? -
dayforce: PolicyAssociation
Fields
- Person string? -
- XRefCode string? -
- Number string? -
- StartDate string? -
- PersonType string? -
- Status string? -
- ExemptedFromDataMinimization boolean? -
- DataManagementCountryCode string? -
dayforce: Position
Fields
- AverageDailyHours decimal? -
- ClockTransferCode string? -
- Department Department? -
- Job Job? -
- EffectiveStart string? -
- EffectiveEnd string? -
- EmploymentIndicator EmploymentIndicator? -
- Executive boolean? -
- FTE decimal? -
- IsNonService boolean? -
- IsWCBExempt boolean? -
- LedgerCode string? -
- Officer boolean? -
- PayClass PayClass? -
- PayGroup PayGroup? -
- PayType PayType? -
- PositionTerm PositionTerm? -
- PPACAFullTime boolean? -
- SemiMonthlyBottomHours decimal? -
- SemiMonthlyTopHours decimal? -
- StandardCostRate decimal? -
- WeeklyHours decimal? -
- DefaultTargetBonus decimal? -
- PositionAssignments PositionAssignmentCollection? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PositionAssignment
Fields
- Location Location? -
- BudgetedHeadCount decimal? -
- EffectiveEnd string? -
- EffectiveStart string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PositionAssignmentCollection
Fields
- Items PositionAssignment[]? -
dayforce: PositionDetails
Fields
- Title string? -
- Company string? -
- Location string? -
- StartDateMonth string? -
- StartDateYear string? -
- EndDateMonth string? -
- EndDateYear string? -
- EndCurrent boolean? -
- Description string? -
dayforce: Positions
Fields
- _Total Signed32? -
- Values PositionDetails[]? -
dayforce: PositionTerm
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PREarningAccumulator
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: PredefinedValue
Fields
- XRefCode string? -
- FriendlyName string? -
dayforce: PRGLSplitSet
Fields
- Location Location? -
- Position Position? -
- EffectiveStart string? -
- EffectiveEnd string? -
- PRGLSplitSetDetails PRGLSplitSetDetailCollection? -
- LastModifiedTimestamp string? -
dayforce: PRGLSplitSetCollection
Fields
- Items PRGLSplitSet[]? -
dayforce: PRGLSplitSetDetail
Fields
- LaborPercentage decimal? -
- IsPrimary boolean? -
- PRGLSplitSetDetailMetricCodes PRGLSplitSetDetailMetricCodeCollection? -
- LastModifiedTimestamp string? -
dayforce: PRGLSplitSetDetailCollection
Fields
- Items PRGLSplitSetDetail[]? -
dayforce: PRGLSplitSetDetailMetricCode
Fields
- LaborMetricsCode LaborMetricsCode? -
- LastModifiedTimestamp string? -
dayforce: PRGLSplitSetDetailMetricCodeCollection
Fields
- Items PRGLSplitSetDetailMetricCode[]? -
dayforce: ProcessResult
Fields
- Code string? -
- Context string? -
- Level string? -
- Message string? -
dayforce: Project
Fields
- LedgerCode string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ProjectAssignmentType
Fields
- IsAssignedAll boolean? -
- XrefCodes string[]? -
dayforce: ProjectForSubmit
Fields
- ProjectXRefCode string? -
- ShortName string? -
- CreationOrgUnitXRefCodes string[]? -
- ClockTransferCode string? -
- ProjectPriority Signed32? -
- AccountNum string? -
- IFRSClassification boolean? -
- LongName string? -
- LedgerCode string? -
- CertifiedPayrollProjectNumber string? -
- ProjectClientXRefCode string? -
- ProjectTypeXRefCode string? -
- ProjectPhaseXRefCode string? -
- ProductGroupXRefCode string? -
- ProductModuleXRefCode string? -
- StartDate string? -
- DueDate string? -
- BudgetHours decimal? -
- BudgetAmount decimal? -
- EmployeeAssignment ProjectAssignmentType? -
- DeptJobAssignment ProjectAssignmentType? -
- DepartmentAssignment ProjectAssignmentType? -
- CompletedDate string? -
- PctComplete decimal? -
- IsDeleted boolean? -
- PayCodeXRefCodeChargeToHours ProjectAssignmentType? -
- PayCodeXRefCodeChargeToAmount ProjectAssignmentType? -
- ParentProjectXRefCode string? -
- TaxLocationAddressXRefCode string? -
- IsResidentAddressUsedForTaxation boolean? -
- IsCOEProject boolean? -
dayforce: Projects
Fields
- CreationOrgUnitXRefCodes string[]? -
- LongName string? -
- ClockTransferCode string? -
- ProjectPriority Signed32? -
- AccountNum string? -
- LedgerCode string? -
- IFRSClassification boolean? -
- CertifiedPayrollProjectNumber string? -
- CompletedDate string? -
- PctComplete decimal? -
- BudgetHours decimal? -
- BudgetAmount decimal? -
- ProjectClientXRefCode string? -
- ProjectTypeXRefCode string? -
- ProjectPhaseXRefCode string? -
- ProductGroupXRefCode string? -
- ProductModuleXRefCode string? -
- Deleted boolean? -
- TaxLocationAddressXRefCode string? -
- ChildProjectXRefCodes string[]? -
- EmployeeXRefCodes ProjectAssignmentType? -
- DeptJobXRefCodes ProjectAssignmentType? -
- DepartmentXRefCodes ProjectAssignmentType? -
- PayCodeXRefCodeChargeToHours ProjectAssignmentType? -
- PayCodeXRefCodeChargeToAmount ProjectAssignmentType? -
- IsResidentAddressUsedForTaxation boolean? -
- IsCOEProject boolean? -
- ProjectXRefCode string? -
- ShortName string? -
- ParentProjectXRefCode string? -
- StartDate string? -
- DueDate string? -
dayforce: ProjectsPostResponse
Fields
- ProjectsPostResponseXRefCode string? -
dayforce: PropertyValue
Fields
- ElementXRefCode string? -
- ElementName string? -
- IsElementActive boolean? -
- ElementDefaultValue string? -
- Value string? -
- SavedAt string? -
- SavedBy Signed32? -
dayforce: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
dayforce: PRPayeeDeduction
Fields
- LongName string? -
- PaymentMethodCode string? -
- PayeeXRefCode string? -
- ShortName string? -
- LastModifiedTimestamp string? -
dayforce: PRPayeeEarning
Fields
- ShortName string? -
- PayeeXRefCode string? -
- LongName string? -
- PaymentMethodCode string? -
- LastModifiedTimestamp string? -
dayforce: PRSIClass
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PRSIExemptReason
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: PRTaxResidentCode
Fields
- XRefCode string? -
- LastModifiedTimestamp string? -
- ShortName string? -
- LongName string? -
dayforce: PublicationDetails
Fields
- Title string? -
- Url string? -
- DateDay string? -
- DateMonth string? -
- DateYear string? -
- Description string? -
dayforce: Publications
Fields
- _Total Signed32? -
- Values PublicationDetails[]? -
dayforce: PunchExtraData
Fields
- DocketXRefCode string? -
- ProjectXRefCode string? -
- PositionXRefCode string? -
- LocationXRefCode string? -
- Quantity decimal? -
- MealWaiver string? -
- BreakAttestation boolean? -
- BioFailure boolean? -
- FaceVerificationFailure boolean? -
- LaborMetrics PunchLaborMetric[]? -
- DocketClockTransferCode string? -
- ProjectClockTransferCode string? -
- PositionClockTransferCode string? -
- LocationClockTransferCode string? -
dayforce: PunchLaborMetric
Fields
- TypeXRefCode string? -
- CodeXRefCode string? -
- TypeClockTransferCode string? -
- CodeClockTransferCode string? -
dayforce: PunchPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: QuestionAnswers
Fields
- Id string? -
- Value string? -
- Values string[]? -
dayforce: QuestionConditionModel
Fields
- id string? -
- value string? -
dayforce: QuestionHierarchicalOptionModel
Fields
- id string? -
- condition QuestionConditionModel? -
- options QuestionOptionModel[]? -
dayforce: QuestionModel
Fields
- id string? -
- 'type string? -
- question string? -
- text string? -
- options QuestionOptionModel[]? -
- required boolean? -
- format string? -
- 'limit Signed32? -
- min string? -
- max string? -
- condition QuestionConditionModel? -
- hierarchicalOptions QuestionHierarchicalOptionModel[]? -
- HTML boolean? -
- fontSize string? -
dayforce: QuestionOptionModel
Fields
- value string? -
- label string? -
dayforce: QuestionsInResponse
Fields
- Questions QuestionModel[]? -
- Url string? -
- RetrievedOnMillis int? -
- Answers QuestionAnswers[]? -
dayforce: RelationshipType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Report
This class encapsulates the report data generated during the execution of the report.
Fields
- XRefCode string? - Reference Code for the Report. This is a unique, human readable, code used to identify the Report.
- Rows record { string... }[]? - An array of Dictionary objects which contain the report data. Each dictionary object represents a row: the key is column name and the values is the column value for the row.
dayforce: ReportColumnMetadata
This class encapsulates the report data generated during the execution of the report.
Fields
- CodeName string? - CodeName is a unique name that can be used with code to refer to this specific column. Its value should be consistent.
- DisplayName string? - This is a name as defined by a user when developing this report. This name would be a human facing name that would be used on a printed report. This field is used as the key to the values dictionary
- DataType string? - This is a string representation of the data type.
dayforce: ReportMetadata
This class provides information about a report.
Fields
- Name string? - Name of the Report. The name will be localized, based on the Session Culture.
- Description string? - Description of the Report. The description will be localized, based on the Session Culture.
- XRefCode string? - Reference Code for the Report. This is a unique, human readable, code used to identify the Report.
- MaxRows Signed32? - Maximum number of rows the Report will return as part of it's ResultSet. If the MaxRows is assigned -1, it will execute to include all possible rows in it's ResultSet.
- ColumnMetadata ReportColumnMetadata[]? - An array of ReportColumnMetadata objects.
- Parameters ReportParameterMetadata[]? - Collection of report parameters defined by the report.
dayforce: ReportParameterMetadata
Report meta data that defines report parameters. Parameter values are supplied by the end-user for execution. All parameters must be supplied during execution. Not all Parameters have default value.
Fields
- Name string? - Name of the parameter.
- DisplayName string? - Human readable name of the parameter.
- ReportParameterMetadataId string? - Unique Id of parameter.
- DataType string? - Data type of the parameter.
- Operator string? - Operator type of the parameter, such as =, <, >=, etc... Not all parameters have operator type. For example, SQL Parameters don't have operators.
- DefaultValue string? - Default value that will be used if no other value is provided for the parameter. Not all parameters have default value. End-user needs to supply a value in here if the parameter doesn't have value. Reference values should contain Ids and be comma separated. E.g "1,2,3"
- IsRequired boolean? - True if a value needs to be supplied.
- AvailableValues ListValue[]? - List of available values a parameter can have.
dayforce: Response
Fields
- Identifier string? -
dayforce: Role
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: SafetyHealthType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ScheduleChangePolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: SchoolYear
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Segment
Fields
- TimeStart string? -
- TimeEnd string? -
- NetHours decimal? -
- DepartmentXRefCode string? -
- JobXRefCode string? -
- PositionXRefCode string? -
- OrgUnitXRefCode string? -
- OrgLocationTypeXRefCode string? -
- PayAdjCodeXRefCode string? -
- DocketXRefCode string? -
- ProjectXRefCode string? -
- Comment string? -
- LaborMetrics EmployeeScheduleSegmentLaborMetric[]? -
dayforce: ShiftRotation
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: ShiftTradePolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Skill
Fields
- SkillType SkillType? -
- SkillLevel SkillLevel[]? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: SkillLevel
Fields
- RankOrder Signed32? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Skills
Fields
- SkillXRefCode string? -
- SkillLevelXRefCode string? -
- IsMandatory boolean? -
dayforce: SkillType
Fields
- IsWFM boolean? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: State
Fields
- Name string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: StateFilingStatus
Fields
- CountryCode string? -
- StateCode string? -
- StateFilingStatusCode string? -
- CalculationCode string? -
- PayrollOutput string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: SubordinateEntityReferences
Fields
- EntityReferences string[]? -
dayforce: SuperannuationContributionCalculationType
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: SuperannuationContributionType
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: SuperannuationType
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: TaxAuthorityInstanceDetails
Fields
- Name string? -
- Description string? -
- EmployerTax boolean? -
- EmployeeTax boolean? -
- TaxAuthorityInstance string? -
- CountryCode string? -
dayforce: TaxProperty
Fields
- PropertyCodeName string? -
- PropertyValue string? -
- LastModifiedTimestamp string? -
dayforce: TaxPropertyCollection
Fields
- Items TaxProperty[]? -
dayforce: TFNExemptionReason
Fields
- PayrollShortName string? -
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: TimeOffPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: TimeZone
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: TipTypeGroup
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: TrainingProgram
Fields
- TrainingProgramCode string? -
- TrainingProgramCourse TrainingProgramCourse[]? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: TrainingProgramCourse
Fields
- Course Course? -
- EffectiveStart string? -
- EffectiveEnd string? -
- LastModifiedTimestamp string? -
dayforce: Transfers
Fields
- TimeStart string? -
- TimeStartRaw string? -
- LocationXRefCode string? -
- PositionXRefCode string? -
- ProjectXRefCode string? -
- DocketXRefCode string? -
- DocketQuantity decimal? -
- PayAdjustmentXRefCode string? -
- EmployeeComment string? -
- ManagerComment string? -
- LaborMetrics LaborMetrics[]? -
dayforce: UploadedBy
Fields
- DisplayName string? -
- XRefCode string? -
- LoginId string? -
dayforce: UserEmployeeAuthorityTypeAssignmentCollection
Fields
- Items AuthorizationAssignment[]? -
dayforce: UserPayAdjCodeGroupCollection
Fields
- Items UserPayAdjustCodeGroup[]? -
dayforce: UserPayAdjustCodeGroup
Fields
- PayAdjCodeGroup PayAdjCodeGroup? -
- LastModifiedTimestamp string? -
dayforce: Validation
Fields
- Warnings string[]? -
- Errors string[]? -
dayforce: VolunteerList
Fields
- EffectiveStart string? -
- EffectiveEnd string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: WithholdingVariationAmountType
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: WithholdingVariationType
Fields
- LastModifiedTimestamp string? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
dayforce: WorkContract
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: WorkContractPremiumPolicy
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: WorkLocationAddress
Fields
- AddressLine1 string? -
- AddressLine2 string? -
- City string? -
- State string? -
- Country string? -
- PostalCode string? -
dayforce: WorkLocationOverride
Fields
- County string? -
- Description string? -
- LocationName string? -
- LocationAddress LocationAddress? -
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: WorkPatternShiftType
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
dayforce: Zone
Fields
- XRefCode string? -
- ShortName string? -
- LongName string? -
- LastModifiedTimestamp string? -
Array types
dayforce: BackgroundScreeningPackageArr
BackgroundScreeningPackageArr
dayforce: BackgroundScreeningBillingCodeArr
BackgroundScreeningBillingCodeArr
dayforce: BackgroundScreeningStatusUrlReportArr
BackgroundScreeningStatusUrlReportArr
dayforce: BackgroundScreeningReportArr
BackgroundScreeningReportArr
dayforce: ExtensiblePropertyArr
ExtensiblePropertyArr
dayforce: JobFeedArr
JobFeedArr
Import
import ballerinax/dayforce;
Metadata
Released date: about 1 year ago
Version: 0.1.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.8.0
GraalVM compatible: Yes
Pull count
Total: 37
Current verison: 37
Weekly downloads
Keywords
HCM
Workforce Management
HRIS
Contributors
Other versions
0.1.0
Dependencies