constantcontact
Module constantcontact
API
Definitions
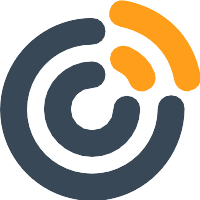
ballerinax/constantcontact Ballerina library
Overview
This is a generated connector for Constant Contact API v3 OpenAPI specification. The Constant Contact, Inc. V3 public API, for building integrations with Constant Contact, the leading small-business email marketing platform.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Constant Contact account
- Obtain tokens by following this guide
Quickstart
To use the Constant Contact connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/constantcontact
module into the Ballerina project.
import ballerinax/constantcontact;
Step 2: Create a new connector instance
Create a constantcontact:ClientConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
constantcontact:ClientConfig clientConfig = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET>, refreshUrl: <REFRESH_URL>, refreshToken: <REFRESH_TOKEN> } }; constantcontact:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get details about all contact lists for the account using the connector.
Return details about all contact lists
public function main() { constantcontact:Contacts|error response = baseClient->getContacts(); if (response is constantcontact:Contacts) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
constantcontact: Client
This is a generated connector for Constant Contact API v3 OpenAPI specification. The Constant Contact, Inc. V3 public API, for building integrations with Constant Contact, the leading small-business email marketing platform.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Constant Contact account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.cc.email/v3" - URL of the target service
getUserPrivileges
function getUserPrivileges() returns UserPrivilegesResource|error
GET User Privileges
Return Type
- UserPrivilegesResource|error - Request successful.
getAccountById
GET a Summary of Account Details
Parameters
- extraFields string? (default ()) - Use the
extra_fields
query parameter to include thephysical_address
and/orcompany_logo
details in the response body. Use a comma separated list to include both (physical_address, company logo).
putCustomerById
function putCustomerById(CustomerPut payload) returns CustomerPut|error
PUT (update) Account Details
Parameters
- payload CustomerPut - In the request body, specify changes to account details by including and modifying all or select
CustomerPut
properties. Changes to read-only fields (encoded_account_id
) are ignored.
Return Type
- CustomerPut|error - Request successful
getPhysicalAddress
function getPhysicalAddress() returns AccountPhysicalAddress|error
GET the Physical Address for the Account
Return Type
- AccountPhysicalAddress|error - Request successful
putPhysicalAddress
function putPhysicalAddress(AccountPhysicalAddress payload) returns AccountPhysicalAddress|error
PUT (update) the Physical Address for an Account
Parameters
- payload AccountPhysicalAddress - Include all
AccountPhysicalAddress
properties required for the specifiedcountry_code
and then update only those properties that you want to change. Excluding a non-read only field from the request body removes it from the physical address.
Return Type
- AccountPhysicalAddress|error - Request successful
retrieveEmailAddresses
function retrieveEmailAddresses(string? confirmStatus, string? roleCode, string? emailAddress) returns AccountEmails|error
GET a Collection of Account Email Addresses
Parameters
- confirmStatus string? (default ()) - Use the
confirm_status
query parameter to search for account emails using the email status. Possible values areCONFIRMED
orUNCONFIRMED
. You can also abbreviate the values of this query parameter and useC
orU
.
- roleCode string? (default ()) - Use the
role_code
query parameter to search for account emails that have a specific role. Each each email address in an account can have multiple roles or no role. Possible values areCONTACT
,BILLING
,REPLY_TO
,JOURNALING
, orOTHER
. You can also abbreviate the value of this query parameter and useC
,B
,R
,J
, orO
.
- emailAddress string? (default ()) - Use the
email_address
query parameter to search for a specific account email address.
Return Type
- AccountEmails|error - Request successful.
addAccountEmailAddress
function addAccountEmailAddress(AccountEmailInput payload) returns AccountEmailCreateResponse|error
POST Add an Account Email Address
Parameters
- payload AccountEmailInput - A JSON request payload containing the new email address you want to add to the Constant Contact account.
Return Type
- AccountEmailCreateResponse|error - Request successful.
getActivityStatusCollection
function getActivityStatusCollection(int 'limit, string? state) returns Activities|error
GET Activity Status Collection
Parameters
- 'limit int (default 50) - Specifies the number of results displayed per page of output, from 1 - 500, default = 50.
- state string? (default ()) - Use this parameter to filter the response to include only activities in one of the following states: cancelled, completed, failed, processing, or timed_out.
Return Type
- Activities|error - Request Successful
getActivity
GET an Activity Status
Parameters
- activityId string - The unique ID of the activity to GET
postContactsExport
function postContactsExport(ContactsExport payload) returns ActivityExportStatus|error
Export Contacts to a File
Parameters
- payload ContactsExport - A JSON payload that specifies the contacts (rows in the CSV file) and contact properties (columns in the CSV file) you want to export.
Return Type
- ActivityExportStatus|error - Request successful, queued for processing.
postContactDelete
function postContactDelete(ContactDelete payload) returns ActivityDeleteStatus|error
Delete Contacts in Bulk
Parameters
- payload ContactDelete - The request body contains an array of contact_ids <em>or</em> list_ids. All contact_ids provided are deleted, or all members of each specified list_id are deleted.
Return Type
- ActivityDeleteStatus|error - Request successful. Activity queued for processing.
contactsCSVImport
function contactsCSVImport(ActivitiesContactsFileImportBody payload) returns ActivityImport|error
Import Contacts using a CSV File
Parameters
- payload ActivitiesContactsFileImportBody - ContactsCSVImport request
Return Type
- ActivityImport|error - Request successful. Activity queued for processing.
bulkImportContactsJSON
function bulkImportContactsJSON(ContactsJsonImport payload) returns ActivityImport|error
Import Contacts using a JSON Payload
Parameters
- payload ContactsJsonImport - The JSON request payload that contains the contact data and contact lists for the import.
Return Type
- ActivityImport|error - Request successful. Activity queued for processing.
postListRemoveContact
function postListRemoveContact(ListActivityRemoveContacts payload) returns ActivityListsMembership|error
Remove Contacts from Lists
Parameters
- payload ListActivityRemoveContacts - The JSON payload used to create the 'remove contacts from lists' activity
Return Type
- ActivityListsMembership|error - Request successful. Activity queued for processing.
postListAddContact
function postListAddContact(ListActivityAddContacts payload) returns ActivityListsMembership|error
Add Contacts to Lists
Parameters
- payload ListActivityAddContacts - The JSON payload used to create the 'add contacts to lists' activity
Return Type
- ActivityListsMembership|error - Request successful. Activity queued for processing.
postTagRemoveContact
function postTagRemoveContact(TagAddRemoveContacts payload) returns ActivityTagging|error
Remove Tags from Contacts
Parameters
- payload TagAddRemoveContacts - The JSON payload used to create an asynchronous activity that removes tags from contacts meeting your specified contact filtering criteria.
Return Type
- ActivityTagging|error - Request successful. Activity queued for processing.
postTagAddContact
function postTagAddContact(TagAddRemoveContacts payload) returns ActivityTagging|error
Add Tags to Contacts
Parameters
- payload TagAddRemoveContacts - The JSON payload used to create an asynchronous activity that adds tags to contacts that meet your specified contact filtering criteria.
Return Type
- ActivityTagging|error - Request successful. Activity queued for processing.
postTagDelete
function postTagDelete(TagIdList500Limit payload) returns ActivityTagging|error
Delete Tags
Parameters
- payload TagIdList500Limit - An array of string values (
tag_id
s) to delete.
Return Type
- ActivityTagging|error - Request successful. Activity queued for processing.
getAccountSegments
function getAccountSegments(string 'limit, string sortBy) returns SegmentsDTO|error
GET all Segments
Parameters
- 'limit string (default "1000") - The number of segments to return on a page.
- sortBy string (default "date") - Specify the segment sort order to use. Sort by name (
sort_by=name
) in ascending order, or sort by date (sort_by=date
) in descending order with the most recently updated segments listed first.
Return Type
- SegmentsDTO|error - Request successful.
createSegment
function createSegment(SegmentData payload) returns SegmentDetail|error
POST (create) a Segment
Parameters
- payload SegmentData - The segment
name
andsegment_criteria
(requires single-string escaped JSON).
Return Type
- SegmentDetail|error - Segment successfully created.
getSegmentDetail
function getSegmentDetail(int segmentId) returns SegmentDetail|error
GET a Segment's Details
Parameters
- segmentId int - The system-generated unique ID that identifies a segment.
Return Type
- SegmentDetail|error - The segment was successfully returned.
updateSegment
function updateSegment(int segmentId, SegmentData payload) returns SegmentDetail|error
PUT (update) a Segment
Parameters
- segmentId int - The system generated ID that uniquely identifies the segment that you want to modify.
- payload SegmentData - Include both the
name
andsegment_criteria
(single-string escaped JSON) in the body request, then make updates to either or both.
Return Type
- SegmentDetail|error - The segment was successfully updated.
deleteSegment
DELETE a Segment
Parameters
- segmentId int - The system generated ID that uniquely identifies the segment.
updateSegmentName
function updateSegmentName(int segmentId, SegmentName payload) returns SegmentDetail|error
PATCH (rename) a Segment
Parameters
- segmentId int - The system generated ID that uniquely identifies the segment that you want to modify.
- payload SegmentName - Include the existing segment
name
in the body request, then rename the segment using a unique new name.
Return Type
- SegmentDetail|error - The segment name was successfully updated.
getContact
function getContact(string contactId, string? include) returns ContactResource|error
GET a Contact
Parameters
- contactId string - Unique ID of contact to GET
- include string? (default ()) - Use
include
to specify which contact sub-resources to include in the response. Use a comma to separate multiple sub-resources. Valid values:custom_fields
,list_memberships
,phone_numbers
,street_addresses
,notes
, andtaggings
.
Return Type
- ContactResource|error - Request successful
putContact
function putContact(string contactId, ContactPutRequest payload) returns ContactResource|error
PUT (update) a Contact
Parameters
- contactId string - Unique ID of contact to update
- payload ContactPutRequest - JSON payload defining the contact object, with updates. Any properties left blank or not included in the PUT payload are overwritten with null value - does not apply to contact subresources.
Return Type
- ContactResource|error - Contact resource has been updated
deleteContact
DELETE a Contact
Parameters
- contactId string - Unique ID of contact to DELETE
getContacts
function getContacts(string? status, string? email, string? lists, string? segmentId, string? tags, string? updatedAfter, string? include, boolean? includeCount, int 'limit) returns Contacts|error
GET Contacts Collection
Parameters
- status string? (default ()) - Use the
status
query parameter to search for contacts by status. This parameter accepts one or more comma separated values:all
,active
,deleted
,not_set
,pending_confirmation
,temp_hold
, andunsubscribed
.
- email string? (default ()) - Use the
email
query parameter to search for a contact using a specific email address.
- lists string? (default ()) - Use the
lists
query parameter to search for contacts that are members of one or more specified lists. Use a comma to separate multiplelist_id
values, up to a maximum of 25.
- segmentId string? (default ()) - Use to get contacts that meet the segment criteria for a single specified
segment_id
. This query parameter can only be combined with the limit query parameter. When using thesegment_id
query parameter, the V3 API may return a 202 response code instead of a 200 response. The 202 response code indicates that your request has been accepted, but not fully completed. Retry sending your API request to return the completed results and a 200 response code.
- tags string? (default ()) - Use to get contact details for up to 50 specified tags. Use a comma to separate each
tag_id
.
- updatedAfter string? (default ()) - Use
updated_after
to search for contacts that have been updated after the date you specify; accepts ISO-8601 formatted dates.
- include string? (default ()) - Use
include
to specify which contact sub-resources to include in the response. Use a comma to separate multiple sub-resources. Valid values:custom_fields
,list_memberships
,taggings
,notes
,phone_numbers
,street_addresses
.
- includeCount boolean? (default ()) - Set
include_count=true
to include the total number of contacts (contacts_count
) that meet all search criteria in the response body.
- 'limit int (default 50) - Specifies the number of results displayed per page of output in the response, from 1 - 500, default = 50.
createContact
function createContact(ContactPostRequest payload) returns ContactResource|error
POST (create) a Contact
Parameters
- payload ContactPostRequest - The JSON payload defining the contact
Return Type
- ContactResource|error - New contact successfully created
createOrUpdateContact
function createOrUpdateContact(ContactCreateOrUpdateInput payload) returns ContactCreateOrUpdateResponse|error
Create or Update a Contact
Parameters
- payload ContactCreateOrUpdateInput - A JSON request body payload that contains the contact resource you are creating or updating. The request body must contain the
email_address
property and thelist_memberships
array.
Return Type
- ContactCreateOrUpdateResponse|error - Contact successfully updated.
getContactIdXrefs
function getContactIdXrefs(string sequenceIds) returns ContactXrefs|error
GET a collection of V2 and V3 API contact IDs
Parameters
- sequenceIds string - Comma delimited list of V2 API contact
ids
to cross-reference with the V3 APIcontact_id
value. Endpoint accepts a maximum of 500 ids at a time.
Return Type
- ContactXrefs|error - Request successful
getCustomField
function getCustomField(string customFieldId) returns CustomFieldResource|error
GET a custom_field
Parameters
- customFieldId string - Unique ID of the
custom_field
on which to operate.
Return Type
- CustomFieldResource|error - Request successful
putCustomField
function putCustomField(string customFieldId, CustomFieldInput payload) returns CustomFieldResource|error
PUT (update) a custom_field
Parameters
- customFieldId string - Unique ID of the
custom_field
on which to operate.
- payload CustomFieldInput - The JSON payload used to update an existing custom field. Any properties omitted in the PUT request are overwritten with a null value.
Return Type
- CustomFieldResource|error - Success - custom_field updated
deleteCustomField
DELETE a custom_field
Parameters
- customFieldId string - Unique ID of the custom_field on which to operate.
getCustomFields
function getCustomFields(int 'limit) returns CustomFields|error
GET custom_fields Collection
Parameters
- 'limit int (default 50) - Specifies the number of results displayed per page of output, from 1 - 100, default = 50.
Return Type
- CustomFields|error - Request successful
postCustomFields
function postCustomFields(CustomFieldInput payload) returns CustomFieldResource|error
POST (create) a custom_field
Parameters
- payload CustomFieldInput - The JSON payload required to create a new
custom_field
Return Type
- CustomFieldResource|error - New custom_field successfully created
getList
function getList(string listId, string? includeMembershipCount) returns ContactList|error
GET a List
Parameters
- listId string - The system generated ID that uniquely identifies a contact list.
- includeMembershipCount string? (default ()) - Use to include the number of contact members per list by setting the
include_membership_count
to eitheractive
, to count only active contacts, orall
to include all contacts in the count.
Return Type
- ContactList|error - Request successful
putList
function putList(string listId, ListInput payload) returns ContactList|error
PUT (update) a List
Parameters
- listId string - Unique ID of the contact list to update
- payload ListInput - JSON payload containing updates to the specified contact list
Return Type
- ContactList|error - Request successful
deleteListActivity
function deleteListActivity(string listId) returns ActivityDeleteListResponse|error
DELETE a List
Parameters
- listId string - Unique ID of the list to delete
Return Type
- ActivityDeleteListResponse|error - Accepted
getLists
function getLists(int 'limit, boolean includeCount, string? includeMembershipCount) returns ContactListArray|error
GET Lists Collection
Parameters
- 'limit int (default 50) - Use to specify the number of results displayed per page of output, from 1 - 500, default = 50.
- includeCount boolean (default false) - Set
include_count
totrue
to return the total number of contact lists that meet your selection criteria.
- includeMembershipCount string? (default ()) - Use to include the number of contact members per list by setting the
include_membership_count
to eitheractive
, to count only active contacts, orall
to include all contacts in the count.
Return Type
- ContactListArray|error - Request successful
createList
function createList(ListInput payload) returns ContactList|error
POST (create) a List
Parameters
- payload ListInput - JSON payload defining the new contact list
Return Type
- ContactList|error - New list successfully created
getListIdXrefs
GET a collection of V2 and V3 API List IDs
Parameters
- sequenceIds string - Comma delimited list of V2 API list
ids
to cross-reference with the V3 APIlist_id
value. Endpoint accepts a maximum of 500 ids at a time.
getTag
GET Tag Details
Parameters
- tagId string - The ID that uniquely identifies a tag (UUID format).
- includeCount boolean (default false) - Use to include (
true
) or exclude (false
) the total number of tagged contacts (contacts_count
) from the results.
putTag
PUT (Update) a Tag
Parameters
- tagId string - The system generated ID used to uniquely identify the tag that you want to rename (UUID format).
- payload TagPut - The JSON payload used to update the tag name (
name
).
deleteTag
function deleteTag(string tagId) returns ActivityGeneric|error
DELETE a Tag
Parameters
- tagId string - The ID that uniquely identifies a tag in UUID format.
Return Type
- ActivityGeneric|error - The asynchronous request was successfully accepted. To view the results of the activity request, use the href link returned in the response.
getTags
GET Details for All Tags
Parameters
- 'limit int (default 50) - Use to specify the number of tag results (up to
500
) to display per page of output. The default is50
.
- includeCount boolean (default false) - Returns the total number of contacts (
contacts_count
) to which a tag applies.
postTag
POST (Create) a Tag
Parameters
- payload TagPost - The JSON payload to use to create a new tag.
retrieveEmailCampaignsUsingGET
function retrieveEmailCampaignsUsingGET(int 'limit, string? beforeDate, string? afterDate) returns PagedEmailCampaignResponse|error
GET a Collection of Email Campaigns
Parameters
- 'limit int (default 50) - Specifies the number of campaigns to display on each page of output that is returned (from return 1 - 500). The default returns 50 campaigns per page.
- beforeDate string? (default ()) - Use to return email campaigns with
updated_at
timestamps that are before a specific date and time (in ISO-8601 format). Use with theafter_date
query parameter to get email campaigns sent within a specific date range.
- afterDate string? (default ()) - Use to return email campaigns with last
updated_at
timestamps that are after a specific date and time (in ISO-8601 format). Use with thebefore_date
query parameter to get email campaigns sent within a specific date range.
Return Type
- PagedEmailCampaignResponse|error - Request successful.
createEmailCampaignUsingPOST
function createEmailCampaignUsingPOST(EmailCampaignComplete payload) returns EmailCampaign|error
POST (Create) a New Email Campaign
Parameters
- payload EmailCampaignComplete - A JSON request body that contains the email content.
Return Type
- EmailCampaign|error - Request successful.
retrieveEmailCampaignUsingGET
function retrieveEmailCampaignUsingGET(string campaignId) returns EmailCampaign|error
GET Details About a Single Email Campaign
Parameters
- campaignId string - The ID (UUID format) that uniquely identifies this email campaign.
Return Type
- EmailCampaign|error - Request successful.
removeEmailCampaignUsingDELETE
DELETE an Email Campaign
Parameters
- campaignId string - The unique ID for the email campaign you are deleting.
renameEmailCampaignUsingPATCH
function renameEmailCampaignUsingPATCH(string campaignId, EmailCampaignName payload) returns EmailCampaign|error
PATCH (Update) an Email Campaign Name
Parameters
- campaignId string - The unique identifier for an email campaign.
- payload EmailCampaignName - A JSON payload that contains the new email campaign name.
Return Type
- EmailCampaign|error - Request successful.
retrieveXrefMappingsUsingGET
function retrieveXrefMappingsUsingGET(string v2EmailCampaignIds) returns CrossReferenceResponse|error
GET a Collection of V2 and V3 API Email Campaign Identifiers
Parameters
- v2EmailCampaignIds string - Comma separated list of V2 API
campaignId
values. You can enter up to 50 V2campaignId
values in each request.
Return Type
- CrossReferenceResponse|error - Request successful.
retrieveEmailCampaignActivityUsingGET
function retrieveEmailCampaignActivityUsingGET(string campaignActivityId, string? include) returns EmailCampaignActivity|error
GET a Single Email Campaign Activity
Parameters
- campaignActivityId string - The unique ID for an email campaign activity.
- include string? (default ()) - Use the
include
query parameter to enter a comma separated list of additional email campaign activity properties for the V3 API to return. Valid values arephysical_address_in_footer
,permalink_url
,html_content
, anddocument_properties
.
Return Type
- EmailCampaignActivity|error - Request successful.
updateEmailCampaignActivityUsingPUT
function updateEmailCampaignActivityUsingPUT(string campaignActivityId, EmailCampaignActivity payload) returns EmailCampaignActivity|error
PUT (Update) An Email Campaign Activity
Parameters
- campaignActivityId string - The unique ID for the email campaign activity you are updating.
- payload EmailCampaignActivity - A request body payload that contains the complete email campaign activity with your changes.
Return Type
- EmailCampaignActivity|error - Email campaign activity successfully updated.
retrieveEmailSchedulesUsingGET
function retrieveEmailSchedulesUsingGET(string campaignActivityId) returns EmailScheduleResponse|error
GET an Email Campaign Activity Schedule
Parameters
- campaignActivityId string - The unique ID for an email campaign activity.
Return Type
- EmailScheduleResponse|error - Request successful.
scheduleEmailCampaignActivityUsingPOST
function scheduleEmailCampaignActivityUsingPOST(string campaignActivityId, EmailScheduleInput payload) returns EmailScheduleResponse|error
POST (Create) an Email Campaign Activity Schedule
Parameters
- campaignActivityId string - The unique ID for an email campaign activity. You can only schedule email campaign activities that have the
primary_email
role.
- payload EmailScheduleInput - A request body payload that contains the date that you want Constant Contact to send your email campaign activity on. Use
"0"
as the date to have Constant Contact immediately send the email campaign activity.
Return Type
- EmailScheduleResponse|error - Request successful.
unscheduleEmailCampaignActivityUsingDELETE
function unscheduleEmailCampaignActivityUsingDELETE(string campaignActivityId) returns Response|error
DELETE an Email Campaign Activity Schedule
Parameters
- campaignActivityId string - The unique ID for an email campaign activity.
Return Type
testSendCampaignActivityUsingPOST
function testSendCampaignActivityUsingPOST(string campaignActivityId, EmailTestSendInput payload) returns Response|error
POST Test Send an Email Campaign Activity
Parameters
- campaignActivityId string - The unique ID for an email campaign activity. You can only test send email campaign activities that have the
primary_email
role.
- payload EmailTestSendInput - A JSON request body that contains the recipients of the test email and an optional personal message.
Return Type
retrieveEmailCampaignActivityPreviewUsingGET
function retrieveEmailCampaignActivityPreviewUsingGET(string campaignActivityId) returns EmailCampaignActivityPreview|error
GET the HTML Preview of an Email Campaign Activity
Parameters
- campaignActivityId string - The unique ID for an email campaign activity.
Return Type
- EmailCampaignActivityPreview|error - Request successful.
retrieveEmailSendHistoryUsingGET
GET the Send History of an Email Campaign Activity
Parameters
- campaignActivityId string - The unique ID for an email campaign activity. You can return the send history for
primary_email
andresend
role email campaign activities.
Return Type
- EmailSendHistory|error - Request successful.
getContactTracking
function getContactTracking(string contactId, string trackingActivitiesList, string 'limit) returns ContactTrackingActivitiesPage|error
GET Contact Activity Details
Parameters
- contactId string - The contact id which is requesting tracking activity data.
- trackingActivitiesList string - The tracking activities list contains the tracking activity types to be specified as part of each request. The tracking activities list is a comma-delimited string containing one ore more tracking activity types. (such as em_sends, em_opens, em_forwards)
- 'limit string (default "100") - The number of tracking activities to return in a single page. Valid values are 1 to 100. Default is 100.
Return Type
- ContactTrackingActivitiesPage|error - successful operation
getContactOpenClickRate
function getContactOpenClickRate(string contactId, string 'start, string end) returns ContactOpenAndClickRates|error
GET Average Open and Click Rates
Parameters
- contactId string - The contact id which is requesting tracking activity data (e.g. aa9ff7b0-478d-11e6-8059-00163e3c8e19)
- 'start string - The starting date, in ISO 8601 format, to use to get campaigns. For example: 2019-01-01T00:00:00-0500.
- end string - The ending date, in ISO 8601 format, to use to get campaigns. For example: 2019-12-01T00:00:00-0500.
Return Type
- ContactOpenAndClickRates|error - Request Successful
getContactTrackingCount
function getContactTrackingCount(string contactId, string 'start, string end) returns ContactCampaignActivitiesSummary|error
GET Contact Action Summary
Parameters
- contactId string - The contact id which is requesting tracking activity data (e.g. aa9ff7b0-478d-11e6-8059-00163e3c8e19)
- 'start string - The starting date, in ISO 8601 format, to use to get campaigns. For example: 2019-01-01T00:00:00-0500.
- end string - The ending date, in ISO 8601 format, to use to get campaigns. For example: 2019-12-01T00:00:00-0500.
Return Type
- ContactCampaignActivitiesSummary|error - successful operation
getCampaignActivityLinkStats
function getCampaignActivityLinkStats(string campaignActivityId) returns EmailLinks|error
GET an Email Links Report
Parameters
- campaignActivityId string - The unique ID for an email campaign activity.
Return Type
- EmailLinks|error - Request was successful
getSends
function getSends(string campaignActivityId, string 'limit) returns SendsTrackingActivitiesPage|error
GET an Email Sends Report
Parameters
- campaignActivityId string - The unique ID for an email campaign activity to use for this report.
- 'limit string (default "500") - The number of tracking activities to return on a page.
Return Type
- SendsTrackingActivitiesPage|error - Request was successful
getOpens
function getOpens(string campaignActivityId, string 'limit) returns OpensTrackingActivitiesPage|error
GET an Email Opens Report
Parameters
- campaignActivityId string - The unique ID for an email campaign activity to use for this report.
- 'limit string (default "500") - The number of tracking activities to return on a page.
Return Type
- OpensTrackingActivitiesPage|error - Request was successful
getUniqueOpens
function getUniqueOpens(string campaignActivityId, string 'limit) returns OpensTrackingActivitiesPage|error
GET an Email Unique Opens Report
Parameters
- campaignActivityId string - The ID that uniquely identifies the email campaign activity to use for this report.
- 'limit string (default "500") - The number of tracking activities to return on a page.
Return Type
- OpensTrackingActivitiesPage|error - Request was successful
getDidNotOpens
function getDidNotOpens(string campaignActivityId, string 'limit) returns DidNotOpensTrackingActivitiesPage|error
GET an Email Did Not Opens Report
Parameters
- campaignActivityId string - The ID that uniquely identifies the email campaign activity to use for this report.
- 'limit string (default "500") - The number of tracking activities to return on a page.
Return Type
- DidNotOpensTrackingActivitiesPage|error - Request was successful
getClicks
function getClicks(string campaignActivityId, int? urlId, string 'limit) returns ClicksTrackingActivitiesPage|error
GET an Email Clicks Report
Parameters
- campaignActivityId string - The ID that uniquely identifies the email campaign activity to use for this report.
- urlId int? (default ()) - The ID that uniquely identifies a single link URL for which you want to get a clicks report.
- 'limit string (default "500") - The number of tracking activities to return on a page.
Return Type
- ClicksTrackingActivitiesPage|error - Request was successful
getForwards
function getForwards(string campaignActivityId, string 'limit) returns ForwardsTrackingActivitiesPage|error
GET an Email Forwards Report
Parameters
- campaignActivityId string - The ID that uniquely identifies the email campaign activity to use for this report.
- 'limit string (default "500") - The number of tracking activities to return on a page.
Return Type
- ForwardsTrackingActivitiesPage|error - Request was successful
getOptouts
function getOptouts(string campaignActivityId, string 'limit) returns OptoutsTrackingActivitiesPage|error
GET an Email Opt-outs Report
Parameters
- campaignActivityId string - The ID that uniquely identifies the email campaign activity to use for this report.
- 'limit string (default "500") - The number of tracking activities to return on a page.
Return Type
- OptoutsTrackingActivitiesPage|error - Request was successful
getBounces
function getBounces(string campaignActivityId, string[]? bounceCode, string 'limit) returns BouncesTrackingActivitiesPage|error
GET an Email Bounces Report
Parameters
- campaignActivityId string - The ID that uniquely identifies the email campaign activity to use for this report.
- bounceCode string[]? (default ()) - To return results for a specific bounce code, select the
bounce_code
from the drop-down list. To return results for multiple codes, repeat the bounce code parameter for each. For example, to return results for bounce codesB
andD
usebounce_code=B&bounce_code=D
.
- 'limit string (default "500") - The number of tracking activities to return on a page.
Return Type
- BouncesTrackingActivitiesPage|error - Request was successful
getAllBulkEmailCampaignSummaries
function getAllBulkEmailCampaignSummaries(string 'limit) returns BulkEmailCampaignSummariesPage|error
GET an Email Campaigns Summary Report
Parameters
- 'limit string (default "50") - Use the
limit
query parameter to limit the number of email campaign summaries to return on a single page. The default is50
and the maximum is500
per page.
Return Type
- BulkEmailCampaignSummariesPage|error - Request was successful.
getEmailSummary
function getEmailSummary(string campaignIds) returns CampaignStatsQueryResultEmail|error
GET an Email Campaign Stats Report
Parameters
- campaignIds string - A comma-separated list of
campaign_id
s (UUID's).
Return Type
- CampaignStatsQueryResultEmail|error - Request was successful.
getEmailCampaignActivitySummary
function getEmailCampaignActivitySummary(string campaignActivityIds) returns CampaignActivityStatsQueryResultEmail|error
GET an Email Campaign Activity Stats Report
Parameters
- campaignActivityIds string - A comma-separated list of
campaign_activity_id
s (UUID's).
Return Type
- CampaignActivityStatsQueryResultEmail|error - Request was successful.
getPartnerSiteOwners
function getPartnerSiteOwners(string? offset, string 'limit) returns PartnerAccount|error
GET Partner Client Accounts
Parameters
- offset string? (default ()) - Depending on the
limit
you specify, the system determines theoffset
parameter to use (number of records to skip) and includes it in the link used to get the next page of results
- 'limit string (default "50") - The number of client accounts to return on each page of results. The default value is
50
. Entering alimit
value less than the minimum (10
) or greater than the maximum (50
) is ignored and the system uses the default values. Depending on thelimit
you specify, the system determines theoffset
parameter to use (number of records to skip) and includes it in the link used to get the next page of results.
Return Type
- PartnerAccount|error - Request successful.
provision
function provision(Provision payload) returns ProvisionResponse|error
POST (create) a Partner Client Account
Parameters
- payload Provision - Create a new Constant Contact client account under your partner account. All required properties must be included in the JSON payload request.
Return Type
- ProvisionResponse|error - Request successful.
getPlan
function getPlan(string encodedAccountId) returns PlanTiersObject|error
GET Billing Plan Details for a Client Account
Parameters
- encodedAccountId string - Specify the client's unique
encoded_account_id
.
Return Type
- PlanTiersObject|error - Request successful.
setPlan
function setPlan(string encodedAccountId, PlanInfo payload) returns PlanTiersObject|error
PUT (update) Billing Plan Details for a Client Account
Parameters
- encodedAccountId string - Specify the client's unique
encoded_account_id
.
- payload PlanInfo - Update the billing plan (
plan_type
) for an existing Constant Contact client account. Options include:
Return Type
- PlanTiersObject|error - Request successful.
cancelAccount
function cancelAccount(string encodedAccountId, AccountCancellation payload) returns AccountCancellation|error
PUT Cancel the Billing Plan for a Client Account
Parameters
- encodedAccountId string - The system generated ID that uniquely identifies the client account.
- payload AccountCancellation - By default, the current date and time is automatically used as the cancellation date. However, you can specify a future date and time to cancel the account (
effective_date
) in the request body in ISO format. You can also enter the client's cancellation reason (reason_id
).
Return Type
- AccountCancellation|error - Request successful
getWebhooksCollection
function getWebhooksCollection() returns WebhooksSubscriptionCollection|error
GET a Collection of Webhook Topic Subscriptions
Return Type
- WebhooksSubscriptionCollection|error - Request successful
getWebhooksTopic
function getWebhooksTopic(string topicId) returns WebhooksSubscriptionResponse|error
GET Webhook Topic Subscription
Parameters
- topicId string - Identifies a webhook topic.
Return Type
- WebhooksSubscriptionResponse|error - Request successful
putWebhooksTopic
function putWebhooksTopic(string topicId, WebhooksSubscriptionBody payload) returns WebhooksSubscriptionPutResp|error
PUT Webhook Topic Subscription
Parameters
- topicId string - Identifies a webhook topic.
- payload WebhooksSubscriptionBody - A JSON payload containing a callback URI. Constant Contact uses this callback URI to notify you about your chosen topic.
Return Type
- WebhooksSubscriptionPutResp|error - Request successful.
deleteWebhooksSubscriptions
DELETE Webhook Topic Subscriptions
Parameters
- topicId string - Identifies a webhook topic.
testSendWebhooksTopic
function testSendWebhooksTopic(string topicId) returns WebhooksTestSend|error
POST Test Send Webhook Notification
Parameters
- topicId string - Identifies a webhook topic.
Return Type
- WebhooksTestSend|error - Test notification successfully sent to your callback URI.
postAuthCode
function postAuthCode(AuthToken payload) returns AuthInfoResponse|error
Access Token Information
Parameters
- payload AuthToken - A JSON request payload containing a valid OAuth2.0 access token.
Return Type
- AuthInfoResponse|error - Request Successful
getAuthCode
function getAuthCode(string responseType, string clientId, string redirectUri) returns Response|error
V3 Authorization service endpoint for OAuth 2.0 flows
Parameters
- responseType string - Specifies the type requested from the authorization service. For server flow set to code; for client flow set to token.
- clientId string - The API key for the client.
- redirectUri string - Tells the Authorization service where to send the user once access is granted. This must be the same redirect_uri associated with your_API_key
Records
constantcontact: AccountCancellation
Specifies the date and time a client requests to cancel their Constant Contact account and changes the account billing_status
to Canceled
. By default, this is the current date and time in ISO format. In the request body, you can optionally specify a future cancellation date (in ISO format) and a reason (reason_code
) that the client wants to cancel their account.
Fields
- reason_id int? - Specifies the reason that the client is canceling their Constant Contact account as follows: <ul> <li><code>1</code> Cost Too High</li> <li><code>2</code> Using A Competitive Service</li> <li><code>3</code> Not Doing Email Marketing</li> <li><code>11</code> Something Missing Or Not Working </li> <li><code>12</code> Doing It In-House</li> <li><code>14</code> Poor Results</li> <li><code>21</code> Too Difficult To Use</li> <li><code>27</code> Canceled Online by Customer</li> <li><code>30</code> Dissatisfied With Billing Policies</li> </ul>
- effective_date string? - The client account cancellation date and time in ISO-8601 format.
constantcontact: AccountEmailCreateResponse
Fields
- email_address string? - An email address associated with a Constant Contact account owner.
- email_id int? - The unique ID for an email address in a Constant Contact account.
- confirm_status string? - The confirmation status of the account email address. When you add a new email address to an account, Constant Contact automatically sends an email to that address with a link to confirm it. You can use any account email address with a <code>CONFIRMED</code> status to create an email campaign.
constantcontact: AccountEmailInput
Fields
- email_address string? - The new email address you want to add to a Constant Contact account.
constantcontact: AccountemailsInner
Fields
- email_address string? - An email address associated with a Constant Contact account owner.
- email_id int? - The unique ID for an email address in a Constant Contact account.
- confirm_status string? - The confirmation status of the account email address. When you add a new email address to an account, Constant Contact automatically sends an email to that address with a link to confirm it. You can use any account email address with a <code>CONFIRMED</code> status to create an email campaign.
- confirm_time string? - The date that the email address changed to <code>CONFIRMED</code> status in ISO-8601 format.
- confirm_source_type string? - Describes who confirmed the email address. Valid values are: <ul> <li>SITE_OWNER — The Constant Contact account owner confirmed the email address.</li> <li>SUPPORT — Constant Contact support staff confirmed the email address.</li> <li>FORCEVERIFY — Constant Contact confirmed the email address without sending a confirmation email.</li> <li>PARTNER — A Constant Contact partner confirmed the email address.</li> </ul>
- roles string[]? - Specifies the current role of a confirmed email address in an account. Each email address can have multiple roles or no role. Possible role values are: <ul> <li>CONTACT — The contact email for the Constant Contact account owner. Each account can only have one <code>CONTACT</code> role email.</li> <li>BILLING — The billing address for the Constant Contact account. Each account can only have one <code>BILLING</code> role email.</li> <li>JOURNALING — An email address that Constant Contact forwards all sent email campaigns to as part of the partner journaling compliance feature.</li> <li>REPLY_TO — The contact email used in the email campaign signature. Each account can only have one <code>REPLY_TO</code> role email.</li> <li>OTHER — An email address that does not fit into the other categories.</li> </ul> You can use any confirmed email address in the email campaign <code>from_email</code> and <code>reply_to_email</code> headers.
- pending_roles string[]? - The planned role for an unconfirmed email address. Possible role values are: <ul> <li>CONTACT — The contact email for the Constant Contact account owner. Each account can only have one <code>CONTACT</code> role email.</li> <li>BILLING — The billing address for the Constant Contact account. Each account can only have one <code>BILLING</code> role email.</li> <li>JOURNALING — The email address that Constant Contact forwards all sent email campaigns to as part of the partner journaling compliance feature.</li> <li>REPLY_TO — The contact email used in the email campaign signature. Each account can only have one <code>REPLY_TO</code> role email. </li> <li>OTHER — An email address that does not fit into the other categories.</li> </ul>
constantcontact: AccountPhysicalAddress
Fields
- address_line1 string - Line 1 of the organization's street address.
- address_line2 string? - Line 2 of the organization's street address.
- address_line3 string? - Line 3 of the organization's street address.
- city string - The city where the organization is located.
- state_code string? - The two letter ISO 3166-1 code for the organization's state and only used if the <code>country_code</code> is <code>US</code> or <code>CA</code>. If not, exclude this property from the request body.
- state_name string? - Use if the state where the organization is physically located is not in the United States or Canada. If <code>country_code</code> is <code>US</code> or <code>CA</code>, exclude this property from the request body.
- postal_code string? - The postal code address (ZIP code) of the organization. This property is required if the <code>state_code</code> is <code>US</code> or <code>CA</code>, otherwise exclude this property from the request body.
- country_code string - The two letter <a href='https://en.wikipedia.org/wiki/ISO_3166-1' target='_blank'>ISO 3166-1 code</a> for the organization's country.
constantcontact: Activities
Fields
- activities ActivitiesActivities[]? - A list of bulk activity jobs and status submitted by the account over the past 10 days.
- _links PagingLinks? - Paging links
constantcontact: ActivitiesActivities
Generic bulk activity status response object
Fields
- activity_id string? - Unique ID for the activity.
- state string? - The state of the request: initialized - request has been received processing - request is being processed completed - job completed cancelled - request was cancelled failed - job failed to complete timed_out - the request timed out before completing
- started_at string? - Timestamp showing when we began processing the activity request, in ISO-8601 format.
- completed_at string? - Timestamp showing when we completed processing the activity, in ISO-8601 format.
- created_at string? - Timestamp showing when we created the activity, in ISO-8601 format.
- updated_at string? - Timestamp showing when we last updated the activity, in ISO-8601 format.
- source_file_name string? - Name of the file used for an add_contacts activity.
- percent_done int? - Shows the percent done for an activity that we are still processing.
- activity_errors string[]? - Array of messages describing the errors that occurred.
- status ActivityStatus? - Activity status
- _links ActivityStatusExportLink? - Activity status export link
constantcontact: ActivitiesContactsFileImportBody
Fields
- file string - The CSV file you are importing. The column headings that you can use in the file are:
first_name
,last_name
,email
,phone
,job_title
,anniversary
,birthday_day
,birthday_month
,company_name
,street
,street2
,city
,state
,zip
, andcountry
. The only required column heading isemail
. You can also use custom fields as column headings. Enter the custom field name prefixed withcf:
as the column heading. For example, usecf:first_name
as the header name if you have a custom field named "first_name". The custom field must already exist in the Constant Contact account you are using. Depending on the custom field data type, you can enter dates or strings as the value of the custom field. Each contact can contain up to 25 different custom fields.
- list_ids string[] - Specify which contact lists you are adding all imported contacts to as an array of up to 50 contact
list_id
values.
constantcontact: Activity
Generic bulk activity status response object
Fields
- activity_id string? - Unique ID for the activity.
- state string? - The state of the request: initialized - request has been received processing - request is being processed completed - job completed cancelled - request was cancelled failed - job failed to complete timed_out - the request timed out before completing
- started_at string? - Timestamp showing when we began processing the activity request, in ISO-8601 format.
- completed_at string? - Timestamp showing when we completed processing the activity, in ISO-8601 format.
- created_at string? - Timestamp showing when we created the activity, in ISO-8601 format.
- updated_at string? - Timestamp showing when we last updated the activity, in ISO-8601 format.
- source_file_name string? - Name of the file used for an add_contacts activity.
- percent_done int? - Shows the percent done for an activity that we are still processing.
- activity_errors string[]? - Array of messages describing the errors that occurred.
- status ActivityStatus? - Activity status
- _links ActivityStatusExportLink? - Activity status export link
constantcontact: ActivityDeleteListResponse
Fields
- activity_id string? - Unique ID for the delete list batch job
- state string? - The state of the delete list request: processing - request is being processed completed - job completed cancelled - request was cancelled failed - job failed to complete timed_out - the request timed out before completing
- created_at string? - Date and time that the request was received, in ISO-8601 formmat.
- updated_at string? - Date and time that the request status was updated, in ISO-8601 format.
- percent_done int? - Job completion percentage
- activity_errors string[]? - Array of messages describing the errors that occurred.
- _links ActivitydeletelistresponseLinks? -
constantcontact: ActivitydeletelistresponseLinks
Fields
- self ActivitydeletelistresponseLinksSelf? - Link to the activity status to use in tracking the request status.
constantcontact: ActivitydeletelistresponseLinksSelf
Link to the activity status to use in tracking the request status.
Fields
- href string? - Link to the activity status to use in tracking the request
constantcontact: ActivityDeleteStatus
Activity status for contact_delete activity
Fields
- activity_id string? - Unique ID for the activity.
- state string? - The state of the request: initialized - request has been received processing - request is being processed completed - job completed cancelled - request was cancelled failed - job failed to complete timed_out - the request timed out before completing
- started_at string? - Timestamp showing when we began processing the activity request, in ISO-8601 format.
- completed_at string? - Timestamp showing when we completed processing the activity, in ISO-8601 format.
- created_at string? - Timestamp showing when we created the activity, in ISO-8601 format.
- updated_at string? - Timestamp showing when we last updated the activity, in ISO-8601 format.
- percent_done int? - Shows the percent done for an activity that we are still processing.
- activity_errors string[]? - Array of messages describing the errors that occurred.
- status ActivitydeletestatusStatus? - Status
- _links ActivitydeletestatusLinks? - Link to the activity status (this object) to retrieve the activity status.
constantcontact: ActivitydeletestatusLinks
Link to the activity status (this object) to retrieve the activity status.
Fields
- self ActivitydeletestatusLinksSelf? - Link to the activity status (this object) to retrieve the activity status.
constantcontact: ActivitydeletestatusLinksSelf
Link to the activity status (this object) to retrieve the activity status.
Fields
- href string? - Link to the activity status (this object)
constantcontact: ActivitydeletestatusStatus
Status
Fields
- list_count int? - The number of lists if request specifies contacts to delete by list_ids.
- items_total_count int? - The total number of contacts to delete.
- items_completed_count int? - The number of contacts deleted.
constantcontact: ActivityErrors
Fields
- message string? - Message describing the error condition.
constantcontact: ActivityExportStatus
Activity status for contact_exports activity
Fields
- activity_id string? - Unique ID for the activity.
- state string? - The state of the request: initialized - request has been received processing - request is being processed completed - job completed cancelled - request was cancelled failed - job failed to complete timed_out - the request timed out before completing
- started_at string? - Timestamp showing when we began processing the activity request, in ISO-8601 format.
- completed_at string? - Timestamp showing when we completed processing the activity, in ISO-8601 format.
- created_at string? - Timestamp showing when we created the activity, in ISO-8601 format.
- updated_at string? - Timestamp showing when we last updated the activity, in ISO-8601 format.
- percent_done int? - Shows the percent done for an activity that we are still processing.
- activity_errors string[]? - Array of messages describing the errors that occurred.
- status ActivityexportstatusStatus? - Status
- _links ActivityStatusExportLink? - Activity status export link
constantcontact: ActivityexportstatusStatus
Status
Fields
- items_total_count int? - The total number of contacts to export.
- items_completed_count int? - The number of contacts processed for export.
constantcontact: ActivityGeneric
Fields
- activity_id string - The ID that uniquely identifies the activity.
- state string - The processing state for the activity.
- created_at string? - The system generated date and time that the resource was created, in ISO-8601 format.
- updated_at string? - The system generated date and time that the resource was last updated, in ISO-8601 format.
- percent_done int? - The percentage complete for the specified activity.
- activity_errors ActivityErrors[]? - An array of error messages if errors occurred for a specified activity. The system returns an empty array if no errors occur.
- status ActivityGenericStatus? -
- _links Activitylinks2? -
constantcontact: ActivityGenericStatus
Fields
- items_total_count int? - The total number of tags that this activity will delete.
- items_completed_count int? - The number of tags that this activity has currently deleted.
constantcontact: ActivityImport
Activity status for contacts_json_import and contacts_file_import activities
Fields
- activity_id string? - Unique ID for the activity.
- state string? - The state of the request: initialized - request has been received processing - request is being processed completed - job completed cancelled - request was cancelled failed - job failed to complete timed_out - the request timed out before completing
- started_at string? - Timestamp showing when we began processing the activity request, in ISO-8601 format.
- completed_at string? - Timestamp showing when we completed processing the activity, in ISO-8601 format.
- created_at string? - Timestamp showing when we created the activity, in ISO-8601 format.
- updated_at string? - Timestamp showing when we last updated the activity, in ISO-8601 format.
- source_file_name string? - Name of the file used for an file_import activity.
- percent_done int? - Shows the percent done for an activity that we are still processing.
- activity_errors string[]? - Array of messages describing the errors that occurred.
- status ActivityimportStatus? - Status
- _links ActivityStatusLink? - Activity status link
constantcontact: ActivityimportStatus
Status
Fields
- items_total_count int? - The total number of rows in the import file.
- person_count int? - The total number of contacts in the import file.
- error_count int? - The number of non-correctable errors encountered during the file import.
- correctable_count int? - The number of correctable errors. Correctable errors are: invalid email address format, birthday or anniversary format error, or does not have minimal contact information (no name or email address). Correctable errors are available in the product UI to correct.
- cannot_add_to_list_count int? - The number of previously unsubscribed contacts in the import - they cannot be added to a list.
constantcontact: ActivityLinks
Fields
- self ActivitylinksSelf? -
- results ActivitylinksResults? -
constantcontact: Activitylinks2
Fields
- self Activitylinks2Self? - The link returned in the response that you use to retrieve the status for the specified activity.
constantcontact: Activitylinks2Self
The link returned in the response that you use to retrieve the status for the specified activity.
Fields
- href string? - The link returned in the response that you use to retrieve the status for the specified activity
constantcontact: ActivitylinksResults
Fields
- href string? - Use this link to view activity results.
constantcontact: ActivitylinksSelf
Fields
- href string? -
constantcontact: ActivityListsMembership
Fields
- activity_id string? - Unique ID for the activity.
- state string? - The state of the request: initialized - request has been received processing - request is being processed completed - job completed cancelled - request was cancelled failed - job failed to complete timed_out - the request timed out before completing
- started_at string? - Timestamp showing when we began processing the activity request, in ISO-8601 format.
- completed_at string? - Timestamp showing when we completed processing the activity, in ISO-8601 format.
- created_at string? - Timestamp showing when we created the activity, in ISO-8601 format.
- updated_at string? - Timestamp showing when we last updated the activity, in ISO-8601 format.
- percent_done int? - Shows the percent done for an activity that we are still processing.
- activity_errors string[]? - Array of messages describing the errors that occurred.
- status ActivitylistsmembershipStatus? -
- _links ActivityStatusLink? - Activity status link
constantcontact: ActivitylistsmembershipStatus
Fields
- items_total_count int? - Total number of contacts to add to or remove from lists.
- items_completed_count int? - The number of contacts processed.
- list_count int? - The number of lists specified in the request.
constantcontact: ActivityReference
Fields
- campaign_activity_id string? - The ID (UUID) that uniquely identifies a campaign activity.
- role string? - The purpose of the individual campaign activity in the larger email campaign effort. Valid values are: <ul> <li>primary_email — The main email marketing campaign that you send to contacts. The <code>primary_email</code> contains the complete email content.</li> <li>permalink — A permanent link to a web accessible version of the <code>primary_email</code> content without any personalized email information. For example, permalinks do not contain any of the contact details that you add to the <code>primary_email</code> email content. </li> <li>resend — An email campaign that you resend to contacts that did not open the email campaign.</li> </ul> Constant Contact creates a <code>primary_email</code> and a <code>permalink</code> role campaign activity when you create an email campaign.
constantcontact: ActivityStatus
Activity status
Fields
- items_total_count int? - The total number of items to be processed.
- items_completed_count int? - The number of items processed in the activity request.
- person_count int? - The total number of contacts in an import contacts request.
- error_count int? - The number of non-correctable errors encountered during an import contacts request.
- correctable_count int? - The number of correctable errors. Correctable errors are: invalid email address format, birthday or anniversary format error, or does not have minimal contact information (no name or email address). Correctable errors are available in the product UI to correct.
- cannot_add_to_list_count int? - The number of contacts that cannot be added to a list because they were previously unsubscribed, valid for contacts_file_ or json_import requests.
- list_count int? - The number of lists processed in an add_ or remove_list_membership activity request.
constantcontact: ActivityStatusExportLink
Activity status export link
Fields
- self ActivitystatusexportlinkSelf? - HATEOS-style link to the activity status (this object).
- results ActivitystatusexportlinkResults? - Link to an activity result resource; as an example, for file_export, the link to the exported contacts file.
constantcontact: ActivitystatusexportlinkResults
Link to an activity result resource; as an example, for file_export, the link to the exported contacts file.
Fields
- href string? - Link to an activity result resource
constantcontact: ActivitystatusexportlinkSelf
HATEOS-style link to the activity status (this object).
Fields
- href string? - HATEOS-style link to the activity status (this object)
constantcontact: ActivityStatusLink
Activity status link
Fields
- self ActivitystatusexportlinkSelf? - HATEOS-style link to the activity status (this object).
constantcontact: ActivityTagging
Fields
- activity_id string? - The system assigned UUID that uniquely identifies an activity.
- state string? - The activity processing state.
- started_at string? - Timestamp showing when processing started for the activity, in ISO-8601 format.
- completed_at string? - Timestamp showing when processing completed for the activity, in ISO-8601 format.
- created_at string? - Timestamp showing when the activity was first requested, in ISO-8601 format.
- updated_at string? - Timestamp showing when the activity was last updated, in ISO-8601 format.
- percent_done int? - The processing percent complete for the activity.
- activity_errors string[]? - An array of error message strings describing the errors that occurred.
- status ActivityTaggingStatus? - Provides the status for the requested activity.
- _links ActivityLinks? -
constantcontact: ActivityTaggingStatus
Provides the status for the requested activity.
Fields
- items_total_count int? - The total number of items processed for the requested activity.
- items_completed_count int? - The total number of items for which processing completed for the requested activity.
constantcontact: AuthInfoResponse
Fields
- scopes string[]? - The scopes associated with your access token as an array containing scope strings.
constantcontact: AuthToken
Fields
- token string - A valid OAuth2.0 access token.
constantcontact: BouncesTrackingActivitiesPage
Fields
- tracking_activities BouncesTrackingActivity[] - The list of email bounce tracking activities associated with a given 'campaign_activity_id'.
- _links Links2 - Links_2
constantcontact: BouncesTrackingActivity
Fields
- contact_id string - The ID that uniquely identifies a contact.
- campaign_activity_id string - The ID that uniquely identifies the email campaign activity.
- tracking_activity_type string - The type of email tracking activity, <code>em_bounces</code>, that this report includes.
- email_address string - The contact's email address that was used when the email campaign activity bounced.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- bounce_code string - The one-character string used to specify the reason for the email bounce. Valid codes include: <ul><li><code>B</code> - Non-existent address; the contact's Internet Service Provider (ISP) indicates that the email address doesn't exist.</li> <li><code>D</code> - Undeliverable; after repeated delivery attempts, no response was received from the contact's ISP.</li> <li><code>F</code> - Full; the contact's mailbox is full.</li> <li><code>S</code> - Suspended; the contact's address was reported as non-existent by the ISP and is suspended from delivery.</li> <li><code>V</code> - Vacation/autoreply; the contact set an autoreply, but the message was delivered.</li> <li><code>X</code> - Other; the contact's ISP specified another reason that the message cannot be delivered.</li> <li><code>Z</code> - Blocked; the recipient's ISP chose not to deliver the email. For example, the ISP may have flagged the email as spam.</li></ul>
- current_email_address string? - The contact's most current email address. If <code>email_address</code> was updated after the email bounce activity occurred, <code>current_ email_address</code> displays the updated address. If updates were not made to <code>email_address</code>, the <code>email_address</code> and <code>current_email_address</code> are the same.
- created_time string - The date and time that the email bounce tracking activity occurred.
- deleted_at string? - If applicable, the date when the contact was deleted.
constantcontact: BulkEmailCampaignSummariesPage
Fields
- bulk_email_campaign_summaries BulkEmailCampaignSummary[] - Lists and provides details about each bulk email campaign activity including total unique counts for tracked activities.
- _links Links2 - Links_2
constantcontact: BulkEmailCampaignSummary
Fields
- campaign_id string - The ID that uniquely identifies an email campaign.
- campaign_type string - Identifies the email campaign type.
- last_sent_date string - The date and time that the email campaign was last sent.
- unique_counts UniqueEmailCounts -
constantcontact: CampaignActivityStatsQueryResultEmail
Fields
- errors StatsError[]? - Array of errors indicating any partial failures in the query
- results CampaignActivityStatsResultGenericStatsEmailActivity[]? - An array of results containing statistics for each requested campaign activity
constantcontact: CampaignActivityStatsResultGenericStatsEmailActivity
Fields
- campaign_id string? - The unique ID used to identify the campaign (UUID).
- campaign_activity_id string? - The unique ID used to identify the campaign activity (UUID).
- stats StatsEmailActivity? -
- last_refresh_time string? - The time at which the campaign activity stats were last refreshed in ISO 8601 format (e.g. '2015-08-25T22:00:09.908Z').
constantcontact: CampaignActivitySummary
Fields
- campaign_activity_id string - The unique id of the activity for an e-mail campaign.
- start_on string - The last date at which the email was sent to this contact.
- em_bounces int - The number of times the email has bounced for this contact.
- em_clicks int - The number of times this contact has clicked a link in this email.
- em_forwards int - The number of times this contact has forwarded this email.
- em_opens int - The number of times this contact has opened this email.
- em_sends int - The number of times the email was sent to this contact.
- em_unsubscribes int - The number of times this contact has opted out.
constantcontact: CampaignStatsQueryResultEmail
Fields
- errors StatsError[]? - An array of errors indicating any partial failures in the query.
- results CampaignStatsResultGenericStatsEmailPercentsEmail[]? - An array of results listing statistics for each requested <code>campaign_id</code>.
constantcontact: CampaignStatsResultGenericStatsEmailPercentsEmail
Fields
- campaign_id string? - The ID that uniquely identifies the campaign (UUID).
- stats StatsEmail? -
- percents PercentsEmail? -
- last_refresh_time string? - The date and time that the campaign stats were last refreshed.
constantcontact: ClicksTrackingActivitiesPage
Fields
- tracking_activities ClicksTrackingActivity[] - The list of click tracking activities
- _links Links2? - Links_2
constantcontact: ClicksTrackingActivity
Fields
- contact_id string - The ID that uniquely identifies a contact.
- campaign_activity_id string - The ID that uniquely identifies the email campaign activity.
- tracking_activity_type string - The type of report tracking activity that is associated with the specified <code>campaign_activity_id</code>.
- email_address string - The email address used to send the email campaign activity to a contact.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- device_type string? - The type of device that the contact was using when they clicked the URL link for the email campaign activity.
- url_id string - The ID used to uniquely identify the URL link.
- link_url string - The text used for the URL link.
- created_time string - The date and time that the contact clicked the specified URL link for the email campaign activity.
- deleted_at string? - If applicable, displays the date that the contact was deleted.
constantcontact: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
constantcontact: CompanyLogo
Fields
- url string? - The internal URL used to get the company logo image file hosted locally in your account's MyLibrary.
- external_url string? - The external URL used to get the company logo image file that is hosted on an external website.
- internal_id string? - The internal ID used to identify the image hosted in your account's MyLibrary.
constantcontact: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
constantcontact: ContactCampaignActivitiesSummary
Fields
- contact_id string - Unique id of the contact that will have their activity summarized.
- campaign_activities CampaignActivitySummary[] - A summary of all the actions for a contact.
- _links Links2? - Links_2
constantcontact: ContactCreateOrUpdateInput
Fields
- email_address string - The email address for the contact. This method identifies each unique contact using their email address. If the email address exists in the account, this method updates the contact. If the email address is new, this method creates a new contact.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- job_title string? - The job title of the contact.
- company_name string? - The name of the company where the contact works.
- phone_number string? - The phone number for the contact.
- list_memberships string[] - The contact lists you want to add the contact to as an array of up to 50 contact <code>list_id</code> values. You must include at least one <code>list_id</code>.
- custom_fields CreateOrUpdateContactCustomField[]? - The custom fields you want to add to the contact as an array of up to 50 custom field objects.
- anniversary string? - The anniversary date for the contact. For example, this value could be the date when the contact first became a customer of an organization in Constant Contact. Valid date formats are MM/DD/YYYY, M/D/YYYY, YYYY/MM/DD, YYYY/M/D, YYYY-MM-DD, YYYY-M-D,M-D-YYYY, or M-DD-YYYY.
- birthday_month int? - The month value for the contact's birthday. Valid values are from 1 through 12. The <code>birthday_month</code> property is required if you use <code>birthday_day</code>.
- birthday_day int? - The day value for the contact's birthday. Valid values are from 1 through 31. The <code>birthday_day</code> property is required if you use <code>birthday_month</code>.
- street_address ContactcreateorupdateinputStreetAddress? -
constantcontact: ContactcreateorupdateinputStreetAddress
Fields
- kind string - The type of street address for the contact. Valid values are home, work, or other.
- street string? - The number and street of the contact's address.
- city string? - The name of the city for the contact's address.
- state string? - The name of the state or province for the contact's address.
- postal_code string? - The zip or postal code for the contact's address.
- country string? - The name of the country for the contact's address.
constantcontact: ContactCreateOrUpdateResponse
Fields
- contact_id string? - The unique identifier for the contact that the V3 API created or updated.
- action string? - Identifies if the V3 API created a new contact or updated an existing contact.
constantcontact: ContactCustomField
Custom fields allow Constant Contact users to add custom content to a contact that can be used to personalize emails in addition to the standard set of variables available for email personalization.
Fields
- custom_field_id string - The custom_field's unique ID
- value string - The custom_field value.
constantcontact: ContactDelete
Fields
- contact_ids string[]? - Specify up to 500 contacts by <code>contact_id</code> to delete; mutually exclusive with <code>list_ids</code>.
- list_ids string[]? - The contacts on the lists (up to 50) specified will be deleted; mutually exclusive with <code>contact_ids</code>.
constantcontact: ContactList
Fields
- list_id string? - Unique ID for the contact list
- name string? - The name given to the contact list
- description string? - Text describing the list.
- favorite boolean? - Identifies whether or not the account has favorited the contact list.
- created_at string? - System generated date and time that the resource was created, in ISO-8601 format.
- updated_at string? - Date and time that the list was last updated, in ISO-8601 format. System generated.
- membership_count int? - The number of contacts in the contact list.
constantcontact: Contactlist2
Fields
- list_id string? - Unique ID for the contact list
- name string? - The name given to the contact list
- description string? - Text describing the list.
- favorite boolean? - Identifies whether or not the account has favorited the contact list.
- created_at string? - System generated date and time that the resource was created, in ISO-8601 format.
- updated_at string? - Date and time that the list was last updated, in ISO-8601 format. System generated.
- membership_count int? - The number of contacts in the contact list.
constantcontact: ContactListArray
Fields
- lists Contactlist2[]? -
- lists_count int? - The total number of contact lists.
- _links PagingLinks? - Paging links
constantcontact: ContactOpenAndClickRates
The average click and open rates for a provided contact. The basic information provided is contact id, open rate, click rate, and emails included in the calculation.
Fields
- contact_id string - The unique ID of the contact for which the report is being generated.
- included_activities_count int - The number of activities included in the calculation.
- average_open_rate decimal - The average rate the contact opened emails sent to them.
- average_click_rate decimal - The average rate the contact clicked on links in emails sent to them.
constantcontact: ContactPostRequest
Fields
- email_address EmailAddressPost? - The contact's email address and related properties.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- job_title string? - The job title of the contact.
- company_name string? - The name of the company where the contact works.
- create_source string? - Describes who added the contact; valid values are <code>Contact</code> or <code>Account</code>. Your integration must accurately identify <code>create_source</code> for compliance reasons; value is set on POST, and is read-only going forward.
- birthday_month int? - The month value for the contact's birthday. Valid values are from 1 through 12. The <code>birthday_month</code> property is required if you use <code>birthday_day</code>.
- birthday_day int? - The day value for the contact's birthday. Valid values are from 1 through 31. The <code>birthday_day</code> property is required if you use <code>birthday_month</code>.
- anniversary string? - The anniversary date for the contact. For example, this value could be the date when the contact first became a customer of an organization in Constant Contact. Valid date formats are MM/DD/YYYY, M/D/YYYY, YYYY/MM/DD, YYYY/M/D, YYYY-MM-DD, YYYY-M-D,M-D-YYYY, or M-DD-YYYY.
- custom_fields ContactCustomField[]? - Array of up to 25 <code>custom_field</code> key value pairs.
- phone_numbers PhoneNumberPut[]? - Array of up to 2 phone_numbers subresources.
- street_addresses StreetAddressPut[]? - Array of street_addresses subresources. A contact can have 1 street address.
- list_memberships string[]? - Array of list_id's to which the contact is being subscribed, up to a maximum of 50.
- taggings string[]? - Array of tags (<code>tag_id</code>) assigned to the contact, up to a maximum of 50.
- notes Note[]? - An array of notes about the contact.
constantcontact: ContactPutRequest
Fields
- email_address EmailAddressPut? - The contact's email address and related properties.
- first_name string? - The contact's first name
- last_name string? - The contact's last name
- job_title string? - The contact's job title
- company_name string? - Name of the company the contact works for.
- birthday_month int? - Accepts values from 1-12; must be used with <code>birthday_day</code>
- birthday_day int? - Accepts values from 1-31; must be used with <code>birthday_month</code>
- anniversary string? - The anniversary date; Accepted formats are MM/DD/YYYY, M/D/YYYY, YYYY/MM/DD, YYYY/M/D, YYYY-MM-DD, YYYY-M-D, MM-DD-YYYY, M-D-YYYY
- update_source string - Identifies who last updated the contact; valid values are <code>Contact</code> or <code>Account</code>.
- custom_fields ContactCustomField[]? - Array of up to 25 custom_field subresources.
- phone_numbers PhoneNumberPut[]? - Array of up to 2 phone_numbers subresources.
- street_addresses StreetAddressPut[]? - Array of street_addresses subresources. A contact can have 1 street address.
- list_memberships string[]? - Array of up to 50 <code>list_ids</code> to which the contact is subscribed.
- taggings string[]? - Array of tags (<code>tag_id</code>) assigned to the contact, up to a maximum of 50.
- notes Note[]? - An array of notes about the contact listed by most recent note first.
constantcontact: ContactResource
Use this endpoint to retrieve (GET), update (PUT), or DELETE an existing contact resource specified using the contact_id
path parameter.
Fields
- contact_id string? - Unique ID for each contact resource
- email_address EmailAddress? - A contact subresource describing the contact's email_address.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- job_title string? - The job title of the contact.
- company_name string? - The name of the company where the contact works.
- birthday_month int? - The month value for the contact's birthday. Valid values are from 1 through 12. You must use this property with <code>birthday_month</code>.
- birthday_day int? - The day value for the contact's birthday. Valid values are from 1 through 12. You must use this property with <code>birthday_day</code>.
- anniversary string? - The anniversary date for the contact. For example, this value could be the date when the contact first became a customer of an organization in Constant Contact. Valid date formats are MM/DD/YYYY, M/D/YYYY, YYYY/MM/DD, YYYY/M/D, YYYY-MM-DD, YYYY-M-D,M-D-YYYY, or M-DD-YYYY.
- update_source string? - Identifies who last updated the contact; valid values are Contact or Account
- create_source string? - Describes who added the contact; valid values are <code>Contact</code> or <code>Account</code>. Your integration must accurately identify <code>create_source</code> for compliance reasons; value is set when contact is created.
- created_at string? - System generated date and time that the resource was created, in ISO-8601 format.
- updated_at string? - System generated date and time that the contact was last updated, in ISO-8601 format.
- deleted_at string? - For deleted contacts (<code>email_address</code> contains <code>opt_out_source</code> and <code>opt_out_date</code>), shows the date of deletion.
- custom_fields ContactCustomField[]? - Array of up to 25 <code>custom_field</code> key value pairs.
- phone_numbers PhoneNumber[]? - Array of phone_numbers subresources. A contact can have up to 2 phone numbers.
- street_addresses StreetAddress[]? - Array of street_addresses subresources. A contact can have 1 street address.
- list_memberships string[]? - Array of list_id's to which the contact is subscribed, up to a maximum of 50.
- taggings string[]? - Array of tags (<code>tag_id</code>) assigned to the contact, up to a maximum of 50.
- notes Note[]? - An array of up to 150 notes about the contact.
constantcontact: Contactresource2
Use this endpoint to retrieve (GET), update (PUT), or DELETE an existing contact resource specified using the contact_id
path parameter.
Fields
- contact_id string? - Unique ID for each contact resource
- email_address EmailAddress? - A contact subresource describing the contact's email_address.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- job_title string? - The job title of the contact.
- company_name string? - The name of the company where the contact works.
- birthday_month int? - The month value for the contact's birthday. Valid values are from 1 through 12. You must use this property with <code>birthday_month</code>.
- birthday_day int? - The day value for the contact's birthday. Valid values are from 1 through 12. You must use this property with <code>birthday_day</code>.
- anniversary string? - The anniversary date for the contact. For example, this value could be the date when the contact first became a customer of an organization in Constant Contact. Valid date formats are MM/DD/YYYY, M/D/YYYY, YYYY/MM/DD, YYYY/M/D, YYYY-MM-DD, YYYY-M-D,M-D-YYYY, or M-DD-YYYY.
- update_source string? - Identifies who last updated the contact; valid values are Contact or Account
- create_source string? - Describes who added the contact; valid values are <code>Contact</code> or <code>Account</code>. Your integration must accurately identify <code>create_source</code> for compliance reasons; value is set when contact is created.
- created_at string? - System generated date and time that the resource was created, in ISO-8601 format.
- updated_at string? - System generated date and time that the contact was last updated, in ISO-8601 format.
- deleted_at string? - For deleted contacts (<code>email_address</code> contains <code>opt_out_source</code> and <code>opt_out_date</code>), shows the date of deletion.
- custom_fields ContactCustomField[]? - Array of up to 25 <code>custom_field</code> key value pairs.
- phone_numbers PhoneNumber[]? - Array of phone_numbers subresources. A contact can have up to 2 phone numbers.
- street_addresses StreetAddress[]? - Array of street_addresses subresources. A contact can have 1 street address.
- list_memberships string[]? - Array of list_id's to which the contact is subscribed, up to a maximum of 50.
- taggings string[]? - Array of tags (<code>tag_id</code>) assigned to the contact, up to a maximum of 50.
- notes Note[]? - An array of up to 150 notes about the contact.
constantcontact: Contacts
Use this endpoint to retrieve (GET) a collection of existing contacts, or to create (POST) a new contact.
Fields
- contacts Contactresource2[]? - Collection of contacts
- contacts_count int? - Total number of contacts in the response.
- _links PagingLinks? - Paging links
- status string? - If you use the <code>segment_id</code> query parameter to filter results based on a segment, this property indicates that the V3 API accepted your request and is still processing it.
constantcontact: ContactsExport
You can export contact objects to a CSV file. By default, all contacts in the user's account are exported if none of the following properties are included:
Fields
- contact_ids string[]? - Exports up to 500 specific contacts. This property is mutually exclusive with <code>list_ids</code>.
- list_ids string[]? - Exports all of the contacts inside of up to 50 contact lists. This property is mutually exclusive with <code>contact_ids</code>.
- segment_id int? - Specify the <code>segment_id</code> from which you want to export all contacts that meet the specified <code>segment_criteria</code>. This property is mutually exclusive with <code>contact_ids</code> and <code>list_ids</code>. You can only specify one <code>segment_id</code>.
- fields string[]? - Use this array to export specific contact fields. You must export <code>email_address</code> to successfully export <code>email_optin_source</code>, <code>email_optin_date</code>, <code>email_optout_source</code>, <code>email_optout_date</code>, or <code>email_optout_reason</code>.
- status string? - Allows you to export only contacts that have a specific status value. Possible values are <code>active</code>, <code>unsubscribed</code>, or <code>removed</code>
constantcontact: ContactsJsonImport
Fields
- import_data JsonImportContact[] - An array containing the contacts to import.
- list_ids string[] - Specify which contact lists you are adding all imported contacts to as an array of up to 50 contact <code>list_id</code> string values.
constantcontact: ContactTrackingActivitiesPage
A page of tracking activities for a contact that can include sends, opens, clicks, bounces, opt-outs and forwards to a friend. If it exists, a link to the next page of tracking activities is provided.
Fields
- tracking_activities ContactTrackingActivity[]? - The list of contact tracking activities in descending date order.
- _links Links2? - Links_2
constantcontact: ContactTrackingActivity
The base contact tracking activity representing sends, opt-outs and forwards to a friend. The basic information provided is campaign activity id, tracking activity type and time the tracking activity occurred.
Fields
- contact_id string - The contact id.
- campaign_activity_id string - The unique id of the activity for an e-mail campaign.
- created_time string? - The time the tracking activity occurred
- tracking_activity_type string - The type of the tracking activity (send, open, click, bounce, opt-out or forward to a friend)
constantcontact: ContactXref
The cross-referenced pair of V3 API list_id
and V2 API sequence_id
for a list. Response is sorted ascending by sequence_id
.
Fields
- sequence_id string? - The V2 API contact unique identifier
- contact_id string? - The V3 API contact unique identifier
constantcontact: ContactXrefs
Fields
- xrefs ContactXref[]? - An array of cross-referenced V3 API <code>contact_id</code> and V2 API <code>sequence_id</code> values. Response is sorted ascending by <code>sequence_id</code>.
constantcontact: CreateOrUpdateContactCustomField
Fields
- custom_field_id string? - The unique ID for the <code>custom_field</code>.
- value string? - The value of the <code>custom_field</code>.
constantcontact: CrossReference
Fields
- v2_email_campaign_id string? - Identifies an email campaign in the V2 API.
- campaign_id string? - <p>Identifies a campaign in the V3 API. In the V3 API, each campaign contains one or more activities. For more information, see <a href="/api_guide/v3_v2_email_campaign_deltas.html" target_"blank">V3 Email Campaign Resource Changes<a/>.<p>
- campaign_activity_id string? - <p>Identifies a campaign activity in the V3 API. In the V3 API, each campaign contains one or more activities. Email type activities represent the detailed information in an email and contain properties like <code>from_email</code> and <code>from_name</code>. For more information, see <a href="/api_guide/v3_v2_email_campaign_deltas.html" target_"blank">V3 Campaign Resource Changes<a/>.<p>
constantcontact: CrossReferenceResponse
Fields
- xrefs CrossReference[]? - An array of objects that contain a <code>v2_email_campaign_id</code> cross-referenced with a V3 <code>campaign_id</code> and a V3 <code>campaign_activity_id</code> value.
constantcontact: Customer
Fields
- contact_email string? - Email addresses that are associated with the Constant Contact account owner.
- contact_phone string? - The account owner's contact phone number (up to 25 characters in length).
- country_code string? - The uppercase two-letter <a href='https://en.wikipedia.org/wiki/ISO_3166-1' target='_blank'>ISO 3166-1 code</a> representing the organization's country.
- encoded_account_id string? - The readOnly encoded account ID that uniquely identifies the account.
- first_name string? - The account owner's first name.
- last_name string? - The account owner's last name.
- organization_name string? - The name of the organization that is associated with this account.
- organization_phone string? - The phone number of the organization that is associated with this account.
- state_code string? - The uppercase two letter <a href='https://en.wikipedia.org/wiki/ISO_3166-1' target='_blank'>ISO 3166-1 code</a> for the organization's state. This property is required if the <code>country_code</code> is US (United States).
- time_zone_id string? - The time zone that is automatically set based on the <code>state_code</code> setting; as defined in the IANA time-zone database (see http://www.iana.org/time-zones).
- website string? - The organization's website URL.
- physical_address CustomerPhysicalAddress? -
- company_logo CompanyLogo? -
constantcontact: CustomerPhysicalAddress
Fields
- address_line1 string - Line 1 of the organization's street address.
- address_line2 string? - Line 2 of the organization's street address.
- address_line3 string? - Line 3 of the organization's street address.
- city string - The city where the organization is located.
- state_code string? - The two letter ISO 3166-1 code for the organization's state and only used if the <code>country_code</code> is <code>US</code> or <code>CA</code>. If not, exclude this property from the request body.
- state_name string? - Use if the state where the organization is physically located is not in the United States or Canada. If <code>country_code</code> is <code>US</code> or <code>CA</code>, exclude this property from the request body.
- postal_code string? - The postal code address (ZIP code) of the organization. This property is required if the <code>state_code</code> is <code>US</code> or <code>CA</code>, otherwise exclude this property from the request body.
- country_code string - The two letter <a href='https://en.wikipedia.org/wiki/ISO_3166-1' target='_blank'>ISO 3166-1 code</a> for the organization's country.
constantcontact: CustomerPut
Fields
- contact_email string? - The confirmed email address that is associated with the account owner.
- contact_phone string? - The account owner's contact phone number (up to 25 characters in length).
- country_code string? - The two-letter <a href='https://en.wikipedia.org/wiki/ISO_3166-1' target='_blank'>ISO 3166-1 code</a> representing the organization's country.
- encoded_account_id string? - The read only encoded account ID that uniquely identifies the account.
- first_name string? - The account owner's first name.
- last_name string? - The account owner's last name.
- organization_name string? - The name of the organization that is associated with this account.
- organization_phone string? - The phone number of the organization that is associated with this account.
- state_code string? - The two letter <a href='https://en.wikipedia.org/wiki/ISO_3166-1' target='_blank'>ISO 3166-1 code</a> used to specify the state to associate with the account. This property is required if the <code>country_code</code> is US (United States).
- time_zone_id string? - The time zone to use for the account; as defined in the IANA time-zone database (see http://www.iana.org/time-zones).
- website string? - The organization's website URL.
constantcontact: CustomFieldInput
Fields
- label string - The display name for the custom_field shown in the UI as free-form text
- 'type string - Specifies the type of value the custom_field field accepts: string or date.
constantcontact: CustomFieldResource
Custom fields allow Constant Contact users to add custom content to a contact that can be used to personalize emails in addition to the standard set of variables available for email personalization.
Fields
- custom_field_id string? - The custom_field's unique ID
- label string - The display name for the custom_field shown in the UI as free-form text
- name string? - Unique name for the custom_field constructed from the label by replacing blanks with underscores.
- 'type string - Specifies the type of value the custom_field field accepts: string or date.
- updated_at string? - System generated date and time that the resource was updated, in ISO-8601 format.
- created_at string? - Date and time that the resource was created, in ISO-8601 format. System generated.
constantcontact: Customfieldresource2
Custom fields allow Constant Contact users to add custom content to a contact that can be used to personalize emails in addition to the standard set of variables available for email personalization.
Fields
- custom_field_id string? - The custom_field's unique ID
- label string - The display name for the custom_field shown in the UI as free-form text
- name string? - Unique name for the custom_field constructed from the label by replacing blanks with underscores.
- 'type string - Specifies the type of value the custom_field field accepts: string or date.
- updated_at string? - System generated date and time that the resource was updated, in ISO-8601 format.
- created_at string? - Date and time that the resource was created, in ISO-8601 format. System generated.
constantcontact: CustomFields
Use this endpoint to retrieve (GET) all custom_fields in the user's account, or to create (POST) a new custom_field.
Fields
- custom_fields Customfieldresource2[]? - CustomFields array
- _links PagingLinks? - Paging links
constantcontact: DidNotOpensTrackingActivitiesPage
Fields
- tracking_activities DidNotOpensTrackingActivity[] - Lists contacts that did not open the specified <code>campaign_activity_id</code>.
- _links Links2? - Links_2
constantcontact: DidNotOpensTrackingActivity
Fields
- contact_id string - The ID that uniquely identifies a contact.
- campaign_activity_id string - The ID that uniquely identifies an email campaign activity.
- tracking_activity_type string - The type of tracking activity that is associated with this <code>campaign_activity_id</code> and used for reporting purposes.
- email_address string - The email address used to send the email campaign activity to a contact.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- created_time string - The date and time that the contact was sent the email campaign activity.
- deleted_at string? - If applicable, displays the date that the contact was deleted.
constantcontact: EmailAddress
A contact subresource describing the contact's email_address.
Fields
- address string - The email address of the contact. The email address must be unique for each contact.
- permission_to_send string? - Identifies the type of permission that the Constant Contact account has to send email to the contact. Types of permission: explicit, implicit, not_set, pending_confirmation, temp_hold, unsubscribed.
- created_at string? - Date and time that the email_address was created, in ISO-8601 format. System generated.
- updated_at string? - Date and time that the email_address was last updated, in ISO-8601 format. System generated.
- opt_in_source string? - Describes who opted-in the email_address; valid values are Contact or Account. Your integration must accurately identify <code>opt_in_source</code> for compliance reasons; value is set on POST, and is read-only going forward.
- opt_in_date string? - Date and time that the email_address was opted-in to receive email from the account, in ISO-8601 format. System generated.
- opt_out_source string? - Describes the source of the unsubscribed/opt-out action: either Account or Contact. If the Contact opted-out, then the account cannot send any campaigns to this contact until the contact opts back in. If the Account, then the account can add the contact back to any lists and send to them. Displayed only if contact has been unsubscribed/opt-out.
- opt_out_date string? - Date and time that the contact unsubscribed/opted-out of receiving email from the account, in ISO-8601 format. Displayed only if contact has been unsubscribed/opt-out. System generated.
- opt_out_reason string? - The reason, as provided by the contact, that they unsubscribed/opted-out of receiving email campaigns.
- confirm_status string? - Indicates if the contact confirmed their email address after they subscribed to receive emails. Possible values: pending, confirmed, off.
constantcontact: EmailAddressPost
The contact's email address and related properties.
Fields
- address string - The contact's email address
- permission_to_send string? - Identifies the type of permission that the Constant Contact account has been granted to send email to the contact. Types of permission: explicit, implicit, not_set, pending_confirmation, temp_hold, unsubscribed.
constantcontact: EmailAddressPut
The contact's email address and related properties.
Fields
- address string - The email address of the contact. The email address must be unique for each contact.
- permission_to_send string? - Identifies the type of permission that the Constant Contact account has to send email to the contact. Types of permission: explicit, implicit, not_set, pending_confirmation, temp_hold, unsubscribed.
- created_at string? - Date and time that the email_address was created, in ISO-8601 format. System generated.
- updated_at string? - Date and time that the email_address was last updated, in ISO-8601 format. System generated.
- opt_in_date string? - Date and time that the email_address was opted-in to receive email from the account, in ISO-8601 format. System generated.
- opt_out_source string? - Describes the source of the unsubscribed/opt-out action: either Account or Contact. If the Contact opted-out, then the account cannot send any campaigns to this contact until the contact opts back in. If the Account, then the account can add the contact back to any lists and send to them. Displayed only if contact has been unsubscribed/opt-out.
- opt_out_date string? - Date and time that the contact unsubscribed/opted-out of receiving email from the account, in ISO-8601 format. Displayed only if contact has been unsubscribed/opt-out. System generated.
- opt_out_reason string? - The reason, as provided by the contact, that they unsubscribed/opted-out of receiving email campaigns.
- confirm_status string? - Indicates if the contact confirmed their email address after they subscribed to receive emails. Possible values: pending, confirmed, off.
constantcontact: EmailCampaign
Fields
- campaign_activities ActivityReference[]? - Lists the role and unique activity ID of each campaign activity that is associated with an Email Campaign.
- campaign_id string? - The unique ID used to identify the email campaign (UUID format).
- created_at string? - The system generated date and time that this email campaign was created. This string is readonly and is in ISO-8601 format.
- current_status string? - The current status of the email campaign. Valid values are: <ul> <li>Draft — An email campaign that you have created but have not sent to contacts.</li> <li>Scheduled — An email campaign that you have scheduled for Constant Contact to send to contacts.</li> <li>Executing — An email campaign that Constant Contact is currently sending to contacts. Email campaign activities are only in this status briefly.</li> <li>Done — An email campaign that you successfully sent to contacts.</li> <li>Error — An email campaign activity that encountered an error.</li> <li>Removed — An email campaign that a user deleted. Users can view and restore deleted emails through the UI.</li> </ul>
- name string? - The descriptive name the user provides to identify this campaign. Campaign names must be unique for each account ID.
- 'type string? - Identifies the type of campaign that you select when creating the campaign. Newsletter and Custom Code email campaigns are the primary types.
- type_code int? - The code used to identify the email campaign
type
. <ul> <li> 1 (Default) </li> <li> 2 (Bulk Email) </li> <li> 10 (Newsletter) </li> <li> 11 (Announcement) </li> <li> 12 (Product/Service News) </li> <li> 14 (Business Letter) </li> <li> 15 (Card) </li> <li> 16 (Press release)</li> <li> 17 (Flyer) </li> <li> 18 (Feedback Request) </li> <li> 19 (Ratings and Reviews) </li> <li> 20 (Event Announcement) </li> <li> 21 (Simple Coupon) </li> <li> 22 (Sale Promotion) </li> <li> 23 (Product Promotion) </li> <li> 24 (Membership Drive) </li> <li> 25 (Fundraiser) </li> <li> 26 (Custom Code Email)</li> <li> 57 (A/B Test)</li> </ul>
- updated_at string? - The system generated date and time showing when the campaign was last updated. This string is read only and is in ISO-8601 format.
constantcontact: EmailCampaignActivity
Fields
- campaign_activity_id string? - Identifies a campaign activity in the V3 API.
- campaign_id string? - Identifies a campaign in the V3 API.
- role string? - The purpose of the individual campaign activity in the larger email campaign effort. Valid values are: <ul> <li>primary_email — The main email marketing campaign that you send to contacts. The <code>primary_email</code> contains the complete email content.</li> <li>permalink — A permanent link to a web accessible version of the <code>primary_email</code> content without any personalized email information. For example, permalinks do not contain any of the contact details that you add to the <code>primary_email</code> email content. </li> <li>resend — An email campaign that you resend to contacts that did not open the email campaign.</li> </ul> Constant Contact creates a <code>primary_email</code> and a <code>permalink</code> role campaign activity when you create an email campaign.
- contact_list_ids string[]? - The contacts that Constant Contact sends the email campaign activity to as an array of contact <code>list_id</code> values. You cannot use contact lists and segments at the same time in an email campaign activity.
- segment_ids int[]? - The contacts that Constant Contact sends the email campaign activity to as an array containing a single <code>segment_id</code> value. Only <code>format_type</code> 3, 4, and 5 email campaign activities support segments. You cannot use contact lists and segments at the same time in an email campaign activity.
- current_status string? - The current status of the email campaign activity. Valid values are: <ul> <li>DRAFT — An email campaign activity that you have created but have not sent to contacts.</li> <li>SCHEDULED — An email campaign activity that you have scheduled for Constant Contact to send to contacts.</li> <li>EXECUTING — An email campaign activity Constant Contact is currently sending to contacts. Email campaign activities are only in this status briefly.</li> <li>DONE — An email campaign activity that you successfully sent to contacts.</li> <li>ERROR — An email campaign activity that encountered an error.</li> <li>REMOVED — An email campaign that a user deleted. Users can view and restore deleted emails through the UI.</li> </ul>
- format_type int? - Identifies the type of email format. Valid values are: <ul> <li>1 - A legacy custom code email created using the V2 API, the V3 API, or the legacy UI HTML editor.</li> <li>2 - An email created using the second generation email editor UI.</li> <li>3 - An email created using the third generation email editor UI. This email editor features an improved drag and drop UI and mobile responsiveness.</li> <li>4 - An email created using the fourth generation email editor UI.</li> <li>5 - A custom code email created using the V3 API or the new UI HTML editor.</li> </ul>
- from_email string - The email "From Email" field for the email campaign activity. You must use a confirmed Constant Contact account email address. Make a GET call to <code>/account/emails</code> to return a collection of account emails and their confirmation status.
- from_name string - The email "From Name" field for the email campaign activity.
- reply_to_email string - The email "Reply To Email" field for the email campaign activity. You must use a confirmed Constant Contact account email address. Make a GET call to <code>/account/emails</code> to return a collection of account emails and their confirmation status.
- subject string - The email "Subject" field for the email campaign activity.
- html_content string? - The HTML or XHTML content for the email campaign activity. Only <code>format_type</code> 1 and 5 (legacy custom code emails or modern custom code emails) can contain <code>html_content</code>.
- template_id string? - Identifies the email layout and design template that the email campaign activity is using as a base.
- permalink_url string? - The permanent link to a web accessible version of the email campaign content without any personalized email information. The permalink URL becomes accessible after you send an email campaign to contacts.
- preheader string? - The email preheader for the email campaign activity. Only <code>format_type</code> 3, 4, and 5 email campaign activities use the preheader property.
- physical_address_in_footer EmailPhysicalAddress? -
- document_properties EmailcampaignactivityDocumentProperties? - An object that contains optional properties for legacy format type emails (<code>format_type</code> 1 and 2). If you attempt to add a property that does apply to the email <code>format_type</code>, the API will ignore the property.
constantcontact: EmailcampaignactivityDocumentProperties
An object that contains optional properties for legacy format type emails (format_type
1 and 2). If you attempt to add a property that does apply to the email format_type
, the API will ignore the property.
Fields
- style_content string? - Contains style sheet elements for XHTML letter format emails. This property applies only to <code>format_type</code> 1.
- letter_format string? - Email message format. Valid values are <code>HTML</code> and <code>XHTML</code>. By default, the value is <code>HTML</code>. For more information, see the <a href="http://www.constantcontact.com/display_media.jsp?id=87" target="_blank">Advanced Editor User's Guide</a>. This property applies only to <code>format_type</code> 1. You cannot change this property after you create an email.
- greeting_salutation string? - The greeting used in the email message. This property applies only to <code>format_type</code> 1.
- greeting_name_type string? - The type of name the campaign uses to greet the contact. Valid values are <code>F</code> (First Name), <code>L</code> (Last Name), <code>B</code> (First and Last Name), or <code>N</code> (No greeting). By default, the value is <code>N</code>. This property applies only to <code>format_type</code> 1.
- greeting_secondary string? - A fallback text string the campaign uses to greet the contact when the <code>greeting_name_type</code> is not available or set to <code>N</code>. This property applies only to <code>format_type</code> 1.
- subscribe_link_enabled string? - If <code>true</code>, the email footer includes a link for subscribing to the list. If <code>false</code>, the message footer does not include a link for subscribing to the list. By default, the value is <code>false</code>. This property applies only to <code>format_type</code> 1.
- subscribe_link_name string? - The text displayed as the name for the subscribe link in the email footer. This property applies only to <code>format_type</code> 1.
- text_content string? - Contains the text content that Constant Contact displays to contacts when their email client cannot display HTML email. If you do not specify text content, Constant Contact displays "Greetings!" as the text content. This property applies only to <code>format_type</code> 1.
- permission_reminder_enabled string? - If <code>true</code>, Constant Contact displays your <code>permission_reminder</code> message to contacts at top of the email. If <code>false</code>, Constant Contact does not display the message. By default, the value is <code>false</code>. This property applies to <code>format_type</code> 1 and 2.
- permission_reminder string? - The message text Constant Contact displays at the top of the email message to remind users that they are subscribed to an email list. This property applies to <code>format_type</code> 1 and 2.
- view_as_webpage_enabled string? - If <code>true</code>, Constant Contact displays the view as web page email message. If <code>false</code> Constant Contact does not display the message. By default, the value is <code>false</code>. This property applies to <code>format_type</code> 1 and 2.
- view_as_webpage_text string? - The text Constant Contact displays before the view as web page link at the top of the email. This property applies to <code>format_type</code> 1 and 2.
- view_as_webpage_link_name string? - The name of the link that users can click to view the email as a web page. This property applies to <code>format_type</code> 1 and 2.
- forward_email_link_enabled string? - If <code>true</code>, when the user forwards an email message the footer includes a link for subscribing to the list. If <code>false</code>, when a user forwards an email message the footer does not include a link for subscribing to the list. By default, the value is <code>false</code>. This property applies to <code>format_type</code> 1 and 2.
- forward_email_link_name string? - The text displayed as the name for the forward email link in the footer when a user forwards an email. This property applies to <code>format_type</code> 1 and 2.
constantcontact: EmailCampaignActivityInput
Fields
- format_type int - The email format you are using to create the email campaign activity. The V3 API supports creating emails using <code>format_type</code> 5 (custom code emails).
- from_name string - The email sender's name to display for the email campaign activity.
- from_email string - The sender's email address to use for the email campaign activity. You must use a confirmed Constant Contact account email address. Make a GET call to <code>/account/emails</code> to return a collection of account emails and their confirmation status.
- reply_to_email string - The sender's email address to use if the contact replies to the email campaign activity. You must use a confirmed Constant Contact account email address. Make a GET call to <code>/account/emails</code> to return a collection of account emails and their confirmation status.
- subject string - The text to display in the subject line that describes the email campaign activity.
- preheader string? - The email preheader for the email campaign activity. Contacts will view your preheader as a short summary that follows the subject line in their email client. Only <code>format_type</code> 3, 4, and 5 email campaign activities use the preheader property.
- html_content string - The HTML content for the email campaign activity. Only <code>format_type</code> 5 (custom code emails) can contain <code>html_content</code>. When creating a <code>format_type</code> 5 custom code email, make sure that you include <code>[[trackingImage]]</code> in the <code><body></code> element of your HTML.
- physical_address_in_footer EmailPhysicalAddress? -
constantcontact: EmailCampaignActivityPreview
Fields
- campaign_activity_id string? - The unique ID for an email campaign activity.
- from_email string? - The "from email" email header for the email campaign activity.
- from_name string? - The "from name" email header for the email campaign activity.
- preheader string? - The email preheader for the email campaign activity. Only <code>format_type</code> 3, 4, and 5 email campaign activities use the preheader property.
- preview_html_content string? - An HTML preview of the email campaign activity.
- preview_text_content string? - A plain text preview of the email campaign activity.
- reply_to_email string? - The email "Reply To Email" field for the email campaign activity.
- subject string? - The email "Subject" field for the email campaign activity.
constantcontact: EmailCampaignComplete
Fields
- name string - The unique and descriptive name that you specify for the email campaign.
- email_campaign_activities EmailCampaignActivityInput[] - The content of the email campaign as an array that contains a single email campaign activity object.
constantcontact: EmailCampaignName
Fields
- name string - The updated email campaign name. The email campaign name must be unique.
constantcontact: EmailCampaigns
Fields
- campaign_id string? - The unique ID used to identify the email campaign (UUID format).
- created_at string? - The system generated date and time that this email campaign was created. This string is readonly and is in ISO-8601 format.
- current_status string? - The current status of the email campaign. Valid values are: <ul> <li>Draft — An email campaign that you have created but have not sent to contacts.</li> <li>Scheduled — An email campaign that you have scheduled for Constant Contact to send to contacts.</li> <li>Executing — An email campaign that Constant Contact is currently sending to contacts. Email campaign activities are only in this status briefly.</li> <li>Done — An email campaign that you successfully sent to contacts.</li> <li>Error — An email campaign activity that encountered an error.</li> <li>Removed — An email campaign that a user deleted. Users can view and restore deleted emails through the UI.</li> </ul>
- name string? - The descriptive name the user provides to identify this campaign. Campaign names must be unique for each account ID.
- 'type string? - Identifies the type of campaign that you select when creating the campaign. Newsletter and Custom Code email campaigns are the primary types.
- type_code int? - The code used to identify the email campaign
type
. <ul> <li> 1 (Default) </li> <li> 2 (Bulk Email) </li> <li> 10 (Newsletter) </li> <li> 11 (Announcement) </li> <li> 12 (Product/Service News) </li> <li> 14 (Business Letter) </li> <li> 15 (Card) </li> <li> 16 (Press release)</li> <li> 17 (Flyer) </li> <li> 18 (Feedback Request) </li> <li> 19 (Ratings and Reviews) </li> <li> 20 (Event Announcement) </li> <li> 21 (Simple Coupon) </li> <li> 22 (Sale Promotion) </li> <li> 23 (Product Promotion) </li> <li> 24 (Membership Drive) </li> <li> 25 (Fundraiser) </li> <li> 26 (Custom Code Email)</li> <li> 57 (A/B Test)</li> </ul>
- updated_at string? - The system generated date and time showing when the campaign was last updated. This string is read only and is in ISO-8601 format.
constantcontact: EmailLinkClickCount
Fields
- link_url string? - The URL of a link in an email campaign activity. This URL is not normalized and appears the same as the URL in the email campaign activity.
- url_id string? - The ID for a unique link URL in an email campaign activity.
- unique_clicks int? - The number of unique contacts that clicked the link.
- list_action string? - If the link uses the click segmentation feature, this property contains the action that contacts trigger when they click the link. Currently the only available action is <code>add</code>, which adds contacts that click the link to a contact list.
- list_id string? - If the link uses the click segmentation feature, this property contains the contact list linked with the <code>list_action</code> property.
- link_tag string? - Link tags are not currently available in email campaigns. By default, this method combines results for duplicate link URLs. Link tags will allow users to get a separate link click report for each unique <code>link_tag</code> value they use, even if URLs are not unique.
constantcontact: EmailLinks
Fields
- campaign_activity_id string? - The unique ID for an email campaign activity.
- link_click_counts EmailLinkClickCount[]? - An array of objects that contain the contact click count and link metadata for each unique link URL in an email campaign activity. By default, this method combines results for duplicate link URLs.
constantcontact: EmailPhysicalAddress
Fields
- address_line1 string - Line 1 of the organization's street address.
- address_line2 string? - Line 2 of the organization's street address.
- address_line3 string? - Line 3 of the organization's street address.
- address_optional string? - An optional address field for the organization. Only <code>format_type</code> 3, 4, and 5 can use this property.
- city string? - The city where the organization sending the email campaign is located.
- country_code string - The uppercase two letter <a href='https://en.wikipedia.org/wiki/ISO_3166-1' target='_blank'>ISO 3166-1 code</a> for the organization's country.
- country_name string? - The full name of the country where the organization sending the email is located. Automatically generated using the <code>country_code</code>.
- organization_name string - The name of the organization that is sending the email campaign.
- postal_code string? - The postal code address (ZIP code) of the organization.
- state_code string? - The uppercase two letter <a href='https://en.wikipedia.org/wiki/ISO_3166-1' target='_blank'>ISO 3166-1 code</a> for the organization's state. This property is required if the <code>country_code</code> is US (United States).
- state_name string? - The full state name for a <code>state_code</code> that is inside the United States. Automatically generated using the <code>state_code</code>.
- state_non_us_name string? - The full state name for a <code>state_code</code> that is outside the United States. This property is not read only.
constantcontact: EmailScheduleInput
Fields
- scheduled_date string - The date when Constant Contact will send the email campaign activity to contacts in ISO-8601 format. For example, <code>2022-05-17</code> and <code>2022-05-17T16:37:59.091Z</code> are valid dates. Use <code>"0"</code> as the date to have Constant Contact immediately send the email campaign activity to contacts.
constantcontact: EmailscheduleresponseInner
Fields
- scheduled_date string? - The date when Constant Contact will send the email campaign activity to contacts in ISO-8601 format. For example, <code>2022-05-17</code> and <code>2022-05-17T16:37:59.091Z</code> are valid dates.
constantcontact: EmailsendhistoryInner
Fields
- send_id int? - Uniquely identifies each send history object using the number of times that you sent the email campaign activity as a sequence starting at <code>1</code>. For example, when you send a specific email campaign activity twice this method returns an object with a <code>send_id</code> of 1 for the first send and an object with a <code>send_id</code> of 2 for the second send.
- contact_list_ids string[]? - The contacts lists that Constant Contact sent email campaign activity to as an array of contact <code>list_id</code> strings.
- segment_ids int[]? - The contact segments that Constant Contact sent the email campaign activity to as an array of <code>segment_id</code> integers.
- count int? - The number of contacts that Constant Contact sent this email campaign activity to. This property is specific to each send history object. When you resend an email campaign activity, Constant Contact only sends it to new contacts in the contact lists or segments you are using.
- run_date string? - The system generated date and time that Constant Contact sent the email campaign activity to contacts in ISO-8601 format.
- send_status string? - The send status for the email campaign activity. Valid values are: <ul> <li><code>COMPLETED</code>: Constant Contact successfully sent the email campaign activity.</li> <li><code>ERRORED</code>: Constant Contact encountered an error when sending the email campaign activity.<li> </ul>
- reason_code int? - The reason why the send attempt completed or encountered an error. This method returns <code>0</code> if Constant Contact successfully sent the email campaign activity to contacts. Possible <code>reason_code</code> values are: <ul> <li>0 — Constant Contact successfully sent the email to contacts.</li> <li>1 — An error occurred when sending this email. Try scheduling it again, or contact <a href='http://support.constantcontact.com' target='_blank'>Customer Support</a>.</li> <li>2 — We were unable to send the email. Please contact our <a href='http://knowledgebase.constantcontact.com/articles/KnowledgeBase/5782-contact-an-account-review-and-deliverability-specialist' target='_blank'>Account Review Team</a> for more information.</li> <li>3 — This Constant Contact account cannot currently send emails. This can be due to billing or product expiration.</li> <li>4 — You're not able to send the email to that many contacts. Remove contacts from the contact lists you are using or select a list with fewer contacts.</li> <li>5 — The email is currently in staging. For more information, see the <a href='http://knowledgebase.constantcontact.com/articles/KnowledgeBase/7402-email-staging' target='_blank>Email Staging Knowledge Base article</a>.</li> <li>6 — Constant Contact was unable to finish sending this email to all of the contacts on your list. Please contact <a href='http://support.constantcontact.com' target='_blank'>Customer Support</a> for more information.</li> <li>7 — The email contains invalid images. This can be caused when one or more images in the email are longer available in your image library.</li> <li>8 — The email contains a link URL that exceeds 1005 characters.</li> <li>9 — Constant Contact was unable to verify your authenticated Sender address. Please contact <a href='http://support.constantcontact.com' target='_blank'>Customer Support</a> for more information.</li> <li>10 — Constant Contact was unable to verify your authenticated Sender address. Please contact <a href='http://support.constantcontact.com' target='_blank'>Customer Support</a> for more information.</li> <li>11 — This Constant Contact account cannot send survey invitations.</li> <li>12 — Constant Contact attempted to send the email, but there were no eligible contacts to send it to. This can be caused by an invalid contact list, a contact list with no contacts, or a contact list with no new contacts during a resend. This method displays <code>reason_code</code> 12 as a send attempt with a <code>send_status</code> of COMPLETED and a <code>count</code> of 0.</li> </ul>
constantcontact: EmailTestSendInput
Fields
- email_addresses string[] - The recipients of the test email as an array of email address strings. You can send a test email to up to 5 different email addresses at a time.
- personal_message string? - A personal message for the recipients of the test email. Constant Contact displays this message before the email campaign activity content.
constantcontact: ForwardsTrackingActivitiesPage
Fields
- tracking_activities ForwardsTrackingActivity[] - The list of contacts that forwarded the specified email campaign activity.
- _links Links2? - Links_2
constantcontact: ForwardsTrackingActivity
Fields
- contact_id string - The ID that uniquely identifies a contact.
- campaign_activity_id string - The ID that uniquely identifies the email campaign activity.
- tracking_activity_type string - The type of report tracking activity that is associated with the specified <code>campaign_activity_id</code>.
- email_address string - The contact's email address.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- created_time string - The time that the contact forwarded the email campaign activity.
- deleted_at string? - If applicable, displays the date that the contact was deleted.
constantcontact: JsonImportContact
Fields
- email string - The email address of the contact. This method identifies each unique contact using their email address.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- job_title string? - The job title of the contact.
- company_name string? - The name of the company where the contact works.
- birthday_month int? - The month value for the contact's birthday. Valid values are from 1 through 12. The <code>birthday_month</code> property is required if you use <code>birthday_day</code>.
- birthday_day int? - The day value for the contact's birthday. Valid values are from 1 through 31. The <code>birthday_day</code> property is required if you use <code>birthday_month</code>.
- anniversary string? - The anniversary date for the contact. For example, this value could be the date when the contact first became a customer of an organization in Constant Contact. Valid date formats are MM/DD/YYYY, M/D/YYYY, YYYY/MM/DD, YYYY/M/D, YYYY-MM-DD, YYYY-M-D,M-D-YYYY, or M-DD-YYYY.
- phone string? - The phone number for the contact.
- street string? - Line one of the street address for the contact.
- street2 string? - Line two of the street address for the contact. This value is automatically appended to the <code>street</code> value.
- city string? - The name of the city where the contact lives.
- state string? - The name of the state or province where the contact lives.
- zip string? - The zip or postal code of the contact.
- country string? - The name of the country where the contact lives.
- 'cf\:custom\_field\_name string? - The name of this property is dynamic based on the custom fields you want to import. Use a key-value pair where the key is an existing custom field name prefixed with <code>cf:</code>, and the value is a custom field string value. For example, if you have a custom field named <code>first_name</code> you can use <code>"cf:first_name":"Joe"</code>. Each contact can contain up to 25 different custom fields.
constantcontact: Link
Link
Fields
- href string? - Link
constantcontact: Link2
Fields
- href string? -
constantcontact: Links
Fields
- next Next -
constantcontact: Links2
Links_2
Fields
- href string? - Link
- next Next2? - The next link in the page or null if there are no additional pages
constantcontact: ListActivityAddContacts
Fields
- 'source ListactivityaddcontactsSource - The <code>source</code> object specifies which contacts you are adding to your targeted lists using one of four mutually exclusive properties.
- list_ids string[] - Specifies which lists (up to 50) you are adding your source contacts to.
constantcontact: ListactivityaddcontactsSource
The source
object specifies which contacts you are adding to your targeted lists using one of four mutually exclusive properties.
Fields
- list_ids string[]? - Specifies which contacts you are adding to lists as an array of up to 50 contact <code>list_id</code> values. This property is mutually exclusive with <code>contact_ids</code>, <code>all_active_contacts</code>, and <code>segment_id</code>.
- all_active_contacts boolean? - Adds all active contacts to your targeted lists. This property is mutually exclusive with <code>contact_ids</code>, <code>list_ids</code>, and <code>segment_id</code>.
- contact_ids string[]? - Specifies which contacts (up to 500) you are adding to lists as an array of <code>contact_id</code> values. This property is mutually exclusive with <code>list_ids</code>, <code>all_active_contacts</code>, and <code>segment_id</code>.
- segment_id int? - Specifies which contacts you are adding to lists as a single <code>segment_id</code> value. This property is mutually exclusive with <code>list_ids</code>, <code>all_active_contacts</code>, and <code>contact_ids</code>.
constantcontact: ListActivityRemoveContacts
Fields
- 'source ListactivityremovecontactsSource - The <code>source</code> object specifies which contacts to remove from your targeted lists using one of three mutually exclusive properties.
- list_ids string[] - Specifies which lists (up to 50) to remove your source contacts from.
constantcontact: ListactivityremovecontactsSource
The source
object specifies which contacts to remove from your targeted lists using one of three mutually exclusive properties.
Fields
- list_ids string[]? - Specifies which contacts to remove from your target lists as an array of up to 50 contact <code>list_id</code> values. This property is mutually exclusive with <code>contact_ids</code> and <code>all_active_contacts</code>.
- all_active_contacts boolean? - Removes all active contacts from your targeted lists. This property is mutually exclusive with <code>contact_ids</code> and <code>list_ids</code>.
- contact_ids string[]? - Specifies which contacts to remove from your target lists as an array of <code>contact_id</code> values. This property is mutually exclusive with <code>list_ids</code> and <code>all_active_contacts</code>.
constantcontact: ListInput
Fields
- name string - The name given to the contact list
- favorite boolean(default false) - Identifies whether or not the account has favorited the contact list.
- description string? - Text describing the list.
constantcontact: ListXref
The cross-referenced pair of V3 API list_id
and V2 API sequence_id
for a list. Response is sorted ascending by sequence_id
.
Fields
- sequence_id string? - The V2 API list unique identifier
- list_id string? - The V3 API list unique identifier
constantcontact: ListXrefs
Fields
- xrefs ListXref[]? - An array of cross-referenced V3 API <code>list_id</code> and V2 API <code>sequence_id</code> properties. Response is sorted ascending by <code>sequence_id</code>.
constantcontact: Next
constantcontact: Next2
The next link in the page or null if there are no additional pages
Fields
- href string - The next link in the page or null if there are no additional pages.
constantcontact: Note
A note about the contact.
Fields
- note_id string? - The ID that uniquely identifies the note (UUID format).
- created_at string? - The date that the note was created.
- content string? - The content for the note.
constantcontact: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://idfed.constantcontact.com/as/token.oauth2") - Refresh URL
constantcontact: OpensTrackingActivitiesPage
Fields
- tracking_activities OpensTrackingActivity[] - Lists contacts that opened the specified <code>campaign_activity_id</code>.
- _links Links2? - Links_2
constantcontact: OpensTrackingActivity
Fields
- contact_id string - The ID that uniquely identifies a contact.
- campaign_activity_id string - The ID that uniquely identifies an email campaign activity.
- tracking_activity_type string - The type of tracking activity that is associated with this <code>campaign_activity_id</code> and used for reporting purposes.
- email_address string - The email address used to send the email campaign activity to a contact.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- device_type string? - The type of device that the contact used to open the email campaign activity.
- created_time string - The date and time that the contact opened the email campaign activity.
- deleted_at string? - If applicable, displays the date that the contact was deleted.
constantcontact: OptoutsTrackingActivitiesPage
Fields
- tracking_activities OptoutsTrackingActivity[] - The list of opt-out tracking activities
- _links Links2 - Links_2
constantcontact: OptoutsTrackingActivity
Fields
- contact_id string - The ID that uniquely identifies a contact.
- campaign_activity_id string - The ID that uniquely identifies the email campaign activity.
- tracking_activity_type string - The type of report tracking activity that is associated with the specified <code>campaign_activity_id</code>.
- email_address string - The contact's email address.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- opt_out_reason string? - The opt-out reason, if the contact entered a reason.
- created_time string - The time that the contact chose to opt-out from receiving future email campaign activities.
- deleted_at string? - If applicable, displays the date that the contact was deleted.
constantcontact: PagedEmailCampaignResponse
Fields
- _links Paginglinks2? -
- campaigns EmailCampaigns[]? -
constantcontact: PaginationLinks
Fields
- next Link2? -
constantcontact: PagingLinks
Paging links
Fields
- next Link? - Link
constantcontact: Paginglinks2
Fields
- next Links2? - Links_2
constantcontact: PartnerAccount
Fields
- site_owner_list PartneraccountSiteOwnerList[] - Lists all Constant Contact client accounts that are managed under a partner account.
- _links PaginationLinks? -
constantcontact: PartneraccountSiteOwnerList
Fields
- encoded_account_id string? - The obfuscated ID used to uniquely identify a client account.
- subscriber_count int? - The total number of subscriber contacts that are associated with a client account.
- organization_name string? - The name of the organization associated with this client account.
- site_owner_name string? - The user name that identifies a client account.
- billing_status string? - The client's account billing status. When you first create a client account the
billing status
defaults toTrial
. Billing status values include: <ul> <li><code>Trial</code> - A non-paying trial client account (default value).</li> <li><code>Open</code> - An active and paying client account.</li> <li><code>Canceled</code> - A canceled client account.</li> <li><code>Trial End</code> - The trial period has ended for this client account.</li> </ul>
- last_login_date string? - The system generated date and time (ISO-8601) showing when the client last logged into their Constant Contact account. If a client has not logged into their account, the value is
null
. This property does not display in the results.
constantcontact: PercentsEmail
Fields
- bounce decimal? - Percentage of emails sent to unique recipients that bounced.
- click decimal? - Percentage of recipients who opened the email who also clicked one or more links in it.
- desktop_click decimal? - Percentage of clicks that came from a standard desktop or laptop computer.
- desktop_open decimal? - Percentage of opens that came from a standard desktop or laptop computer.
- did_not_open decimal? - Percentage of recipients that received the email (did not bounce) but did not open it.
- mobile_click decimal? - Percentage of clicks that came from a mobile phone, tablet computer, or similar small mobile device (e.g. iPhone or iPad).
- mobile_open decimal? - Percentage of opens that came from a mobile phone, tablet computer, or similar small mobile device (e.g. iPhone or iPad).
- open decimal? - Percentage of recipients that received the email (did not bounce) and opened it.
- unsubscribe decimal? - Percentage of recipients that received the email (did not bounce) and chose to unsubscribe.
constantcontact: PhoneNumber
Fields
- phone_number_id string? - Unique ID for the phone number
- phone_number string? - The contact's phone number, 1 of 2 allowed per contact, no more than 25 characters
- kind string? - Describes the type of phone number; valid values are home, work, mobile, or other.
- created_at string? - Date and time that the street address was created, in ISO-8601 format. System generated.
- updated_at string? - Date and time that the phone_number was last updated, in ISO-8601 format. System generated.
- update_source string? - Identifies who last updated the phone_number; valid values are Contact or Account
- create_source string? - Describes who added the phone_number; valid values are Contact or Account.
constantcontact: PhoneNumberPut
Fields
- phone_number string? - The contact's phone number, 1 of 2 allowed per contact, no more than 25 characters
- kind string? - Describes the type of phone number; valid values are home, work, mobile, or other.
constantcontact: PlanInfo
Specifies the type of billing plan (plan_type
) and the billing date (billing_day_of_month
) used for a client account.
Fields
- plan_type string? - The type of billing plan (<code>plan_type</code>) to associate with a client's Constant Contact account. The type of billing plan determines which Constant Contact product features that the client account can access. The billing plan type that you enter must already exist in your plan group or a 400 error message is returned. After changing the <code>plan_type</code> from <code>TRIAL</code> to any other billing plan type, you cannot change it back to <code>TRIAL</code>. <ul> <li><code>TRIAL</code>: A non-billed account with an expiration date that allows clients to try Constant Contact product features.</li> <li><code>GOOD</code>: A billed <b>Email</b> client account plan.</li> <li><code>BETTER</code>: A billed <b>Email Plus</b> client account plan.</li> <li><code>BEST</code>: A billed <b>Personal Marketer</b> client account plan.</li> <li><code>LITE</code>: A <b>Website Builder</b> client account plan.</li> </ul>
- billing_day_of_month int? - This property is required if a client account is not set up to use single billing. You can choose to enter a specific day of the month or accept the default value, which is the day on which the <code>plan_type</code> value changes from a <code>TRIAL</code> plan to a different <code>plan_type</code>. For trial accounts, the value defaults to null. You can only change the <code>billing_day_of_month</code> when changing the <code>plan_type</code> value from <code>TRIAL</code> to a different <code>plan_type</code>, otherwise the value you enter is ignored.
constantcontact: PlanTiersObject
Specifies client billing plan details including the type of plan, the plan tiers used, the current billing status, and the day of the month that the client is billed. When a client account is first provisioned, the plan_type
defaults to a Trial
account. After you change an account billing_status
from Trial
to any other billing_status
, you cannot change it back to a Trial
account.
Fields
- plan_type string? - The billing plan that is associated with a client's Constant Contact account. The billing plan determines which Constant Contact product features that the client account can access. <ul> <li><code>TRIAL</code>: A non-billed account with an expiration date that allows clients to try limited Constant Contact product features.</li> <li><code>GOOD</code>: A billed <b>Email</b> client account plan.</li> <li><code>BETTER</code>: A billed <b>Email Plus</b> client account plan.</li> <li><code>BEST</code>: A billed <b>Personal Marketer</b> client account plan.</li> <li><code>LITE</code>: A <b>Website Builder</b> client account plan.</li> </ul>
- current_tiers TierObject[]? - Lists the billing plan tiers that are currently associated with a client account.
- billing_status string? - The client's account billing status. When you first create a client account the <code>billing_status</code> defaults to <code>Trial</code>. Billing status values include: <ul> <li><code>Trial</code> - A non-paying trial client account (default value).</li> <li><code>Open</code> - An active and paying client account.</li> <li><code>Canceled</code> - A canceled client account.</li> <li><code>Trial End</code> - The trial period has ended for this client account.</li> </ul>
- billing_day_of_month int? - This property is required when an account is not set up to use single billing. For trial accounts, the value is intially set to <code>null</code>. The value can only be changed when changing the <code>plan_type</code> from a trial account to a different type of plan, otherwise the value you enter is ignored. You can choose to enter a specific day of month or except the default value, which is the day that the <code>plan_type</code> value changes from a trial account plan to a different plan. Valid <code>billing_day_of_month</code> values include <code>1</code> through and including <code>31</code>.
constantcontact: Provision
Fields
- contact_email string - A valid email address to associate with the client account.
- contact_phone string? - The contact phone number to associate with the client account.
- country_code string - The two-letter country code (ISO 3166-1 code) that specifies the country in which the client resides.
- organization_name string? - The name of organization that identifies the client account.
- organization_phone string? - The organization phone number. To set the organization phone number using the user interface, select <b>My Settings</b> and in the <b>Organization Information</b> section, select <b>Edit Organization Information</b>.
- state_code string - The two-letter state code that represents the US state (<code>country_code</code> is <code>US</code> ) or Canadian province (<code>country_code</code> is <code>CA</code>) where the client's organization is physically located. Leave the <code>state_code</code> blank for non-US states and Canadian provinces.
- time_zone_id string? - The offical time zone to use to represent the physical location associated with the client account.
- website string? - The client's website URL. Specifying the website URL eliminates the need for clients to provide that information. Requires a valid URL starting with http:// or https://.
- login_name string - A unique login name to associate with the client account. The name must only contain alphanumeric characters and '-', '_', '@','.','+'.
- password string? - Required if not using Single Sign On (SSO) or external authenticator. The password to associate with the client account. Passwords must be a minimum of six characters in length and have no spaces. The password is not returned in the response payload for security reasons. If using SSO authentication, use <code>external_provider</code> and <code>external_id</code> instead of <code>password</code>.
- first_name string? - The client account owner's first name.
- last_name string? - The client account owner's last name.
- partner_account_id string? - The unique client account identifier that partners define and use for billing and reporting purposes.
- billing_locale string? - The currency to use when billing the client account. Valid values are: <code>en_US</code> (default, US Dollars) or <code>en_GB</code> (British Pounds).
- managed_site_owner boolean? - By default, if the client account is setup as a managed account <code>managed_site_owner</code> is automatically set to <code>true</code> and attempting to override the setting with <code>false</code> is ignored. This helps to avoid getting an account into an unknown state.
- enable_single_billing boolean? - If a partner account is setup to allow for single billing and the <code>managed_site_owner</code> property is set to <code>true</code>, use this property to enable the single billing feature for the client account. See your account manager for more information.
- gdpr_opt_out boolean? - When creating accounts for users who have opted-out of any marketing communications, set the <code> gdpr_opt_out</code> to <code>true</code> so that Constant Contact does not send any marketing communications to the account.
- external_id string? - The ID used to uniquely identify the client account for the external authenticator. Do not use the <code>password</code> property when using an external authenticator.
- external_provider string? - The name of the provider who externally authenticates this customer. For example, PayPal or Yahoo. Do not use the <code>password</code> property when using an external authenticator.
constantcontact: ProvisionResponse
Fields
- encoded_account_id string? - The system generated ID used to uniquely identify a client account.
- provision_uuid string? - The system generated ID used to uniquely identify the provisioning of a client account.
constantcontact: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
constantcontact: SegmentData
Fields
- name string - The segment's unique descriptive name.
- segment_criteria string - The <code>segment_criteria</code> specifies the contact data that Constant Contact uses to evaluate and identify contacts that meet your criteria. The <code>segment_criteria</code> must be formatted as single-string escaped JSON. The top-level <code>group</code> <code>type</code> must be <code>add</code>.
constantcontact: SegmentDetail
Fields
- name string? - The segment's unique descriptive name.
- segment_criteria string? - The segment's contact selection criteria formatted as single-string escaped JSON.
- segment_id int? - The system generated number that uniquely identifies the segment.
- created_at string? - The system generated date and time (ISO-8601) that the segment was created.
- edited_at string? - The system generated date and time (ISO-8601) that the segment's <code>name</code> or <code> segment_criteria</code> was last updated.
constantcontact: SegmentMaster
Fields
- name string? - The segment's unique descriptive name.
- segment_id int? - The system generated number that uniquely identifies the segment.
- created_at string? - The system generated date and time that the segment was created (ISO-8601 format).
- edited_at string? - The system generated date and time that the segment's <code>name</code> or <code>segment_criteria</code> was last updated (ISO-8601 format).
constantcontact: SegmentName
Fields
- name string - The segment's unique descriptive name.
constantcontact: SegmentsDTO
Fields
- segments SegmentMaster[] - Lists all segments for the account.
- _links Links? -
constantcontact: SendsTrackingActivitiesPage
Fields
- tracking_activities SendsTrackingActivity[] - Lists the contacts to which the email campaign activity (<code>campaign_activity_id</code>) was sent.
- _links Links2? - Links_2
constantcontact: SendsTrackingActivity
Fields
- contact_id string - The ID that uniquely identifies a contact.
- campaign_activity_id string - The ID that uniquely identifies an email campaign activity.
- tracking_activity_type string - The type of tracking activity that is associated with this <code>campaign_activity_id</code> and used for reporting purposes.
- email_address string - The email address used to send the email campaign activity to a contact.
- first_name string? - The first name of the contact.
- last_name string? - The last name of the contact.
- created_time string - The date and time that you sent the email campaign to the contact.
- deleted_at string? - If applicable, displays the date that the contact was deleted.
constantcontact: StatsEmail
Fields
- em_bounces int? - Number of unique email bounces.
- em_clicks int? - Number of unique recipients who clicked any link in the email.
- em_clicks_all int? - Total number of non-unique email clicks.
- em_clicks_all_computer int? - Number of non-unique email clicks on a standard desktop or laptop computer.
- em_clicks_all_mobile int? - Number of non-unique email clicks on a mobile phone or similar small mobile device (e.g. iPhone).
- em_clicks_all_tablet int? - Number of non-unique email clicks on a small tablet type computer (e.g. iPad).
- em_clicks_all_other int? - Number of non-unique email clicks on an unknown device (e.g. Game Console or Wearable).
- em_clicks_all_none int? - Number of non-unique email clicks for which the device type was not captured. This will account for any clicks prior to when device type was collected and stored.
- em_forwards int? - Number of unique recipients who forwarded the email using the forward to a friend feature (not available for all types of emails).
- em_not_opened int? - Number of unique recipients who did not open the email. This is calculated as follows: <code>em_not_opened</code> is equal to <code>em_sends - em_opens - em_bounces</code>. This value is reported as zero if the calculation results in a negative value.
- em_opens int? - Number of unique recipients who opened the email.
- em_opens_all int? - Total number of non-unique email opens.
- em_opens_all_computer int? - Number of non-unique email opens on a standard desktop or laptop computer.
- em_opens_all_mobile int? - Number of non-unique email opens on a mobile phone or similar small mobile device (e.g. iPhone).
- em_opens_all_tablet int? - Number of non-unique email opens on a small tablet type computer (e.g. iPad).
- em_opens_all_other int? - Number of non-unique email opens on an unknown device (e.g. Game Console or Wearable).
- em_opens_all_none int? - Number of non-unique email opens for which the device type was not captured. This will account for any opens prior to when device type was collected and stored.
- em_optouts int? - Number of unique recipients who unsubscribed due to this email.
- em_sends int? - Number of unique email sends.
constantcontact: StatsEmailActivity
Fields
- em_bounces int? - Number of unique email bounces.
- em_clicks int? - Number of unique recipients who clicked any link in the email.
- em_clicks_all int? - Total number of non-unique email clicks.
- em_clicks_all_computer int? - Number of non-unique email clicks on a standard desktop or laptop computer.
- em_clicks_all_mobile int? - Number of non-unique email clicks on a mobile phone or similar small mobile device (e.g. iPhone).
- em_clicks_all_tablet int? - Number of non-unique email clicks on a small tablet type computer (e.g. iPad).
- em_clicks_all_other int? - Number of non-unique email clicks on an unknown device (e.g. Game Console or Wearable).
- em_clicks_all_none int? - Number of non-unique email clicks for which the device type was not captured. This will account for any clicks prior to when device type was collected and stored.
- em_forwards int? - Number of unique recipients who forwarded the email using the forward to a friend feature (not available for all types of emails).
- em_not_opened int? - Number of unique recipients who did not open the email. This is calculated as follows: <code>em_not_opened</code> is equal to <code>em_sends - em_opens - em_bounces</code>. This value is reported as zero if the calculation results in a negative value.
- em_opens int? - Number of unique recipients who opened the email.
- em_opens_all int? - Total number of non-unique email opens.
- em_opens_all_computer int? - Number of non-unique email opens on a standard desktop or laptop computer.
- em_opens_all_mobile int? - Number of non-unique email opens on a mobile phone or similar small mobile device (e.g. iPhone).
- em_opens_all_tablet int? - Number of non-unique email opens on a small tablet type computer (e.g. iPad).
- em_opens_all_other int? - Number of non-unique email opens on an unknown device (e.g. Game Console or Wearable).
- em_opens_all_none int? - Number of non-unique email opens for which the device type was not captured. This will account for any opens prior to when device type was collected and stored.
- em_optouts int? - Number of unique recipients who unsubscribed due to this email.
- em_sends int? - Number of unique email sends.
- em_abuse int? - Number of abuse (spam) complaints received.
- em_bounces_blocked int? - Unique number bounced because as blocked by the receiving system.
- em_bounces_mailbox_full int? - Unique number that bounced back with a mailbox full message.
- em_bounces_nonexistent_address int? - Unique number that bounced as a non-existent address.
- em_bounces_other int? - Unique number that bounced without an identifiable cause.
- em_bounces_suspended int? - Unique number that bounced as suspended. Email address bounces as suspended when multiple non-existent bounces have been received for the same address.
- em_bounces_undeliverable int? - Unique number that bounced as undeliverable.
- em_bounces_vacation int? - Unique number that bounced back with a vacation or out of office autoreply.
constantcontact: StatsError
Fields
- error_key string? - The unique error key.
- error_message string? - A error description.
constantcontact: StreetAddress
Fields
- street_address_id string? - Unique ID for the street address
- kind string - Describes the type of address; valid values are home, work, or other.
- street string? - Number and street of the address.
- city string? - The name of the city where the contact lives.
- state string? - The name of the state or province where the contact lives.
- postal_code string? - The zip or postal code of the contact.
- country string? - The name of the country where the contact lives.
- created_at string? - Date and time that the street address was created, in ISO-8601 format. System generated.
- updated_at string? - Date and time that the street address was last updated, in ISO-8601 format. System generated.
constantcontact: StreetAddressPut
Fields
- kind string - Describes the type of address; valid values are home, work, or other.
- street string? - Number and street of the address.
- city string? - The name of the city where the contact lives.
- state string? - The name of the state or province where the contact lives.
- postal_code string? - The zip or postal code of the contact.
- country string? - The name of the country where the contact lives.
constantcontact: Tag
Fields
- tag_id string? - The ID that uniquely identifies a tag (UUID format)
- name string? - The unique tag name.
- contacts_count int? - The total number of contacts who are assigned this tag.
- created_at string? - The system generated date and time when the tag was created (ISO-8601 format).
- updated_at string? - The system generated date and time when the tag was last updated (ISO-8601 format).
- tag_source string? - The source used to tag contacts.
constantcontact: Tag2
Fields
- tag_id string? - The ID that uniquely identifies a tag (UUID format)
- name string? - The unique tag name.
- contacts_count int? - The total number of contacts who are assigned this tag.
- created_at string? - The system generated date and time when the tag was created (ISO-8601 format).
- updated_at string? - The system generated date and time when the tag was last updated (ISO-8601 format).
- tag_source string? - The source used to tag contacts.
constantcontact: TagAddRemoveContacts
Fields
- 'source TagaddremovecontactsSource - Select the source used to identify contacts to which a tag is added or removed. Source types are mutually exclusive.
- exclude TagaddremovecontactsExclude? - Use to exclude specified contacts from being added or removed from a tag. Only applicable if the specified source is either <code>all_active_contacts</code> or <code>list_ids</code>.
- tag_ids string[] - An array of tags (<code>tag_id</code>) to add to all contacts meeting the specified source criteria.
constantcontact: TagaddremovecontactsExclude
Use to exclude specified contacts from being added or removed from a tag. Only applicable if the specified source is either all_active_contacts
or list_ids
.
Fields
- contact_ids string[]? - Identifies the contacts, by <code>contact_id</code>, to exclude from the add or remove tags activity.
constantcontact: TagaddremovecontactsSource
Select the source used to identify contacts to which a tag is added or removed. Source types are mutually exclusive.
Fields
- contact_ids string[]? - An array of contacts IDs.
- list_ids string[]? - An array of list IDs ( <code>list_id</code> ).
- tag_ids string[]? - An array of tags ( <code>tag_id</code> ).
- all_active_contacts boolean? - Use to identify contacts with an active status.
- new_subscriber boolean? - Use to identify newly subscribed contacts.
constantcontact: TagIdList500Limit
Fields
- tag_ids string[] - The tag IDs (<code>tag_ids</code>) to delete.
constantcontact: TagPost
Fields
- name string - Specify a unique name to use to identify the tag. Tag names must be at least one character in length and not more than 255 characters.
- tag_source string? - The source used to identify the contacts to tag.
constantcontact: TagPut
Fields
- name string - The new tag name to use. The tag name must be unique.
constantcontact: Tags
Fields
- tags Tag2[]? - Lists all tags and provides tag details.
- _links Paginglinks2? -
constantcontact: TierObject
The usage tier that is associated with a client's Constant Contact account and is used to calculate the monthly billing price.
Fields
- usage_type string? - Identifies the tier usage type that is associated with the billing plan.
- current_usage float? - The number of active contacts (default) used within the current tier.
- tier int? - The billing tier level that is associated with a client account. By default, the system determines the tier level to use based on the number of active contacts currently in the client account at the time of billing. Billing tiers may differ. The following shows an example billing tier: <ul> <li>level <code>1</code> = 0 - 500 contacts</li> <li>level <code>2</code> = 501 - 1000 contacts</li> <li>level <code>3</code> = 1001 - ...</li> </ul>
- tier_min float? - The minimum number of active contacts for the tier level.
- tier_max float? - The maximum number of active contacts for the tier level.
- price float? - The billing price set for the tier used to bill the client account each month.
- currency_code string? - The currency (ISO currency code) used to price the tier for a client account.
constantcontact: UniqueEmailCounts
Fields
- sends int - The total number of unique sends.
- opens int - The total number of unique opens.
- clicks int - The total number of unique clicks.
- forwards int - The total number of unique forwards.
- optouts int - The total number of unique optouts (unsubscribes).
- abuse int - The total number of unique abuse count (spam).
- bounces int - The total number of unique bounce count.
- not_opened int - The total number of unique non-opens
constantcontact: UserprivilegesresourceInner
Fields
- privilege_id int? - Identifies a user privilege in Constant Contact.
- privilege_name string? - The name of the Constant Contact user privilege.
constantcontact: WebhooksSubscription
Fields
- topic_id int? - Identifies the topic using an integer to indicate the type of topic.
- hook_uri string? - Your webhook callback URI. Constant Contact automatically sends POST requests to this URI to notify you about the topic.
- topic_name string? - The name of the topic.
- topic_description string? - A description of what the topic is and when Constant Contact notifies you concerning it.
constantcontact: WebhooksSubscriptionBody
Fields
- hook_uri string? - The callback URI you would like to use to receive webhook notifications. Constant Contact will automatically send POST notifications about your chosen topic to this URI.
constantcontact: WebhooksSubscriptionPutResp
Fields
- topic_id int? - Identifies the topic using an integer to indicate the type of topic.
- hook_uri string? - Your webhook callback URI. Constant Contact automatically sends POST requests to this URI to notify you about the topic.
constantcontact: WebhooksSubscriptionResponse
Fields
- topic_id int? - Identifies the topic using an integer to indicate the type of topic.
- hook_uri string? - Your webhook callback URI. Constant Contact automatically sends POST requests to this URI to notify you about the topic.
- topic_name string? - The name of the topic.
- topic_description string? - A description of what the topic is and when Constant Contact notifies you concerning it.
constantcontact: WebhooksTestSend
Fields
- topic_id int? - Identifies the topic using an integer to indicate the type of topic.
- hook_uri string? - The webhook callback URI. Constant Contact automatically sends POST requests to this URI to notify you about the topic.
- topic_name string? - The name of the topic.
- topic_description string? - A description of what the topic is and when Constant Contact notifies you concerning it. When you use the test send API method, Constant Contact will immediately send a test event to you.
Import
import ballerinax/constantcontact;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Marketing/Marketing Automation
Cost/Paid
Contributors