cloudmersive.virusscan
Module cloudmersive.virusscan
API
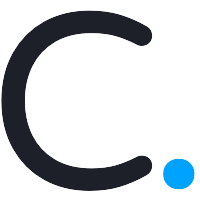
ballerinax/cloudmersive.virusscan Ballerina library
Overview
This is a generated connector from Cloudmersive OpenAPI specification.
The Cloudmersive Virus Scan API lets you scan files and content for viruses and identify security issues with content.
Prerequisites
- Create a Cloudmersive account
- Obtain tokens
- Use this guide to obtain the API key related to your account.
Quickstart
To use the Cloudmersive Virus Scan connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/cloudmersive.virusscan module into the Ballerina project.
import ballerinax/cloudmersive.virusscan;
Step 2 - Create a new connector instance
You can now make the connection configuration using the access token.
virusscan:ApiKeysConfig config = { apikey : "<your apiKey>" }; virusscan:Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
- Get the scan result
virusscan:WebsiteScanRequest address = { Url: "https://www.yahoo.com/" }; virusscan:WebsiteScanResult|error bEvent = baseClient->scanWebsite(address); if (bEvent is virusscan:WebsiteScanResult) { log:printInfo("Result: " + bEvent.toString()); } else { log:printError(msg = bEvent.toString()); }
- Use
bal run
command to compile and run the Ballerina program
Clients
cloudmersive.virusscan: Client
This is a generated connector from Cloudmersive OpenAPI specification. The Cloudmersive Virus Scan API lets you scan files and content for viruses and identify security issues with content.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Cloudmersive account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://testapi.cloudmersive.com/" - URL of the target service
scanWebsite
function scanWebsite(WebsiteScanRequest payload) returns WebsiteScanResult|error
Scan a website for malicious content and threats
Parameters
- payload WebsiteScanRequest -
Return Type
- WebsiteScanResult|error - OK
scanCloudStorageScanAzureBlob
function scanCloudStorageScanAzureBlob(string connectionString, string containerName, string blobPath) returns CloudStorageVirusScanResult|error
Scan an Azure Blob for viruses
Parameters
- connectionString string - Connection string for the Azure Blob Storage Account; you can get this connection string from the Access Keys tab of the Storage Account blade in the Azure Portal.
- containerName string - Name of the Blob container within the Azure Blob Storage account
- blobPath string - Path to the blob within the container, such as 'hello.pdf' or '/folder/subfolder/world.pdf'
Return Type
scanCloudStorageScanAzureBlobAdvanced
function scanCloudStorageScanAzureBlobAdvanced(string connectionString, string containerName, string blobPath, boolean? allowExecutables, boolean? allowInvalidFiles, boolean? allowScripts, boolean? allowPasswordProtectedFiles, boolean? allowMacros, string? restrictFileTypes) returns CloudStorageAdvancedVirusScanResult|error
Advanced Scan an Azure Blob for viruses
Parameters
- connectionString string - Connection string for the Azure Blob Storage Account; you can get this connection string from the Access Keys tab of the Storage Account blade in the Azure Portal.
- containerName string - Name of the Blob container within the Azure Blob Storage account
- blobPath string - Path to the blob within the container, such as 'hello.pdf' or '/folder/subfolder/world.pdf'
- allowExecutables boolean? (default ()) - Set to false to block executable files (program code) from being allowed in the input file. Default is false (recommended).
- allowInvalidFiles boolean? (default ()) - Set to false to block invalid files, such as a PDF file that is not really a valid PDF file, or a Word Document that is not a valid Word Document. Default is false (recommended).
- allowScripts boolean? (default ()) - Set to false to block script files, such as a PHP files, Python scripts, and other malicious content or security threats that can be embedded in the file. Set to true to allow these file types. Default is false (recommended).
- allowPasswordProtectedFiles boolean? (default ()) - Set to false to block password protected and encrypted files, such as encrypted zip and rar files, and other files that seek to circumvent scanning through passwords. Set to true to allow these file types. Default is false (recommended).
- allowMacros boolean? (default ()) - Set to false to block macros and other threats embedded in document files, such as Word, Excel and PowerPoint embedded Macros, and other files that contain embedded content threats. Set to true to allow these file types. Default is false (recommended).
- restrictFileTypes string? (default ()) - Specify a restricted set of file formats to allow as clean as a comma-separated list of file formats, such as .pdf,.docx,.png would allow only PDF, PNG and Word document files. All files must pass content verification against this list of file formats, if they do not, then the result will be returned as CleanResult=false. Set restrictFileTypes parameter to null or empty string to disable; default is disabled.
Return Type
scanCloudStorageScanAwsS3file
function scanCloudStorageScanAwsS3file(string accessKey, string secretKey, string bucketRegion, string bucketName, string keyName) returns CloudStorageVirusScanResult|error
Scan an AWS S3 file for viruses
Parameters
- accessKey string - AWS S3 access key for the S3 bucket; you can get this from My Security Credentials in the AWS console
- secretKey string - AWS S3 secret key for the S3 bucket; you can get this from My Security Credentials in the AWS console
- bucketRegion string - Name of the region of the S3 bucket, such as 'US-East-1'
- bucketName string - Name of the S3 bucket
- keyName string - Key name (also called file name) of the file in S3 that you wish to scan for viruses
Return Type
scanCloudStorageScanAwsS3fileAdvanced
function scanCloudStorageScanAwsS3fileAdvanced(string accessKey, string secretKey, string bucketRegion, string bucketName, string keyName, boolean? allowExecutables, boolean? allowInvalidFiles, boolean? allowScripts, boolean? allowPasswordProtectedFiles, boolean? allowMacros, string? restrictFileTypes) returns CloudStorageAdvancedVirusScanResult|error
Advanced Scan an AWS S3 file for viruses
Parameters
- accessKey string - AWS S3 access key for the S3 bucket; you can get this from My Security Credentials in the AWS console
- secretKey string - AWS S3 secret key for the S3 bucket; you can get this from My Security Credentials in the AWS console
- bucketRegion string - Name of the region of the S3 bucket, such as 'US-East-1'
- bucketName string - Name of the S3 bucket
- keyName string - Key name (also called file name) of the file in S3 that you wish to scan for viruses
- allowExecutables boolean? (default ()) - Set to false to block executable files (program code) from being allowed in the input file. Default is false (recommended).
- allowInvalidFiles boolean? (default ()) - Set to false to block invalid files, such as a PDF file that is not really a valid PDF file, or a Word Document that is not a valid Word Document. Default is false (recommended).
- allowScripts boolean? (default ()) - Set to false to block script files, such as a PHP files, Python scripts, and other malicious content or security threats that can be embedded in the file. Set to true to allow these file types. Default is false (recommended).
- allowPasswordProtectedFiles boolean? (default ()) - Set to false to block password protected and encrypted files, such as encrypted zip and rar files, and other files that seek to circumvent scanning through passwords. Set to true to allow these file types. Default is false (recommended).
- allowMacros boolean? (default ()) - Set to false to block macros and other threats embedded in document files, such as Word, Excel and PowerPoint embedded Macros, and other files that contain embedded content threats. Set to true to allow these file types. Default is false (recommended).
- restrictFileTypes string? (default ()) - Specify a restricted set of file formats to allow as clean as a comma-separated list of file formats, such as .pdf,.docx,.png would allow only PDF, PNG and Word document files. All files must pass content verification against this list of file formats, if they do not, then the result will be returned as CleanResult=false. Set restrictFileTypes parameter to null or empty string to disable; default is disabled.
Return Type
Records
cloudmersive.virusscan: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apikey string - Represents API Key
Apikey
cloudmersive.virusscan: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
cloudmersive.virusscan: CloudStorageAdvancedVirusScanResult
Result of running an advanced virus scan on cloud storage
Fields
- Successful boolean? - True if the operation of retrieving the file, and scanning it were successfully completed, false if the file could not be downloaded from cloud storage, or if the file could not be scanned. Note that successful completion does not mean the file is clean; for the output of the virus scanning operation itself, use the CleanResult and FoundViruses parameters.
- CleanResult boolean? - True if the scan contained no viruses, false otherwise
- ContainsExecutable boolean? - True if the scan contained an executable (application code), which can be a significant risk factor
- ContainsInvalidFile boolean? - True if the scan contained an invalid file (such as a PDF that is not a valid PDF, Word Document that is not a valid Word Document, etc.), which can be a significant risk factor
- ContainsScript boolean? - True if the scan contained a script (such as a PHP script, Python script, etc.) which can be a significant risk factor
- ContainsPasswordProtectedFile boolean? - True if the scan contained a password protected or encrypted file, which can be a significant risk factor
- ContainsRestrictedFileFormat boolean? - True if the uploaded file is of a type that is not allowed based on the optional restrictFileTypes parameter, false otherwise; if restrictFileTypes is not set, this will always be false
- ContainsMacros boolean? - True if the uploaded file contains embedded Macros of other embedded threats within the document, which can be a significant risk factor
- VerifiedFileFormat string? - For file format verification-supported file formats, the contents-verified file format of the file. Null indicates that the file format is not supported for contents verification. If a Virus or Malware is found, this field will always be set to Null.
- FoundViruses CloudStorageVirusFound[]? - Array of viruses found, if any
- ErrorDetailedDescription string? - Detailed error message if the operation was not successful
- FileSize int? - Size in bytes of the file that was retrieved and scanned
cloudmersive.virusscan: CloudStorageVirusFound
Virus positively identified
Fields
- FileName string? - Name of the file containing the virus
- VirusName string? - Name of the virus that was found
cloudmersive.virusscan: CloudStorageVirusScanResult
Result of running a virus scan on cloud storage
Fields
- Successful boolean? - True if the operation of retrieving the file, and scanning it were successfully completed, false if the file could not be downloaded from cloud storage, or if the file could not be scanned. Note that successful completion does not mean the file is clean; for the output of the virus scanning operation itself, use the CleanResult and FoundViruses parameters.
- CleanResult boolean? - True if the scan contained no viruses, false otherwise
- FoundViruses CloudStorageVirusFound[]? - Array of viruses found, if any
- ErrorDetailedDescription string? - Detailed error message if the operation was not successful
- FileSize int? - Size in bytes of the file that was retrieved and scanned
cloudmersive.virusscan: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
cloudmersive.virusscan: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
cloudmersive.virusscan: VirusFound
Virus positively identified
Fields
- FileName string? - Name of the file containing the virus
- VirusName string? - Name of the virus that was found
cloudmersive.virusscan: VirusScanAdvancedResult
Result of running an advanced virus scan
Fields
- CleanResult boolean? - True if the scan contained no viruses, false otherwise
- ContainsExecutable boolean? - True if the scan contained an executable (application code), which can be a significant risk factor
- ContainsInvalidFile boolean? - True if the scan contained an invalid file (such as a PDF that is not a valid PDF, Word Document that is not a valid Word Document, etc.), which can be a significant risk factor
- ContainsScript boolean? - True if the scan contained a script (such as a PHP script, Python script, etc.) which can be a significant risk factor
- ContainsPasswordProtectedFile boolean? - True if the scan contained a password protected or encrypted file, which can be a significant risk factor
- ContainsRestrictedFileFormat boolean? - True if the uploaded file is of a type that is not allowed based on the optional restrictFileTypes parameter, false otherwise; if restrictFileTypes is not set, this will always be false
- ContainsMacros boolean? - True if the uploaded file contains embedded Macros of other embedded threats within the document, which can be a significant risk factor
- ContainsXmlExternalEntities boolean? - True if the uploaded file contains embedded XML External Entity threats of other embedded threats within the document, which can be a significant risk factor
- VerifiedFileFormat string? - For file format verification-supported file formats, the contents-verified file format of the file. Null indicates that the file format is not supported for contents verification. If a Virus or Malware is found, this field will always be set to Null.
- FoundViruses VirusFound[]? - Array of viruses found, if any
cloudmersive.virusscan: VirusScanResult
Result of running a virus scan
Fields
- CleanResult boolean? - True if the scan contained no viruses, false otherwise
- FoundViruses VirusFound[]? - Array of viruses found, if any
cloudmersive.virusscan: WebsiteScanRequest
Request to scan a website for malicious content
Fields
- Url string? - URL of the website to scan; should begin with http:// or https://
cloudmersive.virusscan: WebsiteScanResult
Result of running a website scan
Fields
- CleanResult boolean? - True if the scan contained no threats, false otherwise
- WebsiteThreatType string? - Type of threat returned; can be None, Malware, ForcedDownload or Phishing
- FoundViruses VirusFound[]? - Array of viruses found, if any
- WebsiteHttpResponseCode int? - The remote server URL HTTP reasponse code; useful for debugging issues with scanning; typically if the remote server returns a 200 or 300-series code this means a successful response, while a 400 or 500 series code would represent an error returned from the remote server for the provided URL.
Import
import ballerinax/cloudmersive.virusscan;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 2
Weekly downloads
Keywords
IT Operations/Security & Identity Tools
Cost/Freemium
Contributors
Dependencies