cloudmersive.validate
Module cloudmersive.validate
API
Definitions
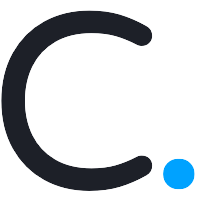
ballerinax/cloudmersive.validate Ballerina library
Overview
This is a generated connector from Cloudmersive OpenAPI specification.
The Cloudmersive Validation APIs help you validate data. Check if an E-mail address is real. Check if a domain is real. Check up on an IP address, and even where it is located. All this and much more is available in the validation API.
Prerequisites
- Create a Cloudmersive account
- Obtain tokens
- Use this guide to obtain the API key related to your account.
Quickstart
To use the Cloudmersive Validate connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/cloudmersive.validate module into the Ballerina project.
import ballerinax/cloudmersive.validate;
Step 2 - Create a new connector instance
You can now make the connection configuration using the access token.
validate:ApiKeysConfig config = { apikey : "<your apiKey>" }; validate:Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
- Obtain the parsed address
validate:ParseAddressRequest address = { AddressString: "Cecilia Chapman 711-2880 Nulla St. Mankato Mississippi 96522 (257) 563-7401", CapitalizationMode: "UpperCase" }; validate:ParseAddressResponse|error bEvent = baseClient->addressParseString(address); if (bEvent is validate:ParseAddressResponse) { log:printInfo("Parsed address: " + bEvent.toString()); } else { log:printError(msg = bEvent.toString()); }
- Use
bal run
command to compile and run the Ballerina program
Clients
cloudmersive.validate: Client
This is a generated connector from Cloudmersive OpenAPI specification. The Cloudmersive Validation APIs help you validate data. Check if an E-mail address is real. Check if a domain is real. Check up on an IP address, and even where it is located. All this and much more is available in the validation API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Cloudmersive account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://testapi.cloudmersive.com/" - URL of the target service
addressParseString
function addressParseString(ParseAddressRequest payload) returns ParseAddressResponse|error
Parse an unstructured input text string into an international, formatted address
Parameters
- payload ParseAddressRequest - Input parse request
Return Type
addressValidateAddress
function addressValidateAddress(ValidateAddressRequest payload) returns ValidateAddressResponse|error
Validate a street address
Parameters
- payload ValidateAddressRequest - Input parse request
Return Type
addressNormalizeAddress
function addressNormalizeAddress(ValidateAddressRequest payload) returns NormalizeAddressResponse|error
Normalize a street address
Parameters
- payload ValidateAddressRequest - Input parse request
Return Type
addressValidateCity
function addressValidateCity(ValidateCityRequest payload) returns ValidateCityResponse|error
Validate a City and State/Province combination, get location information about it
Parameters
- payload ValidateCityRequest - Input parse request
Return Type
addressValidateState
function addressValidateState(ValidateStateRequest payload) returns ValidateStateResponse|error
Validate a state or province, name or abbreviation, get location information about it
Parameters
- payload ValidateStateRequest - Input parse request
Return Type
addressValidatePostalCode
function addressValidatePostalCode(ValidatePostalCodeRequest payload) returns ValidatePostalCodeResponse|error
Validate a postal code, get location information about it
Parameters
- payload ValidatePostalCodeRequest - Input parse request
Return Type
addressCountry
function addressCountry(ValidateCountryRequest payload) returns ValidateCountryResponse|error
Validate and normalize country information, return ISO 3166-1 country codes and country name
Parameters
- payload ValidateCountryRequest - Input request
Return Type
addressCountryList
function addressCountryList() returns CountryListResult|error
Get a list of ISO 3166-1 countries
Return Type
- CountryListResult|error - OK
addressCheckEumembership
function addressCheckEumembership(ValidateCountryRequest payload) returns ValidateCountryResponse|error
Check if a country is a member of the European Union (EU)
Parameters
- payload ValidateCountryRequest - Input request
Return Type
addressGetCountryCurrency
function addressGetCountryCurrency(ValidateCountryRequest payload) returns ValidateCountryResponse|error
Get the currency of the input country
Parameters
- payload ValidateCountryRequest - Input request
Return Type
addressGetCountryRegion
function addressGetCountryRegion(ValidateCountryRequest payload) returns ValidateCountryResponse|error
Get the region, subregion and continent of the country
Parameters
- payload ValidateCountryRequest - Input request
Return Type
addressGetTimezone
function addressGetTimezone(GetTimezonesRequest payload) returns GetTimezonesResponse|error
Gets IANA/Olsen time zones for a country
Parameters
- payload GetTimezonesRequest - Input request
Return Type
addressGeocode
function addressGeocode(ValidateAddressRequest payload) returns ValidateAddressResponse|error
Geocode a street address into latitude and longitude
Parameters
- payload ValidateAddressRequest - Input parse request
Return Type
addressReverseGeocodeAddress
function addressReverseGeocodeAddress(ReverseGeocodeAddressRequest payload) returns ReverseGeocodeAddressResponse|error
Reverse geocode a lattitude and longitude into an address
Parameters
- payload ReverseGeocodeAddressRequest - Input reverse geocoding request
Return Type
dateTimeGetNowSimple
function dateTimeGetNowSimple() returns DateTimeNowResult|error
Get current date and time as of now
Return Type
- DateTimeNowResult|error - OK
dateTimeGetPublicHolidays
function dateTimeGetPublicHolidays(GetPublicHolidaysRequest payload) returns PublicHolidaysResponse|error
Get public holidays in the specified country and year
Parameters
- payload GetPublicHolidaysRequest - Input request
Return Type
dateTimeParseStandardDateTime
function dateTimeParseStandardDateTime(DateTimeStandardizedParseRequest payload) returns DateTimeStandardizedParseResponse|error
Parses a standardized date and time string into a date and time
Parameters
- payload DateTimeStandardizedParseRequest - Input request
Return Type
dateTimeParseNaturalLanguageDateTime
function dateTimeParseNaturalLanguageDateTime(DateTimeNaturalLanguageParseRequest payload) returns DateTimeStandardizedParseResponse|error
Parses a free-form natural language date and time string into a date and time
Parameters
- payload DateTimeNaturalLanguageParseRequest - Input request
Return Type
domainCheck
function domainCheck(string payload) returns CheckResponse|error
Validate a domain name
Parameters
- payload string - Domain name to check, for example "cloudmersive.com". The input is a string so be sure to enclose it in double-quotes.
Return Type
- CheckResponse|error - OK
domainQualityScore
function domainQualityScore(string payload) returns DomainQualityResponse|error
Validate a domain name's quality score
Parameters
- payload string - Domain name to check, for example "cloudmersive.com".
Return Type
domainPost
function domainPost(string payload) returns WhoisResponse|error
Get WHOIS information for a domain
Parameters
- payload string - Domain name to check, for example "cloudmersive.com". The input is a string so be sure to enclose it in double-quotes.
Return Type
- WhoisResponse|error - OK
domainUrlSyntaxOnly
function domainUrlSyntaxOnly(ValidateUrlRequestSyntaxOnly payload) returns ValidateUrlResponseSyntaxOnly|error
Validate a URL syntactically
Parameters
- payload ValidateUrlRequestSyntaxOnly - Input URL information
Return Type
domainUrlFull
function domainUrlFull(ValidateUrlRequestFull payload) returns ValidateUrlResponseFull|error
Validate a URL fully
Parameters
- payload ValidateUrlRequestFull - Input URL request
Return Type
domainGetTopLevelDomainFromUrl
function domainGetTopLevelDomainFromUrl(ValidateUrlRequestSyntaxOnly payload) returns ValidateUrlResponseSyntaxOnly|error
Get top-level domain name from URL
Parameters
- payload ValidateUrlRequestSyntaxOnly - Input URL information
Return Type
domainPhishingCheck
function domainPhishingCheck(PhishingCheckRequest payload) returns PhishingCheckResponse|error
Check a URL for Phishing threats
Parameters
- payload PhishingCheckRequest - Input URL request
Return Type
domainIsAdminPath
function domainIsAdminPath(string payload) returns IsAdminPathResponse|error
Check if path is a high-risk or vulnerable server administration path
Parameters
- payload string - URL or relative path to check, e.g. "/admin/login". The input is a string so be sure to enclose it in double-quotes.
Return Type
- IsAdminPathResponse|error - OK
domainSafetyCheck
function domainSafetyCheck(UrlSafetyCheckRequestFull payload) returns UrlSafetyCheckResponseFull|error
Check a URL for safety threats
Parameters
- payload UrlSafetyCheckRequestFull - Input URL request
Return Type
domainSsrfCheck
function domainSsrfCheck(UrlSsrfRequestFull payload) returns UrlSsrfResponseFull|error
Check a URL for SSRF threats
Parameters
- payload UrlSsrfRequestFull - Input URL request
Return Type
- UrlSsrfResponseFull|error - OK
domainSsrfCheckBatch
function domainSsrfCheckBatch(UrlSsrfRequestBatch payload) returns UrlSsrfResponseBatch|error
Check a URL for SSRF threats in batches
Parameters
- payload UrlSsrfRequestBatch - Input URL request as a batch of multiple URLs
Return Type
domainUrlHtmlSsrfCheck
function domainUrlHtmlSsrfCheck(UrlHtmlSsrfRequestFull payload) returns UrlHtmlSsrfResponseFull|error
Check a URL for HTML embedded SSRF threats
Parameters
- payload UrlHtmlSsrfRequestFull - Input URL request
Return Type
emailPost
function emailPost(string payload) returns AddressVerifySyntaxOnlyResponse|error
Validate email adddress for syntactic correctness only
Parameters
- payload string - Email address to validate, e.g. "support@cloudmersive.com". The input is a string so be sure to enclose it in double-quotes.
Return Type
emailAddressGetServers
function emailAddressGetServers(string payload) returns AddressGetServersResponse|error
Partially check whether an email address is valid
Parameters
- payload string - Email address to validate, e.g. "support@cloudmersive.com". The input is a string so be sure to enclose it in double-quotes.
Return Type
emailFullValidation
function emailFullValidation(string payload) returns FullEmailValidationResponse|error
Fully validate an email address
Parameters
- payload string - Email address to validate, e.g. "support@cloudmersive.com". The input is a string so be sure to enclose it in double-quotes.
Return Type
ipaddressIpIntelligence
function ipaddressIpIntelligence(string payload) returns IPIntelligenceResponse|error
Get intelligence on an IP address
Parameters
- payload string - IP address to process, e.g. "55.55.55.55". The input is a string so be sure to enclose it in double-quotes.
Return Type
ipaddressPost
function ipaddressPost(string payload) returns GeolocateResponse|error
Geolocate an IP address
Parameters
- payload string - IP address to geolocate, e.g. "55.55.55.55". The input is a string so be sure to enclose it in double-quotes.
Return Type
- GeolocateResponse|error - OK
ipaddressGeolocateStreetAddress
function ipaddressGeolocateStreetAddress(string payload) returns GeolocateStreetAddressResponse|error
Geolocate an IP address to a street address
Parameters
- payload string - IP address to geolocate, e.g. "55.55.55.55". The input is a string so be sure to enclose it in double-quotes.
Return Type
ipaddressIsThreat
function ipaddressIsThreat(string payload) returns IPThreatResponse|error
Check if IP address is a known threat
Parameters
- payload string - IP address to check, e.g. "55.55.55.55". The input is a string so be sure to enclose it in double-quotes.
Return Type
- IPThreatResponse|error - OK
ipaddressIsTorNode
function ipaddressIsTorNode(string payload) returns TorNodeResponse|error
Check if IP address is a Tor node server
Parameters
- payload string - IP address to check, e.g. "55.55.55.55". The input is a string so be sure to enclose it in double-quotes.
Return Type
- TorNodeResponse|error - OK
ipaddressIsBot
function ipaddressIsBot(string payload) returns BotCheckResponse|error
Check if IP address is a Bot client
Parameters
- payload string - IP address to check, e.g. "55.55.55.55". The input is a string so be sure to enclose it in double-quotes.
Return Type
- BotCheckResponse|error - OK
ipaddressReverseDomainLookup
function ipaddressReverseDomainLookup(string payload) returns IPReverseDNSLookupResponse|error
Perform a reverse domain name (DNS) lookup on an IP address
Parameters
- payload string - IP address to check, e.g. "55.55.55.55". The input is a string so be sure to enclose it in double-quotes.
Return Type
leadEnrichmentEnrichLead
function leadEnrichmentEnrichLead(LeadEnrichmentRequest payload) returns LeadEnrichmentResponse|error
Enrich an input lead with additional fields of data
Parameters
- payload LeadEnrichmentRequest - Input lead with known fields set, and unknown fields left blank (null)
Return Type
nameValidateFullName
function nameValidateFullName(FullNameValidationRequest payload) returns FullNameValidationResponse|error
Parse and validate a full name
Parameters
- payload FullNameValidationRequest - Validation request information
Return Type
nameValidateFirstName
function nameValidateFirstName(FirstNameValidationRequest payload) returns FirstNameValidationResponse|error
Validate a first name
Parameters
- payload FirstNameValidationRequest - Validation request information
Return Type
nameValidateLastName
function nameValidateLastName(LastNameValidationRequest payload) returns LastNameValidationResponse|error
Validate a last name
Parameters
- payload LastNameValidationRequest - Validation request information
Return Type
nameGetGender
function nameGetGender(GetGenderRequest payload) returns GetGenderResponse|error
Get the gender of a first name
Parameters
- payload GetGenderRequest - Gender request information
Return Type
- GetGenderResponse|error - OK
nameIdentifier
function nameIdentifier(ValidateIdentifierRequest payload) returns ValidateIdentifierResponse|error
Validate a code identifier
Parameters
- payload ValidateIdentifierRequest - Identifier validation request information
Return Type
phoneNumberSyntaxOnly
function phoneNumberSyntaxOnly(PhoneNumberValidateRequest payload) returns PhoneNumberValidationResponse|error
Validate phone number (basic)
Parameters
- payload PhoneNumberValidateRequest - Phone number to validate in a PhoneNumberValidateRequest object. Try a phone number such as "1.800.463.3339", and either leave DefaultCountryCode blank or use "US".
Return Type
textInputCheckSqlInjection
function textInputCheckSqlInjection(string payload, string? detectionLevel) returns SqlInjectionDetectionResult|error
Check text input for SQL Injection (SQLI) attacks
Parameters
- payload string - User-facing text input.
- detectionLevel string? (default ()) - Set to Normal to target a high-security SQL Injection detection level with a very low false positive rate; select High to target a very-high security SQL Injection detection level with higher false positives. Default is Normal (recommended).
Return Type
textInputCheckSqlInjectionBatch
function textInputCheckSqlInjectionBatch(SqlInjectionCheckBatchRequest payload) returns SqlInjectionCheckBatchResponse|error
Check and protect multiple text inputs for SQL Injection (SQLI) attacks in batch
Parameters
- payload SqlInjectionCheckBatchRequest - User-facing text input.
Return Type
textInputCheckXss
function textInputCheckXss(string payload) returns XssProtectionResult|error
Check text input for Cross-Site-Scripting (XSS) attacks
Parameters
- payload string - User-facing text input.
Return Type
- XssProtectionResult|error - OK
textInputProtectXss
function textInputProtectXss(string payload) returns XssProtectionResult|error
Protect text input from Cross-Site-Scripting (XSS) attacks through normalization
Parameters
- payload string - User-facing text input.
Return Type
- XssProtectionResult|error - OK
textInputCheckXssBatch
function textInputCheckXssBatch(XssProtectionBatchRequest payload) returns XssProtectionBatchResponse|error
Check and protect multiple text inputs for Cross-Site-Scripting (XSS) attacks in batch
Parameters
- payload XssProtectionBatchRequest - User-facing text input.
Return Type
textInputCheckHtmlSsrf
function textInputCheckHtmlSsrf(string payload) returns HtmlSsrfDetectionResult|error
Protect html input from Server-side Request Forgery (SSRF) attacks
Parameters
- payload string - User-facing HTML input.
Return Type
textInputCheckXxe
function textInputCheckXxe(string payload, boolean? allowInternetUrls, string? knownSafeUrls, string? knownUnsafeUrls) returns XxeDetectionResult|error
Protect text input from XML External Entity (XXE) attacks
Parameters
- payload string - User-facing text input.
- allowInternetUrls boolean? (default ()) - Optional: Set to true to allow Internet-based dependency URLs for DTDs and other XML External Entitites, set to false to block. Default is false.
- knownSafeUrls string? (default ()) - Optional: Comma separated list of fully-qualified URLs that will automatically be considered safe.
- knownUnsafeUrls string? (default ()) - Optional: Comma separated list of fully-qualified URLs that will automatically be considered unsafe.
Return Type
- XxeDetectionResult|error - OK
textInputCheckXxeBatch
function textInputCheckXxeBatch(XxeDetectionBatchRequest payload) returns XxeDetectionBatchResponse|error
Protect text input from XML External Entity (XXE) attacks
Parameters
- payload XxeDetectionBatchRequest -
Return Type
userAgentParse
function userAgentParse(UserAgentValidateRequest payload) returns UserAgentValidateResponse|error
Parse an HTTP User-Agent string, identify robots
Parameters
- payload UserAgentValidateRequest - Input parse request
Return Type
vatVatLookup
function vatVatLookup(VatLookupRequest payload) returns VatLookupResponse|error
Validate a VAT number
Parameters
- payload VatLookupRequest - Input VAT code
Return Type
- VatLookupResponse|error - OK
Records
cloudmersive.validate: AddressGetServersResponse
Result of a partial email address validation
Fields
- Success boolean? - True if partial address validation was successufl, false otherwise
- Servers string[]? - Email servers for this email address
cloudmersive.validate: AddressVerifySyntaxOnlyResponse
Syntactic validity of email address
Fields
- ValidAddress boolean? - True if the email address is syntactically valid, false if it is not
- Domain string? - Domain name of the email address
- IsFreeEmailProvider boolean? - True if the email domain name is a free provider (typically a free to sign up web email provider for consumers / personal use), false otherwise.
- IsDisposable boolean? - True if the email address is a disposable email address, false otherwise; these disposable providers are not typically used to receive email and so will have a low likelihood of opening mail sent there.
cloudmersive.validate: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apikey string - Represents API Key
Apikey
cloudmersive.validate: BotCheckResponse
Result of performing a Bot check on an IP address
Fields
- IsBot boolean? - True if the input IP address is a Bot or Robot, false otherwise
cloudmersive.validate: CheckResponse
Result of a validation operation
Fields
- ValidDomain boolean? - True if the domain name was valid, false if it is not
cloudmersive.validate: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
cloudmersive.validate: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
cloudmersive.validate: CountryDetails
Details of one country
Fields
- CountryName string? - Name of the country
- ThreeLetterCode string? - Three-letter ISO 3166-1 country code
- ISOTwoLetterCode string? - Two-letter ISO 3166-1 country code
- IsEuropeanUnionMember boolean? - True if this country is currently a member of the European Union (EU), false otherwise
- ISOCurrencyCode string? - ISO 4217 currency three-letter code associated with the country
- CurrencySymbol string? - Symbol associated with the currency
- CurrencyEnglishName string? - Full name of the currency
- Region string? - Region (continent) in which the country is located; possible values are None, Europe, Americas, Asia, Africa, Oceania
- Subregion string? - Subregion in which the country is located; possible values are None, NorthernEurope, WesternEurope, SouthernEurope, EasternEurope, CentralAmerica, NorthernAmerica, SouthAmerica, EasternAfrica, MiddleAfrica, NorthernAfrica , SouthernAfrica , WesternAfrica , CentralAsia , EasternAsia , SouthernAsia , SouthEasternAsia , WesternAsia , Southern , Middle , AustraliaandNewZealand , Melanesia , Polynesia , Micronesia , Caribbean,
cloudmersive.validate: CountryListResult
Result of enumerating available countries
Fields
- Successful boolean? - True if the operation was successful, false otherwise
- Countries CountryDetails[]? - List of current ISO 3166-1 countries in the world
cloudmersive.validate: DateTimeNaturalLanguageParseRequest
Input parameter to a date time parsing request
Fields
- RawDateTimeInput string? - Raw string input of a natural language-formatted date and time for parsing
cloudmersive.validate: DateTimeNowResult
Current date and time response
Fields
- Successful boolean? - True if successful, false otherwise
- Now string? - Current date, time, and time zone in standard JSON date format
- NowGmt string? - Current GMT-time-zone date, time, and time zone in standard JSON date format
cloudmersive.validate: DateTimeStandardizedParseRequest
Input parameter to a date time parsing request
Fields
- RawDateTimeInput string? - Raw string input of a standard-formatted date and time for parsing
- CountryCode string? - Optional: specify the two-letter country code to optimzie date formatting; default is US
cloudmersive.validate: DateTimeStandardizedParseResponse
Result of performing a date time parsing
Fields
- Successful boolean? - True if successful, false otherwise
- ParsedDateResult string? - Result of performing a date time parsing
- Year int? - Year of the parsed date time result
- Month int? - Month of the parsed date time result
- Day int? - Day of the parsed date time result
- Hour int? - Hour of the parsed date time result (24-hour)
- Minute int? - Minute of the parsed date time result
- Second int? - Second of the parsed date time result
- DayOfWeek string? - Day of week
cloudmersive.validate: DomainQualityResponse
Result of performing a domain quality score operation
Fields
- DomainQualityScore decimal? - The quality score of the domain name; possible values are 0.0 to 10.0 with 10.0 being the highest and 0.0 being the lowest quality.
cloudmersive.validate: FirstNameValidationRequest
Request to validate a first name
Fields
- FirstName string? - First name to process
cloudmersive.validate: FirstNameValidationResponse
Result of a first name validation operation
Fields
- Successful boolean? - True if the validation operation was successful, false otherwise
- ValidationResult string? - Possible values are: ValidFirstName, ValidUnknownFirstName, InvalidSpamInput, InvalidCharacters, InvalidEmpty
cloudmersive.validate: FullEmailValidationResponse
Full email addresss validation result
Fields
- ValidAddress boolean? - True if the email address is valid overall, false otherwise
- MailServerUsedForValidation string? - Email server connected to for verification
- Valid_Syntax boolean? - True if the syntax of the email address is valid, false otherwise. This is one component of ValidAddress, but not the only one.
- Valid_Domain boolean? - True if the domain name of the email address is valid, false otherwise. This is one component of ValidAddress, but not the only one.
- Valid_SMTP boolean? - True if the email address was verified by the remote server, false otherwise. This is one component of ValidAddress, but not the only one.
- IsCatchallDomain boolean? - True if the domain is a catch-all domain name, false otherwise. Catch-all domain names, while rare, always accept inbound email to ensure they do not lose any potentially useful emails. Catch-all domain names can occassionally be configured to first accept and store all inbound email, but then later send a bounce email back to the sender after a delayed period of time.
- Domain string? - Domain name of the email address
- IsFreeEmailProvider boolean? - True if the email domain name is a free provider (typically a free to sign up web email provider for consumers / personal use), false otherwise.
- IsDisposable boolean? - True if the email address is a disposable email address, false otherwise; these disposable providers are not typically used to receive email and so will have a low likelihood of opening mail sent there.
cloudmersive.validate: FullNameValidationRequest
Request to validate a full name string
Fields
- FullNameString string? - Full name to process as a free-form string; supports many components such as First Name, Middle Name, Last Name, Title, Nickname, Suffix, and Display Name
cloudmersive.validate: FullNameValidationResponse
Result of a full name validation operation
Fields
- Successful boolean? - True if the validation operation was successful, false otherwise
- ValidationResult_FirstName string? - Possible values are: ValidFirstName, ValidUnknownFirstName, InvalidSpamInput, InvalidCharacters, InvalidEmpty
- ValidationResult_LastName string? - Possible values are: ValidLastName, ValidUnknownLastName, InvalidSpamInput, InvalidCharacters, InvalidEmpty
- Title string? - The person's title (if supplied), e.g. "Mr." or "Ms."
- FirstName string? - The first name (given name)
- MiddleName string? - The middle name(s); if there are multiple names they will be separated by spaces
- LastName string? - The last name (surname)
- NickName string? - Nickname (if supplied)
- Suffix string? - Suffix to the name, e.g. "Jr." or "Sr."
- DisplayName string? - The full display name of the name
cloudmersive.validate: GeolocateResponse
Geolocation result of performing an IP address geolocation operation. This product includes GeoLite2 data created by MaxMind, available from www.maxmind.com.
Fields
- CountryCode string? - Two-letter country code of IP address
- CountryName string? - Country name of IP address
- City string? - City of IP address
- RegionCode string? - State/region code of IP address
- RegionName string? - State/region of IP address
- ZipCode string? - Zip or postal code of IP address
- TimezoneStandardName string? - Timezone of IP address
- Latitude decimal? - Latitude of IP address
- Longitude decimal? - Longitude of IP address
cloudmersive.validate: GeolocateStreetAddressResponse
Geolocation street address result
Fields
- CountryCode string? - Two-letter country code of IP address
- CountryName string? - Country name of IP address
- StreetAddress string? - Street address of IP address
- City string? - City of IP address
- RegionName string? - State/region of IP address
- ZipCode string? - Zip or postal code of IP address
cloudmersive.validate: GetGenderRequest
Request to get the gender from a first name
Fields
- FirstName string? - Input first name (given name) to get the gender of
- CountryCode string? - Optional; the country for this name, possible values are "US", "LY", "NI", "TT", "MK", "KZ", "BO", "UG", "TZ", "CL", "SI", "MA", "RW", "VN", "AW", "CY", "BH", "SG", "ZA", "MU", "BR", "TN", "KH", "US", "TH", "TW", "UY", "DO", "CO", "UA", "QA", "BY", "SN", "SD", "FJ", "LB", "BE", "ML", "LV", "FR", "TM", "NG", "EC", "NO", "SL", "CR", "PA", "GE", "CH", "KR", "RS", "ZM", "FI", "BF", "MC", "AU", "GA", "LS", "RU", "IN", "SE", "LK", "BZ", "MX", "GH", "AF", "TJ", "BN", "DZ", "CM", "GR", "MD", "HN", "AT", "NZ", "SV", "GW", "NA", "AR", "MZ", "PK", "MN", "IQ", "BW", "VE", "PT", "BS", "AL", "TG", "ID", "ET", "CF", "JP", "BB", "PH", "CU", "BD", "AO", "SM", "LC", "ME", "RO", "DA" "NI", "LO", "ES", "EE", "IL", "ZW", "MW", "LU", "IR", "SC", "NL", "JO", "AM", "DE", "GL", "OM", "DK", "HR", "LI", "TD", "KM", "BA", "GM", "GD", "CA", "CZ", "MR", "ST", "IS", "LR", "IE", "VC", "AE", "KG", "DJ", "TR", "KE", "NE", "UZ", "CN", "GQ", "SK", "BJ", "MG", "BT", "EG", "PL", "IT", "SA", "MY", "CI", "AG", "AD", "KS", "HU", "CG", "KP", "DM", "GN", "GT", "NP", "JM", "LA", "GB", "BG", "HT", "PE", "AZ", "LT", "SZ", "PY", "MT", "VA", "SY"
cloudmersive.validate: GetGenderResponse
Result of the GetGender operation
Fields
- Successful boolean? - True if successful, false otherwise
- Gender string? - Gender for this name; possible values are Male, Female, and Neutral (can be applied to Male or Female)
cloudmersive.validate: GetPublicHolidaysRequest
Input parameter to a country validation request
Fields
- RawCountryInput string? - Two-letter code (FIPS 10-4 or ISO 3166-1) of the country; if not specified, defaults to United States
- Year int? - Optional - the year in which to retrieve the holidays; if left blank (0) it will default to the current year
cloudmersive.validate: GetTimezonesRequest
Request to get time zones for a country
Fields
- CountryCodeOrName string? - Can be the two-letter, three-letter country codes or country name
cloudmersive.validate: GetTimezonesResponse
Result of performing a get time zones operation
Fields
- Successful boolean? - True if successful, false otherwise
- CountryFullName string? - Full name of the country
- ISOTwoLetterCode string? - Two-letter ISO 3166-1 country code
- FIPSTwoLetterCode string? - Two-letter FIPS 10-4 country code
- ThreeLetterCode string? - Three-letter ISO 3166-1 country code
- Timezones Timezone[]? - Time zones (IANA/Olsen) in the country
cloudmersive.validate: HtmlSsrfDetectionResult
Result of performing an XXE threat detection operation
Fields
- Successful boolean? - True if the operation was successful, false otherwise
- ContainedThreats boolean? - True if the input contained SSRF threats, false otherwise
cloudmersive.validate: IPIntelligenceResponse
IP address intelligence result
Fields
- IsBot boolean? - True if the IP address is a known bot, otherwise false
- IsTorNode boolean? - True if the IP address is a known Tor exit node, otherwise false
- IsThreat boolean? - True if the IP address is a known threat IP, otherwise false
- IsEU boolean? - True if the IP address is in the European Union, otherwise false
- Location GeolocateResponse? - Geolocation result of performing an IP address geolocation operation. This product includes GeoLite2 data created by MaxMind, available from www.maxmind.com.
- CurrencyCode string? - ISO 4217 currency code for the IP address location
- CurrencyName string? - Name of the currency in English
- RegionArea string? - Region (continent) in which the country is located; possible values are None, Europe, Americas, Asia, Africa, Oceania
- SubregionArea string? - Subregion in which the country is located; possible values are None, NorthernEurope, WesternEurope, SouthernEurope, EasternEurope, CentralAmerica, NorthernAmerica, SouthAmerica, EasternAfrica, MiddleAfrica, NorthernAfrica , SouthernAfrica , WesternAfrica , CentralAsia , EasternAsia , SouthernAsia , SouthEasternAsia , WesternAsia , Southern , Middle , AustraliaandNewZealand , Melanesia , Polynesia , Micronesia , Caribbean,
cloudmersive.validate: IPReverseDNSLookupResponse
Result of performing a Reverse Domain (DNS) lookup on an IP address
Fields
- Successful boolean? - True if a domain was found, false otherwise
- HostName string? - Host name (fully-qualified) associated with the IP address, if any
cloudmersive.validate: IPThreatResponse
Result of performing a IP threat check on an IP address
Fields
- IsThreat boolean? - True if the input IP address is a threat, false otherwise
- ThreatType string? - Specifies the type of IP threat; possible values include Blocklist, Botnet, WebBot
cloudmersive.validate: IsAdminPathResponse
Result of performing an Admin Path operation
Fields
- IsAdminPathNode boolean? - True if the input IP address is an Admin Path, and false otherwise
- Successful boolean? - True if the operation was successful, false otherwise
cloudmersive.validate: LastNameValidationRequest
Request to validate a last name
Fields
- LastName string? - Last name to process
cloudmersive.validate: LastNameValidationResponse
Result of a last name validation operation
Fields
- Successful boolean? - True if the validation operation was successful, false otherwise
- ValidationResult string? - Possible values are: ValidLastName, ValidUnknownLastName, InvalidSpamInput, InvalidCharacters, InvalidEmpty
cloudmersive.validate: LeadEnrichmentRequest
Input lead contact; fill in known fields to extend them with matched field values
Fields
- ContactBusinessEmail string? - The person's business email address for the lead
- ContactFirstName string? - The person's first name for the lead
- ContactLastName string? - The person's last name for the lead
- CompanyName string? - Name of the company for the lead
- CompanyDomainName string? - Domain name / website for the lead
- CompanyHouseNumber string? - House number of the address of the company for the lead
- CompanyStreet string? - Street name of the address of the company for the lead
- CompanyCity string? - City of the address of the company for the lead
- CompanyStateOrProvince string? - State or Province of the address of the company for the lead
- CompanyPostalCode string? - Postal Code of the address of the company for the lead
- CompanyCountry string? - Country of the address of the company for the lead
- CompanyCountryCode string? - Country Code (2-letter ISO 3166-1) of the address of the company for the lead
- CompanyTelephone string? - Telephone of the company office for the lead
- CompanyVATNumber string? - VAT number of the company for the lead
cloudmersive.validate: LeadEnrichmentResponse
Result of the lead enrichment process
Fields
- Successful boolean? - True if the operation was successful, false otherwise
- LeadType string? - The type of the lead; possible types are Junk (a single individual using a disposable/throwaway email address); Individual (a single individual, typically a consumer, not purchasing on behalf of a business); SmallBusiness (a small business, typically with fewer than 100 employees); MediumBusiness (a medium business, larger than 100 employees but fewer than 1000 employees); Enterprise (a large business with greater than 1000 employees); Business (a business customer of unknown size)
- ContactBusinessEmail string? - The person's business email address for the lead
- ContactFirstName string? - The person's first name for the lead
- ContactLastName string? - The person's last name for the lead
- ContactGender string? - Gender for contact name; possible values are Male, Female, and Neutral (can be applied to Male or Female). Requires ContactFirstName.
- CompanyName string? - Name of the company for the lead
- CompanyDomainName string? - Domain name / website for the lead
- CompanyHouseNumber string? - House number of the address of the company for the lead
- CompanyStreet string? - Street name of the address of the company for the lead
- CompanyCity string? - City of the address of the company for the lead
- CompanyStateOrProvince string? - State or Province of the address of the company for the lead
- CompanyPostalCode string? - Postal Code of the address of the company for the lead
- CompanyCountry string? - Country Name of the address of the company for the lead
- CompanyCountryCode string? - Country Code (2-letter ISO 3166-1) of the address of the company for the lead
- CompanyTelephone string? - Telephone of the company office for the lead
- CompanyVATNumber string? - VAT number of the company for the lead
- EmployeeCount int? - Count of employees at the company (estimated), if available
cloudmersive.validate: NormalizeAddressResponse
Result of validating a street address
Fields
- ValidAddress boolean? - True if the address is valid, false otherwise
- Building string? - The name of the building, house or structure if applicable, such as "Cloudmersive Building 2". This will often by null.
- StreetNumber string? - The street number or house number of the address. For example, in the address "1600 Pennsylvania Avenue NW" the street number would be "1600". This value will typically be populated for most addresses.
- Street string? - The name of the street or road of the address. For example, in the address "1600 Pennsylvania Avenue NW" the street number would be "Pennsylvania Avenue NW".
- City string? - The city of the address.
- StateOrProvince string? - The state or province of the address.
- PostalCode string? - The postal code or zip code of the address.
- CountryFullName string? - Country of the address, if present in the address. If not included in the address it will be null.
- ISOTwoLetterCode string? - Two-letter ISO 3166-1 country code
- Latitude decimal? - If the address is valid, the degrees latitude of the validated address, null otherwise
- Longitude decimal? - If the address is valid, the degrees longitude of the validated address, null otherwise
cloudmersive.validate: ParseAddressRequest
Request to parse an address formatted as a string/free text into a structured address
Fields
- AddressString string? - A mailing address or street address formatted as a single text string; this will be parsed into its components
- CapitalizationMode string? - Optional; indicates how the parsed output should be capitalized; default is Title Case; possible values are: "Uppercase" will set the capitalization to UPPER CASE; "Lowercase" will set the capitalization to lower case; "Titlecase" will set the capitalization to Title Case; and "Originalcase" will preserve the original casing as much as possible
cloudmersive.validate: ParseAddressResponse
Result of parsing an address into its component parts
Fields
- Successful boolean? - True if the parsing operation was successful, false otherwise
- Building string? - The name of the building, house or structure if applicable, such as "Cloudmersive Building 2". This will often by null.
- StreetNumber string? - The street number or house number of the address. For example, in the address "1600 Pennsylvania Avenue NW" the street number would be "1600". This value will typically be populated for most addresses.
- Street string? - The name of the street or road of the address. For example, in the address "1600 Pennsylvania Avenue NW" the street number would be "Pennsylvania Avenue NW".
- City string? - The city of the address.
- StateOrProvince string? - The state or province of the address.
- PostalCode string? - The postal code or zip code of the address.
- CountryFullName string? - Country of the address, if present in the address. If not included in the address it will be null.
- ISOTwoLetterCode string? - Two-letter ISO 3166-1 country code
cloudmersive.validate: PhishingCheckRequest
Request to determine if a URL is a Phishing threat
Fields
- URL string? - URL to validate
cloudmersive.validate: PhishingCheckResponse
Result of checking a URL for Phishing threats
Fields
- CleanURL boolean? - True if the URL is clean, false if it is at risk of containing a Phishing threat or attack
- ThreatType string? - Threat type of the Phishing threat; possible values are VerifiedPhishingURL, UnverifiedPhishingURL, or VerifiedPhishingDomain
cloudmersive.validate: PhoneNumberValidateRequest
Request to validate a phone number
Fields
- PhoneNumber string? - Raw phone number string to parse as input for validation
- DefaultCountryCode string? - Optional, default country code. If left blank, will default to "US".
cloudmersive.validate: PhoneNumberValidationResponse
Result from validating a phone number
Fields
- IsValid boolean? - True if the phone number is valid, false otherwise
- Successful boolean? - True if the operation was successful, false if there was an error during validation. See IsValid for validation result.
- PhoneNumberType string? - Type of phone number; possible values are: FixedLine, Mobile, FixedLineOrMobile, TollFree, PremiumRate, SharedCost, Voip, PersonalNumber, Pager, Uan, Voicemail, Unknown
- E164Format string? - E.164 format of the phone number
- InternationalFormat string? - Internaltional format of the phone number
- NationalFormat string? - National format of the phone number
- CountryCode string? - Two digit country code of the phone number
- CountryName string? - User-friendly long name of the country for the phone number
cloudmersive.validate: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
cloudmersive.validate: PublicHolidayOccurrence
Public holiday occurrence
Fields
- EnglishName string? - Name of the holiday in English
- LocalName string? - Local name of the holiday
- OccurrenceDate string? - Date of the holiday (start time)
- HolidayType string? - Type of the holiday; possible values are: Public, Bank, School, Authorities, Optional, Observance
- Nationwaide boolean? - True if the holiday is celebrated in all locales in the country, false otherwise
cloudmersive.validate: PublicHolidaysResponse
Result of performing a get public holidays request operation
Fields
- Successful boolean? - True if successful, false otherwise
- PublicHolidays PublicHolidayOccurrence[]? - Public holidays in the requested country and year
cloudmersive.validate: ReverseGeocodeAddressRequest
Request to reverse geocode a Street Address
Fields
- Latitude decimal? - Latitude coordinate in WGS84 format
- Longitude decimal? - Longitude coordinate in WGS84 format
cloudmersive.validate: ReverseGeocodeAddressResponse
Result of reverse geocoding a street address
Fields
- Successful boolean? - True if the address operation was successful, false otherwise
- StreetAddress string? - Street address to validate, such as '2950 Buskirk Ave.'
- City string? - City part of the addrerss to validate, such as 'Walnut Creek'
- StateOrProvince string? - State or province of the address to validate, such as 'CA' or 'California'
- PostalCode string? - Zip code or postal code of the address to validate, such as '94597'
- CountryFullName string? - Name of the country, such as 'United States'. Global countries are supported.
- CountryCode string? - Three-letter ISO 3166-1 country code
cloudmersive.validate: SqlInjectionCheckBatchRequest
Input to a batch SQL Injection detection operation
Fields
- RequestItems SqlInjectionCheckRequestItem[]? - Multiple items to detect for SQL Injection
- DetectionLevel string? - Set to Normal to target a high-security SQL Injection detection level with a very low false positive rate; select High to target a very-high security SQL Injection detection level with higher false positives. Default is Normal (recommended).
cloudmersive.validate: SqlInjectionCheckBatchResponse
Result of performing a batch XSS protection operation
Fields
- ResultItems SqlInjectionDetectionResult[]? - Results from performing a batch SQL Injection detection operation; order is preserved from input data
cloudmersive.validate: SqlInjectionCheckRequestItem
Individual item to protect for SQL Injection
Fields
- InputText string? - Individual input text item to check for SQL Injection
cloudmersive.validate: SqlInjectionDetectionResult
Result of performing an SQL Injection protection operation
Fields
- Successful boolean? - True if the operation was successful, false otherwise
- ContainedSqlInjectionAttack boolean? - True if the input contained SQL Injection attacks, false otherwise
- OriginalInput string? - Original input string
cloudmersive.validate: Timezone
IANA/Olsen time zone
Fields
- Name string? - Name of the Time Zone
- BaseUTCOffset string? - UTC offset for this time zone
- Now string? - The current time (Now) in this time zone
cloudmersive.validate: TorNodeResponse
Result of performing a Tor node check on an IP address
Fields
- IsTorNode boolean? - True if the input IP address is a Tor exit node, false otherwise
cloudmersive.validate: UrlHtmlSsrfRequestFull
Request to determine if a URL contains HTML-embedded SSRF threats
Fields
- URL string? - URL to validate
cloudmersive.validate: UrlHtmlSsrfResponseFull
Result of checking a URL for HTML-embedded SSRF threats
Fields
- CleanURL boolean? - True if the URL is clean, false if it is at risk of containing an SSRF threat or attack
- HttpResponseCode int? - HTTP response code from the URL
cloudmersive.validate: UrlSafetyCheckRequestFull
Request to determine if a URL is a safety threat check
Fields
- URL string? - URL to validate
cloudmersive.validate: UrlSafetyCheckResponseFull
Result of checking a URL for safety threats
Fields
- CleanURL boolean? - True if the URL is clean, false if it is at risk of containing a safety threat or attack
- ThreatType string? - Threat type identified, if any; possible values are "ForcedDownload", "VirusesAndMalware", "Phishing"
cloudmersive.validate: UrlSsrfRequestBatch
Batch operation to perform SSRF threat checks on multiple URLs
Fields
- InputItems UrlSsrfRequestFull[]? - Input URLs to check for SSRF threats
cloudmersive.validate: UrlSsrfRequestFull
Request to determine if a URL is an SSRF threat check
Fields
- URL string? - URL to validate
- BlockedDomains string[]? - Top level domains that you do not want to allow access to, e.g. mydomain.com - will block all subdomains as well
cloudmersive.validate: UrlSsrfResponseBatch
Result of performing SSRF threat checks on multiple URLs
Fields
- OutputItems UrlSsrfResponseFull[]? - Results of the operation, with indexes matched to input values
cloudmersive.validate: UrlSsrfResponseFull
Result of checking a URL for SSRF threats
Fields
- CleanURL boolean? - True if the URL is clean, false if it is at risk of containing an SSRF threat or attack
- ThreatLevel string? - Threat level of the URL; possible values are High, Medium, Low and None
cloudmersive.validate: UserAgentValidateRequest
User-Agent parse and validation request
Fields
- UserAgentString string? - The user agent string you wish to parse and validate
cloudmersive.validate: UserAgentValidateResponse
The result of a User-Agent validation request
Fields
- Successful boolean? - True if the operation was successful, false otherwise
- IsBot boolean? - True if the request is a known robot, false otherwise
- BotName string? - Optional; name of the robot if the request was from a known robot, otherwise null
- BotURL string? - Optional; if available, the URL to the robot
- OperatingSystem string? - Operating System of the User-Agent (e.g. Windows)
- OperatingSystemCPUPlatform string? - The CPU platform of the User-Agent (e.g. x64)
- OperatingSystemVersion string? - The version of the operating system of the User-Agent (e.g. "10" for Windows 10)
- DeviceType string? - Device type of the User-Agent; possible values are "DESKTOP", "SMARTPHONE", "TABLET"
- DeviceBrandName string? - Brand name of the User-Agent
- DeviceModel string? - Model name or number of the User-Agent
- BrowserName string? - Name of the Browser
- BrowserVersion string? - Version of the Browser
- BrowserEngineName string? - Name of the Browser Engine
- BrowserEngineVersion string? - Version of the Browser Engine
cloudmersive.validate: ValidateAddressRequest
Request to Validate a Street Address
Fields
- StreetAddress string? - Required; Street address to validate, such as '2950 Buskirk Ave.'
- City string? - Required; City part of the addrerss to validate, such as 'Walnut Creek'
- StateOrProvince string? - Required; State or province of the address to validate, such as 'CA' or 'California'
- PostalCode string? - Optional (recommended); Zip code or postal code of the address to validate, such as '94597'
- CountryFullName string? - Optional (recommended); Name of the country, such as 'United States'. If left blank, and CountryCode is also left blank, will default to United States. Global countries are supported.
- CountryCode string? - Optional; two-letter country code (Two-letter ISO 3166-1 country code) of the country. If left blank, and CountryFullName is also left blank, will default to United States. Global countries are supported.
cloudmersive.validate: ValidateAddressResponse
Result of validating a street address
Fields
- ValidAddress boolean? - True if the address is valid, false otherwise
- Latitude decimal? - If the address is valid, the degrees latitude of the validated address, null otherwise
- Longitude decimal? - If the address is valid, the degrees longitude of the validated address, null otherwise
cloudmersive.validate: ValidateCityRequest
Request to Validate a City and State or Province in a country
Fields
- City string? - Required: City of the address to validate, such as 'San Francisco' or 'London'
- StateOrProvince string? - Required: State or province of the address to validate, such as 'California' or 'CA'
- CountryFullName string? - Optional (recommended); Name of the country, such as 'United States'. If left blank, and CountryCode is also left blank, will default to United States. Global countries are supported.
- CountryCode string? - Optional; two-letter country code (Two-letter ISO 3166-1 country code) of the country. If left blank, and CountryFullName is also left blank, will default to United States. Global countries are supported.
cloudmersive.validate: ValidateCityResponse
Result of validating a city
Fields
- ValidCity boolean? - True if the city is valid, false otherwise
- City string? - If valid, City corresponding to the input postal code, such as 'Walnut Creek'
- StateOrProvince string? - If valid; State or province corresponding to the input state name, such as 'CA' or 'California'
- Latitude decimal? - If the postal code is valid, the degrees latitude of the centroid of the state, null otherwise
- Longitude decimal? - If the postal code is valid, the degrees longitude of the centroid of the state, null otherwise
cloudmersive.validate: ValidateCountryRequest
Input parameter to a country validation request
Fields
- RawCountryInput string? - Raw country input - can be a two-letter code (FIPS 10-4 or ISO 3166-1), three-letter code (ISO 3166-1) or country name
cloudmersive.validate: ValidateCountryResponse
Result of performing a country validation operation
Fields
- Successful boolean? - True if successful, false otherwise
- CountryFullName string? - Full name of the country
- ISOTwoLetterCode string? - Two-letter ISO 3166-1 country code
- FIPSTwoLetterCode string? - Two-letter FIPS 10-4 country code
- ThreeLetterCode string? - Three-letter ISO 3166-1 country code
- IsEuropeanUnionMember boolean? - True if this country is currently a member of the European Union (EU), false otherwise
- Timezones Timezone[]? - Time zones (IANA/Olsen) in the country
- ISOCurrencyCode string? - ISO 4217 currency three-letter code associated with the country
- CurrencySymbol string? - Symbol associated with the currency
- CurrencyEnglishName string? - Full name of the currency
- Region string? - Region (continent) in which the country is located; possible values are None, Europe, Americas, Asia, Africa, Oceania
- Subregion string? - Subregion in which the country is located; possible values are None, NorthernEurope, WesternEurope, SouthernEurope, EasternEurope, CentralAmerica, NorthernAmerica, SouthAmerica, EasternAfrica, MiddleAfrica, NorthernAfrica , SouthernAfrica , WesternAfrica , CentralAsia , EasternAsia , SouthernAsia , SouthEasternAsia , WesternAsia , Southern , Middle , AustraliaandNewZealand , Melanesia , Polynesia , Micronesia , Caribbean,
cloudmersive.validate: ValidateIdentifierRequest
Identifier validation request, including the input identifier as well as various identifier rules
Fields
- Input string? - Text string identifier input
- AllowWhitespace boolean? - True if whitespace is allowed in the identifier, false otherwise
- AllowHyphens boolean? - True if hyphens are allowd in the identifier, false otherwise
- AllowUnderscore boolean? - True if underscores are allowed in the identifier, false otherwise
- AllowNumbers boolean? - True if numbers are allowed in the identifier, false otherwise
- AllowPeriods boolean? - True if periods are allowed in the identifier, false otherwise
- MaxLength int? - Optional; maximum length, if any, of the identifier
- MinLength int? - Optional; minimum length, if any, of the identifier
cloudmersive.validate: ValidateIdentifierResponse
Result of performing an identifier validation operation
Fields
- ValidIdentifier boolean? - True if the input identifier is valid, false otherwise
- Error string? - Resulting error from the identifier validation; possible errors are: "InputIsEmpty", "ContainsWhitespace", "ContainsNumbers", "ContainsHyphen", "ContainsUnderscore", "ContainsPeriod", "TooShort", "TooLong", "ContainsSpecialCharacters"
cloudmersive.validate: ValidatePostalCodeRequest
Request to Validate a Postal Code
Fields
- PostalCode string? - Required: Zip code or postal code of the address to validate, such as '94597'
- CountryFullName string? - Optional (recommended); Name of the country, such as 'United States'. If left blank, and CountryCode is also left blank, will default to United States. Global countries are supported.
- CountryCode string? - Optional; two-letter country code (Two-letter ISO 3166-1 country code) of the country. If left blank, and CountryFullName is also left blank, will default to United States. Global countries are supported.
cloudmersive.validate: ValidatePostalCodeResponse
Result of validating a postal code
Fields
- ValidPostalCode boolean? - True if the Postal Code is valid, false otherwise
- City string? - If valid, City corresponding to the input postal code, such as 'Walnut Creek'
- StateOrProvince string? - If valid; State or province corresponding to the input postal code, such as 'CA' or 'California'
- Latitude decimal? - If the postal code is valid, the degrees latitude of the centroid of the postal code, null otherwise
- Longitude decimal? - If the postal code is valid, the degrees longitude of the centroid of the postal code, null otherwise
cloudmersive.validate: ValidateStateRequest
Request to Validate a State or Province in a country
Fields
- StateOrProvince string? - Required: State or province of the address to validate, such as 'California' or 'CA'
- CountryFullName string? - Optional (recommended); Name of the country, such as 'United States'. If left blank, and CountryCode is also left blank, will default to United States. Global countries are supported.
- CountryCode string? - Optional; two-letter country code (Two-letter ISO 3166-1 country code) of the country. If left blank, and CountryFullName is also left blank, will default to United States. Global countries are supported.
cloudmersive.validate: ValidateStateResponse
Result of validating a state
Fields
- ValidState boolean? - True if the address is valid, false otherwise
- StateOrProvince string? - If valid; State or province corresponding to the input state name, such as 'CA' or 'California'
- Latitude decimal? - If the postal code is valid, the degrees latitude of the centroid of the state, null otherwise
- Longitude decimal? - If the postal code is valid, the degrees longitude of the centroid of the state, null otherwise
cloudmersive.validate: ValidateUrlRequestFull
Request to determine if a URL is valid
Fields
- URL string? - URL to validate
cloudmersive.validate: ValidateUrlRequestSyntaxOnly
Request to determine if a URL is valid
Fields
- URL string? - URL to validate
cloudmersive.validate: ValidateUrlResponseFull
Result of validating a URL with full validation
Fields
- ValidURL boolean? - True if the URL has valid syntax, a valid domain, a valid endpoint, and passes virus scan checks; false otherwise
- Valid_Syntax boolean? - True if the URL has valid syntax, false otherwise
- Valid_Domain boolean? - True if the domain name is valid and exists, false otherwise
- Valid_Endpoint boolean? - True if the endpoint is up and responsive and passes a virus scan check, false otherwise
- WellFormedURL string? - Well-formed version of the URL
cloudmersive.validate: ValidateUrlResponseSyntaxOnly
Result of validating a URL with syntax only
Fields
- ValidURL boolean? - True if the URL is valid, false otherwise
- WellFormedURL string? - Well-formed version of the URL
- TopLevelDomainName string? - The top-level domain name of the URL, e.g. mydomain.com
cloudmersive.validate: VatLookupRequest
Input to a VAT lookup request
Fields
- VatCode string? - VAT code to lookup; example "CZ25123891"
cloudmersive.validate: VatLookupResponse
Fields
- CountryCode string? - Two-letter country code
- VatNumber string? - VAT number
- IsValid boolean? - True if the VAT code is valid, false otherwise
- BusinessName string? - Name of the business
- BusinessAddress string? - Business address as a single string
- BusinessBuilding string? - For the business address, the name of the building, house or structure if applicable, such as "Cloudmersive Building 2". This will often by null.
- BusinessStreetNumber string? - For the business address, the street number or house number of the address. For example, in the address "1600 Pennsylvania Avenue NW" the street number would be "1600". This value will typically be populated for most addresses.
- BusinessStreet string? - For the business address, the name of the street or road of the address. For example, in the address "1600 Pennsylvania Avenue NW" the street number would be "Pennsylvania Avenue NW".
- BusinessCity string? - For the business address, the city of the address.
- BusinessStateOrProvince string? - For the business address, the state or province of the address.
- BusinessPostalCode string? - For the business address, the postal code or zip code of the address.
- BusinessCountry string? - For the business address, country of the address, if present in the address. If not included in the address it will be null.
cloudmersive.validate: WhoisResponse
Result of a WHOIS operation
Fields
- ValidDomain boolean? - True if the domain is valid, false if it is not
- RegistrantName string? - Name of the domain registrant
- RegistrantOrganization string? - Organization name of the domain registrant
- RegistrantEmail string? - Email address of the domain registrant
- RegistrantStreetNumber string? - Street number of the address of the domain registrant, if available
- RegistrantStreet string? - Street name of the address of the domain registrant, if available
- RegistrantCity string? - City of the domain registrant, if available
- RegistrantStateOrProvince string? - State or Province of the address of the domain registrant, if available
- RegistrantPostalCode string? - Postal code of the address of the domain registrant, if available
- RegistrantCountry string? - Country of the address of the domain registrant, if available
- RegistrantRawAddress string? - Raw address string of the domain registrant, if available
- RegistrantTelephone string? - Telephone number of the address of the domain registrant
- WhoisServer string? - Server used to lookup WHOIS information (may change based on lookup).
- RawTextRecord string? - WHOIS raw text record
- CreatedDt string? - Creation date for the record
cloudmersive.validate: XssProtectionBatchRequest
Input to a batch XSS protection operation
Fields
- RequestItems XssProtectionRequestItem[]? - Multiple items to protect for XSS
cloudmersive.validate: XssProtectionBatchResponse
Result of performing a batch XSS protection operation
Fields
- ResultItems XssProtectionResult[]? - Results from performing a batch XSS protection operation
cloudmersive.validate: XssProtectionRequestItem
Individual item to protect for XSS
Fields
- InputText string? - Individual input text item to protect from XSS
cloudmersive.validate: XssProtectionResult
Result of performing an XSS protection operation
Fields
- Successful boolean? - True if the operation was successful, false otherwise
- ContainedXss boolean? - True if the input contained XSS scripting, false otherwise
- OriginalInput string? - Original input string
- NormalizedResult string? - Normalized string result, with XSS removed
cloudmersive.validate: XxeDetectionBatchRequest
Input to a batch XXE detection operation
Fields
- RequestItems XxeDetectionRequestItem[]? - Multiple items to protect for XXE
cloudmersive.validate: XxeDetectionBatchResponse
Result of performing a batch XXE detection operation
Fields
- ResultItems XxeDetectionResult[]? - Results from performing a batch XSS protection operation
cloudmersive.validate: XxeDetectionRequestItem
Individual item to detect for XXE
Fields
- InputText string? - Individual input text item to protect from XXE
- AllowInternetUrls boolean? - Optional: Set to true to allow Internet-based dependency URLs for DTDs and other XML External Entitites, set to false to block. Default is false.
- KnownSafeUrls string[]? - Optional: Comma separated list of fully-qualified URLs that will automatically be considered safe.
- KnownUnsafeUrls string[]? - Optional: Comma separated list of fully-qualified URLs that will automatically be considered unsafe.
cloudmersive.validate: XxeDetectionResult
Result of performing an XXE threat detection operation
Fields
- Successful boolean? - True if the operation was successful, false otherwise
- ContainedXxe boolean? - True if the input contained XXE threats, false otherwise
Import
import ballerinax/cloudmersive.validate;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
IT Operations/Security & Identity Tools
Cost/Freemium
Contributors
Dependencies