clever.data
Module clever.data
API
Definitions
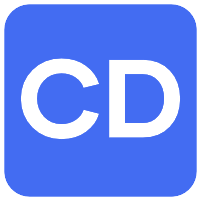
ballerinax/clever.data Ballerina library
Overview
This is a generated connector for Clever Data API v1.2 OpenAPI specification.
Clever Data API provides the capability to retrieve information from Clever.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Clever account
- Obtain tokens - Follow this guide
Quickstart
To use the Clever Data connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/clever.data
module into the Ballerina project.
import ballerinax/clever.data;
Step 2: Create a new connector instance
Create a data:ClientConfig
with the tokens obtained, and initialize the connector with it.
data:ClientConfig configuration = { auth: { token : "<TOKEN>" } }; data:Client cleverClient = check new (configuration);
Step 3: Invoke connector operation
- Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get contacts using the connector.public function main() returns error? { data:StudentContactsResponse response = check cleverClient->getContacts(); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
clever.data: Client
This is a generated connector for Clever Data API v1.2 OpenAPI specification. Clever Data API provides the capability to retrieve information from Clever data store.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Clever account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.clever.com/v1.2" - URL of the target service
getContacts
function getContacts(int? 'limit, string? startingAfter, string? endingBefore) returns StudentContactsResponse|error
Returns a list of student contacts.
Parameters
- 'limit int? (default ()) - Number of contacts
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
Return Type
- StudentContactsResponse|error - StudentContactsResponse or error
getContact
function getContact(string id) returns StudentContactResponse|error
Returns a specific student contact.
Parameters
- id string - Contact ID
Return Type
- StudentContactResponse|error - StudentContactResponse or error
getDistrictForStudentContact
function getDistrictForStudentContact(string id) returns DistrictResponse|error
Returns the district for a student contact.
Parameters
- id string - Contact ID
Return Type
- DistrictResponse|error - DistrictResponse or error
getStudentForContact
function getStudentForContact(string id) returns StudentResponse|error
Returns the student for a student contact.
Parameters
- id string - Contact ID
Return Type
- StudentResponse|error - StudentResponse or error
getDistrictAdmins
function getDistrictAdmins(string? startingAfter, string? endingBefore, string? showLinks) returns DistrictAdminsResponse|error
Returns a list of district admins.
Parameters
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- showLinks string? (default ()) - Show links
Return Type
- DistrictAdminsResponse|error - DistrictAdminsResponse or error
getDistrictAdmin
function getDistrictAdmin(string id) returns DistrictAdminResponse|error
Returns a specific district admin.
Parameters
- id string - District admin ID
Return Type
- DistrictAdminResponse|error - DistrictAdminResponse or error
getDistricts
function getDistricts() returns DistrictsResponse|error
Returns a list of districts.
Return Type
- DistrictsResponse|error - DistrictsResponse or error
getDistrict
function getDistrict(string id, string? include) returns DistrictResponse|error
Returns a specific district.
Return Type
- DistrictResponse|error - DistrictResponse or error
getAdminsForDistrict
function getAdminsForDistrict(string id) returns DistrictAdminsResponse|error
Returns the admins for a district.
Parameters
- id string - District ID
Return Type
- DistrictAdminsResponse|error - DistrictAdminsResponse or error
getSchoolsForDistrict
function getSchoolsForDistrict(string id, int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns SchoolsResponse|error
Returns the schools for a district.
Parameters
- id string - District ID
- 'limit int? (default ()) - Number of schools
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- SchoolsResponse|error - SchoolsResponse or error
getSectionsForDistrict
function getSectionsForDistrict(string id, int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns SectionsResponse|error
Returns the sections for a district.
Parameters
- id string - District ID
- 'limit int? (default ()) - Number of sections
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- SectionsResponse|error - SectionsResponse or error
getDistrictStatus
function getDistrictStatus(string id) returns DistrictStatusResponses|error
Returns the status of the district.
Parameters
- id string - District ID
Return Type
- DistrictStatusResponses|error - DistrictStatusResponses or error
getStudentsForDistrict
function getStudentsForDistrict(string id, int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns StudentsResponse|error
Returns the students for a district.
Parameters
- id string - District ID
- 'limit int? (default ()) - Number of students
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- StudentsResponse|error - StudentsResponse or error
getTeachersForDistrict
function getTeachersForDistrict(string id, int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns TeachersResponse|error
Returns the teachers for a district.
Parameters
- id string - District ID
- 'limit int? (default ()) - Number of teachers
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- TeachersResponse|error - TeachersResponse or error
getSchoolAdmins
function getSchoolAdmins(int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns SchoolAdminsResponse|error
Returns a list of school admins.
Parameters
- 'limit int? (default ()) - Number of admins
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- SchoolAdminsResponse|error - SchoolAdminsResponse or error
getSchoolAdmin
function getSchoolAdmin(string id, string? include) returns SchoolAdminResponse|error
Returns a specific school admin.
Return Type
- SchoolAdminResponse|error - SchoolAdminResponse or error
getSchoolsForSchoolAdmin
function getSchoolsForSchoolAdmin(string id, int? 'limit, string? startingAfter, string? endingBefore) returns SchoolsResponse|error
Returns the schools for a school admin.
Parameters
- id string - School admin ID
- 'limit int? (default ()) - Number of schools
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
Return Type
- SchoolsResponse|error - SchoolsResponse or error
getSchools
function getSchools(int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns SchoolsResponse|error
Returns a list of schools.
Parameters
- 'limit int? (default ()) - Number of schools
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- SchoolsResponse|error - SchoolsResponse or error
getSchool
function getSchool(string id) returns SchoolResponse|error
Returns a specific school.
Parameters
- id string - School ID
Return Type
- SchoolResponse|error - SchoolResponse or error
getDistrictForSchool
function getDistrictForSchool(string id) returns DistrictResponse|error
Returns the district for a school.
Parameters
- id string - School ID
Return Type
- DistrictResponse|error - DistrictResponse or error
getSectionsForSchool
function getSectionsForSchool(string id, int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns SectionsResponse|error
Returns the sections for a school.
Parameters
- id string - School ID
- 'limit int? (default ()) - Number of sections
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- SectionsResponse|error - SectionsResponse or error
getStudentsForSchool
function getStudentsForSchool(string id, int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns StudentsResponse|error
Returns the students for a school.
Parameters
- id string - School ID
- 'limit int? (default ()) - Number of students
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- StudentsResponse|error - StudentsResponse or error
getTeachersForSchool
function getTeachersForSchool(string id, int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns TeachersResponse|error
Returns the teachers for a school.
Parameters
- id string - School ID
- 'limit int? (default ()) - Number of schools
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- TeachersResponse|error - TeachersResponse or error
getSections
function getSections(int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns SectionsResponse|error
Returns a list of sections.
Parameters
- 'limit int? (default ()) - Number of sections
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- SectionsResponse|error - SectionsResponse or error
getSection
function getSection(string id) returns SectionResponse|error
Returns a specific section.
Parameters
- id string - Section ID
Return Type
- SectionResponse|error - SectionResponse or error
getDistrictForSection
function getDistrictForSection(string id) returns DistrictResponse|error
Returns the district for a section.
Parameters
- id string - Section ID
Return Type
- DistrictResponse|error - DistrictResponse or error
getSchoolForSection
function getSchoolForSection(string id) returns SchoolResponse|error
Returns the school for a section
Parameters
- id string - Section ID
Return Type
- SchoolResponse|error - SchoolResponse or error
getStudentsForSection
function getStudentsForSection(string id, int? 'limit, string? startingAfter, string? endingBefore) returns StudentsResponse|error
Returns the students for a section.
Parameters
- id string - Section ID
- 'limit int? (default ()) - Number of students
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
Return Type
- StudentsResponse|error - StudentsResponse or error
getTeacherForSection
function getTeacherForSection(string id) returns TeacherResponse|error
Returns the primary teacher for a section.
Parameters
- id string - Section ID
Return Type
- TeacherResponse|error - TeacherResponse or error
getTeachersForSection
function getTeachersForSection(string id, int? 'limit, string? startingAfter, string? endingBefore) returns TeachersResponse|error
Returns the teachers for a section.
Parameters
- id string - Section ID
- 'limit int? (default ()) - Number of teachers
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
Return Type
- TeachersResponse|error - TeachersResponse or error
getStudents
function getStudents(int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns StudentsResponse|error
Returns a list of students.
Parameters
- 'limit int? (default ()) - Number of students
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- StudentsResponse|error - StudentsResponse or error
getStudent
function getStudent(string id, string? include) returns StudentResponse|error
Returns a specific student.
Return Type
- StudentResponse|error - StudentResponse or error
getContactsForStudent
function getContactsForStudent(string id, int? 'limit) returns StudentContactsForStudentResponse|error
Returns the contacts for a student.
Return Type
- StudentContactsForStudentResponse|error - StudentContactsForStudentResponse or error
getDistrictForStudent
function getDistrictForStudent(string id) returns DistrictResponse|error
Returns the district for a student.
Parameters
- id string - Student ID
Return Type
- DistrictResponse|error - DistrictResponse or error
getSchoolForStudent
function getSchoolForStudent(string id) returns SchoolResponse|error
Returns the primary school for a student.
Parameters
- id string - Student ID
Return Type
- SchoolResponse|error - SchoolResponse or error
getSectionsForStudent
function getSectionsForStudent(string id, int? 'limit, string? startingAfter, string? endingBefore) returns SectionsResponse|error
Returns the sections for a student.
Parameters
- id string - Student ID
- 'limit int? (default ()) - Number of students
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
Return Type
- SectionsResponse|error - SectionsResponse or error
getTeachersForStudent
function getTeachersForStudent(string id, int? 'limit, string? startingAfter, string? endingBefore) returns TeachersResponse|error
Returns the teachers for a student.
Parameters
- id string - Student ID
- 'limit int? (default ()) - Number of teachers
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
Return Type
- TeachersResponse|error - TeachersResponse or error
getTeachers
function getTeachers(int? 'limit, string? startingAfter, string? endingBefore, string? 'where) returns TeachersResponse|error
Returns a list of teachers.
Parameters
- 'limit int? (default ()) - Number of teachers
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
- 'where string? (default ()) - Location
Return Type
- TeachersResponse|error - TeachersResponse or error
getTeacher
function getTeacher(string id, string? include) returns TeacherResponse|error
Returns a specific teacher.
Return Type
- TeacherResponse|error - TeacherResponse or error
getDistrictForTeacher
function getDistrictForTeacher(string id) returns DistrictResponse|error
Returns the district for a teacher.
Parameters
- id string - Teacher ID
Return Type
- DistrictResponse|error - DistrictResponse or error
getGradeLevelsForTeacher
function getGradeLevelsForTeacher(string id) returns GradeLevelsResponse|error
Returns the grade levels for sections a teacher teaches.
Parameters
- id string - Teacher ID
Return Type
- GradeLevelsResponse|error - GradeLevelsResponse or error
getSchoolForTeacher
function getSchoolForTeacher(string id) returns SchoolResponse|error
Retrieves school info for a teacher.
Parameters
- id string - Teacher ID
Return Type
- SchoolResponse|error - SchoolResponse or error
getSectionsForTeacher
function getSectionsForTeacher(string id, int? 'limit, string? startingAfter, string? endingBefore) returns SectionsResponse|error
Returns the sections for a teacher.
Parameters
- id string - Teacher ID
- 'limit int? (default ()) - Number of sections
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
Return Type
- SectionsResponse|error - SectionsResponse or error
getStudentsForTeacher
function getStudentsForTeacher(string id, int? 'limit, string? startingAfter, string? endingBefore) returns StudentsResponse|error
Returns the students for a teacher.
Parameters
- id string - Teacher ID
- 'limit int? (default ()) - Number of students
- startingAfter string? (default ()) - Starting after
- endingBefore string? (default ()) - Ending before
Return Type
- StudentsResponse|error - StudentsResponse or error
Records
clever.data: BadRequest
Fields
- message string? -
clever.data: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
clever.data: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
clever.data: Credentials
Fields
- district_username string? -
clever.data: District
Fields
- id string? -
- mdr_number string? -
- name string? -
clever.data: DistrictAdmin
Fields
- district string? -
- email string? -
- id string? -
- name Name? -
- title string? -
clever.data: DistrictAdminResponse
Fields
- data DistrictAdmin? -
clever.data: DistrictAdminsResponse
Fields
- data DistrictAdmin[]? -
clever.data: DistrictResponse
Fields
- data District? -
clever.data: DistrictsResponse
Fields
- data DistrictResponse[]? -
clever.data: DistrictStatus
Fields
- 'error string? -
- id string? -
- instant_login boolean? -
- last_sync string? -
- launch_date string? -
- pause_end string? -
- pause_start string? -
- sis_type string? -
- state string? -
clever.data: DistrictStatusResponse
Fields
- data DistrictStatus? -
clever.data: DistrictStatusResponses
Fields
- data DistrictStatusResponse[]? -
clever.data: GradeLevelsResponse
Fields
- data string[]? -
clever.data: InternalError
Fields
- message string? -
clever.data: Location
Fields
- address string? -
- city string? -
- lat string? -
- lon string? -
- state string? -
- zip string? -
clever.data: Name
Fields
- first string? -
- last string? -
- middle string? -
clever.data: NotFound
Fields
- message string? -
clever.data: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://clever.com/oauth/tokens") - Refresh URL
clever.data: Principal
Fields
- email string? -
- name string? -
clever.data: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
clever.data: School
Fields
- created string? -
- district string? -
- high_grade string? -
- id string? -
- last_modified string? -
- location Location? -
- low_grade string? -
- mdr_number string? -
- name string? -
- nces_id string? -
- phone string? -
- principal Principal? -
- school_number string? -
- sis_id string? -
- state_id string? -
clever.data: SchoolAdmin
Fields
- credentials Credentials? -
- district string? -
- email string? -
- id string? -
- name Name? -
- schools string[]? -
- staff_id string? -
- title string? -
clever.data: SchoolAdminResponse
Fields
- data SchoolAdmin? -
clever.data: SchoolAdminsResponse
Fields
- data SchoolAdminResponse[]? -
clever.data: SchoolResponse
Fields
- data School? -
clever.data: SchoolsResponse
Fields
- data SchoolResponse[]? -
clever.data: Section
Fields
- course_description string? -
- course_name string? -
- course_number string? -
- created string? -
- district string? -
- grade string? -
- id string? -
- last_modified string? -
- name string? -
- period string? -
- school string? -
- section_number string? -
- sis_id string? -
- students string[]? -
- subject string? -
- teacher string? -
- teachers string[]? -
- term Term? -
clever.data: SectionResponse
Fields
- data Section? -
clever.data: SectionsResponse
Fields
- data SectionResponse[]? -
clever.data: Student
Fields
- created string? -
- credentials Credentials? -
- district string? -
- dob string? -
- ell_status string? -
- email string? -
- gender string? -
- grade string? -
- graduation_year string? -
- hispanic_ethnicity string? -
- id string? -
- last_modified string? -
- location Location? -
- name Name? -
- race string? -
- school string? -
- schools string[]? -
- sis_id string? -
- state_id string? -
- student_number string? -
clever.data: StudentContact
Fields
- district string? -
- email string? -
- id string? -
- name string? -
- phone string? -
- phone_type string? -
- relationship string? -
- sis_id string? -
- student string? -
- 'type string? -
clever.data: StudentContactResponse
Fields
- data StudentContact? -
clever.data: StudentContactsForStudentResponse
Fields
- data StudentContact[]? -
clever.data: StudentContactsResponse
Fields
- data StudentContactResponse[]? -
clever.data: StudentResponse
Fields
- data Student? -
clever.data: StudentsResponse
Fields
- data StudentResponse[]? -
clever.data: Teacher
Fields
- created string? -
- credentials Credentials? -
- district string? -
- email string? -
- id string? -
- last_modified string? -
- name Name? -
- school string? -
- schools string[]? -
- sis_id string? -
- state_id string? -
- teacher_number string? -
- title string? -
clever.data: TeacherResponse
Fields
- data Teacher? -
clever.data: TeachersResponse
Fields
- data TeacherResponse[]? -
clever.data: Term
Fields
- end_date string? -
- name string? -
- start_date string? -
Import
import ballerinax/clever.data;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Education/Elearning
Cost/Paid
Contributors
Dependencies