chaingateway
Module chaingateway
Definitions
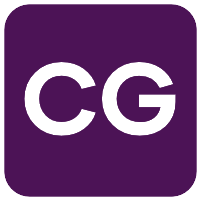
ballerinax/chaingateway Ballerina library
Overview
This is a generated connector for Chaingateway API v1.0 OpenAPI specification.
This is the REST API to build the bridge between Ethereum and the real world. All of the integrations communicates with chaingateway.io through this API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Chaingateway.io Account
- Obtaining tokens Log into Chaingateway.io Account and obtain the necessary API key.
Quickstart
To use the Chaingateway connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/chaingateway module into the Ballerina project.
import ballerinax/chaingateway;
Step 2: Create a new connector instance
You can initialize the client as follows. You can now provide the API key obtained from the Chaingateway.io in the configuration.
chaingateway:ApiKeysConfig config = { authorization: "<API_KEY>" } chaingateway:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
- You can use this function to get gas price of Ethereum.
chaingateway:GetGasPrice gasPrice = check baseClient->getGasPrice("json");
- Use
bal run
command to compile and run the Ballerina program
Clients
chaingateway: Client
This is a generated connector for Chaingateway API v1.0 OpenAPI specification. This is the REST API to build the bridge between Ethereum and the real world. All of the integrations communicates with chaingateway.io through this API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Chaingateway.io Account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://eu.eth.chaingateway.io/v1" - URL of the target service
getToken
function getToken(GetTokenRequest payload) returns GetToken|error
Returns information about a specific ERC20 token like name, symbol, decimal places and total supply.
Parameters
- payload GetTokenRequest - Payload to get a details about ERC20 token like name, symbol, decimal places and total supply
Return Type
getLastBlockNumber
function getLastBlockNumber(string contentType) returns GetLastBlockNumber|error
Returns the block number of the last mined Ethereum block.
Parameters
- contentType string - Result of content type of resuly whether json or xml
Return Type
- GetLastBlockNumber|error - Block number of the last mined Ethereum block
getGasPrice
function getGasPrice(string contentType) returns GetGasPrice|error
Returns the current gas price in GWEI.
Parameters
- contentType string - Result of content type of resuly whether json or xml
Return Type
- GetGasPrice|error - Current gas price in GWEI.
getExchangeRate
function getExchangeRate(GetExchangeRateRequest payload) returns GetExchangeRate|error
Returns the current Ethereum price in Euro or US Dollar.
Parameters
- payload GetExchangeRateRequest - Payload to get current Ethereum price in Euro or US Dollar
Return Type
- GetExchangeRate|error - Current Ethereum price in Euro or US Dollar
getEthereumBalance
function getEthereumBalance(GetEthereumBalanceRequest payload) returns GetEthereumBalance|error
Returns the Ethereum balance of a given address.
Parameters
- payload GetEthereumBalanceRequest - Payload to get Ethereum balance of a given address
Return Type
- GetEthereumBalance|error - Ethereum balance of a given address
getTokenBalance
function getTokenBalance(GetTokenBalanceRequest payload) returns GetTokenBalance|error
Returns the token balance of a given address.
Parameters
- payload GetTokenBalanceRequest - Payload to get token balance of a given address
Return Type
- GetTokenBalance|error - Token balance of a given address
getTransactions
function getTransactions(GetTransactionsRequest payload) returns GetTransactions|error
Returns information like confirmations, token contract address, amount, gas price and more of a given transaction.
Parameters
- payload GetTransactionsRequest - Payload to get a details about given transaction
Return Type
- GetTransactions|error - Information like confirmations, token contract address, amount, gas price and more of a given transaction
getBlock
function getBlock(GetBlockRequest payload) returns GetBlock|error
Returns information of an Ethereum block with or without transactions.
Parameters
- payload GetBlockRequest - Payload to get a Ethereum block
newAddress
function newAddress(NewAddressRequest payload) returns NewAddress|error
Generates a new Ethereum addresses you can use to send or receive funds. Do not lose the password! We can't restore access to an address if you lose it.
Parameters
- payload NewAddressRequest - Payload to create a new Ethereum address
Return Type
- NewAddress|error - Generated new Ethereum addresses you can use to send or receive funds
deleteAddress
function deleteAddress(DeleteAddressRequest payload) returns DeleteAddress|error
Deletes an existing Ethereum address. Be careful when using this function.
Parameters
- payload DeleteAddressRequest - Payload to delete an existing Ethereum address
Return Type
- DeleteAddress|error - Detail of Ethereum addresses deleted
listAddresses
function listAddresses(string contentType) returns ListAddresses|error
Returns all Ethereum addresses created with an account.
Parameters
- contentType string - Result of content type of resuly whether json or xml
Return Type
- ListAddresses|error - Ethereum addresses created with an account
importAddress
function importAddress(ImportAddressRequest payload) returns ImportAddress|error
Imports an existing keystore into system and makes it possible to send transactions from it.
Parameters
- payload ImportAddressRequest - Payload to import a keystore into system
Return Type
- ImportAddress|error - List of Ethereum addresses created with an account
exportAddress
function exportAddress(ExportAddressRequest payload) returns ExportAddress|error
Exports an existing keystore file so you can use it in other wallets.
Parameters
- payload ExportAddressRequest - Payload to export an existing keystore file
Return Type
- ExportAddress|error - List of Ethereum addresses created with an account
subscribeAddress
function subscribeAddress(SubscribeAddressRequest payload) returns SubscribeAddress|error
Creates a new subscription/IPN for the given address (and contractaddress). You will receive a notification to the given url every time a deposit is received. Unsubscribe the address before sending tokens/ETH from it or you won't get reliable notifications anymore.
Parameters
- payload SubscribeAddressRequest - Payload to create a new subscription/IPN
Return Type
- SubscribeAddress|error - Detail about Ethereum address
unsubscribeAddress
function unsubscribeAddress(UnsubscribeAddressRequest payload) returns UnsubscribeAddress|error
Deletes an existing subscription/IPN for the given address (and contractaddress).
Parameters
- payload UnsubscribeAddressRequest - Payload to delete an existing subscription/IPN
Return Type
- UnsubscribeAddress|error - Detail about Ethereum address
listSubscribedAddresses
function listSubscribedAddresses(string contentType) returns ListSubscribedAddresses|error
Returns all subscriptions/IPNs created with an account.
Parameters
- contentType string - Result of content type of resuly whether json or xml
Return Type
- ListSubscribedAddresses|error - Details about all Ethereum addresses
listFailedIPNs
function listFailedIPNs(string contentType) returns ListFailedIPNs|error
Returns all IPNs that couldn't be sent. Our system tries to send IPNs 10 times until they end up failed.
Parameters
- contentType string - Result of content type of resuly whether json or xml
Return Type
- ListFailedIPNs|error - List of IPNs that couldn't be sent
resendFailedIPN
function resendFailedIPN(ResendFailedIPNRequest payload) returns ResendFailedIPN|error
Tries to resend a failed IPN.
Parameters
- payload ResendFailedIPNRequest - Payload to resend a failed IPN
Return Type
- ResendFailedIPN|error - Status of subscriptions/IPNs
sendEthereum
function sendEthereum(SendEthereumRequest payload) returns SendEthereum|error
Sends Ethereum from an address controlled by the account to a specified receiver address.
Parameters
- payload SendEthereumRequest - Payload to send Ethereum transaction from an address
Return Type
- SendEthereum|error - Detail about Ethereum transaction from an address
clearAddress
function clearAddress(ClearAddressRequest payload) returns ClearAddress|error
Sends all available Ethereum funds of an address to a specified receiver address.
Parameters
- payload ClearAddressRequest - Payload to send all available Ethereum funds from an address
Return Type
- ClearAddress|error - Detail about all available Ethereum funds from an address
sendToken
function sendToken(SendTokenRequest payload) returns SendToken|error
Sends ERC20 tokens from an address controlled by the account to a specified receiver address. The token contract address is needed to specify the token. The use of the identifier parameter is recommend and awaits an unique string. Whenever a transaction is beeing sent, the identifier is checked and the transaction gets dropped if there is one with that identifier already.
Parameters
- payload SendTokenRequest - Payload to sends ERC20 tokens from an address
Records
chaingateway: Address
Fields
- ethereumaddress string -
chaingateway: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- authorization string - All requests on the chaingateway.io API needs to include an API key. The API key can be provided as part of the query string or as a request header. The name of the API key needs to be
Authorization
chaingateway: Cipherparams
Fields
- iv string -
chaingateway: ClearAddress
Fields
- ok boolean -
- txid string -
- ethereumaddress string -
- newaddress string -
- amount decimal -
- gas decimal -
- total string -
chaingateway: ClearAddressRequest
Fields
- ethereumaddress string -
- newaddress string -
- password string -
chaingateway: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
chaingateway: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
chaingateway: Content
Fields
- 'version int -
- id string -
- address string -
- crypto Crypto -
chaingateway: Crypto
Fields
- ciphertext string -
- cipherparams Cipherparams -
- cipher string -
- kdf string -
- kdfparams Kdfparams -
- mac string -
chaingateway: DeleteAddress
Fields
- ok boolean -
- ethereumaddress string -
- deleted boolean -
chaingateway: DeleteAddressRequest
Fields
- ethereumaddress string -
- password string -
chaingateway: ExportAddress
Fields
- ok boolean -
- filename string -
- content string -
chaingateway: ExportAddressRequest
Fields
- ethaddress string -
- password string -
chaingateway: FailedIpn
Fields
- id string -
- timestamp string -
- ethereumaddress string -
- contractaddress string -
- amount string -
- url string -
- action string -
chaingateway: GetBlock
Fields
- ok boolean -
- hash string -
- block_number string -
- parent_hash string -
- miner string -
- difficulty string -
- size_in_bytes string -
- gas_limit string -
- gas_used string -
- time_stamp string -
- transactions_count string -
chaingateway: GetBlockRequest
Fields
- block string -
chaingateway: GetEthereumBalance
Fields
- ok boolean -
- ethereumaddress string -
- balance decimal -
chaingateway: GetEthereumBalanceRequest
Fields
- ethereumaddress string -
chaingateway: GetExchangeRate
Fields
- ok boolean -
- currency string -
- rate decimal -
chaingateway: GetExchangeRateRequest
Fields
- currency string -
chaingateway: GetGasPrice
Fields
- ok boolean -
- gasprice int -
chaingateway: GetLastBlockNumber
Fields
- ok boolean -
- blocknumber int -
chaingateway: GetToken
Fields
- ok boolean -
- contractaddress string -
- name string -
- symbol string -
- decimals int -
- supply int -
chaingateway: GetTokenBalance
Fields
- ok boolean -
- contractaddress string -
- ethereumaddress string -
- balance int -
chaingateway: GetTokenBalanceRequest
Fields
- contractaddress string -
- ethereumaddress string -
chaingateway: GetTokenRequest
Fields
- contractaddress string -
chaingateway: GetTransactions
Fields
- ok boolean -
- transactions Transaction[] -
chaingateway: GetTransactionsRequest
Fields
- txid string -
chaingateway: ImportAddress
Fields
- ok boolean -
- ethaddress string -
- filename string -
chaingateway: ImportAddressRequest
Fields
- filename string -
- content Content -
- password string -
chaingateway: Ipn
Fields
- ethereumaddress string -
- contractaddress string -
- url string -
chaingateway: Kdfparams
Fields
- dklen int -
- salt string -
- n int -
- r int -
- p int -
chaingateway: ListAddresses
Fields
- ok boolean -
- addresses Address[] -
chaingateway: ListFailedIPNs
Fields
- ok boolean -
- failed_ipns FailedIpn[] -
chaingateway: ListSubscribedAddresses
Fields
- ok boolean -
- ipns Ipn[] -
chaingateway: NewAddress
Fields
- ok boolean -
- ethereumaddress string -
- password string -
chaingateway: NewAddressRequest
Fields
- password string -
chaingateway: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
chaingateway: ResendFailedIPN
Fields
- ok boolean -
- id int -
chaingateway: ResendFailedIPNRequest
Fields
- id int -
chaingateway: SendEthereum
Fields
- ok boolean -
- txid string -
- 'from string -
- to string -
- amount string -
chaingateway: SendEthereumRequest
Fields
- 'from string -
- to string -
- password string -
- amount decimal -
chaingateway: SendToken
Fields
- ok boolean -
- identifier string -
- txid string -
- contractaddress string -
- 'from string -
- to string -
- amount int -
chaingateway: SendTokenRequest
Fields
- contractaddress string -
- 'from string -
- to string -
- password string -
- amount int -
- identifier string -
chaingateway: SubscribeAddress
Fields
- ok boolean -
- ethereumaddress string -
- contractaddress string -
- url string -
chaingateway: SubscribeAddressRequest
Fields
- ethereumaddress string -
- contractaddress string -
- url string -
chaingateway: Transaction
Fields
- txid string -
- block_number string -
- contract_address string -
- 'type string -
- token_name string -
- token_symbol string -
- token_decimals string -
- token_supply string -
- gas string -
- gas_price string -
- 'from string -
- to string -
- amount string -
chaingateway: UnsubscribeAddress
Fields
- ok boolean -
- ethereumaddress string -
- contractaddress string -
- url string -
- deleted boolean -
chaingateway: UnsubscribeAddressRequest
Fields
- ethereumaddress string -
- contractaddress string -
- url string -
Import
import ballerinax/chaingateway;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Finance/Cryptocurrency
Cost/Freemium
Contributors
Dependencies