capsulecrm
Module capsulecrm
API
Definitions
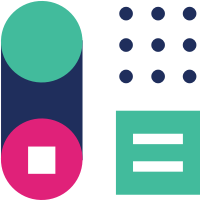
ballerinax/capsulecrm Ballerina library
Overview
This is a generated connector for Capsule CRM API v2 OpenAPI specification. Capsule CRM keeps track of the people and companies you do business with, opportunities in the pipeline and what needs to be done.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Capsule CRM account
- Obtain tokens by following this guide
Quickstart
To use the Capsule CRM connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/capsulecrm
module into the Ballerina project.
import ballerinax/capsulecrm;
Step 2: Create a new connector instance
Create a capsulecrm:ClientConfig
with the Bearer_Token
obtained, and initialize the connector with it.
capsulecrm:ClientConfig clientConfig = { auth: { token: <Bearer_Token> } }; capsulecrm:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve the collection of Cases on the Capsule account using the connector.
Retrieves the collection of Cases on the Capsule account
public function main() returns error? { capsulecrm:Cases response = check baseClient->listCases(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
capsulecrm: Client
This is a generated connector for Capsule CRM API v2 OpenAPI specification. Capsule CRM keeps track of the people and companies you do business with, opportunities in the pipeline and what needs to be done.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Capsule CRM account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.capsulecrm.com" - URL of the target service
listCases
Retrieves the collection of Cases on the Capsule account.
Parameters
- since string? (default ()) - If set then includes only entities that have been changed after this date. Must in be ISO8601 format.
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags ( tags), fields (custom fields and fields in DataTags), party (assigned party), opportunity (the associated opportunity), and missingImportantFields (indicates if a case has any Important custom fields that are missing a value).
createCase
function createCase(CreateCaseRequest payload, string? embed) returns CreatedCase|error
Creates a new case.
Parameters
- payload CreateCaseRequest - An object with a single property kase which must be a Case object. Note that one or more tracks can be applied to the case by including the relevant track ids in a tracks array. This is a convenient shortcut to apply tracks to new cases only, and tracks is not a field of Case objects. Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags ( tags), fields (custom fields and fields in DataTags), party (assigned party), opportunity (the associated opportunity), and missingImportantFields (indicates if a case has any Important custom fields that are missing a value).
Return Type
- CreatedCase|error - Created case stored in Capsule
searchCases
Search for cases on the Capsule account. This will return the same results as the global search inside Capsule.
Parameters
- q string? (default ()) - The value to search for e.g. a name, a postcode or a phone number.
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags ( tags), fields (custom fields and fields in DataTags), party (assigned party), opportunity (the associated opportunity), and missingImportantFields (indicates if a case has any Important custom fields that are missing a value).
showCase
function showCase(string caseId, string? embed) returns CaseObject|error
Show a specific case.
Parameters
- caseId string - The case ID
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags ( tags), fields (custom fields and fields in DataTags), party (assigned party), opportunity (the associated opportunity), and missingImportantFields (indicates if a case has any Important custom fields that are missing a value).
Return Type
- CaseObject|error - A Case object.
listCasesByParty
function listCasesByParty(string partyId, int? page, int? perPage, string? embed) returns Cases|error
Retrieves the collection of all cases associated with the given party.
Parameters
- partyId string - The party ID
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags ( tags), fields (custom fields and fields in DataTags), party (assigned party), opportunity (the associated opportunity), and missingImportantFields (indicates if a case has any Important custom fields that are missing a value).
listOpportunities
function listOpportunities(string? since, int? page, int? perPage, string? embed) returns Opportunities|error
Retrieves the collection of Opportunities on the Capsule account.
Parameters
- since string? (default ()) - If set then includes only entities that have been changed after this date. Must in be ISO8601 format.
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags (tags), fields (custom fields and fields in DataTags), party (assigned party), milestone (the opportunity milestone), and missingImportantFields (indicates if an opportunity has any Important custom fields that are missing a value).
Return Type
- Opportunities|error - An object with a single property opportunity which is an array of Opportunity objects.
createOpportunity
function createOpportunity(CreateOpportunityRequest payload, string? embed) returns CreatedOpportunity|error
Creates a new opportunity.
Parameters
- payload CreateOpportunityRequest - An object with a single property opportunity which must be an Opportunity object. One or more tracks can be applied to the opportunity by including the relevant track ids in a tracks array. This is a convenient shortcut to apply tracks to new opportunities only, and tracks is not a field of Opportunity objects. Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags ( tags), fields (custom fields and fields in DataTags), party (assigned party), opportunity (the associated opportunity), and missingImportantFields (indicates if a case has any Important custom fields that are missing a value).
Return Type
- CreatedOpportunity|error - Created opportunity stored in Capsule
searchOpportunities
function searchOpportunities(string? q, int? page, int? perPage, string? embed) returns Opportunities|error
Search for opportunities on the Capsule account. This will return the same results as the global search inside Capsule. If you're looking for a more structured search endpoint you can use Filters to search by a specific field or tag.
Parameters
- q string? (default ()) - The value to search for e.g. a name, a postcode or a phone number.
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags ( tags), fields (custom fields and fields in DataTags), party (assigned party), opportunity (the associated opportunity), and missingImportantFields (indicates if a case has any Important custom fields that are missing a value).
Return Type
- Opportunities|error - An object with a single property opportunities which is an array of Opportunity objects.
showOpportunity
function showOpportunity(string opportunityId, string? embed) returns OpportunityObject|error
Returns a specific opportunity.
Parameters
- opportunityId string - The opportunity ID
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags (tags), fields (custom fields and fields in DataTags), party (assigned party), milestone (the opportunity milestone), and missingImportantFields (indicates if an opportunity has any Important custom fields that are missing a value).
Return Type
- OpportunityObject|error - An Opportunity object.
updateOpportunity
function updateOpportunity(string opportunityId, UpdateOpportunityRequest payload, string? embed) returns Response|error
Updates an existing opportunity.
Parameters
- opportunityId string - The opportunity ID
- payload UpdateOpportunityRequest - An object with a single property opportunity which must be an Opportunity object. Fields that are not included in the request will remain unchanged. An owner and/or team are required on an opportunity.
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags (tags), fields (custom fields and fields in DataTags), party (assigned party), milestone (the opportunity milestone), and missingImportantFields (indicates if an opportunity has any Important custom fields that are missing a value).
deleteOpportunity
Fully delete a specific opportunity from Capsule.
Parameters
- opportunityId string - The opportunity ID
listOpportunitiesByParty
function listOpportunitiesByParty(string partyId, int? page, int? perPage, string? embed) returns Opportunities|error
Retrieves the collection of all opportunities associated with the given party.
Parameters
- partyId string - The party ID
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags ( tags), fields (custom fields and fields in DataTags), party (assigned party), opportunity (the associated opportunity), and missingImportantFields (indicates if a case has any Important custom fields that are missing a value).
Return Type
- Opportunities|error - An object with a single property opportunities which is an array of Opportunity objects.
listParties
Retrieves the collection of Parties on the Capsule account.
Parameters
- since string? (default ()) - If set then includes only entities that have been changed after this date. Must in be ISO8601 format.
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags (tags), fields (custom fields and fields in DataTags), organisation (extended organisation details for people), and missingImportantFields (indicates if a party has any Important custom fields that are missing a value).
Return Type
createParty
function createParty(CreatePartyRequest payload, string? embed) returns CreatedParty|error
Creates a new party.
Parameters
- payload CreatePartyRequest - An object with a single property party which must be a Party object.
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags (tags), fields (custom fields and fields in DataTags), organisation (extended organisation details for people), and missingImportantFields (indicates if a party has any Important custom fields that are missing a value).
Return Type
- CreatedParty|error - Created party stored in Capsule
searchParties
Search for parties on the Capsule account. This will return the same results as the global search inside Capsule.
Parameters
- q string? (default ()) - The value to search for e.g. a name, a postcode or a phone number.
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags (tags), fields (custom fields and fields in DataTags), organisation (extended organisation details for people), and missingImportantFields (indicates if a party has any Important custom fields that are missing a value).
Return Type
showParty
function showParty(string partyId, string? embed) returns PartyObject|error
Show a specific person or organisation.
Parameters
- partyId string - The party ID
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags (tags), fields (custom fields and fields in DataTags), organisation (extended organisation details for people), and missingImportantFields (indicates if a party has any Important custom fields that are missing a value).
Return Type
- PartyObject|error - An object with a single property party which will contain a Party object.
updateParty
function updateParty(string partyId, UpdatePartyRequest payload, string? embed) returns Response|error
Update an existing party.
Parameters
- partyId string - The party ID
- payload UpdatePartyRequest - object with a single property party which must be a Party object. Fields that are not included in the request will remain unchanged.
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are tags (tags), fields (custom fields and fields in DataTags), organisation (extended organisation details for people), and missingImportantFields (indicates if a party has any Important custom fields that are missing a value).
deleteParty
Fully delete the specific party from Capsule.
Parameters
- partyId string - The party ID
listTasks
Retrieves the collection of Tasks on the Capsule account. By default the body will contain only the open tasks. If you want to retrieve pending and/or completed tasks use the status query parameter.
Parameters
- page int? (default ()) - The page of results to return. Default: 1
- perPage int? (default ()) - The number of entities to return per page. Value must be between 1 and 100. Default: 50
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are party (the party this task is assigned to), opportunity (opportunity this task is on), kase (the case this task is on), owner (the owner of the task) and nextTask (the next task).
- status string? (default ()) - Filter the response based on the status of the task. Must be a comma separated list of strings. Accepted values are open, completed and pending.
createTask
function createTask(CreateTaskRequest payload, string? embed) returns CreatedTask|error
Creates a new task.
Parameters
- payload CreateTaskRequest - An object with a single property task which must be a Task object.
- embed string? (default ()) - Can be used to request additional data that aren’t included in the response by default. If provided, should be a comma separated list of strings. Supported values for this endpoint are party (the party this task is assigned to), opportunity (opportunity this task is on), kase (the case this task is on), owner (the owner of the task) and nextTask (the next task).
Return Type
- CreatedTask|error - Created task stored in Capsule
Records
capsulecrm: Address
Represents a physical address of a contact that is stored in Capsule.
Fields
- id int? - The unique id of this address.
- 'type string? - The address type. Accepted values are Home, Postal, Office, Billing, Shipping
- street string? - The multi-line street address.
- city string? - The city of the address.
- state string? - The state or province of the address.
- country string? - The country of the address. This field should be a valid country name. You can obtain a list of valid countries using the api/v2/countries endpoint.
- zip string? - The zip/postal code.
capsulecrm: Case
Represents a case file that is stored in Capsule.
Fields
- id int? - The unique id of this case.
- party NestedParty - Represents a reference to a party. By default, contains only the id, name and pictureURL. If the field is included in the embed parameter will instead be a full Party object. If the party is restricted for the user calling the API endpoint, the full Party object is not included.
- name string - The name of this case.
- description string? - The description of this case.
- owner NestedUser? - Represents a reference to a user. By default, contains only the id, name and username. If the field is included in the embed parameter will instead be a full User object.
- team NestedTeam? - Represents a reference to a team.
- status string? - The status of the case. Accepted values are OPEN, CLOSED.
- opportunity NestedOpportunity? - Represents a reference to an opportunity. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Opportunity object. If the opportunity is restricted for the user calling the API endpoint the full Opportunity object is not included.
- createdAt string? - The ISO date/time when this case was created.
- updatedAt string? - The ISO date/time when this case was last updated.
- closedOn string? - The ISO date when this case was closed.
- lastContactedAt string? - The ISO date/time when this case was last contacted. This field is automatically set by Capsule based on the attached history entries.
- tags NestedTag[]? - An array of tags that are added to this case. This field is included in responses only if the embed parameter contains tags.
- fields FieldValue[]? - An array of custom fields that are defined for this case. This field is included in responses only if the embed parameter contains fields.
- missingImportantFields boolean? - Indicates if this case has any Important custom fields that are missing a value. This field is included in responses only if the embed parameter contains missingImportantFields.
capsulecrm: CaseObject
A Case object.
Fields
- kase Case? - Represents a case file that is stored in Capsule.
capsulecrm: CaseRequest
An object with a single property kase which must be a Case object. Note that one or more tracks can be applied to the case by including the relevant track ids in a tracks array. This is a convenient shortcut to apply tracks to new cases only, and tracks is not a field of Case objects. Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
Fields
- id int? - The unique id of this case.
- party NestedParty - Represents a reference to a party. By default, contains only the id, name and pictureURL. If the field is included in the embed parameter will instead be a full Party object. If the party is restricted for the user calling the API endpoint, the full Party object is not included.
- name string - The name of this case.
- description string? - The description of this case.
- owner NestedUser? - Represents a reference to a user. By default, contains only the id, name and username. If the field is included in the embed parameter will instead be a full User object.
- team NestedTeam? - Represents a reference to a team.
- status string? - The status of the case. Accepted values are OPEN, CLOSED.
- opportunity NestedOpportunity? - Represents a reference to an opportunity. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Opportunity object. If the opportunity is restricted for the user calling the API endpoint the full Opportunity object is not included.
- createdAt string? - The ISO date/time when this case was created.
- updatedAt string? - The ISO date/time when this case was last updated.
- closedOn string? - The ISO date when this case was closed.
- lastContactedAt string? - The ISO date/time when this case was last contacted. This field is automatically set by Capsule based on the attached history entries.
- tags NestedTag[]? - An array of tags that are added to this case. This field is included in responses only if the embed parameter contains tags.
- fields FieldValue[]? - An array of custom fields that are defined for this case. This field is included in responses only if the embed parameter contains fields.
- missingImportantFields boolean? - Indicates if this case has any Important custom fields that are missing a value. This field is included in responses only if the embed parameter contains missingImportantFields.
- tracks TracksDefinitionObject[]? - One or more tracks can be applied to the case by including the relevant track ids in a tracks array. This is a convenient shortcut to apply tracks to new cases only, and tracks is not a field of Case objects. Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
capsulecrm: Cases
An object with a single property kases which is an array of Case objects.
Fields
- kases Case[]? - An array of Case objects.
capsulecrm: Category
Represents a task category.
Fields
- id int? - The unique id of this category.
- name string - The name of the category.
- colour string? - The hex colour code of the category. Must be a '#' followed by 6 hexadecimal digits.
capsulecrm: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
capsulecrm: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
capsulecrm: CreateCaseRequest
An object with a single property kase which must be a Case object. Note that one or more tracks can be applied to the case by including the relevant track ids in a tracks array. This is a convenient shortcut to apply tracks to new cases only, and tracks is not a field of Case objects. Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
Fields
- kase CaseRequest? - An object with a single property kase which must be a Case object. Note that one or more tracks can be applied to the case by including the relevant track ids in a tracks array. This is a convenient shortcut to apply tracks to new cases only, and tracks is not a field of Case objects. Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
capsulecrm: CreatedCase
Created case stored in Capsule
Fields
- kase Case? - Represents a case file that is stored in Capsule.
capsulecrm: CreatedOpportunity
Created opportunity stored in Capsule
Fields
- opportunity Opportunity? - Represents an opportunity that is stored in Capsule.
capsulecrm: CreatedParty
Created party stored in Capsule
Fields
- party Party? - Represents a contact that is stored in Capsule. This can be either a person or an organisation.
capsulecrm: CreatedTask
Created task stored in Capsule
Fields
- task Task? - Represents a task that is stored in Capsule. These are generally used as a simple way to create a reminder of something the user needs to do. Tasks can be categorized for such things as a meeting, phone call or submission deadline using task categories and can be linked to a party, opportunity or case.
capsulecrm: CreateOpportunityRequest
An object with a single property opportunity which must be an Opportunity object. One or more tracks can be applied to the opportunity by including the relevant track ids in a tracks array. This is a convenient shortcut to apply tracks to new opportunities only, and tracks is not a field of Opportunity objects. Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
Fields
- opportunity OpportunityRequest? - Represents an opportunity that is stored in Capsule.
capsulecrm: CreatePartyRequest
An object with a single property party which must be a Party object.
Fields
- party Party? - Represents a contact that is stored in Capsule. This can be either a person or an organisation.
capsulecrm: CreateTaskRequest
An object with a single property task which must be a Task object.
Fields
- task Task? - Represents a task that is stored in Capsule. These are generally used as a simple way to create a reminder of something the user needs to do. Tasks can be categorized for such things as a meeting, phone call or submission deadline using task categories and can be linked to a party, opportunity or case.
capsulecrm: EmailAddress
Represents an email address of a party.
Fields
- id int? - The unique id of this email address.
- 'type string? - The type of the email address. Accepted values are Home, Work
- address string - The actual email address.
capsulecrm: FieldValue
Represents a value of a custom field. Depending on the custom field definition this can be assignable to parties, opportunities or cases.
Fields
- id int? - The id of this value. Note that field values assignable to different entities (parties, cases or opportunities) can have the same id.
- value string|boolean|decimal - The value of this field. For text custom fields value is a string. For date custom fields value is a string in the format YYYY-MM-DD. For list custom fields value is a string and must match one of the options specified in the custom field definition. For boolean custom fields value is a boolean. For number custom fields value can be passed as either a string or a number, but will always be returned as a string by the API. For link custom fields value is a string URL and can include one of {id} - id, {name} - name, {email} - first email address, {phone} - first phone number, {phone[Mobile]} first mobile number, {custom[name of custom field]} - value of a custom field.
- definition NestedFieldDefinition - Represents a reference to a definition of a custom field.
capsulecrm: NestedCase
Represents a reference to a case. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Case object. If the case is restricted for the user calling the API endpoint the full Case object is not included.
Fields
- id int? - The unique id of this case.
- name string? - The name of this case. This will contain Private when the user calling the API endpoint doesn't have access to the the case record.
- isRestricted boolean? - Defines whether this case is restricted for the user calling the API endpoint.
capsulecrm: NestedFieldDefinition
Represents a reference to a definition of a custom field.
Fields
- id int? - The id of this custom field definition. Note that field definitions assignable to different entities (parties, opportunities or cases) can have the same id.
- name string? - The name of this custom field.
capsulecrm: NestedLostReason
Represents a reference to a lost reason.
Fields
- id int? - The unique id of the lost reason.
- name string? - The name of the lost reason.
capsulecrm: NestedMilestone
Represents a reference to a milestone. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Milestone object.
Fields
- id int? - The unique id of the milestone.
- name string? - The name of the milestone.
capsulecrm: NestedOpportunity
Represents a reference to an opportunity. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Opportunity object. If the opportunity is restricted for the user calling the API endpoint the full Opportunity object is not included.
Fields
- id int? - The unique id of the opportunity.
- name string? - The name of the opportunity. This will contain Private when the user calling the API endpoint doesn't have access to the opportunity record.
- isRestricted boolean? - Defines whether this opportunity is restricted for the user calling the API endpoint.
capsulecrm: NestedParty
Represents a reference to a party. By default, contains only the id, name and pictureURL. If the field is included in the embed parameter will instead be a full Party object. If the party is restricted for the user calling the API endpoint, the full Party object is not included.
Fields
- id int? - The unique id of the party.
- firstName string? - The first name of the person. This field is present only when type is person.
- lastName string? - The last name of the person. This field is present only when type is person.
- name string? - The name of the organisation. This field is present only when type is organisation. This will contain Private when the user calling the API endpoint doesn't have access to the party record.
- 'type string? - Represents if this party is a person or organisation. Accepted values are person, organisation
- pictureURL string? - A URL that represents the location of the profile picture for this party.
- isRestricted boolean? - Indicates whether this party is restricted for the user calling the API endpoint.
capsulecrm: NestedTag
Represents an attached tag. Depending on the tag type this can be assignable to parties, opportunities or cases.
Fields
- id int? - The id of this tag. Note that tags assignable to different entities (parties, cases or opportunities) can have the same id.
- name string - The name of this tag.
- description string? - A longer description of this tag.
capsulecrm: NestedTask
Represents a reference to a task. By default, contains only the id and description. If the field name is included in the embed parameter will instead be a full Task object.
Fields
- id int? - The unique id of this task.
- description string? - A short description of the task.
capsulecrm: NestedTeam
Represents a reference to a team.
Fields
- id int? - The unique id of this team.
- name string? - The name of the team.
capsulecrm: NestedUser
Represents a reference to a user. By default, contains only the id, name and username. If the field is included in the embed parameter will instead be a full User object.
Fields
- id int? - The unique id of this user.
- username string? - The username of the user.
- name string? - The name of the user.
capsulecrm: Opportunities
An object with a single property opportunities which is an array of Opportunity objects.
Fields
- opportunities Opportunity[]? - An array of Opportunity objects.
capsulecrm: Opportunity
Represents an opportunity that is stored in Capsule.
Fields
- id int? - The unique id of this opportunity.
- createdAt string? - The ISO date/time this opportunity was created.
- updatedAt string? - The ISO DateTime when this opportunity was last updated.
- party NestedParty - Represents a reference to a party. By default, contains only the id, name and pictureURL. If the field is included in the embed parameter will instead be a full Party object. If the party is restricted for the user calling the API endpoint, the full Party object is not included.
- lostReason NestedLostReason? - Represents a reference to a lost reason.
- name string - The name of this opportunity.
- description string? - The description of this opportunity.
- owner NestedUser? - Represents a reference to a user. By default, contains only the id, name and username. If the field is included in the embed parameter will instead be a full User object.
- team NestedTeam? - Represents a reference to a team.
- milestone NestedMilestone - Represents a reference to a milestone. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Milestone object.
- value OpportunityValue? - Represents the value of an opportunity.
- expectedCloseOn string? - The expected close date of this opportunity.
- probability int? - The probability of winning this opportunity.
- durationBasis string? - The time unit used by the duration field. Accepted values are FIXED, HOUR, DAY, WEEK, MONTH, QUARTER, YEAR.
- duration int? - The duration of this opportunity. Must be null if durationBasis is set to FIXED.
- closedOn string? - The date this opportunity was closed.
- tags NestedTag[]? - An array of tags that are added to this opportunity. This field is included in responses only if the embed parameter contains tags.
- fields FieldValue[]? - An array of custom fields that are defined for this opportunity. This field is included in responses only if the embed parameter contains fields.
- lastContactedAt string? - The ISO DateTime when this opportunity was last contacted.
- lastStageChangedAt string? - The ISO DateTime when this opportunity last had its milestone changes.
- lastOpenMilestone NestedMilestone? - Represents a reference to a milestone. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Milestone object.
- missingImportantFields boolean? - Indicates if this opportunity has any Important custom fields that are missing a value. This field is included in responses only if the embed parameter contains missingImportantFields.
capsulecrm: OpportunityObject
An Opportunity object.
Fields
- opportunity Opportunity? - Represents an opportunity that is stored in Capsule.
capsulecrm: OpportunityRequest
Represents an opportunity that is stored in Capsule.
Fields
- id int? - The unique id of this opportunity.
- createdAt string? - The ISO date/time this opportunity was created.
- updatedAt string? - The ISO DateTime when this opportunity was last updated.
- party NestedParty - Represents a reference to a party. By default, contains only the id, name and pictureURL. If the field is included in the embed parameter will instead be a full Party object. If the party is restricted for the user calling the API endpoint, the full Party object is not included.
- lostReason NestedLostReason? - Represents a reference to a lost reason.
- name string - The name of this opportunity.
- description string? - The description of this opportunity.
- owner NestedUser? - Represents a reference to a user. By default, contains only the id, name and username. If the field is included in the embed parameter will instead be a full User object.
- team NestedTeam? - Represents a reference to a team.
- milestone NestedMilestone - Represents a reference to a milestone. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Milestone object.
- value OpportunityValue? - Represents the value of an opportunity.
- expectedCloseOn string? - The expected close date of this opportunity.
- probability int? - The probability of winning this opportunity.
- durationBasis string? - The time unit used by the duration field. Accepted values are FIXED, HOUR, DAY, WEEK, MONTH, QUARTER, YEAR.
- duration int? - The duration of this opportunity. Must be null if durationBasis is set to FIXED.
- closedOn string? - The date this opportunity was closed.
- tags NestedTag[]? - An array of tags that are added to this opportunity. This field is included in responses only if the embed parameter contains tags.
- fields FieldValue[]? - An array of custom fields that are defined for this opportunity. This field is included in responses only if the embed parameter contains fields.
- lastContactedAt string? - The ISO DateTime when this opportunity was last contacted.
- lastStageChangedAt string? - The ISO DateTime when this opportunity last had its milestone changes.
- lastOpenMilestone NestedMilestone? - Represents a reference to a milestone. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Milestone object.
- missingImportantFields boolean? - Indicates if this opportunity has any Important custom fields that are missing a value. This field is included in responses only if the embed parameter contains missingImportantFields.
- tracks TracksDefinitionObject[]? - One or more tracks can be applied to the case by including the relevant track ids in a tracks array. This is a convenient shortcut to apply tracks to new cases only, and tracks is not a field of Case objects. Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
capsulecrm: OpportunityValue
Represents the value of an opportunity.
Fields
- amount decimal - The amount the opportunity is worth.
- currency string? - The currency type of the opportunity.
capsulecrm: Parties
An object with a single property parties which is an array of Party objects.
Fields
- parties Party[]? - An array of Party objects.
capsulecrm: Party
Represents a contact that is stored in Capsule. This can be either a person or an organisation.
Fields
- id int? - The unique id of this party.
- 'type string - Represents if this party is a person or an organisation. Accepted values are person, organisation.
- firstName string? - The first name of the person. This field is present only when type is person.
- lastName string? - The last name of the person. This field is present only when type is person.
- title string? - The title of the person. This field is present only when type is person. Accepted values are existing custom titles.
- jobTitle string? - The job title of the person. This field is present only when type is person.
- organisation NestedParty? - Represents a reference to a party. By default, contains only the id, name and pictureURL. If the field is included in the embed parameter will instead be a full Party object. If the party is restricted for the user calling the API endpoint, the full Party object is not included.
- name string? - The name of the organisation. This field is present only when type is organisation.
- about string? - A short description of the party.
- createdAt string? - The ISO date/time when this party was created.
- updatedAt string? - The ISO date/time when this party was last updated.
- lastContactedAt string? - The ISO date/time when this party was last contacted.
- addresses Address[]? - An array of all the addresses associated with this party.
- phoneNumbers PhoneNumber[]? - An array of all the phone numbers associated with this party.
- websites Website[]? - An array of the websites and social network accounts associated with this party.
- emailAddresses EmailAddress[]? - An array of all the email addresses associated with this party.
- pictureURL string? - A URL that represents the location of the profile picture for this party. This field is automatically derived by Capsule.
- tags NestedTag[]? - An array of tags that are added to this party. This field is included in responses only if the embed parameter contains tags.
- fields FieldValue[]? - An array of custom fields that are defined for this party. This field is included in responses only if the embed parameter contains fields.
- owner NestedUser? - Represents a reference to a user. By default, contains only the id, name and username. If the field is included in the embed parameter will instead be a full User object.
- team NestedTeam? - Represents a reference to a team.
- missingImportantFields boolean? - Indicates if this party has any Important custom fields that are missing a value. This field is included in responses only if the embed parameter contains missingImportantFields.
capsulecrm: PartyObject
A Party object.
Fields
- party Party? - Represents a contact that is stored in Capsule. This can be either a person or an organisation.
capsulecrm: PhoneNumber
Represents a phone number of a party.
Fields
- id int? - The unique id of this phone number.
- 'type string? - The type of the phone number. Accepted values are Home, Work, Mobile, Fax, Direct.
- number string - The actual phone number.
capsulecrm: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
capsulecrm: Task
Represents a task that is stored in Capsule. These are generally used as a simple way to create a reminder of something the user needs to do. Tasks can be categorized for such things as a meeting, phone call or submission deadline using task categories and can be linked to a party, opportunity or case.
Fields
- id int? - The unique id of this task.
- createdAt string? - The ISO date/time when this task was created.
- updatedAt string? - The ISO date/time when this task was last updated.
- description string - A short description of the task.
- detail string? - More details about the task.
- category Category? - Represents a task category.
- dueOn string - The date (without a time element) when this task is due.
- dueTime string? - The time (without a date element) when this task is due. Note that the time is in the user's timezone, and not UTC.
- status string? - Specify whether this task is open, completed or pending. A task is pending when it was not completed by the user, but is not relevant anymore (i.e. linked to a closed opportunity). Accepted values are OPEN, COMPLETED, PENDING.
- party NestedParty? - Represents a reference to a party. By default, contains only the id, name and pictureURL. If the field is included in the embed parameter will instead be a full Party object. If the party is restricted for the user calling the API endpoint, the full Party object is not included.
- opportunity NestedOpportunity? - Represents a reference to an opportunity. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Opportunity object. If the opportunity is restricted for the user calling the API endpoint the full Opportunity object is not included.
- kase NestedCase? - Represents a reference to a case. By default, contains only the id and name. If the field is included in the embed parameter will instead be a full Case object. If the case is restricted for the user calling the API endpoint the full Case object is not included.
- owner NestedUser? - Represents a reference to a user. By default, contains only the id, name and username. If the field is included in the embed parameter will instead be a full User object.
- completedBy string? - The username of the user that completed this task.
- completedAt string? - The ISO DateTime when this task was completed. By default, this will be the time of the request that marked the task as completed. However, if you provide offline capability, you might want to override this field when completing the task.
- hasTrack boolean? - Determines if the task is part of a track associated with the parent kase or opportunity.
- nextTask NestedTask? - Represents a reference to a task. By default, contains only the id and description. If the field name is included in the embed parameter will instead be a full Task object.
- taskDelayRule string? - For tasks that are part of a track, this indicates if the dueOn date is calculated based on the start date of the track (TRACK_START), an end date specified when adding the track (END_DATE), or relative to the date the previous task in the track is completed (LAST_TASK). Accepted values are TRACK_START, END_DATE, LAST_TASK.
- daysAfter int? - If this task is part of a track with a taskDelayRule of LAST_TASK, indicates the number of days after the completion of the previous task that this task is due. If this task is part of a track with a taskDelayRule of START_DATE or END_DATE, indicates the number of days between the start or end date of the track and the due date of this task respectively.
capsulecrm: Tasks
An object with a single property tasks which is an array of Task objects.
Fields
- tasks Task[]? - An array of Task objects.
capsulecrm: TrackDefinitionId
Track Definition ID
Fields
- id int? - Track ID
capsulecrm: TracksDefinitionObject
Track definition object
Fields
- definition int|TrackDefinitionId? - Tracks can be specified using a definition object or the ID shorthand, or a mixture of both.
capsulecrm: UpdateOpportunityRequest
An object with a single property opportunity which must be an Opportunity object. Fields that are not included in the request will remain unchanged. An owner and/or team are required on an opportunity.
Fields
- opportunity Opportunity? - Represents an opportunity that is stored in Capsule.
capsulecrm: UpdatePartyRequest
An object with a single property party which must be a Party object. Fields that are not included in the request will remain unchanged.
Fields
- party Party? - Represents a contact that is stored in Capsule. This can be either a person or an organisation.
capsulecrm: Website
Represents a website or a social network account.
Fields
- id int? - The unique id of this website.
- 'service string - Defines what social network this website represents. Accepted values are URL, SKYPE, TWITTER, LINKED_IN, FACEBOOK, XING, FEED, GOOGLE_PLUS, FLICKR, GITHUB, YOUTUBE, INSTAGRAM, PINTEREST.
- address string - For Twitter, Github, Youtube, Pinterest, Instagram this is the account username. For all other services this is the profile or web page URL.
- 'type string? - Accepted values are Home, Work
- url string? - Generated URL that links to the website or profile page.
Import
import ballerinax/capsulecrm;
Metadata
Released date: almost 2 years ago
Version: 1.4.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.2.1
GraalVM compatible: Yes
Pull count
Total: 2172
Current verison: 165
Weekly downloads
Keywords
Sales & CRM/Customer Relationship Management
Cost/Freemium
Contributors