browshot
Module browshot
API
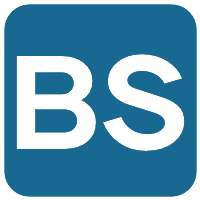
ballerinax/browshot Ballerina library
Overview
This is a generated connector for Browshot API v1.17.0 OpenAPI specification. Browshot API allows to get screenshots of any website in real time.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Browshot Account
- Obtaining tokens
- Log into Browshot Account
- After login token can be obtained by navigating to
Settings
by following this guide.
Quickstart
To use the Browshot connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/browshot module into the Ballerina project.
import ballerinax/browshot;
Step 2: Create a new connector instance
You can initialize the client as follows. You can now provide the API key obtained from the Browshot dashboard in the configuration.
browshot:ApiKeysConfig apiKeyConfig = { key: "<API_KEY>" } browshot:Client baseClient = check new Client(apiKeyConfig, serviceUrl = "https://api.browshot.com/api/v1");
Step 3: Invoke connector operation
- You can get all browsers.
browshot:BrowserList browserList = check baseClient->getBrowsersInfo();
- Use
bal run
command to compile and run the Ballerina program.
Clients
browshot: Client
This is a generated connector for Browshot API v1.17.0 OpenAPI specification. The Browshot API provides services to get screenshots of any website in real time.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Browshot Account and obtain token by navigating to Settings
by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.browshot.com/api/v1" - URL of the target service
createScreenshot
function createScreenshot(string url, int instanceId, string size, int cache, int delay, int flashDelay, int screenWidth, int screenHeight, int? priority, string? referer, string? postData, string? cookie, string? script, int details, int html, int maxWait, string? headers, int shots, int shotInterval, string? hosting, int? hostingHeight, int? hostingWidth, float hostingScale, string? hostingBucket, string? hostingFile, string? hostingHeaders) returns Screenshot|error
Request a screenshot
Parameters
- url string - URL of the page to get a screenshot for
- instanceId int - instance ID to use
- size string (default "screen") - screenshot size - "screen" (default) or "page"
- cache int (default 86400) - use a previous screenshot (same URL, same instance) if it was done within <cache_value> seconds. The default value is 24hours. Specify cache=0 if you want a new screenshot.
- delay int (default 5) - number of seconds to wait after the page has loaded. This is used to let JavaScript run longer before taking the screenshot. Use delay=0 to take screenshots faster.
- flashDelay int (default 10) - number of seconds to wait after the page has loaded if Flash elements are present. Use flash_delay=0 to take screenshots faster.
- screenWidth int (default 1024) - width of the browser window. For desktop browsers only.
- screenHeight int (default 768) - height of the browser window. For desktop browsers only. (Note: full-page screenshots can have a height of up to 15,000px)
- priority int? (default ()) - assign priority to the screenshot (for private instances only)
- referer string? (default ()) - use a custom referrer header - paid screenshots only
- postData string? (default ()) - send a POST requests with post_data, useful for filling out forms - paid screenshots only
- cookie string? (default ()) - set a cookie for the URL requested (see Custom POST Data, Referer and Cookie) Cookies should be separated by a ; - paid screenshots only
- script string? (default ()) - URL of javascript file to execute after the page load event
- details int (default 2) - level of information available with screenshot/info
- html int (default 0) - saves the HTML of the rendered page which can be retrieved by the API call screenshot/html. This feature costs 1 credit per screenshot.
- maxWait int (default 0) - maximum number of seconds to wait before triggering the PageLoad event. Note that delay will still be used. (default: 0 = disabled)
- headers string? (default ()) - any custom HTTP headers. (Not supported with Internet Explorer)
- shots int (default 1) - take multiple screenshots of the same page. This costs 1 additional credit for every 2 additional screenshots.
- shotInterval int (default 5) - number of seconds between 2 screenshots
- hosting string? (default ()) - hosting option - s3 or browshot
- hostingHeight int? (default ()) - maximum height of the thumbnail to host
- hostingWidth int? (default ()) - maximum height of the thumbnail to host
- hostingScale float (default 1.0) - scale of the thumbnail to host
- hostingBucket string? (default ()) - S3 bucket to upload the screenshot or thumbnail (required for S3)
- hostingFile string? (default ()) - file name to use (for S3 only)
- hostingHeaders string? (default ()) - list of headers to add to the S3 object (for S3 only)
Return Type
- Screenshot|error - Request accepted
createMultipleScreenshots
function createMultipleScreenshots(string url, int instanceId, string size, int cache, int delay, int flashDelay, int screenWidth, int screenHeight, int? priority, string? referer, string? postData, string? cookie, string? script, int details, int html, int maxWait, string? headers, string? hosting, int? hostingHeight, int? hostingWidth, float hostingScale, string? hostingBucket, string? hostingFile, string? hostingHeaders) returns ScreenshotList|error
Request multiple screenshots
Parameters
- url string - URL of the page to get a screenshot for. You can specify multiple url parameters (up to 10).
- instanceId int - instance ID to use. You can specify multiple instance_id parameters (up to 10).
- size string (default "screen") - screenshot size - "screen" (default) or "page"
- cache int (default 86400) - use a previous screenshot (same URL, same instance) if it was done within <cache_value> seconds. The default value is 24hours. Specify cache=0 if you want a new screenshot.
- delay int (default 5) - number of seconds to wait after the page has loaded. This is used to let JavaScript run longer before taking the screenshot. Use delay=0 to take screenshots faster.
- flashDelay int (default 10) - number of seconds to wait after the page has loaded if Flash elements are present. Use flash_delay=0 to take screenshots faster.
- screenWidth int (default 1024) - width of the browser window. For desktop browsers only.
- screenHeight int (default 768) - height of the browser window. For desktop browsers only. (Note: full-page screenshots can have a height of up to 15,000px)
- priority int? (default ()) - assign priority to the screenshot (for private instances only)
- referer string? (default ()) - use a custom referrer header - paid screenshots only
- postData string? (default ()) - send a POST requests with post_data, useful for filling out forms - paid screenshots only
- cookie string? (default ()) - set a cookie for the URL requested (see Custom POST Data, Referer and Cookie) Cookies should be separated by a ; - paid screenshots only
- script string? (default ()) - URL of javascript file to execute after the page load event
- details int (default 2) - level of information available with screenshot/info
- html int (default 0) - saves the HTML of the rendered page which can be retrieved by the API call screenshot/html. This feature costs 1 credit per screenshot.
- maxWait int (default 0) - maximum number of seconds to wait before triggering the PageLoad event. Note that delay will still be used. (default: 0 = disabled)
- headers string? (default ()) - any custom HTTP headers. (Not supported with Internet Explorer)
- hosting string? (default ()) - hosting option - s3 or browshot
- hostingHeight int? (default ()) - maximum height of the thumbnail to host
- hostingWidth int? (default ()) - maximum height of the thumbnail to host
- hostingScale float (default 1.0) - scale of the thumbnail to host
- hostingBucket string? (default ()) - S3 bucket to upload the screenshot or thumbnail (required for S3)
- hostingFile string? (default ()) - file name to use (for S3 only)
- hostingHeaders string? (default ()) - list of headers to add to the S3 object (for S3 only)
Return Type
- ScreenshotList|error - Request accepted
getScreenshotInfo
function getScreenshotInfo(int id, int details) returns Screenshot[]|error
Query screenshot status
Parameters
- id int - screenshot ID received from /api/v1/screenshot/create
- details int (default 2) - level of details about the screenshot and the page
Return Type
- Screenshot[]|error - Screenshot found
getMultipleScreenshotsInfo
function getMultipleScreenshotsInfo(int 'limit, string? status) returns ScreenshotList[]|error
Get information about screenshots
Parameters
- 'limit int (default 100) - maximum number of screenshots' information to return
- status string? (default ()) - get list of screenshot in a given status (error, finished, in_process)
Return Type
- ScreenshotList[]|error - list of screenshot information
searchScreenshot
function searchScreenshot(string url, int 'limit, string? status) returns ScreenshotList[]|error
Search for screenshots
Parameters
- url string - look for a string matching the URL requested
- 'limit int (default 50) - maximum number of screenshots' information to return
- status string? (default ()) - get list of screenshot in a given status (error, finished, in_process)
Return Type
- ScreenshotList[]|error - list of screenshot information
hostScreenshot
function hostScreenshot(int id, string hosting, int? width, int? height, decimal scale, string? bucket, string? file, string? headers) returns ScreenshotHost[]|error
Host thumbnails on your own S3 account or on Browshot.
Parameters
- id int - screenshot ID
- hosting string - hosting option: s3 or browshot
- width int? (default ()) - width of the thumbnail
- height int? (default ()) - height of the thumbnail
- scale decimal (default 1.0) - scale of the thumbnail
- bucket string? (default ()) - S3 bucket to upload the screenshot or thumbnail - required with hosting=s3
- file string? (default ()) - file name to use - optional, used with hosting=s3
- headers string? (default ()) - HTTP headers to add to your S3 object - optional, used with hosting=s3
Return Type
- ScreenshotHost[]|error - list of screenshot information
getThumbnail
function getThumbnail(int id, int? width, int? height, decimal scale, int zoom, string ratio, int left, int right, int top, int? bottom, string format, int shot, int quality) returns Response|error
Retrieve a thumbnail image
Parameters
- id int - screenshot ID
- width int? (default ()) - width of the thumbnail
- height int? (default ()) - height of the thumbnail
- scale decimal (default 1.0) - scale of the thumbnail
- zoom int (default 100) - zoom 1 to 100 percent
- ratio string (default "fit") - Use fit to keep the original page ration, and fill to get a thumbnail for the exact width and height. specified. If you provide both width and height, you need to specify the ratio: fit to keep the original width/height ratio (the thumbnail might be smaller than the specified width and height), or fill to crop the image if necessary.
- left int (default 0) - left edge of the area to be cropped
- right int (default 0) - right edge of the area to be cropped
- top int (default 0) - top edge of the area to be cropped
- bottom int? (default ()) - bottom edge of the area to be cropped
- format string (default "png") - image as PNG or JPEG
- shot int (default 1) - get the second or third screenshot if multiple screenshots were requested
- quality int (default 100) - JPEG quality factor (for JPEG thumbnails only)
shareScreenshot
function shareScreenshot(int id, string? note) returns ScreenshotHost[]|error
Share a screenshot
Return Type
- ScreenshotHost[]|error - list of screenshot information
deleteScreenshot
function deleteScreenshot(int id, string data) returns ScreenshotShort[]|error
Delete screenshot data
Parameters
- id int - screenshot ID
- data string (default "image") - data to remove. You can specify multiple of them (separated by a ,): image (image files), url (url requested), metadata (time added, time finished, post data, cookie and referer used for the screenshot), all (all data and files)
Return Type
- ScreenshotShort[]|error - list of screenshot information
getHTML
Get the HTML code
Parameters
- id int - screenshot ID
getBatchInfo
Get the batch status
Parameters
- id int - batch ID
getAccountInfo
Get information about your account
Parameters
- details int (default 1) - level of information returned
getInstanceInfo
Get information about an instance
Parameters
- id int - instance ID
getInstancesInfo
function getInstancesInfo() returns InstanceList|error
Get all instances
Return Type
- InstanceList|error - Instance information
getBrowserInfo
Get information about a browser
Parameters
- id int - browser ID
getBrowsersInfo
function getBrowsersInfo() returns BrowserList|error
Get all browsers
Return Type
- BrowserList|error - Instance information
Records
browshot: Account
Fields
- balance int - number of credits left on your account
- free_screenshots_left int - number of free screenshots available for the current month
- private_instances int - 1 is your account is authorized to create and use private instances, 0 otherwise (default)
- hosting_browshot int - 1 is your account is authorized to request hosting on Browshot, 0 otherwise (default)
- instances Instance[]? - list of private instances as returned by /api/v1/instance/list
- browsers Browser[]? - list of custom browsers as returned by /api/v1/browser/list
- screenshots Screenshot[]? - list of 10 latest screenshots requests as returned by /api/v1/screenshot/list
browshot: AccountError
Fields
- 'error string? - description of the problem that occurred
- status string? - status of the request - error
browshot: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- 'key string - All requests on the Browshot API needs to include an API key. The API key can be provided as part of the query string or as a request header. The name of the API key needs to be
key
.
browshot: Batch
Fields
- id int? - batch ID
- status string? - status of the request - "in_queue", "processing", "finished", "error"
- started int? - time of processing (UNIX timestamp)
- finished int? - time of batch completed (UNIX timestamp)
- count int? - number of unique URLs in the batch
- processed int? - number of screenshots finishe
- failed int? - number of screenshots failed
- urls string[]? - URLs to download the batch
browshot: BatchError
Fields
- 'error string? - description of the problem that occurred
- status string? - status of the request - "in_queue", "processing", "finished", "error"
browshot: Browser
Fields
- id int? - browser ID
- name string? - browser name and version: Firefox 45, etc.
- javascript int? - JavaScript support: 1 if enabled, 0 if disabled
- flash int? - Flash support: 1 if enabled, 0 if disabled
- mobile int? - Mobile browser: 1 if true, 0 if false
browshot: BrowserError
Fields
- 'error string? - description of the problem that occurred
- status string? - status of the request - error
browshot: BrowserList
Fields
- default int? -
browshot: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
browshot: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
browshot: Instance
Fields
- id int? - instance ID (required to requests screenshots)
- width int? - screen width in pixels
- height int? - screen height in pixels
- load float? - instance load: < 1: new screenshot requests will be processed immediately, 1-2: new screenshot requests will be processed in about two minutes, 2-3: new screenshot requests will be processed in about four minutes, 3-4: new screenshot requests will be processed in about six minutes, etc.
- browser Browser? -
- 'type string? - public, shared or private
- screenshot_cost int? - number of credits for each screenshot
- country string? - instance's country of origin
browshot: InstanceError
Fields
- 'error string? - description of the problem that occurred
- status string? - status of the request - error
browshot: InstanceList
Fields
- shared Instance[]? -
- free Instance[]? -
- 'private Instance[]? -
browshot: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
browshot: Screenshot
Fields
- id int - screenshot ID
- status string - status of the request: "in_queue", "processing", "finished", "error"
- screenshot_url record {}? - URL to download the screenshot
- 'error string? - description of the problem that occurred
- priority int? - priority given to the screenshot: high (1) to low (3)
- url string - original URL requested
- size string? - screenshot size requested
- width int? - screenshot width
- height int? - screenshot height
- final_url string? - URL of the screenshot (redirections can occur)
- scale decimal? - image scale. Always 1 for desktop browsers; mobiles may change the scale (zoom in or zoom out) to fit the page on the screen
- instance_id int - instance ID used for the screenshot
- cost int? - number of credits spent for the screenshot
- referer string? - custom referrer used (see Custom POST Data, Referer and Cookie)
- post_data string? - POST data sent (see Custom POST Data, Referer and Cookie)
- cookie string? - custom cookie used (see Custom POST Data, Referer and Cookie)
- delay int? - number of seconds to wait after page load
- flash_delay int? - number of seconds to wait after page load if Flash elements are present
- details int? - level of details about the screenshot and the page
- script string? - URL of optional javascript file executed after the page load event
- shared_url string? - if the screenshot was shared, show the public URL
browshot: ScreenshotError
Fields
- 'error string? - description of the problem that occurred
- status string? - status of the request - "in_queue", "processing", "finished", "error"
- priority decimal? - priority given to the screenshot - high (1) to low (3)
- cost decimal? - number of credits taken
browshot: ScreenshotHost
Fields
- id int? - screenshot ID
- status string? - status of the request: "error", "ok" or "in_queue"
- url string? - URL to the hosted screenshot or thumbnail
browshot: ScreenshotInfoError
Fields
- id int? - screenshot ID
- 'error string? - description of the problem that occurred
- status string? - status of the request - "in_queue", "processing", "finished", "error"
browshot: ScreenshotList
Fields
- default decimal? -
browshot: ScreenshotShort
Fields
- id int? - screenshot ID
- status string? - status of the request
Import
import ballerinax/browshot;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
IT Operations/Browser Tools
Cost/Freemium
Contributors