bitbucket
Module bitbucket
Definitions
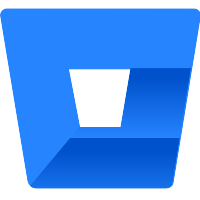
ballerinax/bitbucket Ballerina library
Overview
This is a generated connector for Bitbucket API v2.0 OpenAPI Specification.
Code against the Bitbucket API to automate simple tasks, embed Bitbucket data into your own site, build mobile or desktop apps, or even add custom UI add-ons into Bitbucket itself using the Connect framework.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a BitBucket account
- Obtain tokens
-
Go to the Bitbucket account and navigate to your workspace.
-
Then, Go to settings in the workspace.
-
Select OAuth consumers from Apps and Features list.
-
Create a new consumers and copy your key and secret.
-
Use the following code to generate the refresh token. The full-blown 3-LO flow. Request authorization from the end user by sending their browser to: https://bitbucket.org/site/oauth2/authorize?client_id={client_id}&response_type=code
The callback includes the ?code={} query parameter that you can swap for an access token:
$ curl -X POST -u "client_id:secret" https://bitbucket.org/site/oauth2/access_token -d grant_type=authorization_code -d code={code}
-
Use the following link as the refresh URL
https://bitbucket.org/site/oauth2/access_token
-
For more information, Refer this link
Quickstart
To use the BitBucket connector in your Ballerina application, update the .bal file as follows: Add steps to create a simple sample
Step 1 - Import connector
Import the ballerinax/bitbucket
module into the Ballerina project.
import ballerinax/bitbucket;
Step 2 - Create a new connector instance
bitbucket:ClientConfig configuration = { auth: { clientId: "<Client Id>", clientSecret: "<Client Secret>", refreshUrl: "<Refresh Url>", refreshToken: "<Refresh Token>" } }; bitbucket:Client bitBucketClient = check new (clientConfig);
Step 3 - Invoke connector operation
- Invoke connector operations using the client
public function main() { bitbucket;Issue|error response = bitBucketClient->getIssueByID(issueId, repoWithIssueTracker, workspace); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
bitbucket: Client
This is a generated connector for Bitbucket API v2.0 OpenAPI Specification. Code against the Bitbucket API to automate simple tasks, embed Bitbucket data into your own site, build mobile or desktop apps, or even add custom UI add-ons into Bitbucket itself using the Connect framework.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Bitbucket account and obtain tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.bitbucket.org/2.0" - URL of the target service
listWorkspaces
function listWorkspaces(string workspace, string? role, string? q, string? sort) returns PaginatedRepositories|error
Returns a list of all repositories
Parameters
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
.
- role string? (default ()) - Filters the result based on the authenticated user's role on each repository. member-returns repositories to which the user has explicit read access. contributor- returns repositories to which the user has explicit write access. admin- returns repositories to which the user has explicit administrator access. owner-returns all repositories owned by the current user
- q string? (default ()) - Query string to narrow down the response as https://developer.atlassian.com/bitbucket/api/2/reference/meta/filtering
- sort string? (default ()) - Field by which the results should be sorted as https://developer.atlassian.com/bitbucket/api/2/reference/meta/filtering
Return Type
- PaginatedRepositories|error - The repositories owned by the specified account.
listPullrequets
function listPullrequets(string repoSlug, string workspace, string? state) returns PaginatedPullrequests|error
Returns all pull requests
Parameters
- repoSlug string - This can either be the repository slug or the UUID of the repository, surrounded by curly-braces, for example:
{repository UUID}
.
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
.
- state string? (default ()) - Only return pull requests that are in this state. This parameter can be repeated.
Return Type
- PaginatedPullrequests|error - All pull requests on the specified repository.
getPullRequestByID
function getPullRequestByID(int pullRequestId, string repoSlug, string workspace) returns Pullrequest|error
Returns the specified pull request
Parameters
- pullRequestId int - The id of the pull request
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
Return Type
- Pullrequest|error - The pull request object
getRepositoryDetail
function getRepositoryDetail(string repoSlug, string workspace) returns Repository|error
Returns the object describing this repository
Parameters
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example: {repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example: {workspace UUID}
Return Type
- Repository|error - The repository object
createNewRepository
function createNewRepository(string repoSlug, string workspace, Repository payload) returns Repository|error
Creates a new repository
Parameters
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
- payload Repository - The repository that is to be created. Note that most object elements are optional. Elements "owner" and "full_name" are ignored as the URL implies them
Return Type
- Repository|error - The newly created repository.
deleteRepository
Deletes the repository
Parameters
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
getIssueByID
Returns the specified issue
Parameters
- issueId string - The issue id
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
deleteIssue
Deletes the specified issue
Parameters
- issueId string - The issue id
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
listIssues
function listIssues(string repoSlug, string workspace, string? q, string? sort) returns PaginatedIssues|error
Returns the issues in the issue tracker
Parameters
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
- q string? (default ()) - Query string to narrow down the response as https://developer.atlassian.com/bitbucket/api/2/reference/meta/filtering
- sort string? (default ()) - Field by which the results should be sorted as https://developer.atlassian.com/bitbucket/api/2/reference/meta/filtering
Return Type
- PaginatedIssues|error - A paginated list of the issues matching any filter criteria that were provided
createIssue
Creates a new issue
Parameters
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
- payload Issue - The new issue. The only required element is
title
. All other elements can be omitted from the body
listComments
function listComments(string issueId, string repoSlug, string workspace) returns PaginatedIssueComments|error
Returns a list of all comments on an issue
Parameters
- issueId string - The issue id
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
Return Type
- PaginatedIssueComments|error - A paginated list of issue comments
createNewIssueComment
function createNewIssueComment(string issueId, string repoSlug, string workspace, IssueComment payload) returns Response|error
Creates a new issue comment
Parameters
- issueId string - The issue id
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
- payload IssueComment - The new issue comment object
getCommentByID
function getCommentByID(int commentId, string issueId, string repoSlug, string workspace) returns IssueComment|error
Returns the specified issue comment object
Parameters
- commentId int - The id of the comment
- issueId string - The issue id
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
Return Type
- IssueComment|error - The issue comment
updateComment
function updateComment(int commentId, string issueId, string repoSlug, string workspace, IssueComment payload) returns IssueComment|error
Updates the content of the specified issue comment
Parameters
- commentId int - The id of the comment
- issueId string - The issue id
- repoSlug string - This can either be the repository slug or the UUID of the repository surrounded by curly-braces, for example:
{repository UUID}
- workspace string - This can either be the workspace ID (slug) or the workspace UUID surrounded by curly-braces, for example:
{workspace UUID}
- payload IssueComment - The updated comment
Return Type
- IssueComment|error - The updated issue comment
deleteComment
function deleteComment(int commentId, string issueId, string repoSlug, string workspace) returns Response|error
Deletes the specified comment
Parameters
- commentId int - The id of the comment
- issueId string - The issue id
- repoSlug string - This can either be the repository slug or the UUID of the repository
- workspace string - This can either be the workspace ID (slug) or the workspace UUID
getWorkSpaceByID
Returns the requested workspace
Parameters
- workspace string - This can either be the workspace ID (slug) or the workspace UUID
getProjectByProjectKey
Returns the requested project
Parameters
- projectKey string - The project in question. This is the actual
key
assigned to the project
- workspace string - This can either be the workspace ID (slug) or the workspace UUID
createOrUpdateProject
function createOrUpdateProject(string projectKey, string workspace, Project payload) returns Project|error
Creates or Updates a project
Parameters
- projectKey string - The project in question. This is the actual
key
assigned to the project.
- workspace string - This can either be the workspace ID (slug) or the workspace UUID
- payload Project - The project object
deleteProject
Deletes a project.
Records
bitbucket: Account
Fields
- links Links? - links to a resource related to comment object.
- username string? - Username of the account
- nickname string? - Account name defined by the owner. Should be used instead of the "username" field. Note that "nickname" cannot be used in place of "username" in URLs and queries, as "nickname" is not guaranteed to be unique.
- account_status string? - The status of the account. Currently the only possible value is "active", but more values may be added in the future
- display_name string? - Display name of the account
- website string? - Website of the account
- created_on string? - The created date of the account
- uuid string? - The UUID of the account
- has_2fa_enabled boolean? - Indicates whether two factor authentication is on
bitbucket: Author
Fields
- raw string? - The raw author value from the repository. This may be the only value available if the author does not match a user in Bitbucket
- user Account? -
bitbucket: BaseCommit
Fields
- hash string? - The hash value of the base commit
- date string? - The date of the base commit
- author Author? -
- message string? - The message of the base commit
- summary Summary? - The summary of the pull request
- parents BaseCommit[]? - The parent base commit
bitbucket: Branch
Fields
- Fields Included from *Ref
- merge_strategies string[]? - Available merge strategies for pull requests targeting this branch.
- default_merge_strategy string? - The default merge strategy for pull requests targeting this branch.
bitbucket: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
bitbucket: Comment
Fields
- id int? - The ID of the comment
- created_on string? - The created date of the comment
- updated_on string? - The updated date of the comment
- content Content? - The content of the issue
- user User? -
- deleted boolean? - Indicates whether the comment is deleted
- parent Comment? -
- inline Inline? - The comment's anchor line detail
- links Links? - links to a resource related to comment object.
bitbucket: Commit
Fields
- Fields Included from *BaseCommit
- repository Repository? -
- participants Participant[]? - The participants of the commit
bitbucket: Component
Fields
- links Links? - links to a resource related to comment object.
- name string? - The name of the component
- id int? - The ID of the component
bitbucket: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
bitbucket: Content
The content of the issue
Fields
- raw string? - The text as it was typed by a user.
- markup string? - The type of markup language the raw content is to be interpreted in.
- html string? - The user's content rendered as HTML
bitbucket: Description
Fields
- raw string? - The text as it was typed by a user
- markup string? - The type of markup language the raw content is to be interpreted in.
- html string? - The user's content rendered as HTML
bitbucket: Error
Base type for most resource objects. It defines the common type
element that identifies an object's type. It also identifies the element as Swagger's discriminator
.
Fields
- 'type string - The type of the error
- 'error ErrorDetail? - The error detail
bitbucket: ErrorDetail
The error detail
Fields
- message string -
- detail string? -
bitbucket: Inline
The comment's anchor line detail
Fields
- to int? - The comment's anchor line in the new version of the file. If the 'from' line is also provided, this value will be removed.
- 'from int? - The comment's anchor line in the old version of the file.
- path string - The path of the file this comment is anchored to.
bitbucket: Issue
Fields
- links Links? - links to a resource related to comment object.
- id int? - The ID of the issue
- repository Repository? -
- title string? - The title of the issue
- reporter User? -
- assignee User? -
- created_on string? - The created date/time of the issue
- updated_on string? - The last updated date/time of the issue
- edited_on string? - The last edited date/time of the issue
- state string? - The state of the issue
- kind string? - The kind of the issue
- priority string? - The priority level of the issue
- milestone Milestone? -
- 'version Version? -
- component Component? -
- votes int? - The number of votes
- content Content? - The content of the issue
bitbucket: IssueComment
Fields
- Fields Included from *Comment
- issue Issue? -
bitbucket: Link
A link to a resource related to this object.
Fields
- href string? -
- name string? -
bitbucket: Links
links to a resource related to comment object.
Fields
- self Link? - A link to a resource related to this object.
- html Link? - A link to a resource related to this object.
- code Link? - A link to a resource related to this object.
- commits Link? - A link to a resource related to this object.
- approve Link? - A link to a resource related to this object.
- diff Link? - A link to a resource related to this object.
- diffstat Link? - A link to a resource related to this object.
- comments Link? - A link to a resource related to this object.
- activity Link? - A link to a resource related to this object.
- merge Link? - A link to a resource related to this object.
- decline Link? - A link to a resource related to this object.
- name string? -
- avatar Link? - A link to a resource related to this object.
- followers Link? - A link to a resource related to this object.
- following Link? - A link to a resource related to this object.
- repositories Link? - A link to a resource related to this object.
- pullrequests Link? - A link to a resource related to this object.
- forks Link? - A link to a resource related to this object.
- watchers Link? - A link to a resource related to this object.
- downloads Link? - A link to a resource related to this object.
- clone Clone? -
- hooks Link? - A link to a resource related to this object.
- attachments Link? - A link to a resource related to this object.
- watch Link? - A link to a resource related to this object.
- vote Link? - A link to a resource related to this object.
- members Link? - A link to a resource related to this object.
- owners Link? - A link to a resource related to this object.
- projects Link? - A link to a resource related to this object.
- snippets Link? - A link to a resource related to this object.
bitbucket: MergeCommit
The pull request commit
Fields
- hash string? -
bitbucket: Milestone
Fields
- links Links? - links to a resource related to comment object.
- name string? - The name of the milestone
- id int? - The ID of the milestone
bitbucket: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://bitbucket.org/site/oauth2/access_token") - Refresh URL
bitbucket: PaginatedIssueComments
A paginated list of issue comments
Fields
- size int? - Total number of objects in the response. This is an optional element that is not provided in all responses, as it can be expensive to compute
- page int? - Page number of the current results. This is an optional element that is not provided in all responses
- pagelen int? - Current number of objects on the existing page. The default value is 10 with 100 being the maximum allowed value. Individual APIs may enforce different values
- next string? - Link to the next page if it exists. The last page of a collection does not have this value. Use this link to navigate the result set and refrain from constructing your own URLs
- previous string? - Link to previous page if it exists. A collections first page does not have this value. This is an optional element that is not provided in all responses. Some result sets strictly support forward navigation and never provide previous links. Clients must anticipate that backwards navigation is not always available. Use this link to navigate the result set and refrain from constructing your own URLs
- values IssueComment[]? - An array of issue comment objects returned by the operation
bitbucket: PaginatedIssues
A paginated list of issues.
Fields
- size int? - Total number of objects in the response. This is an optional element that is not provided in all responses, as it can be expensive to compute
- page int? - Page number of the current results. This is an optional element that is not provided in all responses
- pagelen int? - Current number of objects on the existing page. The default value is 10 with 100 being the maximum allowed value. Individual APIs may enforce different values
- next string? - Link to the next page if it exists. The last page of a collection does not have this value. Use this link to navigate the result set and refrain from constructing your own URLs
- previous string? - Link to previous page if it exists. A collections first page does not have this value. This is an optional element that is not provided in all responses. Some result sets strictly support forward navigation and never provide previous links. Clients must anticipate that backwards navigation is not always available. Use this link to navigate the result set and refrain from constructing your own URLs
- values Issue[]? - An array of issues
bitbucket: PaginatedPullrequests
A paginated list of pullrequests.
Fields
- size int? - Total number of objects in the response. This is an optional element that is not provided in all responses, as it can be expensive to compute.
- page int? - Page number of the current results. This is an optional element that is not provided in all responses.
- pagelen int? - Current number of objects on the existing page. The default value is 10 with 100 being the maximum allowed value. Individual APIs may enforce different values.
- next string? - Link to the next page if it exists. The last page of a collection does not have this value. Use this link to navigate the result set and refrain from constructing your own URLs.
- previous string? - Link to previous page if it exists. A collections first page does not have this value. This is an optional element that is not provided in all responses. Some result sets strictly support forward navigation and never provide previous links. Clients must anticipate that backwards navigation is not always available. Use this link to navigate the result set and refrain from constructing your own URLs.
- values Pullrequest[]? -
bitbucket: PaginatedRepositories
A paginated list of repositories.
Fields
- size int? - Total number of objects in the response. This is an optional element that is not provided in all responses, as it can be expensive to compute.
- page int? - Page number of the current results. This is an optional element that is not provided in all responses.
- pagelen int? - Current number of objects on the existing page. The default value is 10 with 100 being the maximum allowed value. Individual APIs may enforce different values.
- next string? - Link to the next page if it exists. The last page of a collection does not have this value. Use this link to navigate the result set and refrain from constructing your own URLs.
- previous string? - Link to previous page if it exists. A collections first page does not have this value. This is an optional element that is not provided in all responses. Some result sets strictly support forward navigation and never provide previous links. Clients must anticipate that backwards navigation is not always available. Use this link to navigate the result set and refrain from constructing your own URLs.
- values Repository[]? -
bitbucket: Participant
Fields
- user User? -
- role string? - The type of the participant
- approved boolean? - Indicates whether participant is approved
- state string? - The state of the participant
- participated_on string? - The ISO8601 timestamp of the participant's action. For approvers, this is the time of their approval. For commenters and pull request reviewers who are not approvers, this is the time they last commented, or null if they have not commented.
bitbucket: Project
Fields
- links Links? - links to a resource related to comment object.
- uuid string? - The project's immutable id
- 'key string? - The project's key.
- owner Team? - A team object.
- name string? - The name of the project
- description string? - The project description
- is_private boolean? - Indicates whether the project is publicly accessible, or whether it is private to the team and consequently only visible to team members. Note that private projects cannot contain public repositories.
- created_on string? - The created date/time of the project
- updated_on string? - The last updated date/time of the project
bitbucket: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
bitbucket: Pullrequest
Fields
- links Links? - links to a resource related to comment object.
- id int? - The pull request's unique ID. Note that pull request IDs are only unique within their associated repository
- title string? - Title of the pull request
- rendered Rendered? - User provided pull request text, interpreted in a markup language and rendered in HTML
- summary Summary? - The summary of the pull request
- state string? - The pull request's current status
- author Account? -
- 'source PullrequestEndpoint? -
- destination PullrequestEndpoint? -
- merge_commit MergeCommit? - The pull request commit
- comment_count int? - The number of comments for a specific pull request
- task_count int? - The number of open tasks for a specific pull request
- close_source_branch boolean? - A boolean flag indicating if merging the pull request closes the source branch
- closed_by Account? -
- reason string? - Explains why a pull request was declined. This field is only applicable to pull requests in rejected state
- created_on string? - The ISO8601 timestamp the request was created
- updated_on string? - The ISO8601 timestamp the request was last updated
- reviewers Account[]? - The list of users that were added as reviewers on this pull request when it was created. For performance reasons, the API only includes this list on a pull request's
self
URL.
- participants Participant[]? - The list of users that are collaborating on this
bitbucket: PullrequestBranch
Fields
- name string? -
- merge_strategies string[]? - Available merge strategies, when this endpoint is the destination of the pull request
- default_merge_strategy string? - The default merge strategy, when this endpoint is the destination of the pull request
bitbucket: PullrequestCommit
Fields
- hash string? -
bitbucket: PullrequestEndpoint
Fields
- repository Repository? -
- branch PullrequestBranch? -
- 'commit PullrequestCommit? -
bitbucket: Reason
Fields
- raw string? - The text as it was typed by a user
- markup string? - The type of markup language the raw content is to be interpreted in.
- html string? - The user's content rendered as HTML
bitbucket: Ref
A ref object, representing a branch or tag in a repository
Fields
- 'type string - The type of the ref object
- links Links? - links to a resource related to comment object.
- name string? - The name of the ref.
- target Commit? -
bitbucket: Rendered
User provided pull request text, interpreted in a markup language and rendered in HTML
Fields
- title Title? -
- description Description? -
- reason Reason? -
bitbucket: Repository
Fields
- links Links? - links to a resource related to comment object.
- uuid string? - The repository's immutable id. This can be used as a substitute for the slug segment in URLs. Doing this guarantees your URLs will survive renaming of the repository by its owner, or even transfer of the repository to a different user
- full_name string? - The concatenation of the repository owner's username and the slugified name, e.g. "evzijst/interruptingcow". This is the same string used in Bitbucket URLs
- is_private boolean? - Indicates whether the repository is private
- parent Repository? -
- scm string? - source control
- owner Account? -
- name string? - The name of the repository
- description string? - The description of the repository
- created_on string? - The created data/time of the repository
- updated_on string? - The last updated date/time of the repository
- size int? - The size of the repository
- language string? - The language of the repository
- has_issues boolean? - Indicates whether repository includes issues
- has_wiki boolean? - Indicates whether repository has a wiki
- fork_policy string? - Controls the rules for forking this repository. ()allow_forks)-unrestricted forking (no_public_forks) - restrict forking to private forks (forks cannot be made public later) (no_forks) -deny all forking
- project Project? -
- mainbranch Branch? -
bitbucket: Summary
The summary of the pull request
Fields
- raw string? - The text as it was typed by a user
- markup string? - The type of markup language the raw content is to be interpreted in.
- html string? - The user's content rendered as HTML
bitbucket: Team
A team object.
Fields
- Fields Included from *Account
bitbucket: Title
Fields
- raw string? - The text as it was typed by a user
- markup string? - The type of markup language the raw content is to be interpreted in
- html string? - The user's content rendered as HTML
bitbucket: User
Fields
- Fields Included from *Account
- is_staff boolean? - Indicates whether the user represents staff
- account_id string? - The user's Atlassian account ID
bitbucket: Version
Fields
- links Links? - links to a resource related to comment object.
- name string? - The name of the version
- id int? - The ID of the version
bitbucket: Workspace
Fields
- links Links? - links to a resource related to comment object.
- uuid string? - The workspace's immutable ID
- name string? - The name of the workspace
- slug string? - The short label that identifies this workspace
- is_private boolean? - Indicates whether the workspace is publicly accessible, or whether it is private to the members and consequently only visible to members. Note that private workspaces cannot contain public repositories
- created_on string? - The created date/time of the workspace
- updated_on string? - The last updated date/time of the workspace
Import
import ballerinax/bitbucket;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 13
Current verison: 6
Weekly downloads
Keywords
IT Operations/Source Control
Cost/Freemium
Contributors