bisstats
Module bisstats
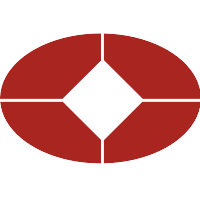
ballerinax/bisstats Ballerina library
Overview
This is a generated connector for BIS SDMX RESTful API v1 OpenAPI specification. The BIS SDMX RESTful API is a subset of the official SDMX RESTful API v1.4.0, released in June 2019. This service offers programmatic access to the BIS statistical data and metadata published on the BIS statistics pages released to the public. For additional information about the SDMX RESTful API, check the official sdmx-rest specification or the dedicated Wiki, including useful tips for consumers.
This module supports BIS SDMX RESTful API v1. The BIS SDMX RESTful API v1 is a subset of the official SDMX RESTful API v1.4.0
Quickstart
To use the BIS Stats connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/bisstats
module into the Ballerina project.
import ballerinax/bisstats;
Step 2: Create a new connector instance
Initialize the connector.
bisstats:Client baseClient = check new Client();
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get statistical Data from BIS using the connector. You can find more information about the parameters to pass here.
Get Statistical Data from BIS
public function main() { string|error response = baseClient->getData("BISWEB_EERDATAFLOW", "M.N.B.CH"); if (response is string) { log:printInfo(response); xml|error x = 'xml:fromString(response); } else { log:printError(response.message()); } }
Following is an example on how to get information about statistical data availability in BIS using the connector. You can find more information about the parameters to pass here.
Get Information about Statistical Data Availability in BIS
public function main() { string|error response = baseClient->getDataAvailabilityInformation("BISWEB_EERDATAFLOW", "M.N.B.CH", "all"); if (response is string) { log:printInfo(response); xml|error x = 'xml:fromString(response); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
bisstats: Client
This is a generated connector for BIS SDMX RESTful API v1 OpenAPI specification. The BIS SDMX RESTful API is a subset of the official SDMX RESTful API v1.4.0, released in June 2019. This service offers programmatic access to the BIS statistical data and metadata published on the BIS statistics pages released to the public. For additional information about the SDMX RESTful API, check the official sdmx-rest specification or the dedicated Wiki, including useful tips for consumers.
Constructor
Gets invoked to initialize the connector
.
The connector initialization doesn't require setting the API credentials.
Please refer the API documentation for more information.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://stats.bis.org/api/v1" - URL of the target service
getData
function getData(string flow, string 'key, string? startPeriod, string? endPeriod, int? firstNObservations, int? lastNObservations, string detail, string acceptEncoding) returns string|error
Get data
Parameters
- flow string - The statistical domain (aka dataflow) of the data to be returned. Examples: *
BISWEB_EERDATAFLOW
: The ID of the domain *BIS,BISWEB_EERDATAFLOW
: The BISWEB_EERDATAFLOW domain, maintained by the BIS *BIS,BISWEB_EERDATAFLOW,1.0
: Version 1.0 of the BISWEB_EERDATAFLOW domain, maintained by the BIS
- 'key string - The (possibly partial) key identifying the data to be returned. The keyword
all
can be used to indicate that all data belonging to the specified dataflow and provided by the specified provider must be returned. The examples below are based on the following key: Frequency, Type, Basket, Country. *M.N.B.CH
: Full key, matching exactly one series, i.e. the monthly (M
) nominal rate (N
) in the broad basket (B
) for Switzerland (CH
). *M.N+R.B.CH
: Retrieves both nominal and real rates (N+R
), matching exactly two series. *M.N.B.
: The last dimension is wildcarded, and it will therefore match all countries.
- startPeriod string? (default ()) - The start of the period for which results should be supplied (inclusive). Can be expressed using 8601 dates or SDMX reporting periods. Examples: *
2000
: Year (ISO 8601) *2000-01
: Month (ISO 8601) *2000-01-01
: Date (ISO 8601) *2000-Q1
: Quarter (SDMX) *2000-W01
: Week (SDMX)
- endPeriod string? (default ()) - The end of the period for which results should be supplied (inclusive). Can be expressed using 8601 dates or SDMX reporting periods. Examples: *
2000
: Year (ISO 8601) *2000-01
: Month (ISO 8601) *2000-01-01
: Date (ISO 8601) *2000-S1
: Semester (SDMX) *2000-D001
: Day (SDMX)
- firstNObservations int? (default ()) - The maximum number of observations to be returned starting from the oldest one
- lastNObservations int? (default ()) - The maximum number of observations to be returned starting from the most recent one
- detail string (default "full") - The amount of information to be returned. Possible options are: *
full
: All data and documentation *dataonly
: Everything except attributes *serieskeysonly
: The series keys. This is useful to return the series that match a certain query, without returning the actual data (e.g. overview page) *nodata
: The series, including attributes and annotations, without observations.
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getDataAvailabilityInformation
function getDataAvailabilityInformation(string flow, string 'key, string componentID, string mode, string references, string? startPeriod, string? endPeriod, string acceptEncoding) returns string|error
Get information about data availability
Parameters
- flow string - The statistical domain (aka dataflow) of the data to be returned. Examples: *
BISWEB_EERDATAFLOW
: The ID of the domain *BIS,BISWEB_EERDATAFLOW
: The BISWEB_EERDATAFLOW domain, maintained by the BIS *BIS,BISWEB_EERDATAFLOW,1.0
: Version 1.0 of the BISWEB_EERDATAFLOW domain, maintained by the BIS
- 'key string - The (possibly partial) key identifying the data to be returned. The keyword
all
can be used to indicate that all data belonging to the specified dataflow and provided by the specified provider must be returned. The examples below are based on the following key: Frequency, Type, Basket, Country. *M.N.B.CH
: Full key, matching exactly one series, i.e. the monthly (M
) nominal rate (N
) in the broad basket (B
) for Switzerland (CH
). *M.N+R.B.CH
: Retrieves both nominal and real rates (N+R
), matching exactly two series. *M.N.B.
: The last dimension is wildcarded, and it will therefore match all countries.
- componentID string - The id of the Dimension for which to obtain availability information about. Use all to indicate that data availability should be provided for all dimensions.
- mode string (default "exact") - Instructs the web service to return a ContentConstraint which defines a Cube Region containing values which will be returned by executing the query (mode="exact") vs a Cube Region showing what values remain valid selections that could be added to the data query (mode="available"). A valid selection is one which results in one or more series existing for the selected value, based on the current data query selection state defined by the current path parameters.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the ContentConstraint to be returned.
- startPeriod string? (default ()) - The start of the period for which results should be supplied (inclusive). Can be expressed using 8601 dates or SDMX reporting periods. Examples: *
2000
: Year (ISO 8601) *2000-01
: Month (ISO 8601) *2000-01-01
: Date (ISO 8601) *2000-Q1
: Quarter (SDMX) *2000-W01
: Week (SDMX)
- endPeriod string? (default ()) - The end of the period for which results should be supplied (inclusive). Can be expressed using 8601 dates or SDMX reporting periods. Examples: *
2000
: Year (ISO 8601) *2000-01
: Month (ISO 8601) *2000-01-01
: Date (ISO 8601) *2000-S1
: Semester (SDMX) *2000-D001
: Day (SDMX)
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getDataStructures
function getDataStructures(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get data structures
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getDataFlows
function getDataFlows(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get dataflows
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getCategorisations
function getCategorisations(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get categorisations
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getContentConstraints
function getContentConstraints(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get content constraints
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getActualConstraints
function getActualConstraints(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get actual constraints
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getAllowedConstraints
function getAllowedConstraints(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get allowed constraints
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getStructures
function getStructures(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get structures
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getConceptSchemes
function getConceptSchemes(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get concept schemes
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getCodelists
function getCodelists(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get codelists
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getCategorySchemes
function getCategorySchemes(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get category schemes
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getHierarchicalCodelists
function getHierarchicalCodelists(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get hierarchical codelists
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getAgencySchemes
function getAgencySchemes(string agencyID, string resourceID, string 'version, string references, string detail, string acceptEncoding) returns string|error
Get agency schemes
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getConcepts
function getConcepts(string agencyID, string resourceID, string 'version, string itemID, string references, string detail, string acceptEncoding) returns string|error
Get concepts
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- itemID string - The id of the item to be returned. It is possible to set more than one id, using
+
as separator (e.g. A+Q+M). The keywordall
can be used to return all items of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getCodes
function getCodes(string agencyID, string resourceID, string 'version, string itemID, string references, string detail, string acceptEncoding) returns string|error
Get codes
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- itemID string - The id of the item to be returned. It is possible to set more than one id, using
+
as separator (e.g. A+Q+M). The keywordall
can be used to return all items of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getCategories
function getCategories(string agencyID, string resourceID, string 'version, string itemID, string references, string detail, string acceptEncoding) returns string|error
Get categories
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- itemID string - The id of the item to be returned. It is possible to set more than one id, using
+
as separator (e.g. A+Q+M). The keywordall
can be used to return all items of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
getHierarchies
function getHierarchies(string agencyID, string resourceID, string 'version, string itemID, string references, string detail, string acceptEncoding) returns string|error
Get hierarchies
Parameters
- agencyID string - The agency maintaining the artefact to be returned. It is possible to set more than one agency, using
+
as separator (e.g. BIS+ECB). The keywordall
can be used to indicate that artefacts maintained by any maintenance agency should be returned.
- resourceID string - The id of the artefact to be returned. It is possible to set more than one id, using
+
as separator (e.g. CL_FREQ+CL_CONF_STATUS). The keywordall
can be used to indicate that any artefact of the specified resource type, {agencyID} and {version} should be returned.
- 'version string - The version of the artefact to be returned. It is possible to set more than one version, using
+
as separator (e.g. 1.0+2.1). The keywordall
can be used to return all versions of the matching resource. The keywordlatest
can be used to return the latest production version of the matching resource.
- itemID string - The id of the item to be returned. It is possible to set more than one id, using
+
as separator (e.g. A+Q+M). The keywordall
can be used to return all items of the matching resource.
- references string (default "none") - Instructs the web service to return (or not) the artefacts referenced by the artefact to be returned. Possible values are: *
none
: No references will be returned *parents
: Returns the artefacts that use the artefact matching the query *parentsandsiblings
: Returns the artefacts that use the artefact matching the query, as well as the artefacts referenced by these artefacts *children
: Returns the artefacts referenced by the artefact to be returned *descendants
: References of references, up to any level, will be returned *all
: The combination of parentsandsiblings and descendants * In addition, a concrete type of resource may also be used (for example, references=codelist).
- detail string (default "full") - The amount of information to be returned. Possible values are: *
allstubs
: All artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencestubs
: Referenced artefacts should be returned as stubs, containing only identification information, as well as the artefacts' name *referencepartial
: Referenced item schemes should only include items used by the artefact to be returned. For example, a concept scheme would only contain the concepts used in a DSD, and its isPartial flag would be set totrue
*allcompletestubs
: All artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *referencecompletestubs
: Referenced artefacts should be returned as complete stubs, containing identification information, the artefacts' name, description, annotations and isFinal information *full
: All available information for all artefacts should be returned
- acceptEncoding string (default "identity") - Specifies whether the response should be compressed and how.
identity
(the default) indicates that no compression will be performed.
Records
bisstats: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
bisstats: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
bisstats: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Import
import ballerinax/bisstats;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Business Intelligence/Analytics
Cost/Free
Contributors
Dependencies