azure_storage_service.files
Modules
Module azure_storage_service.files
Declarations
Definitions
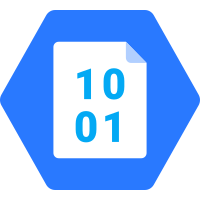
ballerinax/azure_storage_service.files Ballerina library
Overview
This module allows you to access the Azure Storage File REST API through Ballerina. Azure Files offers fully managed file shares in the cloud that are accessible via the Server Message Block (SMB) protocol or Network File System (NFS) protocol. This module provides you the capability to execute operations like getFileList, getDirectoryList, createDirectory, directUpload and getFile etc using the FileClient. It also allows you to execute management operations such as createShare and deleteShare etc using the ManagementClient.
This module supports Azure Storage Service REST API 2019-12-12 version.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Obtain
Shared Access Signature
(SAS
) or use one of the Accesskeys for authentication.
Quickstart
To use this connector in your Ballerina application, update the .bal file as follows:
Step1: Import connector
Import the ballerinax/azure_storage_service.files
module into the Ballerina project.
import ballerinax/azure_storage_service.files as azure_files;
Step2: Create a new connector instance
Create an azure_files:ConnectionConfig
with the obtained Shared Access Signature or Access Key, base URL and account name.
- If you are using Shared Access Signature, use the follwing format.
azure_files:ConnectionConfig fileServiceConfig = { accessKeyOrSAS: "ACCESS_KEY_OR_SAS", accountName: "ACCOUNT_NAME", authorizationMethod: "SAS" };
- If you are using one of the Access Key, use the follwing format.
azure_files:ConnectionConfig fileServiceConfig = { accessKeyOrSAS: "ACCESS_KEY_OR_SAS", accountName: "ACCOUNT_NAME", authorizationMethod: "accessKey" };
Create the FileClient using the fileServiceConfig you have created as shown above.
azure_files:FileClient fileClient = check new (fileServiceConfig);
Step3: Invoke connector operation
- Now you can use the operations available within the connector. Note that they are in the form of remote operations. Following is an example on how to list all the directories in a file share using the connector.
public function main() returns error? { azure_files:ConnectionConfig fileServiceConfig = { accessKeyOrSAS: "ACCESS_KEY_OR_SAS", accountName: "ACCOUNT_NAME", authorizationMethod: "accessKey" }; azure_files:FileClient fileClient = check new (fileServiceConfig); azure_files:DirectoryList result = check fileClient->getDirectoryList(fileShareName = "demoshare"); }
- Use
bal run
command to compile and run the Ballerina program.
Quick reference
- Get list of directories in a file share
azure_files:DirectoryList result = check fileClient->getDirectoryList(fileShareName = "demoshare");
- Get list of files in a file share
azure_files:FileList result = check fileClient->getFileList(fileShareName = "demoshare");
- Create a directory
_ = check fileClient->createDirectory(fileShareName = "demoshare", newDirectoryName = "demoDirectory");
- Upload a file to a fileshare.
_ = check fileClient->directUpload(fileShareName = "demoshare", localFilePath = "resources/uploads/test.txt", azureFileName = "testfile.txt");
- Download a file from a fileshare.
_ = check fileClient->getFile(fileShareName = "demoshare", fileName = "testfile.txt", localFilePath = "resources/downloads/downloadedFile.txt");
Clients
azure_storage_service.files: FileClient
Azure Storage File connector allows you to access the Azure Files REST API. This connector lets you to access data stored in Azure file shares.
Constructor
Initializes the connector.
Create an Azure account following this guide.
Create an Azure Storage account following this guide
Obtain Shared Access Signature
(SAS
) or use one of the Accesskeys for authentication.
init (ConnectionConfig config)
- config ConnectionConfig -
getDirectoryList
function getDirectoryList(string fileShareName, string? azureDirectoryPath, GetFileListURIParameters uriParameters) returns DirectoryList|Error
Lists directories within the share or specified directory.
Parameters
- fileShareName string - Name of the FileShare
- azureDirectoryPath string? (default ()) - Path of the Azure directory
- uriParameters GetFileListURIParameters (default {}) - Map of the optional URI parameters record
Return Type
- DirectoryList|Error - If success, DirectoryList record with Details and the marker. Else an error.
getFileList
function getFileList(string fileShareName, string? azureDirectoryPath, GetFileListURIParameters uriParameters) returns FileList|Error
Lists files within the share or specified directory.
Parameters
- fileShareName string - Name of the FileShare
- azureDirectoryPath string? (default ()) - Path of the Azure directory
- uriParameters GetFileListURIParameters (default {}) - Map of the optional URI parameters record
createDirectory
function createDirectory(string fileShareName, string newDirectoryName, string? azureDirectoryPath) returns Error?
Creates a directory in the share or parent directory.
Parameters
- fileShareName string - Name of the fileshare
- newDirectoryName string - New directory name in azure
- azureDirectoryPath string? (default ()) - Path to the new directory
Return Type
- Error? - If success true. Else an error
deleteDirectory
function deleteDirectory(string fileShareName, string directoryName, string? azureDirectoryPath) returns Error?
Deletes the directory. Only supported for empty directories.
Parameters
- fileShareName string - Name of the FileShare
- directoryName string - Name of the Directory to be deleted
- azureDirectoryPath string? (default ()) - Path of the Azure directory
Return Type
- Error? - If success true. Else an error
createFile
function createFile(string fileShareName, string newFileName, int fileSizeInByte, string? azureDirectoryPath) returns Error?
Creates a new file or replaces a file. This operation only initializes a file. putRange should be used to add content.
Parameters
- fileShareName string - Name of the fileShare
- newFileName string - Name of the file
- fileSizeInByte int - Size of the file in Bytes
- azureDirectoryPath string? (default ()) - Path of the Azure directory
Return Type
- Error? - If success true. Else an error
getFileMetadata
function getFileMetadata(string fileShareName, string fileName, string? azureDirectoryPath, OptionalURIParametersFileMetaData uriParameters) returns FileMetadataResult|Error
Gets File Metadata.
Parameters
- fileShareName string - Name of the FileShare
- fileName string - Name of the File
- azureDirectoryPath string? (default ()) - Path of the azure directory
- uriParameters OptionalURIParametersFileMetaData (default {}) - Optional URI Parameter Description
Return Type
- FileMetadataResult|Error - If successful, File Metadata. Else an Error
putRange
function putRange(string fileShareName, string localFilePath, string azureFileName, string? azureDirectoryPath) returns Error?
Writes the content (a range of bytes) to a file initialized earlier.
Parameters
- fileShareName string - Name of the FileShare
- localFilePath string - Path of the local file
- azureFileName string - Name of the file in azure
- azureDirectoryPath string? (default ()) - Path of the azure directory
Return Type
- Error? - If success true. Else an error
listRange
function listRange(string fileShareName, string fileName, string? azureDirectoryPath) returns RangeList|Error
Provides a list of valid ranges (in bytes) of a file.
Parameters
- fileShareName string - Name of the FileShare
- fileName string - Name of the file
- azureDirectoryPath string? (default ()) - Path of the Azure directory
deleteFile
function deleteFile(string fileShareName, string fileName, string? azureDirectoryPath) returns Error?
Deletes a file from the fileshare.
Parameters
- fileShareName string - Name of the FileShare
- fileName string - Name of the file
- azureDirectoryPath string? (default ()) - Path of the Azure directory
Return Type
- Error? - If success true. Else an error
getFile
function getFile(string fileShareName, string fileName, string localFilePath, string? azureDirectoryPath) returns Error?
Downloads a file from fileshare to a specified location.
Parameters
- fileShareName string - Name of the FileShare
- fileName string - Name of the file
- localFilePath string - Path to the local destination location
- azureDirectoryPath string? (default ()) - Path of azure directory
Return Type
- Error? - If success true. Else an error
getFileAsByteArray
function getFileAsByteArray(string fileShareName, string fileName, string? azureDirectoryPath, ContentRange? range) returns byte[]|Error
Downloads a file from fileshare to a specified location as an Byte array
Parameters
- fileShareName string - Name of the FileShare
- fileName string - Name of the file
- azureDirectoryPath string? (default ()) - Path of azure directory
- range ContentRange? (default ()) - The specified byte range to be returned the file data
Return Type
- byte[]|Error - If successful, file content as a byte array. Else an error
copyFile
function copyFile(string fileShareName, string sourceURL, string destFileName, string? destDirectoryPath) returns Error?
Copies a file to another location in fileShare.
Parameters
- fileShareName string - Name of the fileShare
- sourceURL string - source file url in the fileShare
- destFileName string - Name of the destination file
- destDirectoryPath string? (default ()) - Path of the destination in fileShare
Return Type
- Error? - If success, true. Else an error
directUpload
function directUpload(string fileShareName, string localFilePath, string azureFileName, string? azureDirectoryPath) returns Error?
Upload a file directly to the fileshare.
Parameters
- fileShareName string - Name of the fileShare
- localFilePath string - The path of the file to be uploaded
- azureFileName string - The name of the file in Azure
- azureDirectoryPath string? (default ()) - Directory path in Azure
Return Type
- Error? - If success true. Else an error
directUploadFileAsByteArray
function directUploadFileAsByteArray(string fileShareName, byte[] fileContent, string azureFileName, string? azureDirectoryPath) returns Error?
Uploads a byte array directly to the fileshare.
Parameters
- fileShareName string - Name of the fileShare
- fileContent byte[] - File content as a byte array
- azureFileName string - Name of the file in Azure
- azureDirectoryPath string? (default ()) - Directory path in Azure
Return Type
- Error? - If success true. Else an error
azure_storage_service.files: ManagementClient
Azure Storage File Service Management Client.
Constructor
Initialize Azure Client using the provided azureConfiguration by user
init (ConnectionConfig config)
- config ConnectionConfig -
listShares
function listShares(ListShareURIParameters uriParameters) returns SharesList|Error
Lists all the file shares in the storage account.
Parameters
- uriParameters ListShareURIParameters (default {}) - URI Parameters
Return Type
- SharesList|Error - If success, returns ShareList record with basic details. Else returns an error.
getFileServiceProperties
function getFileServiceProperties() returns FileServicePropertiesList|Error
Gets the File service properties for the storage account.
Return Type
- FileServicePropertiesList|Error - If success, returns FileServicePropertiesList record with details. Else returns error.
setFileServiceProperties
function setFileServiceProperties(FileServicePropertiesList fileServicePropertiesList) returns Error?
Sets the File service properties for the storage account.
Parameters
- fileServicePropertiesList FileServicePropertiesList - fileServicePropertiesList record with detail to be set
Return Type
- Error? - If success, returns true. Else returns error.
createShare
function createShare(string fileShareName, RequestHeaders? fileShareRequestHeaders) returns Error?
Creates a new share in a storage account.
Parameters
- fileShareName string - Name of the fileshare
- fileShareRequestHeaders RequestHeaders? (default ()) - Optional. Map of the user defined optional headers
Return Type
- Error? - If success, returns true. Else returns error.
getShareProperties
function getShareProperties(string fileShareName) returns FileServicePropertiesList|Error
Returns all user-defined metadata and system properties of a share.
Parameters
- fileShareName string - Name of the FileShare
Return Type
- FileServicePropertiesList|Error - If success, returns FileServicePropertiesList record with Details. Else returns error.
deleteShare
Deletes the share and any files and directories it contains.
Parameters
- fileShareName string - Name of the fileshare
Return Type
- Error? - If success, returns true. Else returns error.
Enums
azure_storage_service.files: AuthorizationMethod
Azure storage service authorization methods
Members
Records
azure_storage_service.files: ConnectionConfig
Azure File Service Configuration.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth never? -
- accessKeyOrSAS string - Access key or Shared Access Signature for Azure Storage Account
- accountName string - Name of the Azure Storage account
- authorizationMethod AuthorizationMethod - Holds the used authorization method from the enum AuthorizationMethod
- httpVersion HttpVersion(default http:HTTP_1_1) - The HTTP version understood by the client
azure_storage_service.files: ContentRange
Defines byte range of a file content
Fields
- startByte int - Start position, Example: 0
- endByte int - End position, Example: 255
azure_storage_service.files: CoreRulesType
Represents a CORS rules.
Fields
- AllowedOrigins string? - A comma-separated list of origin domains that will be allowed via CORS, or "*" to allow all domains
- AllowedMethods string? - A comma-separated list of response headers to expose to CORS clients
- MaxAgeInSeconds string? - The number of seconds that the client/browser should cache a preflight response
- AllowedHeaders string? - A comma-separated list of headers allowed to be part of the cross-origin request
- ExposedHeaders string? - A comma-separated list of HTTP methods that are allowed to be executed by the origin
azure_storage_service.files: CorsType
Contains the CORS rules.
Fields
- CorsRules CoreRulesType? - Represents the CORS rules
azure_storage_service.files: Directory
Represnts an Azure directory.
Fields
- Name string - Name of the azure directory
- Properties PropertiesFileItem|EMPTY_STRING? - Properties of the directory
azure_storage_service.files: DirectoryList
Represents a list of azure directories.
Fields
- Marker string? - Marker for the list
- MaxResults int? - limits number of results in the list
azure_storage_service.files: File
Represents an Azure file.
Fields
- Name string - Name of the azure file
- Properties PropertiesFileItem|EMPTY_STRING? - Properties of the azure file
azure_storage_service.files: FileList
Represents a list of files.
Fields
- Marker string? - Marker for the list
- MaxResults int? - limits number of results in the list
azure_storage_service.files: FileMetadataResult
Represents File Metadata Result.
Fields
- eTag string - ETag
- lastModified string - Date/time that the file was last modified
- responseHeaders ResponseHeaders - Response headers from Azure
azure_storage_service.files: FileServicePropertiesList
Represents the file service properties list.
Fields
- StorageServiceProperties StorageServicePropertiesType - Storage Service Properties record
azure_storage_service.files: GetDirectoryListURIParameters
Represents optional URI parameters for GetDirectoryList operation.
Fields
- prefix string? - Filters the results to return only directories whose name begins with the specified prefix
- sharesnapshot string? - The share snapshot to query for the list of directories
- marker string? - A string value that identifies the portion of the list to be returned with the next list operation
- maxresults string? - The maximum number of shares to return. Maximum limit and default is 5000.
- timeout string? - The timeout parameter is expressed in seconds
azure_storage_service.files: GetFileListURIParameters
Represents optional URI parameters for GetFileList operation.
Fields
- prefix string? - Filters the results to return only files whose name begins with the specified prefix
- sharesnapshot string? - The share snapshot to query for the list of files and directories
- marker string? - A string value that identifies the portion of the list to be returned with the next list operation
- maxresults string? - The maximum number of shares to return. Maximum limit and default is 5000.
- timeout string? - The timeout parameter is expressed in seconds
azure_storage_service.files: ListShareURIParameters
Represents optional URI parameters for ListShares operation.
Fields
- prefix string? - Filters the results to return only shares whose name begins with the specified prefix
- marker string? - A string value that identifies the portion of the list to be returned with the next list operation
- maxresults string? - Specifies the maximum number of shares to return. Maximum limit and default is 5000.
- include string? - Specifies one or more datasets to include in the response like metadata, snapshots, deleted
- timeout string? - The timeout parameter is expressed in seconds
azure_storage_service.files: MetricsType
Represents the Storage Analytics HourMetrics/MinuteMetrics settings.
Fields
- Version string - The version of Storage Analytics to configure
- RetentionPolicy RetentionPolicyType? - Indicates whether metrics should generate summary statistics for called API operations
azure_storage_service.files: MultichannelType
Contains the settings for SMB multichannel.
Fields
- Enabled string? - Toggles the state of SMB multichannel
azure_storage_service.files: NoSharesFoundErrorData
Represents a record for share not found error information.
Fields
- storageAccountName string - Name of the fileshare that error is related
azure_storage_service.files: OptionalURIParametersFileMetaData
Represents optional URI parameters for get meta data operation.
Fields
- sharesnapshot string? - The sharesnapshot parameter is an opaque DateTime value that, when present, specifies the share snapshot to query to retrieve the metadata
- timeout string? - The timeout parameter is expressed in seconds
azure_storage_service.files: PropertiesFileItem
Represents the details of the Properties.
Fields
- 'Content\-Length string? -
azure_storage_service.files: PropertiesItem
Represents Properties of the share.
Fields
- 'Last\-Modified string -
- Quota string - Quota of the fileShare
- Etag string? - Etag given by the fileShare
- AccessTier string? - AccessTier of the fileShare
azure_storage_service.files: ProtocolSettingsType
Groups the settings for file system protocols.
Fields
- SMB SMBType? - Represents SMB type variable
azure_storage_service.files: RangeItem
Represents a range item as a record.
Fields
- Start string - Start byte
- End string - End byte
azure_storage_service.files: RangeItemList
Represents a range item list as a record.
Fields
- Range RangeItem - Range item
azure_storage_service.files: RangeList
Represents a range of a file content.
Fields
- Ranges string|RangeItemList - A list of Ranges
azure_storage_service.files: RequestHeaders
Represents optional request headers for CreateShareHeaders operation.
Fields
- 'x\-ms\-share\-quota string? -
- 'x\-ms\-access\-tier string? -
- 'x\-ms\-enabled\-protocols string? -
azure_storage_service.files: RequestParameterList
Represents different types of Request parameters.
Fields
- fileShareName string - Name of the fileshare
- azureDirectoryName string? - Name of the azure directory
- azureFileName string? - Name of the file name
- azureDirectoryPath string? - Path of the azure directory
- SearchPrefix string? - Search prefix word
- maxResult int? - Maximum number of search results in the response
- marker int? - Marker to the left items if any
- newDirectoryName string? - Name of the new directory to be created
- fileSizeInByte int? - Size of the file in bytes
- localFilePath string? - Path to the local location of a file
azure_storage_service.files: ResponseHeaders
Represents Response headers.
Fields
- Date string - A UTC date/time value that indicates the time at which the response was initiated
- x\-ms\-version string - Indicates the service version that was used to execute the request
- x\-ms\-request\-id string - Uniquely identifies the request that was made, and can be used for troubleshooting
azure_storage_service.files: RetentionPolicyType
Contains the Retention Policy details.
Fields
- Enabled string? - Indicates whether metrics are enabled for the File service
- Days string? - Indicates the number of days that metrics data should be retained
azure_storage_service.files: ServerErrorDetail
Defines the details of an File Service error message.
Fields
- httpStatus int - HTTP status code associated with the error
- errorCode string - Azure File Service error code
- message string - Associated error message
azure_storage_service.files: ShareItem
Represents a share.
Fields
- Name string - Name of the share
- Properties PropertiesItem - Properties of the share
azure_storage_service.files: Shares
Represents a File share or FileShare array.
Fields
azure_storage_service.files: SharesList
Represents a list of FileShares.
Fields
- Shares Shares - Shares type record
azure_storage_service.files: SMBType
Groups the settings for SMB.
Fields
- Multichannel MultichannelType? - Contains multi channel type record
azure_storage_service.files: StorageServicePropertiesType
Represents the storage service properties type record.
Fields
- HourMetrics MetricsType? - Provides a summary of request statistics grouped by API in hourly aggregates
- MinuteMetrics MetricsType? - Provides a summary of request statistics grouped by API for each minute
- ProtocolSettings ProtocolSettingsType? - Groups the settings for file system protocols
Errors
azure_storage_service.files: BadRequestError
Error for Http Status 400
azure_storage_service.files: ConflictError
Error for Http Status 409
azure_storage_service.files: Error
Super type error returned by connector
azure_storage_service.files: ForbiddenError
Error for Http Status 403
azure_storage_service.files: InternalServerError
Error for Http Status 500
azure_storage_service.files: NoSharesFoundError
Defines an error for ShareNotFound
azure_storage_service.files: NotFoundError
Error for Http Status 404
azure_storage_service.files: ProcessingError
Error created by connector when processing, transforming data
azure_storage_service.files: ServerError
Error created by connector depending on server responses
Union types
azure_storage_service.files: AzureRecord
AzureRecord
The types of records, which support the azure operations.
azure_storage_service.files: URIRecord
URIRecord
Defines the type of URIRecord for ListShareURIParameters, GetDirectoryListURIParameters, GetFileListURIParameters
azure_storage_service.files: ClientError
ClientError
Error created by connector when processing or invoking
Type descriptor types
azure_storage_service.files: AzureRecordType
AzureRecordType
The type description of the nested records.
Import
import ballerinax/azure_storage_service.files;
Metadata
Released date: over 1 year ago
Version: 4.3.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: 2201.4.1
Pull count
Total: 10659
Current verison: 4949
Weekly downloads
Keywords
Content & Files/File Management & Storage
Cost/Paid
Vendor/Microsoft
Contributors