azure_storage_service.blobs
Modules
Module azure_storage_service.blobs
Definitions
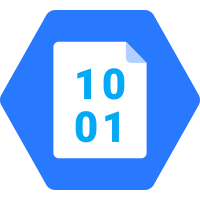
ballerinax/azure_storage_service.blobs Ballerina library
Overview
This module allows you to access the Azure Blob service REST API through Ballerina. The Azure Blob service stores text and binary data as objects in the cloud. The Blob service offers the following three resources: the storage account, containers, and blobs. Within your storage account, containers provide a way to organize sets of blobs. This module provides you the capability to execute operations such as listContainers, listBlobs, putBlob, deleteBlob etc. It also allows you to execute management operations such as createContainer and deleteContainer etc.
This module supports Azure Storage Service REST API 2019-12-12 version.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Obtain
Shared Access Signature
(SAS
) or use one of the Accesskeys for authentication.
Quickstart
To use this connector in your Ballerina application, update the .bal file as follows:
Step1: Import connector
Import the ballerinax/azure_storage_service.blobs
module into the Ballerina project
import ballerinax/azure_storage_service.blobs as azure_blobs;
Step2: Create a new connector instance
Create a azure_blobs:ConnectionConfig
with the obtained Shared Access Signature or Access Key,
base URL and account name.
If you are using Shared Access Signature, use the follwing format.
azure_blobs:ConnectionConfig blobServiceConfig = { accessKeyOrSAS: "ACCESS_KEY_OR_SAS", accountName: "ACCOUNT_NAME", authorizationMethod: "SAS" };
- If you are using one of the Access Key, use the follwing format.
azure_blobs:ConnectionConfig blobServiceConfig = { accessKeyOrSAS: "ACCESS_KEY_OR_SAS", accountName: "ACCOUNT_NAME", authorizationMethod: "accessKey" };
- Create the BlobClient using the blobServiceConfig you have created as shown above.
azure_blobs:BlobClient blobClient = check new (blobServiceConfig);
Step3: Invoke connector operation
- Now you can use the operations available within the connector. Note that they are in the form of remote operations. Following is an example on how to list all the containers using the connector.
public function main() returns error? { azure_blobs:ConnectionConfig blobServiceConfig = { accessKeyOrSAS: os:getEnv("ACCESS_KEY_OR_SAS"), accountName: os:getEnv("ACCOUNT_NAME"), authorizationMethod: "accessKey" }; azure_blobs:BlobClient blobClient = check new (blobServiceConfig); azure_blobs:ListContainerResult result = blobClient->listContainers(); }
- Use
bal run
command to compile and run the Ballerina program.
Quick reference
- Get the list of containers in the storage account
azure_blobs:ListContainerResult result = check blobClient->listContainers();
- Get the list of blobs from a container using container name
azure_blobs:ListBlobResult result = check blobClient->listBlobs("container-1");
- Upload a blob
byte[] testBlob = "hello".toBytes(); map<json> result = check blobClient->putBlob(containerName, "hello.txt", "BlockBlob", testBlob);
- Get a blob using container name and blob name
azure_blobs:BlobResult result = check blobClient->getBlob("container-1", "hello.txt");
- Delete a blob
map<json> deleteBlobResult = check blobClient->deleteBlob("container-1", "hello.txt");
Functions
addAuthorizationHeader
function addAuthorizationHeader(Request request, HttpOperation verb, string accountName, string accessKey, string resourceString, map<string> uriParameters) returns ProcessingError?
Add authentication header to the request if it uses Shared Key Authentication.
Parameters
- request Request - HTTP request
- verb HttpOperation - HTTP verb
- accountName string - Azure account name
- accessKey string - Shared Key
- resourceString string - Resource String
Return Type
- ProcessingError? - Returns error if unsuccessful
generateUriParametersString
Generates URI parameter string from the given map
Return Type
- string - Returns URI Parameter string
getMetaDataHeaders
Get metaData headers from a request.
Parameters
- response Response - HTTP response
preparePath
function preparePath(string authorizationMethod, string accessKeyOrSAS, map<string> uriParameters, string resourcePath) returns string
Create path according to the authorization method.
Parameters
- authorizationMethod string - Authorization method
- accessKeyOrSAS string - Azure Storage account's Access Key or Shared Access Signature
- resourcePath string - Resource path
Return Type
- string - Returns path
setDefaultHeaders
function setDefaultHeaders(Request request)
Add default headers to the request.
Parameters
- request Request - HTTP request
setMetaDataHeaders
Set metadata headers to a request
setOptionalHeaders
Clients
azure_storage_service.blobs: BlobClient
Azure Storage Blob connector allows you to access the Azure Blob service REST API. The Blob service offers the following three resources: the storage account, containers, and blobs. This connector lets you execute operations against the storage account, containers, and blobs.
Constructor
Initializes the connector.
Create an Azure account following this guide.
Create an Azure Storage account following this guide
Obtain Shared Access Signature
(SAS
) or use one of the Accesskeys for authentication.
init (ConnectionConfig config)
- config ConnectionConfig -
listContainers
function listContainers(int? maxResults, string? marker, string? prefix) returns ListContainerResult|Error
Gets list of containers of a storage account.
Parameters
- maxResults int? (default ()) - Optional. Maximum number of containers to return
- marker string? (default ()) - Optional. nextMarker value specified in the previous response
- prefix string? (default ()) - Optional. filters results to return only containers whose name begins with the specified prefix
Return Type
- ListContainerResult|Error - If successful, ListContainerResult. Else an error
listBlobs
function listBlobs(string containerName, int? maxResults, string? marker, string? prefix) returns ListBlobResult|Error
Gets list of blobs (metadata and properties of a blob) from a container.
Parameters
- containerName string - Name of the container
- maxResults int? (default ()) - Optional. Maximum number of containers to return
- marker string? (default ()) - Optional. nextMarker value specified in the previous response
- prefix string? (default ()) - Optional. filters results to return only containers whose name begins with the specified prefix
Return Type
- ListBlobResult|Error - If successful ListBlobResult else an error
getBlob
function getBlob(string containerName, string blobName, ByteRange? byteRange) returns BlobResult|Error
Gets a blob from a container.
Parameters
- containerName string - Name of the container
- blobName string - Name of the blob
- byteRange ByteRange? (default ()) - Optional. The range of the byte to get. If not given, entire blob content will be returned
Return Type
- BlobResult|Error - If successful, blob as a byte array. Else an Error
getBlobMetadata
function getBlobMetadata(string containerName, string blobName) returns BlobMetadataResult|Error
Gets Blob Metadata.
Return Type
- BlobMetadataResult|Error - If successful, Blob Metadata. Else an Error
getBlobProperties
Gets Blob Properties.
getBlockList
function getBlockList(string containerName, string blobName) returns BlockListResult|Error
Gets Block List.
Return Type
- BlockListResult|Error - If successful, Block List. Else an Error
putBlob
function putBlob(string containerName, string blobName, BlobType blobType, byte[] blob, Properties? properties, int? pageBlobLength) returns map<json>|Error
Uploads a blob to a container as a single byte array.
Parameters
- containerName string - Name of the container
- blobName string - Name of the blob
- blobType BlobType - Type of the Blob ("BlockBlob" or "AppendBlob" or "PageBlob")
- blob byte[] (default []) - Blob as a byte[]
- properties Properties? (default ()) - Optional. Additional properties.
- pageBlobLength int? (default ()) - Optional. Length of PageBlob. (Required only for Page Blobs)
putBlobFromURL
function putBlobFromURL(string containerName, string blobName, string sourceBlobURL, Properties? properties) returns map<json>|Error
Puts Blob From URL - creates a new Block Blob where the content of the blob is read from a given URL.
Parameters
- containerName string - Name of the container
- blobName string - Name of the blob
- sourceBlobURL string - Url of source blob
- properties Properties? (default ()) - Optional. Additional properties.
setBlobMetadata
function setBlobMetadata(string containerName, string blobName, map<string> metadata) returns map<json>|Error
Sets Blob Metadata.
deleteBlob
Deletes a blob from a container.
copyBlob
function copyBlob(string containerName, string blobName, string sourceBlobURL) returns CopyBlobResult|Error
Copies a blob from a URL.
Parameters
- containerName string - Name of the container
- blobName string - Name of the blob
- sourceBlobURL string - URL of source blob
Return Type
- CopyBlobResult|Error - If successful, Response Headers. Else an Error
putBlock
function putBlock(string containerName, string blobName, string blockId, byte[] content) returns map<json>|Error
Commits a new block to be commited as part of a blob.
Parameters
- containerName string - Name of the container
- blobName string - Name of the blob
- blockId string - A string value that identifies the block (should be less than 64 bytes in size)
- content byte[] - Blob content
putBlockFromURL
function putBlockFromURL(string containerName, string blobName, string blockId, string sourceBlobURL, ByteRange? byteRange) returns map<json>|Error
Commits a new block to be commited as part of a blob where the content is read from a URL.
Parameters
- containerName string - Name of the container
- blobName string - Name of the blob
- blockId string - A string value that identifies the block (should be less than 64 bytes in size)
- sourceBlobURL string - URL of the source blob
- byteRange ByteRange? (default ()) - Optional. The byte range to get blob content. If not given, entire blob content will be added.
putBlockList
function putBlockList(string containerName, string blobName, string[] blockIdList, Properties? properties) returns map<json>|Error
Writes a blob by specifying the list of blockIDs that make up the blob.
Parameters
- containerName string - Name of the container
- blobName string - Name of the blob
- blockIdList string[] - List of blockIds
- properties Properties? (default ()) - Optional. Additional properties.
putPage
function putPage(string containerName, string pageBlobName, PageOperation operation, ByteRange byteRange, byte[]? content) returns PutPageResult|Error
Updates or adds a new page Blob.
Parameters
- containerName string - Name of the container
- pageBlobName string - Name of the page blob
- operation PageOperation - It can be 'update' or 'clear'
- byteRange ByteRange - Byte range to write
- content byte[]? (default ()) - Blob content
Return Type
- PutPageResult|Error - If successful, Response Headers. Else an Error.
getPageRanges
function getPageRanges(string containerName, string blobName, ByteRange? byteRange) returns PageRangeResult|Error
Gets list of valid page ranges for a page blob.
Parameters
- containerName string - Name of the container
- blobName string - Name of the page blob
- byteRange ByteRange? (default ()) - Optional. The byte range over which to list ranges.
Return Type
- PageRangeResult|Error - If successful, page ranges. Else an Error.
appendBlock
function appendBlock(string containerName, string blobName, byte[] block) returns AppendBlockResult|error
Commits a new block of data to the end of an existing append blob.
Parameters
- containerName string - Name of the container
- blobName string - Name of the append blob
- block byte[] - Content of the block
Return Type
- AppendBlockResult|error - If successful, Response Headers. Else an Error.
appendBlockFromURL
function appendBlockFromURL(string containerName, string blobName, string sourceBlobURL) returns AppendBlockResult|Error
Commits a new block of data (from a URL) to the end of an existing append blob.
Parameters
- containerName string - Name of the container
- blobName string - Name of the append blob
- sourceBlobURL string - URL of the source blob
Return Type
- AppendBlockResult|Error - If successful Response Headers. Else an Error.
uploadLargeBlob
function uploadLargeBlob(string containerName, string blobName, string filePath, Properties? properties) returns error?
Uploads large blob from a file path.
Parameters
- containerName string - name of the container
- blobName string - Name of the blob
- filePath string - Path to the file which should be uploaded
- properties Properties? (default ()) - Optional. Additional properties.
Return Type
- error? - error if unsuccessful
azure_storage_service.blobs: ManagementClient
Azure Storage Blob Management Client Object.
getAccountInformation
function getAccountInformation(string? clientRequestId) returns AccountInformationResult|Error
Get Account Information of the azure storage account.
Parameters
- clientRequestId string? (default ()) - Provides a client-generated, opaque value with a 1 KiB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
Return Type
- AccountInformationResult|Error - If successful, returns AccountInformation. Else returns Error.
createContainer
function createContainer(string containerName, AccessLevel? accessLevel, map<string>? metadata, string? clientRequestId) returns map<json>|Error
Create a container in the azure storage account.
Parameters
- containerName string - Name of the container
- accessLevel AccessLevel? (default ()) - Specifies whether data in the container can be accessed publicly and the level of access
- clientRequestId string? (default ()) - Provides a client-generated, opaque value with a 1 KiB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
deleteContainer
function deleteContainer(string containerName, string? clientRequestId, string? leaseId) returns map<json>|Error
Delete a container from the azure storage account.
Parameters
- containerName string - Name of the container
- clientRequestId string? (default ()) - Provides a client-generated, opaque value with a 1 KiB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
- leaseId string? (default ()) - If the container has an active lease
getContainerProperties
function getContainerProperties(string containerName, string? clientRequestId, string? leaseId) returns ContainerPropertiesResult|Error
Get Container Properties.
Parameters
- containerName string - Name of the container
- clientRequestId string? (default ()) - Provides a client-generated, opaque value with a 1 KiB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
- leaseId string? (default ()) - If the container has an active lease
Return Type
- ContainerPropertiesResult|Error - If successful, returns Container Properties. Else returns Error.
getContainerMetadata
function getContainerMetadata(string containerName, string? clientRequestId, string? leaseId) returns ContainerMetadataResult|Error
Get Container Metadata.
Parameters
- containerName string - Name of the container
- clientRequestId string? (default ()) - Provides a client-generated, opaque value with a 1 KiB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
- leaseId string? (default ()) - If the container has an active lease
Return Type
- ContainerMetadataResult|Error - If successful, returns Container Metadata. Else returns Error.
getContainerACL
function getContainerACL(string containerName, string? clientRequestId, string? leaseId) returns ContainerACLResult|Error
Get Container ACL (gets the permissions for the specified container).
Parameters
- containerName string - Name of the container
- clientRequestId string? (default ()) - Provides a client-generated, opaque value with a 1 KiB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
- leaseId string? (default ()) - If the container has an active lease
Return Type
- ContainerACLResult|Error - If successful, returns container ACL. Else returns Error.
getBlobServiceProperties
function getBlobServiceProperties(string? clientRequestId) returns BlobServicePropertiesResult|Error
Get Blob Service Properties.
Parameters
- clientRequestId string? (default ()) - Provides a client-generated, opaque value with a 1 KiB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
Return Type
- BlobServicePropertiesResult|Error - If successful, returns Blob Service Properties. Else returns Error.
Enums
azure_storage_service.blobs: AccessLevel
Members
azure_storage_service.blobs: AuthorizationMethod
Represents the authorization method used to access azure blob service.
Members
azure_storage_service.blobs: BlobType
Represents the type of a blob.
Members
azure_storage_service.blobs: PageOperation
Represents an operation done on page blob.
Members
Records
azure_storage_service.blobs: AccountInformationResult
Represents Azure Storage Account Information.
Fields
- skuName string - skuName of the specified account
- accountKind string - accountKind of the specified account
- isHNSEnabled string - indicates if the account has a hierarchical namespace enabled
- responseHeaders map<json> - reponse headers and values related to the operation
azure_storage_service.blobs: AppendBlockResult
Represents AppendBlock Result.
Fields
- blobAppendOffset string - Offset at which the block was committed, in bytes
- blobCommitedBlockCount string - The number of committed blocks present in the blob
- eTag string - ETag
- lastModified string - Date/time that the blob was last modified
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: Blob
Represents Azure Storage Blob.
Fields
- Name string - Name of the Blob
- Properties map<json> - Properties of the blob
- Snapshot string? - A date time value
- VersionId string? - A date time value
- IsCurrentVersion string? - if it is the current version or not
- Deleted string? - If it is deleted or not
azure_storage_service.blobs: BlobMetadataResult
Represents Blob Metadata Result.
Fields
- eTag string - ETag
- lastModified string - Date/time that the blob was last modified
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: BlobProperties
Represents Azure Storage Blob Properties.
Fields
- Etag string - Etag
- BlobType string - Type of the blob
- AccessTier string - AccessTier of the blob
- AccessTierInferred string - AccessTierInferred of the blob
- LeaseStatus string - LeaseStatus of the Blob
- LeaseState string - LeaseState of the blob
- ServerEncrypted string - indicates if the server is encrypted
azure_storage_service.blobs: BlobResult
Represents Blob Result.
Fields
- blobContent byte[] - Content of the blob
- responseHeaders map<json> - Response headers from Azure
- properties Properties - Blob properties
azure_storage_service.blobs: BlobServicePropertiesResult
Represents Blob Service Properties Result.
Fields
- storageServiceProperties json - Storage Service properties
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: BlockListResult
Represents Block List Result.
Fields
- blockList json - List of Blocks
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: ByteRange
Represents Byte Range of a blob
Fields
- startByte int - From which byte
- endByte int - Upto which byte
azure_storage_service.blobs: ConnectionConfig
Azure Blob Service Configuration.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth never? -
- accessKeyOrSAS string - Access key or Shared Access Signature for Azure Storage Account
- accountName string - Name of the Azure Storage account
- authorizationMethod AuthorizationMethod - Holds the used authorization method from the enum AuthorizationMethod
- httpVersion HttpVersion(default http:HTTP_1_1) - The HTTP version understood by the client
azure_storage_service.blobs: Container
Represents Azure Storage Container.
Fields
- Name string - The name of the container
- Properties map<json> - Properties of the container
- Version string? - Container version
- Deleted string? - Is the container deleted
- Metadata map<json>? - Container Metadata
azure_storage_service.blobs: ContainerACLResult
Represents Container ACL Result.
Fields
- signedIdentifiers json? - Signed Identifiers
- publicAccess string? - Public access of container
- eTag string - ETag
- lastModified string - Date/time that the blob was last modified
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: ContainerMetadataResult
Represents Container Metadata Result.
Fields
- eTag string - ETag
- lastModified string - Date/time that the blob was last modified
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: ContainerPropertiesResult
Represents Container Properties Result.
Fields
- eTag string - ETag
- lastModified string - Date/time that the blob was last modified
- leaseStatus string - Lease status of the container
- leaseState string - Lease state of the container
- leaseDuration string? - Lease duration of the container
- publicAccess string? - Public access of the container
- hasImmutabilityPolicy string - If it has immutability policy
- hasLegalHold string - If it has legal hold
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: CopyBlobResult
Represents Copy Blob Result.
Fields
- copyId string - String identifier for this copy operation
- copyStatus string - State of the copy operation
- lastModified string - Date/time that the blob was last modified
- eTag string - ETag
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: ListBlobResult
Represents List Blob Result.
Fields
- blobList Blob[] - List of Blobs
- nextMarker string - Offset value
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: ListContainerResult
Represents List Container Result.
Fields
- containerList Container[] - List of Containers
- nextMarker string - Offset value
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: PageRangeResult
Represents Page Range Result.
Fields
- pageList json - List of page ranges
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: Properties
Represents additional properties of a blob.
Fields
- blobContentType string? - Blob Content Type
- blobContentEncoding string? - Blob Content Encoding
- blobContentMd5 string? - Blob Content MD5
azure_storage_service.blobs: PutPageResult
Represents PutPage Result.
Fields
- blobSequenceNumber string - The current sequence number for the page blob
- eTag string - ETag
- lastModified string - Date/time that the blob was last modified
- responseHeaders map<json> - Response headers from Azure
azure_storage_service.blobs: ServerErrorDetail
Defines the details of an error message.
Fields
- httpStatus int - HTTP status code associated with the error
- errorCode string - Azure Blob Storage error code
- message string - Associated error message
Errors
azure_storage_service.blobs: BadRequestError
Error for Http Status 400
azure_storage_service.blobs: ClientError
Error created by connector when processing or invoking
azure_storage_service.blobs: ConflictError
Error for Http Status 409
azure_storage_service.blobs: Error
Super type error returned by connector
azure_storage_service.blobs: ForbiddenError
Error for Http Status 403
azure_storage_service.blobs: InternalServerError
Error for Http Status 500
azure_storage_service.blobs: NotFoundError
Error for Http Status 404
azure_storage_service.blobs: PreconditionFailedError
Error types as per https://learn.microsoft.com/en-us/rest/api/storageservices/blob-service-error-codes Error for Http Status 412
azure_storage_service.blobs: ProcessingError
Error created by connector when processing, transforming data
azure_storage_service.blobs: RequestedRangeNotSatisfiableError
Error for Http Status 416
azure_storage_service.blobs: ServerError
Error created by connector depending on server responses
Import
import ballerinax/azure_storage_service.blobs;
Metadata
Released date: over 1 year ago
Version: 4.1.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: 2201.3.1
Pull count
Total: 10717
Current verison: 285
Weekly downloads
Keywords
Content & Files/File Management & Storage
Cost/Paid
Vendor/Microsoft
Contributors