azure.textanalytics
Module azure.textanalytics
API
Definitions
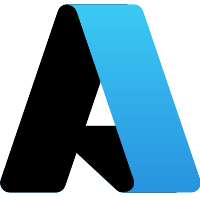
ballerinax/azure.textanalytics Ballerina library
Overview
This is a generated connector for Azure Text Analytics API v1 OpenAPI specification. The Text Analytics API is a suite of natural language processing (NLP) services built with best-in-class Microsoft machine learning algorithms. The API can be used to analyze unstructured text for tasks such as sentiment analysis, key phrase extraction and language detection. Functionality for analysis of text specific to the healthcare domain and personal information are also available in the API. Further documentation can be found here
This module supports Azure Text Analytics API v1.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Create an Azure account
-
Create a Text Analysis resource
-
Obtain tokens by following this guide
Quickstart
To use the Azure Text Analytics connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/azure.textanalytics
module into the Ballerina project.
import ballerinax/azure.textanalytics;
Step 2: Create a new connector instance
You can now make the connection configuration using Ocp-Apim-Subscription-Key
.
You can do this step in two ways. You can use any one of this.
-
Option 1 : Configure API Keys in ballerina file directly.
nalytics:ApiKeysConfig apiKeyConfig = { cpApimSubscriptionKey:"<AZURE_API_KEY>" ubscriptionKey: "<Subscription Key>" nalytics:Client myClient = check new Client(apiKeyConfig, serviceUrl = "https://<REGION>.api.cognitive.microsoft.com/text/analytics/v3.1");
-
Option 2 : Configure API Keys in
Config.toml
file and configure it in ballerina file, using configurables.- Set up API Keys in
Config.toml
as shown below.
[apiKeyConfig] ocpApimSubscriptionKey = "<AZURE_API_KEY>" subscriptionKey = "<Subscription Key>"
- Configure the client in ballerina file as shown below.
configurable ApiKeysConfig & readonly apiKeyConfig = ?; textanalytics:Client myClient = check new Client(apiKeyConfig, serviceUrl = "https://<REGION>.api.cognitive.microsoft.com/text/analytics/v3.1");
- Set up API Keys in
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to use sentiment prediction. The API returns a sentiment prediction, as well as sentiment scores for each sentiment class (Positive, Negative, and Neutral) for the document and each sentence within it.
Sentiment Prediction
public function main() returns error? { textanalytics:MultiLanguageBatchInput payload = { "documents": [ { "id": "string", "text": "string", "language": "string" } ]}; textanalytics:SentimentResponse res = check baseClient->sentiment(payload); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
azure.textanalytics: Client
This is a generated connector for Azure Text Analytics API v1 OpenAPI specification. The Text Analytics API is a suite of natural language processing (NLP) services built with best-in-class Microsoft machine learning algorithms. The API can be used to analyze unstructured text for tasks such as sentiment analysis, key phrase extraction and language detection. Functionality for analysis of text specific to the healthcare domain and personal information are also available in the API. Further documentation can be found in https://docs.microsoft.com/en-us/azure/cognitive-services/text-analytics/overview
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Azure Text Analytics account and obtain API key following this guide.
init (ApiKeysConfig apiKeyConfig, string serviceUrl, ConnectionConfig config)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- serviceUrl string - URL of the target service
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
healthStatus
function healthStatus(string jobId, int top, int skip, boolean? showStats) returns HealthcareJobState|error
Get healthcare analysis job status and results
Parameters
- jobId string - Format - uuid. Job ID
- top int (default 20) - (Optional) Set the maximum number of results per task. When both $top and $skip are specified, $skip is applied first.
- skip int (default 0) - (Optional) Set the number of elements to offset in the response. When both $top and $skip are specified, $skip is applied first.
- showStats boolean? (default ()) - (Optional) if set to true, response will contain request and document level statistics.
Return Type
- HealthcareJobState|error - OK
cancelHealthJob
Cancel healthcare prediction job
Parameters
- jobId string - Format - uuid. Job ID
languages
function languages(LanguageBatchInput payload, string? modelVersion, boolean? showStats, boolean? loggingOptOut) returns LanguageResult|error
Detect Language
Parameters
- payload LanguageBatchInput - Collection of documents to analyze for language endpoint.
- modelVersion string? (default ()) - (Optional) This value indicates which model will be used for scoring. If a model-version is not specified, the API should default to the latest, non-preview version.
- showStats boolean? (default ()) - (Optional) if set to true, response will contain request and document level statistics.
- loggingOptOut boolean? (default ()) - (Optional) If set to true, you opt-out of having your text input logged for troubleshooting. By default, Text Analytics logs your input text for 48 hours, solely to allow for troubleshooting issues in providing you with the Text Analytics natural language processing functions. Setting this parameter to true, disables input logging and may limit our ability to remediate issues that occur. Please see Cognitive Services Compliance and Privacy notes at https://aka.ms/cs-compliance for additional details, and Microsoft Responsible AI principles at https://www.microsoft.com/en-us/ai/responsible-ai.
Return Type
- LanguageResult|error - A successful call results in the detected language with the highest probability for each valid document
entitiesRecognitionPii
function entitiesRecognitionPii(MultiLanguageBatchInput payload, string? modelVersion, boolean? showStats, boolean? loggingOptOut, string? domain, string stringIndexType, string[]? piiCategories) returns PiiResult|error
Entities containing personal information
Parameters
- payload MultiLanguageBatchInput - Collection of documents to analyze.
- modelVersion string? (default ()) - (Optional) This value indicates which model will be used for scoring. If a model-version is not specified, the API should default to the latest, non-preview version.
- showStats boolean? (default ()) - (Optional) if set to true, response will contain request and document level statistics.
- loggingOptOut boolean? (default ()) - (Optional) If set to true, you opt-out of having your text input logged for troubleshooting. By default, Text Analytics logs your input text for 48 hours, solely to allow for troubleshooting issues in providing you with the Text Analytics natural language processing functions. Setting this parameter to true, disables input logging and may limit our ability to remediate issues that occur. Please see Cognitive Services Compliance and Privacy notes at https://aka.ms/cs-compliance for additional details, and Microsoft Responsible AI principles at https://www.microsoft.com/en-us/ai/responsible-ai.
- domain string? (default ()) - (Optional) if specified, will set the PII domain to include only a subset of the entity categories. Possible values include: 'PHI', 'none'.
- stringIndexType string (default "TextElement_v8") - (Optional) Specifies the method used to interpret string offsets. Defaults to Text Elements (Graphemes) according to Unicode v8.0.0. For additional information see https://aka.ms/text-analytics-offsets
- piiCategories string[]? (default ()) - (Optional) describes the PII categories to return
Return Type
analyzeStatus
function analyzeStatus(string jobId, boolean? showStats, int top, int skip) returns AnalyzeJobState|error
Get analysis status and results
Parameters
- jobId string - Job ID for Analyze
- showStats boolean? (default ()) - (Optional) if set to true, response will contain request and document level statistics.
- top int (default 20) - (Optional) Set the maximum number of results per task. When both $top and $skip are specified, $skip is applied first.
- skip int (default 0) - (Optional) Set the number of elements to offset in the response. When both $top and $skip are specified, $skip is applied first.
Return Type
- AnalyzeJobState|error - Analysis job status and metadata.
keyPhrases
function keyPhrases(MultiLanguageBatchInput payload, string? modelVersion, boolean? showStats, boolean? loggingOptOut) returns KeyPhraseResult|error
Key Phrases
Parameters
- payload MultiLanguageBatchInput - Collection of documents to analyze.
- modelVersion string? (default ()) - (Optional) This value indicates which model will be used for scoring. If a model-version is not specified, the API should default to the latest, non-preview version.
- showStats boolean? (default ()) - (Optional) if set to true, response will contain request and document level statistics.
- loggingOptOut boolean? (default ()) - (Optional) If set to true, you opt-out of having your text input logged for troubleshooting. By default, Text Analytics logs your input text for 48 hours, solely to allow for troubleshooting issues in providing you with the Text Analytics natural language processing functions. Setting this parameter to true, disables input logging and may limit our ability to remediate issues that occur. Please see Cognitive Services Compliance and Privacy notes at https://aka.ms/cs-compliance for additional details, and Microsoft Responsible AI principles at https://www.microsoft.com/en-us/ai/responsible-ai.
Return Type
- KeyPhraseResult|error - A successful response results in 0 or more key phrases identified in each valid document
entitiesLinking
function entitiesLinking(MultiLanguageBatchInput payload, string? modelVersion, boolean? showStats, boolean? loggingOptOut, string stringIndexType) returns EntityLinkingResult|error
Linked entities from a well known knowledge base
Parameters
- payload MultiLanguageBatchInput - Collection of documents to analyze.
- modelVersion string? (default ()) - (Optional) This value indicates which model will be used for scoring. If a model-version is not specified, the API should default to the latest, non-preview version.
- showStats boolean? (default ()) - (Optional) if set to true, response will contain request and document level statistics.
- loggingOptOut boolean? (default ()) - (Optional) If set to true, you opt-out of having your text input logged for troubleshooting. By default, Text Analytics logs your input text for 48 hours, solely to allow for troubleshooting issues in providing you with the Text Analytics natural language processing functions. Setting this parameter to true, disables input logging and may limit our ability to remediate issues that occur. Please see Cognitive Services Compliance and Privacy notes at https://aka.ms/cs-compliance for additional details, and Microsoft Responsible AI principles at https://www.microsoft.com/en-us/ai/responsible-ai.
- stringIndexType string (default "TextElement_v8") - (Optional) Specifies the method used to interpret string offsets. Defaults to Text Elements (Graphemes) according to Unicode v8.0.0. For additional information see https://aka.ms/text-analytics-offsets
Return Type
- EntityLinkingResult|error - A successful call results in a list of recognized entities with links to a well known knowledge base returned for each valid document
entitiesRecognitionGeneral
function entitiesRecognitionGeneral(MultiLanguageBatchInput payload, string? modelVersion, boolean? showStats, boolean? loggingOptOut, string stringIndexType) returns EntitiesResult|error
Named Entity Recognition
Parameters
- payload MultiLanguageBatchInput - Collection of documents to analyze.
- modelVersion string? (default ()) - (Optional) This value indicates which model will be used for scoring. If a model-version is not specified, the API should default to the latest, non-preview version.
- showStats boolean? (default ()) - (Optional) if set to true, response will contain request and document level statistics.
- loggingOptOut boolean? (default ()) - (Optional) If set to true, you opt-out of having your text input logged for troubleshooting. By default, Text Analytics logs your input text for 48 hours, solely to allow for troubleshooting issues in providing you with the Text Analytics natural language processing functions. Setting this parameter to true, disables input logging and may limit our ability to remediate issues that occur. Please see Cognitive Services Compliance and Privacy notes at https://aka.ms/cs-compliance for additional details, and Microsoft Responsible AI principles at https://www.microsoft.com/en-us/ai/responsible-ai.
- stringIndexType string (default "TextElement_v8") - (Optional) Specifies the method used to interpret string offsets. Defaults to Text Elements (Graphemes) according to Unicode v8.0.0. For additional information see https://aka.ms/text-analytics-offsets
Return Type
- EntitiesResult|error - A successful call results in a list of recognized entities returned for each valid document.
sentiment
function sentiment(MultiLanguageBatchInput payload, string? modelVersion, boolean? showStats, boolean? loggingOptOut, boolean? opinionMining, string stringIndexType) returns SentimentResponse|error
Sentiment
Parameters
- payload MultiLanguageBatchInput - Collection of documents to analyze.
- modelVersion string? (default ()) - (Optional) This value indicates which model will be used for scoring. If a model-version is not specified, the API should default to the latest, non-preview version.
- showStats boolean? (default ()) - (Optional) if set to true, response will contain request and document level statistics.
- loggingOptOut boolean? (default ()) - (Optional) If set to true, you opt-out of having your text input logged for troubleshooting. By default, Text Analytics logs your input text for 48 hours, solely to allow for troubleshooting issues in providing you with the Text Analytics natural language processing functions. Setting this parameter to true, disables input logging and may limit our ability to remediate issues that occur. Please see Cognitive Services Compliance and Privacy notes at https://aka.ms/cs-compliance for additional details, and Microsoft Responsible AI principles at https://www.microsoft.com/en-us/ai/responsible-ai.
- opinionMining boolean? (default ()) - (Optional) if set to true, response will contain not only sentiment prediction but also opinion mining (aspect-based sentiment analysis) results.
- stringIndexType string (default "TextElement_v8") - (Optional) Specifies the method used to interpret string offsets. Defaults to Text Elements (Graphemes) according to Unicode v8.0.0. For additional information see https://aka.ms/text-analytics-offsets
Return Type
- SentimentResponse|error - A successful call results in a document sentiment prediction, as well as sentiment scores for each sentiment class (Positive, Negative, and Neutral)
analyze
function analyze(AnalyzeBatchInput payload) returns Response|error
Submit analysis job
Parameters
- payload AnalyzeBatchInput - Collection of documents to analyze and tasks to execute.
Return Type
health
function health(MultiLanguageBatchInput payload, string? modelVersion, string stringIndexType, boolean? loggingOptOut) returns Response|error
Submit healthcare analysis job
Parameters
- payload MultiLanguageBatchInput - Collection of documents to analyze.
- modelVersion string? (default ()) - (Optional) This value indicates which model will be used for scoring. If a model-version is not specified, the API should default to the latest, non-preview version.
- stringIndexType string (default "TextElement_v8") - (Optional) Specifies the method used to interpret string offsets. Defaults to Text Elements (Graphemes) according to Unicode v8.0.0. For additional information see https://aka.ms/text-analytics-offsets
- loggingOptOut boolean? (default ()) - (Optional) If set to true, you opt-out of having your text input logged for troubleshooting. By default, Text Analytics logs your input text for 48 hours, solely to allow for troubleshooting issues in providing you with the Text Analytics natural language processing functions. Setting this parameter to true, disables input logging and may limit our ability to remediate issues that occur. Please see Cognitive Services Compliance and Privacy notes at https://aka.ms/cs-compliance for additional details, and Microsoft Responsible AI principles at https://www.microsoft.com/en-us/ai/responsible-ai.
Records
azure.textanalytics: AnalysisInput
Fields
- analysisInput MultiLanguageBatchInput - Contains a set of input documents to be analyzed by the service.
azure.textanalytics: AnalyzeBatchInput
Fields
- Fields Included from *JobDescriptor
- displayName string
- anydata...
- Fields Included from *AnalysisInput
- analysisInput MultiLanguageBatchInput
- anydata...
- Fields Included from *JobManifest
- tasks JobmanifestTasks
- anydata...
azure.textanalytics: AnalyzeJobDisplayName
Fields
- displayName string? -
azure.textanalytics: AnalyzeJobErrorsAndStatistics
Fields
- errors TextAnalyticsError[]? -
- statistics RequestStatistics? - if showStats=true was specified in the request this field will contain information about the request payload.
azure.textanalytics: AnalyzeJobMetadata
Fields
- Fields Included from *JobMetadata
- Fields Included from *AnalyzeJobDisplayName
- displayName string
- anydata...
azure.textanalytics: AnalyzeJobState
Fields
- Fields Included from *AnalyzeJobMetadata
- Fields Included from *TasksState
- tasks TasksstateTasks
- anydata...
- Fields Included from *AnalyzeJobErrorsAndStatistics
- errors TextAnalyticsError[]
- statistics RequestStatistics
- anydata...
- Fields Included from *Pagination
- \@nextLink string
- anydata...
azure.textanalytics: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- ocpApimSubscriptionKey string - Represents API Key
Ocp-Apim-Subscription-Key
- subscriptionKey string - Represents API Key
subscription-key
azure.textanalytics: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
azure.textanalytics: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
azure.textanalytics: DetectedLanguage
Fields
- name string - Long name of a detected language (e.g. English, French).
- iso6391Name string - A two letter representation of the detected language according to the ISO 639-1 standard (e.g. en, fr).
- confidenceScore decimal - A confidence score between 0 and 1. Scores close to 1 indicate 100% certainty that the identified language is true.
azure.textanalytics: DocumentEntities
Fields
- id string - Unique, non-empty document identifier.
- entities Entity[] - Recognized entities in the document.
- warnings TextAnalyticsWarning[] - Warnings encountered while processing document.
- statistics DocumentStatistics? - if showStats=true was specified in the request this field will contain information about the document payload.
azure.textanalytics: DocumentError
Fields
- id string - Document Id.
- 'error TextAnalyticsError -
azure.textanalytics: DocumentHealthcareEntities
Fields
- id string - Unique, non-empty document identifier.
- entities HealthcareEntity[] - Healthcare entities.
- relations HealthcareRelation[] - Healthcare entity relations.
- warnings TextAnalyticsWarning[] - Warnings encountered while processing document.
- statistics DocumentStatistics? - if showStats=true was specified in the request this field will contain information about the document payload.
azure.textanalytics: DocumentKeyPhrases
Fields
- id string - Unique, non-empty document identifier.
- keyPhrases string[] - A list of representative words or phrases. The number of key phrases returned is proportional to the number of words in the input document.
- warnings TextAnalyticsWarning[] - Warnings encountered while processing document.
- statistics DocumentStatistics? - if showStats=true was specified in the request this field will contain information about the document payload.
azure.textanalytics: DocumentLanguage
Fields
- id string - Unique, non-empty document identifier.
- detectedLanguage DetectedLanguage -
- warnings TextAnalyticsWarning[] - Warnings encountered while processing document.
- statistics DocumentStatistics? - if showStats=true was specified in the request this field will contain information about the document payload.
azure.textanalytics: DocumentLinkedEntities
Fields
- id string - Unique, non-empty document identifier.
- entities LinkedEntity[] - Recognized well known entities in the document.
- warnings TextAnalyticsWarning[] - Warnings encountered while processing document.
- statistics DocumentStatistics? - if showStats=true was specified in the request this field will contain information about the document payload.
azure.textanalytics: DocumentSentiment
Fields
- id string - Unique, non-empty document identifier.
- sentiment string - Predicted sentiment for document (Negative, Neutral, Positive, or Mixed).
- statistics DocumentStatistics? - if showStats=true was specified in the request this field will contain information about the document payload.
- confidenceScores SentimentConfidenceScorePerLabel - Represents the confidence scores between 0 and 1 across all sentiment classes: positive, neutral, negative.
- sentences SentenceSentiment[] - Sentence level sentiment analysis.
- warnings TextAnalyticsWarning[] - Warnings encountered while processing document.
azure.textanalytics: DocumentStatistics
if showStats=true was specified in the request this field will contain information about the document payload.
Fields
- charactersCount int - Number of text elements recognized in the document.
- transactionsCount int - Number of transactions for the document.
azure.textanalytics: EntitiesResult
Fields
- documents DocumentEntities[] - Response by document
- errors DocumentError[] - Errors by document id.
- statistics RequestStatistics? - if showStats=true was specified in the request this field will contain information about the request payload.
- modelVersion string - This field indicates which model is used for scoring.
azure.textanalytics: EntitiesTask
Fields
- parameters EntitiestaskParameters? -
- taskName string? -
azure.textanalytics: EntitiestaskParameters
Fields
- 'model\-version string? -
- loggingOptOut boolean? -
- stringIndexType StringIndexType? -
azure.textanalytics: EntitiesTaskResult
Fields
- results EntitiesResult? -
azure.textanalytics: Entity
Fields
- text string - Entity text as appears in the request.
- category string - Entity type.
- subcategory string? - (Optional) Entity sub type.
- offset int - Start position for the entity text. Use of different 'stringIndexType' values can affect the offset returned.
- length int - Length for the entity text. Use of different 'stringIndexType' values can affect the length returned.
- confidenceScore decimal - Confidence score between 0 and 1 of the extracted entity.
azure.textanalytics: EntityLinkingResult
Fields
- documents DocumentLinkedEntities[] - Response by document
- errors DocumentError[] - Errors by document id.
- statistics RequestStatistics? - if showStats=true was specified in the request this field will contain information about the request payload.
- modelVersion string - This field indicates which model is used for scoring.
azure.textanalytics: EntityLinkingTask
Fields
- parameters EntitiestaskParameters? -
- taskName string? -
azure.textanalytics: EntityLinkingTaskResult
Fields
- results EntityLinkingResult? -
azure.textanalytics: ErrorResponse
Fields
- 'error TextAnalyticsError -
azure.textanalytics: HealthcareAssertion
Fields
- conditionality string? - Describes any conditionality on the entity.
- certainty string? - Describes the entities certainty and polarity.
- association string? - Describes if the entity is the subject of the text or if it describes someone else.
azure.textanalytics: HealthcareEntity
Fields
- text string - Entity text as appears in the request.
- category string - Healthcare Entity Category.
- subcategory string? - (Optional) Entity sub type.
- offset int - Start position for the entity text. Use of different 'stringIndexType' values can affect the offset returned.
- length int - Length for the entity text. Use of different 'stringIndexType' values can affect the length returned.
- confidenceScore decimal - Confidence score between 0 and 1 of the extracted entity.
- Fields Included from *HealthcareLinkingProperties
- assertion HealthcareAssertion
- name string
- links HealthcareEntityLink[]
- anydata...
azure.textanalytics: HealthcareEntityLink
Fields
- dataSource string - Entity Catalog. Examples include: UMLS, CHV, MSH, etc.
- id string - Entity id in the given source catalog.
azure.textanalytics: HealthcareJobState
Fields
- Fields Included from *JobMetadata
- Fields Included from *HealthcareTaskResult
- results HealthcareResult
- errors TextAnalyticsError[]
- anydata...
- Fields Included from *Pagination
- \@nextLink string
- anydata...
azure.textanalytics: HealthcareLinkingProperties
Fields
- assertion HealthcareAssertion? -
- name string? - Preferred name for the entity. Example: 'histologically' would have a 'name' of 'histologic'.
- links HealthcareEntityLink[]? - Entity references in known data sources.
azure.textanalytics: HealthcareRelation
Every relation is an entity graph of a certain relationType, where all entities are connected and have specific roles within the relation context.
Fields
- relationType string - Type of relation. Examples include:
DosageOfMedication
or 'FrequencyOfMedication', etc.
- entities HealthcareRelationEntity[] - The entities in the relation.
azure.textanalytics: HealthcareRelationEntity
Fields
- ref string - Reference link object, using a JSON pointer RFC 6901 (URI Fragment Identifier Representation), pointing to the entity .
- role string - Role of entity in the relationship. For example: 'CD20-positive diffuse large B-cell lymphoma' has the following entities with their roles in parenthesis: CD20 (GeneOrProtein), Positive (Expression), diffuse large B-cell lymphoma (Diagnosis).
azure.textanalytics: HealthcareResult
Fields
- documents DocumentHealthcareEntities[] - Response by document
- errors DocumentError[] - Errors by document id.
- statistics RequestStatistics? - if showStats=true was specified in the request this field will contain information about the request payload.
- modelVersion string - This field indicates which model is used for scoring.
azure.textanalytics: HealthcareTaskResult
Fields
- results HealthcareResult? -
- errors TextAnalyticsError[]? -
azure.textanalytics: InnerError
Fields
- code string - Error code.
- message string - Error message.
- details record {}? - Error details.
- target string? - Error target.
- innererror InnerError? -
azure.textanalytics: JobDescriptor
Fields
- displayName string? - Optional display name for the analysis job.
azure.textanalytics: JobManifest
Fields
- tasks JobmanifestTasks - The set of tasks to execute on the input documents. Cannot specify the same task more than once.
azure.textanalytics: JobmanifestTasks
The set of tasks to execute on the input documents. Cannot specify the same task more than once.
Fields
- entityRecognitionTasks EntitiesTask[]? - Array of EntityRecognitionTasks records
- entityRecognitionPiiTasks PiiTask[]? - Array of EntityRecognitionPiiTasks records
- keyPhraseExtractionTasks KeyPhrasesTask[]? - Array of EntityRecognitionTasks records
- entityLinkingTasks EntityLinkingTask[]? - Array of EntityLinkingTasks records
- sentimentAnalysisTasks SentimentAnalysisTask[]? - Array of SentimentAnalysisTasks records
azure.textanalytics: JobMetadata
Fields
- createdDateTime string -
- expirationDateTime string? -
- jobId string -
- lastUpdateDateTime string -
- status string -
azure.textanalytics: KeyPhraseResult
Fields
- documents DocumentKeyPhrases[] - Response by document
- errors DocumentError[] - Errors by document id.
- statistics RequestStatistics? - if showStats=true was specified in the request this field will contain information about the request payload.
- modelVersion string - This field indicates which model is used for scoring.
azure.textanalytics: KeyPhrasesTask
Fields
- parameters KeyphrasestaskParameters? -
- taskName string? -
azure.textanalytics: KeyphrasestaskParameters
Fields
- 'model\-version string? -
- loggingOptOut boolean? -
azure.textanalytics: KeyPhraseTaskResult
Fields
- results KeyPhraseResult? -
azure.textanalytics: LanguageBatchInput
Fields
- documents LanguageInput[] -
azure.textanalytics: LanguageInput
Fields
- id string - Unique, non-empty document identifier.
- text string -
- countryHint string? -
azure.textanalytics: LanguageResult
Fields
- documents DocumentLanguage[] - Response by document
- errors DocumentError[] - Errors by document id.
- statistics RequestStatistics? - if showStats=true was specified in the request this field will contain information about the request payload.
- modelVersion string - This field indicates which model is used for scoring.
azure.textanalytics: LinkedEntity
Fields
- name string - Entity Linking formal name.
- matches Match[] - List of instances this entity appears in the text.
- language string - Language used in the data source.
- id string? - Unique identifier of the recognized entity from the data source.
- url string - URL for the entity's page from the data source.
- dataSource string - Data source used to extract entity linking, such as Wiki/Bing etc.
- bingId string? - Bing Entity Search API unique identifier of the recognized entity.
azure.textanalytics: Match
Fields
- confidenceScore decimal - If a well known item is recognized, a decimal number denoting the confidence level between 0 and 1 will be returned.
- text string - Entity text as appears in the request.
- offset int - Start position for the entity match text.
- length int - Length for the entity match text.
azure.textanalytics: MultiLanguageBatchInput
Contains a set of input documents to be analyzed by the service.
Fields
- documents MultiLanguageInput[] - The set of documents to process as part of this batch.
azure.textanalytics: MultiLanguageInput
Contains an input document to be analyzed by the service.
Fields
- id string - A unique, non-empty document identifier.
- text string - The input text to process.
- language string? - (Optional) This is the 2 letter ISO 639-1 representation of a language. For example, use "en" for English; "es" for Spanish etc. If not set, use "en" for English as default.
azure.textanalytics: Pagination
Fields
- '\@nextLink string? -
azure.textanalytics: PiiDocumentEntities
Fields
- id string - Unique, non-empty document identifier.
- redactedText string - Returns redacted text.
- entities Entity[] - Recognized entities in the document.
- warnings TextAnalyticsWarning[] - Warnings encountered while processing document.
- statistics DocumentStatistics? - if showStats=true was specified in the request this field will contain information about the document payload.
azure.textanalytics: PiiResult
Fields
- documents PiiDocumentEntities[] - Response by document
- errors DocumentError[] - Errors by document id.
- statistics RequestStatistics? - if showStats=true was specified in the request this field will contain information about the request payload.
- modelVersion string - This field indicates which model is used for scoring.
azure.textanalytics: PiiTask
Fields
- parameters PiitaskParameters? -
- taskName string? -
azure.textanalytics: PiitaskParameters
Fields
- domain string? -
- 'model\-version string? -
- loggingOptOut boolean? -
- piiCategories PiiCategories? - (Optional) describes the PII categories to return
- stringIndexType StringIndexType? -
azure.textanalytics: PiiTaskResult
Fields
- results PiiResult? -
azure.textanalytics: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
azure.textanalytics: RequestStatistics
if showStats=true was specified in the request this field will contain information about the request payload.
Fields
- documentsCount int - Number of documents submitted in the request.
- validDocumentsCount int - Number of valid documents. This excludes empty, over-size limit or non-supported languages documents.
- erroneousDocumentsCount int - Number of invalid documents. This includes empty, over-size limit or non-supported languages documents.
- transactionsCount int - Number of transactions for the request.
azure.textanalytics: SentenceAssessment
Fields
- sentiment string - Assessment sentiment in the sentence.
- confidenceScores TargetConfidenceScoreLabel - Represents the confidence scores across all sentiment classes: positive, neutral, negative.
- offset int - The assessment offset from the start of the sentence.
- length int - The length of the assessment.
- text string - The assessment text detected.
- isNegated boolean - The indicator representing if the assessment is negated.
azure.textanalytics: SentenceSentiment
Fields
- text string - The sentence text.
- sentiment string - The predicted Sentiment for the sentence.
- confidenceScores SentimentConfidenceScorePerLabel - Represents the confidence scores between 0 and 1 across all sentiment classes: positive, neutral, negative.
- offset int - The sentence offset from the start of the document.
- length int - The length of the sentence.
- targets SentenceTarget[]? - The array of sentence targets for the sentence.
- assessments SentenceAssessment[]? - The array of assessments for the sentence.
azure.textanalytics: SentenceTarget
Fields
- sentiment string - Targeted sentiment in the sentence.
- confidenceScores TargetConfidenceScoreLabel - Represents the confidence scores across all sentiment classes: positive, neutral, negative.
- offset int - The target offset from the start of the sentence.
- length int - The length of the target.
- text string - The target text detected.
- relations TargetRelation[] - The array of either assessment or target objects which is related to the target.
azure.textanalytics: SentimentAnalysisTask
Fields
- parameters SentimentanalysistaskParameters? -
- taskName string? -
azure.textanalytics: SentimentanalysistaskParameters
Fields
- 'model\-version string? -
- loggingOptOut boolean? -
- opinionMining boolean? -
- stringIndexType StringIndexType? -
azure.textanalytics: SentimentConfidenceScorePerLabel
Represents the confidence scores between 0 and 1 across all sentiment classes: positive, neutral, negative.
Fields
- positive decimal - Positive value
- neutral decimal - Nuetral value
- negative decimal - Negative value
azure.textanalytics: SentimentResponse
Fields
- documents DocumentSentiment[] - Sentiment analysis per document.
- errors DocumentError[] - Errors by document id.
- statistics RequestStatistics? - if showStats=true was specified in the request this field will contain information about the request payload.
- modelVersion string - This field indicates which model is used for scoring.
azure.textanalytics: SentimentTaskResult
Fields
- results SentimentResponse? -
azure.textanalytics: TargetConfidenceScoreLabel
Represents the confidence scores across all sentiment classes: positive, neutral, negative.
Fields
- positive decimal - Positive
- negative decimal - Negative
azure.textanalytics: TargetRelation
Fields
- relationType string - The type related to the target.
- ref string - The JSON pointer indicating the linked object.
azure.textanalytics: TasksState
Fields
- tasks TasksstateTasks -
azure.textanalytics: TasksstateTasks
Fields
- completed int -
- failed int -
- inProgress int -
- total int -
- entityRecognitionTasks record {}[]? -
- entityRecognitionPiiTasks record {}[]? -
- keyPhraseExtractionTasks record {}[]? -
- entityLinkingTasks record {}[]? -
- sentimentAnalysisTasks record {}[]? -
azure.textanalytics: TaskState
Fields
- lastUpdateDateTime string -
- taskName string? -
- status string -
azure.textanalytics: TextAnalyticsError
Fields
- code string - Error code.
- message string - Error message.
- target string? - Error target.
- innererror InnerError? -
- details TextAnalyticsError[]? - Details about specific errors that led to this reported error.
azure.textanalytics: TextAnalyticsWarning
Fields
- code string - Error code.
- message string - Warning message.
- targetRef string? - A JSON pointer reference indicating the target object.
String types
azure.textanalytics: StringIndexType
StringIndexType
Import
import ballerinax/azure.textanalytics;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 45
Current verison: 1
Weekly downloads
Keywords
IT Operations/Cloud Services
Cost/Paid
Vendor/Microsoft
Contributors
Dependencies