azure.qnamaker
Module azure.qnamaker
Definitions
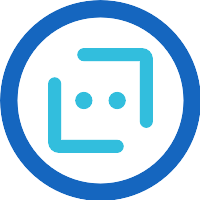
ballerinax/azure.qnamaker Ballerina library
Overview
This is a generated connector for Azure QnA Maker API v4 OpenAPI specification. The Azure QnA Maker API is a cloud-based Natural Language Processing (NLP) service that allows you to create a natural conversational layer over your data. It is used to find the most appropriate answer for any input from your custom knowledge base (KB) of information. QnA Maker is commonly used to build conversational client applications, which include social media applications, chat bots, and speech-enabled desktop applications.
This module supports Azure QnA Maker API v4.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Create an Azure account
-
Create a resource
-
Obtain tokens by following this guide
Quickstart
To use this connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/azure.qnamaker
module into the Ballerina project.
import ballerinax/azure.qnamaker;
Step 2: Create a new connector instance
You can now make the connection configuration using Ocp-Apim-Subscription-Key
.
You can do this step in two ways. You can use any one of this.
-
Option 1 : Configure API Keys in ballerina file directly.
ker:ApiKeysConfig apiKeyConfig = { cpApimSubscriptionKey:"<AZURE_API_KEY>" ubscriptionKey: "<Subscription Key>" ker:Client myClient = check new Client(apiKeyConfig, serviceUrl = "https://<REGION>.api.cognitive.microsoft.com/qnamaker/v4.0");
-
Option 2 : Configure API Keys in
Config.toml
file and configure it in ballerina file, using configurables.- Set up API Keys in
Config.toml
as shown below.
[apiKeyConfig] ocpApimSubscriptionKey = "<AZURE_API_KEY>" subscriptionKey = "<Subscription Key>"
- Configure the client in ballerina file as shown below.
configurable ApiKeysConfig & readonly apiKeyConfig = ?; qnamaker:Client myClient = check new Client(apiKeyConfig, serviceUrl = "https://<REGION>.api.cognitive.microsoft.com/qnamaker/v4.0");
- Set up API Keys in
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get Knowledgebase Details from API.
Get Knowledgebase Details
public function main() returns error? { qnamaker:KnowledgebaseDTO res = check baseClient->getKnowledgebaseDetail(kID); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
azure.qnamaker: Client
This is a generated connector for Azure QnA Maker API v4 OpenAPI specification. The Azure QnA Maker API is a cloud-based Natural Language Processing (NLP) service that allows you to create a natural conversational layer over your data. It is used to find the most appropriate answer for any input from your custom knowledge base (KB) of information. QnA Maker is commonly used to build conversational client applications, which include social media applications, chat bots, and speech-enabled desktop applications. QnA Maker REST API V4.0
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Azure Text Analytics account and obtain API key following this guide.
init (ApiKeysConfig apiKeyConfig, string serviceUrl, ConnectionConfig config)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- serviceUrl string - URL of the target service
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
getKnowledgebaseDetail
function getKnowledgebaseDetail(string kbId) returns KnowledgebaseDTO|error
Get Knowledgebase Details
Parameters
- kbId string - Knowledgebase id.
Return Type
- KnowledgebaseDTO|error - Details of the knowledgebase.
replaceKnowledgebase
function replaceKnowledgebase(string kbId, ReplaceKbDTO payload) returns Response|error
Replace Knowledgebase
Parameters
- kbId string - Knowledgebase id
- payload ReplaceKbDTO - An instance of ReplaceKbDTO which contains list of qnas to be uploaded
publishKnowledgebase
Publish Knowledgebase
Parameters
- kbId string - Knowledgebase id
deleteKnowledgebase
Delete Knowledgebase
Parameters
- kbId string - Knowledgebase id.
updateKnowledgebase
function updateKnowledgebase(string kbId, UpdateKbOperationDTO payload) returns Operation|error
Update Knowledgebase
downloadKnowledgebase
function downloadKnowledgebase(string kbId, string environment) returns QnADocumentsDTO|error
Download Knowledgebase
Parameters
- kbId string - Knowledgebase id
- environment string - Specifies whether environment is Test or Prod.
Return Type
- QnADocumentsDTO|error - Collection of all Q-A in the knowledgebase.
getEndpointKeys
function getEndpointKeys() returns EndpointKeysDTO|error
Get Endpoint Keys
Return Type
- EndpointKeysDTO|error - response with endpoint info in it.
getKnowledgebaseDetails
function getKnowledgebaseDetails() returns KnowledgebasesDTO|error
Get Knowledgebases for user
Return Type
- KnowledgebasesDTO|error - Collection of knowlegebases.
getOperationDetails
Get Operation Details
Parameters
- operationId string - Operation id.
refreshEndpointKeys
function refreshEndpointKeys(string keyType) returns EndpointKeysDTO|error
Refresh Endpoint Keys
Parameters
- keyType string - type of Key
Return Type
- EndpointKeysDTO|error - Details of the endpoint keys generated.
downloadAlterations
function downloadAlterations() returns WordAlterationsDTO|error
Download Alterations
Return Type
- WordAlterationsDTO|error - Alterations data.
replaceAlterations
function replaceAlterations(WordAlterationsDTO payload) returns Response|error
Replace Alterations
Parameters
- payload WordAlterationsDTO - New alterations data.
createKnowledgebase
function createKnowledgebase(CreateKbDTO payload) returns Operation|error
Create Knowledgebase
Parameters
- payload CreateKbDTO - Post body of the request.
getEndpointSettings
function getEndpointSettings() returns EndpointSettingsDTO|error
Get Endpoint Settings
Return Type
- EndpointSettingsDTO|error - response with endpoint settings info in it.
updateEndpointSettings
function updateEndpointSettings(EndpointSettingsDTO payload) returns Response|error
Update Endpoint Settings
Parameters
- payload EndpointSettingsDTO - PATCH body of the request.
Records
azure.qnamaker: ActiveLearningSettingsDTO
Active Learning settings.
Fields
- enable string? - A True or False parameter denoting if Active Learning is enabled.
azure.qnamaker: AlterationsDTO
Collection of words that are synonyms.
Fields
- alterations string[] - Words that are synonymous with each other.
azure.qnamaker: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- ocpApimSubscriptionKey string - Represents API Key
Ocp-Apim-Subscription-Key
- subscriptionKey string - Represents API Key
subscription-key
azure.qnamaker: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
azure.qnamaker: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
azure.qnamaker: ContextDTO
Context associated with Qna.
Fields
- isContextOnly boolean? - To mark if a prompt is relevant only with a previous question or not. true - Do not include this QnA as search result for queries without context false - ignores context and includes this QnA in search result
- prompts PromptDTO[]? - List of prompts associated with the answer.
azure.qnamaker: CreateKbDTO
Post body schema for CreateKb operation.
Fields
- name string - Friendly name for the knowledgebase.
- qnaList QnADTO[]? - List of Q-A (QnADTO) to be added to the knowledgebase. Q-A Ids are assigned by the service and should be omitted.
- urls string[]? - List of URLs to be used for extracting Q-A.
- files FileDTO[]? - List of files from which to Extract Q-A.
azure.qnamaker: CreateKbInputDTO
Input to create KB.
Fields
- qnaList QnADTO[]? - list of QNA to be added to the index. Ids are generated by the service and should be omitted.
- urls string[]? - List of URLs to be added to knowledgebase.
- files FileDTO[]? - List of files to be added to knowledgebase.
azure.qnamaker: DeleteKbContentsDTO
PATCH body schema of Delete Operation in UpdateKb
Fields
- ids int[]? - List of Qna Ids to be deleted
- sources string[]? - List of sources to be deleted from knowledgebase.
azure.qnamaker: EndpointKeysDTO
Schema for EndpointKeys generate/refresh operations.
Fields
- primaryEndpointKey string? - Primary Access Key.
- secondaryEndpointKey string? - Secondary Access Key.
- installedVersion string? - Current version of runtime.
- lastStableVersion string? - Latest version of runtime.
azure.qnamaker: EndpointSettingsDTO
Endpoint settings.
Fields
- activeLearning ActiveLearningSettingsDTO? - Active Learning settings.
azure.qnamaker: Error
The error object. As per Microsoft One API guidelines - https://github.com/Microsoft/api-guidelines/blob/vNext/Guidelines.md#7102-error-condition-responses.
Fields
- code ErrorCode - Human readable error code.
- message string? - A human-readable representation of the error.
- target string? - The target of the error.
- details Error[]? - An array of details about specific errors that led to this reported error.
- innerError InnerErrorModel? - An object containing more specific information about the error. As per Microsoft One API guidelines - https://github.com/Microsoft/api-guidelines/blob/vNext/Guidelines.md#7102-error-condition-responses.
azure.qnamaker: ErrorResponse
Error response. As per Microsoft One API guidelines - https://github.com/Microsoft/api-guidelines/blob/vNext/Guidelines.md#7102-error-condition-responses.
Fields
- 'error Error? - The error object. As per Microsoft One API guidelines - https://github.com/Microsoft/api-guidelines/blob/vNext/Guidelines.md#7102-error-condition-responses.
azure.qnamaker: FileDTO
DTO to hold details of uploaded files.
Fields
- fileName string - File name. Supported file types are ".tsv", ".pdf", ".txt", ".docx", ".xlsx".
- fileUri string - Public URI of the file.
azure.qnamaker: InnerErrorModel
An object containing more specific information about the error. As per Microsoft One API guidelines - https://github.com/Microsoft/api-guidelines/blob/vNext/Guidelines.md#7102-error-condition-responses.
Fields
- code string? - A more specific error code than was provided by the containing error.
- innerError InnerErrorModel? - An object containing more specific information about the error. As per Microsoft One API guidelines - https://github.com/Microsoft/api-guidelines/blob/vNext/Guidelines.md#7102-error-condition-responses.
azure.qnamaker: KnowledgebaseDTO
Response schema for CreateKb operation.
Fields
- id string? - Unique id that identifies a knowledgebase.
- hostName string? - URL host name at which the knowledgebase is hosted.
- lastAccessedTimestamp string? - Time stamp at which the knowledgebase was last accessed (UTC).
- lastChangedTimestamp string? - Time stamp at which the knowledgebase was last modified (UTC).
- lastPublishedTimestamp string? - Time stamp at which the knowledgebase was last published (UTC).
- name string? - Friendly name of the knowledgebase.
- userId string? - User who created / owns the knowledgebase.
- urls string[]? - URL sources from which Q-A were extracted and added to the knowledgebase.
- sources string[]? - Custom sources from which Q-A were extracted or explicitly added to the knowledgebase.
azure.qnamaker: KnowledgebasesDTO
Collection of knowledgebases owned by a user.
Fields
- knowledgebases KnowledgebaseDTO[]? - Collection of knowledgebase records.
azure.qnamaker: MetadataDTO
Name - value pair of metadata.
Fields
- name string - Metadata name.
- value string - Metadata value.
azure.qnamaker: Operation
Record to track long running operation.
Fields
- operationState OperationState - Enumeration of operation states.
- createdTimestamp string - Timestamp when the operation was created.
- lastActionTimestamp string - Timestamp when the current state was entered.
- resourceLocation string? - Relative URI to the target resource location for completed resources.
- userId string? - User Id
- operationId string? - Operation Id.
- errorResponse ErrorResponse? - Error response. As per Microsoft One API guidelines - https://github.com/Microsoft/api-guidelines/blob/vNext/Guidelines.md#7102-error-condition-responses.
azure.qnamaker: PromptDTO
Prompt for an answer.
Fields
- displayOrder int? - Index of the prompt - used in ordering of the prompts
- qnaId int? - Qna id corresponding to the prompt - if QnaId is present, QnADTO object is ignored.
- qna QnADTO? - Q-A object.
- displayText string? - Text displayed to represent a follow up question prompt
azure.qnamaker: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
azure.qnamaker: QnADocumentsDTO
List of QnADTO
Fields
- qnaDocuments QnADTO[]? - List of answers.
azure.qnamaker: QnADTO
Q-A object.
Fields
- id int? - Unique id for the Q-A.
- answer string - Answer text
- 'source string? - Source from which Q-A was indexed. eg. https://docs.microsoft.com/en-us/azure/cognitive-services/QnAMaker/FAQs
- questions string[] - List of questions associated with the answer.
- metadata MetadataDTO[]? - List of metadata associated with the answer.
- context ContextDTO? - Context associated with Qna.
azure.qnamaker: ReplaceKbDTO
Post body schema for Replace KB operation.
Fields
- qnaList QnADTO[] - List of Q-A (QnADTO) to be added to the knowledgebase. Q-A Ids are assigned by the service and should be omitted.
azure.qnamaker: UpdateContextDTO
Update Body schema to represent context to be updated
Fields
- promptsToDelete int[]? - List of prompts associated with qna to be deleted
- promptsToAdd PromptDTO[]? - List of prompts to be added to the qna.
- isContextOnly boolean? - To mark if a prompt is relevant only with a previous question or not. true - Do not include this QnA as search result for queries without context false - ignores context and includes this QnA in search result
azure.qnamaker: UpdateKbContentsDTO
PATCH body schema for Update operation in Update Kb
Fields
- name string? - Friendly name for the knowledgebase.
- qnaList UpdateQnaDTO[]? - List of Q-A (UpdateQnaDTO) to be added to the knowledgebase.
- urls string[]? - List of existing URLs to be refreshed. The content will be extracted again and re-indexed.
azure.qnamaker: UpdateKbOperationDTO
Contains list of QnAs to be updated
Fields
- add CreateKbInputDTO? - Input to create KB.
- delete DeleteKbContentsDTO? - PATCH body schema of Delete Operation in UpdateKb
- update UpdateKbContentsDTO? - PATCH body schema for Update operation in Update Kb
azure.qnamaker: UpdateMetadataDTO
PATCH Body schema to represent list of Metadata to be updated
Fields
- delete MetadataDTO[]? - List of Metadata associated with answer to be deleted
- add MetadataDTO[]? - List of Metadat associated with answer to be added
azure.qnamaker: UpdateQnaDTO
PATCH Body schema for Update Qna List
Fields
- id int? - Unique id for the Q-A
- answer string? - Answer text
- 'source string? - Source from which Q-A was indexed. eg. https://docs.microsoft.com/en-us/azure/cognitive-services/QnAMaker/FAQs
- questions UpdateQuestionsDTO? - PATCH Body schema for Update Kb which contains list of questions to be added and deleted
- metadata UpdateMetadataDTO? - PATCH Body schema to represent list of Metadata to be updated
- context UpdateContextDTO? - Update Body schema to represent context to be updated
azure.qnamaker: UpdateQuestionsDTO
PATCH Body schema for Update Kb which contains list of questions to be added and deleted
Fields
- add string[]? - List of questions to be added
- delete string[]? - List of questions to be deleted.
azure.qnamaker: WordAlterationsDTO
Collection of word alterations.
Fields
- wordAlterations AlterationsDTO[] - Collection of word alterations.
String types
Import
import ballerinax/azure.qnamaker;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
IT Operations/Cloud Services
Cost/Freemium
Vendor/Microsoft
Contributors