azure.ad
Module azure.ad
Declarations
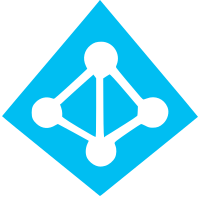
ballerinax/azure.ad Ballerina library
Overview
Ballerina connector for Azure Active Directory (AD) is connecting the Azure AD REST API in Microsoft Graph v1.0 via Ballerina language. It provides capability to perform management operations on user and group resources in an Azure AD tenent (Organization).
The connector uses the Microsoft Graph REST web API that provides the capability to access Microsoft Cloud service resources. This version of the connector only supports the operations on users and groups in an Azure AD.
This module supports Microsoft Graph API v1.0
.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Create an Azure account to register an application in the Azure portal
-
Obtain tokens
- Use this guide to register an application with the Microsoft identity platform
- The necessary scopes for this connector are shown below
Permissions name Type Description User.ReadWrite.All Delegated Create channels Group.ReadWrite.All Delegated Read and write all users' full profiles
Quickstart
To use the Azure AD connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
Import the ballerinax/aad module into the Ballerina project.
import ballerinax/azure.aad;
Step 2 - Create a new connector instance
You can now make the connection configuration using the OAuth2 refresh token grant config.
aad:ConnectionConfig configuration = { auth: { refreshUrl: <REFRESH_URL>, refreshToken : <REFRESH_TOKEN>, clientId : <CLIENT_ID>, clientSecret : <CLIENT_SECRET> } }; aad:Client aadClient = check new (config);
Step 3 - Invoke connector operation
- Create an Azure AD User
ad:NewUser info = { accountEnabled: true, displayName: "<DISPLAY_NAME>", userPrincipalName: "<USER_PRINCIPAL_NAME>", mailNickname: "<MAIL_NICKNAME>", passwordProfile: { password: "<PASSWORD>", forceChangePasswordNextSignIn: true }, surname: "<SURNAME>" }; ad:User|error userInfo = aadClient->createUser(info); if (userInfo is ad:User) { log:printInfo("User succesfully created " + userInfo?.id.toString()); } else { log:printError(userInfo.message()); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
azure.ad: Client
This is connecting to the Microsoft Graph RESTful web API that enables you to access Microsoft Cloud service resources.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Azure account
and obtain tokens following this guide. Configure the Access token to
have the required permission.
init (ConnectionConfig config)
- config ConnectionConfig - Configurations required to initialize the
Client
endpoint
createUser
Creates a new user.
Parameters
- info NewUser - The basic information about a user
getUser
Gets information about a user.
Parameters
- userId string - The object ID if the user
updateUser
function updateUser(string userId, UpdateUser info) returns error?
Updates information about a user.
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
listUsers
Lists information about users.
Parameters
deleteUser
Deletes a user.
Parameters
- userId string - The object ID if the user
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
createGroup
Creates a new group.
Parameters
- info NewGroup - The basic information about a group
getGroup
Gets information about a group.
Parameters
- groupId string - The object ID if the group
updateGroup
function updateGroup(string groupId, UpdateGroup info) returns error?
Updates information about a group.
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
listGroups
Lists information about groups.
Parameters
deleteGroup
Deletes a group.
Parameters
- groupId string - The object ID if the group
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
renewGroup
Renews a group's expiration.
When a group is renewed, the group expiration is extended by the number of days
defined in the policy.
Parameters
- groupId string - The object ID if the group
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
listParentGroups
function listParentGroups(DirctoryObject 'type, string objectId, string? queryParams) returns stream<Group, error?>|error
Lists groups that the group is a direct member of.
listTransitiveParentGroups
function listTransitiveParentGroups(DirctoryObject 'type, string objectId, string? queryParams) returns stream<Group, error?>|error
Gets groups that the group is a member of.
This operation is transitive and will also include all groups
that this groups is a nested member of.
addGroupMember
Adds a member to a Microsoft 365 group or a security group through the members navigation property.
Parameters
- groupId string - The object ID if the group
- memberIds string... - The object IDs of members to add to the group
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
listGroupMembers
Gets a list of the group's direct members.
Parameters
- groupId string - The object ID if the group
listTransitiveGroupMembers
function listTransitiveGroupMembers(string groupId, string? queryParams) returns stream<User, error?>|error
Gets a list of the group's members.
Parameters
- groupId string - The object ID if the group
removeGroupMember
Removes a member from a group via the members navigation property.
Parameters
- groupId string - The object ID if the group
- memberId string - The object ID of member to remove from the group
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
addGroupOwner
Adds a user or service principal to the group's owners.
The owners are a set of users or service principals who are
allowed to modify the group object.
Parameters
- groupId string - The object ID if the group
- ownerId string - The object ID of owner to add to the group
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
listGroupOwners
Retrieves a list of the group's owners.
Parameters
- groupId string - The object ID if the group
removeGroupOwner
Removes an owner from a group via the members navigation property.
Parameters
- groupId string - The object ID if the group
- ownerId string - The object ID of owner to remove from the group
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
listPermissionGrants
function listPermissionGrants(string groupId, string? queryParams) returns stream<PermissionGrant, error?>|error
Lists all resource-specific permission grants on the group.
This list specifies the Azure AD apps that have access
to the group, along with the corresponding kind of resource-specific access that each app has.
Parameters
- groupId string - The object ID if the group
Return Type
- stream<PermissionGrant, error?>|error - A stream of
aad:PermissionGrant
if sucess. Elseerror
.
Enums
azure.ad: AgeGroup
The age group of the user.
Members
azure.ad: CapabilityStatus
Condition of the capability assignment.
Members
azure.ad: ConcentForMinor
Consent which has been obtained for minors.
Members
azure.ad: DirctoryObject
Specifies the visibility of a Microsoft 365 group.
Members
azure.ad: EmployeeType
Represent the enterprise worker type.
Members
azure.ad: GroupType
Specifies the group type and its membership.
Members
azure.ad: GroupVisibility
Specifies the visibility of a Microsoft 365 group.
Members
- Owner permission is needed to join the group.
- Non-members cannot view the contents of the group.
- Owner permission is needed to join the group.
- Non-members cannot view the contents of the group.
- Non-members cannot see the members of the group.
- Administrators (global, company, user, and helpdesk) can view the membership of the group.
- The group appears in the global address book (GAL).
azure.ad: MembershipRuleProcessingState
The dynamic membership processing state.
Members
on
paused
azure.ad: PasswordPolicy
Specifies password policies for the user.
Members
azure.ad: PermissionType
The type of permission.
Members
azure.ad: ResourceBehaviorOption
The group behaviors that can be set for a Microsoft 365 group during creation.
Members
azure.ad: ResourceProvisionOption
The group resources that are provisioned as part of Microsoft 365 group creation, that are not normally part of default group creation.
Members
azure.ad: Theme
Specifies a Microsoft 365 group's color theme.
Members
Records
azure.ad: AssignedLabel
Sensitivity label assigned to an Microsoft 365 group.
Fields
- labelId string - The unique identifier of the label
- displayName string - The display name of the label
azure.ad: AssignedLicense
License assigned to a user.
Fields
- disabledPlans string[] - A collection of the unique identifiers for plans that have been disabled
- skuId string - The unique identifier for the SKU
azure.ad: BaseGroupData
Common Azure Active Directory (Azure AD) group information.
Fields
- visibility GroupVisibility? - Specifies the group join policy and group content visibility for groups
- description string? - An optional description for the group
- isAssignableToRole boolean? - Indicates whether this group can be assigned to an Azure Active Directory role or not
- owners string[]? - The owners of the group. The owners are a set of non-admin users who are allowed to modify this object. Limited to 100 owners.
- members string[]? - Users and groups that are members of this group
- classification string? - Describes a classification for the group (such as low, medium or high business impact)
- membershipRule string? - The rule that determines members for this group if the group is a dynamic group
- membershipRuleProcessingState MembershipRuleProcessingState? - Indicates whether the dynamic membership processing is on or paused
- preferredDataLocation string? - The preferred data location for the group
- preferredLanguage string? - The preferred language for a Microsoft 365 group. Should follow ISO 639-1 Code.
Example:
en-US
.
- resourceProvisioningOptions ResourceProvisionOption[]? - Specifies the group resources that are provisioned as part of Microsoft 365 group creation, that are not normally part of default group creation.
- theme Theme? - Specifies a Microsoft 365 group's color theme
azure.ad: BaseUserData
Common Azure AD user account information.
Fields
- onPremisesImmutableId string? - This property is used to associate an on-premise active directory user account to its Azure AD user object
- passwordProfile PasswordProfile? - Specifies the password profile for the user
- aboutMe string? - A freeform text entry field for the user to describe themselves
- ageGroup AgeGroup? - Sets the age group of the user
- birthday string? - The birthday of the user. The Timestamp type represents date and time information using ISO 8601 format
and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is
2014-01-01T00:00:00Z
.
- businessPhones string[]? - The telephone numbers for the user. NOTE: Although this is a string collection, only one number can be set for this property.
- city string? - The city in which the user is located. Maximum length is 128 characters.
- companyName string? - The company name which the user is associated. This property can be useful for describing the company that an external user comes from. The maximum length of the company name is 64 characters.
- consentProvidedForMinor ConcentForMinor? - Sets whether consent has been obtained for minors
- country string? - The country/region in which the user is located; for example, US or UK. Maximum length is 128 characters.
- department string? - The name for the department in which the user works. Maximum length is 64 characters.
- employeeId string? - The employee identifier assigned to the user by the organization
- employeeType EmployeeType? - Captures enterprise worker type
- givenName string? - The given name (first name) of the user. Maximum length is 64 characters.
- hireDate string? - The hire date of the user. The Timestamp type represents date and time information using ISO 8601 format
and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is
2014-01-01T00:00:00Z
.
- interests string[]? - A list for the user to describe their interests
- jobTitle string? - The user's job title. Maximum length is 128 characters.
- mail string? - The SMTP address for the user, for example, jeff@contoso.onmicrosoft.com
- mobilePhone string? - The primary cellular telephone number for the user. Read-only for users synced from on-premises directory. Maximum length is 64 characters.
- mySite string? - The URL for the user's personal site
- officeLocation string? - The office location in the user's place of business
- otherMails string? - A list of additional email addresses for the user Example: ["bob@contoso.com", "Robert@fabrikam.com"]
- passwordPolicies PasswordPolicy? - Specifies password policies for the user
- pastProjects string[]? - A list for the user to enumerate their past projects
- postalCode string? - The postal code for the user's postal address
- preferredLanguage string? - The preferred language for the user. Should follow ISO 639-1 Code; for example
en-US
.
- responsibilities string[]? - A list for the user to enumerate their responsibilities
- schools string[]? - A list for the user to enumerate the schools they have attended
- skills string[]? - A list for the user to enumerate their skills
- state string? - The state or province in the user's address. Maximum length is 128 characters
- streetAddress string? - The street address of the user's place of business. Maximum length is 1024 characters
- usageLocation string? - A two letter country code (ISO standard 3166). Required for users that will be assigned licenses due to legal requirement to check for availability of services in countries Examples: US, JP, and GB.
- surname string? - The user's surname (family name or last name). Maximum length is 64 characters
azure.ad: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
azure.ad: ExtentionAttriute
Custom extention attributes of the user.
Source of authority for this set of properties is the on-premises Active
Directory which is synchronized to Azure AD
Fields
- extensionAttribute1 string? - First customizable extension attribute
- extensionAttribute2 string? - Second customizable extension attribute
- extensionAttribute3 string? - Third customizable extension attribute
- extensionAttribute4 string? - Fourth customizable extension attribute
- extensionAttribute5 string? - Fifth customizable extension attribute
- extensionAttribute6 string? - Sixth customizable extension attribute
- extensionAttribute7 string? - Seventh customizable extension attribute
- extensionAttribute8 string? - Eighth customizable extension attribute
- extensionAttribute9 string? - Ninth customizable extension attribute
- extensionAttribute10 string? - Tenth customizable extension attribute
- extensionAttribute11 string? - Eleventh customizable extension attribute
- extensionAttribute12 string? - Twelfth customizable extension attribute
- extensionAttribute13 string? - Thirteenth customizable extension attribute
- extensionAttribute14 string? - Fourteenth customizable extension attribute
- extensionAttribute15 string? - Fifteenth customizable extension attribute
azure.ad: Group
Azure Active Directory (Azure AD) group.
Fields
- id string? - The unique identifier for the group
- Fields Included from *BaseGroupData
- visibility ""|"HiddenMembership"|"Public"|"Private"|()
- description string|()
- isAssignableToRole boolean|()
- owners string[]
- members string[]
- classification string|()
- membershipRule string|()
- membershipRuleProcessingState "Paused"|"On"|()
- preferredDataLocation string|()
- preferredLanguage string|()
- resourceProvisioningOptions ResourceProvisionOption[]
- theme "Red"|"Orange"|"Blue"|"Green"|"Pink"|"Purple"|"Teal"|()
- anydata...
- resourceBehaviorOptions string[]? - Specifies the group behaviors that can be set for a Microsoft 365 group during creation
- allowExternalSenders boolean? - Indicates if people external to the organization can send messages to the group
- assignedLabels AssignedLabel[]? - The list of sensitivity label pairs (label ID, label name) associated with a Microsoft 365 group
- assignedLicenses AssignedLicense[]? - The licenses that are assigned to the group
- autoSubscribeNewMembers boolean? - Indicates if new members added to the group will be auto-subscribed to receive email notifications
- createdDateTime string? - Timestamp of when the group was created. Represents date and time information using ISO 8601
format and is always in UTC time. For example, midnight UTC on Jan 1,
2014 is 2014-01-01T00:00:00Z
.
- deletedDateTime string? - The date and time when the object was deleted
- renewedDateTime string? - Timestamp of when the group was last renewed
- expirationDateTime string? - Timestamp of when the group is set to expire
- hideFromAddressLists string? - True if the group is not displayed in certain parts of the Outlook UI: the Address Book, address lists for selecting message recipients, and the Browse Groups dialog for searching groups; otherwise, false.
- hideFromOutlookClients string? - True if the group is not displayed in Outlook clients, such as Outlook for Windows and Outlook on the web; otherwise, false.
- isSubscribedByMail boolean? - Indicates whether the signed-in user is subscribed to receive email conversations. Default
value is
true
- licenseProcessingState string? - Indicates status of the group license assignment to all members of the group. Default value
is
false
- onPremisesDomainName string? - On premises domain name
- onPremisesLastSyncDateTime string? - On premises last sync date/time
- onPremisesNetBiosName string? - On premises net bios name
- onPremisesSamAccountName string? - On premises SAM account name
- onPremisesSecurityIdentifier string? - On premises security identifier
- onPremisesSyncEnabled boolean? - On premises sync enabled
- proxyAddresses string[]? - Email addresses for the group that direct to the same group mailbox. Example: ["SMTP: bob@contoso.com", "smtp: bob@sales.contoso.com"]
- mailEnabled boolean - Specifies whether the group is mail-enabled
azure.ad: NewGroup
New group to be added to the Active AD.
Fields
- Fields Included from *BaseGroupData
- visibility ""|"HiddenMembership"|"Public"|"Private"|()
- description string|()
- isAssignableToRole boolean|()
- owners string[]
- members string[]
- classification string|()
- membershipRule string|()
- membershipRuleProcessingState "Paused"|"On"|()
- preferredDataLocation string|()
- preferredLanguage string|()
- resourceProvisioningOptions ResourceProvisionOption[]
- theme "Red"|"Orange"|"Blue"|"Green"|"Pink"|"Purple"|"Teal"|()
- anydata...
- groupTypes GroupType[] - Specifies the group type and its membership
- displayName string - The display name for the group
- mailEnabled boolean - Specifies whether the group is mail-enabled
- securityEnabled boolean - Specifies whether the group is a security group
- mailNickname string - The mail alias for the group
- resourceBehaviorOptions ResourceBehaviorOption[]? - Specifies the group behaviors that can be set for a Microsoft 365 group during creation
- ownerIds string[]? - Represents the owners for the group at creation time.
- memberIds string[]? - Represents the members for the group at creation time
azure.ad: NewUser
New user to be added to the Active AD.
Fields
- accountEnabled boolean - True if the account is enabled or else false
- mailNickname string - The mail alias for the user
- displayName string - The name displayed in the address book for the user
- userPrincipalName string - The user principal name (UPN) of the user
- Fields Included from *BaseUserData
- onPremisesImmutableId string|()
- passwordProfile PasswordProfile|()
- aboutMe string|()
- ageGroup "adult"|"notAdult"|"minor"|"null"|()
- birthday string|()
- businessPhones string[]
- city string|()
- companyName string|()
- consentProvidedForMinor "notRequired"|"denied"|"granted"|"null"|()
- country string|()
- department string|()
- employeeId string|()
- employeeType "Vendor"|"Consultant"|"Contractor"|"Employee"|()
- givenName string|()
- hireDate string|()
- interests string[]
- jobTitle string|()
- mail string|()
- mobilePhone string|()
- mySite string|()
- officeLocation string|()
- otherMails string|()
- passwordPolicies "DisablePasswordExpiration, DisableStrongPassword"|"DisablePasswordExpiration"|"DisableStrongPassword"|()
- pastProjects string[]
- postalCode string|()
- preferredLanguage string|()
- responsibilities string[]
- schools string[]
- skills string[]
- state string|()
- streetAddress string|()
- usageLocation string|()
- surname string|()
- anydata...
azure.ad: PasswordProfile
Password profile associated with a user.
Fields
- forceChangePasswordNextSignIn boolean? -
true
if the user must change the password on the next login or elsefalse
- forceChangePasswordNextSignInWithMfa boolean? - If
true
, at the next sign-in, the users must perform a multi-factor authentication (MFA) before being forced to change their password
- password string - The password of the user
azure.ad: PermissionGrant
Permission that has been granted to a specific AzureAD app for an instance of a resource in Microsoft Graph.
Fields
- id string - The unique identifier of the resource-specific permission grant
- deletedDateTime string? - Deleted date/time
- clientId string? - ID of the Azure AD app that has been granted access
- clientAppId string? - ID of the service principal of the Azure AD app that has been granted access
- resourceAppId string? - ID of the Azure AD app that is hosting the resource
- permissionType PermissionType? - The type of permission
- permission string? - The name of the resource-specific permission
azure.ad: UpdateGroup
Updatable Azure AD group information.
Fields
- groupTypes GroupType[]? - Specifies the group type and its membership
- description string? - An optional description for the group
- displayName string? - The display name for the group
- mailEnabled boolean? - Specifies whether the group is mail-enabled
- securityEnabled boolean? - Specifies whether the group is a security group
- mailNickname string? - The mail alias for the group
- allowExternalSenders boolean? - Indicates if people external to the organization can send messages to the group.
Default value is
false
- autoSubscribeNewMembers boolean? - Indicates if new members added to the group will be auto-subscribed to receive email notifications
- visibility GroupVisibility? - Specifies the group join policy and group content visibility for groups
azure.ad: UpdateUser
Updatable Azure AD user account information.
Fields
- accountEnabled boolean? - True if the account is enabled or else false
- mailNickname string? - The mail alias for the user
- displayName string? - The name displayed in the address book for the user. Maximum length is 256 characters.
- userPrincipalName string? - The user principal name (UPN) of the user
- Fields Included from *BaseUserData
- onPremisesImmutableId string|()
- passwordProfile PasswordProfile|()
- aboutMe string|()
- ageGroup "adult"|"notAdult"|"minor"|"null"|()
- birthday string|()
- businessPhones string[]
- city string|()
- companyName string|()
- consentProvidedForMinor "notRequired"|"denied"|"granted"|"null"|()
- country string|()
- department string|()
- employeeId string|()
- employeeType "Vendor"|"Consultant"|"Contractor"|"Employee"|()
- givenName string|()
- hireDate string|()
- interests string[]
- jobTitle string|()
- mail string|()
- mobilePhone string|()
- mySite string|()
- officeLocation string|()
- otherMails string|()
- passwordPolicies "DisablePasswordExpiration, DisableStrongPassword"|"DisablePasswordExpiration"|"DisableStrongPassword"|()
- pastProjects string[]
- postalCode string|()
- preferredLanguage string|()
- responsibilities string[]
- schools string[]
- skills string[]
- state string|()
- streetAddress string|()
- usageLocation string|()
- surname string|()
- anydata...
azure.ad: User
Azure AD user account.
Fields
- id string? - The unique identifier for the user
- displayName string? - The name displayed in the address book for the user. Maximum length is 256 characters.
- userPrincipalName string? - The user principal name (UPN) of the user
- Fields Included from *BaseUserData
- onPremisesImmutableId string|()
- passwordProfile PasswordProfile|()
- aboutMe string|()
- ageGroup "adult"|"notAdult"|"minor"|"null"|()
- birthday string|()
- businessPhones string[]
- city string|()
- companyName string|()
- consentProvidedForMinor "notRequired"|"denied"|"granted"|"null"|()
- country string|()
- department string|()
- employeeId string|()
- employeeType "Vendor"|"Consultant"|"Contractor"|"Employee"|()
- givenName string|()
- hireDate string|()
- interests string[]
- jobTitle string|()
- mail string|()
- mobilePhone string|()
- mySite string|()
- officeLocation string|()
- otherMails string|()
- passwordPolicies "DisablePasswordExpiration, DisableStrongPassword"|"DisablePasswordExpiration"|"DisableStrongPassword"|()
- pastProjects string[]
- postalCode string|()
- preferredLanguage string|()
- responsibilities string[]
- schools string[]
- skills string[]
- state string|()
- streetAddress string|()
- usageLocation string|()
- surname string|()
- anydata...
- onPremisesExtensionAttributes ExtentionAttriute? - Contains extensionAttributes 1-15 for the user
Import
import ballerinax/azure.ad;
Metadata
Released date: 12 months ago
Version: 2.5.0
License: Apache-2.0
Compatibility
Platform: java17
Ballerina version: 2201.8.0
GraalVM compatible: Yes
Pull count
Total: 18
Current verison: 4
Weekly downloads
Keywords
IT Operations/Security & Identity Tools
Cost/Freemium
Vendor/Microsoft
Contributors