aws.secretmanager
Module aws.secretmanager
Declarations
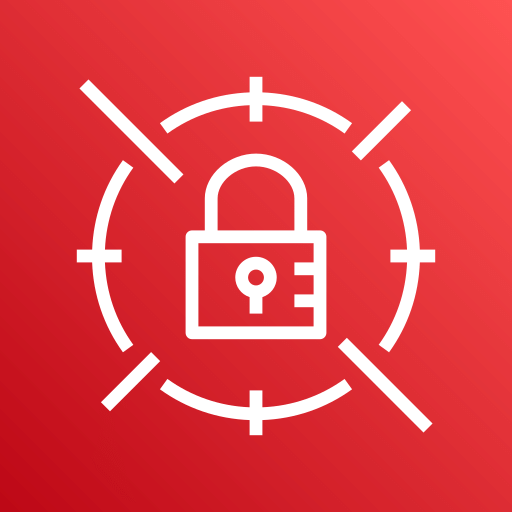
ballerinax/aws.secretmanager Ballerina library
Overview
AWS Secrets Manager is a service that helps you protect sensitive information, such as database credentials, API keys, and other secrets, by securely storing and managing access to them.
The ballerinax/aws.secretmanager
package provides APIs to interact with AWS Secrets Manager, enabling developers to programmatically manage secrets, including creating, retrieving, updating, and deleting secrets in their applications.
Setup guide
Before using this connector in your Ballerina application, complete the following:
- Create an AWS account
- Obtain tokens
Quickstart
To use the aws.secretmanager
connector in your Ballerina project, modify the .bal
file as follows:
Step 1: Import the module
Import the ballerinax/aws.secretmanager
module into your Ballerina project.
import ballerinax/aws.secretmanager;
Step 2: Instantiate a new connector
Create a new secretmanager:Client
by providing the access key ID, secret access key, and the region.
configurable string accessKeyId = ?; configurable string secretAccessKey = ?; secretmanager:Client secretmanager = check new(region = secretmanager:US_EAST_1, auth = { accessKeyId, secretAccessKey });
Step 3: Invoke the connector operation
Now, utilize the available connector operations.
secretmanager:DescribeSecretResponse response = check secretManager->describeSecret("<secret-id>");
Step 4: Run the Ballerina application
Use the following command to compile and run the Ballerina program.
bal run
Clients
aws.secretmanager: Client
AWS Secret Manager client.
Constructor
Initialize the Ballerina AWS Secret Manager client.
secretmanager:Client secretmanager = check new(region = secretmanager:US_EAST_1, auth = {
accessKeyId: "<aws-access-key>",
secretAccessKey: "<aws-secret-key>"
});
init (*ConnectionConfig configs)
- configs *ConnectionConfig - The AWS Secret Manager client configurations
describeSecret
function describeSecret(SecretId secretId) returns DescribeSecretResponse|Error
Retrieves the details of a secret. It does not include the encrypted secret value. Secrets Manager only returns fields that have a value in the response.
secretmanager:DescribeSecretResponse response = check secretmanager->describeSecret("<aws-secret-id>");
Parameters
- secretId SecretId - The ARN or name of the secret
Return Type
- DescribeSecretResponse|Error - An
secretmanager:DescribeSecretResponse
containing the details of the secret, or ansecretmanager:Error
if the request validation or the operation failed
getSecretValue
function getSecretValue(SecretId secretId, *SecretVersionSelector versionSelector) returns SecretValue|Error
Retrieves the contents of the encrypted fields from the specified version of a secret.
secretmanager:SecretValue secret = check secretmanager->getSecretValue("<aws-secret-id>");
Parameters
- secretId SecretId - The ARN or name of the secret
- versionSelector *SecretVersionSelector - Details for selecting a specific version of the secret
Return Type
- SecretValue|Error - An
secretmanager:SecretValue
containing the content of the secret, or ansecretmanager:Error
if the request validation or the operation failed
batchGetSecretValue
function batchGetSecretValue(*BatchGetSecretValueRequest request) returns BatchGetSecretValueResponse|Error
Retrieves the contents of the encrypted fields for up to 20 secrets.
secretmanager:BatchGetSecretValueResponse secret = check secretmanager->batchGetSecretValue( secretIds = ["<aws-secret-id>"]);
Parameters
- request *BatchGetSecretValueRequest - The filters or secret IDs used to identify the secrets to retrieve
Return Type
- BatchGetSecretValueResponse|Error - An
secretmanager:BatchGetSecretValueResponse
containing the contents of the secrets, or ansecretmanager:Error
if the request validation or the operation failed
close
function close() returns Error?
Closes the AWS Secret Manager client resources.
check secretmanager->close();
Return Type
- Error? - A
secretmanager:Error
if there is an error while closing the client resources or else nil
Enums
aws.secretmanager: Region
An Amazon Web Services region that hosts a set of Amazon services.
Members
aws.secretmanager: StagingStatus
Represents the staging label that indicates the version of the secret in AWS Secrets Manager.
Members
Records
aws.secretmanager: ApiError
Represents an error encountered by Secrets Manager while retrieving an individual secret.
Fields
- errorCode? string - The error code returned by Secrets Manager indicating the issue encountered.
- message? string - A descriptive message explaining the nature of the error.
- secretId? SecretId - The ARN or name of the secret for which the error occurred.
aws.secretmanager: AuthConfig
Represents the Authentication configurations for AWS Marketplace Entitlement service.
Fields
- accessKeyId string - The AWS access key, used to identify the user interacting with AWS
- secretAccessKey string - The AWS secret access key, used to authenticate the user interacting with AWS
- sessionToken? string - The AWS session token, retrieved from an AWS token service, used for authenticating a user with temporary permission to a resource
aws.secretmanager: BatchGetSecretValueRequest
Represents the request parameters for the batchGetSecretValue
API of the AWS Secrets Manager connector.
Fields
- filters? SecretValueFilter[] - The filters to choose which secrets to retrieve
- maxResults? int - The number of results to include in the response. If there are more results available,
in the response, Secrets Manager includes
nextToken
. To use this parameter, you must also use thefilters
parameter
- nextToken? string - A token that indicates where the output should continue from, if a previous call did not show all results
- secretIds? SecretId[] - The ARN or names of the secrets to retrieve. You must include
filters
orsecretIds
, but not both
aws.secretmanager: BatchGetSecretValueResponse
Represents the response returned by the batchGetSecretValue
API of the AWS Secrets Manager connector.
Fields
- errors? ApiError[] - A list of errors encountered by Secrets Manager while attempting to retrieve individual secrets. Each error provides details such as the error code, message, and the affected secret's identifier.
- nextToken? string - A token indicating that more results are available than what is included in the current response.
Use this token to retrieve the next set of results by making another call to
batchGetSecretValue
. This token might be present even if no values are returned, such as when filtering a long list
- secretValues? SecretValue[] - A list of retrieved secret values. Each value represents a specific secret returned by the API.
aws.secretmanager: ConnectionConfig
Represents the Client configurations for AWS Marketplace Entitlement service.
Fields
- region Region - The AWS region with which the connector should communicate
- auth AuthConfig - The authentication configurations for the AWS Marketplace Entitlement service
aws.secretmanager: DescribeSecretResponse
Represents the results retrieved from GetEntitlements
operation.
Fields
- arn string - The ARN of the secret
- createdDate Utc - The date the secret was created
- deletedDate? Utc - The date the secret is scheduled for deletion
- description string - The description of the secret
- kmsKeyId? string - The key ID or alias ARN of the AWS KMS key that Secrets Manager uses to encrypt the secret value
- lastAccessedDate? Utc - The date that the secret was last accessed in the Region
- lastChangedDate? Utc - The last date and time that this secret was modified in any way
- lastRotatedDate? Utc - The last date and time that Secrets Manager rotated the secret
- name string - The name of the secret
- nextRotationDate? Utc - The next rotation is scheduled to occur on or before this date
- owningService string - The ID of the service that created this secret
- primaryRegion Region - The Region the secret is in. If a secret is replicated to other Regions, the replicas are listed in
replicationStatus
- replicationStatus? ReplicationStatus[] - A list of the replicas of this secret and their status
- rotationEnabled boolean - Specifies whether automatic rotation is turned on for this secret
- rotationLambdaArn? string - The ARN of the Lambda function that Secrets Manager invokes to rotate the secret
- rotationRules? RotationRules - The rotation schedule and Lambda function for this secret
- tags? Tag[] - The list of tags attached to the secret
- versionToStages? map<StagingStatus[]> - A list of the versions of the secret that have staging labels attached
aws.secretmanager: ErrorDetails
The error details type for the AWS Secret Manager module.
Fields
- httpStatusCode? int - The HTTP status code for the error
- httpStatusText? string - The HTTP status text returned from the service
- errorCode? string - The error code associated with the response
- errorMessage? string - The human-readable error message provided by the service
aws.secretmanager: ReplicationStatus
Represents the replication status of a secret in AWS Secrets Manager.
Fields
- kmsKeyId? string - The ARN, key ID, or an alias ARN of the AWS KMS key that Secrets Manager uses to encrypt the secret value
- lastAccessedDate? Utc - The date that the secret was last accessed in the Region
- region? Region - The Region where replication occurs
- status? "InSync"|"Failed"|"InProgress" - The replication status
- statusMessage? string - The status message
aws.secretmanager: RotationRules
Represents the rotation rules for a secret in AWS Secrets Manager
Fields
- automaticallyAfterDays? int - The number of days between rotations of the secret
- duration? string - The length of the rotation window in hours
- scheduleExpresssion? string - A
cron
orrate
expression that defines the schedule for rotating your secret
aws.secretmanager: SecretValue
Represents the details of a secret retrieved from AWS Secrets Manager.
Fields
- arn string - The ARN of the secret
- createdDate Utc - The date and time that this version of the secret was created
- name string - The friendly name of the secret
- value byte[]|string - The decrypted secret value
- versionId string - The unique identifier of this version of the secret
- versionStages string[] - A list of all the staging labels currently attached to this version of the secret
aws.secretmanager: SecretValueFilter
Represents a filter used to match specific secrets based on a key and its values.
Fields
- 'key? FilterKey - The key used to filter secrets
- values? FilterValue[] - A list of values associated with the filter key
aws.secretmanager: SecretVersionSelector
Represents the request to retrieve a secret value from AWS Secrets Manager.
Fields
- versionId? string - The unique identifier of the version of the secret
- versionStage? string - The staging label of the version of the secret
aws.secretmanager: Tag
Represents a tag associated with an AWS resource.
Fields
- 'key? string - The key identifier, or name, of the tag
- value? string - The string value associated with the key of the tag
Errors
aws.secretmanager: Error
Represents a AWS Secret Manager distinct error.
Union types
aws.secretmanager: FilterKey
FilterKey
The allowed filter keys for SecretValueFilter
.
String types
Import
import ballerinax/aws.secretmanager;
Metadata
Released date: 6 months ago
Version: 0.1.0
License: Apache-2.0
Compatibility
Platform: java17
Ballerina version: 2201.10.0
GraalVM compatible: Yes
Pull count
Total: 6
Current verison: 2
Weekly downloads
Keywords
AWS
Secret Manager
Cloud/Subscriptions
Contributors
Dependencies