aws.s3
Module aws.s3
API
Declarations
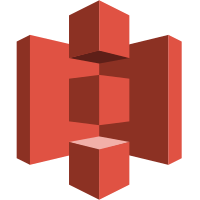
ballerinax/aws.s3 Ballerina library
Overview
The Ballerina AWS S3 provides the capability to manage buckets and objects in AWS S3.
This module supports Amazon S3 REST API 2006-03-01
version.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an AWS account
- Obtain tokens
Quickstart
To use the AWS S3 connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/aws.s3
module into the Ballerina project.
import ballerinax/aws.s3;
Step 2: Create a new connector instance
Create a s3:ConnectionConfig
with the tokens obtained, and initialize the connector with it.
s3:ConnectionConfig amazonS3Config = { accessKeyId: <ACCESS_KEY_ID>, secretAccessKey: <SECRET_ACCESS_KEY>, region: <REGION> }; s3:Client amazonS3Client = check new(amazonS3Config);
IAM role-based authentication can be used as below if the code is running within an EC2 instance.
s3:ConnectionConfig amazonS3Config = { authType: s3:EC2_IAM_ROLE, region: <REGION> } ;
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create a bucket using the connector.string bucketName = "name"; public function main() returns error? { _ = check amazonS3Client->createBucket(bucketName); }
-
Use
bal run
command to compile and run the Ballerina program.
Functions
sort
Returns sorted string array after performing bucket sort repeatedly. By default, sorting is done on the english alphabet order. If any character outside the alphabet isfound, it will get the least priority in sorting.
Parameters
- unsortedArray string[] - The unsorted string array.
Return Type
- string[] - The sorted string array.
Clients
aws.s3: Client
Ballerina Amazon S3 connector provides the capability to access AWS S3 API. This connector lets you to get authorized access to AWS S3 buckets and objects.
Constructor
Initializes the connector. During initialization you have to pass access key id and secret access key Create an AWS account and obtain tokens following this guide.
init (ConnectionConfig config)
- config ConnectionConfig -
listBuckets
Retrieves a list of all Amazon S3 buckets that the authenticated user of the request owns.
createBucket
Creates a bucket.
Parameters
- bucketName string - A unique name for the bucket
- cannedACL CannedACL? (default ()) - The access control list of the new bucket
Return Type
- error? - An error on failure or else
()
listObjects
function listObjects(string bucketName, string? delimiter, string? encodingType, int? maxKeys, string? prefix, string? startAfter, boolean? fetchOwner, string? continuationToken) returns S3Object[]|error
Retrieves the existing objects in a given bucket.
Parameters
- bucketName string - The name of the bucket
- delimiter string? (default ()) - A delimiter is a character you use to group keys
- encodingType string? (default ()) - The encoding method to be applied to the response
- maxKeys int? (default ()) - The maximum number of keys to include in the response
- prefix string? (default ()) - The prefix of the objects to be listed. If unspecified, all objects are listed
- startAfter string? (default ()) - Object key from which to begin listing
- fetchOwner boolean? (default ()) - Set to true, to retrieve the owner information in the response. By default the API does not return the Owner information in the response
- continuationToken string? (default ()) - When the response to this API call is truncated (that is, the IsTruncated response element value is true), the response also includes the NextContinuationToken element. To list the next set of objects, you can use the NextContinuationToken element in the next request as the continuation-token
getObject
function getObject(string bucketName, string objectName, ObjectRetrievalHeaders? objectRetrievalHeaders, int? byteArraySize) returns stream<byte[], Error?>|error
Retrieves objects from Amazon S3.
Parameters
- bucketName string - The name of the bucket
- objectName string - The name of the object
- objectRetrievalHeaders ObjectRetrievalHeaders? (default ()) - Optional headers for the get object
- byteArraySize int? (default ()) - A defaultable parameter to state the size of the byte array. Default size is 8KB
createObject
function createObject(string bucketName, string objectName, string|xml|json|byte[]|stream<Block, Error?> payload, CannedACL? cannedACL, ObjectCreationHeaders? objectCreationHeaders, map<string> userMetadataHeaders) returns error?
Creates an object.
Parameters
- bucketName string - The name of the bucket
- objectName string - The name of the object
- cannedACL CannedACL? (default ()) - The access control list of the new object
- objectCreationHeaders ObjectCreationHeaders? (default ()) - Optional headers for the
createObject
function
Return Type
- error? - An error on failure or else
()
deleteObject
Deletes an object.
Parameters
- bucketName string - The name of the bucket
- objectName string - The name of the object
- versionId string? (default ()) - The specific version of the object to delete, if versioning is enabled
Return Type
- error? - An error on failure or else
()
deleteBucket
Deletes a bucket.
Parameters
- bucketName string - The name of the bucket
Return Type
- error? - An error on failure or else
()
createPresignedUrl
function createPresignedUrl(string bucketName, string objectName, ObjectAction|ObjectCreationHeaders|ObjectRetrievalHeaders action, int expires, int? partNo, string? uploadId) returns string|error
Generates a presigned URL for the object.
Parameters
- bucketName string - The name of the bucket
- objectName string - The name of the object
- action ObjectAction|ObjectCreationHeaders|ObjectRetrievalHeaders - The action to be done on the object (
RETRIEVE
for object retrieval orCREATE
for object creation) or the relevant headers for object retrieval or creation
- expires int (default 1800) - The time period for which the presigned URL is valid, in seconds
- partNo int? (default ()) - The part number of the object, when uploading multipart objects
- uploadId string? (default ()) - The upload ID of the multipart upload
createMultipartUpload
function createMultipartUpload(string objectName, string bucketName, CannedACL? cannedACL, MultipartUploadHeaders? multipartUploadHeaders) returns string|error
Initiates a multipart upload and returns an upload ID.
Parameters
- objectName string - The name of the object
- bucketName string - The name of the bucket
- cannedACL CannedACL? (default ()) - The access control list of the new object
- multipartUploadHeaders MultipartUploadHeaders? (default ()) - Optional headers for multipart uploads
uploadPart
function uploadPart(string objectName, string bucketName, string|xml|json|byte[]|stream<Block, Error?> payload, string uploadId, int partNumber, UploadPartHeaders? uploadPartHeaders) returns CompletedPart|error
Completes a multipart upload by assembling previously uploaded parts.
Parameters
- objectName string - The name of the object
- bucketName string - The name of the bucket
- uploadId string - The upload ID of the multipart upload
- partNumber int - The part number of the object
- uploadPartHeaders UploadPartHeaders? (default ()) - Optional headers for the upload
Return Type
- CompletedPart|error - An error on failure or else
()
completeMultipartUpload
function completeMultipartUpload(string objectName, string bucketName, string uploadId, CompletedPart[] completedParts) returns error?
Completes a multipart upload by assembling previously uploaded parts.
Parameters
- objectName string - The name of the object
- bucketName string - The name of the bucket
- uploadId string - The upload ID of the multipart upload
- completedParts CompletedPart[] - An array containing the part number and ETag of each uploaded part
Return Type
- error? - An error on failure or else
()
abortMultipartUpload
Aborts a multipart upload.
Parameters
- objectName string - The name of the object
- bucketName string - The name of the bucket
- uploadId string - The upload ID of the multipart upload
Return Type
- error? - An error on failure or else
()
Constants
aws.s3: ACL_AUTHENTICATED_READ
aws.s3: ACL_BUCKET_OWNER_FULL_CONTROL
aws.s3: ACL_BUCKET_OWNER_READ
aws.s3: ACL_LOG_DELIVERY_WRITE
aws.s3: ACL_PRIVATE
aws.s3: ACL_PUBLIC_READ
aws.s3: ACL_PUBLIC_READ_WRITE
aws.s3: AWS_STATIC_AUTH
aws.s3: EC2_IAM_ROLE
Enums
aws.s3: ObjectAction
The action to be carried out on the object.
Members
Records
aws.s3: Bucket
Defines bucket.
Fields
- name string - The name of the bucket
- creationDate string - The creation date of the bucket
aws.s3: CompletedPart
Represents the details of a part uploaded through the UploadPart
function.
Fields
- partNumber int - The part number of the file part
- ETag string - Represents the hash value of the object, which reflects modifications made exclusively to the contents of the object
aws.s3: ConnectionConfig
Represents the AmazonS3 Connector configurations.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth? never - auth
- accessKeyId? string - The access key of the Amazon S3 account
- secretAccessKey? string - The secret access key of the Amazon S3 account
- region? string - The AWS Region. If you don't specify an AWS region, Client uses US East (N. Virginia) as default region
- httpVersion HttpVersion(default http:HTTP_1_1) - The HTTP version understood by the client
- authType AWS_STATIC_AUTH|EC2_IAM_ROLE(default AWS_STATIC_AUTH) - The type of authentication to be used
- sessionToken? string - The session token for temporary credentials
aws.s3: GetHeaders
Define record for GET method together with ObjectRetrievalHeaders.
Fields
- method GET(default GET) - HTTP method
- headers ObjectRetrievalHeaders - ObjectRetrievalHeaders
aws.s3: MultipartUploadHeaders
Represents the optional headers specific to CreateMultipartUpload
function.
Fields
- cacheControl? string - Can be used to specify caching behavior along the request/reply chain
- contentDisposition? string - Specifies presentational information for the object
- contentEncoding? string - Specifies what content encodings have been applied to the object and thus what decoding mechanisms must be applied to obtain the media-type referenced by the Content-Type header field
- contentLanguage? string - The language the content is in
- contentType? string - The MIME type of the content
- expires? string - The date and time at which the object is no longer cacheable
aws.s3: ObjectCreationHeaders
Represents the optional headers specific to createObject function.
Fields
- cacheControl? string - Can be used to specify caching behavior along the request/reply chain
- contentDisposition? string - Specifies presentational information for the object.
- contentEncoding? string - Specifies what content encodings have been applied to the object and thus what decoding mechanisms must be applied to obtain the media-type referenced by the Content-Type header field
- contentLength? string - The size of the object, in bytes
- contentMD5? string - The base64-encoded 128-bit MD5 digest of the message (without the headers)
- expect? string - When your application uses 100-continue, it does not send the request body until it receives an acknowledgment.The date and time at which the object is no longer able to be cached
- expires? string - The date and time at which the object is no longer cacheable
- contentType? string - The MIME type of the content
aws.s3: ObjectRetrievalHeaders
Represents the optional headers specific to getObject function.
Fields
- modifiedSince? string - Return the object only if it has been modified since the specified time
- unModifiedSince? string - Return the object only if it has not been modified since the specified time
- ifMatch? string - Return the object only if its entity tag (ETag) is the same as the one specified
- ifNoneMatch? string - Return the object only if its entity tag (ETag) is different from the one specified
- range? string - Downloads the specified range bytes of an object
aws.s3: PutHeaders
Define record for PUT method together with ObjectCreationHeaders.
Fields
- method PUT(default PUT) - HTTP method
- headers ObjectCreationHeaders - ObjectCreationHeaders
aws.s3: S3Object
Define S3Object.
Fields
- objectName? string - The name of the object
- lastModified? string - The last modified date of the object
- eTag? string - The etag of the object
- objectSize? string - The size of the object
- ownerId? string - The id of the object owner
- ownerDisplayName? string - The display name of the object owner
- storageClass? string - The storage class of the object
- content? byte[] - The content of the object
aws.s3: UploadPartHeaders
Represents the optional headers specific to UploadPart
function.
Fields
- contentLength? string - The size of the object, in bytes
- contentMD5? string - The base64-encoded 128-bit MD5 digest of the message (without the headers)
Union types
Import
import ballerinax/aws.s3;
Metadata
Released date: 29 days ago
Version: 3.5.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 12510
Current verison: 198
Weekly downloads
Keywords
Content & Files/File Management & Storage
Cost/Paid
Vendor/Amazon
Contributors