avaza
Module avaza
API
Definitions
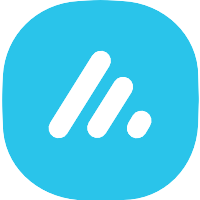
ballerinax/avaza Ballerina library
Overview
This is a generated connector for Avaza API v1 OpenAPI specification. Avaza API allows to collaborate on projects, chat, schedule resources, track time, manage expenses & invoice customers.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Avaza Account
- Obtaining tokens
- Log into Avaza Account
- After login token can be obtained by navigating to
Settings
->Manage Developer Apps
->Developer Apps
.
Quickstart
To use the Avaza connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/avaza module into the Ballerina project.
import ballerinax/avaza;
Step 2: Create a new connector instance
Add the project configuration file by creating a Config.toml
file. Config file should have following configurations. Add the tokens obtained in the previous step to the Config.toml
file.
[auth] token = "<Bearer_token">
configurable http:BearerTokenConfig & readonly auth = ?; avaza:ClientConfig clientConfig = {auth : auth}; avaza:Client baseClient = check new Client(clientConfig, serviceUrl = "https://api.avaza.com/");
Step 3: Invoke connector operation
- You can get playlist created in your avaza account.
avaza:CompanyList contactGet = check baseClient->companyGet();
- Use
bal run
command to compile and run the Ballerina program.
Clients
avaza: Client
This is a generated connector for Avaza API v1 OpenAPI specification. Avaza API allows to collaborate on projects, chat, schedule resources, track time, manage expenses & invoice customers.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Avaza account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.avaza.com/" - URL of the target service
accountGet
function accountGet() returns AccountDetails|error
Account Details
Return Type
- AccountDetails|error - Success
companyLookup
function companyLookup(int? pageSize, int? pageNumber, string? search) returns CompanyDropdownList|error
Gets minimal list of Companies.
Parameters
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- search string? (default ()) - Search string to match against Company title
Return Type
- CompanyDropdownList|error - OK
companyGet
function companyGet(string? updatedAfter, int? pageSize, int? pageNumber, string? sort) returns CompanyList|error
Gets list of Companies
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- pageSize int? (default ()) - Number of results per page
- pageNumber int? (default ()) - 1 based page number to retrieve
- sort string? (default ()) - (optional) Supply one of: "DateUpdated", "DateCreated", "CompanyName","DateUpdated desc","DateCreated desc", "CompanyName desc"
Return Type
- CompanyList|error - Success
companyPut
function companyPut(UpdateCompany payload) returns Company|error
Update a Company record.
Parameters
- payload UpdateCompany - A record of type
UpdateCompany
which contains the necessary data to update company
companyPost
function companyPost(NewCompany payload) returns Company|error
Create a Company
Parameters
- payload NewCompany - A record of type
NewCompany
which contains the necessary data to create company
companyGetbyid
Gets Company by Company ID
Parameters
- id int - Company ID Number
contactGet
function contactGet(string? updatedAfter, int? pageSize, int? pageNumber, string? sort, int? companyIDFK) returns ContactList|error
Gets list of Contacts
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - Sort method
- companyIDFK int? (default ()) - Company related to contacts
Return Type
- ContactList|error - Success
contactPost
function contactPost(NewCompanyContact payload) returns CompanyContact|error
Create a Contact
Parameters
- payload NewCompanyContact - A record of type
NewCompanyContact
which contains the necessary data to create contact
Return Type
- CompanyContact|error - Success
contactGetbyid
function contactGetbyid(int id) returns CompanyContact|error
Gets Contact by Contact ID
Parameters
- id int - Contact ID number
Return Type
- CompanyContact|error - Success
creditnoteGet
function creditnoteGet(string? updatedAfter, int? pageSize, int? pageNumber) returns CreditNoteList|error
Gets list of CreditNotes
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
Return Type
- CreditNoteList|error - Success
creditnoteGetbyid
function creditnoteGetbyid(int id) returns CreditNote|error
Gets Credit Note by CreditNoteID
Parameters
- id int - Credit Note ID Number
Return Type
- CreditNote|error - Success
currencyGet
function currencyGet() returns CurrencyList|error
Gets list of Currencies
Return Type
- CurrencyList|error - Success
estimateGet
function estimateGet(string? updatedAfter, int? pageSize, int? pageNumber, string? sort, int? companyIDFK) returns EstimateList|error
Gets list of Estimates
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - Sort method
- companyIDFK int? (default ()) - Company related to estimates
Return Type
- EstimateList|error - Success
estimatePost
function estimatePost(NewEstimate payload) returns EstimateDetails|error
Create a new draft Estimate
Parameters
- payload NewEstimate - A record of type
NewEstimate
which contains the necessary data to create a new draft estimate
Return Type
- EstimateDetails|error - OK
estimateGetbyid
Gets Estimate by Estimate ID
Parameters
- id int - Estimate Estimate ID number
expenseAttachment
function expenseAttachment(ExpenseAttachmentBody payload) returns ExpenseAttachmentUploadResult|error
Create expense attachment
Parameters
- payload ExpenseAttachmentBody - Request to create expense attachment
Return Type
expenseApproval
Submit Expenses for Approval.
Parameters
- payload int[] - A collection of ExpenseID's that should be submitted for approval. If not provided, submits all verified expenses for approval.
- userID int? (default ()) - The user to submit the Expenses for. Defaults to current user. Only allowed to be different from the current user when the current user has rights to Impersonate other users.
- sendNotifications boolean? (default ()) - Send email alerts to expense approvers. Defaults to true
Return Type
- json|error - OK
expenseGet
function expenseGet(string? updatedAfter, string? expenseDateFrom, string? expenseDateTo, string? userEmail, int? userID, string? categoryName, int? customerID, int? projectID, boolean? isChargeable, boolean? isInvoiced, int? expenseReimbursementIDFK, int? expensePaymentMethodIDFK, string? expenseApprovalStatusCode, string? search, int? pageSize, int? pageNumber, string? sort) returns ExpenseList|error
Gets list of Expenses
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- expenseDateFrom string? (default ()) - Start date to filter
- expenseDateTo string? (default ()) - End date to filter
- userEmail string? (default ()) - User email address
- userID int? (default ()) - User Id
- categoryName string? (default ()) - Category name
- customerID int? (default ()) - Customer Id
- projectID int? (default ()) - Project Id
- isChargeable boolean? (default ()) - Chargeable status
- isInvoiced boolean? (default ()) - Invoiced status
- expenseReimbursementIDFK int? (default ()) - Expense reimbursement
- expensePaymentMethodIDFK int? (default ()) - Expense payment method
- expenseApprovalStatusCode string? (default ()) - Expense apprval status code
- search string? (default ()) - Search query
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - Sort method
Return Type
- ExpenseList|error - Success
expensePut
function expensePut(UpdateExpense payload) returns ExpenseDetails|error
Update an Expense
Parameters
- payload UpdateExpense - A record of type
UpdateExpense
which contains the necessary data to update expense
Return Type
- ExpenseDetails|error - OK
expensePost
function expensePost(NewExpense payload) returns ExpenseDetails|error
Create an Expense
Parameters
- payload NewExpense - A record of type
NewExpense
which contains the necessary data to create expense
Return Type
- ExpenseDetails|error - OK
expenseDelete
function expenseDelete() returns ExpenseDeleteResultSet|error
Delete a Timesheet Entry
Return Type
expenseGetbyid
function expenseGetbyid(int id) returns ExpenseDetails|error
Gets an Expense Entry by Expense ID
Parameters
- id int - Expense ID number
Return Type
- ExpenseDetails|error - Success
expensecategoryGet
function expensecategoryGet(boolean? isEnabled) returns ExpenseCategoryList|error
Gets list of Expense Categories
Parameters
- isEnabled boolean? (default ()) - Optional filter on for enabled/disabled categories. Defaults to true.
Return Type
- ExpenseCategoryList|error - Success
expenseGroupLookup
function expenseGroupLookup(int? pageSize, int? pageNumber, string? search) returns ExpenseGroupDropdownList|error
Gets minimal list of Expense Groups.
Parameters
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- search string? (default ()) - Search string to match against Expense Group Name
Return Type
expenseMerchangeLookup
function expenseMerchangeLookup(int? pageSize, int? pageNumber, string? search) returns ExpenseMerchantDropdownList|error
Gets minimal list of Expense Merchants.
Parameters
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- search string? (default ()) - Search string to match against Expense Group Name
Return Type
expensePaymentMethodLookup
function expensePaymentMethodLookup() returns ExpensePaymentMethodDropdownList|error
Gets minimal list of Expense Payment Methods.
Return Type
expensesummaryGet
function expensesummaryGet(string[]? modelGroupby, string? modelExpensedatefrom, string? modelExpensedateto, int[]? modelUserid, int? modelProjectid) returns ExpenseSummaryResult|error
Gets Basic Summary of Expense Statistics
Parameters
- modelGroupby string[]? (default ()) - (Optional) Combine one, two or three levels of Grouping. Combine these possible grouping values: "Category", "ChargeableStatus", "Merchant", "ApprovalStatus", "ReimbursementStatus", "Customer", "Project", "User", "Task", "Year", "Month", "Day", "Week".
- modelExpensedatefrom string? (default ()) - (Required) Filter for expenses with expense dates greater or equal to the specified date. e.g. 2019-01-25.
- modelExpensedateto string? (default ()) - (Required) Filter for expenses with an expense date smaller or equal to the specified date. e.g. 2019-01-25.
- modelUserid int[]? (default ()) - (Optional) Defaults to the current user. Provide one or more UserIDs of Users whose expenses should be retrieved. If the current user doesn't have impersonation rights, then they will only see their own data.
- modelProjectid int? (default ()) - (Optional) Filter by Project
Return Type
fixedamountGet
function fixedamountGet(string? updatedAfter, string? entryDateFrom, string? entryDateTo, int? projectID, int? taskID, boolean? isInvoiced, int? pageSize, int? pageNumber, string? sort) returns FixedAmountList|error
Gets list of Fixed Amounts
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- entryDateFrom string? (default ()) - Start date to filter
- entryDateTo string? (default ()) - End date to filter
- projectID int? (default ()) - (Optional) The ProjectID of a Project to filter Fixed Amounts for
- taskID int? (default ()) - (Optional) The TaskID of a Task to filter Fixed Amounts for
- isInvoiced boolean? (default ()) - Invoiced status
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - Optional sorting instruction. Currently possible values: "DateUpdated", "DateCreated", "DateUpdated desc", "DateCreated desc","EntryDate", "EntryDate desc", "StartTimeLocal","StartTimeLocal desc", "TimeSheetEntryID", "TimeSheetEntryID desc"
Return Type
- FixedAmountList|error - Success
inventoryGet
function inventoryGet(string? updatedAfter, int? pageSize, int? pageNumber) returns InventoryList|error
Gets list of Inventory
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
Return Type
- InventoryList|error - Success
inventoryGetbyid
Gets InventoryItem by InventoryItem ID
Parameters
- id int - InventoryItem ID number
invoiceGet
function invoiceGet(string? updatedAfter, int? pageSize, int? pageNumber, string? sort, int? companyIDFK) returns InvoiceList|error
Gets list of Invoices
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - Sort method
- companyIDFK int? (default ()) - Invoice issued company
Return Type
- InvoiceList|error - Success
invoicePost
function invoicePost(NewInvoice payload) returns Invoice|error
Create a new draft invoice
Parameters
- payload NewInvoice - A record of type
NewInvoice
which contains the necessary data to create invoice
invoiceGetbyid
Gets Invoice by Invoice ID
Parameters
- id int - Invoice Transaction ID number
paymentGet
function paymentGet(string? updatedAfter, int? pageSize, int? pageNumber) returns PaymentList|error
Gets list of Payments
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
Return Type
- PaymentList|error - Success
paymentPost
function paymentPost(NewPayment payload) returns Payment|error
Create new Payment and optionally assign payment allocations to Invoices
Parameters
- payload NewPayment - A record of type
NewPayment
which contains the necessary data to create payment
paymentGetbyid
Gets Payment by Payment Transaction ID
Parameters
- id int - Invoice Transaction ID Number
projectLookup
function projectLookup(int? pageSize, int? pageNumber, int? timesheetUserID, int? companyIDFK, string? search) returns ProjectDropdownList|error
Gets minimal list of active Projects for the current user
Parameters
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- timesheetUserID int? (default ()) - Optionally Filter to the projects that the supplied UserID can add timesheets to
- companyIDFK int? (default ()) - Optionally Filter for a specific Company ID
- search string? (default ()) - Search string to match against Project title and Customer name
Return Type
- ProjectDropdownList|error - OK
projectGet
function projectGet(string? updatedAfter, int? pageSize, int? pageNumber, string? sort, int? timesheetUserID, boolean? includeArchived) returns ProjectList|error
Gets list of Projects
Parameters
- updatedAfter string? (default ()) - Only show project records updated after a certain date (UTC)
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - A column to sort on. Current possible values: "DateUpdated", "DateCreated", "DateUpdated desc", "DateCreated desc"
- timesheetUserID int? (default ()) - Filter to the projects that the supplied UserID can add timesheets to
- includeArchived boolean? (default ()) - Include Archived Projects in the results
Return Type
- ProjectList|error - OK
projectPut
function projectPut(UpdateProjectModel payload) returns ProjectDetails|error
Update an Project
Parameters
- payload UpdateProjectModel - A record of type
UpdateProjectModel
which contains the necessary data to update project
Return Type
- ProjectDetails|error - OK
projectPost
function projectPost(NewProjectModel payload) returns ProjectDetails|error
Create a Project
Parameters
- payload NewProjectModel - A record of type
NewProjectModel
which contains the necessary data to create project
Return Type
- ProjectDetails|error - Success
projectGetbyid
function projectGetbyid(int id) returns ProjectDetails|error
Gets Project by Project ID
Parameters
- id int - Project ID number
Return Type
- ProjectDetails|error - Success
projectmemberPost
function projectmemberPost(NewProjectMember payload) returns ProjectMemberDetails|error
Assign a user as a Member of a Project
Parameters
- payload NewProjectMember - A record of type
NewProjectMember
which contains the necessary data to assign user to project
Return Type
projecttimesheetcategoryGet
function projecttimesheetcategoryGet(int? projectID) returns ProjectTimesheetCategoryList|error
Gets list of Project Timesheet Categories
Parameters
- projectID int? (default ()) - Get categories filtered by ProjectID
Return Type
- ProjectTimesheetCategoryList|error - Success
scheduleassignmentGet
function scheduleassignmentGet(string? updatedAfter, string? scheduleDateFrom, string? scheduleDateTo, int? scheduleSeriesID, int? userID, string? userEmail, int? pageSize, int? pageNumber, string? sort) returns ScheduleAssignmentList|error
Gets list of Schedule Assignments.
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- scheduleDateFrom string? (default ()) - Filter for schedule assignement that are on or after a specific date
- scheduleDateTo string? (default ()) - Filter for schedules that are on or before a specific date
- scheduleSeriesID int? (default ()) - Filter to records for a particular Schedule Series
- userID int? (default ()) - The UserID of a schedule user to filter assignments for. Only api users with Admin role can see all schedules across all users. Users with ScheduleUser role can access their own ScheduleSeries.
- userEmail string? (default ()) - The email of the user who has been scheduled
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - Optional sorting instruction. Currently possible values: "DateUpdated", "DateCreated", "DateUpdated desc", "DateCreated desc"
Return Type
- ScheduleAssignmentList|error - Success
scheduleseriesAddbooking
function scheduleseriesAddbooking(CreateBooking payload) returns ScheduleSeriesDetails|error
Create new Schedule Booking
Parameters
- payload CreateBooking - A record of type
CreateBooking
which contains the necessary data to create schedule booking
Return Type
scheduleseriesAddleave
function scheduleseriesAddleave(CreateLeave payload) returns ScheduleSeriesDetails|error
Create new Leave Booking
Parameters
- payload CreateLeave - A record of type
CreateLeave
which contains the necessary data to create leave booking
Return Type
scheduleseriesEditleave
function scheduleseriesEditleave(EditLeave payload) returns ScheduleSeriesDetails|error
Edit Leave Booking
Parameters
- payload EditLeave - A record of type
EditLeave
which contains the necessary data to edit leave booking
Return Type
scheduleseriesEditbooking
function scheduleseriesEditbooking(EditBooking payload) returns ScheduleSeriesDetails|error
Edit Booking
Parameters
- payload EditBooking - A record of type
EditBooking
which contains the necessary data to edit booking
Return Type
scheduleseriesGet
function scheduleseriesGet(string? updatedAfter, string? scheduleStartDateFrom, string? scheduleStartDateTo, string? scheduleEndDateFrom, string? scheduleEndDateTo, int? userID, string? userEmail, int? timeSheetCategoryID, string? timeSheetCategoryName, int? leaveTypeID, int? projectID, int? companyID, int? pageSize, int? pageNumber, string? sort) returns ScheduleSeriesList|error
Gets list of Schedule Series
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- scheduleStartDateFrom string? (default ()) - Filter for schedules that start on or after a specific date
- scheduleStartDateTo string? (default ()) - Filter for schedules that start on or before a specific date
- scheduleEndDateFrom string? (default ()) - Filter for schedules that end on or after a specific date
- scheduleEndDateTo string? (default ()) - Filter for schedules that end on or before a specific date
- userID int? (default ()) - The UserID of a schedule user to filter assignments for. Only api users with Admin role can see all schedules across all users. Users with ScheduleUser role can access their own ScheduleSeries.
- userEmail string? (default ()) - The email of the user who has been scheduled
- timeSheetCategoryID int? (default ()) - Filter for schedule records linked to a specific timesheeet category
- timeSheetCategoryName string? (default ()) - Filter for schedule records with a specific timesheeet category name (exact string match)
- leaveTypeID int? (default ()) - Filter to records of a particular leave type
- projectID int? (default ()) - Filter to only include books linked to a specific project
- companyID int? (default ()) - Filter to only include records linked to projects, where that project belongs to a specific customer company
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - Optional sorting instruction. Currently possible values: "DateUpdated", "DateCreated", "DateUpdated desc", "DateCreated desc"
Return Type
- ScheduleSeriesList|error - Success
sectionGet
function sectionGet(int projectID) returns SectionList|error
Gets list of Sections
Parameters
- projectID int - Get sections for Project with ProjectID
Return Type
- SectionList|error - Success
sectionPost
function sectionPost(NewSection payload) returns SectionDetails|error
Create a Section
Parameters
- payload NewSection - A record of type
NewSection
which contains the necessary data to create section
Return Type
- SectionDetails|error - OK
sectionDelete
Delete a Section
Parameters
- sectionID int - Unique identifier for section
Return Type
- json|error - OK
taskLookup
function taskLookup(int projectID, int? pageSize, int? pageNumber, boolean? hideCompleted, string? search) returns TaskDropdownList|error
Gets minimal list of Tasks for the current user
Parameters
- projectID int - (required) The ProjectID to use when filtering Tasks
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- hideCompleted boolean? (default ()) - (optional) true/false to hide completed tasks. Defaults false
- search string? (default ()) - (optional) Search string to match against Task title. Performs begins-with match
Return Type
- TaskDropdownList|error - OK
taskGet
function taskGet(string? updatedAfter, int? pageSize, int? pageNumber, string? sort, boolean? isComplete, int? projectID) returns TaskList|error
Gets list of Tasks
Parameters
- updatedAfter string? (default ()) - Optional filter to records updated after a specific date.
- pageSize int? (default ()) - Number of items per page. Defaults to 20.
- pageNumber int? (default ()) - Page to display. Starts from 1. Defaults to 1
- sort string? (default ()) - Optional sorting instruction. Currently possible values: "DateUpdated", "DateCreated", "DateUpdated desc", "DateCreated desc", "SectionTitle", "Title"
- isComplete boolean? (default ()) - Optional filter to only display tasks linked to a Task Status where isComplete=false, or where isComplete=true
- projectID int? (default ()) - Optional filter to only display tasks belonging to a specific ProjectID
taskPut
function taskPut(UpdateTask payload) returns TaskDetails|error
Update a Task.
Parameters
- payload UpdateTask - A record of type
UpdateTask
which contains the necessary data to update task
Return Type
- TaskDetails|error - OK
taskPost
function taskPost(NewTask payload) returns TaskDetails|error
Create a Task
Parameters
- payload NewTask - A record of type
NewTask
which contains the necessary data to create task
Return Type
- TaskDetails|error - OK
taskDelete
Delete a Task
Parameters
- taskID int - Task Id
Return Type
- json|error - OK
taskGetbyid
function taskGetbyid(int id) returns TaskDetails|error
Gets Task by Task ID
Parameters
- id int - Task ID number
Return Type
- TaskDetails|error - Success
taskstatusGet
function taskstatusGet() returns TaskStatusList|error
Gets list of Task Statuses
Return Type
- TaskStatusList|error - Success
tasktypeGet
function tasktypeGet() returns TaskTypeList|error
Gets list of Task Types
Return Type
- TaskTypeList|error - Success
taxGet
Get List of Taxes configured in the Avaza account.
timesheetGet
function timesheetGet(string? updatedAfter, string? entryDateFrom, string? entryDateTo, int? userID, string? userEmail, string? categoryName, int? projectID, boolean? isBillable, boolean? isInvoiced, boolean? isTimerRunning, int? pageSize, int? pageNumber, string? sort) returns TimesheetList|error
Gets list of Timsheets
Parameters
- updatedAfter string? (default ()) - Limit results to records updated after the specified date
- entryDateFrom string? (default ()) - Timesheet start entry date to filter
- entryDateTo string? (default ()) - Timesheet end entry date to filter
- userID int? (default ()) - The UserID of a timesheet user to filter timesheets for. Only api users with certain higher roles can see timesheets across multiple users.
- userEmail string? (default ()) - User email address
- categoryName string? (default ()) - Category name
- projectID int? (default ()) - Project Id
- isBillable boolean? (default ()) - Billable status
- isInvoiced boolean? (default ()) - Invoiced status
- isTimerRunning boolean? (default ()) - Timer running status
- pageSize int? (default ()) - Number of items per page (max 1000)
- pageNumber int? (default ()) - Page to display. Starts from 1.
- sort string? (default ()) - Optional sorting instruction. Currently possible values: "DateUpdated", "DateCreated", "DateUpdated desc", "DateCreated desc","EntryDate", "EntryDate desc", "StartTimeLocal","StartTimeLocal desc", "TimeSheetEntryID", "TimeSheetEntryID desc"
Return Type
- TimesheetList|error - Success
timesheetPut
function timesheetPut(UpdateTimesheetModel payload) returns TimesheetDetails|error
Update a Timesheet
Parameters
- payload UpdateTimesheetModel - A record of type
UpdateTimesheetModel
which contains the necessary data to update timesheet
Return Type
- TimesheetDetails|error - OK
timesheetPost
function timesheetPost(NewTimesheet payload) returns TimesheetDetails|error
Create a new Timesheet Entry
Parameters
- payload NewTimesheet - A record of type
NewTimesheet
which contains the necessary data to create timesheet
Return Type
- TimesheetDetails|error - OK
timesheetGetbyid
function timesheetGetbyid(int id) returns TimesheetDetails|error
Gets a Timesheet Entry by Timesheet ID
Parameters
- id int - Timesheet ID number
Return Type
- TimesheetDetails|error - Success
timesheetDelete
Delete a Timesheet Entry
Parameters
- id int - The id of the timesheet entry to be deleted
Return Type
- json|error - OK
timesheetsubmissionPost
function timesheetsubmissionPost(boolean? sendNotifications, string? wholeWeekOf, string? wholeDayOf, int? userID) returns json|error
Submit Timesheets for Approval.
Parameters
- sendNotifications boolean? (default ()) - Send email alerts to timesheet approvers. Defaults to true
- wholeWeekOf string? (default ()) - A date (yyyy-MM-dd) that falls within a Week to have all timesheets in that week submitted. Respects the First Day of Week setting in your account Timesheet Settings to determine the week range.
- wholeDayOf string? (default ()) - A date (yyyy-MM-dd) to submit all timesheets on this day
- userID int? (default ()) - The user to submit timesheets for. Defaults to current user. Only allowed to be different from the current user when the current user has rights to Impersonate other users.
Return Type
- json|error - OK
timesheetsummaryGet
function timesheetsummaryGet(string[]? modelGroupby, string? modelEntrydatefrom, string? modelEntrydateto, int[]? modelUserid, int? modelProjectid, boolean? modelIsbillable, boolean? modelIsinvoiced) returns TimesheetSummaryResult|error
Gets Basic Summary of Timesheet Statistics
Parameters
- modelGroupby string[]? (default ()) - (Optional) Combine one, two or three levels of Grouping. Combine these possible grouping values: "Customer", "Project", "Category", "User", "Task", "Year", "Month", "Day", "Week".
- modelEntrydatefrom string? (default ()) - (Required) Filter for timesheets greater or equal to the specified date. e.g. 2019-01-25. You can optionally include a time component, otherwise it assumes 00:00
- modelEntrydateto string? (default ()) - (Required) Filter for timesheets with an entry date smaller or equal to the specified date. e.g. 2019-01-25. You can optionally include a time component, otherwise it assumes 00:00
- modelUserid int[]? (default ()) - (Optional) Defaults to the current user. Provide one or more UserIDs of Users whose timesheets should be retrieved. If the current user doesn't have impersonation rights, then they will only see their own data.
- modelProjectid int? (default ()) - (Optional) Filter by Project
- modelIsbillable boolean? (default ()) - (Optional) Filter by the billable status of Timesheets.
- modelIsinvoiced boolean? (default ()) - (Optional) Filter for timesheets by whether they have been Invoiced or not.
Return Type
timesheettimerGetrunningtimer
Gets the Running Timer if there is one for a user.
Parameters
- userID int? (default ()) - Optional - User ID number if impersonating a different user. Otherwise assumes the current user. Only users with certain security roles have permission to impersonate other users
Return Type
- json|error - Success
timesheettimerStarttimer
Starts a Timer running on an existing Timesheet Entry
Parameters
- id int - id of timesheet entry that should be used as the basis for running a timer. If the existing timesheet is not on the current day, or you have start/end times enabled, then a new timesheet will be created for the timer.
- userID int? (default ()) - Optional - User ID number if impersonating a different user. Otherwise assumes the current user. Only users with certain security roles have permission to impersonate other users
Return Type
- json|error - Success
timesheettimerStoptimer
Stop the timer running on an existing Timesheet Entry
Parameters
- id int - The ID of the existing timesheet entry that needs its timer stopped
- userID int? (default ()) - Optional - User ID number if impersonating a different user. Otherwise assumes the current user. Only users with certain security roles have permission to impersonate other users
Return Type
- json|error - Success
userprofileGet
function userprofileGet(string? roles, string? tags, boolean? currentUserOnly, int? companyIDFK) returns UserList|error
Get Collection of Users who have roles in the current Avaza account.
Parameters
- roles string? (default ()) - Optional list of comma separated role codes to filter users by (e.g. "TimesheetUser,Admin")
- tags string? (default ()) - User role tags
- currentUserOnly boolean? (default ()) - Optional boolean (true/false) to filter to only show current authenticated user (always true for non Admin/InvoiceManager users)
- companyIDFK int? (default ()) - Optionally filter by Company ID
webhookGet
function webhookGet() returns WebhookList|error
Get list of Webhook Subscriptions
Return Type
- WebhookList|error - OK
webhookPost
function webhookPost(CreateSubscription payload) returns SubscribeResult|error
Subscribe to Webhook. On success, returns ID of webhook subscription.
Parameters
- payload CreateSubscription - A record of type
CreateSubscription
which contains the necessary data to subscribe to webhook
Return Type
- SubscribeResult|error - Subscription created
webhookDeletebyurl
Delete webhook subscription by URL
Parameters
- targetUrl string - Target URL that should be used to delete subscriptions
Return Type
- json|error - Subscription Deleted OK
webhookGetbyid
function webhookGetbyid(int id) returns WebhookList|error
Get Webhook Subscription by SubscriptionID
Parameters
- id int - Subscription Id
Return Type
- WebhookList|error - OK
webhookDelete
Delete Webhook Subscription by ID
Parameters
- id int - Subscription id to be deleted
Return Type
- json|error - Subscription deleted ok
Records
avaza: AccountDetails
Fields
- AccountID int? -
- CompanyName string? -
- Subdomain string? -
- AccountEmail string? -
- hasStartEndTimesheets boolean? -
- has24HourTimesheetFormat boolean? -
- WeeklyTimesheetReminder boolean? -
- LockApprovedTimesheets boolean? -
- TimesheetDayOfWeek int? -
- TimesheetDisplayFormatCode string? -
- AllowHidingCompletedTasksOnTimesheet boolean? -
- CurrentServerTimeISO string? - Format: ISO "YYYY-MM-DD HH:mm:ss.SSS"
- SC string? -
- DefaultCurrencyCode string? - ISO 3 letter base Currency Code for the account
- ExpenseApprovalRequired boolean? - Whether the account requires expenses be approved.
- LockApprovedExpenses boolean? - Approved expenses get locked from subsequent editing by standard expense users.
avaza: AccountTaskTypeDetails
Fields
- AccountTaskTypeID int? -
- Name string? -
- Icon string? -
- IconType string? -
- isDefault boolean? -
avaza: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
avaza: Company
Fields
- CompanyID int? -
- CompanyName string? -
- BillingAddressLine string? -
- BillingAddressCity string? -
- BillingAddressState string? -
- BillingAddressPostCode string? -
- BillingCountryCode string? -
- BillingAddress string? -
- Phone string? -
- Fax string? -
- website string? -
- TaxNumber string? -
- Comments string? -
- CurrencyCode string? -
- DefaultTradingTermIDFK int? -
- DateCreated string? -
- DateUpdated string? -
- Contacts CompanyContact[]? -
avaza: CompanyContact
Fields
- ContactID int? -
- CompanyIDFK int? -
- CompanyName string? -
- Firstname string? -
- Lastname string? -
- Email string? -
- Phone string? -
- Mobile string? -
- PositionTitle string? -
- TimeZone string? -
- DateCreated string? -
- DateUpdated string? -
avaza: CompanyDropdownList
Fields
- Companies CompanyMinimal[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: CompanyList
Fields
- Companies Company[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: CompanyMinimal
Fields
- CompanyID int? -
- CompanyName string? -
avaza: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
avaza: ContactList
Fields
- Contacts CompanyContact[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: CreateBooking
Fields
- UserIDFK int? -
- HoursPerDay decimal? -
- TotalDuration decimal? -
- DurationType string? -
- ScheduleOnDaysOff boolean? -
- ProjectIDFK int? -
- CategoryIDFK int? -
- TaskIDFK int? -
- Notes string? -
- StartDate string? -
- EndDate string? -
avaza: CreateLeave
Fields
- LeaveUserIDFK int? -
- LeaveNotify boolean? -
- LeaveHoursPerDay decimal? -
- LeaveTypeIDFK int? -
- LeaveNotes string? -
- LeaveStartDate string? -
- LeaveEndDate string? -
avaza: CreateSubscription
New Subscription to be Created
Fields
- target_url string - The URL that should be notified of the event.
- event string - The event code to be notified about. Possible values: company_created, contact_created, invoice_created, invoice_sent, project_created, task_created
- secret string? - Optional Secret string (255 char max). If provided, the secret will be BASE 64 encoded and used as a basic authentication http header with webhook notifications. i.e. Authorization Basic [BASE64 of Secret]"
avaza: CreditNote
Fields
- TransactionID int? -
- TransactionPrefix string? -
- CreditNoteNumber string? -
- CustomerIDFK int? -
- DateIssued string? -
- TransactionStatusCode string? -
- Balance decimal? -
- CurrencyCode string? -
- TotalAmount decimal? -
- Notes string? -
- DateCreated string? -
- DateUpdated string? -
- CreditNoteAllocations CreditNoteAllocation[]? -
- CreditNoteLineItems CreditNoteLineItem[]? -
avaza: CreditNoteAllocation
Fields
- TransactionAllocationID int? -
- InvoiceTransactionIDFK int? -
- CreditNoteTransactionIDFK int? -
- AllocationDate string? -
- AllocationAmount decimal? -
avaza: CreditNoteLineItem
Fields
- TransactionLineItemID int? -
- Description string? -
- Quantity decimal? -
- UnitPrice decimal? -
- TaxAmount decimal? -
- TaxIDFK int? -
- Amount decimal? -
- Discount decimal? -
avaza: CreditNoteList
Fields
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
- CreditNotes CreditNote[]? -
avaza: Currency
Fields
- CurrencyCode string? -
- Name string? -
- Symbol string? -
- Symbol2 string? -
- DecimalPlaces int? -
avaza: CurrencyList
Fields
- Currencies Currency[]? -
avaza: EditBooking
Fields
- ScheduleSeriesID int? -
- UserIDFK int? -
- HoursPerDay decimal? -
- TotalDuration decimal? -
- DurationType string? -
- ScheduleOnDaysOff boolean? -
- ProjectIDFK int? -
- CategoryIDFK int? -
- TaskIDFK int? -
- Notes string? -
- StartDate string? -
- EndDate string? -
avaza: EditLeave
Fields
- ScheduleSeriesID int? -
- UserIDFK int? -
- HoursPerDay decimal? -
- LeaveTypeIDFK int? -
- Notes string? -
- StartDate string? -
- EndDate string? -
avaza: EstimateDetails
Fields
- EstimateID int? -
- AccountIDFK int? -
- EstimatePrefix string? -
- EstimateItemNumber string? -
- CompanyIDFK int? -
- CompanyName string? -
- Subject string? -
- DateIssued string? -
- DateSent string? -
- DueDate string? -
- EstimateStatusCode string? -
- TaxAmount decimal? -
- EstimateTaxConfigCode string? -
- Balance decimal? -
- CurrencyCode string? -
- TotalAmount decimal? -
- ExchangeRate decimal? -
- Notes string? -
- DateCreated string? -
- DateUpdated string? -
- LineItems EstimateLineItemDetails[]? -
- Links EstimateLinks? -
- Issuer IssuerDetails? -
- Recipient RecipientDetails? -
avaza: EstimateLineItemDetails
Fields
- EstimateLineItemID int? -
- InventoryItemIDFK int? -
- InventoryItemName string? -
- InventoryItemSKU string? -
- Description string? -
- Quantity decimal? -
- UnitPrice decimal? -
- TaxAmount decimal? -
- TaxIDFK int? -
- TaxCode string? -
- TaxName string? -
- Amount decimal? -
- Discount decimal? -
avaza: EstimateLinks
Fields
- ClientView string? -
- View string? -
- Edit string? -
avaza: EstimateList
Fields
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
- Estimates EstimateDetails[]? -
avaza: ExpenseAttachmentBody
Fields
- File string - Upload software package
avaza: ExpenseAttachmentUploadResult
Fields
- FileAttachments FileAttachmentDetails[]? -
avaza: ExpenseCategoryDetails
Fields
- ExpenseCategoryID int? -
- Name string? -
- Enabled boolean? -
- hasUnitPrice boolean? -
- UnitPrice decimal? -
- UnitName string? -
avaza: ExpenseCategoryList
Fields
- Categories ExpenseCategoryDetails[]? -
avaza: ExpenseDeleteResult
Fields
- ExpenseID int? -
- Success boolean? -
- ErrorMessage string? -
avaza: ExpenseDeleteResultSet
Fields
- Results ExpenseDeleteResult[]? -
avaza: ExpenseDetails
Fields
- ExpenseID int? -
- UserIDFK int? -
- Firstname string? -
- Lastname string? -
- Email string? -
- CustomerIDFK int? -
- CustomerName string? -
- ProjectIDFK int? -
- ProjectTitle string? -
- ProjectCode string? -
- TaskIDFK int? -
- TaskTitle string? -
- ExpenseCategoryIDFK int? -
- ExpenseCategoryName string? -
- ExpenseCategoryHasUnitPrice boolean? -
- ExpenseCategoryUnitPrice decimal? -
- ExpenseCategoryUnitName string? -
- CurrencyCode string? -
- ExchangeRate decimal? -
- Quantity decimal? -
- Amount decimal? -
- TaxAmount decimal? -
- TaxIDFK int? -
- TaxName string? -
- TransactionTaxConfigCode string? -
- TransactionTaxConfigName string? -
- isOfficialExchangeRate boolean? -
- ExpenseApprovalStatusCode string? -
- ExpensePaymentMethodIDFK int? -
- ExpensePaymentMethodName string? -
- isChargeable boolean? -
- ChargeableStatusCode string? -
- isReimbursable boolean? -
- ExpenseReimbursementStatusCode string? -
- ExpenseReimbursementIDFK int? -
- ExpenseDate string? -
- FileAttachmentIDFK int? -
- AttachmentURL string? -
- AttachmentPreviewURL string? -
- Merchant string? -
- MerchantTaxNumber string? -
- Notes string? -
- ExpenseReportIDFK int? -
- ExpenseReportName string? -
- DateCreated string? -
- DateUpdated string? -
avaza: ExpenseGroupDropdownList
Fields
- ExpenseGroups ExpenseGroupMinimal[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: ExpenseGroupMinimal
Fields
- ExpenseGroupID int? -
- Name string? -
avaza: ExpenseList
Fields
- Expenses ExpenseDetails[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: ExpenseMerchantDropdownList
Fields
- ExpenseMerchants ExpenseMerchantMinimal[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: ExpenseMerchantMinimal
Fields
- MerchantName string? -
avaza: ExpensePaymentMethodDropdownList
Fields
- ExpensePaymentMethods ExpensePaymentMethodMinimal[]? -
avaza: ExpensePaymentMethodMinimal
Fields
- ExpensePaymentMethodID int? -
- Name string? -
avaza: ExpenseSummaryGroup
Fields
- GroupID string? -
- GroupName string? -
- TotalAmount decimal? -
- GroupData ExpenseSummaryGroup[]? -
avaza: ExpenseSummaryRequest
Fields
- GroupBy string[]? - (Optional) Combine one, two or three levels of Grouping. Combine these possible grouping values: "Category", "ChargeableStatus", "Merchant", "ApprovalStatus", "ReimbursementStatus", "Customer", "Project", "User", "Task", "Year", "Month", "Day", "Week".
- ExpenseDateFrom string? - (Required) Filter for expenses with expense dates greater or equal to the specified date. e.g. 2019-01-25.
- ExpenseDateTo string? - (Required) Filter for expenses with an expense date smaller or equal to the specified date. e.g. 2019-01-25.
- UserID int[]? - (Optional) Defaults to the current user. Provide one or more UserIDs of Users whose expenses should be retrieved. If the current user doesn't have impersonation rights, then they will only see their own data.
- ProjectID int? - (Optional) Filter by Project
avaza: ExpenseSummaryResult
Fields
- ExpenseDateFrom string? -
- ExpenseDateTo string? -
- TotalAmount decimal? -
- UserID int[]? -
- GroupingLevels string[]? -
- GroupData ExpenseSummaryGroup[]? -
avaza: FileAttachmentDetails
Fields
- FileAttachmentID int? -
- SizeBytes int? -
- OriginalFilename string? -
- PublicFileURL string? -
- PreviewBaseURL string? -
avaza: FixedAmountDetails
Fields
- FixedAmountID int? -
- ProjectIDFK int? -
- ProjectTitle string? -
- ProjectCode string? -
- TaskIDFK int? -
- TaskTitle string? -
- InventoryItemIDFK int? -
- InventoryItemName string? -
- Amount decimal? -
- Notes string? -
- isInvoiced boolean? -
- DateCreated string? -
- DateUpdated string? -
- UpdatedByUserIDFK int? -
avaza: FixedAmountList
Fields
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
- FixedAmounts FixedAmountDetails[]? -
avaza: InventoryItem
Fields
- InventoryItemID int? -
- Name string? -
- Description string? -
- SKU string? -
- CostPrice decimal? -
- SalePrice decimal? -
- SaleTaxIDFK int? -
- isHidden boolean? -
- DateCreated string? -
- DateUpdated string? -
avaza: InventoryList
Fields
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
- Inventory InventoryItem[]? -
avaza: Invoice
Fields
- TransactionID int? -
- AccountIDFK int? -
- TransactionPrefix string? -
- InvoiceNumber string? -
- CompanyIDFK int? -
- CompanyName string? -
- Subject string? -
- DateIssued string? -
- DateSent string? -
- DueDate string? -
- TransactionStatusCode string? -
- TaxAmount decimal? -
- TransactionTaxConfigCode string? -
- Balance decimal? -
- CurrencyCode string? -
- TotalAmount decimal? -
- ExchangeRate decimal? -
- Notes string? -
- CustomerPONumber string? -
- DateCreated string? -
- DateUpdated string? -
- LineItems InvoiceLineItem[]? -
- Links InvoiceLinks? -
- Issuer IssuerDetails? -
- Recipient RecipientDetails? -
avaza: InvoiceLineItem
Fields
- TransactionLineItemID int? -
- InventoryItemIDFK int? -
- InventoryItemName string? -
- InventoryItemSKU string? -
- Description string? -
- Quantity decimal? -
- UnitPrice decimal? -
- TaxAmount decimal? -
- TaxIDFK int? -
- TaxCode string? -
- TaxName string? -
- ProjectIDFK int? -
- ProjectTitle string? -
- Amount decimal? -
- Discount decimal? -
avaza: InvoiceLinks
Fields
- ClientView string? -
- View string? -
- Edit string? -
avaza: InvoiceList
Fields
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
- Invoices Invoice[]? -
avaza: IssuerDetails
Fields
- BillingAddress string? -
- BillingAddressLine string? -
- BillingAddressCity string? -
- BillingAddressState string? -
- BillingAddressPostCode string? -
- BillingCountryCode string? -
- TaxNumber string? -
avaza: NewCompany
Fields
- CompanyName string -
- CurrencyCode string? -
- BillingAddressLine string? -
- BillingAddressCity string? -
- BillingAddressState string? -
- BillingAddressPostCode string? -
- BillingCountryCode string? -
- BillingAddress string? -
- Phone string? -
- Fax string? -
- website string? -
- TaxNumber string? -
- Comments string? -
avaza: NewCompanyContact
Fields
- CompanyIDFK int? -
- CompanyName string? -
- CurrencyCode string? -
- CompanyBillingAddress string? -
- CompanyBillingAddressLine string? -
- CompanyBillingAddressCity string? -
- CompanyBillingAddressState string? -
- CompanyBillingAddressPostCode string? -
- CompanyBillingAddressCountryCode string? -
- ContactEmail string -
- Firstname string -
- Lastname string -
- PositionTitle string? -
- Mobile string? -
- Phone string? -
- UpdateExisting boolean? -
avaza: NewEstimate
New Estimate to be created
Fields
- EstimatePrefix string? - A prefix for the Estimate number. e.g. 'INV'. If left blank it will be set to the account default. Max length 20 characters.
- EstimateNumber string? - Pass any string. If left blank it will use the next number in the auto incrementing sequence. If an integer is passed then the largest integer will be use as the seed to auto generate the next Estimate number in the sequence.
- CompanyIDFK int? - If left blank then you must specify Company Name.
- CompanyName string? - If left blank then you must specify Company ID. Specified Name will be used to match existing customer record. If not matched then it will be used to create a new customer. First Name, Last Name and Email will only be used if it is a new company. If the Company name appears multiple times we will check the email address to find a matching company. If email address doesn't identify a matching company then the Estimate creation will be rejected.
- Firstname string? - Specified value will be used to create a new customer contact only if a new customer is being created.
- Lastname string? - Specified value will be used to create a new customer contact only if a new customer is being created.
- Email string? - Specified value will be used to create a new customer contact only if a new customer is being created.
- CurrencyCode string? - Expects ISO Standard 3 character currency code. If left blank the currency will default to account's currency in general setting. For existing companies this field will be ignored and the Estimate will use the currency of the customer. For new customers if the currency is not specified then account currency will be used otherwise the specified currency will be used.
- ExchangeRate decimal? - Exchange rate is only valid for Estimates in currency other than default account currency. If not specified it will get the market rate based on the Date Issued.
- InvoiceTemplateIDFK int? - If left blank the account default Estimate template will be used.
- Subject string? - Plain UTF8 text. (no HTML). 255 characters max
- CustomerPONumber string? - Plain UTF8 text. 100 characters max
- DateIssued string? - If not specified it will use today's date. The date should be specified as local date.
- DueDate string? - It will be auto calculated based on the payment term and issue date. Due Date must be greater than or equal to Issue Date. If the Due Date is specified then Payment Terms will be set to -1 (Custom)
- EstimateTaxConfigCode string? - Possible values are (EX --- Tax Exclusive, INC --- Tax Inclusive). If left empty it will use the account default.
- Notes string? - Plain UTF8 text. (no HTML). Max 2000 characters
- LineItems NewEstimateLineItem[]? - Line item to be added to new Estimate
avaza: NewEstimateLineItem
Line item to be added to new Estimate
Fields
- InventoryItemIDFK int? - If not specified then Inventory Item Name must be specified.
- InventoryItemName string? - If not specified then Inventory item ID must be specified. If specified and not matched to any existing inventory items then a new inventory item will be created. Max 200 characters.
- Description string? - Plain UTF8 text. (no HTML)
- Quantity decimal - The quantity for the line item
- UnitPrice decimal - The unit price for the lineitem.
- TaxIDFK int? - If specified then it must match an existing Tax ID. If not specified then Tax Name and Tax Percent must be specified.
- TaxName string? - Must be specified if the Tax ID is blank. If the Tax Name is specified it will be matched to an existing Tax Name or else a new Tax will be created.
- TaxPercent decimal? - The Tax Percent will only be used if a new tax is being created.
- Discount decimal? - Enter 10.5 to give a 10.5% discount
avaza: NewExpense
Fields
- ExpenseDate string? - The date of the expense entry (Required)
- UserIDFK int? - UserID for a Timesheet/Expense user in Avaza. If not provided, UserEmail field must be provided
- UserEmail string? - The email address of a Timesheet/Expense user in Avaza. If not provided, UserIDFK field must be provided.
- ExpenseCategoryIDFK int? - The expense category to link the Expense to. If not provided, ExpenseCategoryName must be provided
- ExpenseCategoryName string? - Must match an existing expense category name otherwise a new category will be created. If left blank Expense Category ID must be provided.
- isChargeable boolean? - aka Billable. Defaults to false if not provided. If set to true, a CustomerIDFK or CustomerName must be provided.
- isReimbursable boolean? - Defaults to false if not provided.
- Quantity decimal? - Conditional - available for expenses that are assigned a unit priced based expense category. e.g Mileage
- CustomerIDFK int? - The Avaza Customer ID to associate the Expense with. Either this field or CustomerName can be provided.
- CustomerName string? - The name of an existing customer in Avaza. Must be an exact (case insensitive) match.
- ProjectIDFK int? - The Avaza project ID to associate the Expense with.
- ProjectName string? - Can work for matching an expense to a project, but only if it's an exact match for a single project under the customer.
- TaskIDFK int? - (optional) TaskID of a Task to link the new Expense to. A Customer and Project must be provided also.
- CurrencyCode string? - A 3-letter ISO CurrencyCode for the expense currency. (e.g. USD). If not provided, defaults to the Account base currency.
- ExchangeRate decimal? - Optional (Only relevant if the expense currency is different to your account currency. If not provided we will look up the market exchange rate for you based on the expense date.) Exchange Rate = Expense Currency Amount / Base Currency Amount (e.g. if Expense currency is in AUD, and Base Currency is in USD, Exchange Rate = AUD $140 / USD $100 = 1.4)
- Amount decimal? - Expense Amount (Required). Must be >= 0
- TaxIDFK int? - Avaza Tax ID the expense belongs to. If left blank then Tax Name must be provided.
- TaxName string? - Must exactly match an existing Tax Name that you have configured in Avaza Tax settings. If left blank then Tax ID must be provided.
- TransactionTaxConfigCode string? - Optional - Enter "INC" if the tax amount is included in the expense amount otherwise enter "EX" when the amount exlcudes the tax. Defaults to "Ex". The tax amount on the expense will be autocalculated.
- GroupTripName string? - Links the expense to a Grouping/Trip report. If no matching name found, creates a new Group/Trip Report name.
- ExpensePaymentMethodIDFK int? - (Optional) ID of Expense Payment Method.
- Merchant string? - The name of the merchant.
- MerchantTaxNumber string? - A Tax number identifier for the merchant.
- Notes string? - Expense Notes
- VerifyAndSave boolean? - Pass false if creating a draft expense. True otherwise.
- FileAttachmentIDs int[]? - Array of File Attachment IDs to associate with this expense. The files need to have already been uploaded. Currently only accepts a single file.
avaza: NewInvoice
New invoice to be created
Fields
- TransactionPrefix string? - A prefix for the Invoice number. e.g. 'INV'. If left blank it will be set to the account default. Max length 20 characters.
- InvoiceNumber string? - Pass any string. If left blank it will use the next number in the auto incrementing sequence. If an integer is passed then the largest integer will be use as the seed to auto generate the next invoice number in the sequence.
- CompanyIDFK int? - If left blank then you must specify Company Name.
- CompanyName string? - If left blank then you must specify Company ID. Specified Name will be used to match existing customer record. If not matched then it will be used to create a new customer. First Name, Last Name and Email will only be used if it is a new company. If the Company name appears multiple times we will check the email address to find a matching company. If email address doesn't identify a matching company then the invoice creation will be rejected.
- Firstname string? - Specified value will be used to create a new customer contact only if a new customer is being created.
- Lastname string? - Specified value will be used to create a new customer contact only if a new customer is being created.
- Email string? - Specified value will be used to create a new customer contact only if a new customer is being created.
- CurrencyCode string? - Expects ISO Standard 3 character currency code. If left blank the currency will default to account's currency in general setting. For existing companies this field will be ignored and the invoice will use the currency of the customer. For new customers if the currency is not specified then account currency will be used otherwise the specified currency will be used.
- ExchangeRate decimal? - Exchange rate is only valid for invoices in currency other than default account currency. If not specified it will get the market rate based on the Date Issued.
- InvoiceTemplateIDFK int? - If left blank the account default invoice template will be used.
- Subject string? - Plain UTF8 text. (no HTML). 255 characters max
- CustomerPONumber string? - Plain UTF8 text. 100 characters max
- DateIssued string? - If not specified it will use today's date. The date should be specified as local date.
- PaymentTerms int? - "If left blank we will set it to customer default. If specified then it must match one of your existing pre configured payment term periods. Your account starts with: (-1 --- Custom, 0 --- Upon Receipt, 7 --- 7 Days, 15 --- 15 Days, 30 --- 30 Days, 45 --- 45 Days, 60 --- 60 Days)
- DueDate string? - It will be auto calculated based on the payment term and issue date. Due Date must be greater than or equal to Issue Date. If the Due Date is specified then Payment Terms will be set to -1 (Custom)
- TransactionTaxConfigCode string? - Possible values are (EX --- Tax Exclusive, INC --- Tax Inclusive). If left empty it will use the account default.
- Notes string? - Plain UTF8 text. (no HTML). Max 2000 characters
- LineItems NewInvoiceLineItem[]? - Line item to be added to new invoice
avaza: NewInvoiceLineItem
Line item to be added to new invoice
Fields
- InventoryItemIDFK int? - If not specified then Inventory Item Name must be specified.
- InventoryItemName string? - If not specified then Inventory item ID must be specified. If specified and not matched to any existing inventory items then a new inventory item will be created. Max 200 characters.
- Description string? - Plain UTF8 text. (no HTML)
- Quantity decimal - The quantity for the line item
- UnitPrice decimal - The unit price for the lineitem.
- TaxIDFK int? - If specified then it must match an existing Tax ID. If not specified then Tax Name and Tax Percent must be specified.
- TaxName string? - Must be specified if the Tax ID is blank. If the Tax Name is specified it will be matched to an existing Tax Name or else a new Tax will be created.
- TaxPercent decimal? - The Tax Percent will only be used if a new tax is being created.
- Discount decimal? - Enter 10.5 to give a 10.5% discount
- ProjectIDFK int? - Optional. Project ID of an Avaza Project that belongs to this customer, so line item is attributed to that Project for reporting.
avaza: NewPayment
Fields
- Amount decimal? -
- PaymentNumber string? - Optional. If not specified will be automatically generated
- DateIssued string? - Date of Payment. If not specified, assumes today.
- TransactionPrefix string? - Optional to override the default prefix added to Payment Numbers
- CustomerIDFK int? - Only required if no invoice allocations specified.
- ExchangeRate decimal? - Optional. Only used when the Customer's currecy is different from the Avaza account's base currency. Specifies the exchange rate that should apply between the customer currency and base currency. If not provided we will obtain an up to date exchange rate for the Payment Issue Date.
- TransactionReference string? - Optional for storing the reference # of the payment method.
- Notes string? -
- PaymentProviderCode string? - Optional for storing the payment provider who was the source of funds.
- PaymentAllocations NewPaymentAllocation[]? - List of amounts within this payment that are allocated to invoices. The sum of these be less than or equal to the payment amount.
avaza: NewPaymentAllocation
Fields
- InvoiceTransactionIDFK int? - The Avaza Invoice TransactionID that is having a payment amount allocated to it.
- AllocationAmount decimal? - The Amount being allocated to the invoice. Expects same currency as invoice currency
- AllocationDate string? - Optional. Defaults to the current time in the Avaza account's timezone. The date the allocation is applied to the invoice. Can be difference from the Payment Date when doing prepayments etc.
avaza: NewProjectMember
Fields
- isProjectManager boolean? -
- isTimesheetAllowed boolean? -
- isTimesheetApprover boolean? -
- isTimesheetApprovalRequired boolean? -
- canCreateTasks boolean? -
- canDeleteTasks boolean? -
- canCommentOnTasks boolean? -
- canUpdateTasks boolean? -
- ProjectIDFK int? - Required. The ProjectID
- UserIDFK int? - Required. The UserID to assign
- CostAmount decimal? - Optional. If not provided, defaults to the User's default Cost Amount.
- RateAmount decimal? - Optional. If not provided, defaults to the User's default Rate Amount.
- BudgetAmount decimal? - Optional
avaza: NewProjectModel
Fields
- CompanyIDFK int? - An ID of a company in Avaza to create the Project under. You must provide either a CompanyID, or a CompanyName
- CompanyName string? - The name for a Company to create the project under. Will create company unless it matches an existing company name
- CurrencyCode string? - The ISO 3 letter currency code to use when creating a new Company. If not provided, the account's default currency will be used.
- ProjectTitle string - The title of the new project. (255 characters max)
- ProjectCode string? - Used when Manual Project Codes are enabled
- ProjectNotes string? - Any descriptive notes about the project. (2000 characters max)
- TimesheetApprovalRequiredbyDefault boolean? -
- PopulateDefaultProjectMembers boolean? - Defaults to true.
- isTaskRequiredOnTimesheet boolean? -
- StartDate string? -
- EndDate string? -
- BudgetAmount decimal? -
- BudgetHours decimal? -
- ProjectStatusCode string? -
- ProjectCategoryIDFK int? -
avaza: NewSection
Fields
- ProjectIDFK int? -
- Title string? -
- StartDateUTC string? -
- EndDateUTC string? -
avaza: NewTag
Fields
- Name string? -
- Color string? - Hex color code in format #000000
avaza: NewTask
Fields
- ProjectIDFK int -
- SectionIDFK int -
- AccountTaskTypeIDFK int? -
- Title string -
- Description string? -
- AssignedToUserIDFK int? -
- TaskPriorityCode string? -
- DateStart string? -
- DateDue string? -
- EstimatedEffort decimal? - Decimal hours
- Tags NewTag[]? - Collection of tags specifying Name and Color (Hex)
avaza: NewTimesheet
Fields
- UserIDFK int? - UserID for a Timesheet user in Avaza
- ProjectIDFK int? - The project to associate the timesheet with.
- TimesheetCategoryIDFK int? - The Project timesheet category to link the timesheet to
- Duration decimal? - The duration of the timesheet, in decimal hours. If null or 0, a timer will be started.
- isInvoiced boolean? - Optional. False by default. Allows you to mark the timesheet as invoiced in an external system.
- EntryDate string? - The date of the timesheet entry, with an optional start time component.
- hasStartEndTime boolean? - If true, the start time will be take from the time component of the Entry Date field, and the end time will be calculated by adding the Duration to the StartDate
- Notes string? - Timesheet Notes
- TaskIDFK int? - Optional. Link the timesheet to a specific task
- CustomMetadata string? - Optional. free nvarchar field available via Api to store any additional metadata against a timesheet. We suggest you use Json or your preferred serialisation format. 1000 characters max.
avaza: Payment
Fields
- TransactionID int? -
- AccountIDFK int? -
- TransactionPrefix string? -
- PaymentNumber string? -
- TransactionReference string? -
- CustomerIDFK int? -
- DateIssued string? -
- TransactionStatusCode string? -
- PaymentProviderCode string? -
- ExchangeRate decimal? -
- Balance decimal? -
- CurrencyCode string? -
- TotalAmount decimal? -
- Notes string? -
- DateCreated string? -
- DateUpdated string? -
- PaymentAllocations PaymentAllocation[]? -
avaza: PaymentAllocation
Fields
- TransactionAllocationID int? -
- InvoiceTransactionIDFK int? -
- PaymentTransactionIDFK int? -
- AllocationDate string? -
- AllocationAmount decimal? -
avaza: PaymentList
Fields
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
- Payments Payment[]? -
avaza: ProjectCompanyGroup
Fields
- CompanyID int? -
- CompanyName string? -
- projects ProjectDropdownSelection[]? -
avaza: ProjectDetails
Fields
- ProjectID int? -
- Title string? -
- ProjectCode string? -
- Notes string? -
- CompanyName string? -
- CompanyIDFK int? -
- ProjectOwnerUserIDFK int? -
- ProjectStatusCode string? - Possible values: NotStarted, InProgress, Complete
- isArchived boolean? -
- ProjectBillableTypeCode string? - Possible values: CategoryHourly, NoRate, NotBillable, PersonHourly, ProjectHourly
- ProjectBudgetTypeCode string? - Possible Values: CategoryHours, NoBudget, PersonHours, ProjectFees, ProjectHours
- BudgetAmount decimal? -
- BudgetHours decimal? -
- ProjectHourlyRate decimal? -
- ProjectCategoryIDFK int? -
- ProjectCategoryName string? -
- isTaskRequiredOnTimesheet boolean? -
- DefaultAccountTaskTypeIDFK int? -
- DefaultAccountTaskTypeName string? -
- ProjectCategoryColor string? - Html Hex Color Code starting with #
- StartDate string? -
- EndDate string? -
- Sections ProjectSectionDetails[]? -
- Members ProjectMemberDetails[]? -
- ProjectTags ProjectTagItem[]? -
- DateCreated string? -
- DateUpdated string? -
avaza: ProjectDropdownList
Fields
- companies ProjectCompanyGroup[]? - List of Projects grouped by Customer Name
- pageNumber int? - Current page number (1 based)
- PageSize int? - Current page size
- hasMore boolean? - More records probably exist
avaza: ProjectDropdownSelection
Fields
- ProjectID int? -
- ProjectCode string? -
- Title string? -
avaza: ProjectList
Fields
- Projects ProjectListDetails[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: ProjectListDetails
Fields
- ProjectID int? -
- ProjectCode string? -
- Title string? -
- isArchived boolean? -
- Notes string? -
- CompanyName string? -
- CompanyIDFK int? -
- isTaskRequiredOnTimesheet boolean? -
- DefaultAccountTaskTypeIDFK int? -
- DefaultAccountTaskTypeName string? -
- DateCreated string? -
- DateUpdated string? -
avaza: ProjectMemberDetails
Fields
- UserIDFK int? -
- Firstname string? -
- Lastname string? -
- Fullname string? -
- Email string? -
- CostAmount decimal? -
- RateAmount decimal? -
- BudgetAmount decimal? -
- isMemberDisabled boolean? -
- isProjectManager boolean? -
- isTimesheetAllowed boolean? -
- isTimesheetApprover boolean? -
- isTimesheetApprovalRequired boolean? -
- canCreateTasks boolean? -
- canDeleteTasks boolean? -
- canCommentOnTasks boolean? -
- canUpdateTasks boolean? -
avaza: ProjectSectionDetails
Fields
- SectionID int? -
- Title string? -
- StartDate string? -
- EndDate string? -
- DisplayOrder int? -
avaza: ProjectTagItem
Fields
- ProjectTagID int? -
- Name string? -
avaza: ProjectTimesheetCategoryDetails
Fields
- TimeSheetCategoryIDFK int? -
- AccountIDFK int? -
- ProjectIDFK int? -
- Name string? -
- isBillable boolean? -
- RateAmount decimal? -
- BudgetHours decimal? -
- CostAmount decimal? -
avaza: ProjectTimesheetCategoryList
Fields
- Categories ProjectTimesheetCategoryDetails[]? -
avaza: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
avaza: RecipientDetails
Fields
- CompanyIDFK int? -
- CompanyName string? -
- RecipientFormattedBillingAddress string? -
- RecipientBillingAddressLine string? -
- RecipientBillingAddressCity string? -
- RecipientBillingAddressState string? -
- RecipientBillingAddressPostCode string? -
- RecipientBillingAddressCountryCode string? -
avaza: RoleDetails
Fields
- RoleCode string? -
- RoleName string? -
avaza: ScheduleAssignmentDetails
Fields
- ScheduleAssignmentID int? -
- AccountIDFK int? -
- UserIDFK int? -
- ScheduleSeriesIDFK int? -
- ScheduleDate string? -
- Duration decimal? -
- DateCreated string? -
- DateUpdated string? -
avaza: ScheduleAssignmentList
Fields
- ScheduleAssignments ScheduleAssignmentDetails[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: ScheduleSeriesDetails
Fields
- ScheduleSeriesID int? -
- AccountIDFK int? -
- UserIDFK int? -
- Firstname string? -
- Lastname string? -
- ProjectIDFK int? -
- ProjectTitle string? -
- CompanyIDFK int? -
- CompanyName string? -
- TimeSheetCategoryIDFK int? -
- TimeSheetCategoryName string? -
- LeaveTypeIDFK int? -
- LeaveTypeName string? -
- StartDate string? -
- EndDate string? -
- HoursPerDay decimal? -
- TotalDuration decimal? -
- ScheduleOnDaysOff boolean? -
- Notes string? -
- TaskIDFK int? -
- TaskTitle string? -
- DateCreated string? -
- DateUpdated string? -
- UpdatedByUserIDFK int? -
avaza: ScheduleSeriesList
Fields
- ScheduleSeries ScheduleSeriesDetails[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: SectionDetails
Fields
- SectionID int? -
- ProjectIDFK int? -
- Title string? -
- DisplayOrder int? -
- StartDateUTC string? -
- StartDate string? -
- EndDateUTC string? -
- EndDate string? -
avaza: SectionList
Fields
- Sections SectionDetails[]? -
- TotalCount int? -
avaza: SubscribeResult
Fields
- ID int? -
avaza: TagItem
Fields
- TagID int? -
- Name string? -
- Color string? -
avaza: TaskDetails
Fields
- TaskID int? -
- ProjectIDFK int? -
- ProjectTitle string? -
- ProjectCode string? -
- SectionTitle string? -
- SectionIDFK int? -
- Title string? -
- Description string? -
- DescriptionNoHTML string? -
- AssignedToFirstname string? -
- AssignedToLastname string? -
- AssignedToEmail string? -
- AssignedToUserIDFK string? -
- DateStart string? -
- DateDue string? -
- DateCompleted string? -
- EstimatedEffort decimal? -
- ActualTime decimal? -
- Tags TagItem[]? -
- AccountTaskTypeIDFK int? -
- TaskStatusCode string? -
- TaskStatusName string? -
- isCompleteStatus boolean? -
- PercentComplete decimal? -
- TaskPriorityCode string? -
- TaskPriorityName string? -
- DateCreated string? -
- DateUpdated string? -
avaza: TaskDropdownList
Fields
- sections TaskSectionGroup[]? - List of Task grouped by Section
- pageNumber int? - Current page number (1 based)
- PageSize int? - Current page size
- hasMore boolean? - More records probably exist
avaza: TaskDropdownSelection
Fields
- TaskID string? -
- Title string? -
avaza: TaskList
Fields
- Tasks TaskDetails[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: TaskSectionGroup
Fields
- SectionTitle string? -
- tasks TaskDropdownSelection[]? -
avaza: TaskStatusDetails
Fields
- TaskStatusCode string? -
- Name string? -
- Color string? -
- DisplayOrder int? -
- isComplete boolean? -
- AccountTaskTypeIDFK int? -
- TaskTypeName string? -
avaza: TaskStatusList
Fields
- statuses TaskStatusDetails[]? -
avaza: TaskTypeList
Fields
- tasktypes AccountTaskTypeDetails[]? -
avaza: TaxComponent
Fields
- TaxComponentID int? -
- TaxIDFK int? -
- ComponentTaxCode string? -
- Name string? -
- Percentage decimal? -
- isCompound boolean? -
avaza: TaxItem
Fields
- TaxID int? -
- TaxCode string? -
- Name string? -
- CalculatedPercent decimal? -
- TaxComponents TaxComponent[]? -
avaza: TaxList
Fields
- Taxes TaxItem[]? -
avaza: TimesheetDetails
Fields
- TimesheetEntryID int? -
- UserIDFK int? -
- Firstname string? -
- Lastname string? -
- Email string? -
- ProjectIDFK int? -
- ProjectTitle string? -
- ProjectCode string? -
- CustomerIDFK int? -
- CustomerName string? -
- TimesheetCategoryIDFK int? -
- CategoryName string? -
- Duration decimal? -
- TimesheetEntryApprovalStatusCode string? -
- HasTimer boolean? -
- TimerStartedAtUTC string? -
- isBillable boolean? -
- isInvoiced boolean? -
- EntryDate string? -
- StartTimeLocal string? -
- StartTimeUTC string? -
- EndTimeLocal string? -
- EndTimeUTC string? -
- TimesheetUserTimeZone string? -
- Notes string? -
- TaskIDFK int? -
- TaskTitle string? -
- InvoiceIDFK int? -
- InvoiceLineItemIDFK int? -
- DateCreated string? -
- DateUpdated string? -
- DateApproved string? -
- ApprovedBy string? -
- CustomMetadata string? -
avaza: TimesheetList
Fields
- Timesheets TimesheetDetails[]? -
- TotalCount int? -
- PageNumber int? -
- PageSize int? -
avaza: TimesheetSummaryGroup
Fields
- GroupID string? -
- GroupName string? -
- TotalHours decimal? -
- BillableHours decimal? -
- GroupData TimesheetSummaryGroup[]? -
avaza: TimesheetSummaryRequest
Fields
- GroupBy string[]? - (Optional) Combine one, two or three levels of Grouping. Combine these possible grouping values: "Customer", "Project", "Category", "User", "Task", "Year", "Month", "Day", "Week".
- EntryDateFrom string? - (Required) Filter for timesheets greater or equal to the specified date. e.g. 2019-01-25. You can optionally include a time component, otherwise it assumes 00:00
- EntryDateTo string? - (Required) Filter for timesheets with an entry date smaller or equal to the specified date. e.g. 2019-01-25. You can optionally include a time component, otherwise it assumes 00:00
- UserID int[]? - (Optional) Defaults to the current user. Provide one or more UserIDs of Users whose timesheets should be retrieved. If the current user doesn't have impersonation rights, then they will only see their own data.
- ProjectID int? - (Optional) Filter by Project
- isBillable boolean? - (Optional) Filter by the billable status of Timesheets.
- isInvoiced boolean? - (Optional) Filter for timesheets by whether they have been Invoiced or not.
avaza: TimesheetSummaryResult
Fields
- EntryDateFrom string? -
- EntryDateTo string? -
- TotalHours decimal? -
- BillableHours decimal? -
- UserID int[]? -
- GroupingLevels string[]? -
- GroupData TimesheetSummaryGroup[]? -
avaza: UpdateCompany
Fields
- CompanyID int? -
- FieldsToUpdate string[]? -
- CompanyName string? -
- BillingAddressLine string? -
- BillingAddressCity string? -
- BillingAddressState string? -
- BillingAddressPostCode string? -
- BillingCountryCode string? -
- BillingAddress string? -
- Phone string? -
- Fax string? -
- website string? -
- TaxNumber string? -
- Comments string? -
avaza: UpdateExpense
Fields
- ExpenseID int -
- FieldsToUpdate string[] -
- ExpenseDate string? - The date of the expense entry
- ExpenseCategoryIDFK int? - The expense category to link the Expense to.
- isChargeable boolean? - aka Billable. Defaults to false if not provided. If set to true, a CustomerIDFK or CustomerName must be provided.
- isReimbursable boolean? - Defaults to false if not provided.
- Quantity decimal? - Conditional - available for expenses that are assigned a unit priced based expense category. e.g Mileage
- CustomerIDFK int? - The Avaza Customer ID to associate the Expense with.
- ProjectIDFK int? - The Avaza project ID to associate the Expense with.
- TaskIDFK int? - (optional) TaskID of a Task to link the new Expense to. A Customer and Project must be provided also.
- CurrencyCode string? - A 3-letter ISO CurrencyCode for the expense currency. (e.g. USD). If not provided, defaults to the Account base currency.
- ExchangeRate decimal? - Optional (Only relevant if the expense currency is different to your account currency. If not provided we will look up the market exchange rate for you based on the expense date.) Exchange Rate = Expense Currency Amount / Base Currency Amount (e.g. if Expense currency is in AUD, and Base Currency is in USD, Exchange Rate = AUD $140 / USD $100 = 1.4)
- Amount decimal? - Expense Amount (Required). Must be >= 0
- TaxIDFK int? - Avaza Tax ID the expense belongs to.
- TransactionTaxConfigCode string? - Optional - Enter "INC" if the tax amount is included in the expense amount otherwise enter "EX" when the amount exlcudes the tax. Defaults to "Ex". The tax amount on the expense will be autocalculated.
- GroupTripName string? - Links the expense to a Grouping/Trip report. If no matching name found, creates a new Group/Trip Report name.
- ExpensePaymentMethodIDFK int? - (Optional) ID of Expense Payment Method.
- Merchant string? - The name of the merchant.
- MerchantTaxNumber string? - A Tax number identifier for the merchant.
- Notes string? - Expense Notes
- VerifyAndSave boolean? - Pass false if creating a draft expense. True otherwise.
- FileAttachmentIDs int[]? - Array of File Attachment IDs to associate with this expense. The files need to have already been uploaded. Currently only accepts a single file.
avaza: UpdateProjectModel
Fields
- ProjectID int? - The ID of the Project to update
- FieldsToUpdate string[]? -
- ProjectTitle string? - (optional) An updated project title. (255 characters max)
- ProjectNotes string? - (optional) Any descriptive notes about the project. (2000 characters max)
- TimesheetApprovalRequiredbyDefault boolean? - Whether timesheet approval should be required by default for newly added project members.
- isTaskRequiredOnTimesheet boolean? - Whether timesheets entered against this project require a task to be selected.
- StartDate string? -
- EndDate string? -
- BudgetAmount decimal? -
- BudgetHours decimal? -
- ProjectStatusCode string? - Update the project status (string, optional): (Possible values: NotStarted, InProgress, Complete, OnHold)
- ProjectCategoryIDFK int? -
- ProjectBillableTypeCode string? - The billing method of the project. (string, optional) Possible values: CategoryHourly, NoRate, NotBillable, PersonHourly, ProjectHourly
- ProjectBudgetTypeCode string? - The project budgeting type. (string, optional) Possible values: NoBudget, PersonHours, ProjectFees, ProjectHours, CategoryHours
avaza: UpdateTask
Fields
- TaskID int -
- FieldsToUpdate string[] -
- SectionIDFK int? -
- Title string? -
- Description string? -
- AssignedToUserIDFK int? -
- DateStart string? -
- DateDue string? -
- TaskPriorityCode string? -
- EstimatedEffort decimal? - Decimal hours
- TaskStatusCode string? -
- PercentComplete int? -
- Tags NewTag[]? -
avaza: UpdateTimesheetModel
Fields
- TimeSheetEntryID int -
- FieldsToUpdate string[] -
- ProjectIDFK int -
- TimesheetCategoryIDFK int? -
- TaskIDFK int? -
- Duration decimal? -
- EntryDate string? -
- hasStartEndTime boolean? -
- Notes string? -
- CustomMetadata string? - Optional. free nvarchar field available via Api to store any additional metadata against a timesheet. We suggest you use Json or your preferred serialisation format. 1000 characters max.
avaza: UserDetails
Fields
- UserID int? -
- AccountIDFK int? -
- Email string? -
- Firstname string? -
- Lastname string? -
- PositionTitle string? -
- Phone string? -
- Mobile string? -
- TimeZone string? - Windows Timezone ID
- IANATimezone string? - IANA tz database timezone name
- isTeamMember boolean? -
- CompanyIDFK int? -
- CompanyName string? -
- DefaultBillableRate decimal? -
- DefaultCostRate decimal? -
- MondayAvailableHours decimal? -
- TuesdayAvailableHours decimal? -
- WednesdayAvailableHours decimal? -
- ThursdayAvailableHours decimal? -
- FridayAvailableHours decimal? -
- SaturdayAvailableHours decimal? -
- SundayAvailableHours decimal? -
- Roles RoleDetails[]? -
- Tags UserTagDetails[]? -
avaza: UserList
Fields
- Users UserDetails[]? -
avaza: UserTagDetails
Fields
- UserTagID int? -
- UserTagName string? -
avaza: WebhookDetails
Fields
- SubscriptionID int? -
- EventCode string? -
- NotificationURL string? -
- UserIDFK int? -
avaza: WebhookList
Fields
- Webhooks WebhookDetails[]? -
Import
import ballerinax/avaza;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Productivity/Project Management
Cost/Freemium
Contributors
Dependencies