atspoke
Module atspoke
API
Definitions
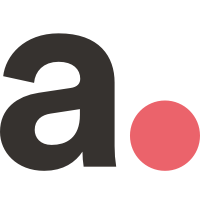
ballerinax/atspoke Ballerina library
Overview
This is a generated connector for atSpoke API v0.1.0 OpenAPI specification. The atSpoke REST API provides a broad set of operations including:
- Creation, manipulation, and deletion of requests in atSpoke
- Management of users in atSpoke
- Creation, manipulation, and deletion of knowledge resources in atSpoke
The public API is served from https://api.askspoke.com/api/v1 – note
api
in the host name, not your usual organization id.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an atSpoke account
- Obtain tokens by following this guide
Quickstart
To use the atSpoke connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/atspoke
module into the Ballerina project.
import ballerinax/atspoke;
Step 2: Create a new connector instance
Create a atspoke:ApiKeysConfig
with the API key obtained, and initialize the connector with it.
atspoke:ApiKeysConfig config = { apiKey: "<API_KEY>" } atspoke:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to return a list of teams using the connector.
Return a list of teams
public function main() returns error? { atspoke:InlineResponse2006 response = check baseClient->getTeams(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
atspoke: Client
This is a generated connector for atSpoke API v0.1.0 OpenAPI specification. The atSpoke REST API provides a broad set of operations including:
- Creation, manipulation, and deletion of requests in atSpoke
- Management of users in atSpoke
- Creation, manipulation, and deletion of knowledge resources in atSpoke
The public API is served from https://api.askspoke.com/api/v1 – note
api
in the host name, not your usual organization id.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a atSpoke account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.askspoke.com/api/v1" - URL of the target service
getRequests
function getRequests(string filter, string status, string? team, string? tag, string? q, int 'start, int 'limit, string sort) returns InlineResponse200|error
List requests
Parameters
- filter string (default "inbox") - Parameter to filter queries
- status string (default "OPEN") - Parameter to get requests by status. Should be a comma-separated string of statuses.
- team string? (default ()) - Get requests belonging to this team
- tag string? (default ()) - Get requests tagged with this tag. Accepts a comma-separated string of many tags, and returns requests that match any provided tag. The keyword
none
can also be passed to return requests that are not tagged.
- q string? (default ()) - Searches and returns request with this text
- 'start int (default 0) - The index of the request to start with
- 'limit int (default 25) - The number of requests to return at once. Max is 100.
- sort string (default "updated") - The order by which requests should be returned. When querying with a
q
param, results will be sorted by their similarity to the query when no othersort
is specified.
Return Type
- InlineResponse200|error - List of requests
addRequest
function addRequest(RequestsBody payload) returns Request|error
Create new request
Parameters
- payload RequestsBody - AddRequest payload
getOpenTasks
function getOpenTasks(int? 'start, int? 'limit, boolean? byDueDate) returns InlineResponse2001|error
Get open tasks
Parameters
- 'start int? (default ()) - The index of the task to start with.
- 'limit int? (default ()) - The number of tasks to return.
- byDueDate boolean? (default ()) - Whether to sort tasks by due date or not.
Return Type
- InlineResponse2001|error - List of pending tasks.
getRequest
Get a request
Parameters
- requestId string - the ID of the request, 24 characters, hexadecimal
deleteRequest
Delete a request
Parameters
- requestId string - the ID of the request, 24 characters, hexadecimal
updateRequest
function updateRequest(string requestId, RequestPatchBody payload) returns Request|error
Update a request
Parameters
- requestId string - the ID of the request, 24 characters, hexadecimal
- payload RequestPatchBody - RequestPatchBody payload
bulkAddTags
function bulkAddTags(RequestsBulkAddTagBody payload) returns Request|error
Bulk add tags
Parameters
- payload RequestsBulkAddTagBody - A payload containing the tag id of the tag to be added and a list of request ids that the tag will be added to.
getMergedRequests
function getMergedRequests(string requestId, int? 'start, int? 'limit) returns InlineResponse2002|error
Get merged requests
Parameters
- requestId string - the request id of the request for which you wish to see the merged requests of
- 'start int? (default ()) - The index of merged requests to start with
- 'limit int? (default ()) - The number of requests merged with your initial request to return. Defaults to 25.
Return Type
- InlineResponse2002|error - List of requests.
postRequestMessage
function postRequestMessage(string requestId, RequestidMessagesBody payload) returns Update|error
Post a message
Parameters
- requestId string - ID of the request.
- payload RequestidMessagesBody - PostRequestMessage payload
mergeRequest
function mergeRequest(string requestId, RequestidMergeBody payload) returns Response|error
Merge requests
Parameters
- requestId string - the request id of the request you wish to merge in to
- payload RequestidMergeBody - MergeRequest payload
addTagsToRequest
function addTagsToRequest(string requestId, RequestidTagsBody payload) returns Response|error
Add tags
Parameters
- requestId string - ID of the request that needs to be updated.
- payload RequestidTagsBody - AddTagsToRequest payload
deleteTagFromRequest
Delete tag
Parameters
- requestId string - ID of the request that needs to be updated.
- tagId string - ID of the tag to remove from the request.
addSubscriberToRequest
Add a subscriber
Parameters
- requestId string - ID of the request that will have the new subscriber.
- userId string - ID of the user to subscribe to the request.
Return Type
- SubscriberList|error - All request subscribers
removeSubscriberFromRequest
Remove a subscriber
Parameters
- requestId string - ID of the request that will have the subscriber removed.
- userId string - ID of the user to remove as a subscriber.
Return Type
- SubscriberList|error - All request subscribers
getRequestTypes
function getRequestTypes(string? q, string? team, int? 'start, int? 'limit, string? sort) returns InlineResponse2003|error
List request types
Parameters
- q string? (default ()) - Parameter for text-searching requestTypes
- team string? (default ()) - Get request types belonging to a team.
- 'start int? (default ()) - The index of the request type to start with.
- 'limit int? (default ()) - The number of request types to return. Defaults to 25.
- sort string? (default ()) - sort order for the returned request types
Return Type
- InlineResponse2003|error - List of request types.
getRequestType
function getRequestType(string requestTypeId) returns RequestType|error
Get a request type.
Parameters
- requestTypeId string - id of the request type
Return Type
- RequestType|error - A request type object.
getResources
function getResources(string? q, boolean? ai, decimal? status, string? team, string? author, int? reviewBy, int? 'start, int? 'limit) returns InlineResponse2004|error
List resources
Parameters
- q string? (default ()) - A search query to search resources for.
- ai boolean? (default ()) - Whether or not to use AI-based search. Requires q to be set as well.
- status decimal? (default ()) - Parameter to get resources by status. 0 = ok, 1 = needs_review, 2 = deprecated..
- team string? (default ()) - Get resources belonging to one or more teams. Send comma-separated string for multiple teams, "none" for no team
- author string? (default ()) - Get resources created one or more authors. Send comma-separated string for multiple authors.
- reviewBy int? (default ()) - Get resources up for review before this timestamp (ms since epoch).
- 'start int? (default ()) - The index of the request to start with.
- 'limit int? (default ()) - The number of parameters to return. Defaults to 25.
Return Type
- InlineResponse2004|error - List of resources.
addResource
function addResource(ResourcePostBody payload) returns Resource|error
Create a resource
Parameters
- payload ResourcePostBody - ResourcePostBody payload
getResource
Get a resource
Parameters
- resourceId string - ID of the resource.
deleteResource
Delete a resource
Parameters
- resourceId string - ID of resource that needs to be deleted.
updateResource
function updateResource(string resourceId, ResourcePostBody payload) returns Resource|error
Update a resource
Parameters
- resourceId string - ID of the resource that needs to be updated.
- payload ResourcePostBody - ResourcePostBody payload
getTags
function getTags(int? 'start, int? 'limit, string? q, string? tagId) returns InlineResponse2005|error
List tags
Parameters
- 'start int? (default ()) - The index of the tag to start with.
- 'limit int? (default ()) - The number of tags to return. Defaults to 25.
- q string? (default ()) - A search query to search tags for
- tagId string? (default ()) - A comma separated list of tags
Return Type
- InlineResponse2005|error - List of tags.
getTeams
function getTeams(string? q, boolean? ai, string? slug, int? 'start, int? 'limit) returns InlineResponse2006|error
List teams
Parameters
- q string? (default ()) - Text search to search teams for.
- ai boolean? (default ()) - Whether or not to use AI-based search. Requires q to be set as well.
- slug string? (default ()) - Comma-separated list of team slugs to get.
- 'start int? (default ()) - The index of the request to start with. Ignored if ai is set to true.
- 'limit int? (default ()) - The number of parameters to return. Defaults to 25. Ignored if ai is set to true.
Return Type
- InlineResponse2006|error - List of teams.
getTeam
Get a team
Parameters
- teamId string - ID of the team.
updateTeam
function updateTeam(string teamId, TeamPatchBody payload) returns Team|error
Update a team
getUsers
function getUsers(string? q, string? status, string? team, int? 'start, int? 'limit) returns InlineResponse2007|error
List users
Parameters
- q string? (default ()) - Text search to search user for.
- status string? (default ()) - Filter user based on status.
- team string? (default ()) - Filter user based on team.
- 'start int? (default ()) - The index of the request to start with.
- 'limit int? (default ()) - The number of parameters to return. Defaults to 10.
Return Type
- InlineResponse2007|error - List of users.
getUser
Get a user
Parameters
- userId string - ID of the user.
updateUser
function updateUser(string userId, UsersUseridBody payload) returns User|error
Update a user
Parameters
- userId string - ID of user that needs to be updated.
- payload UsersUseridBody - UpdateUser payload
getUserByEmail
Get a user by email
Parameters
- address string - Email address of the user. Case insensitive.
whoami
function whoami() returns InlineResponse2008|error
Get API user details
Return Type
- InlineResponse2008|error - User and Org details
getConfigLists
function getConfigLists(string? q, int? 'start, int? 'limit, string? listId, string? withItem) returns InlineResponse2009|error
List config lists
Parameters
- q string? (default ()) - Parameter for text-searching lists by name or item name
- 'start int? (default ()) - The index of the list to start with.
- 'limit int? (default ()) - The number of lists to return. Defaults to 25.
- listId string? (default ()) - comma-separated list of listids to return
- withItem string? (default ()) - comma-separated list of itemids to return
Return Type
- InlineResponse2009|error - List of config lists.
addConfigList
function addConfigList(string listId, ConfigList payload) returns ConfigList|error
Create a config list.
Return Type
- ConfigList|error - A config list object.
deleteConfigList
Delete a config list.
Parameters
- listId string - the ID of the configlist, 24 characters, hexadecimal
updateConfigList
function updateConfigList(string listId, ConfigList payload) returns ConfigList|error
Update a config list.
Parameters
- listId string - the ID of the configlist, 24 characters, hexadecimal
- payload ConfigList - ConfigList payload
Return Type
- ConfigList|error - A config list object.
getWebhooks
function getWebhooks() returns InlineResponse20010|error
Lists webhook subscriptions
Return Type
- InlineResponse20010|error - Lists all the webhook subscriptions for this user on this org.
addWebhook
function addWebhook(WebhookSubscriptionPostBody payload) returns WebhookSubscription|error
Creates a new webhook subscription
Parameters
- payload WebhookSubscriptionPostBody - WebhookSubscriptionPostBody payload
Return Type
- WebhookSubscription|error - Webhook subscription detail.
getWebhook
function getWebhook(string webhookId) returns WebhookSubscription|error
Get a webhook subscription
Parameters
- webhookId string - The ID of the webhook to get.
Return Type
- WebhookSubscription|error - Webhook Subscription object.
deleteWebhook
Delete a webhook subscription.
Parameters
- webhookId string - ID of webhook subscription to deleted.
updateWebhook
function updateWebhook(string webhookId, WebhookSubscriptionPostBody payload) returns WebhookSubscription|error
Update a webhook subscription
Parameters
- webhookId string - ID of the webhook subscription that needs to be updated.
- payload WebhookSubscriptionPostBody - WebhookSubscriptionPostBody payload
Return Type
- WebhookSubscription|error - Updated webhook subscription object.
Records
atspoke: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apiKey string - Users on atSpoke's Standard and Plus plans can generate an API key by navigating to "My Profile" and then selecting the API tab. All API actions are taken by the API user. If a new key is generated or the user is deactivated, the old API key is deactivated automatically.<br/><br/> Requests made to atSpoke's public API are rate-limited to no more than 6000 requests per minute per API key.<br/><br/> <b> Warning:</b> All private items viewable by the user whose API key is being used will be viewable via the API. This means that if an admin uses their key to connect atSpoke to an external system, requests and resources that are private to a team in atSpoke will accessible in the external system. Keep this in mind when building with the API so that private data does not become public.
atspoke: BadRequest
Bad request
Fields
- code int? - atSpoke error code.
- message string? - Error message.
atspoke: BulkAddLabelBody
Body to bulk add labels
Fields
- requests string[] - A list of request ids to add a label to.
- label string - Label id to add to the requests.
atspoke: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
atspoke: ConfigList
Configuration Item List
Fields
- id string? - ID
- name string? - Name
- status string? - Status
- items ConfigListItem[]? - Items
- externalData ConfigListExternalData? - Third-party data associated with a configuration list
- org string? - Org
atspoke: ConfigListExternalData
Third-party data associated with a configuration list
Fields
- sourceIntegrationId string? - Integration id of the third party app this list or item is synced from
- lastSyncedAt string? - Most recent timestamp when this list was synced with the third-party system
atspoke: ConfigListItem
A single item in a configuration list
Fields
- id string? - ID
- name string? - Name
- status string? - Status
- externalData ConfigListItemExternalData? - Third-party data associated with a configuration list
atspoke: ConfigListItemExternalData
Third-party data associated with a configuration list
Fields
- sourceIntegrationId string? - Integration id of the third party app this list or item is synced from
- remoteId string? - Unique id in the third-party system for this item
- remoteLink string? - Unique link for this item in the third-party system
- lastSyncedAt string? - Most recent timestamp when this list was synced with the third-party system
atspoke: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
atspoke: CustomNavList
A custom nav list used to organize a user’s requests
Fields
- name string - Name
- slug string - Slug
- filters CustomnavlistFilters? - Filters
atspoke: CustomnavlistFilters
Filters
Fields
- status CustomnavlistFiltersStatus? - Status
- team CustomnavlistFiltersTeam? - Team
- requestType CustomnavlistFiltersRequesttype? - Request type
- assignee CustomnavlistFiltersAssignee? - Assignee
- requester CustomnavlistFiltersRequester? - Requester
- follower CustomnavlistFiltersFollower? - Follower
- taskAssignee CustomnavlistFiltersTaskassignee? - Task assignee
- tag CustomnavlistFiltersTag? - Tag
- query string? - Query
atspoke: CustomnavlistFiltersAssignee
Assignee
Fields
- includeNoValue boolean? - Include no value
- values string[]? - Values
atspoke: CustomnavlistFiltersFollower
Follower
Fields
- includeNoValue boolean? - Include no value
- values string[]? - Values
atspoke: CustomnavlistFiltersRequester
Requester
Fields
- includeNoValue boolean? - Include no value
- values string[]? - Values
atspoke: CustomnavlistFiltersRequesttype
Request type
Fields
- includeNoValue boolean? - Include no value
- values string[]? - Values
atspoke: CustomnavlistFiltersStatus
Status
Fields
- includeNoValue boolean? - Include no value
- values string[]? - Values
atspoke: CustomnavlistFiltersTag
Tag
Fields
- includeNoValue boolean? - Include no value
- values string[]? - Values
atspoke: CustomnavlistFiltersTaskassignee
Task assignee
Fields
- includeNoValue boolean? - Include no value
- values string[]? - Values
atspoke: CustomnavlistFiltersTeam
Team
Fields
- includeNoValue boolean? - Include no value
- values string[]? - Values
atspoke: Forbidden
Forbidden
Fields
- code int? - atSpoke error code.
- message string? - Error message.
atspoke: InlineResponse200
Fields
- results Request[]? - The list of requests itself.
- total int? - The total number of requests.
- 'start int? - The start offset for this set of requests.
- 'limit int? - The number of requests returned.
atspoke: InlineResponse2001
Fields
- results RequestTask[]? -
- total int? - The total number of tasks.
- 'start int? - The start offset for this set of tasks.
- 'limit int? - The number of tasks returned.
atspoke: InlineResponse20010
Fields
- results WebhookSubscription[]? -
atspoke: InlineResponse2002
Fields
- results Request[]? -
- total int? - The total number of the requests.
- 'start int? - The start offset for this set of requests.
- 'limit int? - The number of requests returned.
atspoke: InlineResponse2003
Fields
- results RequestType[]? -
- total int? - The total number of the request types.
- 'start int? - The start offset for this set of request types.
- 'limit int? - The number of request types returned.
atspoke: InlineResponse2004
Fields
- results Resource[]? -
- total int? - The total number of the resources.
- 'start int? - The start offset for this set of resouces.
- 'limit int? - The number of resouces returned.
- newStart int? - Where to start next request for pagination.
atspoke: InlineResponse2005
Fields
- results Tag[]? -
- total int? - The total number of the tags.
- 'start int? - The start offset for this set of tags.
- count int? - The number of tags returned.
atspoke: InlineResponse2006
Fields
- results Team[]? -
- total int? - The total number of the teams.
- 'start int? - The start offset for this set of teams.
- 'limit int? - The limit of teams returned.
atspoke: InlineResponse2007
Fields
- results User[]? -
- total int? - The total number of the users.
- 'start int? - The start offset for this set of users.
- 'limit int? - The number of users returned.
atspoke: InlineResponse2008
Fields
- org string? - current org id
- user string? - user id
- displayName string? - user name
- role string? - user role
- status string? - user status
atspoke: InlineResponse2009
Fields
- results ConfigList[]? -
- total int? - The total number of config lists.
- 'start int? - The start offset for this set of lists.
- 'limit int? - The number of lists returned.
atspoke: MessageContent
The content of the update
Fields
- message MessagecontentMessage? - An object that holds various types of updates. Only the text type is supported via the public API. Attachments are not supported.
atspoke: MessagecontentMessage
An object that holds various types of updates. Only the text type is supported via the public API. Attachments are not supported.
Fields
- text string? - a plain text string of the message to be posted to the request
atspoke: NotFound
Not Found
Fields
- code int? - atSpoke error code.
- message string? - Error message.
atspoke: PaymentRequired
Payment required
Fields
- code int? - atSpoke error code.
- message string? - Error message.
atspoke: Profile
User profile object.
Fields
- id string? - ID
- jobTitle string? - Job title
- location string? - Location
- department string? - Department
- manager ProfileManager? - Manager
- startDate string? - Start date
atspoke: ProfileManager
Manager
Fields
- name string? - Name
- email string? - Email
atspoke: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
atspoke: Request
Request object.
Fields
- id string? - ID
- requester string - Requester
- owner string? - Owner
- subject string - Subject
- status RequestStatus? - Request statuses.
- requestType record {}? - Request type
- requestTypeInfo RequestTypeInfoResponse? - Request type form answer values.
- privacyLevel string? - Privacy level
- team string? - Team
- org string? - Org
- isAutoResolved boolean? - Is auto resolved
- isFiled boolean? - is filed
- email string? - Email
- permalink string? - Permalink
- createdAt string? - Created at
- updatedAt string? - Updated at
- resolveTime int? - Resolve time
- teamResponseTimeInMs int? - Team response time in ms
- lastResolvedAt string? - Last resolved at
- tags string[]? - Tags
- taskInstances TaskInstanceResponse[]? - Task instances
atspoke: RequestidMergeBody
Fields
- requests string[] - Request ids to be merged into the request in the path parameter
atspoke: RequestidMessagesBody
Fields
- actor UpdateActor - Describes the actor that led to an update.
- content MessageContent - The content of the update
atspoke: RequestidTagsBody
Fields
- tags RequestsrequestidtagsTags[] - Tag ids to add
atspoke: RequestPatchBody
Request PATCH object.
Fields
- requester string? - New requester user id
- owner string? - New request owner user id
- subject string? - New request subject
- status RequestStatus? - Request statuses.
- requestType record {}? - New request type id
- requestTypeInfo RequestTypeInfo? - Request type form values.
- taskInstances TaskInstance[]? - Task instances
- privacyLevel string? - New privacy level
- team string? - New team id
atspoke: RequestsBody
Fields
- subject string - Request subject.
- body string? - a longer description of the request
- requester string - user id of person who will be the requester
- owner string? - user id of the person the request will be assigned to. Will be automatically delegated if left blank
- team string? - ID of the team to assign the request to.
- privacyLevel string? - Privacy level for the request.
- requestType string? - ID of the request type form.
- requestTypeInfo RequestTypeInfo? - Request type form values.
atspoke: RequestsBulkAddTagBody
Fields
- requests string[] - the IDs of the requests to be updated, 24 characters, hexadecimal
- tag string - ID of the tag to add to the requests.
atspoke: RequestsrequestidtagsTags
Fields
- _id string? - a tag id
atspoke: RequestTask
Request containing single task object.
Fields
- id string? - ID
- subject string - Subject
- permalinkId int? - Permalink ID
- taskInstances TaskInstance? - Task instances
- sortField string? - Sort field
atspoke: RequestType
Request type object
Fields
- id string? - ID
- title string - Title
- form RequesttypeForm? - Form
- taskTemplates TaskTemplate[]? - Task template
- status string? - Status
- icon string? - Icon
- team string - Team
- description string? - Description
- org string - Org
- hasForm boolean? - Has form
- createdAt string? - Created at
- updatedAt string? - Updated at
atspoke: RequesttypeForm
Form
Fields
- fields RequesttypeFormFields[]? - Fields
atspoke: RequesttypeFormFields
Fields
- uuid string? -
- 'type string? -
- label string? -
- 'select RequesttypeFormSelect? -
atspoke: RequesttypeFormSelect
Fields
- choices string[]? -
atspoke: RequestTypeInfo
Request type form values.
Fields
- answeredFields RequesttypeinfoAnsweredfields[]? - Answered fields
atspoke: RequesttypeinfoAnsweredfields
Fields
- fieldId string? -
- value string? -
atspoke: RequestTypeInfoResponse
Request type form answer values.
Fields
- answeredFields RequesttypeinforesponseAnsweredfields[]? - Answered fields
atspoke: RequesttypeinforesponseAnsweredfields
Fields
- simpleValue string? -
- 'field RequesttypeinforesponseField? -
atspoke: RequesttypeinforesponseField
Fields
- uuid string? -
- 'type string? -
- label string? -
- 'select RequesttypeFormSelect? -
- assignmentRule RequesttypeinforesponseFieldAssignmentrule? -
atspoke: RequesttypeinforesponseFieldAssignmentrule
Fields
- 'type string? -
- team string? -
- user string? -
atspoke: Resource
Resource object.
Fields
- id string? - ID
- 'type string? - Type
- author string? - Author
- title string? - Title
- body string? - Body
- file record {}? - File
- link record {}? - Link
- keywords string[]? - Keywords
- team string? - Team
- org string? - Org
- isDeprecated boolean? - Is deprecated
- needsReview boolean? - Needs review
- reviewStatus string? - Review status
- createdAt string? - Created at
- updatedAt string? - Updated at
atspoke: ResourcePostBody
Resource object.
Fields
- 'type string? - The type of the resource. atSpoke supports many types of resources but only text-based ones can be created via API.
- author string - The user id of the author of this resource.
- title string - The title of this resource.
- body string? - The body of this resource.
- team string? - The id of the team this resource should belong to.
atspoke: SearchProposal
A DB record for storing search recommendations.
Fields
- query string? - Query
- channelId string? - Channel ID
- user string? - User
- org string? - Org
- pivot SearchProposalPivot? - The item created from the search proposal.
- interactionId string? - Interaction ID
- proposals SearchProposalItem[]? - Proposals
atspoke: SearchProposalItem
The item created from the search proposal.
Fields
- action string? - Action
- itemType string? - Item type
- ref string? - Ref
atspoke: SearchProposalPivot
The item created from the search proposal.
Fields
- kind string? - Kind
- ref string? - Ref
atspoke: SegmentStringField
A string field in a VisibleSegment
Fields
- operator string? - Operator
- value string? - Value
atspoke: SegmentTeamField
A team field in a VisibleSegment
Fields
- operator string? - Operator
- value Team? - Team object.
atspoke: SubscriberlistInner
Fields
- subscriber string? - The user id of a user subscribed to this request
- unsubscribed boolean? - whether this user has unsubscribed from this request
- reason string? - why this user was subscribed
atspoke: Tag
Request tag.
Fields
- id string? - ID
- color string? - Color
- status string? - Status
- text string? - Text
- org string? - Org
atspoke: TaskInstance
Task instances
Fields
- uuid string - UUID
- owner string? - Owner
- status string? - Status
- dueDate string? - Due date
atspoke: TaskInstanceResponse
A representation of a single task on a request
Fields
- uuid string? - UUID
- subject string - Subject
- taskTemplate TaskTemplate? - the template a RequestType uses to create a task. Copied onto the created TaskInstance at instantiation
- requestTypeId string? - Request type ID
- isRequired boolean? - Is required
- parentTaskUUIDs string? - Parent task UUIDs
- isApproval boolean? - Is Approval
- approvalState string? - Approval State
- owner string? - Owner
- dueDate string? - Due date
- wasManuallyReassigned boolean? - Was manually reassigned
- status string? - Status
- timestamps string? - Timestamps
atspoke: TaskTemplate
the template a RequestType uses to create a task. Copied onto the created TaskInstance at instantiation
Fields
- uuid string? - UUID
- subject string? - Subject
- assignmentRule TasktemplateAssignmentrule? - Assignment rule
atspoke: TasktemplateAssignmentrule
Assignment rule
Fields
- 'type string? - Type
- team string? - Team
- user string? - User
atspoke: Team
Team object.
Fields
- id string? - ID
- name string - Name
- slug string? - Slug
- description string? - Description
- keywords string[]? - Keywords
- icon string? - Icon
- color string? - Color
- status string? - Status
- goals record {}? - Goals
- agentList record {}[]? - Agent list
- createdAt string? - Created at
- updatedAt string? - Updated at
- owner string - Owner
- org string? - Org
- email string? - Email
- permalink string? - Permalink
- settings TeamSettings? - Team settings
atspoke: TeamAgent
Describes an agent in a team.
Fields
- user User? - User object.
- status TeamAgentStatus? - Team agent statuses.
- lastAssignedAt string? - last request assigned at timestamp
- joinedAt string? - timestamp when this agent joined the team
- timestamps TeamagentTimestamps? - Timestamps
- isExcluded boolean? - Whether this agent is excluded from rotation
- numRequests int? - number of open requests assigned to this user on this team
atspoke: TeamagentTimestamps
Timestamps
Fields
- lastAvailableAt string? - when this agent became available most recently
- lastAwayAt string? - when this agent went away most recently
atspoke: TeamDelegation
Team delegation settings
Fields
- strategy TeamDelegationStrategy? - Team delegation strategies.
- specificUser string? - user id, valid only for SPECIFIC_USER strategy
- excludedUsers string[]? - user ids of excluded users, valid only for ROUND_ROBIN strategy
atspoke: TeamPatchBody
Team PATCH object
Fields
- settings TeamSettings? - Team settings
atspoke: TeamSettings
Team settings
Fields
- delegation TeamDelegation? - Team delegation settings
- flags TeamsettingsFlags? - Flags
atspoke: TeamsettingsFlags
Flags
Fields
- AUTO_DELEGATION boolean? - whether this team should use auto delegation
atspoke: Update
Update object
Fields
- content MessageContent? - The content of the update
- actor UpdateActor? - Describes the actor that led to an update.
atspoke: UpdateActor
Describes the actor that led to an update.
Fields
- kind string? - Kind
- ref string? - A team or user id
atspoke: User
User object.
Fields
- id string? - ID
- displayName string - Display name
- email string - Email
- isEmailVerified boolean? - Is email verified
- isProfileCompleted boolean? - Is profile completed
- status string? - Status
- profile Profile? - User profile object.
- memberships string[]? - Memberships
- createdAt string? - Created at
atspoke: UsersUseridBody
Fields
- displayName string? - New display name.
- phoneNumber string? - New phone number of the user.
- countryId string? - country of the users phone number.
- email string? - New email of the user.
- profile Profile? - User profile object.
atspoke: VisibleSegment
VisibleSegment object.
Fields
- id string? - ID
- org string - Org
- name string? - Name
- teams SegmentTeamField[]? - Teams
- locations SegmentStringField[]? - SegmentStringField
- departments SegmentStringField[]? - Departments
- createdAt string? - Created at
- updatedAt string? - Updated at
atspoke: WebhookSubscription
Webhook Subscription object.
Fields
- id string? - The ID of the webhook subscription.
- 'client string? - A description of the client software that created the webhook.
- org string? - The subscriptions org.
- user string? - The user who owns the subscription.
- url string? - The URL to which webhooks will be posted.
- enabled boolean? - Whether the webhook is enabled. If disabled, webhooks will not be sent, but one can always re-enable the subscription later.
- topics string[]? - An array of the topics to which to subscribe.
- description string? - A description of this subscription in particular
- createdAt string? - When the subscription was created
- authentication WebhooksubscriptionAuthentication? - Webhook authentication mechanism
atspoke: WebhooksubscriptionAuthentication
Webhook authentication mechanism
Fields
- 'type string? - The type of authentication for this webhook. Currently, client_secret is the default and only supported value. This means that a secret will be sent in the headers of each request (as the "Client-Secret" header).
- clientSecret string? - The secret sent with each request. Set only if authentication.type is client_secret.
atspoke: WebhookSubscriptionPostBody
Webhook Subscription object.
Fields
- 'client string - A description of the client software that created the webhook.
- url string - The URL to which webhooks will be posted. Must be HTTPS
- topics string[]? - An array of the topics to which to subscribe. If this is left empty, it will default to an empty array.
- request.created is fired when a request is created
- resource.created is fired when a resource is created
- request.updated is fired when a request is updated
- Change in request status
- Change in associated requestType
- Change in request tags
- Change in form fields
- description string? - A description of this subscription in particular
- enabled boolean? - if false, webhook will not actually be sent.
- authentication WebhooksubscriptionpostbodyAuthentication? - Authentication info for this webhook.
atspoke: WebhooksubscriptionpostbodyAuthentication
Authentication info for this webhook.
Fields
- clientSecret string? - A secret that will be sent with every fired webhook. Use this to confirm that webhooks are coming from atSpoke.
String types
Import
import ballerinax/atspoke;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1
Current verison: 1
Weekly downloads
Keywords
Support/Customer Support
Cost/Paid
Contributors
Dependencies