asana
Module asana
API
Definitions
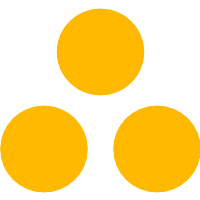
ballerinax/asana Ballerina library
Overview
This is a generated connector for Asana API v1.0 OpenAPI specification.
This API enables you to help teams organize, track and manage their work. For additional help getting started with the API, visit Asana API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Asana Account
- Obtaining tokens
- Log into Asana Account
- Token can be obtained from Developer app console by creating an app and get an access token.
Quickstart
To use the Asana connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/asana module into the Ballerina project.
import ballerinax/asana;
Step 2: Create a new connector instance
configurable http:BearerTokenConfig & readonly auth = ?; asana:ClientConfig clientConfig = { auth : auth }; asana:Client myclient = check new asana:Client(clientConfig, "https://app.asana.com/api/1.0");
Step 3: Invoke connector operation
- You can get tasks related to a specific project.
asana:InlineResponse20018 result = check myclient->getTasks(completedSince="2021-07-16T01:25:40+05:30", project="1200611263773935");
- Use
bal run
command to compile and run the Ballerina program.
Clients
asana: Client
This is a generated connector for Asana API v1.0 OpenAPI specification. This API enables you to help teams organize, track and manage their work. For additional help getting started with the API, visit Asana API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Asana API Account and obtain tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://app.asana.com/api/1.0" - URL of the target service
getAttachment
function getAttachment(string attachmentGid, boolean? optPretty, string[]? optFields) returns InlineResponse200|error
Get an attachment
Parameters
- attachmentGid string - Globally unique identifier for the attachment.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse200|error - Successfully retrieved the record for a single attachment.
deleteAttachment
function deleteAttachment(string attachmentGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete an attachment
Parameters
- attachmentGid string - Globally unique identifier for the attachment.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully deleted the specified attachment.
createBatchRequest
function createBatchRequest(BatchBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2002|error
Submit parallel requests
Parameters
- payload BatchBody - The requests to batch together via the Batch API.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2002|error - Successfully completed the requested batch API operations.
createCustomField
function createCustomField(CustomFieldsBody payload, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse201|error
Create a custom field
Parameters
- payload CustomFieldsBody - The custom field object to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse201|error - Custom field successfully created.
getCustomField
function getCustomField(string customFieldGid, boolean? optPretty, string[]? optFields) returns InlineResponse201|error
Get a custom field
Parameters
- customFieldGid string - Globally unique identifier for the custom field.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse201|error - Successfully retrieved the complete definition of a custom field’s metadata.
updateCustomField
function updateCustomField(string customFieldGid, CustomFieldsCustomFieldGidBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse201|error
Update a custom field
Parameters
- customFieldGid string - Globally unique identifier for the custom field.
- payload CustomFieldsCustomFieldGidBody - The custom field object with all updated properties.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse201|error - The custom field was successfully updated.
deleteCustomField
function deleteCustomField(string customFieldGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete a custom field
Parameters
- customFieldGid string - Globally unique identifier for the custom field.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - The custom field was successfully deleted.
createEnumOptionForCustomField
function createEnumOptionForCustomField(string customFieldGid, CustomFieldGidEnumOptionsBody payload, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2011|error
Create an enum option
Parameters
- customFieldGid string - Globally unique identifier for the custom field.
- payload CustomFieldGidEnumOptionsBody - The enum option object to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2011|error - Custom field enum option successfully created.
insertEnumOptionForCustomField
function insertEnumOptionForCustomField(string customFieldGid, EnumOptionsInsertBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2011|error
Reorder a custom field's enum
Parameters
- customFieldGid string - Globally unique identifier for the custom field.
- payload EnumOptionsInsertBody - The enum option object to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2011|error - Custom field enum option successfully reordered.
updateEnumOption
function updateEnumOption(string enumOptionGid, EnumOptionsEnumOptionGidBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2011|error
Update an enum option
Parameters
- enumOptionGid string - Globally unique identifier for the enum option.
- payload EnumOptionsEnumOptionGidBody - The enum option object to update
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2011|error - Successfully updated the specified custom field enum.
getEvents
function getEvents(string 'resource, string? sync, boolean? optPretty, string[]? optFields) returns InlineResponse2003|error
Get events on a resource
Parameters
- 'resource string - A resource ID to subscribe to. The resource can be a task or project.
- sync string? (default ()) - A sync token received from the last request, or none on first sync. Events will be returned from the point in time that the sync token was generated. Note: On your first request, omit the sync token. The response will be the same as for an expired sync token, and will include a new valid sync token.If the sync token is too old (which may happen from time to time) the API will return a
412 Precondition Failed
error, and include a fresh sync token in the response.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2003|error - Successfully retrieved events.
getJob
function getJob(string jobGid, boolean? optPretty, string[]? optFields) returns InlineResponse2004|error
Get a job by id
Parameters
- jobGid string - Globally unique identifier for the job.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2004|error - Successfully retrieved Job.
createOrganizationExport
function createOrganizationExport(OrganizationExportsBody payload, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2012|error
Create an organization export request
Parameters
- payload OrganizationExportsBody - The organization to export.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2012|error - Successfully created organization export request.
getOrganizationExport
function getOrganizationExport(string organizationExportGid, boolean? optPretty, string[]? optFields) returns InlineResponse2012|error
Get details on an org export request
Parameters
- organizationExportGid string - Globally unique identifier for the organization export.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2012|error - Successfully retrieved organization export object.
getTeamsForOrganization
function getTeamsForOrganization(string workspaceGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2005|error
Get teams in an organization
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2005|error - Returns the team records for all teams in the organization or workspace accessible to the authenticated user.
getPortfolioMemberships
function getPortfolioMemberships(string? portfolio, string? workspace, string? user, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2006|error
Get multiple portfolio memberships
Parameters
- portfolio string? (default ()) - The portfolio to filter results on.
- workspace string? (default ()) - The workspace to filter results on.
- user string? (default ()) - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2006|error - Successfully retrieved portfolio memberships.
getPortfolioMembership
function getPortfolioMembership(string portfolioMembershipGid, boolean? optPretty, string[]? optFields) returns InlineResponse2007|error
Get a portfolio membership
Parameters
- portfolioMembershipGid string - Globally unique identifier for the portfolio membership
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2007|error - Successfully retrieved the requested portfolio membership.
getPortfolios
function getPortfolios(string workspace, string owner, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2008|error
Get multiple portfolios
Parameters
- workspace string - The workspace or organization to filter portfolios on.
- owner string - The user who owns the portfolio. Currently, API users can only get a list of portfolios that they themselves own.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2008|error - Successfully retrieved portfolios.
createPortfolio
function createPortfolio(PortfoliosBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2013|error
Create a portfolio
Parameters
- payload PortfoliosBody - The portfolio to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2013|error - Successfully created portfolio.
getPortfolio
function getPortfolio(string portfolioGid, boolean? optPretty, string[]? optFields) returns InlineResponse2013|error
Get a portfolio
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2013|error - Successfully retrieved the requested portfolio.
updatePortfolio
function updatePortfolio(string portfolioGid, PortfoliosPortfolioGidBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2013|error
Update a portfolio
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- payload PortfoliosPortfolioGidBody - The updated fields for the portfolio.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2013|error - Successfully updated the portfolio.
deletePortfolio
function deletePortfolio(string portfolioGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete a portfolio
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully deleted the specified portfolio.
addCustomFieldSettingForPortfolio
function addCustomFieldSettingForPortfolio(string portfolioGid, PortfolioGidAddcustomfieldsettingBody payload, boolean? optPretty) returns InlineResponse2001|error
Add a custom field to a portfolio
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- payload PortfolioGidAddcustomfieldsettingBody - Information about the custom field setting.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
Return Type
- InlineResponse2001|error - Successfully added the custom field to the portfolio.
addItemForPortfolio
function addItemForPortfolio(string portfolioGid, PortfolioGidAdditemBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Add a portfolio item
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- payload PortfolioGidAdditemBody - Information about the item being inserted.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully added the item to the portfolio.
addMembersForPortfolio
function addMembersForPortfolio(string portfolioGid, PortfolioGidAddmembersBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Add users to a portfolio
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- payload PortfolioGidAddmembersBody - Information about the members being added.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully added members to the portfolio.
getCustomFieldSettingsForPortfolio
function getCustomFieldSettingsForPortfolio(string portfolioGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2009|error
Get a portfolio's custom fields
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2009|error - Successfully retrieved custom field settings objects for a portfolio.
getItemsForPortfolio
function getItemsForPortfolio(string portfolioGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20010|error
Get portfolio items
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20010|error - Successfully retrieved the requested portfolio's items.
getPortfolioMembershipsForPortfolio
function getPortfolioMembershipsForPortfolio(string portfolioGid, string? user, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2006|error
Get memberships from a portfolio
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- user string? (default ()) - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2006|error - Successfully retrieved the requested portfolio's memberships.
removeCustomFieldSettingForPortfolio
function removeCustomFieldSettingForPortfolio(string portfolioGid, PortfolioGidRemovecustomfieldsettingBody payload, boolean? optPretty) returns InlineResponse2001|error
Remove a custom field from a portfolio
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- payload PortfolioGidRemovecustomfieldsettingBody - Information about the custom field setting being removed.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
Return Type
- InlineResponse2001|error - Successfully removed the custom field from the portfolio.
removeItemForPortfolio
function removeItemForPortfolio(string portfolioGid, PortfolioGidRemoveitemBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove a portfolio item
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- payload PortfolioGidRemoveitemBody - Information about the item being removed.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully removed the item from the portfolio.
removeMembersForPortfolio
function removeMembersForPortfolio(string portfolioGid, PortfolioGidRemovemembersBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove users from a portfolio
Parameters
- portfolioGid string - Globally unique identifier for the portfolio.
- payload PortfolioGidRemovemembersBody - Information about the members being removed.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully removed the members from the portfolio.
getProjectMembership
function getProjectMembership(string projectMembershipGid, boolean? optPretty, string[]? optFields) returns InlineResponse20011|error
Get a project membership
Parameters
- projectMembershipGid string - Globally unique identifier for the project membership.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20011|error - Successfully retrieved the requested project membership.
getProjectStatus
function getProjectStatus(string projectStatusGid, boolean? optPretty, string[]? optFields) returns InlineResponse20012|error
Get a project status
Parameters
- projectStatusGid string - The project status update to get.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20012|error - Successfully retrieved the specified project's status updates.
deleteProjectStatus
function deleteProjectStatus(string projectStatusGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete a project status
Parameters
- projectStatusGid string - The project status update to get.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully deleted the specified project status.
getProjects
function getProjects(boolean? optPretty, string[]? optFields, int? 'limit, string? offset, string? workspace, string? team, boolean? archived) returns InlineResponse20010|error
Get multiple projects
Parameters
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
- workspace string? (default ()) - The workspace or organization to filter projects on.
- team string? (default ()) - The team to filter projects on.
- archived boolean? (default ()) - Only return projects whose
archived
field takes on the value of this parameter.
Return Type
- InlineResponse20010|error - Successfully retrieved projects.
createProject
function createProject(ProjectsBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2014|error
Create a project
Parameters
- payload ProjectsBody - The project to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2014|error - Successfully retrieved projects.
getProject
function getProject(string projectGid, boolean? optPretty, string[]? optFields) returns InlineResponse2014|error
Get a project
Parameters
- projectGid string - Globally unique identifier for the project.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2014|error - Successfully retrieved the requested project.
updateProject
function updateProject(string projectGid, ProjectsProjectGidBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2014|error
Update a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectsProjectGidBody - The updated fields for the project.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2014|error - Successfully updated the project.
deleteProject
function deleteProject(string projectGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete a project
Parameters
- projectGid string - Globally unique identifier for the project.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully deleted the specified project.
addCustomFieldSettingForProject
function addCustomFieldSettingForProject(string projectGid, ProjectGidAddcustomfieldsettingBody payload, boolean? optPretty) returns InlineResponse20013|error
Add a custom field to a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidAddcustomfieldsettingBody - Information about the custom field setting.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
Return Type
- InlineResponse20013|error - Successfully added the custom field to the project.
addFollowersForProject
function addFollowersForProject(string projectGid, ProjectGidAddfollowersBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Add followers to a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidAddfollowersBody - Information about the followers being added.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully added followers to the project.
addMembersForProject
function addMembersForProject(string projectGid, ProjectGidAddmembersBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Add users to a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidAddmembersBody - Information about the members being added.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully added members to the project.
getCustomFieldSettingsForProject
function getCustomFieldSettingsForProject(string projectGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2009|error
Get a project's custom fields
Parameters
- projectGid string - Globally unique identifier for the project.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2009|error - Successfully retrieved custom field settings objects for a project.
duplicateProject
function duplicateProject(string projectGid, ProjectGidDuplicateBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2004|error
Duplicate a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidDuplicateBody - Describes the duplicate's name and the elements that will be duplicated.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2004|error - Successfully created the job to handle duplication.
getProjectMembershipsForProject
function getProjectMembershipsForProject(string projectGid, string? user, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20014|error
Get memberships from a project
Parameters
- projectGid string - Globally unique identifier for the project.
- user string? (default ()) - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20014|error - Successfully retrieved the requested project's memberships.
getProjectStatusesForProject
function getProjectStatusesForProject(string projectGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20015|error
Get statuses from a project
Parameters
- projectGid string - Globally unique identifier for the project.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20015|error - Successfully retrieved the specified project's status updates.
createProjectStatusForProject
function createProjectStatusForProject(string projectGid, ProjectGidProjectStatusesBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20012|error
Create a project status
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidProjectStatusesBody - The project status to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20012|error - Successfully created a new story.
removeCustomFieldSettingForProject
function removeCustomFieldSettingForProject(string projectGid, ProjectGidRemovecustomfieldsettingBody payload, boolean? optPretty) returns InlineResponse2001|error
Remove a custom field from a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidRemovecustomfieldsettingBody - Information about the custom field setting being removed.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
Return Type
- InlineResponse2001|error - Successfully removed the custom field from the project.
removeFollowersForProject
function removeFollowersForProject(string projectGid, ProjectGidRemovefollowersBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove followers from a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidRemovefollowersBody - Information about the followers being removed.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully removed followers from the project.
removeMembersForProject
function removeMembersForProject(string projectGid, ProjectGidRemovemembersBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove users from a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidRemovemembersBody - Information about the members being removed.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully removed the members from the project.
getSectionsForProject
function getSectionsForProject(string projectGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20016|error
Get sections in a project
Parameters
- projectGid string - Globally unique identifier for the project.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20016|error - Successfully retrieved sections in project.
createSectionForProject
function createSectionForProject(string projectGid, ProjectGidSectionsBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2015|error
Create a section in a project
Parameters
- projectGid string - Globally unique identifier for the project.
- payload ProjectGidSectionsBody - The section to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2015|error - Successfully created the specified section.
insertSectionForProject
function insertSectionForProject(string projectGid, SectionsInsertBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Move or Insert sections
Parameters
- projectGid string - Globally unique identifier for the project.
- payload SectionsInsertBody - The section's move action.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully moved the specified section.
getTaskCountsForProject
function getTaskCountsForProject(string projectGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20017|error
Get task count of a project
Parameters
- projectGid string - Globally unique identifier for the project.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20017|error - Successfully retrieved the requested project's task counts.
getTasksForProject
function getTasksForProject(string projectGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20018|error
Get tasks from a project
Parameters
- projectGid string - Globally unique identifier for the project.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20018|error - Successfully retrieved the requested project's tasks.
getSection
function getSection(string sectionGid, boolean? optPretty, string[]? optFields) returns InlineResponse2015|error
Get a section
Parameters
- sectionGid string - The globally unique identifier for the section.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2015|error - Successfully retrieved section.
updateSection
function updateSection(string sectionGid, SectionsSectionGidBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2015|error
Update a section
Parameters
- sectionGid string - The globally unique identifier for the section.
- payload SectionsSectionGidBody - The section to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2015|error - Successfully updated the specified section.
deleteSection
function deleteSection(string sectionGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete a section
Parameters
- sectionGid string - The globally unique identifier for the section.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully deleted the specified section.
addTaskForSection
function addTaskForSection(string sectionGid, SectionGidAddtaskBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Add task to section
Parameters
- sectionGid string - The globally unique identifier for the section.
- payload SectionGidAddtaskBody - The task and optionally the insert location.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully added the task.
getTasksForSection
function getTasksForSection(string sectionGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20018|error
Get tasks from a section
Parameters
- sectionGid string - The globally unique identifier for the section.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20018|error - Successfully retrieved the section's tasks.
getStory
function getStory(string storyGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20019|error
Get a story
Parameters
- storyGid string - Globally unique identifier for the story.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20019|error - Successfully retrieved the specified story.
updateStory
function updateStory(string storyGid, StoriesStoryGidBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20019|error
Update a story
Parameters
- storyGid string - Globally unique identifier for the story.
- payload StoriesStoryGidBody - The comment story to update.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20019|error - Successfully retrieved the specified story.
deleteStory
function deleteStory(string storyGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete a story
Parameters
- storyGid string - Globally unique identifier for the story.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully deleted the specified story.
getTags
function getTags(boolean? optPretty, string[]? optFields, int? 'limit, string? offset, string? workspace) returns InlineResponse20020|error
Get multiple tags
Parameters
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
- workspace string? (default ()) - The workspace to filter tags on.
Return Type
- InlineResponse20020|error - Successfully retrieved the specified set of tags.
createTag
function createTag(TagsBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2016|error
Create a tag
Parameters
- payload TagsBody - The tag to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2016|error - Successfully created the newly specified tag.
getTag
function getTag(string tagGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2016|error
Get a tag
Parameters
- tagGid string - Globally unique identifier for the tag.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2016|error - Successfully retrieved the specified tag.
updateTag
function updateTag(string tagGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2016|error
Update a tag
Parameters
- tagGid string - Globally unique identifier for the tag.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2016|error - Successfully updated the specified tag.
deleteTag
function deleteTag(string tagGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2001|error
Delete a tag
Parameters
- tagGid string - Globally unique identifier for the tag.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2001|error - Successfully deleted the specified tag.
getTasksForTag
function getTasksForTag(string tagGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20018|error
Get tasks from a tag
Parameters
- tagGid string - Globally unique identifier for the tag.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20018|error - Successfully retrieved the tasks associated with the specified tag.
getTasks
function getTasks(boolean? optPretty, string[]? optFields, int? 'limit, string? offset, string? assignee, string? project, string? section, string? workspace, string? completedSince, string? modifiedSince) returns InlineResponse20018|error
Get multiple tasks
Parameters
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
- assignee string? (default ()) - The assignee to filter tasks on. Note: If you specify
assignee
, you must also specify theworkspace
to filter on.
- project string? (default ()) - The project to filter tasks on.
- section string? (default ()) - The section to filter tasks on. Note: Currently, this is only supported in board views.
- workspace string? (default ()) - The workspace to filter tasks on. Note: If you specify
workspace
, you must also specify theassignee
to filter on.
- completedSince string? (default ()) - Only return tasks that are either incomplete or that have been completed since this time.
- modifiedSince string? (default ()) - Only return tasks that have been modified since the given time. Note: A task is considered “modified” if any of its properties change, or associations between it and other objects are modified (e.g. a task being added to a project). A task is not considered modified just because another object it is associated with (e.g. a subtask) is modified. Actions that count as modifying the task include assigning, renaming, completing, and adding stories.
Return Type
- InlineResponse20018|error - Successfully retrieved requested tasks.
createTask
function createTask(TasksBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2017|error
Create a task
Parameters
- payload TasksBody - Create Task Request
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2017|error - Successfully created a new task.
getTask
function getTask(string taskGid, boolean? optPretty, string[]? optFields) returns InlineResponse2017|error
Get a task
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2017|error - Successfully retrieved the specified task.
updateTask
function updateTask(string taskGid, TasksTaskGidBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2017|error
Update a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TasksTaskGidBody - The task to update.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2017|error - Successfully updated the specified task.
deleteTask
function deleteTask(string taskGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete a task
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully deleted the specified task.
addDependenciesForTask
function addDependenciesForTask(string taskGid, TaskGidAdddependenciesBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Set dependencies for a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidAdddependenciesBody - The list of tasks to set as dependencies.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully set the specified dependencies on the task.
addDependentsForTask
function addDependentsForTask(string taskGid, TaskGidAdddependentsBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20018|error
Set dependents for a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidAdddependentsBody - The list of tasks to add as dependents.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20018|error - Successfully set the specified dependents on the given task.
addFollowersForTask
function addFollowersForTask(string taskGid, TaskGidAddfollowersBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Add followers to a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidAddfollowersBody - The followers to add to the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully added the specified followers to the task.
addProjectForTask
function addProjectForTask(string taskGid, TaskGidAddprojectBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Add a project to a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidAddprojectBody - The project to add the task to.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully added the specified project to the task.
addTagForTask
function addTagForTask(string taskGid, TaskGidAddtagBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Add a tag to a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidAddtagBody - The tag to add to the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully added the specified tag to the task.
getAttachmentsForTask
function getAttachmentsForTask(string taskGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20021|error
Get attachments for a task
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20021|error - Successfully retrieved the compact records for all attachments on the task.
createAttachmentForTask
function createAttachmentForTask(string taskGid, AttachmentRequest payload, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse200|error
Upload an attachment
Parameters
- taskGid string - Globally unique identifier for the task.
- payload AttachmentRequest - The file you want to upload.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse200|error - Successfully uploaded the attachment to the task.
getDependenciesForTask
function getDependenciesForTask(string taskGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20018|error
Get dependencies from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20018|error - Successfully retrieved the specified task's dependencies.
getDependentsForTask
function getDependentsForTask(string taskGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20018|error
Get dependents from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20018|error - Successfully retrieved the specified dependents of the task.
duplicateTask
function duplicateTask(string taskGid, TaskGidDuplicateBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2004|error
Duplicate a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidDuplicateBody - Describes the duplicate's name and the fields that will be duplicated.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2004|error - Successfully created the job to handle duplication.
getProjectsForTask
function getProjectsForTask(string taskGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20010|error
Get projects a task is in
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20010|error - Successfully retrieved the projects for the given task.
removeDependenciesForTask
function removeDependenciesForTask(string taskGid, TaskGidRemovedependenciesBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20022|error
Unlink dependencies from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidRemovedependenciesBody - The list of tasks to unlink as dependencies.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20022|error - Successfully unlinked the dependencies from the specified task.
removeDependentsForTask
function removeDependentsForTask(string taskGid, TaskGidRemovedependentsBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20022|error
Unlink dependents from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidRemovedependentsBody - The list of tasks to remove as dependents.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20022|error - Successfully unlinked the specified tasks as dependents.
removeFollowerForTask
function removeFollowerForTask(string taskGid, TaskGidRemovefollowersBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove followers from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidRemovefollowersBody - The followers to remove from the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully removed the specified followers from the task.
removeProjectForTask
function removeProjectForTask(string taskGid, TaskGidRemoveprojectBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove a project from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidRemoveprojectBody - The project to remove the task from.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully removed the specified project from the task.
removeTagForTask
function removeTagForTask(string taskGid, TaskGidRemovetagBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove a tag from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidRemovetagBody - The tag to remove from the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully removed the specified tag from the task.
setParentForTask
function setParentForTask(string taskGid, TaskGidSetparentBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2017|error
Set the parent of a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidSetparentBody - The new parent of the subtask.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2017|error - Successfully changed the parent of the specified subtask.
getStoriesForTask
function getStoriesForTask(string taskGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20023|error
Get stories from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20023|error - Successfully retrieved the specified task's stories.
createStoryForTask
function createStoryForTask(string taskGid, TaskGidStoriesBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20019|error
Create a story on a task
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidStoriesBody - The story to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20019|error - Successfully created a new story.
getSubtasksForTask
function getSubtasksForTask(string taskGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20018|error
Get subtasks from a task
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20018|error - Successfully retrieved the specified task's subtasks.
createSubtaskForTask
function createSubtaskForTask(string taskGid, TaskGidSubtasksBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2017|error
Create a subtask
Parameters
- taskGid string - Globally unique identifier for the task.
- payload TaskGidSubtasksBody - The new subtask to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2017|error - Successfully created the specified subtask.
getTagsForTask
function getTagsForTask(string taskGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20020|error
Get a task's tags
Parameters
- taskGid string - Globally unique identifier for the task.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20020|error - Successfully retrieved the tags for the given task.
getTeamMemberships
function getTeamMemberships(boolean? optPretty, string[]? optFields, int? 'limit, string? offset, string? team, string? user, string? workspace) returns InlineResponse20024|error
Get team memberships
Parameters
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
- team string? (default ()) - Globally unique identifier for the team.
- user string? (default ()) - A string identifying a user. This can either be the string "me", an email, or the gid of a user. This parameter must be used with the workspace parameter.
- workspace string? (default ()) - Globally unique identifier for the workspace. This parameter must be used with the user parameter.
Return Type
- InlineResponse20024|error - Successfully retrieved the requested team memberships.
getTeamMembership
function getTeamMembership(string teamMembershipGid, boolean? optPretty, string[]? optFields) returns InlineResponse20025|error
Get a team membership
Parameters
- teamMembershipGid string - Globally unique identifier for the team membership.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20025|error - Successfully retrieved the requested team membership.
createTeam
function createTeam(TeamsBody payload, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2018|error
Create a team
Parameters
- payload TeamsBody - The team to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2018|error - Successfully created a new team.
getTeam
function getTeam(string teamGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2018|error
Get a team
Parameters
- teamGid string - Globally unique identifier for the team.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2018|error - Successsfully retrieved the record for a single team.
addUserForTeam
function addUserForTeam(string teamGid, TeamGidAdduserBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20026|error
Add a user to a team
Parameters
- teamGid string - Globally unique identifier for the team.
- payload TeamGidAdduserBody - The user to add to the team.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20026|error - Returns the full user record for the added user.
getProjectsForTeam
function getProjectsForTeam(string teamGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset, boolean? archived) returns InlineResponse20010|error
Get a team's projects
Parameters
- teamGid string - Globally unique identifier for the team.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
- archived boolean? (default ()) - Only return projects whose
archived
field takes on the value of this parameter.
Return Type
- InlineResponse20010|error - Successfully retrieved the requested team's projects.
createProjectForTeam
function createProjectForTeam(string teamGid, TeamGidProjectsBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2014|error
Create a project in a team
Parameters
- teamGid string - Globally unique identifier for the team.
- payload TeamGidProjectsBody - The new project to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2014|error - Successfully created the specified project.
removeUserForTeam
function removeUserForTeam(string teamGid, TeamGidRemoveuserBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove a user from a team
Parameters
- teamGid string - Globally unique identifier for the team.
- payload TeamGidRemoveuserBody - The user to remove from the team.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Returns an empty data record
getTeamMembershipsForTeam
function getTeamMembershipsForTeam(string teamGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20024|error
Get memberships from a team
Parameters
- teamGid string - Globally unique identifier for the team.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20024|error - Successfully retrieved the requested team's memberships.
getUsersForTeam
function getUsersForTeam(string teamGid, boolean? optPretty, string[]? optFields, string? offset) returns InlineResponse20027|error
Get users in a team
Parameters
- teamGid string - Globally unique identifier for the team.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20027|error - Returns the user records for all the members of the team, including guests and limited access users
getUserTaskList
function getUserTaskList(string userTaskListGid, boolean? optPretty, string[]? optFields) returns InlineResponse20028|error
Get a user task list
Parameters
- userTaskListGid string - Globally unique identifier for the user task list.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20028|error - Successfully retrieved the user task list.
getTasksForUserTaskList
function getTasksForUserTaskList(string userTaskListGid, string? completedSince, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20018|error
Get tasks from a user task list
Parameters
- userTaskListGid string - Globally unique identifier for the user task list.
- completedSince string? (default ()) - Only return tasks that are either incomplete or that have been completed since this time. Accepts a date-time string or the keyword now.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20018|error - Successfully retrieved the user task list's tasks.
getUsers
function getUsers(string? workspace, string? team, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20027|error
Get multiple users
Parameters
- workspace string? (default ()) - The workspace or organization ID to filter users on.
- team string? (default ()) - The team ID to filter users on.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20027|error - Successfully retrieved the requested user records.
getUser
function getUser(string userGid, boolean? optPretty, string[]? optFields) returns InlineResponse20026|error
Get a user
Parameters
- userGid string - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20026|error - Returns the user specified.
getFavoritesForUser
function getFavoritesForUser(string userGid, string resourceType, string workspace, boolean? optPretty, string[]? optFields) returns InlineResponse20029|error
Get a user's favorites
Parameters
- userGid string - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- resourceType string - The resource type of favorites to be returned.
- workspace string - The workspace in which to get favorites.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20029|error - Returns the specified user's favorites.
getTeamMembershipsForUser
function getTeamMembershipsForUser(string userGid, string workspace, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20024|error
Get memberships from a user
Parameters
- userGid string - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- workspace string - Globally unique identifier for the workspace.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20024|error - Successfully retrieved the requested users's memberships.
getTeamsForUser
function getTeamsForUser(string userGid, string organization, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse2005|error
Get teams for a user
Parameters
- userGid string - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- organization string - The workspace or organization to filter teams on.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse2005|error - Returns the team records for all teams in the organization or workspace to which the given user is assigned.
getUserTaskListForUser
function getUserTaskListForUser(string userGid, string workspace, boolean? optPretty, string[]? optFields) returns InlineResponse20028|error
Get a user's task list
Parameters
- userGid string - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- workspace string - The workspace in which to get the user task list.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20028|error - Successfully retrieved the user's task list.
getWorkspaceMembershipsForUser
function getWorkspaceMembershipsForUser(string userGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20030|error
Get workspace memberships for a user
Parameters
- userGid string - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20030|error - Successfully retrieved the requested user's workspace memberships.
getWebhooks
function getWebhooks(string workspace, boolean? optPretty, string[]? optFields, int? 'limit, string? offset, string? 'resource) returns InlineResponse20031|error
Get multiple webhooks
Parameters
- workspace string - The workspace to query for webhooks in.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
- 'resource string? (default ()) - Only return webhooks for the given resource.
Return Type
- InlineResponse20031|error - Successfully retrieved the requested webhooks.
createWebhook
function createWebhook(WebhooksBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2019|error
Establish a webhook
Parameters
- payload WebhooksBody - The webhook workspace and target.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2019|error - Successfully created the requested webhook.
getWebhook
function getWebhook(string webhookGid, boolean? optPretty, string[]? optFields) returns InlineResponse2019|error
Get a webhook
Parameters
- webhookGid string - Globally unique identifier for the webhook.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2019|error - Successfully retrieved the requested webhook.
deleteWebhook
function deleteWebhook(string webhookGid, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Delete a webhook
Parameters
- webhookGid string - Globally unique identifier for the webhook.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - Successfully retrieved the requested webhook.
getWorkspaceMembership
function getWorkspaceMembership(string workspaceMembershipGid, boolean? optPretty, string[]? optFields) returns InlineResponse20032|error
Get a workspace membership
Parameters
- workspaceMembershipGid string - Globally unique identifier for the workspace membership
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20032|error - Successfully retrieved the requested workspace membership.
getWorkspaces
function getWorkspaces(boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20033|error
Get multiple workspaces
Parameters
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20033|error - Return all workspaces visible to the authorized user.
getWorkspace
function getWorkspace(string workspaceGid, boolean? optPretty, string[]? optFields) returns InlineResponse20034|error
Get a workspace
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20034|error - Return the full workspace record.
updateWorkspace
function updateWorkspace(string workspaceGid, WorkspacesWorkspaceGidBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20034|error
Update a workspace
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- payload WorkspacesWorkspaceGidBody - The workspace object with all updated properties.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20034|error - Update for the workspace was successful.
addUserForWorkspace
function addUserForWorkspace(string workspaceGid, WorkspaceGidAdduserBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse20026|error
Add a user to a workspace or organization
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- payload WorkspaceGidAdduserBody - The user to add to the workspace.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20026|error - The user was added successfully to the workspace or organization.
getCustomFieldsForWorkspace
function getCustomFieldsForWorkspace(string workspaceGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20035|error
Get a workspace's custom fields
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20035|error - Successfully retrieved all custom fields for the given workspace.
getProjectsForWorkspace
function getProjectsForWorkspace(string workspaceGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset, boolean? archived) returns InlineResponse20010|error
Get all projects in a workspace
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
- archived boolean? (default ()) - Only return projects whose
archived
field takes on the value of this parameter.
Return Type
- InlineResponse20010|error - Successfully retrieved the requested workspace's projects.
createProjectForWorkspace
function createProjectForWorkspace(string workspaceGid, WorkspaceGidProjectsBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2014|error
Create a project in a workspace
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- payload WorkspaceGidProjectsBody - The new project to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2014|error - Successfully created a new project in the specified workspace.
removeUserForWorkspace
function removeUserForWorkspace(string workspaceGid, WorkspaceGidRemoveuserBody payload, boolean? optPretty, string[]? optFields) returns InlineResponse2001|error
Remove a user from a workspace or organization
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- payload WorkspaceGidRemoveuserBody - The user to remove from the workspace.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse2001|error - The user was removed successfully to the workspace or organization.
getTagsForWorkspace
function getTagsForWorkspace(string workspaceGid, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20020|error
Get tags in a workspace
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20020|error - Successfully retrieved the specified set of tags.
createTagForWorkspace
function createTagForWorkspace(string workspaceGid, WorkspaceGidTagsBody payload, boolean? optPretty, string[]? optFields) returns WorkspaceGidTagsBody|error
Create a tag in a workspace
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- payload WorkspaceGidTagsBody - The tag to create.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- WorkspaceGidTagsBody|error - Successfully created the newly specified tag.
searchTasksForWorkspace
function searchTasksForWorkspace(string workspaceGid, boolean? optPretty, string[]? optFields, string? text, string resourceSubtype, string? assigneeAny, string? assigneeNot, string? portfoliosAny, string? projectsAny, string? projectsNot, string? projectsAll, string? sectionsAny, string? sectionsNot, string? sectionsAll, string? tagsAny, string? tagsNot, string? tagsAll, string? teamsAny, string? followersAny, string? followersNot, string? createdByAny, string? createdByNot, string? assignedByAny, string? assignedByNot, string? likedByAny, string? likedByNot, string? commentedOnByAny, string? commentedOnByNot, string? dueOnBefore, string? dueOnAfter, string? dueOn, string? dueAtBefore, string? dueAtAfter, string? startOnBefore, string? startOnAfter, string? startOn, string? createdOnBefore, string? createdOnAfter, string? createdOn, string? createdAtBefore, string? createdAtAfter, string? completedOnBefore, string? completedOnAfter, string? completedOn, string? completedAtBefore, string? completedAtAfter, string? modifiedOnBefore, string? modifiedOnAfter, string? modifiedOn, string? modifiedAtBefore, string? modifiedAtAfter, boolean? isBlocking, boolean? isBlocked, boolean? hasAttachment, boolean? completed, boolean? isSubtask, string sortBy, boolean sortAscending) returns InlineResponse20018|error
Search tasks in a workspace
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- text string? (default ()) - Performs full-text search on both task name and description
- resourceSubtype string (default "milestone") - Filters results by the task's resource_subtype
- assigneeAny string? (default ()) - Comma-separated list of user identifiers
- assigneeNot string? (default ()) - Comma-separated list of user identifiers
- portfoliosAny string? (default ()) - Comma-separated list of portfolio IDs
- projectsAny string? (default ()) - Comma-separated list of project IDs
- projectsNot string? (default ()) - Comma-separated list of project IDs
- projectsAll string? (default ()) - Comma-separated list of project IDs
- sectionsAny string? (default ()) - Comma-separated list of section or column IDs
- sectionsNot string? (default ()) - Comma-separated list of section or column IDs
- sectionsAll string? (default ()) - Comma-separated list of section or column IDs
- tagsAny string? (default ()) - Comma-separated list of tag IDs
- tagsNot string? (default ()) - Comma-separated list of tag IDs
- tagsAll string? (default ()) - Comma-separated list of tag IDs
- teamsAny string? (default ()) - Comma-separated list of team IDs
- followersAny string? (default ()) - Comma-separated list of user identifiers
- followersNot string? (default ()) - Comma-separated list of user identifiers
- createdByAny string? (default ()) - Comma-separated list of user identifiers
- createdByNot string? (default ()) - Comma-separated list of user identifiers
- assignedByAny string? (default ()) - Comma-separated list of user identifiers
- assignedByNot string? (default ()) - Comma-separated list of user identifiers
- likedByAny string? (default ()) - Comma-separated list of user identifiers
- likedByNot string? (default ()) - Comma-separated list of user identifiers
- commentedOnByAny string? (default ()) - Comma-separated list of user identifiers
- commentedOnByNot string? (default ()) - Comma-separated list of user identifiers
- dueOnBefore string? (default ()) - ISO 8601 date string
- dueOnAfter string? (default ()) - ISO 8601 date string
- dueOn string? (default ()) - ISO 8601 date string or
null
- dueAtBefore string? (default ()) - ISO 8601 datetime string
- dueAtAfter string? (default ()) - ISO 8601 datetime string
- startOnBefore string? (default ()) - ISO 8601 date string
- startOnAfter string? (default ()) - ISO 8601 date string
- startOn string? (default ()) - ISO 8601 date string or
null
- createdOnBefore string? (default ()) - ISO 8601 date string
- createdOnAfter string? (default ()) - ISO 8601 date string
- createdOn string? (default ()) - ISO 8601 date string or
null
- createdAtBefore string? (default ()) - ISO 8601 datetime string
- createdAtAfter string? (default ()) - ISO 8601 datetime string
- completedOnBefore string? (default ()) - ISO 8601 date string
- completedOnAfter string? (default ()) - ISO 8601 date string
- completedOn string? (default ()) - ISO 8601 date string or
null
- completedAtBefore string? (default ()) - ISO 8601 datetime string
- completedAtAfter string? (default ()) - ISO 8601 datetime string
- modifiedOnBefore string? (default ()) - ISO 8601 date string
- modifiedOnAfter string? (default ()) - ISO 8601 date string
- modifiedOn string? (default ()) - ISO 8601 date string or
null
- modifiedAtBefore string? (default ()) - ISO 8601 datetime string
- modifiedAtAfter string? (default ()) - ISO 8601 datetime string
- isBlocking boolean? (default ()) - Filter to incomplete tasks with dependents
- isBlocked boolean? (default ()) - Filter to tasks with incomplete dependencies
- hasAttachment boolean? (default ()) - Filter to tasks with attachments
- completed boolean? (default ()) - Filter to completed tasks
- isSubtask boolean? (default ()) - Filter to subtasks
- sortBy string (default "modified_at") - One of
due_date
,created_at
,completed_at
,likes
, ormodified_at
, defaults tomodified_at
- sortAscending boolean (default false) - Default
false
Return Type
- InlineResponse20018|error - Successfully retrieved the section's tasks.
typeaheadForWorkspace
function typeaheadForWorkspace(string workspaceGid, string resourceType, string 'type, string? query, int? count, boolean? optPretty, string[]? optFields) returns InlineResponse20036|error
Get objects via typeahead
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- resourceType string - The type of values the typeahead should return. You can choose from one of the following:
custom_field
,project
,portfolio
,tag
,task
, anduser
. Note that unlike in the names of endpoints, the types listed here are in singular form (e.g.task
). Using multiple types is not yet supported.
- 'type string (default "user") - Deprecated: new integrations should prefer the resource_type field.
- query string? (default ()) - The string that will be used to search for relevant objects. If an empty string is passed in, the API will currently return an empty result set.
- count int? (default ()) - The number of results to return. The default is 20 if this parameter is omitted, with a minimum of 1 and a maximum of 100. If there are fewer results found than requested, all will be returned.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
Return Type
- InlineResponse20036|error - Successfully retrieved objects via a typeahead search algorithm.
getUsersForWorkspace
function getUsersForWorkspace(string workspaceGid, boolean? optPretty, string[]? optFields, string? offset) returns InlineResponse20027|error
Get users in a workspace or organization
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20027|error - Return the users in the specified workspace or org.
getWorkspaceMembershipsForWorkspace
function getWorkspaceMembershipsForWorkspace(string workspaceGid, string? user, boolean? optPretty, string[]? optFields, int? 'limit, string? offset) returns InlineResponse20030|error
Get the workspace memberships for a workspace
Parameters
- workspaceGid string - Globally unique identifier for the workspace or organization.
- user string? (default ()) - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
- optPretty boolean? (default ()) - Provides the response in a “pretty” format. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging.
- optFields string[]? (default ()) - Defines fields to return. Some requests return compact representations of objects in order to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The id of included objects will always be returned, regardless of the field options.
- 'limit int? (default ()) - Results per page. The number of objects to return per page. The value must be between 1 and 100.
- offset string? (default ()) - Offset token. An offset to the next page returned by the API. A pagination request will return an offset token, which can be used as an input parameter to the next request. If an offset is not passed in, the API will return the first page of results. 'Note: You can only pass in an offset that was returned to you via a previously paginated request.'
Return Type
- InlineResponse20030|error - Successfully retrieved the requested workspace's memberships.
Records
asana: AddCustomFieldSettingRequest
Fields
- custom_field string - The custom field to associate with this container.
- insert_after string? - A gid of a Custom Field Setting on this container, after which the new Custom Field Setting will be added.
insert_before
andinsert_after
parameters cannot both be specified.
- insert_before string? - A gid of a Custom Field Setting on this container, before which the new Custom Field Setting will be added.
insert_before
andinsert_after
parameters cannot both be specified.
- is_important boolean? - Whether this field should be considered important to this container (for instance, to display in the list view of items in the container).
asana: AddFollowersRequest
Fields
- followers string - An array of strings identifying users. These can either be the string "me", an email, or the gid of a user.
asana: AddMembersRequest
Fields
- members string - An array of strings identifying users. These can either be the string "me", an email, or the gid of a user.
asana: AsanaNamedResource
A generic Asana Resource, containing a globally unique identifier.
Fields
- Fields Included from *AsanaResource
- name string? - The name of the object.
asana: AsanaResource
A generic Asana Resource, containing a globally unique identifier.
Fields
- gid string? - Globally unique identifier of the resource, as a string.
- resource_type string? - The base type of this resource.
asana: AttachmentCompact
Fields
- Fields Included from *AsanaResource
- name string? - The name of the file.
- resource_subtype anydata? - The service hosting the attachment. Valid values are
asana
,dropbox
,gdrive
,onedrive
,box
, andexternal
.external
attachments are a beta feature currently limited to specific integrations.
asana: AttachmentRequest
Fields
- file string? -
asana: AttachmentResponse
Fields
- created_at string? - The time at which this resource was created.
- download_url string? - The URL containing the content of the attachment. Note: May be null if the attachment is hosted by Box. If present, this URL may only be valid for two minutes from the time of retrieval. You should avoid persisting this URL somewhere and just refresh it on demand to ensure you do not keep stale URLs.
- host string? - The service hosting the attachment. Valid values are
asana
,dropbox
,gdrive
andbox
.
- parent TaskCompact? - The task is the basic object around which many operations in Asana are centered.
- view_url string? - The URL where the attachment can be viewed, which may be friendlier to users in a browser than just directing them to a raw file. May be null if no view URL exists for the service.
asana: BatchBody
Fields
- data BatchRequest? - A request object for use in a batch request.
asana: BatchRequest
A request object for use in a batch request.
Fields
- actions BatchRequestAction[]? - Batch request actions
asana: BatchRequestAction
An action object for use in a batch request.
Fields
- data record {}? - For
GET
requests, this should be a map of query parameters you would have normally passed in the URL. Options and pagination are not accepted here; put them inoptions
instead. ForPOST
,PATCH
, andPUT
methods, this should be the content you would have normally put in the data field of the body.
- method string - The HTTP method you wish to emulate for the action.
- options BatchrequestactionOptions? - Pagination (
limit
andoffset
) and output options (fields
orexpand
) for the action. “Pretty” JSON output is not an available option on individual actions; if you want pretty output, specify that option on the parent request.
- relative_path string - The path of the desired endpoint relative to the API’s base URL. Query parameters are not accepted here; put them in
data
instead.
asana: BatchrequestactionOptions
Pagination (limit
and offset
) and output options (fields
or expand
) for the action. “Pretty” JSON output is not an available option on individual actions; if you want pretty output, specify that option on the parent request.
Fields
- fields string[]? - The fields to retrieve in the request.
- 'limit int? - Pagination limit for the request.
- offset int? - Pagination offset for the request.
asana: BatchResponse
A response object returned from a batch request.
Fields
- body record {}? - The JSON body that the invoked endpoint returned.
- headers record {}? - A map of HTTP headers specific to this result. This is primarily used for returning a
Location
header to accompany a201 Created
result. The parent HTTP response will contain all common headers.
- status_code int? - The HTTP status code that the invoked endpoint returned.
asana: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
asana: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
asana: CustomFieldBase
Fields
- Fields Included from *CustomFieldCompact
- currency_code string? - ISO 4217 currency code to format this custom field. This will be null if the
format
is notcurrency
.
- custom_label string? - This is the string that appears next to the custom field value. This will be null if the
format
is notcustom
.
- custom_label_position string? - Only relevant for custom fields with
custom
format. This depicts where to place the custom label. This will be null if theformat
is notcustom
.
- enum_options EnumOption[]? - Conditional. Only relevant for custom fields of type enum. This array specifies the possible values which an
enum
custom field can adopt. To modify the enum options, refer to working with enum options.
- format string? - The format of this custom field.
- has_notifications_enabled boolean? - Conditional. This flag describes whether a follower of a task with this field should receive inbox notifications from changes to this field.
- is_global_to_workspace boolean? - This flag describes whether this custom field is available to every container in the workspace. Before project-specific custom fields, this field was always true.
- precision int? - Only relevant for custom fields of type ‘Number’. This field dictates the number of places after the decimal to round to, i.e. 0 is integer values, 1 rounds to the nearest tenth, and so on. Must be between 0 and 6, inclusive. For percentage format, this may be unintuitive, as a value of 0.25 has a precision of 0, while a value of 0.251 has a precision of 1. This is due to 0.25 being displayed as 25%. The identifier format will always have a precision of 0.
asana: CustomFieldCompact
Custom Fields store the metadata that is used in order to add user-specified information to tasks in Asana. Be sure to reference the Custom Fields developer documentation for more information about how custom fields relate to various resources in Asana.
Fields
- Fields Included from *AsanaResource
- display_value string? - A string representation for the value of the custom field. Integrations that don't require the underlying type should use this field to read values. Using this field will future-proof an app against new custom field types.
- enabled boolean? - Conditional. Determines if the custom field is enabled or not.
- enum_options EnumOption[]? - Conditional. Only relevant for custom fields of type enum. This array specifies the possible values which an
enum
custom field can adopt. To modify the enum options, refer to working with enum options.
- name string? - The name of the custom field.
- number_value decimal? - Conditional. This number is the value of a number custom field.
- resource_subtype string? - The type of the custom field. Must be one of the given values.
- text_value string? - Conditional. This string is the value of a text custom field.
- 'type string? - Deprecated: new integrations should prefer the resource_subtype field. The type of the custom field. Must be one of the given values.
asana: CustomFieldGidEnumOptionsBody
Fields
- data EnumOptionRequest? -
asana: CustomFieldRequest
Fields
- Fields Included from *CustomFieldBase
- currency_code string|()
- custom_label string|()
- custom_label_position string
- description string
- enum_options EnumOption[]
- format string
- has_notifications_enabled boolean
- is_global_to_workspace boolean
- precision int
- display_value string
- enabled boolean
- name string
- number_value decimal
- resource_subtype string
- text_value string
- type string
- gid string
- resource_type string
- anydata...
- workspace string - Create-Only The workspace to create a custom field in.
asana: CustomFieldResponse
Fields
- Fields Included from *CustomFieldBase
- currency_code string|()
- custom_label string|()
- custom_label_position string
- description string
- enum_options EnumOption[]
- format string
- has_notifications_enabled boolean
- is_global_to_workspace boolean
- precision int
- display_value string
- enabled boolean
- name string
- number_value decimal
- resource_subtype string
- text_value string
- type string
- gid string
- resource_type string
- anydata...
- created_by UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- enum_value EnumOption? - Enum options are the possible values which an enum custom field can adopt.
asana: CustomFieldsBody
Fields
- data CustomFieldRequest? -
asana: CustomFieldsCustomFieldGidBody
Fields
- data CustomFieldRequest? -
asana: CustomFieldSettingCompact
Fields
- Fields Included from *AsanaResource
asana: CustomFieldSettingResponse
Fields
- custom_field CustomFieldResponse? -
- is_important boolean? -
is_important
is used in the Asana web application to determine if this custom field is displayed in the list/grid view of a project or portfolio.
- parent ProjectCompact? - A project represents a prioritized list of tasks in Asana or a board with columns of tasks represented as cards. It exists in a single workspace or organization and is accessible to a subset of users in that workspace or organization, depending on its permissions.
- project ProjectCompact? - A project represents a prioritized list of tasks in Asana or a board with columns of tasks represented as cards. It exists in a single workspace or organization and is accessible to a subset of users in that workspace or organization, depending on its permissions.
asana: EmptyResponse
An empty object. Some endpoints do not return an object on success. The success is conveyed through a 2-- status code and returning an empty object.
asana: EnumOption
Enum options are the possible values which an enum custom field can adopt.
Fields
- Fields Included from *AsanaResource
- color string? - The color of the enum option. Defaults to ‘none’.
- enabled boolean? - Whether or not the enum option is a selectable value for the custom field.
- name string? - The name of the enum option.
asana: EnumOptionInsertRequest
Fields
- after_enum_option string? - An existing enum option within this custom field after which the new enum option should be inserted. Cannot be provided together with before_enum_option.
- before_enum_option string? - An existing enum option within this custom field before which the new enum option should be inserted. Cannot be provided together with after_enum_option.
- enum_option string - The gid of the enum option to relocate.
asana: EnumOptionRequest
Fields
- insert_after string? - An existing enum option within this custom field after which the new enum option should be inserted. Cannot be provided together with before_enum_option.
- insert_before string? - An existing enum option within this custom field before which the new enum option should be inserted. Cannot be provided together with after_enum_option.
asana: EnumOptionsEnumOptionGidBody
Fields
- data EnumOptionRequest? -
asana: EnumOptionsInsertBody
Fields
- data EnumOptionInsertRequest? -
asana: Error
Fields
- help string? - Additional information directing developers to resources on how to address and fix the problem, if available.
- message string? - Message providing more detail about the error that occurred, if available.
- phrase string? - 500 errors only. A unique error phrase which can be used when contacting developer support to help identify the exact occurrence of the problem in Asana’s logs.
asana: ErrorResponse
Sadly, sometimes requests to the API are not successful. Failures can occur for a wide range of reasons. In all cases, the API should return an HTTP Status Code that indicates the nature of the failure, with a response body in JSON format containing additional information.
In the event of a server error the response body will contain an error phrase. These phrases are automatically generated using the node-asana-phrase library and can be used by Asana support to quickly look up the incident that caused the server error.
Fields
- errors Error[]? - Array of errors when requests to the API are not successful.
asana: EventResponse
An event is an object representing a change to a resource that was observed by an event subscription or delivered asynchronously to the target location of an active webhook.
The event may be triggered by a different user
than the
subscriber. For example, if user A subscribes to a task and user B
modified it, the event’s user will be user B. Note: Some events
are generated by the system, and will have null
as the user. API
consumers should make sure to handle this case.
The resource
that triggered the event may be different from the one
that the events were requested for or the webhook is subscribed to. For
example, a subscription to a project will contain events for tasks
contained within the project.
Note: pay close attention to the relationship between the fields
Event.action
and Event.change.action
.
Event.action
represents the action taken on the resource
itself, and Event.change.action
represents how the information
within the resource's fields have been modified.
For instance, consider these scenarios:
-
When at task is added to a project,
Event.action
will beadded
,Event.parent
will be on object with theid
andtype
of the project, and there will be nochange
field. -
When an assignee is set on the task,
Event.parent
will benull
,Event.action
will bechanged
,Event.change.action
will bechanged
, andchanged_value
will be an object with the user'sid
andtype
. -
When a collaborator is added to the task,
Event.parent
will benull
,Event.action
will bechanged
,Event.change.action
will beadded
, andadded_value
will be an object with the user'sid
andtype
.
Fields
- action string? - The type of action taken on the resource that triggered the event. This can be one of
changed
,added
,removed
,deleted
, orundeleted
depending on the nature of the event.
- change EventresponseChange? - Information about the type of change that has occurred. This field is only present when the value of the property
action
, describing the action taken on the resource, ischanged
.
- created_at string? - The timestamp when the event occurred.
- parent AsanaNamedResource? - A generic Asana Resource, containing a globally unique identifier.
- 'resource AsanaNamedResource? - A generic Asana Resource, containing a globally unique identifier.
- 'type string? - Deprecated: Refer to the resource_type of the resource. The type of the resource that generated the event.
- user UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
asana: EventresponseChange
Information about the type of change that has occurred. This field is only present when the value of the property action
, describing the action taken on the resource, is changed
.
Fields
- action string? - The type of action taken on the field which has been changed. This can be one of
changed
,added
, orremoved
depending on the nature of the change.
- added_value anydata? - Conditional. This property is only present when the field's
action
isadded
and theadded_value
is an Asana resource. This will be only thegid
andresource_type
of the resource when the events come from webhooks; this will be the compact representation (and can have fields expanded with opt_fields) when using the Events resource.
- 'field string? - The name of the field that has changed in the resource.
- new_value anydata? - Conditional. This property is only present when the field's
action
ischanged
and thenew_value
is an Asana resource. This will be only thegid
andresource_type
of the resource when the events come from webhooks; this will be the compact representation (and can have fields expanded with opt_fields) when using the Events resource.
- removed_value anydata? - Conditional. This property is only present when the field's
action
isremoved
and theremoved_value
is an Asana resource. This will be only thegid
andresource_type
of the resource when the events come from webhooks; this will be the compact representation (and can have fields expanded with opt_fields) when using the Events resource.
asana: InlineResponse200
Fields
- data AttachmentResponse? -
asana: InlineResponse2001
Fields
- data EmptyResponse? - An empty object. Some endpoints do not return an object on success. The success is conveyed through a 2-- status code and returning an empty object.
asana: InlineResponse20010
Fields
- data ProjectCompact[]? -
asana: InlineResponse20011
Fields
- data ProjectMembershipResponse? -
asana: InlineResponse20012
Fields
- data ProjectStatusResponse? -
asana: InlineResponse20013
Fields
- data CustomFieldSettingResponse? -
asana: InlineResponse20014
Fields
- data ProjectMembershipCompact[]? -
asana: InlineResponse20015
Fields
- data ProjectStatusCompact[]? -
asana: InlineResponse20016
Fields
- data SectionCompact[]? -
asana: InlineResponse20017
Fields
- data TaskCountResponse? - A response object returned from the task count endpoint.
asana: InlineResponse20018
Fields
- data TaskCompact[]? -
asana: InlineResponse20019
Fields
- data StoryResponse? - Represents activity associated with an object in the Asana system
asana: InlineResponse2002
Fields
- data BatchResponse[]? -
asana: InlineResponse20020
Fields
- data TagCompact[]? -
asana: InlineResponse20021
Fields
- data AttachmentCompact[]? -
asana: InlineResponse20022
Fields
- data EmptyResponse[]? -
asana: InlineResponse20023
Fields
- data StoryCompact[]? -
asana: InlineResponse20024
Fields
- data TeamMembershipCompact[]? -
asana: InlineResponse20025
Fields
- data TeamMembershipResponse? -
asana: InlineResponse20026
Fields
- data UserResponse? -
asana: InlineResponse20027
Fields
- data UserCompact[]? -
asana: InlineResponse20028
Fields
- data UserTaskListResponse? -
asana: InlineResponse20029
Fields
- data AsanaNamedResource[]? -
asana: InlineResponse2003
The full record for all events that have occurred since the sync token was created.
Fields
- data EventResponse[]? - An organization_export object represents a request to export the complete data of an organization.
- sync string? - A sync token to be used with the next call to the events endpoint.
asana: InlineResponse20030
Fields
- data WorkspaceMembershipCompact[]? -
asana: InlineResponse20031
Fields
- data WebhookResponse[]? -
asana: InlineResponse20032
Fields
- data WorkspaceMembershipResponse? -
asana: InlineResponse20033
Fields
- data WorkspaceCompact[]? -
asana: InlineResponse20034
Fields
- data WorkspaceResponse? -
asana: InlineResponse20035
Fields
- data CustomFieldResponse[]? -
asana: InlineResponse20036
Fields
- data AsanaNamedResource[]? - The data containing generic Asana Resource, containing a globally unique identifier.
asana: InlineResponse2004
Fields
- data JobResponse? -
asana: InlineResponse2005
Fields
- data TeamCompact[]? -
asana: InlineResponse2006
Fields
- data PortfolioMembershipCompact[]? -
asana: InlineResponse2007
Fields
- data PortfolioMembershipResponse? -
asana: InlineResponse2008
Fields
- data PortfolioCompact[]? -
asana: InlineResponse2009
Fields
- data CustomFieldSettingResponse[]? -
asana: InlineResponse201
Fields
- data CustomFieldResponse? -
asana: InlineResponse2011
Fields
- data EnumOption? - Enum options are the possible values which an enum custom field can adopt.
asana: InlineResponse2012
Fields
- data OrganizationExportResponse? -
asana: InlineResponse2013
Fields
- data PortfolioResponse? -
asana: InlineResponse2014
Fields
- data ProjectResponse? -
asana: InlineResponse2015
Fields
- data SectionResponse? -
asana: InlineResponse2016
Fields
- data TagResponse? -
asana: InlineResponse2017
Fields
- data TaskResponse? -
asana: InlineResponse2018
Fields
- data TeamResponse? -
asana: InlineResponse2019
Fields
- data WebhookResponse? -
asana: JobCompact
Fields
- Fields Included from *AsanaResource
- new_project ProjectCompact? - A project represents a prioritized list of tasks in Asana or a board with columns of tasks represented as cards. It exists in a single workspace or organization and is accessible to a subset of users in that workspace or organization, depending on its permissions.
- new_task TaskCompact? - The task is the basic object around which many operations in Asana are centered.
- resource_subtype string? - The subtype of this resource. Different subtypes retain many of the same fields and behavior, but may render differently in Asana or represent resources with different semantic meaning.
- status string? - Status of job.
asana: Like
An object to represent a user's like.
Fields
- gid string? - Globally unique identifier of the object, as a string.
- user UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
asana: ModifyDependenciesRequest
Fields
- dependencies string[]? - An array of task gids that a task depends on.
asana: ModifyDependentsRequest
A set of dependent tasks.
Fields
- dependents string[]? - An array of task gids that are dependents of the given task.
asana: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://app.asana.com/-/oauth_token") - Refresh URL
asana: OrganizationExportCompact
Fields
- Fields Included from *AsanaResource
- created_at string? - The time at which this resource was created.
- download_url string? - Download this URL to retreive the full export of the organization in JSON format. It will be compressed in a gzip (.gz) container. Note: May be null if the export is still in progress or failed. If present, this URL may only be valid for 1 hour from the time of retrieval. You should avoid persisting this URL somewhere and rather refresh on demand to ensure you do not keep stale URLs.
- organization WorkspaceCompact? - The workspace in which the user task list is located.
- state string? - The current state of the export.
asana: OrganizationExportRequest
An organization_export request starts a job to export the complete data of the given Organization.
Fields
- organization string? - Globally unique identifier for the workspace or organization.
asana: OrganizationExportsBody
Fields
- data OrganizationExportRequest? - An organization_export request starts a job to export the complete data of the given Organization.
asana: PortfolioAddItemRequest
Fields
- insert_after string? - An id of an item in this portfolio. The new item will be added after the one specified here.
insert_before
andinsert_after
parameters cannot both be specified.
- insert_before string? - An id of an item in this portfolio. The new item will be added before the one specified here.
insert_before
andinsert_after
parameters cannot both be specified.
- item string - The item to add to the portfolio.
asana: PortfolioBase
Fields
- Fields Included from *PortfolioCompact
- color string? - Color of the portfolio.
asana: PortfolioCompact
A portfolio gives a high-level overview of the status of multiple initiatives in Asana.
Fields
- Fields Included from *AsanaResource
- name string? - The name of the portfolio.
asana: PortfolioGidAddcustomfieldsettingBody
Fields
- data AddCustomFieldSettingRequest? -
asana: PortfolioGidAdditemBody
Fields
- data PortfolioAddItemRequest? -
asana: PortfolioGidAddmembersBody
Fields
- data AddMembersRequest? -
asana: PortfolioGidRemovecustomfieldsettingBody
Fields
- data RemoveCustomFieldSettingRequest? -
asana: PortfolioGidRemoveitemBody
Fields
- data PortfolioRemoveItemRequest? -
asana: PortfolioGidRemovemembersBody
Fields
- data RemoveMembersRequest? -
asana: PortfolioMembershipCompact
Fields
- Fields Included from *AsanaResource
- portfolio PortfolioCompact? - A portfolio gives a high-level overview of the status of multiple initiatives in Asana.
- user UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
asana: PortfolioRemoveItemRequest
Fields
- item string - The item to remove from the portfolio.
asana: PortfolioRequest
Fields
- Fields Included from *PortfolioBase
- members string[]? - An array of strings identifying users. These can either be the string "me", an email, or the gid of a user.
- workspace string? - Gid of an object.
asana: PortfolioResponse
Fields
- Fields Included from *PortfolioBase
- created_at string? - The time at which this resource was created.
- created_by UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- custom_field_settings CustomFieldSettingResponse[]? - Array of custom field settings applied to the portfolio.
- due_on string? - The localized day on which this portfolio is due. This takes a date with format YYYY-MM-DD.
- members UserCompact[]? -
- owner UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- permalink_url string? - A url that points directly to the object within Asana.
- start_on string? - The day on which work for this portfolio begins, or null if the portfolio has no start date. This takes a date with
YYYY-MM-DD
format. Note:due_on
must be present in the request when setting or unsetting thestart_on
parameter. Additionally, start_on and due_on cannot be the same date.
- workspace WorkspaceCompact? - The workspace in which the user task list is located.
asana: PortfoliosBody
Fields
- data PortfolioRequest? -
asana: PortfoliosPortfolioGidBody
Fields
- data PortfolioRequest? -
asana: Preview
A collection of rich text that will be displayed as a preview to another app.
This is read-only except for a small group of whitelisted apps.
Fields
- fallback string? - Some fallback text to display if unable to display the full preview.
- footer string? - Text to display in the footer.
- header string? - Text to display in the header.
- header_link string? - Where the header will link to.
- html_text string? - HTML formatted text for the body of the preview.
- text string? - Text for the body of the preview.
- title string? - Text to display as the title.
- title_link string? - Where to title will link to.
asana: ProjectBase
Fields
- Fields Included from *ProjectCompact
- archived boolean? - True if the project is archived, false if not. Archived projects do not show in the UI by default and may be treated differently for queries.
- color string? - Color of the project.
- created_at string? - The time at which this resource was created.
- current_status ProjectStatusResponse? -
- custom_field_settings CustomFieldSettingCompact[]? - Array of Custom Field Settings (in compact form).
- default_view string? - The default view (list, board, calendar, or timeline) of a project.
- due_date string? - Deprecated: new integrations should prefer the due_on field.
- due_on string? - The day on which this project is due. This takes a date with format YYYY-MM-DD.
- members UserCompact[]? - Array of users who are members of this project.
- modified_at string? - The time at which this project was last modified. Note: This does not currently reflect any changes in associations such as tasks or comments that may have been added or removed from the project.
- notes string? - More detailed, free-form textual information associated with the project.
- 'public boolean? - True if the project is public to the organization. If false, do not share this project with other users in this organization without explicitly checking to see if they have access.
- start_on string? - The day on which work for this project begins, or null if the project has no start date. This takes a date with
YYYY-MM-DD
format. Note:due_on
ordue_at
must be present in the request when setting or unsetting thestart_on
parameter. Additionally, start_on and due_on cannot be the same date.
- workspace WorkspaceCompact? - The workspace in which the user task list is located.
asana: ProjectCompact
A project represents a prioritized list of tasks in Asana or a board with columns of tasks represented as cards. It exists in a single workspace or organization and is accessible to a subset of users in that workspace or organization, depending on its permissions.
Fields
- Fields Included from *AsanaResource
- name string? - Name of the project. This is generally a short sentence fragment that fits on a line in the UI for maximum readability. However, it can be longer.
asana: ProjectDuplicateRequest
Fields
- include string? - The elements that will be duplicated to the new project. Tasks are always included.
- name string - The name of the new project.
- schedule_dates ProjectduplicaterequestScheduleDates? - A dictionary of options to auto-shift dates.
task_dates
must be included to use this option. Requires eitherstart_on
ordue_on
, but not both.
- team string? - Sets the team of the new project. If team is not defined, the new project will be in the same team as the the original project.
asana: ProjectduplicaterequestScheduleDates
A dictionary of options to auto-shift dates. task_dates
must be included to use this option. Requires either start_on
or due_on
, but not both.
Fields
- due_on string? - Sets the last due date in the duplicated project to the given date. The rest of the due dates will be offset by the same amount as the due dates in the original project.
- should_skip_weekends boolean - Determines if the auto-shifted dates should skip weekends.
- start_on string? - Sets the first start date in the duplicated project to the given date. The rest of the start dates will be offset by the same amount as the start dates in the original project.
asana: ProjectGidAddcustomfieldsettingBody
Fields
- data AddCustomFieldSettingRequest? -
asana: ProjectGidAddfollowersBody
Fields
- data AddFollowersRequest? -
asana: ProjectGidAddmembersBody
Fields
- data AddMembersRequest? -
asana: ProjectGidDuplicateBody
Fields
- data ProjectDuplicateRequest? -
asana: ProjectGidProjectStatusesBody
Fields
- data ProjectStatusRequest? -
asana: ProjectGidRemovecustomfieldsettingBody
Fields
- data RemoveCustomFieldSettingRequest? -
asana: ProjectGidRemovefollowersBody
Fields
- data RemoveFollowersRequest? -
asana: ProjectGidRemovemembersBody
Fields
- data RemoveMembersRequest? -
asana: ProjectGidSectionsBody
Fields
- data SectionRequest? -
asana: ProjectMembershipCompact
Fields
- Fields Included from *AsanaResource
- user UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
asana: ProjectMembershipResponse
Fields
- project ProjectCompact? - A project represents a prioritized list of tasks in Asana or a board with columns of tasks represented as cards. It exists in a single workspace or organization and is accessible to a subset of users in that workspace or organization, depending on its permissions.
- write_access string? - Whether the user has full access to the project or has comment-only access.
asana: ProjectRequest
Fields
- Fields Included from *ProjectBase
- archived boolean
- color string|()
- created_at string
- current_status ProjectStatusResponse|()
- custom_field_settings CustomFieldSettingCompact[]
- default_view string
- due_date string|()
- due_on string|()
- html_notes string
- is_template boolean
- members UserCompact[]
- modified_at string
- notes string
- public boolean
- start_on string|()
- workspace WorkspaceCompact
- name string
- gid string
- resource_type string
- anydata...
- custom_fields record {}? - An object where each key is a Custom Field gid and each value is an enum gid, string, or number.
- followers string? - Create-only. Comma separated string of users. Followers are a subset of members who receive all notifications for a project, the default notification setting when adding members to a project in-product.
- owner string? - The current owner of the project, may be null.
- team string? - Create-only. The team that this project is shared with. This field only exists for projects in organizations.
asana: ProjectResponse
Fields
- Fields Included from *ProjectBase
- archived boolean
- color string|()
- created_at string
- current_status ProjectStatusResponse|()
- custom_field_settings CustomFieldSettingCompact[]
- default_view string
- due_date string|()
- due_on string|()
- html_notes string
- is_template boolean
- members UserCompact[]
- modified_at string
- notes string
- public boolean
- start_on string|()
- workspace WorkspaceCompact
- name string
- gid string
- resource_type string
- anydata...
- custom_fields CustomFieldCompact[]? - Array of Custom Fields.
- followers UserCompact[]? - Array of users following this project. Followers are a subset of members who receive all notifications for a project, the default notification setting when adding members to a project in-product.
- icon string? - The icon for a project.
- owner UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- permalink_url string? - A url that points directly to the object within Asana.
- team TeamCompact? - A team is used to group related projects and people together within an organization.
asana: ProjectsBody
Fields
- data ProjectRequest? -
asana: ProjectSectionInsertRequest
Fields
- after_section string? - Insert the given section immediately after the section specified by this parameter.
- before_section string? - Insert the given section immediately before the section specified by this parameter.
- project string - The project in which to reorder the given section.
- section string - The section to reorder.
asana: ProjectsProjectGidBody
Fields
- data ProjectRequest? -
asana: ProjectStatusBase
Fields
- Fields Included from *ProjectStatusCompact
- author UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- color string - The color associated with the status update.
- modified_at anydata? - The time at which this project status was last modified. Note: This does not currently reflect any changes in associations such as comments that may have been added or removed from the project status.
- text string - The text content of the status update.
asana: ProjectStatusCompact
Fields
- Fields Included from *AsanaResource
- title string? - The title of the project status update.
asana: ProjectStatusResponse
Fields
- Fields Included from *ProjectStatusBase
- created_at string? - The time at which this resource was created.
- created_by UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
asana: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
asana: RemoveCustomFieldSettingRequest
Fields
- custom_field string - The custom field to remove from this portfolio.
asana: RemoveFollowersRequest
Fields
- followers string - An array of strings identifying users. These can either be the string "me", an email, or the gid of a user.
asana: RemoveMembersRequest
Fields
- members string - An array of strings identifying users. These can either be the string "me", an email, or the gid of a user.
asana: SectionCompact
A section is a subdivision of a project that groups tasks together.
Fields
- Fields Included from *AsanaResource
- name string? - The name of the section (i.e. the text displayed as the section header).
asana: SectionGidAddtaskBody
Fields
- data SectionTaskInsertRequest? -
asana: SectionRequest
Fields
- insert_after string? - An existing section within this project after which the added section should be inserted. Cannot be provided together with insert_before.
- insert_before string? - An existing section within this project before which the added section should be inserted. Cannot be provided together with insert_after.
- name string - The text to be displayed as the section name. This cannot be an empty string.
- project string - Create-Only The project to create the section in
asana: SectionResponse
Fields
- created_at string? - The time at which this resource was created.
- project ProjectCompact? - A project represents a prioritized list of tasks in Asana or a board with columns of tasks represented as cards. It exists in a single workspace or organization and is accessible to a subset of users in that workspace or organization, depending on its permissions.
- projects ProjectCompact[]? - Deprecated - please use project instead
asana: SectionsInsertBody
Fields
- data ProjectSectionInsertRequest? -
asana: SectionsSectionGidBody
Fields
- data SectionRequest? -
asana: SectionTaskInsertRequest
Fields
- insert_after string? - An existing task within this section after which the added task should be inserted. Cannot be provided together with insert_before.
- insert_before string? - An existing task within this section before which the added task should be inserted. Cannot be provided together with insert_after.
- task string - The task to add to this section.
asana: StoriesStoryGidBody
Fields
- data StoryRequest? -
asana: StoryBase
Fields
- Fields Included from *AsanaResource
- created_at string? - The time at which this resource was created.
- is_pinned boolean? - Conditional. Whether the story should be pinned on the resource.
- resource_subtype string? - The subtype of this resource. Different subtypes retain many of the same fields and behavior, but may render differently in Asana or represent resources with different semantic meaning.
- sticker_name string? - The name of the sticker in this story.
null
if there is no sticker.
- text string? - The plain text of the comment to add. Cannot be used with html_text.
asana: StoryCompact
A story represents an activity associated with an object in the Asana system.
Fields
- Fields Included from *AsanaResource
- created_at string? - The time at which this resource was created.
- created_by UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- resource_subtype string? - The subtype of this resource. Different subtypes retain many of the same fields and behavior, but may render differently in Asana or represent resources with different semantic meaning.
- text string? - Create-only. Human-readable text for the story or comment.
This will not include the name of the creator.
Note: This is not guaranteed to be stable for a given type of story. For example, text for a reassignment may not always say “assigned to …” as the text for a story can both be edited and change based on the language settings of the user making the request.
Use the
resource_subtype
property to discover the action that created the story.
asana: StoryResponse
Represents activity associated with an object in the Asana system
Fields
- Fields Included from *StoryBase
- assignee UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- created_by UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- custom_field CustomFieldCompact? - Custom Fields store the metadata that is used in order to add user-specified information to tasks in Asana. Be sure to reference the Custom Fields developer documentation for more information about how custom fields relate to various resources in Asana.
- dependency TaskCompact? - The task is the basic object around which many operations in Asana are centered.
- duplicate_of TaskCompact? - The task is the basic object around which many operations in Asana are centered.
- duplicated_from TaskCompact? - The task is the basic object around which many operations in Asana are centered.
- follower UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- hearted boolean? - Deprecated - please use likes instead Conditional. True if the story is hearted by the authorized user, false if not.
- hearts Like[]? - Deprecated - please use likes instead Conditional. Array of likes for users who have hearted this story.
- is_edited boolean? - Conditional. Whether the text of the story has been edited after creation.
- liked boolean? - Conditional. True if the story is liked by the authorized user, false if not.
- likes Like[]? - Conditional. Array of likes for users who have liked this story.
- new_approval_status string? - Conditional
- new_dates StoryResponseDates? - Conditional
- new_enum_value EnumOption? - Enum options are the possible values which an enum custom field can adopt.
- new_name string? - Conditional
- new_number_value int? - Conditional
- new_resource_subtype string? - Conditional
- new_section SectionCompact? - A section is a subdivision of a project that groups tasks together.
- new_text_value string? - Conditional
- num_hearts int? - Deprecated - please use likes instead Conditional. The number of users who have hearted this story.
- num_likes int? - Conditional. The number of users who have liked this story.
- old_approval_status string? - Conditional
- old_dates StoryResponseDates? - Conditional
- old_enum_value EnumOption? - Enum options are the possible values which an enum custom field can adopt.
- old_name string? - Conditional'
- old_number_value int? - Conditional
- old_resource_subtype string? - Conditional
- old_section SectionCompact? - A section is a subdivision of a project that groups tasks together.
- old_text_value string? - Conditional
- previews Preview[]? - Conditional. A collection of previews to be displayed in the story. Note: This property only exists for comment stories.
- project ProjectCompact? - A project represents a prioritized list of tasks in Asana or a board with columns of tasks represented as cards. It exists in a single workspace or organization and is accessible to a subset of users in that workspace or organization, depending on its permissions.
- 'source string? - The component of the Asana product the user used to trigger the story.
- story StoryCompact? - A story represents an activity associated with an object in the Asana system.
- tag TagCompact? - A tag is a label that can be attached to any task in Asana.
- target StoryresponseTarget? - The object this story is associated with. Currently may only be a task.
- task TaskCompact? - The task is the basic object around which many operations in Asana are centered.
asana: StoryResponseDates
Conditional
Fields
- due_at string? - Date and time representing activity occurance
- due_on string? - Date representing activity occurance
- start_on string? - Date representing activity start
asana: StoryresponseTarget
The object this story is associated with. Currently may only be a task.
Fields
- gid string? - The gid of the object this story is associated with.
- name string? - The name of the object this story is associated with.
asana: TagBase
Fields
- Fields Included from *TagCompact
- color string? - Color of the tag.
asana: TagCompact
A tag is a label that can be attached to any task in Asana.
Fields
- Fields Included from *AsanaResource
- name string? - Name of the tag. This is generally a short sentence fragment that fits on a line in the UI for maximum readability. However, it can be longer.
asana: TagRequest
Fields
- Fields Included from *TagBase
- followers string[]? - An array of strings identifying users. These can either be the string "me", an email, or the gid of a user.
- workspace string? - Gid of an object.
asana: TagResponse
Fields
- Fields Included from *TagBase
- followers UserCompact[]? - Array of users following this tag.
- permalink_url string? - A url that points directly to the object within Asana.
- workspace WorkspaceCompact? - The workspace in which the user task list is located.
asana: TagsBody
Fields
- data TagRequest? -
asana: TaskAddFollowersRequest
Fields
- followers string[] - An array of strings identifying users. These can either be the string "me", an email, or the gid of a user.
asana: TaskAddProjectRequest
Fields
- insert_after string? - A task in the project to insert the task after, or
null
to insert at the beginning of the list.
- insert_before string? - A task in the project to insert the task before, or
null
to insert at the end of the list.
- project string - The project to add the task to.
- section string? - A section in the project to insert the task into. The task will be inserted at the bottom of the section.
asana: TaskAddTagRequest
Fields
- tag string - The tag to add to the task.
asana: TaskBase
Fields
- Fields Included from *TaskCompact
- approval_status string? - Conditional Reflects the approval status of this task. This field is kept in sync with
completed
, meaningpending
translates to false whileapproved
,rejected
, andchanges_requested
translate to true. If you set completed to true, this field will be set toapproved
.
- assignee_status string? - Deprecated Scheduling status of this task for the user it is assigned to. This field can only be set if the assignee is non-null. Setting this field to "inbox" or "upcoming" inserts it at the top of the section, while the other options will insert at the bottom.
- completed boolean? - True if the task is currently marked complete, false if not.
- completed_at string? - The time at which this task was completed, or null if the task is incomplete.
- completed_by UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- created_at string? - The time at which this resource was created.
- dependencies AsanaResource[]? - Opt In. Array of resources referencing tasks that this task depends on. The objects contain only the gid of the dependency.
- dependents AsanaResource[]? - Opt In. Array of resources referencing tasks that depend on this task. The objects contain only the ID of the dependent.
- due_at string? - The UTC date and time on which this task is due, or null if the task has no due time. This takes an ISO 8601 date string in UTC and should not be used together with
due_on
.
- due_on string? - The localized date on which this task is due, or null if the task has no due date. This takes a date with
YYYY-MM-DD
format and should not be used together with due_at.
- 'external TaskbaseExternal? - OAuth Required. Conditional. This field is returned only if external values are set or included by using [Opt In] (/docs/input-output-options).
The external field allows you to store app-specific metadata on tasks, including a gid that can be used to retrieve tasks and a data blob that can store app-specific character strings. Note that you will need to authenticate with Oauth to access or modify this data. Once an external gid is set, you can use the notation
external:custom_gid
to reference your object anywhere in the API where you may use the original object gid. See the page on Custom External Data for more details.
- hearted boolean? - Deprecated - please use liked instead True if the task is hearted by the authorized user, false if not.
- hearts Like[]? - Deprecated - please use likes instead Array of likes for users who have hearted this task.
- is_rendered_as_separator boolean? - Opt In. In some contexts tasks can be rendered as a visual separator; for instance, subtasks can appear similar to sections without being true
section
objects. If atask
object is rendered this way in any context it will have the propertyis_rendered_as_separator
set totrue
.
- liked boolean? - True if the task is liked by the authorized user, false if not.
- likes Like[]? - Array of likes for users who have liked this task.
- memberships TaskbaseMemberships[]? - Create-only. Array of projects this task is associated with and the section it is in. At task creation time, this array can be used to add the task to specific sections. After task creation, these associations can be modified using the
addProject
andremoveProject
endpoints. Note that over time, more types of memberships may be added to this property.
- modified_at string? - The time at which this task was last modified. Note: This does not currently reflect any changes in associations such as projects or comments that may have been added or removed from the task.
- name string? - Name of the task. This is generally a short sentence fragment that fits on a line in the UI for maximum readability. However, it can be longer.
- notes string? - More detailed, free-form textual information associated with the task.
- num_hearts int? - Deprecated - please use likes instead The number of users who have hearted this task.
- num_likes int? - The number of users who have liked this task.
- resource_subtype string? - The subtype of this resource. Different subtypes retain many of the same fields and behavior, but may render differently in Asana or represent resources with different semantic meaning.
The resource_subtype
milestone
represent a single moment in time. This means tasks with this subtype cannot have a start_date.
- start_at string? - Date and time on which work begins for the task, or null if the task has no start time. This takes a UTC timestamp format.
Note:
due_at
must be present in the request when setting or unsetting thestart_at
parameter.
- start_on string? - The day on which work begins for the task , or null if the task has no start date. This takes a date with
YYYY-MM-DD
format. Note:due_on
ordue_at
must be present in the request when setting or unsetting thestart_on
parameter.
asana: TaskbaseExternal
OAuth Required. Conditional. This field is returned only if external values are set or included by using [Opt In] (/docs/input-output-options).
The external field allows you to store app-specific metadata on tasks, including a gid that can be used to retrieve tasks and a data blob that can store app-specific character strings. Note that you will need to authenticate with Oauth to access or modify this data. Once an external gid is set, you can use the notation external:custom_gid
to reference your object anywhere in the API where you may use the original object gid. See the page on Custom External Data for more details.
Fields
- data string? - The data of external field allows you to store app-specific metadata on tasks.
- gid string? - Globally unique identifier of the resource, as a string.
asana: TaskbaseMemberships
Fields
- project ProjectCompact? - A project represents a prioritized list of tasks in Asana or a board with columns of tasks represented as cards. It exists in a single workspace or organization and is accessible to a subset of users in that workspace or organization, depending on its permissions.
- section SectionCompact? - A section is a subdivision of a project that groups tasks together.
asana: TaskCompact
The task is the basic object around which many operations in Asana are centered.
Fields
- Fields Included from *AsanaResource
- name string? - The name of the task.
asana: TaskCountResponse
A response object returned from the task count endpoint.
Fields
- num_completed_milestones int? - The number of completed milestones in a project.
- num_completed_tasks int? - The number of completed tasks in a project.
- num_incomplete_milestones int? - The number of incomplete milestones in a project.
- num_incomplete_tasks int? - The number of incomplete tasks in a project.
- num_milestones int? - The number of milestones in a project.
- num_tasks int? - The number of tasks in a project.
asana: TaskDuplicateRequest
Fields
- include string? - The fields that will be duplicated to the new task.
- name string? - The name of the new task.
asana: TaskGidAdddependenciesBody
Fields
- data ModifyDependenciesRequest? -
asana: TaskGidAdddependentsBody
Fields
- data ModifyDependentsRequest? - A set of dependent tasks.
asana: TaskGidAddfollowersBody
Fields
- data TaskAddFollowersRequest? -
asana: TaskGidAddprojectBody
Fields
- data TaskAddProjectRequest? -
asana: TaskGidAddtagBody
Fields
- data TaskAddTagRequest? -
asana: TaskGidDuplicateBody
Fields
- data TaskDuplicateRequest? -
asana: TaskGidRemovedependenciesBody
Fields
- data ModifyDependenciesRequest? -
asana: TaskGidRemovedependentsBody
Fields
- data ModifyDependentsRequest? - A set of dependent tasks.
asana: TaskGidRemovefollowersBody
Fields
- data TaskRemoveFollowersRequest? -
asana: TaskGidRemoveprojectBody
Fields
- data TaskRemoveProjectRequest? -
asana: TaskGidRemovetagBody
Fields
- data TaskRemoveTagRequest? -
asana: TaskGidSetparentBody
Fields
- data TaskSetParentRequest? -
asana: TaskGidStoriesBody
Fields
- data StoryRequest? -
asana: TaskGidSubtasksBody
Fields
- data TaskRequest? -
asana: TaskRemoveFollowersRequest
Fields
- followers string[] - An array of strings identifying users. These can either be the string "me", an email, or the gid of a user.
asana: TaskRemoveProjectRequest
Fields
- project string - The project to remove the task from.
asana: TaskRemoveTagRequest
Fields
- tag string - The tag to remove from the task.
asana: TaskRequest
Fields
- Fields Included from *TaskBase
- approval_status string
- assignee_status string
- completed boolean
- completed_at string|()
- completed_by UserCompact|()
- created_at string
- dependencies AsanaResource[]
- dependents AsanaResource[]
- due_at string|()
- due_on string|()
- external TaskbaseExternal
- hearted boolean
- hearts Like[]
- html_notes string
- is_rendered_as_separator boolean
- liked boolean
- likes Like[]
- memberships TaskbaseMemberships[]
- modified_at string
- name string
- notes string
- num_hearts int
- num_likes int
- num_subtasks int
- resource_subtype string
- start_at string|()
- start_on string|()
- gid string
- resource_type string
- anydata...
- assignee string? - Gid of a user.
- custom_fields record {}? - An object where each key is a Custom Field gid and each value is an enum gid, string, or number.
- followers string[]? - Create-Only An array of strings identifying users. These can either be the string "me", an email, or the gid of a user. In order to change followers on an existing task use
addFollowers
andremoveFollowers
.
- parent string? - Gid of a task.
- projects string[]? - Create-Only Array of project gids. In order to change projects on an existing task use
addProject
andremoveProject
.
- tags string[]? - Create-Only Array of tag gids. In order to change tags on an existing task use
addTag
andremoveTag
.
- workspace string? - Gid of a workspace.
asana: TaskResponse
Fields
- Fields Included from *TaskBase
- approval_status string
- assignee_status string
- completed boolean
- completed_at string|()
- completed_by UserCompact|()
- created_at string
- dependencies AsanaResource[]
- dependents AsanaResource[]
- due_at string|()
- due_on string|()
- external TaskbaseExternal
- hearted boolean
- hearts Like[]
- html_notes string
- is_rendered_as_separator boolean
- liked boolean
- likes Like[]
- memberships TaskbaseMemberships[]
- modified_at string
- name string
- notes string
- num_hearts int
- num_likes int
- num_subtasks int
- resource_subtype string
- start_at string|()
- start_on string|()
- gid string
- resource_type string
- anydata...
- assignee UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- custom_fields CustomFieldResponse[]? - Array of custom field values applied to the task. These represent the custom field values recorded on this project for a particular custom field. For example, these custom field values will contain an
enum_value
property for custom fields of type enum, atext_value
property for custom fields of type text, and so on. Please note that thegid
returned on each custom field value is identical to thegid
of the custom field, which allows referencing the custom field metadata through the/custom_fields/custom_field-gid
endpoint.
- followers UserCompact[]? - Array of users following this task.
- parent TaskCompact? - The task is the basic object around which many operations in Asana are centered.
- permalink_url string? - A url that points directly to the object within Asana.
- projects ProjectCompact[]? - Create-only. Array of projects this task is associated with. At task creation time, this array can be used to add the task to many projects at once. After task creation, these associations can be modified using the addProject and removeProject endpoints.
- tags TagCompact[]? - Array of tags associated with this task. In order to change tags on an existing task use
addTag
andremoveTag
.
- workspace WorkspaceCompact? - The workspace in which the user task list is located.
asana: TasksBody
Fields
- data TaskRequest? -
asana: TaskSetParentRequest
Fields
- insert_after string? - A subtask of the parent to insert the task after, or
null
to insert at the beginning of the list.
- insert_before string? - A subtask of the parent to insert the task before, or
null
to insert at the end of the list.
- parent string - The new parent of the task, or
null
for no parent.
asana: TasksTaskGidBody
Fields
- data TaskRequest? -
asana: TeamAddUserRequest
A user identification object for specification with the addUser/removeUser endpoints.
Fields
- user string? - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
asana: TeamCompact
A team is used to group related projects and people together within an organization.
Fields
- Fields Included from *AsanaResource
- name string? - The name of the team.
asana: TeamGidAdduserBody
Fields
- data TeamAddUserRequest? - A user identification object for specification with the addUser/removeUser endpoints.
asana: TeamGidProjectsBody
Fields
- data ProjectRequest? -
asana: TeamGidRemoveuserBody
Fields
- data TeamRemoveUserRequest? - A user identification object for specification with the addUser/removeUser endpoints.
asana: TeamMembershipCompact
Fields
- Fields Included from *AsanaResource
- is_guest boolean? - Describes if the user is a guest in the team.
- team TeamCompact? - A team is used to group related projects and people together within an organization.
- user UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
asana: TeamRemoveUserRequest
A user identification object for specification with the addUser/removeUser endpoints.
Fields
- user string? - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
asana: TeamRequest
Fields
- description string? - The description of the team.
- html_description string? - The description of the team with formatting as HTML.
- organization string? - The organization/workspace the team belongs to.
asana: TeamResponse
Fields
- organization WorkspaceCompact? - The workspace in which the user task list is located.
- permalink_url string? - A url that points directly to the object within Asana.
asana: TeamsBody
Fields
- data TeamRequest? -
asana: UserCompact
The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
Fields
- Fields Included from *AsanaResource
- name string? - Read-only except when same user as requester. The user’s name.
asana: UserResponse
Fields
- Fields Included from *UserCompact
- email string? - The user's email address.
- photo UserresponsePhoto? - A map of the user’s profile photo in various sizes, or null if no photo is set. Sizes provided are 21, 27, 36, 60, and 128. Images are in PNG format.
- workspaces WorkspaceCompact[]? - Workspaces and organizations this user may access. Note: The API will only return workspaces and organizations that also contain the authenticated user.
asana: UserresponsePhoto
A map of the user’s profile photo in various sizes, or null if no photo is set. Sizes provided are 21, 27, 36, 60, and 128. Images are in PNG format.
Fields
- image_128x128 string? - User profile image of size 128x128
- image_21x21 string? - User profile image of size 21x21
- image_27x27 string? - User profile image of size 27x27
- image_36x36 string? - User profile image of size 36x36
- image_60x60 string? - User profile image of size 60x60
asana: UserTaskListCompact
Fields
- Fields Included from *AsanaResource
- name string? - The name of the user task list.
- owner UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- workspace WorkspaceCompact? - The workspace in which the user task list is located.
asana: WebhookCompact
Fields
- Fields Included from *AsanaResource
- active boolean? - If true, the webhook will send events - if false it is considered inactive and will not generate events.
- 'resource AsanaNamedResource? - A generic Asana Resource, containing a globally unique identifier.
- target string? - The URL to receive the HTTP POST.
asana: WebhookFilter
A WebhookFilter can be passed on creation of a webhook in order to filter the types of actions that trigger delivery of an Event
Fields
- fields string[]? - Conditional. A whitelist of fields for events which will pass the filter when the resource is changed. These can be any combination of the fields on the resources themselves. This field is only valid for
action
of type 'changed'
- resource_subtype string? - The resource subtype of the resource that the filter applies to. This should be set to the same value as is returned on the
resource_subtype
field on the resources themselves.
- resource_type string? - The type of the resource which created the event when modified; for example, to filter to changes on regular tasks this field should be set to
task
.
asana: WebhookRequest
Fields
- filters WebhookFilter[]? - An array of WebhookFilter objects to specify a whitelist of filters to apply to events from this webhook. If a webhook event passes any of the filters the event will be delivered; otherwise no event will be sent to the receiving server.
- 'resource string - A resource ID to subscribe to. Many Asana resources are valid to create webhooks on, but higher-level resources require filters.
- target string - The URL to receive the HTTP POST. The full URL will be used to deliver events from this webhook (including parameters) which allows encoding of application-specific state when the webhook is created.
asana: WebhookResponse
Fields
- Fields Included from *WebhookCompact
- active boolean
- resource AsanaNamedResource
- target string
- gid string
- resource_type string
- anydata...
- created_at string? - The time at which this resource was created.
- filters WebhookFilter[]? - Whitelist of filters to apply to events from this webhook. If a webhook event passes any of the filters the event will be delivered; otherwise no event will be sent to the receiving server.
- last_failure_at string? - The timestamp when the webhook last received an error when sending an event to the target.
- last_failure_content string? - The contents of the last error response sent to the webhook when attempting to deliver events to the target.
- last_success_at string? - The timestamp when the webhook last successfully sent an event to the target.
asana: WebhooksBody
Fields
- data WebhookRequest? -
asana: WorkspaceAddUserRequest
A user identification object for specification with the addUser/removeUser endpoints.
Fields
- user string? - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
asana: WorkspaceCompact
The workspace in which the user task list is located.
Fields
- Fields Included from *AsanaResource
- name string? - The name of the workspace.
asana: WorkspaceGidAdduserBody
Fields
- data WorkspaceAddUserRequest? - A user identification object for specification with the addUser/removeUser endpoints.
asana: WorkspaceGidProjectsBody
Fields
- data ProjectRequest? -
asana: WorkspaceGidRemoveuserBody
Fields
- data WorkspaceRemoveUserRequest? - A user identification object for specification with the addUser/removeUser endpoints.
asana: WorkspaceGidTagsBody
Fields
- data TagResponse? -
asana: WorkspaceMembershipCompact
Fields
- Fields Included from *AsanaResource
- user UserCompact? - The owner of the user task list, i.e. the person whose My Tasks is represented by this resource.
- workspace WorkspaceCompact? - The workspace in which the user task list is located.
asana: WorkspaceMembershipResponse
Fields
- is_active boolean? - Reflects if this user still a member of the workspace.
- is_admin boolean? - Reflects if this user is an admin of the workspace.
- is_guest boolean? - Reflects if this user is a guest of the workspace.
- user_task_list UserTaskListResponse? -
asana: WorkspaceRemoveUserRequest
A user identification object for specification with the addUser/removeUser endpoints.
Fields
- user string? - A string identifying a user. This can either be the string "me", an email, or the gid of a user.
asana: WorkspaceResponse
Fields
- email_domains string[]? - The email domains that are associated with this workspace.
- is_organization boolean? - Whether the workspace is an organization.
asana: WorkspacesWorkspaceGidBody
Fields
- data WorkspaceRequest? -
Import
import ballerinax/asana;
Metadata
Released date: over 1 year ago
Version: 1.6.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1700
Current verison: 277
Weekly downloads
Keywords
Productivity/Project Management
Cost/Freemium
Contributors