adobe.analytics
Module adobe.analytics
API
Definitions
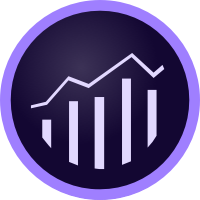
ballerinax/adobe.analytics Ballerina library
Overview
This is a generated connector for Adobe Analytics API OpenAPI specification.
The Adobe Analytics 2.0 APIs allow you to directly call Adobe's servers to perform almost any action that you can perform in the user interface. You can create reports to explore, get insights, or answer important questions about your data. You can also manage components of Adobe Analytics, such as the creation of segments or calculated metrics.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Adobe Developer account.
- Obtain tokens by following this guide.
Quickstart
To use the Adobe Analytics connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/adobe.analytics
module into the Ballerina project.
import ballerinax/adobe.analytics;
Step 2 - Create a new connector instance
You can now make the connection configuration using the <ACCESS_TOKEN>.
analytics:ClientConfig clientConfig = { auth : { token: `<ACCESS_TOKEN>` } }; analytics:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to list all calculated metric functions the connector.
public function main() { analytics:CalcMetricFunction[]|error result = baseClient->getCalculatedMetricFunctions(); if (result is analytics:CalcMetricFunction[]) { log:printInfo(result.toString()); } else { log:printInfo(result.toString()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
adobe.analytics: Client
The Adobe Analytics 2.0 APIs allow you to directly call Adobe's servers to perform almost any action that you can perform in the user interface. You can create reports to explore, get insights, or answer important questions about your data. You can also manage components of Adobe Analytics, such as the creation of segments or calculated metrics.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Adobe Developer account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://analytics.adobe.io/" - URL of the target service
findCalculatedMetrics
function findCalculatedMetrics(string? rsids, int? ownerId, string? filterByIds, string? toBeUsedInRsid, string locale, string? name, string? tagNames, boolean? favorite, boolean? approved, int 'limit, int page, string sortDirection, string sortProperty, string[]? expansion, string[]? includeType) returns AnalyticsCalculatedMetric[]|error
Retrieve many calculated metrics
Parameters
- rsids string? (default ()) - Filter list to only include calculated metrics tied to specified RSID list (comma-delimited)
- ownerId int? (default ()) - Filter list to only include calculated metrics owned by the specified loginId
- filterByIds string? (default ()) - Filter list to only include calculated metrics in the specified list (comma-delimited list of IDs) (this is the same as calculatedMetricFilter, and is overwritten by calculatedMetricFilter
- toBeUsedInRsid string? (default ()) - The report suite where the calculated metric intended to be used. This report suite will be used to determine things like compatibility and permissions. If it is not specified then the permissions will be calculated based on the union of all metrics authorized in all groups the user belongs to. If the compatibility expansion is specified and toBeUsedInRsid is not then the compatibility returned is based off the compatibility from the last time the calculated metric was saved.
- locale string (default "en_US") - Locale
- name string? (default ()) - Filter list to only include calculated metrics that contains the Name
- tagNames string? (default ()) - Filter list to only include calculated metrics that contains one of the tags
- favorite boolean? (default ()) - Filter list to only include calculated metrics that are favorites
- approved boolean? (default ()) - Filter list to only include calculated metrics that are approved
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
- sortDirection string (default "ASC") - Sort direction (ASC or DESC)
- sortProperty string (default "id") - Property to sort by (name, modified_date, id is currently allowed)
- expansion string[]? (default ()) - Comma-delimited list of additional calculated metric metadata fields to include on response.
- includeType string[]? (default ()) - Include additional calculated metrics not owned by user. The "all" option takes precedence over "shared"
Return Type
- AnalyticsCalculatedMetric[]|error - successful operation
createCalculatedMetric
function createCalculatedMetric(AnalyticsCalculatedMetric payload, string locale, string[]? expansion) returns AnalyticsCalculatedMetric|error
Create a new Calculated Metric
Parameters
- payload AnalyticsCalculatedMetric - JSON-formatted Object containing key/value pairs for calculated metric creation.
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional calculated metric metadata fields to include on response.
Return Type
- AnalyticsCalculatedMetric|error - successful operation
getCalculatedMetricFunctions
function getCalculatedMetricFunctions(string locale) returns CalcMetricFunction[]|error
Retrieve calculated metric functions
Parameters
- locale string (default "en_US") - Locale
Return Type
- CalcMetricFunction[]|error - successful operation
getCalculatedMetricFunction
function getCalculatedMetricFunction(string id, string locale) returns CalcMetricFunction|error
Retrieve a calculated metric function by id
Return Type
- CalcMetricFunction|error - successful operation
validateCalculatedMetric
function validateCalculatedMetric(AnalyticsCalculatedMetric payload, string locale, boolean migrating) returns AnalyticsCalculatedMetric|error
Validate a calculated metric definition
Parameters
- payload AnalyticsCalculatedMetric - JSON-formatted Object containing key/value pairs for calculated metric validation.
- locale string (default "en_US") - Locale
- migrating boolean (default false) - Include migration functions in validation
Return Type
- AnalyticsCalculatedMetric|error - successful operation
findOneCalculatedMetric
function findOneCalculatedMetric(string id, string locale, string[]? expansion) returns AnalyticsCalculatedMetric|error
Retrieve a single calculated metric by id
Parameters
- id string - The calculated metric ID to retrieve
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional calculated metric metadata fields to include on response.
Return Type
- AnalyticsCalculatedMetric|error - successful operation
updateCalculatedMetric
function updateCalculatedMetric(string id, AnalyticsCalculatedMetric payload, string locale, string[]? expansion) returns AnalyticsCalculatedMetric|error
Update an existing calculated metric
Parameters
- id string - Calculated Metric ID to be updated
- payload AnalyticsCalculatedMetric - JSON-formatted Object containing key/value pairs to be updated.
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional calculated metric metadata fields to include on response.
Return Type
- AnalyticsCalculatedMetric|error - successful operation
deleteCalculatedMetric
function deleteCalculatedMetric(string id, string locale) returns DeleteResponse|error
Deletion of Calculated Metrics by Id
Parameters
- id string - The calculated metric ID to be deleted
- locale string (default "en_US") - Locale
Return Type
- DeleteResponse|error - successful operation
listReportSuites
function listReportSuites(string? rsids, string? rsidContains, int 'limit, int page, string[]? expansion) returns SuiteCollectionItem|error
Retrieves report suites that match the given filters.
Parameters
- rsids string? (default ()) - Filter list to only include suites in this RSID list (comma-delimited)
- rsidContains string? (default ()) - Filter list to only include suites whose rsid contains rsidContains.
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
- expansion string[]? (default ()) - Comma-delimited list of additional metadata fields to include on response.
Return Type
- SuiteCollectionItem|error - successful operation
getReportSuite
function getReportSuite(string rsid, string[]? expansion) returns SuiteCollectionItem|error
Retrieves report suite by id
Parameters
- rsid string - The rsid of the suite to return
- expansion string[]? (default ()) - Comma-delimited list of additional metadata fields to include on response.
Return Type
- SuiteCollectionItem|error - successful operation
searchComponentTags
function searchComponentTags(ComponentSearch payload, int 'limit, int page) returns TaggedComponent|error
search for tags for several components at once
Parameters
- payload ComponentSearch - items to search for
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
Return Type
- TaggedComponent|error - successful operation
findAllTagsForCompany
Returns a list of tags for the current user's company
Parameters
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
saveTagListForCompany
Saves the given tag(s) for the current user's company
Parameters
- payload Tag[] - JSON-formatted array of Tag objects containing key-value pairs
deleteTagItemsFromComponents
function deleteTagItemsFromComponents(string componentIds, string componentType) returns record {}[]|error
Disassociates all tags from the given components
Parameters
- componentIds string - Comma-separated list of componentIds to operate on.
- componentType string - The component type to operate on.
Return Type
- record {}[]|error - successful operation
getTagListByComponentIdAndComponentType
function getTagListByComponentIdAndComponentType(string componentId, string componentType) returns Tag[]|error
Retrieves a tags for a given component by componentId and componentType
Parameters
- componentId string - The componentId to operate on. Currently this is just the segmentId.
- componentType string - The component type to operate on.
saveTagComponentList
function saveTagComponentList(TaggedComponent[] payload) returns TaggedComponent[]|error
Tag a component with one or many tags at once. WARNING: Authoritative; deletes/overwrites all pre-existing associations
Parameters
- payload TaggedComponent[] - JSON-formatted object containing key-value pairs that conform to the schema
Return Type
- TaggedComponent[]|error - successful operation
getTagById
Retrieves an tag by its id
Parameters
- id int - Tag ID to be retrieved
deleteTag
Removes the tagId and all associations from that tag to any components
Parameters
- id int - The tagId to be deleted
Return Type
- record {}[]|error - successful operation
getComponentsByTagName
Retrieves component ids associated with the given tag names
Parameters
- tagNames string - Comma separated list of tag names.
- componentType string - The component type to operate on.
searchComponentShares
function searchComponentShares(ComponentSearch payload, int 'limit, int page) returns SharedComponent|error
search for shares for several components at once
Parameters
- payload ComponentSearch - items to search for
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
Return Type
- SharedComponent|error - successful operation
getComponentsSharedToMe
get components shared with the current user by type
Parameters
- componentType string - Component type to get shared ids for
findAllSharesForCompany
Returns a list of shares for the current user's company
Parameters
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
updateSharesForComponents
function updateSharesForComponents(byte[] payload) returns SharedComponent|error
Share components with users. WARNING: Authoritative; deletes/overwrites all pre-existing shares for the given components
Parameters
- payload byte[] - JSON-formatted array of Share objects containing key-value pairs
Return Type
- SharedComponent|error - successful operation
saveShareMyCompany
Saves the given share for the current user's company
Parameters
- payload Share - JSON-formatted array of Share objects containing key-value pairs
getShareById
Retrieves an share by its id
Parameters
- id int - Share ID to be retrieved
deleteShare
function deleteShare(int id) returns InlineResponse200|error
Removes the shareId and all associations from that share
Parameters
- id int - The shareId to be deleted
Return Type
- InlineResponse200|error - successful operation
getDateRangesForUser
function getDateRangesForUser(string locale, string? filterByIds, int 'limit, int page, string[]? expansion, string[]? includeType) returns AnalyticsDateRange|error
Returns a list of dateranges for the user
Parameters
- locale string (default "en_US") - Locale
- filterByIds string? (default ()) - Filter list to only include date ranges in the specified list (comma-delimited list of IDs)
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
- expansion string[]? (default ()) - Comma-delimited list of additional date range metadata fields to include on response.
- includeType string[]? (default ()) - Include additional date ranges not owned by user. The "all" option takes precedence over "shared"
Return Type
- AnalyticsDateRange|error - successful operation
createDateRange
function createDateRange(byte[] payload, string locale, string[]? expansion) returns AnalyticsDateRange|error
Creates configuration for a DateRange.
Parameters
- payload byte[] - JSON-formatted array of Date Range objects containing key-value pairs
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional date range metadata fields to include on response.
Return Type
- AnalyticsDateRange|error - successful operation
getDateRange
function getDateRange(string dateRangeId, string locale, string[]? expansion) returns AnalyticsDateRange|error
Retrieves configuration for a DateRange.
Parameters
- dateRangeId string - The DateRange id for which to retrieve information
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional date range metadata fields to include on response.
Return Type
- AnalyticsDateRange|error - successful operation
updateDateRange
function updateDateRange(string dateRangeId, byte[] payload, string locale, string[]? expansion) returns AnalyticsDateRange|error
Updates configuration for a DateRange.
Parameters
- dateRangeId string - The DateRange id for which to retrieve information
- payload byte[] - JSON-formatted array of Date Range objects containing key-value pairs
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional date range metadata fields to include on response.
Return Type
- AnalyticsDateRange|error - successful operation
deleteDateRange
function deleteDateRange(string dateRangeId) returns InlineResponse2001|error
Deletes a DateRange.
Parameters
- dateRangeId string - The id of the date range to delete
Return Type
- InlineResponse2001|error - successful operation
getDimensionsForReportSuite
function getDimensionsForReportSuite(string rsid, string locale, boolean? segmentable, boolean? reportable, boolean classifiable, string[]? expansion) returns AnalyticsDimension[]|error
Returns a list of dimensions for a given report suite.
Parameters
- rsid string - A Report Suite ID
- locale string (default "en_US") - Locale
- segmentable boolean? (default ()) - Only include dimensions that are valid within a segment.
- reportable boolean? (default ()) - Only include dimensions that are valid within a report.
- classifiable boolean (default false) - Only include classifiable dimensions.
- expansion string[]? (default ()) - Add extra metadata to items (comma-delimited list)
Return Type
- AnalyticsDimension[]|error - successful operation
getDimensionForReportSuite
function getDimensionForReportSuite(string dimensionId, string rsid, string locale, string[]? expansion) returns AnalyticsDimension|error
Returns a dimension for the given report suite
Parameters
- dimensionId string - The dimension ID. For example a valid id is a value like 'evar1'
- rsid string - The report suite ID.
- locale string (default "en_US") - The locale to use for returning system named dimensions.
- expansion string[]? (default ()) - Add extra metadata to items (comma-delimited list)
Return Type
- AnalyticsDimension|error - successful operation
getMetricsForReportSuite
function getMetricsForReportSuite(string rsid, string locale, boolean segmentable, string[]? expansion) returns AnalyticsMetric|error
Returns a list of metrics for the given report suite
Parameters
- rsid string - ID of desired report suite ie. myrsid
- locale string (default "en_US") - Locale that system named metrics should be returned in
- segmentable boolean (default false) - Filter the metrics by if they are valid in a segment.
- expansion string[]? (default ()) - Add extra metadata to items (comma-delimited list)
Return Type
- AnalyticsMetric|error - successful operation
getMetricForReportSuite
function getMetricForReportSuite(string id, string rsid, string locale, string[]? expansion) returns AnalyticsMetric|error
Returns a metric for the given report suite
Parameters
- id string - The id of the metric for which to retrieve info. Note ids are values like pageviews, not metrics/pageviews
- rsid string - ID of desired report suite ie. myrsid
- locale string (default "en_US") - Locale that system named metrics should be returned in
- expansion string[]? (default ()) - Add extra metadata to items (comma-delimited list)
Return Type
- AnalyticsMetric|error - successful operation
getProjectConfiguration
function getProjectConfiguration(string projectId, string[]? expansion, string locale) returns AnalyticsProject|error
Retrieves configuration for a Project.
Parameters
- projectId string - The Project id for which to retrieve information
- expansion string[]? (default ()) - Comma-delimited list of additional project metadata fields to include on response.
- locale string (default "en_US") - Locale
Return Type
- AnalyticsProject|error - successful operation
updateProjectConfiguration
function updateProjectConfiguration(string projectId, byte[] payload, string[]? expansion, string locale) returns AnalyticsProject|error
Updates configuration for a project.
Parameters
- projectId string - The Project id for which to retrieve information
- payload byte[] - JSON-formatted Object containing project keys/value pairs to be updated.
- expansion string[]? (default ()) - Comma-delimited list of additional project metadata fields to include on response.
- locale string (default "en_US") - Locale
Return Type
- AnalyticsProject|error - successful operation
deleteProject
function deleteProject(string projectId, string locale) returns DeleteResponse|error
deletes a project.
Parameters
- projectId string - The Project id for which to retrieve information
- locale string (default "en_US") - Locale
Return Type
- DeleteResponse|error - successful operation
validateProjectDefinition
function validateProjectDefinition(ProjectsValidateBody payload, string locale) returns ProjectCompatibility|error
Validates a Project definition
Parameters
- payload ProjectsValidateBody - JSON-formatted Object containing key/value pairs for Project validation.
- locale string (default "en_US") - Locale
Return Type
- ProjectCompatibility|error - successful operation
getProjectsOfUser
function getProjectsOfUser(string[]? includeType, string[]? expansion, string? filterByIds, string locale, string pagination, int? ownerId, int 'limit, int page) returns AnalyticsProject[]|error
Returns a list of projects for the user
Parameters
- includeType string[]? (default ()) - Include additional projects not owned by user. The "all" option takes precedence over "shared". If neither guided, or project is included, both types are returned
- expansion string[]? (default ()) - Comma-delimited list of additional project metadata fields to include on response.
- filterByIds string? (default ()) - Filter list to only include projects in the specified list (comma-delimited list of IDs)
- locale string (default "en_US") - Locale
- pagination string (default "false") - return paginated results
- ownerId int? (default ()) - Filter list to only include projects owned by the specified loginId
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
Return Type
- AnalyticsProject[]|error - successful operation
createProject
function createProject(byte[] payload, string[]? expansion, string locale) returns AnalyticsProject|error
Creates a single project.
Parameters
- payload byte[] - JSON-formatted Object containing project keys/value pairs to be updated.
- expansion string[]? (default ()) - Comma-delimited list of additional project metadata fields to include on response.
- locale string (default "en_US") - Locale
Return Type
- AnalyticsProject|error - successful operation
runReport
function runReport(RankedRequest payload) returns RankedReportData|error
Runs a report for the request in the post body
Parameters
- payload RankedRequest - Ranked report request
Return Type
- RankedReportData|error - Successful operation
runTopItemReport
function runTopItemReport(string rsid, string dimension, string locale, string? dateRange, string? searchClause, string? startDate, string? endDate, string? searchAnd, string? searchOr, string? searchNot, string? searchPhrase, boolean lookupNoneValues, int 'limit, int page) returns UnhashReportData|error
Runs a top items report for the request in the post body
Parameters
- rsid string - ID of desired report suite ie. myrsid
- dimension string - Dimension to run the report against. Example: 'variables/page'
- locale string (default "en_US") - Locale that system named metrics should be returned in
- dateRange string? (default ()) - Format: YYYY-MM-DD/YYYY-MM-DD
- searchClause string? (default ()) - General search string; wrap with single quotes. Example: 'PageABC'
- startDate string? (default ()) - Format: YYYY-MM-DD
- endDate string? (default ()) - Format: YYYY-MM-DD
- searchAnd string? (default ()) - Search terms that will be AND-ed together. Space delimited.
- searchOr string? (default ()) - Search terms that will be OR-ed together. Space delimited.
- searchNot string? (default ()) - Search terms that will be treated as NOT including. Space delimited.
- searchPhrase string? (default ()) - A full search phrase that will be searched for.
- lookupNoneValues boolean (default true) - Controls None values to be included
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
Return Type
- UnhashReportData|error - Successful operation
getAllSegments
function getAllSegments(string? rsids, string? segmentFilter, string locale, string? name, string? tagNames, string filterByPublishedSegments, int 'limit, int page, string sortDirection, string sortProperty, string[]? expansion, string[]? includeType) returns AnalyticsSegmentResponseItem|error
Retrieve All Segments
Parameters
- rsids string? (default ()) - Filter list to only include segments tied to specified RSID list (comma-delimited)
- segmentFilter string? (default ()) - Filter list to only include segments in the specified list (comma-delimited list of IDs)
- locale string (default "en_US") - Locale
- name string? (default ()) - Filter list to only include segments that contains the Name
- tagNames string? (default ()) - Filter list to only include segments that contains one of the tags
- filterByPublishedSegments string (default "all") - Filter list to only include segments where the published field is set to one of the allowable values (all, true, false).
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
- sortDirection string (default "ASC") - Sort direction (ASC or DESC
- sortProperty string (default "id") - Property to sort by (name, modified_date, id is currently allowed)
- expansion string[]? (default ()) - Comma-delimited list of additional segment metadata fields to include on response.
- includeType string[]? (default ()) - Include additional segments not owned by user. The "all" option takes precedence over "shared"
Return Type
- AnalyticsSegmentResponseItem|error - successful operation
createSegment
function createSegment(AnalyticsSegment payload, string locale, string[]? expansion) returns AnalyticsSegmentResponseItem|error
Creates Segment
Parameters
- payload AnalyticsSegment - JSON-formatted Object containing key/value pairs for segment creation.
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional segment metadata fields to include on response.
Return Type
- AnalyticsSegmentResponseItem|error - successful operation
validateSegment
function validateSegment(string rsid, string payload) returns SegmentCompatibility|error
Validate a Segment
Return Type
- SegmentCompatibility|error - successful operation
getSegment
function getSegment(string id, string locale, string[]? expansion) returns AnalyticsSegmentResponseItem|error
Get a Single Segment
Parameters
- id string - The segment ID to retrieve
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional segment metadata fields to include on response.
Return Type
- AnalyticsSegmentResponseItem|error - successful operation
updateSegment
function updateSegment(string id, json payload, string locale, string[]? expansion) returns AnalyticsSegmentResponseItem|error
Update a Segment
Parameters
- id string - Segment ID to be updated
- payload json - JSON-formatted Object containing key/value pairs to be updated.
- locale string (default "en_US") - Locale
- expansion string[]? (default ()) - Comma-delimited list of additional segment metadata fields to include on response.
Return Type
- AnalyticsSegmentResponseItem|error - successful operation
deleteSegment
Delete Segment
listAllUsersForCompany
function listAllUsersForCompany(int 'limit, int page) returns AnalyticsUser[]|error
Returns a list of users for the current user's login company
Parameters
- 'limit int (default 10) - Number of results per page
- page int (default 0) - Page number (base 0 - first page is "0")
Return Type
- AnalyticsUser[]|error - successful operation
getCurrentUser
function getCurrentUser() returns AnalyticsUser|error
Get the current user
Return Type
- AnalyticsUser|error - successful operation
getUsageAccessLogs
function getUsageAccessLogs(string startDate, string endDate, string? login, string? ip, string? rsid, string? eventType, string? event, int 'limit, int page) returns ResponsePageUsageLogDto|error
Retrieves usage and access logs for the search criteria provided.
Parameters
- startDate string - Start date for the maximum of a 3 month period.
- endDate string - End date for the maximum of a 3 month period.
- login string? (default ()) - The login value of the user you want to filter logs by.
- ip string? (default ()) - The IP address you want to filter logs by.
- rsid string? (default ()) - The report suite ID you want to filter logs by.
- eventType string? (default ()) - The numeric id for the event type you want to filter logs by.
- event string? (default ()) - The event description you want to filter logs by. No wildcards permitted.
- 'limit int (default 10) - Number of results per page.
- page int (default 0) - Page number (base 0 - first page is "0").
Return Type
- ResponsePageUsageLogDto|error - Successful
Records
adobe.analytics: AnalyticsCalculatedMetric
Fields
- id string? - System generated id
- name string? -
- description string? -
- rsid string? - The report suite id for which the component was created/updated
- reportSuiteName string? - The report suite name for which the component was created/updated
- owner Owner? - Owner
- polarity string? - Set metric polarity, which indicates whether it's good or bad if a given metric goes up. Default=positive
- precision int? - Number of decimal places to include in calculated metric result
- 'type string? -
- definition CalculatedMetricDef -
- categories string[]? -
- tags Tag[]? -
- siteTitle string? -
- modified string? -
- created string? - Calculated metric creation date
adobe.analytics: AnalyticsDateRange
Fields
- id string? - System generated id
- name string? - Name
- description string? - Description
- rsid string? - The report suite id for which the component was created/updated
- reportSuiteName string? - The report suite name for which the component was created/updated
- owner Owner? - Owner
- definition record {}? - Definition
- tags Tag[]? - Tags
- siteTitle string? - Site Title
- modified string? - Modified date time
- created string? - Created date time
adobe.analytics: AnalyticsDateRangeDefinition
Fields
- 'start RollingDateFunction[]? -
- end RollingDateFunction[]? -
- calendarType CalendarType? -
- 'version string? -
adobe.analytics: AnalyticsDimension
Fields
- id string? -
- title string? -
- name string? -
- 'type string? -
- category string? -
- categories string[]? -
- support string[]? -
- pathable boolean? -
- parent string? -
- extraTitleInfo string? -
- segmentable boolean? -
- reportable string[]? -
- description string? -
- allowedForReporting boolean? -
- noneSettings NoneSettings? -
- tags Tag[]? -
adobe.analytics: AnalyticsMetric
Fields
- id string? -
- title string? -
- name string? -
- 'type string? -
- extraTitleInfo string? -
- category string? -
- categories string[]? -
- support string[]? -
- allocation boolean? -
- precision int? -
- calculated boolean? -
- segmentable boolean? -
- description string? -
- polarity string? -
- helpLink string? -
- allowedForReporting boolean? -
- tags Tag[]? -
adobe.analytics: AnalyticsProject
Fields
- id string? - System generated id
- name string? - Name
- description string? - Description
- rsid string? - The report suite id for which the component was created/updated
- reportSuiteName string? - The report suite name for which the component was created/updated
- owner Owner? - Owner
- 'type string? - Type
- definition JsonNode? -
- externalReferences record {}? -
- accessLevel string? - Access Level
- versionNotes string? -
- tags record {}[]? -
- shares record {}[]? -
- modified string? -
- created string? -
adobe.analytics: AnalyticsSegment
Fields
- name string? - A name for the segment.
- description string? - A description of the segment.
- rsid string? - The report suite id.
- reportSuiteName string? - The friendly name for the report suite id.
- owner Owner? - Owner
- definition AnalyticsSegmentDefinition? - Analytics segment definition
- compatibility SegmentCompatibility? - Segment compatibility.
- definitionLastModified string? -
- categories string[]? -
- siteTitle string? - A name for the report suite. This is deprecated and should use the report suite name instead.
- tags Tag[]? - All existing tags associated with the segment.
- modified string? -
- created string? -
adobe.analytics: AnalyticsSegmentDefinition
Analytics segment definition
Fields
- container AnalyticssegmentdefinitionContainer? - Analytics segment definition container
- func string? - Func
- 'version int[]? - Version
adobe.analytics: AnalyticssegmentdefinitionContainer
Analytics segment definition container
Fields
- context string? - Context
- func string? - Func
- pred AnalyticssegmentdefinitionContainerPred? - Pred
adobe.analytics: AnalyticssegmentdefinitionContainerPred
Pred
Fields
- val AnalyticssegmentdefinitionContainerPredVal? - Val
- str string? - Str
- func string? - Func
adobe.analytics: AnalyticssegmentdefinitionContainerPredVal
Val
Fields
- description string? - Description
- name string? - Name
- func string? - Func
adobe.analytics: AnalyticsSegmentResponseItem
Fields
- id string? - Id of the segment.
- name string? - A name for the segment.
- description string? - A description of the segment.
- rsid string? - The report suite id.
- reportSuiteName string? - The friendly name for the report suite id.
- owner Owner? - Owner
- definition AnalyticsSegmentDefinition? - Analytics segment definition
- compatibility SegmentCompatibility? - Segment compatibility.
- definitionLastModified string? -
- categories string[]? -
- siteTitle string? - A name for the report suite. This is deprecated and should use the report suite name instead.
- tags Tag[]? - All existing tags associated with the segment.
- modified string? - Modified date and time.
- created string? - Created date and time
adobe.analytics: AnalyticsUser
Fields
- companyid int? -
- loginId int? -
- login string? -
- changePassword boolean? -
- createDate string? -
- disabled boolean? -
- email string? -
- firstName string? -
- fullName string? -
- imsUserId string? -
- lastName string? -
- lastAccess string? -
- phoneNumber string? -
- tempLoginEnd string? -
- title string? -
adobe.analytics: AnalyticsVirtualReportSuite
Fields
- id string? - System generated virtual report suite id
- name string? -
- description string? -
- rsid string? - The report suite id for which the component was created/updated
- reportSuiteName string? - The report suite name for which the component was created/updated
- owner Owner? - Owner
- parentRsid string? - Parent report suite id for virtual report suite
- parentRsidName string? - Parent report suite name
- timezone int? -
- segmentList string[]? - List of segments applied to this virtual report suite
- currency string? -
- calendarType CalendarType? -
- timezoneZoneInfo string? - Suite friendly timezone name
- tags Tag[]? -
- siteTitle string? -
- modified string? -
- created string? -
adobe.analytics: CalcMetricCompatibility
Fields
- valid boolean? -
- message string? -
- identityMetrics IdentityMetric[]? -
- identityDimensions string[]? -
- segments string[]? -
- functions string[]? -
- validator_version string? -
- supported_products string[]? -
- supported_schema string[]? -
adobe.analytics: CalcMetricFunction
Fields
- id string? - Calculated Metric Function ID
- category string? - Calculated Metric Function category
- persistable boolean? - If a Calculated Metric Function is persistable
- name string? - Calculated Metric Function name
- namespace string? - Calculated Metric Function namespace
- description string? - Calculated Metric Function description
- exampleKey string? - Calculated Metric Function example key
- example string? - Calculated Metric Function example
- definition CalcMetricFunctionDef? -
adobe.analytics: CalcMetricFunctionDef
Fields
- func string? -
- parameters CalcMetricFunctionParameter[]? -
- formula record {}? -
- 'version int[]? -
adobe.analytics: CalcMetricFunctionParameter
Fields
- func string? -
- name string? -
- 'type string? -
- friendlyNameKey string? -
- descKey string? -
- friendlyName string? -
- description string? -
- 'default\-value record {}? -
adobe.analytics: CalculatedMetricDef
adobe.analytics: CalculatedMetricErrorStatus
Fields
- errorCode string? -
- errorDescription string? -
- errorId string? -
- errorDetails record {}? -
- rootCauseService string? -
adobe.analytics: CalendarType
Fields
- rsid string? -
- 'type string? -
- anchorDate string? -
adobe.analytics: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
adobe.analytics: Column
Fields
- 'type string? -
- id string? -
- title string? -
- segmentIds string[]? -
adobe.analytics: ComponentSearch
Fields
- componentType string? -
- componentIds string[]? -
adobe.analytics: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
adobe.analytics: DeleteResponse
Fields
- result string? -
- message string? -
adobe.analytics: IdentityMetric
Fields
- identity string? -
- dimensionView string? -
- allocationModel string? -
adobe.analytics: InlineResponse200
Fields
- shareId int? -
- success InlineResponse200Success? -
adobe.analytics: InlineResponse2001
Fields
- result string? -
adobe.analytics: InlineResponse200Success
Fields
- success string? -
adobe.analytics: JsonNode
adobe.analytics: Locale
Fields
- language string? -
- script string? -
- country string? -
- variant string? -
- extensionKeys string[]? -
- unicodeLocaleAttributes string[]? -
- unicodeLocaleKeys string[]? -
- iso3Language string? -
- iso3Country string? -
- displayLanguage string? -
- displayScript string? -
- displayCountry string? -
- displayVariant string? -
- displayName string? -
adobe.analytics: NoneSettings
Fields
- includeNoneByDefault boolean? -
- noneChangeable boolean? -
adobe.analytics: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://account-d.docusign.com/oauth/token") - Refresh URL
adobe.analytics: Owner
Owner
Fields
- id int - the login id of the owner
- name string? - the friendly full login name of the owner, included when the expansion parameter ownerFullName is true
- login string? - the friendly full login name of the owner, included when the expansion parameter ownerFullName is true
adobe.analytics: Pageable
Fields
- offset int? -
- sort Sort? -
- pageNumber int? -
- pageSize int? -
adobe.analytics: PredictiveSettings
Fields
- trainingPeriods int? -
- highAnomalies boolean? -
- lowAnomalies boolean? -
adobe.analytics: ProjectCompatibility
Fields
- valid boolean? -
- validatorVersion string? -
- message string? -
adobe.analytics: ProjectsValidateBody
Fields
- project record {}? -
adobe.analytics: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
adobe.analytics: PublishingStatus
Fields
- published boolean? -
- publishedDate string? -
- lookbackPeriod int? -
- lookbackGranularity string? -
adobe.analytics: RankedColumnError
Fields
- columnId string? -
- errorCode string? -
- errorId string? -
- errorDescription string? -
adobe.analytics: RankedColumnMetaData
Fields
- dimension ReportDimension? -
- columnIds string[]? -
- columnErrors RankedColumnError[]? -
adobe.analytics: RankedReportData
Fields
- totalPages int? -
- firstPage boolean? -
- lastPage boolean? -
- numberOfElements int? -
- number int? -
- totalElements int? -
- message string? -
- request RankedRequest? -
- reportId string? -
- columns RankedColumnMetaData? -
- rows Row[]? -
adobe.analytics: RankedRequest
Fields
- rsid string? -
- dimension string? -
- locale Locale? -
- globalFilters ReportFilter[]? -
- search ReportSearch? -
- settings RankedSettings? -
- statistics RankedStatistics? -
- metricContainer ReportMetrics? -
- rowContainer ReportRows? -
- anchorDate string? -
adobe.analytics: RankedSettings
Fields
- 'limit int? -
- page int? -
- dimensionSort string? -
- countRepeatInstances boolean? -
- reflectRequest boolean? -
- includeAnomalyDetection boolean? -
- includePercentChange boolean? -
- includeLatLong boolean? -
- nonesBehavior string? -
adobe.analytics: RankedStatistics
Fields
- functions string[]? -
- ignoreZeroes boolean? -
adobe.analytics: ReportDimension
Fields
- id string? -
- 'type string? -
adobe.analytics: ReportErrorStatus
Fields
- errorCode string? -
- errorDescription string? -
- errorId string? -
- errorDetails record {}? -
- rootCauseService string? -
adobe.analytics: ReportFilter
Fields
- id string? -
- 'type string? -
- dimension string? -
- itemId string? -
- itemIds string[]? -
- segmentId string? -
- segmentDefinition record {}? -
- dateRange string? -
- excludeItemIds string[]? -
adobe.analytics: ReportMetric
Fields
- id string? -
- columnId string? -
- filters string[]? -
- sort string? -
- metricDefinition record {}? -
- predictive ReportMetricPredictiveSettings? -
adobe.analytics: ReportMetricPredictiveSettings
Fields
- anomalyConfidence decimal? -
adobe.analytics: ReportMetrics
Fields
- metricFilters ReportFilter[]? -
- metrics ReportMetric[]? -
adobe.analytics: ReportRow
Fields
- rowId string? -
- filters string[]? -
adobe.analytics: ReportRows
Fields
- rowFilters ReportFilter[]? -
- rows ReportRow[]? -
adobe.analytics: ReportSearch
Fields
- clause string? -
- excludeItemIds string[]? -
- itemIds string[]? -
- includeSearchTotal boolean? -
- empty boolean? -
adobe.analytics: ResponsePageUsageLogDto
Fields
- content UsageLogDto[]? -
- totalElements int? -
- lastPage boolean? -
- numberOfElements int? -
- totalPages int? -
- firstPage boolean? -
- sort string? -
- size int? -
- number int? -
adobe.analytics: RollingDateFunction
Fields
- 'function string? -
- granularity string? -
- offset int? -
- dow string? -
- date string? -
adobe.analytics: Row
Fields
- itemId string? -
- value string? -
- rowId string? -
- data decimal[]? -
- dataExpected decimal[]? -
- dataUpperBound decimal[]? -
- dataLowerBound decimal[]? -
- dataAnomalyDetected boolean[]? -
- percentChange decimal[]? -
- latitude decimal? -
- longitude decimal? -
adobe.analytics: RowItem
Fields
- itemId string? -
- value string? -
adobe.analytics: SegmentCompatibility
Segment compatibility.
Fields
- valid boolean? - Validity
- message string? - Message
- validator_version string? - Validator version
- supported_products string[]? - Suported products
- supported_schema string[]? - Supported schema
- supported_features string[]? - Supported features
adobe.analytics: Share
Share Model
Fields
- id int? - the share id
- shareToId int? - the id of the user/group the component is shared with
- shareToType string? - the type of entity shared with (user/group/all)
- shareToDisplayName string? - full name of the entity shared with
- componentType string? - the type of component being shared
- componentId string? - the id of the component being shared
- accessLevel string? - Level of rights shared with entity. (for projects only)
adobe.analytics: SharedComponent
Fields
- componentType string? -
- componentId string? -
- shares Share[]? -
adobe.analytics: Sort
adobe.analytics: SuiteCollectionItem
Fields
- name string? -
- timezoneZoneInfo string? - Suite friendly timezone name
- parentRsid string? - Parent report suite id for virtual report suite
- collectionItemType string? - Suite type
- currency string? -
- calendarType CalendarType? -
- rsid string? -
adobe.analytics: Tag
Tag Model
Fields
- id int? - the tag id
- name string? - the tag name
- description string? - the tag description
- components TaggedComponent[]? - the list of components that have been tagged with this tag
adobe.analytics: TaggedComponent
Fields
- componentType string? -
- componentId string? -
- tags Tag[]? -
adobe.analytics: UnhashReportData
Fields
- totalPages int? -
- firstPage boolean? -
- lastPage boolean? -
- numberOfElements int? -
- number int? -
- totalElements int? -
- message string? -
- reportId string? -
- searchAnd string? -
- searchOr string? -
- searchNot string? -
- searchPhrase string? -
- oberonRequestXML string? -
- oberonResponseXML string? -
- rows RowItem[]? -
adobe.analytics: UsageLogDto
Fields
- dateCreated string? -
- eventDescription string? -
- ipAddress string? -
- rsid string? -
- eventType string? -
- login string? -
Import
import ballerinax/adobe.analytics;
Metadata
Released date: about 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 6
Current verison: 6
Weekly downloads
Keywords
Business Intelligence/Analytics
Cost/Freemium
Contributors
Dependencies