activecampaign
Module activecampaign
API
Definitions
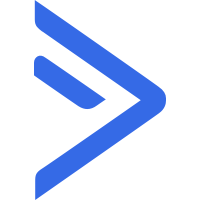
ballerinax/activecampaign Ballerina library
Overview
This is a generated connector for ActiveCampaign API v3 OpenAPI specification.
ActiveCampaign is an integrated email marketing, automation, sales software, and CRM platform. It lets users perform powerful automation, email marketing, and customer relationship management.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an ActiveCampaign Account.
- Obtain tokens by following this guide.
Quickstart
To use the ActiveCampaign connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/activecampaign
module into the Ballerina project.
import ballerinax/activecampaign;
Step 2: Create a new connector instance
You can now make the connection configuration using the <API_TOKEN>.
activecampaign:ApiKeysConfig apiKeyConfig = { apiToken:"<API_TOKEN>" }; activecampaign:Client baseClient = check new (clientConfig);
Step 3: Invoke connector operation
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to list contacts using the connector.
public function main() { activecampaign:AccountListResponse|error result = baseClient->listAccounts(); if (result is activecampaign:AccountListResponse) { log:printInfo(result.toString()); } else { log:printInfo(result.toString()); } }
- Use
bal run
command to compile and run the Ballerina program.
Clients
activecampaign: Client
This is a generated connector for ActiveCampaign API version 3 OpenAPI specification. ActiveCampaignis an integrated email marketing, automation, sales software, and CRM platform. It lets users perform powerful automation, email marketing, and customer relationship management.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an ActiveCampaign account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, string serviceUrl, ConnectionConfig config)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- serviceUrl string - URL of the target service
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
retrieveAccount
function retrieveAccount(string id) returns AccountData|error
Retrieve an account
Parameters
- id string - Account's ID
Return Type
- AccountData|error - Account data
updateAccount
function updateAccount(string id, UpdateAccountRequest payload) returns AccountData|error
Update an account
Return Type
- AccountData|error - Account data
deleteAccount
Delete an account
Parameters
- id string - Account's ID
listAccounts
function listAccounts(string? search, string? countDeals) returns AccountListResponse|error
List all accounts
Parameters
- search string? (default ()) - Search by name
- countDeals string? (default ()) - Whether to compute the contactCount and dealCount counts for the number of contacts/deals associated with each account. Set it to true to include the right counts. If set to false or omitted from the call, then contactCount and dealCount will not be counted and be simply displayed as 0.
Return Type
- AccountListResponse|error - Accounts
createAccount
function createAccount(CreateAccountRequest payload) returns AccountData|error
Create an account
Parameters
- payload CreateAccountRequest - Create account payload
Return Type
- AccountData|error - Account data
createAccountNote
function createAccountNote(string id, CreateAccountNoteRequest payload) returns AccountNoteData|error
Create a new note for an account
Parameters
- id string - Account's id to assign new note to
- payload CreateAccountNoteRequest - Create Account Note payload
Return Type
- AccountNoteData|error - Account Note data
updateAccoutNote
function updateAccoutNote(string id, string noteId, UpdateAccountNoteRequest payload) returns AccountNoteData|error
Update an account note
Parameters
- id string - Account's id to assign new note to
- noteId string - Account note's id to update
- payload UpdateAccountNoteRequest - Update Account Note payload
Return Type
- AccountNoteData|error - Account Note data
getContacts
function getContacts(string? ids, string? email, string? emailLike, int? exclude, int? formid, int? idGreater, int? idLess, string? listid, string? search, int? segmentid, int? seriesid, int? status, int? tagid, string? filtersCreatedBefore, string? filtersCreatedAfter, string? filtersUpdatedBefore, string? filtersUpdatedAfter, int? waitid, string? ordersCdate, string? ordersEmail, string? ordersFirstName, string? ordersLastName, string? ordersName, string? fordersScore, string? inGroupLists) returns ContactListResponse|error
List, search, and filter contacts
Parameters
- ids string? (default ()) - Filter contacts by ID. Can be repeated for multiple IDs.
- email string? (default ()) - Filter contacts by ID. Can be repeated for multiple IDs.
- emailLike string? (default ()) - Filter contacts that contain the given value in the email address
- exclude int? (default ()) - Exclude from the response the contact with the given ID
- formid int? (default ()) - Filter contacts associated with the given form
- idGreater int? (default ()) - Only include contacts with an ID greater than the given ID
- idLess int? (default ()) - Only include contacts with an ID less than the given ID
- listid string? (default ()) - Filter contacts associated with the given list
- search string? (default ()) - Filter contacts that match the given value in the contact names, organization, phone or email
- segmentid int? (default ()) - Return only contacts that match a list segment (this param initially returns segment information, when it is run a second time it will return contacts that match the segment)
- seriesid int? (default ()) - Filter contacts associated with the given automation
- status int? (default ()) - Status
- tagid int? (default ()) - Filter contacts associated with the given tag
- filtersCreatedBefore string? (default ()) - Filter contacts that were created prior to this date
- filtersCreatedAfter string? (default ()) - Filter contacts that were created after this date
- filtersUpdatedBefore string? (default ()) - Filter contacts that were updated before this date
- filtersUpdatedAfter string? (default ()) - Filter contacts that were updated after this date
- waitid int? (default ()) - Filter by contacts in the wait queue of an automation block
- ordersCdate string? (default ()) - Order contacts by creation date
- ordersEmail string? (default ()) - Order contacts by email
- ordersFirstName string? (default ()) - Order contacts by first name
- ordersLastName string? (default ()) - Order contacts by last name
- ordersName string? (default ()) - Order contacts by full name
- fordersScore string? (default ()) - Order contacts by score
- inGroupLists string? (default ()) - Set this to "true" in order to return only contacts that the current user has permissions to see.
Return Type
- ContactListResponse|error - Contacts
createContact
function createContact(CreateContactRequest payload) returns ContactReadResponse|error
Create Contact
Parameters
- payload CreateContactRequest - Create Contact payload
Return Type
- ContactReadResponse|error - Contact data
getContactById
function getContactById(string contactId) returns ContactReadResponse|error
Retrieve an existing contact
Parameters
- contactId string - ID of the contact
Return Type
- ContactReadResponse|error - Contact data
deleteContact
Delete Contact
Parameters
- contactId int - Delete an existing contact
getContactData
function getContactData(int contactId) returns ContactDataResponse|error
Retrieve a contact's data
Parameters
- contactId int - ID of the contact
Return Type
- ContactDataResponse|error - Contact data
getContactBounceLogs
function getContactBounceLogs(int contactId) returns BounceLogResponse|error
Get Contact Bounce Logs
Parameters
- contactId int - ID of the contact
Return Type
- BounceLogResponse|error - Bounce Log data
getContactDataGoals
function getContactDataGoals(int contactId) returns ContactGoalResponse|error
Retrieve a contact's goals
Parameters
- contactId int - ID of the contact
Return Type
- ContactGoalResponse|error - Contact Goals data
listContactAutomations
function listContactAutomations() returns ContactAutomationListResponse|error
List all automations a contact is in
Return Type
- ContactAutomationListResponse|error - Contact Automations
addContactToAutomation
function addContactToAutomation(AddContactAutomationRequest payload) returns ContactAutomationResponse|error
Add a contact to an automation
Parameters
- payload AddContactAutomationRequest - Add Contact to Automation payload
Return Type
- ContactAutomationResponse|error - Contact Automation data
getContactAutomation
function getContactAutomation(int contactAutomationId) returns ContactAutomationResponse|error
Retrieve an automation a contact is in
Parameters
- contactAutomationId int - ID of the contactAutomation to retrieve
Return Type
- ContactAutomationResponse|error - Contact Automation data
deleteContactAutomation
Remove a contact from an automation
Parameters
- contactAutomationId int - ID of the contactAutomation to delete
listEmailActivities
List all email activities
Parameters
- filtersSubscriberid int - Set this parameter to return only email activities belonging to a given subscriber.
- filtersDealid int - Set this parameter to return only email activities belonging to a given deal.
Return Type
- json|error - Email Activities
createOrUpdateContact
function createOrUpdateContact(UpdateContactRequest payload) returns ContactReadResponse|error
Create or update a contact
Parameters
- payload UpdateContactRequest - Update Contact payload
Return Type
- ContactReadResponse|error - Contact data
subscribeOrUnsubscribeContactFromList
function subscribeOrUnsubscribeContactFromList(SubscribeOrUnsubscribeContactRequest payload) returns SubscribeOrUnsubscribeContactResponse|error
Subscribe a contact to a list or unsubscribe a contact from a list.
Parameters
- payload SubscribeOrUnsubscribeContactRequest - Subscribe or Unsubscribe from list payload
Return Type
- SubscribeOrUnsubscribeContactResponse|error - List subscription data
bulkImportStatusList
function bulkImportStatusList() returns BulkImportStatus|error
Bulk import status list. After using the POST endpoint to send bulk data, you can use this endpoint to monitor progress.
Return Type
- BulkImportStatus|error - BulkImport status response
bulkImportContacts
function bulkImportContacts(BulkImportRequest payload) returns BulkImportResponse|error
Bulk import contacts
Parameters
- payload BulkImportRequest - Bulk import payload
Return Type
- BulkImportResponse|error - Bulk import data
bulkImportStatusInfo
function bulkImportStatusInfo(string batchId) returns BulkImportStatusInfo|error
This endpoint returns a specific bulk import's status including the contact IDs of any newly created contacts, and emails of any contacts that failed to be created.
Parameters
- batchId string - Batch ID
Return Type
- BulkImportStatusInfo|error - BulkImport status list
getCampaigns
function getCampaigns(string? ordersSdate, string? ordersLdate, string? filtersAutomation) returns CampaignListResponse|error
List all campaigns
Parameters
- ordersSdate string? (default ()) - Order campaigns by send date
- ordersLdate string? (default ()) - Order campaigns by last send date
- filtersAutomation string? (default ()) - Filter to return automation campaigns
Return Type
- CampaignListResponse|error - Campaigns
getCampaignById
function getCampaignById(int campaignId) returns CampaignReadResponse|error
Retrieve a campaign
Parameters
- campaignId int - ID of campaign to retrieve
Return Type
- CampaignReadResponse|error - Campaign data
getMessages
function getMessages() returns MessageListResponse|error
List all messages
Return Type
- MessageListResponse|error - Message List
addTagToContact
function addTagToContact(AddTagToContactRequest payload) returns ContactTagReadResponse|error
Add a tag to a contact
Parameters
- payload AddTagToContactRequest - Contact tag data payload
Return Type
- ContactTagReadResponse|error - Contact Tag data
removeTagFromContact
Remove a tag from a contact
Parameters
- tagId string - The contactTag id
getLists
function getLists(string? filtersName) returns ListListResponse|error
Retrieve all lists
Parameters
- filtersName string? (default ()) - Filter by the name of the list
Return Type
- ListListResponse|error - Lists
createList
function createList(CreateListRequest payload) returns ListCreateResponse|error
Create a list
Parameters
- payload CreateListRequest - Create List payload
Return Type
- ListCreateResponse|error - List
Records
activecampaign: Account
Fields
- name string - Account's name
- accountUrl string? - Account's website
- fields Field[]? - Account's custom field values {customFieldId- int, fieldValue- string, fieldCurrency?-string}[]
activecampaign: AccountData
Fields
- account AccountdataAccount? -
activecampaign: AccountdataAccount
Fields
- name string? -
- accountUrl string? -
- createdTimestamp string? -
- updatedTimestamp string? -
- links string[]? -
- id string? -
- fields Field[]? -
activecampaign: AccountListResponse
Fields
- accounts AccountData[]? -
activecampaign: AccountNoteData
Fields
- accounts AccountData[]? -
- note NoteData? -
activecampaign: AddContactAutomationRequest
Fields
- contactAutomation ContactAutomation -
activecampaign: AddTagToContactRequest
Fields
- contactTag ContactTag -
activecampaign: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apiToken string - Provide your API Key as Api-Token. Eg: {apiToken : <Your API Key>} Your API key can be found in your account on the Settings page under the Developer tab.
activecampaign: Batch
Fields
- forDate string? -
- batches string? -
- contacts string? -
activecampaign: BounceLog
Fields
- tstamp string? -
- bounceid string? -
- subscriberid string? -
- campaignid string? -
- messageid string? -
- codeid string? -
- email string? -
- 'error string? -
- 'source string? -
- created_timestamp string? -
- updated_timestamp string? -
- created_by string? -
- updated_by string? -
- links Links? -
- id string? -
- bounce string? -
- contact string? -
- campaign string? -
- message string? -
- code string? -
activecampaign: BounceLogResponse
Fields
- bounceLogs BounceLog[]? -
activecampaign: BulkContactData
Fields
- email string - The contact's email.
- first_name string? - The contact's first name
- last_name string? - The contact's last name.
- phone string? - The contact’s phone number.
- customer_acct_name string? - The name of the contact’s account.
- tags string[]? - Each string in the array will be added as a single tag to the contact. New tags will be created if they do not already exist.
- fields FieldValue[]? - A list of custom fields to apply to the contact. Each field must contain two fields- Each contact may have up to N custom fields.
- subscribe Subscribe[]? - An array of lists to subscribe the contact to. Contacts may not be subscribed to lists which they have previously unsubscribed from. Each list object contains a single field.
- unsubscribe Subscribe[]? - An array of lists to unsubscribe the contact to. Each list object contains a single field.
activecampaign: BulkImportRequest
Fields
- contacts BulkContactData[] -
- callback string? -
activecampaign: BulkImportResponse
Fields
- Success int? -
- queued_contacts int? -
- batchId string? -
- message string? -
activecampaign: BulkImportStatus
Fields
- outstanding Batch[]? -
- recentlyCompleted Batch[]? -
activecampaign: BulkImportStatusInfo
Fields
- status string? -
- success string[]? -
- failure string[]? -
activecampaign: CampaignData
Fields
- 'type string? -
- userid string? -
- segmentid string? -
- bounceid string? -
- realcid string? -
- sendid string? -
- threadid string? -
- seriesid string? -
- formid string? -
- basetemplateid string? -
- basemessageid string? -
- addressid string? -
- 'source string? -
- name string? -
- cdate string? -
- mdate string? -
- sdate string? -
- ldate string? -
- send_amt string? -
- total_amt string? -
- opens string? -
- uniqueopens string? -
- linkclicks string? -
- uniquelinkclicks string? -
- subscriberclicks string? -
- forwards string? -
- uniqueforwards string? -
- hardbounces string? -
- softbounces string? -
- unsubscribes string? -
- unsubreasons string? -
- updates string? -
- socialshares string? -
- replies string? -
- uniquereplies string? -
- status string? -
- 'public string? -
- mail_transfer string? -
- mail_send string? -
- mail_cleanup string? -
- mailer_log_file string? -
- tracklinks string? -
- tracklinksanalytics string? -
- trackreads string? -
- trackreadsanalytics string? -
- analytics_campaign_name string? -
- tweet string? -
- facebook string? -
- survey string? -
- embed_images string? -
- htmlunsub string? -
- textunsub string? -
- htmlunsubdata string? -
- textunsubdata string? -
- recurring string? -
- willrecur string? -
- split_type string? -
- split_content string? -
- split_offset string? -
- split_offset_type string? -
- split_winner_messageid string? -
- split_winner_awaiting string? -
- responder_offset string? -
- responder_type string? -
- responder_existing string? -
- reminder_field string? -
- reminder_format string? -
- reminder_type string? -
- reminder_offset string? -
- reminder_offset_type string? -
- reminder_offset_sign string? -
- reminder_last_cron_run string? -
- activerss_interval string? -
- activerss_url string? -
- activerss_items string? -
- ip4 string? -
- laststep string? -
- managetext string? -
- schedule string? -
- scheduleddate string? -
- waitpreview string? -
- deletestamp string? -
- replysys string? -
- links Links? -
- id string? -
- user string? -
- automation string? -
activecampaign: CampaignListResponse
Fields
- campaigns CampaignData[]? -
- meta Meta? -
activecampaign: CampaignReadResponse
Fields
- campaign CampaignData? -
activecampaign: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
activecampaign: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
activecampaign: Contact
Fields
- email string - Email address of the new contact. Example- 'test@example.com'
- firstName string? - First name of the new contact.
- lastName string? - Last name of the new contact.
- phone int? - Phone number of the contact.
- fieldValues FieldValue[]? -
activecampaign: ContactAutomation
Fields
- contact string? - Contact ID of the Contact, to be linked to the contactAutomation
- automation string? - Automation ID of the automation, to be linked to the contactAutomation
activecampaign: ContactAutomationData
Fields
- contact string? -
- seriesid string? -
- startid string? -
- status string? -
- adddate string? -
- remdate string? -
- timespan string? -
- lastblock string? -
- lastdate string? -
- completedElements string? -
- totalElements string? -
- completed int? -
- completeValue int? -
- links Links? -
- id string? -
- automation string? -
activecampaign: ContactAutomationListResponse
Fields
- contactAutomations ContactAutomationData[]? -
- meta Meta? -
activecampaign: ContactAutomationResponse
Fields
- contacts ContactRead[]? -
- contactAutomation ContactAutomationData? -
activecampaign: ContactData
Fields
- contact string? -
- tstamp string? -
- geoTstamp string? -
- geoIp4 string? -
- geoCountry2 string? -
- geo_country string? -
- geoState string? -
- geoCity string? -
- geoZip string? -
- geoArea string? -
- geoLat string? -
- geoLon string? -
- geoTz string? -
- geoTzOffset string? -
- ga_campaign_source string? -
- ga_campaign_name string? -
- ga_campaign_medium string? -
- ga_campaign_term string? -
- ga_campaign_content string? -
- ga_campaign_customsegment string? -
- ga_first_visit string? -
- ga_times_visited string? -
- fb_id string? -
- fb_name string? -
- tw_id string? -
- created_timestamp string? -
- updated_timestamp string? -
- created_by string? -
- updated_by string? -
- links Links? -
- id string? -
activecampaign: ContactDataResponse
Fields
- contactDatum ContactData? -
activecampaign: ContactGoal
Fields
- goalid string? -
- seriesid string? -
- subscriberid string? -
- subscriberseriesid string? -
- timespan string? -
- tstamp string? -
- links Links? -
- id string? -
- goal string? -
- automation string? -
- contact string? -
- contactAutomation string? -
activecampaign: ContactGoalResponse
Fields
- contactGoals ContactGoal[]? -
activecampaign: ContactList
Fields
- contact string? -
- list string? -
- form string? -
- seriesid string? -
- sdate string? -
- udate string? -
- status string? -
- responder string? -
- sync string? -
- unsubreason string? -
- campaign string? -
- message string? -
- first_name string? -
- last_name string? -
- ip4Sub string? -
- sourceid string? -
- autosyncLog string? -
- ip4_last string? -
- ip4Unsub string? -
- unsubscribeAutomation string? -
- links Links? -
- id string? -
- automation string? -
activecampaign: ContactListData
Fields
- list int - ID of the list to subscribe the contact to
- contact int - ID of the contact to subscribe to the list
- status int - Set to "1" to subscribe the contact to the list. Set to "2" to unsubscribe the contact from the list. WARNING- If you change a status from unsubscribed to active, you can re-subscribe a contact to a list from which they had manually unsubscribed.
- sourceid int? - Set to "4" when re-subscribing a contact to a list
activecampaign: ContactListListResponse
Fields
- contactLists ContactList[]? -
activecampaign: ContactListResponse
Fields
- scoreValues anydata[]? -
- contacts ContactRead[]? -
- meta Meta? -
activecampaign: ContactRead
Fields
- cdate string? -
- email string? -
- phone string? -
- firstName string? -
- lastName string? -
- orgid string? -
- segmentio_id string? -
- bounced_hard string? -
- bounced_soft string? -
- bounced_date string? -
- ip string? -
- ua string? -
- hash string? -
- socialdata_lastcheck string? -
- email_local string? -
- email_domain string? -
- sentcnt string? -
- rating_tstamp string? -
- gravatar string? -
- deleted string? -
- anonymized string? -
- udate string? -
- deleted_at string? -
- scoreValues anydata[]? -
- links Links? -
- id string? -
- organization string? -
activecampaign: ContactReadResponse
Fields
- contactAutomations ContactAutomation[]? -
- contactLists ContactList[]? -
- deals Deal[]? -
- fieldValues FieldValue[]? -
- geoAddresses GeoAddress[]? -
- geoIps GeoIp[]? -
- contact ContactRead? -
activecampaign: ContactTag
Fields
- contact string? - The id of the Contact
- tag string? - The id of the tag
activecampaign: ContactTagData
Fields
- cdate string? -
- contact string? -
- id string? -
- links Links? -
- tag string? -
activecampaign: ContactTagReadResponse
Fields
- contactTag ContactTagData? -
activecampaign: CreateAccountNoteRequest
Fields
- note string - Account note's content
activecampaign: CreateAccountRequest
Fields
- account Account -
activecampaign: CreateContactRequest
Fields
- contact Contact -
activecampaign: CreateListRequest
Fields
- list List -
activecampaign: Deal
Fields
- owner string? -
- contact string? -
- organization string? -
- group string? -
- title string? -
- nexttaskid string? -
- currency string? -
- status string? -
- links Links? -
- id string? -
- nextTask string? -
activecampaign: Field
Fields
- customFieldId int - Field ID, ID of the Custom Field Meta Data
- fieldCurrency string? - Required only for the currency field type. The three letter currency code for the currency value
activecampaign: FieldValue
Fields
- 'field string - Field name
- value string - Value related to field name
activecampaign: GeoAddress
Fields
- ip4 string? -
- country2 string? -
- country string? -
- state string? -
- city string? -
- zip string? -
- area string? -
- lat string? -
- lon string? -
- tz string? -
- tstamp string? -
- links Links? -
- id string? -
activecampaign: GeoIp
Fields
- contact string? -
- campaignid string? -
- messageid string? -
- geoaddrid string? -
- ip4 string? -
- tstamp string? -
- geoAddress string? -
- links Links? -
- id string? -
activecampaign: Links
Fields
- bounceLogs string? -
- contactAutomations string? -
- contactData string? -
- contactGoals string? -
- contactLists string? -
- contactLogs string? -
- contactTags string? -
- contactDeals string? -
- deals string? -
- fieldValues string? -
- geoIps string? -
- notes string? -
- organization string? -
- plusAppend string? -
- trackingLogs string? -
- scoreValues string? -
activecampaign: List
Fields
- name string - Name of the list to create
- stringid string - URL-safe list name. Example- 'list-name-sample'
- sender_url string - The website URL this list is for.
- sender_reminder string - A reminder for your contacts as to why they are on this list and you are messaging them.
- user int? - User Id of the list owner. A list owner is able to control campaign branding. A property of list. userid also exists on this object; both properties map to the same list owner field and are being maintained in the response object for backward compatibility. If you post values for both list.user and list.userid, the value of list.user will be used.
- send_last_broadcast boolean? - Boolean value indicating whether or not to send the last sent campaign to this list to a new subscriber upon subscribing. 1 = yes, 0 = no
- carboncopy string? - Comma-separated list of email addresses to send a copy of all mailings to upon send
- subscription_notify string? - Comma-separated list of email addresses to notify when a new subscriber joins this list.
- unsubscription_notify string? - Comma-separated list of email addresses to notify when a subscriber unsubscribes from this list.
activecampaign: ListCreateResponse
Fields
- list ListData? -
activecampaign: ListData
Fields
- stringid string? -
- userid string? -
- name string? -
- cdate string? -
- p_use_tracking string? -
- p_use_analytics_read string? -
- p_use_analytics_link string? -
- p_use_twitter string? -
- p_use_facebook string? -
- p_embed_image string? -
- p_use_captcha string? -
- send_last_broadcast string? -
- 'private string? -
- analytics_domains string? -
- analytics_source string? -
- analytics_ua string? -
- twitter_token string? -
- twitter_token_secret string? -
- facebook_session string? -
- carboncopy string? -
- subscription_notify string? -
- unsubscription_notify string? -
- require_name string? -
- get_unsubscribe_reason string? -
- to_name string? -
- optinoptout string? -
- sender_name string? -
- sender_addr1 string? -
- sender_addr2 string? -
- sender_city string? -
- sender_state string? -
- sender_zip string? -
- sender_country string? -
- sender_phone string? -
- sender_url string? -
- sender_reminder string? -
- fulladdress string? -
- optinmessageid string? -
- optoutconf string? -
- deletestamp string? -
- udate string? -
- links Links? -
- id string? -
- user string? -
activecampaign: ListListResponse
Fields
- lists ListData[]? -
- meta Meta? -
activecampaign: ListReadResponse
Fields
- list ListData? -
activecampaign: MessageData
Fields
- userid string? -
- ed_instanceid string? -
- ed_version string? -
- cdate string? -
- mdate string? -
- name string? -
- fromname string? -
- fromemail string? -
- reply2 string? -
- priority string? -
- charset string? -
- encoding string? -
- format string? -
- subject string? -
- preheader_text string? -
- text string? -
- html string? -
- htmlfetch string? -
- textfetch string? -
- hidden string? -
- preview_mime string? -
- preview_data string? -
activecampaign: MessageListResponse
Fields
- messages MessageData[]? -
- meta Meta? -
activecampaign: MessageReadResponse
Fields
- message MessageData? -
activecampaign: Meta
Fields
- total string? -
- page_input MetaPageInput? -
activecampaign: MetaPageInput
Fields
- segmentid int? -
- formid int? -
- listid int? -
- tagid int? -
- 'limit int? -
- offset int? -
- search string? -
- sort string? -
- seriesid int? -
- waitid int? -
- status int? -
- forceQuery int? -
- cacheid string? -
activecampaign: NoteData
Fields
- note NotedataNote? -
activecampaign: NotedataNote
Fields
- cdate string? -
- id string? -
- links NotedataNoteLinks? -
- mdate string? -
- note string? -
- owner NotedataNoteOwner? -
- relid record {}? -
- reltype record {}? -
- user record {}? -
- userid record {}? -
activecampaign: NotedataNoteLinks
Fields
- activities string? -
- mentions string? -
- notes string? -
- owner string? -
- user string? -
activecampaign: NotedataNoteOwner
Fields
- id string? -
- 'type string? -
activecampaign: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
activecampaign: Subscribe
Fields
- listid string - The ID of the list to subscribe the contact to or unsubscribe the contact from. Lists must be referenced by the ID that ActiveCampaign assigns to them. You can find the list ID by clicking the list in your ActiveCampaign account then viewing the URL bar. It will look something like this-
/app/contacts/?listid=19&status=1
You can also retrieve the ID number for a list by using the "Retrieve all lists" API call.
activecampaign: SubscribeOrUnsubscribeContactRequest
Fields
- contactList ContactListData -
activecampaign: SubscribeOrUnsubscribeContactResponse
Fields
- contacts ContactRead[]? -
- contactList ContactList? -
activecampaign: UpdatableAccount
Fields
- name string? - Account's name
- accountUrl string? - Account's website
- fields Field[]? - Account's custom field values {customFieldId- int, fieldValue- string, fieldCurrency?-string}[]
activecampaign: UpdateAccountNoteRequest
Fields
- note string? - Account note's content
activecampaign: UpdateAccountRequest
Fields
- account UpdatableAccount -
activecampaign: UpdateContactData
Fields
- email string? - Email address of the contact to sync
- firstName string? - First name of the new contact.
- lastName string? - Last name of the new contact.
- phone int? - Phone number of the contact.
- fieldValues FieldValue[]? - Contact's custom field values [{field, value}]
activecampaign: UpdateContactRequest
Fields
- contact UpdateContactData -
Import
import ballerinax/activecampaign;
Metadata
Released date: about 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1373
Current verison: 252
Weekly downloads
Keywords
Marketing/Marketing Automation
Cost/Freemium
Contributors
Dependencies