Module ably
API
Definitions
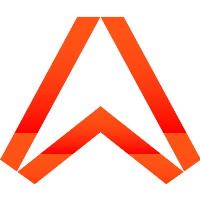
ballerinax/ably Ballerina library
Overview
This is a generated connector for Ably REST API v1.1.0 OpenAPI specification. The Ably REST API provides a way for a wide range of server and client devices to communicate with the Ably service over REST. The REST API does not provide a realtime long-lived connection to Ably, but in all other respects is a simple subset of the full realtime messaging API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Ably account
- Obtain tokens following this guide.
Note: The application key can be split at the first colon, with the initial segment being used as the username, and the remaining string (without the leading colon) used as the password.
Quickstart
To use the Ably connector in your Ballerina application, update the .bal file as follows
Step 1: Import connector
First, import the ballerinax/ably
module into the Ballerina project.
import ballerinax/ably;
Step 2: Create a new connector instance
Create a ably:ClientConfig
with the application key split at the first colon as username and password, and initialize the connector with it.
ably:ClientConfig configuration = { auth: { username: <ABLY_USERNAME>, password: <ABLY_PASSWORD> } }; ably:Client ablyClient = check new(configuration);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to publish a new message using the connector.
Publish a new message
public function main() { ably:Message message = { name: "my_event_name", data: "my_event_data" }; ably:InlineResponse2xx1|error response = baseClient->publishMessagesToChannel("rest-example", message); }
Following is an example on how to retrieve message history for a channel using the connector.
Retrieve message history for a channel
public function main() { Message[]|error response = baseClient->getMessagesByChannel("rest-example"); if (response is Message[]) { foreach var item in response { log:printInfo(item.toString()); } } else { log:printError(response.message()); } } -
Use
bal run
command to compile and run the Ballerina program.
Clients
ably: Client
This is a generated connector for Ably REST API v1.1.0 OpenAPI specification. The Ably REST API provides a way for a wide range of server and client devices to communicate with the Ably service over REST. The REST API does not provide a realtime long-lived connection to Ably, but in all other respects is a simple subset of the full realtime messaging API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Ably account and obtain tokens following this guide.
init (ClientConfig clientConfig, string serviceUrl)
- clientConfig ClientConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://rest.ably.io" - URL of the target service
getMetadataOfAllChannels
function getMetadataOfAllChannels(string? xAblyVersion, string? format, int 'limit, string? prefix, string? 'by) returns InlineResponse2xx|error
Enumerate all active channels of the application
Parameters
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- 'limit int (default 100) - Optionally specifies the maximum number of results to return. A limit greater than 1000 is unsupported
- prefix string? (default ()) - Optionally limits the query to only those channels whose name starts with the given prefix
- 'by string? (default ()) - optionally specifies whether to return just channel names (by=id) or ChannelDetails (by=value)
Return Type
- InlineResponse2xx|error - OK
getMetadataOfChannel
function getMetadataOfChannel(string channelId, string? xAblyVersion, string? format) returns ChannelDetails|error
Get metadata of a channel
Parameters
- channelId string - The Channel's ID.
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
Return Type
- ChannelDetails|error - OK
getMessagesByChannel
function getMessagesByChannel(string channelId, string? xAblyVersion, string? format, string? 'start, int? 'limit, string end, string direction) returns Message[]|error
Get message history for a channel
Parameters
- channelId string - The Channel's ID.
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- 'start string? (default ()) - Beginning of time The start of the query interval as a time in milliseconds since the epoch. A message qualifies as a member of the result set if it was received at or after this time.
- 'limit int? (default ()) - The maximum number of records to return. A limit greater than 1,000 is invalid.
- end string (default "now") - The end of the query interval as a time in milliseconds since the epoch. A message qualifies as a member of the result set if it was received at or before this time.
- direction string (default "backwards") - The direction of this query. The direction determines the order of the returned result array, but also determines which end of the query interval is the start point for the search. For example, a forwards query uses start as the start point, whereas a backwards query uses end as the start point.
publishMessagesToChannel
function publishMessagesToChannel(string channelId, Message payload, string? xAblyVersion, string? format) returns InlineResponse2xx1|error
Publish a message to a channel
Parameters
- channelId string - The Channel's ID.
- payload Message - Message
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
Return Type
- InlineResponse2xx1|error - OK
getPresenceOfChannel
function getPresenceOfChannel(string channelId, string? xAblyVersion, string? format, string? clientId, string? connectionId, int 'limit) returns PresenceMessage[]|error
Get presence of a channel
Parameters
- channelId string - The Channel's ID.
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- clientId string? (default ()) - Optional filter to restrict members present with that clientId
- connectionId string? (default ()) - Optional filter to restrict members present with that connectionId
- 'limit int (default 100) - The maximum number of records to return. A limit greater than 1,000 is invalid.
Return Type
- PresenceMessage[]|error - OK
getPresenceHistoryOfChannel
function getPresenceHistoryOfChannel(string channelId, string? xAblyVersion, string? format, string? 'start, int? 'limit, string end, string direction) returns PresenceMessage[]|error
Get presence history of a channel
Parameters
- channelId string - The Channel's ID.
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- 'start string? (default ()) - Beginning of time The start of the query interval as a time in milliseconds since the epoch. A message qualifies as a member of the result set if it was received at or after this time.
- 'limit int? (default ()) - The maximum number of records to return. A limit greater than 1,000 is invalid.
- end string (default "now") - The end of the query interval as a time in milliseconds since the epoch. A message qualifies as a member of the result set if it was received at or before this time.
- direction string (default "backwards") - The direction of this query. The direction determines the order of the returned result array, but also determines which end of the query interval is the start point for the search. For example, a forwards query uses start as the start point, whereas a backwards query uses end as the start point.
Return Type
- PresenceMessage[]|error - OK
requestAccessToken
function requestAccessToken(string keyName, KeynameRequesttokenBody payload, string? xAblyVersion, string? format) returns TokenDetails|error
Request an access token
Parameters
- payload KeynameRequesttokenBody - Request Body
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
Return Type
- TokenDetails|error - OK
getPushSubscriptionsOnChannels
function getPushSubscriptionsOnChannels(string? xAblyVersion, string? format, string? 'channel, string? deviceId, string? clientId, int 'limit) returns DeviceDetails|error
List channel subscriptions
Parameters
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- 'channel string? (default ()) - Filter to restrict to subscriptions associated with that channel.
- deviceId string? (default ()) - Optional filter to restrict to devices associated with that deviceId. Cannot be used with clientId.
- clientId string? (default ()) - Optional filter to restrict to devices associated with that clientId. Cannot be used with deviceId.
- 'limit int (default 100) - The maximum number of records to return.
Return Type
- DeviceDetails|error - OK
subscribePushDeviceToChannel
function subscribePushDeviceToChannel(PushChannelsubscriptionsBody payload, string? xAblyVersion, string? format) returns Response|error
Subscribe a device to a channel
Parameters
- payload PushChannelsubscriptionsBody - Request Body
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
deletePushDeviceDetails
function deletePushDeviceDetails(string? xAblyVersion, string? format, string? 'channel, string? deviceId, string? clientId) returns Response|error
Delete a registered device's update token
Parameters
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- 'channel string? (default ()) - Filter to restrict to subscriptions associated with that channel.
- deviceId string? (default ()) - Must be set when clientId is empty, cannot be used with clientId.
- clientId string? (default ()) - Must be set when deviceId is empty, cannot be used with deviceId.
getChannelsWithPushSubscribers
function getChannelsWithPushSubscribers(string? xAblyVersion, string? format) returns string[]|error
List all channels with at least one subscribed device
Parameters
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
getRegisteredPushDevices
function getRegisteredPushDevices(string? xAblyVersion, string? format, string? deviceId, string? clientId, int 'limit) returns DeviceDetails|error
List devices registered for receiving push notifications
Parameters
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- deviceId string? (default ()) - Optional filter to restrict to devices associated with that deviceId.
- clientId string? (default ()) - Optional filter to restrict to devices associated with that clientId.
- 'limit int (default 100) - The maximum number of records to return.
Return Type
- DeviceDetails|error - OK
registerPushDevice
function registerPushDevice(DeviceDetails payload, string? xAblyVersion, string? format) returns DeviceDetails|error
Register a device for receiving push notifications
Parameters
- payload DeviceDetails - Device Details
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
Return Type
- DeviceDetails|error - OK
unregisterAllPushDevices
function unregisterAllPushDevices(string? xAblyVersion, string? format, string? deviceId, string? clientId) returns Response|error
Unregister matching devices for push notifications
Parameters
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- deviceId string? (default ()) - Optional filter to restrict to devices associated with that deviceId. Cannot be used with clientId.
- clientId string? (default ()) - Optional filter to restrict to devices associated with that clientId. Cannot be used with deviceId.
getPushDeviceDetails
function getPushDeviceDetails(string deviceId, string? xAblyVersion, string? format) returns DeviceDetails|error
Get a device registration
Parameters
- deviceId string - Device's ID.
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
Return Type
- DeviceDetails|error - OK
putPushDeviceDetails
function putPushDeviceDetails(string deviceId, DeviceDetails payload, string? xAblyVersion, string? format) returns DeviceDetails|error
Update a device registration
Parameters
- deviceId string - Device's ID.
- payload DeviceDetails - Device Details
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
Return Type
- DeviceDetails|error - OK
unregisterPushDevice
function unregisterPushDevice(string deviceId, string? xAblyVersion, string? format) returns Response|error
Unregister a single device for push notifications
Parameters
- deviceId string - Device's ID.
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
patchPushDeviceDetails
function patchPushDeviceDetails(string deviceId, DeviceDetails payload, string? xAblyVersion, string? format) returns DeviceDetails|error
Update a device registration
Parameters
- deviceId string - Device's ID.
- payload DeviceDetails - Device Details
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
Return Type
- DeviceDetails|error - OK
updatePushDeviceDetails
function updatePushDeviceDetails(string deviceId, string? xAblyVersion, string? format) returns DeviceDetails|error
Reset a registered device's update token
Parameters
- deviceId string - Device's ID.
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
Return Type
- DeviceDetails|error - OK
publishPushNotificationToDevices
function publishPushNotificationToDevices(PushPublishBody payload, string? xAblyVersion, string? format) returns Response|error
Publish a push notification to device(s)
Parameters
- payload PushPublishBody - Request Body
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
getStats
function getStats(string? xAblyVersion, string? format, string? 'start, int? 'limit, string end, string direction, string unit) returns json|error
Retrieve usage statistics for an application
Parameters
- xAblyVersion string? (default ()) - The version of the API you wish to use.
- format string? (default ()) - The response format you would like
- 'start string? (default ()) - Beginning of time The start of the query interval as a time in milliseconds since the epoch. A message qualifies as a member of the result set if it was received at or after this time.
- 'limit int? (default ()) - The maximum number of records to return. A limit greater than 1,000 is invalid.
- end string (default "now") - The end of the query interval as a time in milliseconds since the epoch. A message qualifies as a member of the result set if it was received at or before this time.
- direction string (default "backwards") - The direction of this query. The direction determines the order of the returned result array, but also determines which end of the query interval is the start point for the search. For example, a forwards query uses start as the start point, whereas a backwards query uses end as the start point.
- unit string (default "minute") - Specifies the unit of aggregation in the returned results.
Return Type
- json|error - OK
getTime
Get the service time
Records
ably: ChannelDetails
Fields
- channelId string - The required name of the channel including any qualifier, if any.
- isGlobalMaster boolean? - In events relating to the activity of a channel in a specific region, this optionally identifies whether or not that region is responsible for global coordination of the channel.
- region string? - In events relating to the activity of a channel in a specific region, this optionally identifies the region.
- status ChannelStatus? - A ChannelStatus instance.
ably: ChannelStatus
A ChannelStatus instance.
Fields
- isActive boolean - A required boolean value indicating whether the channel that is the subject of the event is active. For events indicating regional activity of a channel this indicates activity in that region, not global activity.
- occupancy Occupancy? - An Occupancy instance indicating the occupancy of a channel. For events indicating regional activity of a channel this indicates activity in that region, not global activity.
ably: ClientConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|CredentialsConfig - Configurations related to client authentication
- httpVersion string(default "1.1") - The HTTP version understood by the client
- http1Settings ClientHttp1Settings(default {}) - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings(default {}) - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- followRedirects FollowRedirects?(default ()) - Configurations associated with Redirection
- poolConfig PoolConfiguration?(default ()) - Configurations associated with request pooling
- cache CacheConfig(default {}) - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig?(default ()) - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig?(default ()) - Configurations associated with retrying
- cookieConfig CookieConfig?(default ()) - Configurations associated with cookies
- responseLimits ResponseLimitConfigs(default {}) - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket?(default ()) - SSL/TLS-related options
ably: DeviceDetails
Fields
- clientId string? - Optional trusted client identifier for the device.
- deviceSecret string? - Secret value for the device.
- formFactor string? - Form factor of the push device.
- id string? - Unique identifier for the device generated by the device itself.
- metadata record {}? - Optional metadata object for this device. The metadata for a device may only be set by clients with push-admin privileges and will be used more extensively in the future with smart notifications.
- platform string? - Platform of the push device.
- pushRecipient Recipient? - Push recipient details for a device.
- pushState string? - the current state of the push device.
ably: Error
Returned error from failed REST.
Fields
- code int? - Error code.
- href string? - Link to help with error.
- message string? - Message explaining the error's cause.
- serverId string? - Server ID with which error was encountered.
- statusCode int? - Status error code.
ably: Extras
Extras object. Currently only allows for push extra.
Fields
- push Push? - Push
ably: InlineResponse2xx1
Fields
- 'channel string? -
- messageId string? -
ably: Message
Message object.
Fields
- connectionId string? - The connection ID of the publisher of this message.
- data string? - The string encoded payload, with the encoding specified below.
- encoding string? - This will typically be empty as all messages received from Ably are automatically decoded client-side using this value. However, if the message encoding cannot be processed, this attribute will contain the remaining transformations not applied to the data payload.
- id string? - A Unique ID that can be specified by the publisher for idempotent publishing.
- name string? - The event name, if provided.
- timestamp int? - Timestamp when the message was received by the Ably, as milliseconds since the epoch.
ably: Notification
Notification
Fields
- body string? - Text below title on the expanded notification.
- collapseKey string? - Platform-specific, used to group notifications together.
- icon string? - Platform-specific icon for the notification.
- sound string? - Platform-specific sound for the notification.
- title string? - Title to display at the notification.
ably: Occupancy
An Occupancy instance indicating the occupancy of a channel. For events indicating regional activity of a channel this indicates activity in that region, not global activity.
Fields
- presenceConnections int? - The number of connections that are authorised to enter members into the presence channel.
- presenceMembers int? - The number of members currently entered into the presence channel.
- presenceSubscribers int? - The number of connections that are authorised to subscribe to presence messages.
- publishers int? - The number of connections attached to the channel that are authorised to publish.
- subscribers int? - The number of connections attached that are authorised to subscribe to messages.
ably: PresenceMessage
Fields
- action string? - The event signified by a PresenceMessage.
- clientId string? - The client ID of the publisher of this presence update.
- connectionId string? - The connection ID of the publisher of this presence update.
- data string? - The presence update payload, if provided.
- encoding string? - This will typically be empty as all presence updates received from Ably are automatically decoded client-side using this value. However, if the message encoding cannot be processed, this attribute will contain the remaining transformations not applied to the data payload.
- id string? - Unique ID assigned by Ably to this presence update.
- timestamp int? - Timestamp when the presence update was received by Ably, as milliseconds since the epoch.
ably: Push
Push
Fields
- apns PushApns? - Extends and overrides generic values when delivering via APNs. See examples
- data string? - Arbitrary key-value string-to-string payload.
- fcm PushFcm? - Extends and overrides generic values when delivering via GCM/FCM. See examples
- notification Notification? - Notification
- web PushWeb? - Extends and overrides generic values when delivering via web. See examples
ably: PushApns
Extends and overrides generic values when delivering via APNs. See examples
Fields
- notification Notification? - Notification
ably: PushFcm
Extends and overrides generic values when delivering via GCM/FCM. See examples
Fields
- notification Notification? - Notification
ably: PushPublishBody
Fields
- push Push? - Push
- recipient Recipient - Push recipient details for a device.
ably: PushPublishBody1
Fields
- push Push? - Push
- recipient Recipient - Push recipient details for a device.
ably: PushPublishBody2
Fields
- push Push? - Push
- recipient Recipient - Push recipient details for a device.
ably: PushWeb
Extends and overrides generic values when delivering via web. See examples
Fields
- notification Notification? - Notification
ably: Recipient
Push recipient details for a device.
Fields
- clientId string? - Client ID of recipient
- deviceId string? - Client ID of recipient
- deviceToken string? - when using APNs, specifies the required device token.
- registrationToken string? - when using GCM or FCM, specifies the required registration token.
- transportType string? - Defines which push platform is being used.
ably: SignedTokenRequest
Fields
- Fields Included from *TokenRequest
- mac string - A signature, generated as an HMAC of each of the above components, using the key secret value.
ably: TokenDetails
Fields
- capability string? - Regular expression representation of the capabilities of the token.
- expires int? - Timestamp of token expiration.
- issued int? - Timestamp of token creation.
- keyName string? - Name of the key used to create the token
- token string? - The Ably Token.
ably: TokenRequest
Fields
- capability record {} - The capabilities (i.e. a set of channel names/namespaces and, for each, a set of operations) which should be a subset of the set of capabilities associated with the key specified in keyName.
- keyName string - Name of the key used for the TokenRequest. The keyName comprises of the app ID and key ID on an API Key.
- nonce string - An unquoted, un-escaped random string of at least 16 characters. Used to ensure the Ably TokenRequest cannot be reused.
- timestamp int - Time of creation of the Ably TokenRequest.
Union types
ably: InlineResponse2xx
InlineResponse2xx
ably: KeynameRequesttokenBody
KeynameRequesttokenBody
ably: PushChannelsubscriptionsBody
PushChannelsubscriptionsBody
Import
import ballerinax/ably;
Metadata
Released date: over 3 years ago
Version: 1.1.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: slbeta6
GraalVM compatible: Yes
Pull count
Total: 8
Current verison: 0
Weekly downloads
Keywords
Business Intelligence/Analytics
Cost/Freemium
Contributors
Dependencies