aayu.mftg.as2
Module aayu.mftg.as2
API
Declarations
Definitions
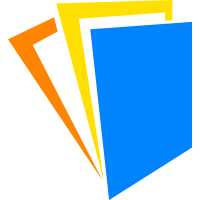
ballerinax/aayu.mftg.as2 Ballerina library
Overview
This is a generated connector for MFT Gateway (by Aayu Technologies) REST API v1.0 OpenAPI specification. The MFT Gateway REST API provides a secure AS2 secured channel for B2B communications, multiple ways to upload/download files and automate the exchange through integration mechanisms.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a account on MFT Gateway (by Aayu technologies)
- Create a Station in the MFT Gateway account.
Quickstart
To use the MFT gateway connector in your Ballerina application, update the .bal file as follows
Step 1: Import connector
First, import the ballerinax/aayu.mftg.as2
module into the Ballerina project.
import ballerinax/aayu.mftg.as2 as mftg;
Step 2: Create a new connector instance
Create a mftg:Client
instance using username, password (used to sign in MFTgateway) and Station identifier.
mftg:Client mftgClient = check new (username, password, stationIdentifier);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on sending an AS2 message.
Send AS2 Message
public function main() returns error? { byte[] filePayload = check io:fileReadBytes(filePath); mftg:SuccessfulMessageSubmitResponse successfulMessageSubmitResponse = check mftgClient->sendAS2Message("<PARTNER_AS2_ID>", "<CONTENT_TYPE>", filePayload); io:println(successfulMessageSubmitResponse); }
Following is an example on sending an AS2 message with attachements larger than 3MB.
Send AS2 Message
public function main() returns error? { stream<io:Block, io:Error?> fileReadBlocksAsStream = check io:fileReadBlocksAsStream(<FILE_PATH>); check mftgClient->sendLargeAS2Message(<PARTNER_AS2_ID>, fileReadBlocksAsStream, <ACCESS_KEY_ID>, <SECRET_ACCESS_KEY>, <MFTG_BUCKET>, <ATTACHMENT_NAME>); }
Following is an example on listing received messages.
List Received Messages
public function main() { mftg:SuccessfulMessageListRetrievalResponse messageResponse = check mftgClient->listReceivedMessages(); if messageResponse.messages == [] { io:println("No messages received!!"); return; } foreach var messageId in messageResponse.messages { io:println("MESSAGE ID : " + messageId); } }
Following is an example on single message retrieval.
Retrieve an Inbox (Received) Message
public function main() { mftg:AS2Message messageResponse = check mftgClient->retrieveInboxMessage(messageResponse1.messages[0]); io:println(messageResponse); }
Following is an example on listing certificates available.
List Certificates
public function main() { mftg:SuccessfulCertListRetrievalResponse listCertificates = check mftgClient->listCertificates(); io:println(listCertificates); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
aayu.mftg.as2: Client
This is a generated connector for MFT REST API v1.0 OpenAPI specification. The MFT REST API provides a secure AS2 secured channel for such communications, and offers your company, multiple ways to upload/download files, or automate the exchange through integration mechanisms.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a MFT account and obtain tokens following this guide.
(Note : Token Validity is 1h)
init (string username, string password, string as2From, ConnectionConfig config, string serviceUrl)
- username string - Valid email address used to sign in MFTG console
- password string - Valid Password used to sign in MFTG console
- as2From string - Station AS2 identifier (which message is send from)
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.mftgateway.com" - URL of the target service
sendAS2Message
function sendAS2Message(string as2To, string contentType, byte[] payload, string? subject, string? attachmentName) returns SuccessfulMessageSubmitResponse|error
Sends AS2 Message. Recommend less than 3MB.
Parameters
- as2To string - Partner AS2 identifier which intends to receive the message
- contentType string - Content type of the message payload. For multiple attachments, content type should be multipart/form-data with valid form content
- payload byte[] - File payload as byte array
- subject string? (default ()) - Subject of the message. If not specified, the default subject configured for the intended partner will be applied.
- attachmentName string? (default ()) - Name of the message attachment (Only applicable when sending a message with single attachment)
Return Type
- SuccessfulMessageSubmitResponse|error - Accepted for Processing
sendLargeAS2Message
function sendLargeAS2Message(string as2To, stream<Block, Error?> payload, string awsAccessKeyID, string awsSecretAccessKey, string awsS3BucketName, string attachmentName) returns error?
Sends AS2 Message. Recommend for large messages. The message will be uploading to AWS S3 bucket.
As a prerequisite, Enable (AWS S3 integration)[https://console.mftgateway.com/integration/s3] in MFT gateway.
Create new credentials & obtain awsAccessKeyID
, awsSecretAcessKey
Parameters
- as2To string - Partner AS2 identifier which intends to receive the message
- awsAccessKeyID string - AWS access key ID (Enable & download credentials from https://console.mftgateway.com/integration/s3)
- awsSecretAccessKey string - AWS secret access key (Enable & download credentials from https://console.mftgateway.com/integration/s3)
- awsS3BucketName string - Name of the AWS S3 bucket (Enable AWS S3 integration https://console.mftgateway.com/integration/s3, Obtain the S3 bucket name from console)
- attachmentName string - Name of the message attachment
Return Type
- error? - An error on failure or else
()
listReceivedMessages
function listReceivedMessages(string sortDir, int pageLength, int pageOffset, string? stationAS2Id, string? partnerAS2Id, string? as2MessageId, string? subject, boolean fetchAll) returns SuccessfulMessageListRetrievalResponse|error
List Received Messages
Parameters
- sortDir string (default "desc") - Sort direction of messages
- pageLength int (default 10) - Length of a page. An integer value greater than or equal to 1 and less than or equal to 100 is accepted.
- pageOffset int (default 0) - Page offset, supports non-negative integer values
- stationAS2Id string? (default ()) - AS2 identifier of the AS2 Station that received these messages
- partnerAS2Id string? (default ()) - AS2 identifier of the AS2 Partner that sent these messages
- as2MessageId string? (default ()) - AS2 message identifier of the required AS2 message(s)
- subject string? (default ()) - Any subject string to filter messages against
- fetchAll boolean (default false) - Fetch all messages including already been "marked as read" within the API scope
Return Type
- SuccessfulMessageListRetrievalResponse|error - Successful
retrieveInboxMessage
function retrieveInboxMessage(string as2MessageId, boolean markAsRead) returns AS2Message|error
Retrieve an Inbox (Received) Message
Parameters
- as2MessageId string - AS2 ID of the message to be retrieved
- markAsRead boolean (default true) - If this is set to true, the message will be "marked as read" upon retrieval. Any message list queries with
fetchAll
query param set tofalse
, will not return the corresponding message afterwards. You can combine this and thefetchAll
query parameter, to ensure one-time processing of received messages. (Note that this marking will be applied only at API scope; "marked as read" messages may still appear as unread on the MFT Gateway webapp inbox.)
Return Type
- AS2Message|error - Successfully Retrieved
deleteInboxMessage
function deleteInboxMessage(string as2MessageId) returns SuccessfulMessageDeletionResponse|error
Delete Inbox (Received) Message
Parameters
- as2MessageId string - AS2 ID of the message to be deleted
Return Type
- SuccessfulMessageDeletionResponse|error - Successfully Deleted
markReceivedMessageAsUnRead
function markReceivedMessageAsUnRead(string as2MessageId) returns ResponseWithMessage|error
Mark Received Message As UnRead
Parameters
- as2MessageId string - AS2 ID of the message to be marked as unread
Return Type
- ResponseWithMessage|error - Successfully marked as Unread
retrieveInboxMessageAttachments
function retrieveInboxMessageAttachments(string as2MessageId) returns SuccessfulAttachmentListResponse|error
Retrieve Inbox (Received) Message Attachment(s)
Parameters
- as2MessageId string - AS2 ID of the message to retrieve attachments of
Return Type
- SuccessfulAttachmentListResponse|error - Successfully Retrieved
retrieveInboxMessageMDN
function retrieveInboxMessageMDN(string as2MessageId) returns SuccessfulMDNRetrievalResponse|error
Retrieve Inbox (Received) Message MDN
Parameters
- as2MessageId string - AS2 ID of the message to retrieve MDN of
Return Type
- SuccessfulMDNRetrievalResponse|error - Successfully Retrieved
batchDeleteInboxMessages
function batchDeleteInboxMessages(MessageBatchOperationRequest payload) returns SuccessfulMessageBatchDeletionResponse|error
Batch Delete Inbox (Received) Messages
Parameters
- payload MessageBatchOperationRequest - AS2 IDs of the messages to be deleted
Return Type
- SuccessfulMessageBatchDeletionResponse|error - Successfully Deleted
listSentMessages
function listSentMessages(string sortDir, int pageLength, int pageOffset, string? stationAS2Id, string? partnerAS2Id, string? as2MessageId, string? subject) returns SuccessfulMessageListRetrievalResponse|error
List Sent Messages
Parameters
- sortDir string (default "desc") - Sort direction of messages
- pageLength int (default 10) - Length of a page. An integer value greater than or equal to 1 and less than or equal to 100 is accepted.
- pageOffset int (default 0) - Page offset, supports non-negative integer values
- stationAS2Id string? (default ()) - AS2 identifier of the AS2 Station that sent these messages
- partnerAS2Id string? (default ()) - AS2 identifier of the AS2 Partner that received these messages
- as2MessageId string? (default ()) - AS2 message identifier of the required AS2 message(s)
- subject string? (default ()) - Any subject string to filter messages against
Return Type
- SuccessfulMessageListRetrievalResponse|error - Successful
listQueuedMessages
function listQueuedMessages(string sortDir, int pageLength, int pageOffset, string? stationAS2Id, string? partnerAS2Id, string? as2MessageId, string? subject) returns SuccessfulMessageListRetrievalResponse|error
List Queued Messages
Parameters
- sortDir string (default "desc") - Sort direction of messages
- pageLength int (default 10) - Length of a page. An integer value greater than or equal to 1 and less than or equal to 100 is accepted.
- pageOffset int (default 0) - Page offset, supports non-negative integer values
- stationAS2Id string? (default ()) - AS2 identifier of the AS2 Station that will be sending these messages
- partnerAS2Id string? (default ()) - AS2 identifier of the AS2 Partner that will be receiving these messages
- as2MessageId string? (default ()) - AS2 message identifier of the required AS2 message(s)
- subject string? (default ()) - Any subject string to filter messages against
Return Type
- SuccessfulMessageListRetrievalResponse|error - Successful
listFailedMessages
function listFailedMessages(string sortDir, int pageLength, int pageOffset, string? stationAS2Id, string? partnerAS2Id, string? as2MessageId, string? subject) returns SuccessfulMessageListRetrievalResponse|error
List Failed Messages
Parameters
- sortDir string (default "desc") - Sort direction of messages
- pageLength int (default 10) - Length of a page. An integer value greater than or equal to 1 and less than or equal to 100 is accepted.
- pageOffset int (default 0) - Page offset, supports non-negative integer values
- stationAS2Id string? (default ()) - AS2 identifier of the AS2 Station that that was intended to sent these messages
- partnerAS2Id string? (default ()) - AS2 identifier of the AS2 Partner that was intended to receive these messages
- as2MessageId string? (default ()) - AS2 message identifier of the required AS2 message(s)
- subject string? (default ()) - Any subject string to filter messages against
Return Type
- SuccessfulMessageListRetrievalResponse|error - Successful
listincompletedmessages
function listincompletedmessages(string sortDir, int pageLength, int pageOffset, string? stationAS2Id, string? partnerAS2Id, string? as2MessageId, string? subject) returns SuccessfulMessageListRetrievalResponse|error
List Incompleted Messages
Parameters
- sortDir string (default "desc") - Sort direction of messages
- pageLength int (default 10) - Length of a page. An integer value greater than or equal to 1 and less than or equal to 100 is accepted.
- pageOffset int (default 0) - Page offset, supports non-negative integer values
- stationAS2Id string? (default ()) - AS2 identifier of the AS2 Station that sent these messages
- partnerAS2Id string? (default ()) - AS2 identifier of the AS2 Partner that received these messages
- as2MessageId string? (default ()) - AS2 message identifier of the required AS2 message(s)
- subject string? (default ()) - Any subject string to filter messages against
Return Type
- SuccessfulMessageListRetrievalResponse|error - Successful
retrieveOutboxMessage
function retrieveOutboxMessage(string as2MessageId) returns AS2Message|error
Retrieve Outbox (Sent/Queued/Failed/Incomplete) Message
Parameters
- as2MessageId string - AS2 ID of the message to be retrieved
Return Type
- AS2Message|error - Successfully Retrieved
deleteOutboxMessage
function deleteOutboxMessage(string as2MessageId) returns SuccessfulMessageDeletionResponse|error
Delete Outbox (Sent/Queued/Failed) Message
Parameters
- as2MessageId string - AS2 ID of the message to be deleted
Return Type
- SuccessfulMessageDeletionResponse|error - Successfully Deleted
retrieveOutboxMessageAttachments
function retrieveOutboxMessageAttachments(string as2MessageId) returns SuccessfulAttachmentListResponse|error
Retrieve Outbox (Sent/Queued/Failed) Message Attachment(s)
Parameters
- as2MessageId string - AS2 ID of the message to retrieve attachments of
Return Type
- SuccessfulAttachmentListResponse|error - Successfully Retrieved
retrieveOutboxMessageMDN
function retrieveOutboxMessageMDN(string as2MessageId) returns SuccessfulMDNRetrievalResponse|error
Retrieve Outbox (Sent/Queued/Failed) Message MDN
Parameters
- as2MessageId string - AS2 ID of the message to retrieve MDN of
Return Type
- SuccessfulMDNRetrievalResponse|error - Successfully Retrieved
batchDeleteOutboxMessages
function batchDeleteOutboxMessages(MessageBatchOperationRequest payload) returns SuccessfulMessageBatchDeletionResponse|error
Batch Delete Outbox (Sent/Queued/Failed) Messages
Parameters
- payload MessageBatchOperationRequest - AS2 IDs of the messages to be deleted
Return Type
- SuccessfulMessageBatchDeletionResponse|error - Successfully Deleted
listCertificates
List Certificates
Parameters
- certType string? (default ()) - Certificate type to filter. Allowed values: 'STATION', 'PARTNER', 'HTTPS', 'PARTNER_CHAIN' and 'HTTPS_CHAIN' Defaults to null (all certificates)
Return Type
- SuccessfulCertListRetrievalResponse|error - Successful Certificate List Retrieval
retrieveCertificateMetadata
function retrieveCertificateMetadata(string alias) returns SuccessfulCertRetrievalResponse|error
Retrieve Certificate Metadata
Parameters
- alias string - Alias of the certificate to retrieve
Return Type
- SuccessfulCertRetrievalResponse|error - A certificate with given alias found
createStation
function createStation(CreateStationRequest payload) returns SuccessfulStationCreationResponse|error
Create Station
Parameters
- payload CreateStationRequest - Station metadata in form on a record
CreateStationRequest
Return Type
- SuccessfulStationCreationResponse|error - Successfully Created
createPartner
function createPartner(CreatePartnerRequest payload) returns SuccessfulPartnerCreationResponse|error
Create Partner
Parameters
- payload CreatePartnerRequest - Partner metadata in form on a record
Return Type
- SuccessfulPartnerCreationResponse|error - Successfully Created
Enums
aayu.mftg.as2: CertificateType
Members
Records
aayu.mftg.as2: AS2Message
Fields
- id int -
- as2MessageId string -
- incoming boolean -
- msgStatus string -
- mdnStatus string -
- receiverAS2Id string -
- senderAS2Id string -
- subject string -
- failures int -
- attachmentPaths string[] -
- compressed boolean -
- signed boolean -
- encrypted boolean -
- mic string -
- micMatches boolean -
- transportStatusReceived int -
- userAgent string -
- timestamp int -
- apiFetched boolean -
- transportHeaders TransportHeader? -
- mdnMessage MdnMessage? -
aayu.mftg.as2: Attachment
Fields
- name string -
- url string -
aayu.mftg.as2: AuthorizeRequest
Fields
- username string -
- password string -
aayu.mftg.as2: CertificateFromCertStoreForStation
Fields
- 'type CertificateType(default FROM_CERTIFICATE_STORE) - Certificate configuration type
- alias string - Existing MFT Gateway IDENTITY type certificate alias
aayu.mftg.as2: CertificateFromKeystoreForStation
Fields
- 'type CertificateType(default FROM_KEYSTORE) - Certificate configuration type
- keystore string - S3 key of the existing keystore in your MFT Gateway S3 bucket
- keystorePassword string - Keystore password
- alias string - Certificate alias
- privateKeyPassword string - Existing private key password
- newPrivateKeyPassword string? - New private key password. If not provided, the existing private key password will be used as new password.
aayu.mftg.as2: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
aayu.mftg.as2: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
aayu.mftg.as2: CreatePartnerRequest
Fields
- name string -
- as2Identifier string -
- description string? -
- url string - Trading partner message delivery endpoint
- encryptionCertificate string - Base64 encoded encryption certificate
- encryptMessage boolean? - Whether to encrypt outbound messages
- encryptionAlgorithm string? - Encryption algorithm to be used
- signMessage boolean? - Whether to sign outbound messages
- signatureAlgorithm string? - Signature algorithm to be used
- useDiffCertAsSignCert boolean? - Whether to use a different certificate to verify signature of the inbound messages. If set to
false
, encryptionCertificate will be used to validate incoming message signatures.
- signatureCertificate string? - Base64 encoded signature certificate (Required if useDiffCertAsSignCert set to
true
)
- httpsCertificate string? - Base64 encoded SSL certificate
- encryptSignChainCertificates string[]? - Base64 encoded encryption/sign chain certificate(s)
- httpsChainCertificates string[]? - Base64 encoded SSL chain certificate(s)
- validateTrustAnchor boolean? - Whether to validate trust anchor of the uploaded certificates
- messageSubject string? - Default message subject for trading partner
- compressBefore boolean? - Whether to compress messages before encryption/sign
- compressAfter boolean? - Whether to compress messages after encryption/sign
- requestMDN boolean? - Whether to request Message Disposition Notification
- requestSignedMDN boolean? - Whether to request signed Message Disposition Notification
- requestAsyncMDN boolean? - Whether to request asynchronous Disposition Notification
- useStaticIP boolean? - Whether to use Static IP for outbound messages (Business/Enterprise tier feature)
- transmissionTimeout int? - Maximum waiting time (in seconds) before closing the outbound connection
- deleteAttachmentsOnSuccessMdn boolean? - Whether to delete Attachments from the S3 bucket when a success MDN is received
- autoRetryIncompleteMessages boolean? - Whether to auto retry incomplete messages.
Incomplete messages may have successfully processed by the trading partner but failed to acknowledge before
the configured timeout. If sets to
true
there is a possibility of duplicating outbound messages.
- customHeaders CreatepartnerrequestCustomheaders[]? - Custom transport headers to be included in the outbound messages to this trading partner. Following header names are reserved and cannot be used. 'as2-from', 'as2-to', 'as2-version', 'content-transfer-encoding', 'content-type', 'disposition-notification-options', 'mime-version', 'message-id', 'receipt-delivery-option', 'destination'
aayu.mftg.as2: CreatepartnerrequestCustomheaders
Fields
- headerName string -
- headerValue string -
aayu.mftg.as2: CreateStationRequest
Fields
- name string -
- as2Identifier string -
- email string - Comma separated list of emails (up to maximum of 3 emails)
- certificate NewCertificateForStation|CertificateFromKeystoreForStation|CertificateFromCertStoreForStation - Nested JSON object with station identity certificate configuration
- description string? -
- receivedMessageNotifications boolean? - Whether to enable email notifications for received messages
- failedMessageNotifications boolean? - Whether to enable email notifications for send failures
- largePayloadSupport boolean? - Whether to receive messages with payload size larger than 3MB through static IP address (Business/Enterprise tier feature)
aayu.mftg.as2: CreationErrorResponse
Fields
- message string -
- errors RequestFieldError[] -
aayu.mftg.as2: MdnMessage
Fields
- mdnId string -
- messageId int -
- as2MessageId string -
- disposition string -
- humanMessage string -
- rawMdnS3Key string -
- mic string -
- signed boolean -
- incoming boolean -
- status string -
- timestamp int -
aayu.mftg.as2: MessageBatchOperationRequest
Fields
- as2MessageIds string[] - AS2 IDs of the messages
aayu.mftg.as2: MessageSubmitBody
Fields
- File byte[] -
aayu.mftg.as2: NewCertificateForStation
Fields
- 'type CertificateType(default NEW_SELF_SIGN_CERTIFICATE) - Certificate configuration type
- commonName string - Certificate Common Name
- password string - Private key password
- keyLength int - Certificate key length
- validity int - Certificate validity in years
- orgUnit string? - Organization unit
- orgName string? - Organization name
- city string? - City
- state string? - State code ISO 3166-1 alpha-2
- country string? - Country code ISO 3166-1 alpha-2
aayu.mftg.as2: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
aayu.mftg.as2: RefreshAuthTokenRequest
Fields
- username string -
- refreshToken string -
aayu.mftg.as2: RequestFieldError
aayu.mftg.as2: ResponseWithMessage
Fields
- message string -
aayu.mftg.as2: SuccessfulAttachmentListResponse
Fields
- total int -
- attachments Attachment[] -
aayu.mftg.as2: SuccessfulAuthorizationResponse
Fields
- api_token string -
- refresh_token string -
aayu.mftg.as2: SuccessfulcertlistretrievalresponseInner
Fields
- alias string? -
- 'type string? -
aayu.mftg.as2: SuccessfulCertRetrievalResponse
Fields
- alias string -
- 'type string -
- serial_number string -
- subject_common_name string -
- subject_distinguish_name string -
- issuer_common_name string -
- issuer_distinguish_name string -
- valid_from string -
- expiry string -
- belongsTo string[] - To which entities this certificate belongs to
aayu.mftg.as2: SuccessfulMDNRetrievalResponse
Fields
- url string -
aayu.mftg.as2: SuccessfulMessageBatchDeletionResponse
Fields
- deleted string[] - AS2 IDs of the deleted messages
aayu.mftg.as2: SuccessfulMessageDeletionResponse
Fields
- deleted string - AS2 ID of the deleted message
aayu.mftg.as2: SuccessfulMessageListRetrievalResponse
Fields
- messages string[] - Array of AS2 IDs of the messages
aayu.mftg.as2: SuccessfulMessageSubmitResponse
Fields
- message string? -
- as2MessageId string? -
aayu.mftg.as2: SuccessfulPartnerCreationResponse
Fields
- message string -
- partnerId int? -
aayu.mftg.as2: SuccessfulStationCreationResponse
Fields
- message string -
- stationId int? -
aayu.mftg.as2: TransportHeader
Fields
- 'AS2\-From string -
- 'User\-Agent string -
- 'Accept\-Encoding string? -
- 'Content\-Transfer\-Encoding string? -
- 'Message\-ID string? -
- 'Disposition\-Notification\-Options string? -
- 'AS2\-To string -
- 'Content\-Type string -
- 'Disposition\-Notification\-To string? -
- 'AS2\-Version string -
- Host string? -
- From string -
- 'MIME\-Version string -
- Subject string -
Import
import ballerinax/aayu.mftg.as2;
Metadata
Released date: about 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1441
Current verison: 300
Weekly downloads
Keywords
IT Operations/Gateway
Cost/Paid
Contributors